Step-Through Debugging of GLSL Shaders
Total Page:16
File Type:pdf, Size:1020Kb
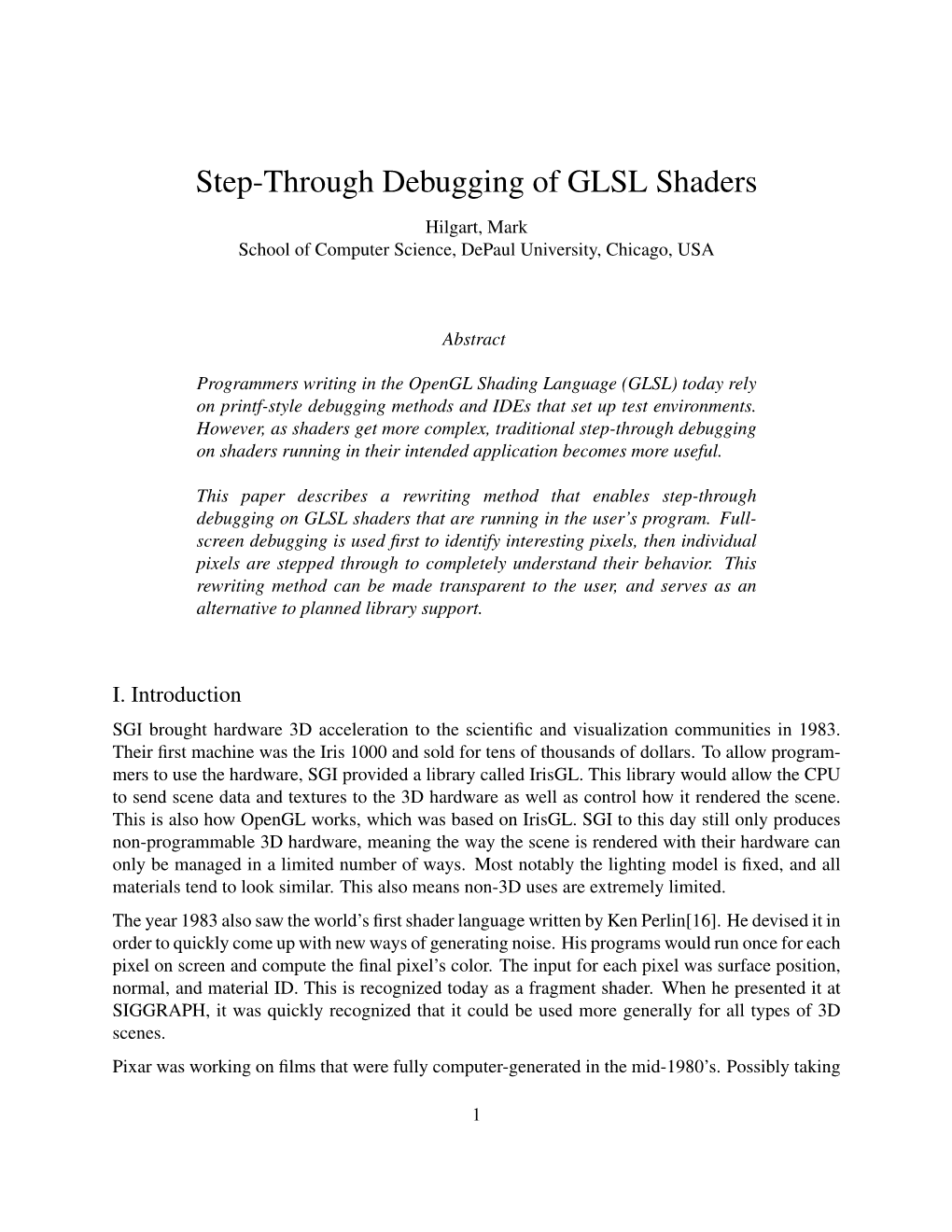
Load more
Recommended publications
-
Master Thesis
Faculty of Computer Science and Management Field of study: COMPUTER SCIENCE Specialty: Information Systems Design Master Thesis Multithreaded game engine architecture Adrian Szczerbiński keywords: game engine multithreading DirectX 12 short summary: Project, implementation and research of a multithreaded 3D game engine architecture using DirectX 12. The goal is to create a layered architecture, parallelize it and compare the results in order to state the usefulness of multithreading in game engines. Supervisor ...................................................... ............................ ……………………. Title/ degree/ name and surname grade signature The final evaluation of the thesis Przewodniczący Komisji egzaminu ...................................................... ............................ ……………………. dyplomowego Title/ degree/ name and surname grade signature For the purposes of archival thesis qualified to: * a) Category A (perpetual files) b) Category BE 50 (subject to expertise after 50 years) * Delete as appropriate stamp of the faculty Wrocław 2019 1 Streszczenie W dzisiejszych czasach, gdy społeczność graczy staje się coraz większa i stawia coraz większe wymagania, jak lepsza grafika, czy ogólnie wydajność gry, pojawia się potrzeba szybszych i lepszych silników gier, ponieważ większość z obecnych jest albo stara, albo korzysta ze starych rozwiązań. Wielowątkowość jest postrzegana jako trudne zadanie do wdrożenia i nie jest w pełni rozwinięta. Programiści często unikają jej, ponieważ do prawidłowego wdrożenia wymaga wiele pracy. Według mnie wynikający z tego wzrost wydajności jest warty tych kosztów. Ponieważ nie ma wielu silników gier, które w pełni wykorzystują wielowątkowość, celem tej pracy jest zaprojektowanie i zaproponowanie wielowątkowej architektury silnika gry 3D, a także przedstawienie głównych systemów używanych do stworzenia takiego silnika gry 3D. Praca skupia się na technologii i architekturze silnika gry i jego podsystemach wraz ze strukturami danych i algorytmami wykorzystywanymi do ich stworzenia. -
Driver Riva Tnt2 64
Driver riva tnt2 64 click here to download The following products are supported by the drivers: TNT2 TNT2 Pro TNT2 Ultra TNT2 Model 64 (M64) TNT2 Model 64 (M64) Pro Vanta Vanta LT GeForce. The NVIDIA TNT2™ was the first chipset to offer a bit frame buffer for better quality visuals at higher resolutions, bit color for TNT2 M64 Memory Speed. NVIDIA no longer provides hardware or software support for the NVIDIA Riva TNT GPU. The last Forceware unified display driver which. version now. NVIDIA RIVA TNT2 Model 64/Model 64 Pro is the first family of high performance. Drivers > Video & Graphic Cards. Feedback. NVIDIA RIVA TNT2 Model 64/Model 64 Pro: The first chipset to offer a bit frame buffer for better quality visuals Subcategory, Video Drivers. Update your computer's drivers using DriverMax, the free driver update tool - Display Adapters - NVIDIA - NVIDIA RIVA TNT2 Model 64/Model 64 Pro Computer. (In Windows 7 RC1 there was the build in TNT2 drivers). http://kemovitra. www.doorway.ru Use the links on this page to download the latest version of NVIDIA RIVA TNT2 Model 64/Model 64 Pro (Microsoft Corporation) drivers. All drivers available for. NVIDIA RIVA TNT2 Model 64/Model 64 Pro - Driver Download. Updating your drivers with Driver Alert can help your computer in a number of ways. From adding. Nvidia RIVA TNT2 M64 specs and specifications. Price comparisons for the Nvidia RIVA TNT2 M64 and also where to download RIVA TNT2 M64 drivers. Windows 7 and Windows Vista both fail to recognize the Nvidia Riva TNT2 ( Model64/Model 64 Pro) which means you are restricted to a low. -
Eindhoven University of Technology MASTER 3D-Graphics Rendering On
Eindhoven University of Technology MASTER 3D-graphics rendering on a multiprocessor architecture van Heesch, F.H. Award date: 2003 Link to publication Disclaimer This document contains a student thesis (bachelor's or master's), as authored by a student at Eindhoven University of Technology. Student theses are made available in the TU/e repository upon obtaining the required degree. The grade received is not published on the document as presented in the repository. The required complexity or quality of research of student theses may vary by program, and the required minimum study period may vary in duration. General rights Copyright and moral rights for the publications made accessible in the public portal are retained by the authors and/or other copyright owners and it is a condition of accessing publications that users recognise and abide by the legal requirements associated with these rights. • Users may download and print one copy of any publication from the public portal for the purpose of private study or research. • You may not further distribute the material or use it for any profit-making activity or commercial gain "77S0 TUIe technische universiteit eindhoven Faculty of Electrical Engineering Section Design Technology For Electronic Systems (ICS/ES) ICS-ES 817 Master's Thesis 3D-GRAPHICS RENDE RING ON A MULTI PROCESSOR ARCHITECTURE. F. van Heesch Coach: Ir. E. Jaspers (Philips Research Laboratories) Dr. E. van der Tol (Philips Research Laboratories) Supervisor: prof.dr.ir. G. de Haan Date: March 2003 The Faculty of Electrical Engineering of the Eindhoven Universily of Technology does not accept any responsibility regarding the contents of Masters Theses Abstract Real-time 3D-graphics rendering is a highly computationally intensive task, with a high memory bandwidth requirement. -
Development of Synthetic Cameras for Virtual Commissioning
DEVELOPMENT OF SYNTHETHIC CAMERAS FOR VIRTUAL COMMISSIONING Bachelor Degree Project in Automation Engineer 2020 DEVELOPMENT OF SYNTHETIC CAMERAS FOR VIRTUAL COMMISSIONING Bachelor Degree Project in Automation Engineering Bachelor Level 30 ECTS Spring term 2020 Francisco Vico Arjona Daniel Pérez Torregrosa Company supervisor: Mikel Ayani University supervisor: Wei Wang Examiner: Stefan Ericson 1 DEVELOPMENT OF SYNTHETHIC CAMERAS FOR VIRTUAL COMMISSIONING Bachelor Degree Project in Automation Engineer 2020 Abstract Nowadays, virtual commissioning has become an incredibly useful technology which has raised its importance hugely in the latest years. Creating virtual automated systems, as similar to reality as possible, to test their behaviour has become into a great tool for avoiding waste of time and cost in the real commissioning stage of any manufacturing system. Currently, lots of virtual automated systems are controlled by different vision tools, however, these tools are not integrated in most of emulation platforms, so it precludes testing the performance of numerous virtual systems. This thesis intends to give a solution to this limitation that nowadays exists for virtual commissioning. The main goal is the creation of a synthetic camera that allows to obtain different types of images inside any virtual automated system in the same way it would have been obtained in a real system. Subsequently, a virtual demonstrator of a robotic cell controlled by computer vision is developed to show the immense opportunities that synthetic camera can open for testing vision systems. 2 DEVELOPMENT OF SYNTHETIC CAMERAS FOR VIRTUAL COMMISSIONING Bachelor Degree Project in Automation Engineering 2020 Certify of Authenticity This thesis has been submitted by Francisco Vico Arjona and Daniel Pérez Torregrosa to the University of Skövde as a requirement for the degree of Bachelor of Science in Production Engineering. -
Programmation Amigaos
Découverte de la Programmation AmigaOS Version 1.0 - 07/11/2003 http://www.guru-meditation.net Avant-propos Bonjour à tous, A l’occasion de l’Alchimie, nous sommes très heureux de faire voir le jour à ce document en français sur la programmation Amiga. Il devrait représenter une précieuse source d’informations et il sera complété et corrigé au fil du temps. Le but de ce livret est de vous offrir des clés, des pistes pour partir sur les chemins du développement en les balisant. Par exemple, vous ne trouverez pas ici de tutoriels sur l’utilisation des bibliothèques ou des devices du système. Nous donnons ici des principes et des conseils mais pas de code : pour des sources et des exemples, nous vous renvoyons à notre site (http://www.guru-meditation.net) et un chapitre est en plus réservé à la recherche d’informations. Nous essaierons de prendre en compte un maximum de configurations possible, de signaler par exemple les spécificités de MorphOS, ... Quelque soit le système, on peut d’ors et déjà déconseiller à tous de “coder comme à la belle époque” comme on entend parfois, c’est à dire en outrepassant le système. Nous souhaitons, par le développement, contribuer à un avenir plus serein de l’Amiga. C’est pourquoi, parfois avec un pincement, nous omettrons de parler d’outils ou de pratiques “révolus”. On conseillera avant tout ceux qui sont maintenus et modernes ... ou encore, à défaut, anciens mais indispensables. Objectif : futur. Ce livret est très porté vers le langage C mais donne malgré tout de nombreux éclairages sur la programmation en général. -
3D Computer Graphics Compiled By: H
animation Charge-coupled device Charts on SO(3) chemistry chirality chromatic aberration chrominance Cinema 4D cinematography CinePaint Circle circumference ClanLib Class of the Titans clean room design Clifford algebra Clip Mapping Clipping (computer graphics) Clipping_(computer_graphics) Cocoa (API) CODE V collinear collision detection color color buffer comic book Comm. ACM Command & Conquer: Tiberian series Commutative operation Compact disc Comparison of Direct3D and OpenGL compiler Compiz complement (set theory) complex analysis complex number complex polygon Component Object Model composite pattern compositing Compression artifacts computationReverse computational Catmull-Clark fluid dynamics computational geometry subdivision Computational_geometry computed surface axial tomography Cel-shaded Computed tomography computer animation Computer Aided Design computerCg andprogramming video games Computer animation computer cluster computer display computer file computer game computer games computer generated image computer graphics Computer hardware Computer History Museum Computer keyboard Computer mouse computer program Computer programming computer science computer software computer storage Computer-aided design Computer-aided design#Capabilities computer-aided manufacturing computer-generated imagery concave cone (solid)language Cone tracing Conjugacy_class#Conjugacy_as_group_action Clipmap COLLADA consortium constraints Comparison Constructive solid geometry of continuous Direct3D function contrast ratioand conversion OpenGL between -
V2.1 User Handbook
V2.1 User Handbook August 24, 2007 i The EMC Team This handbook is a work in progress. If you are able to help with writing, editing, or graphic preparation please contact any member of the writing team or join and send an email to emc- [email protected]. Copyright (c) 2000-6 LinuxCNC.org Permission is granted to copy, distribute and/or modify this document under the terms of the GNU Free Documentation License, Version 1.1 or any later version published by the Free Software Foundation; with no Invariant Sections, no Front-Cover Texts, and one Back-Cover Text: "This EMC Handbook is the product of several authors writing for linuxCNC.org. As you find it to be of value in your work, we invite you to contribute to its revision and growth." A copy of the license is included in the section entitled "GNU Free Documentation License". If you do not find the license you may order a copy from Free Software Foundation, Inc. 59 Temple Place, Suite 330 Boston, MA 02111-1307 Contents I Introduction & installing EMC2 1 1 The Enhanced Machine Control 2 1.1 Introduction ........................................... 2 1.2 The Big CNC Picture ....................................... 2 1.3 Computer Operating Systems ................................. 3 1.4 History of the Software ..................................... 3 1.5 How the EMC2 Works ...................................... 4 1.5.1 Graphical User Interfaces ............................... 5 1.5.2 Motion Controller EMCMOT .............................. 6 1.5.3 Discrete I/O Controller EMCIO ............................ 7 1.5.4 Task Executor EMCTASK ............................... 7 1.6 Thinking Like a Machine Operator .............................. 8 1.6.1 Modes of Operation .................................. -
Graphical Process Unit a New Era
Nov 2014 (Volume 1 Issue 6) JETIR (ISSN-2349-5162) Graphical Process Unit A New Era Santosh Kumar, Shashi Bhushan Jha, Rupesh Kumar Singh Students Computer Science and Engineering Dronacharya College of Engineering, Greater Noida, India Abstract - Now in present days every computer is come with G.P.U (graphical process unit). The graphics processing unit (G.P.U) has become an essential part of today's mainstream computing systems. Over the past 6 years, there has been a marked increase in the performance and potentiality of G.P.U. The modern G.P.Us is not only a powerful graphics engine but also a deeply parallel programmable processor showing peak arithmetic and memory bandwidth that substantially outpaces its CPU counterpart. The G.P.U's speedy increase in both programmability and capability has spawned a research community that has successfully mapped a broad area of computationally demanding, mixed problems to the G.P.U. This effort in general-purpose computing on the G.P.Us, also known as G.P.U computing, has positioned the G.P.U as a compelling alternative to traditional microprocessors in high-performance computer systems of the future. We illustrate the history, hardware, and programming model for G.P.U computing, abstract the state of the art in tools and techniques, and present 4 G.P.U computing successes in games physics and computational physics that deliver order-of- magnitude performance gains over optimized CPU applications. Index Terms - G.P.U, History of G.P.U, Future of G.P.U, Problems in G.P.U, eG.P.U, Integrated graphics ________________________________________________________________________________________________________ I. -
Performance Comparison on Rendering Methods for Voxel Data
fl Performance comparison on rendering methods for voxel data Oskari Nousiainen School of Science Thesis submitted for examination for the degree of Master of Science in Technology. Espoo July 24, 2019 Supervisor Prof. Tapio Takala Advisor Dip.Ins. Jukka Larja Aalto University, P.O. BOX 11000, 00076 AALTO www.aalto.fi Abstract of the master’s thesis Author Oskari Nousiainen Title Performance comparison on rendering methods for voxel data Degree programme Master’s Programme in Computer, Communication and Information Sciences Major Computer Science Code of major SCI3042 Supervisor Prof. Tapio Takala Advisor Dip.Ins. Jukka Larja Date July 24, 2019 Number of pages 50 Language English Abstract There are multiple ways in which 3 dimensional objects can be presented and rendered to screen. Voxels are a representation of scene or object as cubes of equal dimensions, which are either full or empty and contain information about their enclosing space. They can be rendered to image by generating surface geometry and passing that to rendering pipeline or using ray casting to render the voxels directly. In this paper, these methods are compared to each other by using different octree structures for voxel storage. These methods are compared in different generated scenes to determine which factors affect their relative performance as measured in real time it takes to draw a single frame. These tests show that ray casting performs better when resolution of voxel model is high, but rasterisation is faster for low resolution voxel models. Rasterisation scales better with screen resolution and on higher screen resolutions most of the tested voxel resolutions where faster to render with with rasterisation. -
Mediashield User's Guide
ForceWare Software MediaShield User’s Guide Version 5.4 NVIDIA Corporation June 6, 2007 NVIDIA Applications MediaShield User’s Guide Version 5.4 Published by NVIDIA Corporation 2701 San Tomas Expressway Santa Clara, CA 95050 Notice ALL NVIDIA DESIGN SPECIFICATIONS, REFERENCE BOARDS, FILES, DRAWINGS, DIAGNOSTICS, LISTS, AND OTHER DOCUMENTS (TOGETHER AND SEPARATELY, “MATERIALS”) ARE BEING PROVIDED “AS IS.” NVIDIA MAKES NO WARRANTIES, EXPRESSED, IMPLIED, STATUTORY, OR OTHERWISE WITH RESPECT TO THE MATERIALS, AND EXPRESSLY DISCLAIMS ALL IMPLIED WARRANTIES OF NONINFRINGEMENT, MERCHANTABILITY, AND FITNESS FOR A PARTICULAR PURPOSE. Information furnished is believed to be accurate and reliable. However, NVIDIA Corporation assumes no responsibility for the consequences of use of such information or for any infringement of patents or other rights of third parties that may result from its use. No license is granted by implication or otherwise under any patent or patent rights of NVIDIA Corporation. Specifications mentioned in this publication are subject to change without notice. This publication supersedes and replaces all information previously supplied. NVIDIA Corporation products are not authorized for use as critical components in life support devices or systems without express written approval of NVIDIA Corporation. Trademarks NVIDIA, the NVIDIA logo, MediaShield, 3DFX, 3DFX INTERACTIVE, the 3dfx Logo, STB, STB Systems and Design, the STB Logo, the StarBox Logo, NVIDIA nForce, GeForce, NVIDIA Quadro, NVDVD, NVIDIA Personal Cinema, NVIDIA Soundstorm, -
Voodoo 3 2000-3000 Reviewers Guide
Voodoo3™ 2000 /3000 Reviewer’s Guide For reviewers of: Voodoo3 2000 Voodoo3 3000 DRAFT - DATED MATERIAL THE CONTENTS OF THIS REVIEWER’S GUIDE IS INTENDED SOLELY FOR REFERENCE WHEN REVIEWING SHIPPING VERSIONS OF VOODOO3 REFERENCE BOARDS. THIS INFORMATION WILL BE REGULARLY UPDATED, AND REVIEWERS SHOULD CONTACT THE PERSONS LISTED IN THIS GUIDE FOR UPDATES BEFORE EVALUATING ANY VOODOO3 BOARD. 3dfx Interactive, Inc. 4435 Fortran Dr. San Jose, CA 95134 408-935-4400 www.3dfx.com Copyright 1999 3dfx Interactive, Inc. All Rights Reserved. All other trademarks are the property of their respective owners. Voodoo3™ Reviewers Guide August 1999 Table of Contents INTRODUCTION Page 4 SECTION 1: Voodoo3 Board Overview Page 4 • Features • 2D Performance • 3D Performance • Video Performance • Target Audience • Pricing & Availability • Warranty • Technical Support SECTION 2: About the Voodoo3 Board Page 7 • Board Layout - Hardware Configuration & Components - System Requirements • Display Mode Table • Software Drivers • 3dfx Tools Summary SECTION 3: About the Voodoo3 Chip Page 10 • Overview SECTION 4: Installation and Start-Up Page 11 • Installing the Board • Start-Up SECTION 5: Testing Recommendations Page 14 • Testing the Voodoo3 Board • Cures to common benchmarking and image quality mistakes - 2 - Voodoo3™ Reviewers Guide August 1999 Table of Contents (cont.) SECTION 6: FAQ Page 16 SECTION 7: Glossary of 3D Terms Page 19 SECTION 8: Contacts Page 20 APPENDICES 1: Current Benchmark Page 21 • 3D WinBench • 3D Mark • Winbench 99 • Speedy • Game Gauge 1 • Quake II Time Demo 1 at 1600 x 1200 • Quake II Time Demo 1 at 1280 x 1024 Expected Performance of Popular Benchmarks 2: Errata: Known Problems Page 23 3: 3dfx Tools User Guide Page 24 - 3 - Voodoo3™ Reviewers Guide August 1999 INTRODUCTION: The Voodoo3 2000/3000 Reviewer’s Guide is a concise guide to the Voodoo3 143MHz, and 166MHz graphics accelerator boards. -
An Overview Study of Game Engines
Faizi Noor Ahmad Int. Journal of Engineering Research and Applications www.ijera.com ISSN : 2248-9622, Vol. 3, Issue 5, Sep-Oct 2013, pp.1673-1693 RESEARCH ARTICLE OPEN ACCESS An Overview Study of Game Engines Faizi Noor Ahmad Student at Department of Computer Science, ACNCEMS (Mahamaya Technical University), Aligarh-202002, U.P., India ABSTRACT We live in a world where people always try to find a way to escape the bitter realities of hubbub life. This escapism gives rise to indulgences. Products of such indulgence are the video games people play. Back in the past the term ―game engine‖ did not exist. Back then, video games were considered by most adults to be nothing more than toys, and the software that made them tick was highly specialized to both the game and the hardware on which it ran. Today, video game industry is a multi-billion-dollar industry rivaling even the Hollywood. The software that drives these three dimensional worlds- the game engines-have become fully reusable software development kits. In this paper, I discuss the specifications of some of the top contenders in video game engines employed in the market today. I also try to compare up to some extent these engines and take a look at the games in which they are used. Keywords – engines comparison, engines overview, engines specification, video games, video game engines I. INTRODUCTION 1.1.2 Artists Back in the past the term ―game engine‖ did The artists produce all of the visual and audio not exist. Back then, video games were considered by content in the game, and the quality of their work can most adults to be nothing more than toys, and the literally make or break a game.