Optimizing Indirect Branch Prediction Accuracy in Virtual Machine Interpreters
Total Page:16
File Type:pdf, Size:1020Kb
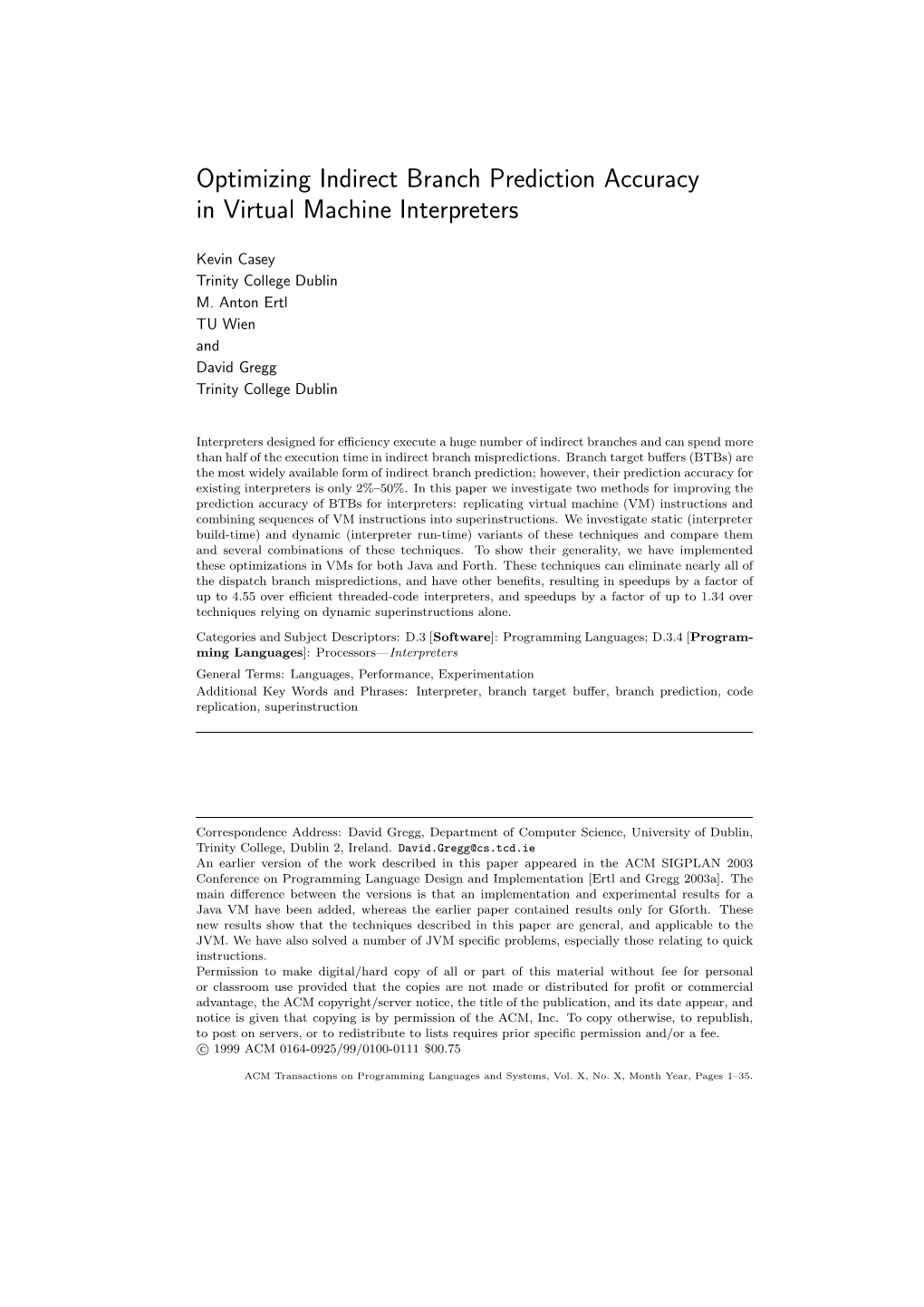
Load more
Recommended publications
-
2. Instruction Set Architecture
!1 2. ISA Gebotys ECE 222 2. Instruction Set Architecture “Ideally, your initial instruction set should be an exemplar, …” " Instruction set architecture (ISA) defines the interface between the hardware and software# " instruction set is the language of the computer# " RISCV instructions are 32-bits, instruction[31:0]# " RISC-V assembly1 language notation # " uses 64-bit registers, 64-bits refer to double word, 32-bits refers to word (8-bits is byte).# " there are 32 registers, namely x0-x31, where x0 is always zero # " to perform arithmetic operations (add, sub, shift, logical) data must always be in registers # " the number of variables in programs is typically larger than 32, hence ‘less frequently used’ [or those used later] variables are ‘spilled’ into memory [spilling registers]# " registers are faster and more energy e$cient than memory# " for embedded applications where code size is important, a 16-bit instruction set exists, RISC-V compressed (e.g. others exist also ARM Thumb and Thumb2)# " byte addressing is used, little endian (where address of 64-bit word refers to address of ‘little' or rightmost byte, [containing bit 0 of word]) so sequential double word accesses di%er by 8 e.g. byte address 0 holds the first double word and byte address 8 holds next double word. (Byte addressing allows the supports of two byte instructions)# " memory contains 261 memory words - using load/store instructions e.g. 64-bits available (bits 63 downto 0, 3 of those bits are used for byte addressing, leaving 61 bits)# " Program counter register (PC) -
Exploiting Branch Target Injection Jann Horn, Google Project Zero
Exploiting Branch Target Injection Jann Horn, Google Project Zero 1 Outline ● Introduction ● Reverse-engineering branch prediction ● Leaking host memory from KVM 2 Disclaimer ● I haven't worked in CPU design ● I don't really understand how CPUs work ● Large parts of this talk are based on guesses ● This isn't necessarily how all CPUs work 3 Variants overview Spectre Meltdown ● CVE-2017-5753 ● CVE-2017-5715 ● CVE-2017-5754 ● Variant 1 ● Variant 2 ● Variant 3 ● Bounds Check ● Branch Target ● Rogue Data Cache Bypass Injection Load ● Primarily affects ● Primarily affects ● Affects kernels (and interpreters/JITs kernels/hypervisors architecturally equivalent software) 4 Performance ● Modern consumer CPU clock rates: ~4GHz ● Memory is slow: ~170 clock cycles latency on my machine ➢ CPU needs to work around high memory access latencies ● Adding parallelism is easier than making processing faster ➢ CPU needs to do things in parallel for performance ● Performance optimizations can lead to security issues! 5 Performance Optimization Resources ● everyone wants programs to run fast ➢ processor vendors want application authors to be able to write fast code ● architectural behavior requires architecture documentation; performance optimization requires microarchitecture documentation ➢ if you want information about microarchitecture, read performance optimization guides ● Intel: https://software.intel.com/en-us/articles/intel-sdm#optimization ("optimization reference manual") ● AMD: https://developer.amd.com/resources/developer-guides-manuals/ ("Software Optimization Guide") 6 (vaguely based on optimization manuals) Out-of-order execution front end out-of-order engine port (scheduler, renaming, ...) port instruction stream add rax, 9 add rax, 8 inc rbx port inc rbx sub rax, rbx mov [rcx], rax port cmp rax, 16 .. -
Simty: Generalized SIMT Execution on RISC-V Caroline Collange
Simty: generalized SIMT execution on RISC-V Caroline Collange To cite this version: Caroline Collange. Simty: generalized SIMT execution on RISC-V. CARRV 2017: First Work- shop on Computer Architecture Research with RISC-V, Oct 2017, Boston, United States. pp.6, 10.1145/nnnnnnn.nnnnnnn. hal-01622208 HAL Id: hal-01622208 https://hal.inria.fr/hal-01622208 Submitted on 7 Oct 2020 HAL is a multi-disciplinary open access L’archive ouverte pluridisciplinaire HAL, est archive for the deposit and dissemination of sci- destinée au dépôt et à la diffusion de documents entific research documents, whether they are pub- scientifiques de niveau recherche, publiés ou non, lished or not. The documents may come from émanant des établissements d’enseignement et de teaching and research institutions in France or recherche français ou étrangers, des laboratoires abroad, or from public or private research centers. publics ou privés. Simty: generalized SIMT execution on RISC-V Caroline Collange Inria [email protected] ABSTRACT and programming languages to target GPU-like SIMT cores. Be- We present Simty, a massively multi-threaded RISC-V processor side simplifying software layers, the unification of CPU and GPU core that acts as a proof of concept for dynamic inter-thread vector- instruction sets eases prototyping and debugging of parallel pro- ization at the micro-architecture level. Simty runs groups of scalar grams. We have implemented Simty in synthesizable VHDL and threads executing SPMD code in lockstep, and assembles SIMD synthesized it for an FPGA target. instructions dynamically across threads. Unlike existing SIMD or We present our approach to generalizing the SIMT model in SIMT processors like GPUs or vector processors, Simty vector- Section 2, then describe Simty’s microarchitecture is Section 3, and izes scalar general-purpose binaries. -
Ep 2182433 A1
(19) & (11) EP 2 182 433 A1 (12) EUROPEAN PATENT APPLICATION published in accordance with Art. 153(4) EPC (43) Date of publication: (51) Int Cl.: 05.05.2010 Bulletin 2010/18 G06F 9/38 (2006.01) (21) Application number: 07768016.3 (86) International application number: PCT/JP2007/063241 (22) Date of filing: 02.07.2007 (87) International publication number: WO 2009/004709 (08.01.2009 Gazette 2009/02) (84) Designated Contracting States: • AOKI, Takashi AT BE BG CH CY CZ DE DK EE ES FI FR GB GR Kawasaki-shi HU IE IS IT LI LT LU LV MC MT NL PL PT RO SE Kanagawa 211-8588 (JP) SI SK TR Designated Extension States: (74) Representative: Ward, James Norman AL BA HR MK RS Haseltine Lake LLP Lincoln House, 5th Floor (71) Applicant: Fujitsu Limited 300 High Holborn Kawasaki-shi, Kanagawa 211-8588 (JP) London WC1V 7JH (GB) (72) Inventors: • TOYOSHIMA, Takashi Kawasaki-shi Kanagawa 211-8588 (JP) (54) INDIRECT BRANCHING PROGRAM, AND INDIRECT BRANCHING METHOD (57) A processor 100 reads an interpreter program the indirect branch instruction in a link register 120c (the 200a to start an interpreter. The interpreter makes branch processor 100 internally stacks the branch destination prediction by executing, in place of an indirect branch addresses also in a return address stack 130) in inverse instruction that is necessary for execution of a source order and reading the addresses from the return address program 200b, storing branch destination addresses in stack 130 in one at a time manner. EP 2 182 433 A1 Printed by Jouve, 75001 PARIS (FR) 1 EP 2 182 433 A1 2 Description vised and brought into practical use. -
Digital Design & Computer Arch. Lecture 17: Branch Prediction II
Digital Design & Computer Arch. Lecture 17: Branch Prediction II Prof. Onur Mutlu ETH Zürich Spring 2020 24 April 2020 Required Readings n This week q Smith and Sohi, “The Microarchitecture of Superscalar Processors,” Proceedings of the IEEE, 1995 q H&H Chapters 7.8 and 7.9 q McFarling, “Combining Branch Predictors,” DEC WRL Technical Report, 1993. 2 Recall: How to Handle Control Dependences n Critical to keep the pipeline full with correct sequence of dynamic instructions. n Potential solutions if the instruction is a control-flow instruction: n Stall the pipeline until we know the next fetch address n Guess the next fetch address (branch prediction) n Employ delayed branching (branch delay slot) n Do something else (fine-grained multithreading) n Eliminate control-flow instructions (predicated execution) n Fetch from both possible paths (if you know the addresses of both possible paths) (multipath execution) 3 Recall: Fetch Stage with BTB and Direction Prediction Direction predictor (taken?) taken? PC + inst size Next Fetch Address Program hit? Counter Address of the current branch target address Cache of Target Addresses (BTB: Branch Target Buffer) 4 Recall: More Sophisticated Direction Prediction n Compile time (static) q Always not taken q Always taken q BTFN (Backward taken, forward not taken) q Profile based (likely direction) q Program analysis based (likely direction) n Run time (dynamic) q Last time prediction (single-bit) q Two-bit counter based prediction q Two-level prediction (global vs. local) q Hybrid q Advanced algorithms -
Leveraging Indirect Branch Locality in Dynamic Binary Translators
Leveraging Indirect Branch Locality in Dynamic Binary Translators Balaji Dhanasekaran Kim Hazelwood University of Virginia University of Virginia [email protected] [email protected] Abstract an application depending on how frequent a particular Dynamic Binary Translators (DBTs) have a wide range of code path is executed. It is extremely difficult to make applications like program instrumentation, dynamic opti- optimization decisions like these at compile time. But, mization, and security. One of the main issues with DBTs with the help of binary translators, we can instrument is their performance overhead. A significant part of this the application at runtime and choose the appropriate overhead is caused by indirect branch (IB) translation. In optimization based on the execution characteristics of the this paper, we show that the percentage of instructions application. DBTs provide enormous power in the hands spent in translating indirect branches is as high as 50% of of the users to analyze and optimize their applications at the total guest application’s instructions. The time spent runtime. DBTs are also used in other applications like in indirect branch translation is so high that in some ap- instrumentation (Pin [8] and DynamoRIO [2]), security plications, bounding the code cache size actually results (Strata [9]), dynamic translation (Rosetta [1]), and design in an increased performance, since code cache flushes also space exploration (Daisy [4]). remove stale indirect branch information along with the One of the main problems with DBTs is their perfor- translated code. In order to improve the performance of in- mance overhead. Figure 1 shows the relative performance direct branches, we analyze the locality of indirect branch of the SPEC2006 INT benchmarks when executed under targets and show that the locality is as high as 70%. -
Intel Itanium 2 Processor Reference Manual
Intel® Itanium® 2 Processor Reference Manual For Software Development and Optimization May 2004 Order Number: 251110-003 THIS DOCUMENT IS PROVIDED “AS IS” WITH NO WARRANTIES WHATSOEVER, INCLUDING ANY WARRANTY OF MERCHANTABILITY, FITNESS FOR ANY PARTICULAR PURPOSE, OR ANY WARRANTY OTHERWISE ARISING OUT OF ANY PROPOSAL, SPECIFICATION OR SAMPLE. Information in this document is provided in connection with Intel® products. No license, express or implied, by estoppel or otherwise, to any intellectual property rights is granted by this document. Except as provided in Intel's Terms and Conditions of Sale for such products, Intel assumes no liability whatsoever, and Intel disclaims any express or implied warranty, relating to sale and/or use of Intel products including liability or warranties relating to fitness for a particular purpose, merchantability, or infringement of any patent, copyright or other intellectual property right. Intel products are not intended for use in medical, life saving, or life sustaining applications. Intel may make changes to specifications and product descriptions at any time, without notice. Designers must not rely on the absence or characteristics of any features or instructions marked “reserved” or “undefined.” Intel reserves these for future definition and shall have no responsibility whatsoever for conflicts or incompatibilities arising from future changes to them. The Pentium, Itanium and IA-32 architecture processors may contain design defects or errors known as errata which may cause the product to deviate from published specifications. Current characterized errata are available on request. Contact your local Intel sales office or your distributor to obtain the latest specifications and before placing your product order. -
Understanding and Predicting Indirect Branch Behavior
Understanding and Predicting Indirect Branch Behavior Adrian FLOREA, Lucian N. VINTAN “Lucian Blaga” University of Sibiu, Computer Science Department, Str. E. Cioran, No. 4, Sibiu-550025, ROMANIA, Tel./Fax: +40-269-212716, E-mail: [email protected], [email protected], [email protected] Abstract: Understanding program behavior is at the foundation of computer architecture and program optimization. Machine code usually has much less semantic information than source code, which makes it much more difficult to discover control flow or data flow information. The main aim of this paper consists in extracting some corpus program and features from procedural and object- oriented applications or execution characteristics of desktop applications that generate indirect function call (unavoidable breaks in control) at machine level. We also made a comparative analysis of C and C++ languages behavior from the execution point of view on instruction level parallelism architectures. The simulation part of the paper mainly refers to measuring the locality degree of target addresses on series of architectural resources but specially to adapting some knowing prediction schemes (Two Context Predictors named Target Cache and, respectively, the complete PPM Predictor) to predict indirect jump instructions. PPM predictor seems to be an almost ultimate limit of target prediction, and, thus, a good frame for deriving practical prediction schemes. Keywords Procedural and object-oriented programs, indirect branches / function calls, virtual functions, dynamic branch prediction, instruction level parallel architectures, SPEC benchmarks, dynamic link libraries (DLL). 1. Introduction As the average instruction issue rate and depth of the pipeline in multiple-instruction-issue (MII) processors increase, accurate dynamic branch prediction becomes more and more essential. -
Design of Digital Circuits Lecture 19: Branch Prediction II, VLIW, Fine-Grained Multithreading
Design of Digital Circuits Lecture 19: Branch Prediction II, VLIW, Fine-Grained Multithreading Prof. Onur Mutlu ETH Zurich Spring 2018 4 May 2018 Agenda for Today & Next Few Lectures n Single-cycle Microarchitectures n Multi-cycle and Microprogrammed Microarchitectures n Pipelining n Issues in Pipelining: Control & Data Dependence Handling, State Maintenance and Recovery, … n Out-of-Order Execution n Other Execution Paradigms 2 Readings for Today n Smith and Sohi, “The Microarchitecture of Superscalar Processors,” Proceedings of the IEEE, 1995 q More advanced pipelining q Interrupt and exception handling q Out-of-order and superscalar execution concepts n H&H Chapters 7.8 and 7.9 n Optional: q Kessler, “The Alpha 21264 Microprocessor,” IEEE Micro 1999. q McFarling, “Combining Branch Predictors,” DEC WRL Technical Report, 1993. 3 Approaches to (Instruction-Level) Concurrency n Pipelining n Out-of-order execution n Dataflow (at the ISA level) n Superscalar Execution n VLIW n Fine-Grained Multithreading n SIMD Processing (Vector and array processors, GPUs) n Decoupled Access Execute n Systolic Arrays 4 Branch Prediction Wrap-Up 5 Recall: Alpha 21264 Tournament Predictor n Minimum branch penalty: 7 cycles n Typical branch penalty: 11+ cycles n 48K bits of target addresses stored in I-cache n Predictor tables are reset on a context switch n Kessler, “The Alpha 21264 Microprocessor,” IEEE Micro 1999. 6 Recall: Are We Done w/ Branch Prediction? n Hybrid branch predictors work well q E.g., 90-97% prediction accuracy on average n Some “difficult” -
Instruction Set Define Computer
Instruction set define computer Continue A set of abstract symbols, describing the operations of a computer program for the processor, should not be confused with the architecture of industry standards. Machine Code General Concepts Instruction set Opcode Illegal opcode Opcode table Operand Instructions NOP Branch Indirect Branch vte In Computer Sciences, The Architecture Set of Instructions (ISA) is an abstract computer model. It is also called architecture or computer architecture. Implementing an ISA, such as a CPU, is called implementation. In general, the ISA identifies supported data types, registers, hardware support for basic memory management, core functions (such as memory consistency, processing modes, virtual memory) and the ISA implementation family I/O model. IsA determines the behavior of the machine code running on the implementations of this ISA in a way that does not depend on the characteristics of this implementation, providing binary compatibility between implementations. This allows multiple ISA implementations that differ in performance, physical size and cash costs (among other things), but which are capable of running the same machine code, so that lower performance, lower cost machines can be replaced by higher cost, higher machine performance without replacing the software. It also allows the evolution of microarchitectures to implement this ISA, so that new, higher performance ISA implementations can run software that works on previous generations of implementations. If the operating system supports the standard and compatible binary application interface (ABI) for a specific ISA, the machine code for this ISA and operating system will work on future implementations of this ISA and new versions of this operating system. -
AMD Architecture Guidelines for Indirect Branch Control
White Paper | AMD64 TECHNOLOGY INDIRECT BRANCH CONTROL EXTENSION REVISION 4.10.18 AMD64 TECHNOLOGY INDIRECT BRANCH CONTROL EXTENSION This document describes an indirect branch control feature designed to mitigate indirect branch target injection on AMD products. There are three defined mechanisms: Indirect Branch Prediction Barrier (IBPB), Indirect Branch Restricted Speculation (IBRS), and Single Thread Indirect Branch Prediction mode (STIBP). PRESENCE The presence of the three features are indicated through three CPUID bits. AMD does enumerate these features differently than other x86 vendors. IBPB support is indicated by CPUID Function 8000_0008, EBX[12]=1. IBRS support is indicated by CPUID Function 8000_0008, EBX[14]=1. STIBP support is indicated by CPUID Function 8000_0008, EBX[15]=1. Support for IBPB implies that MSR 0x49 exists in the architecture. Support for IBRS or STIBP implies MSR 0x48 exists. Here is a simple representation in table form: FEATURE AMD VERSION MSR EXIST IBPB 8000_0008 EBX[12]=1 PRED_CMD (MSR 49) IBRS 8000_0008 EBX[14]=1 SPEC_CTRL (MSR 48) STIBP 8000_0008 EBX[15]=1 SPEC_CTRL (MSR 48) USAGE The indirect branch control extension is essentially three features that allow for increased control of indirect branches. IBPB Indirect branch prediction barrier (IBPB) exists at MSR 0x49 (PRED_CMD) bit 0. This is a write only MSR that both GP faults when software reads it or if software tries to write any of the bits in 63:1. When bit zero is written, the processor guarantees that older indirect branches cannot influence predictions of indirect branches in the future. This applies to jmp indirects, call indirects and returns. -
Predicated Code
Computer Architecture: Branch Prediction (II) and Predicated Execution Prof. Onur Mutlu Carnegie Mellon University A Note on This Lecture These slides are partly from 18-447 Spring 2013, Computer Architecture, Lecture 12: Predicated Execution Video of that lecture: http://www.youtube.com/watch?v=xtA1arYjq-M 2 Last Lecture Branch prediction 3 Today’s Agenda Wrap up control dependence handling State recovery mechanisms, interrupts, exceptions 4 Control Dependence Handling 5 Review: How to Handle Control Dependences Critical to keep the pipeline full with correct sequence of dynamic instructions. Potential solutions if the instruction is a control-flow instruction: Stall the pipeline until we know the next fetch address Guess the next fetch address (branch prediction) Employ delayed branching (branch delay slot) Do something else (fine-grained multithreading) Eliminate control-flow instructions (predicated execution) Fetch from both possible paths (if you know the addresses of both possible paths) (multipath execution) 6 Review: Importance of The Branch Problem Assume a 5-wide superscalar pipeline with 20-cycle branch resolution latency How long does it take to fetch 500 instructions? Assume no fetch breaks and 1 out of 5 instructions is a branch 100% accuracy 100 cycles (all instructions fetched on the correct path) No wasted work 99% accuracy 100 (correct path) + 20 (wrong path) = 120 cycles 20% extra instructions fetched 98% accuracy 100 (correct path) + 20 * 2 (wrong path) = 140 cycles 40% extra instructions