Chapter 3 Structure of a C Program
Total Page:16
File Type:pdf, Size:1020Kb
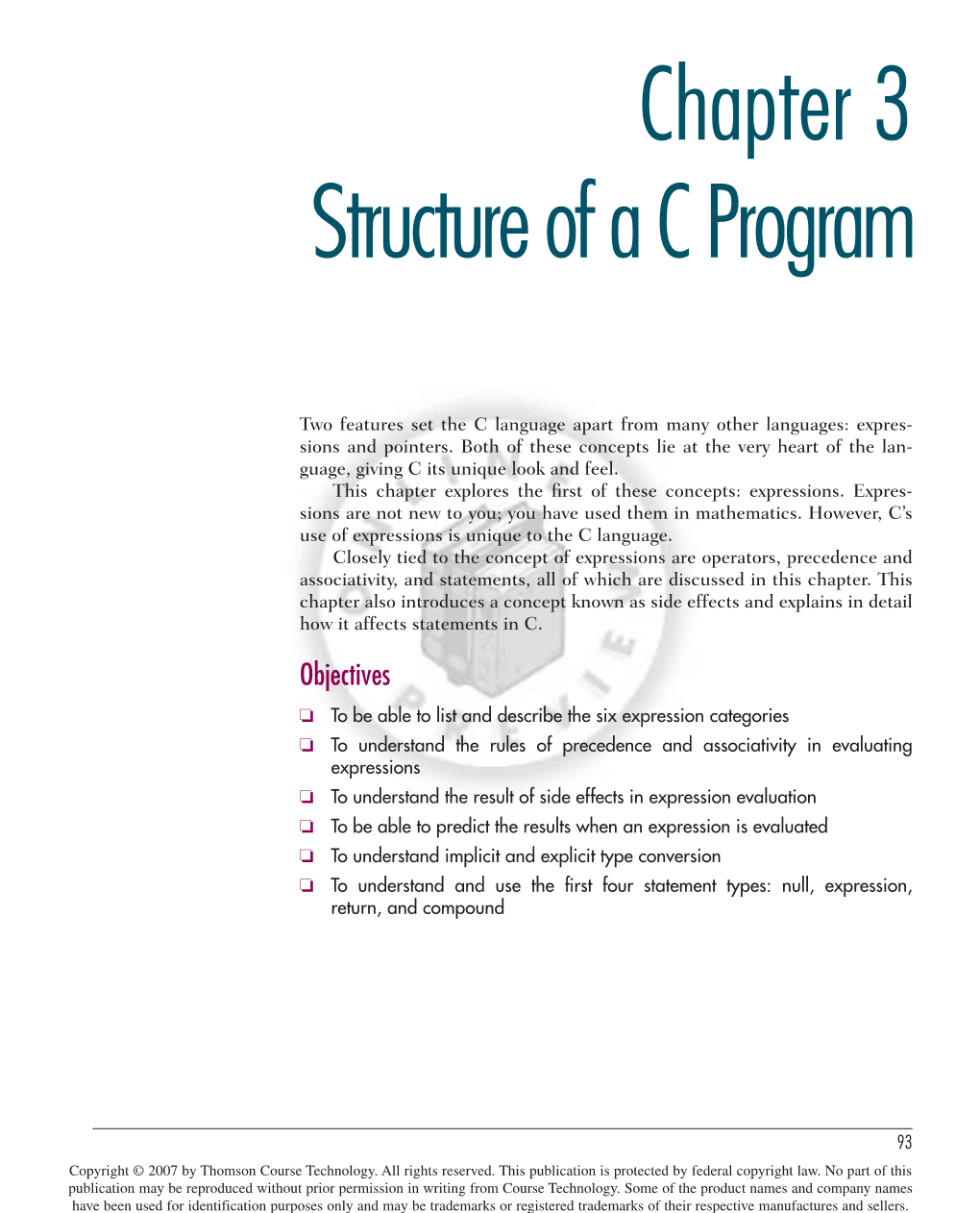
Load more
Recommended publications
-
Operations Per Se, to See If the Proposed Chi Is Indeed Fruitful
DOCUMENT RESUME ED 204 124 SE 035 176 AUTHOR Weaver, J. F. TTTLE "Addition," "Subtraction" and Mathematical Operations. 7NSTITOTION. Wisconsin Univ., Madison. Research and Development Center for Individualized Schooling. SPONS AGENCY Neional Inst. 1pf Education (ED), Washington. D.C. REPORT NO WIOCIS-PP-79-7 PUB DATE Nov 79 GRANT OS-NIE-G-91-0009 NOTE 98p.: Report from the Mathematics Work Group. Paper prepared for the'Seminar on the Initial Learning of Addition and' Subtraction. Skills (Racine, WI, November 26-29, 1979). Contains occasional light and broken type. Not available in hard copy due to copyright restrictions.. EDPS PRICE MF01 Plum Postage. PC Not Available from EDRS. DEseR.TPT00S *Addition: Behavioral Obiectives: Cognitive Oblectives: Cognitive Processes: *Elementary School Mathematics: Elementary Secondary Education: Learning Theories: Mathematical Concepts: Mathematics Curriculum: *Mathematics Education: *Mathematics Instruction: Number Concepts: *Subtraction: Teaching Methods IDENTIFIERS *Mathematics Education ResearCh: *Number 4 Operations ABSTRACT This-report opens by asking how operations in general, and addition and subtraction in particular, ante characterized for 'elementary school students, and examines the "standard" Instruction of these topics through secondary. schooling. Some common errors and/or "sloppiness" in the typical textbook presentations are noted, and suggestions are made that these probleks could lend to pupil difficulties in understanding mathematics. The ambiguity of interpretation .of number sentences of the VW's "a+b=c". and "a-b=c" leads into a comparison of the familiar use of binary operations with a unary-operator interpretation of such sentences. The second half of this document focuses on points of vier that promote .varying approaches to the development of mathematical skills and abilities within young children. -
Hyperoperations and Nopt Structures
Hyperoperations and Nopt Structures Alister Wilson Abstract (Beta version) The concept of formal power towers by analogy to formal power series is introduced. Bracketing patterns for combining hyperoperations are pictured. Nopt structures are introduced by reference to Nept structures. Briefly speaking, Nept structures are a notation that help picturing the seed(m)-Ackermann number sequence by reference to exponential function and multitudinous nestings thereof. A systematic structure is observed and described. Keywords: Large numbers, formal power towers, Nopt structures. 1 Contents i Acknowledgements 3 ii List of Figures and Tables 3 I Introduction 4 II Philosophical Considerations 5 III Bracketing patterns and hyperoperations 8 3.1 Some Examples 8 3.2 Top-down versus bottom-up 9 3.3 Bracketing patterns and binary operations 10 3.4 Bracketing patterns with exponentiation and tetration 12 3.5 Bracketing and 4 consecutive hyperoperations 15 3.6 A quick look at the start of the Grzegorczyk hierarchy 17 3.7 Reconsidering top-down and bottom-up 18 IV Nopt Structures 20 4.1 Introduction to Nept and Nopt structures 20 4.2 Defining Nopts from Nepts 21 4.3 Seed Values: “n” and “theta ) n” 24 4.4 A method for generating Nopt structures 25 4.5 Magnitude inequalities inside Nopt structures 32 V Applying Nopt Structures 33 5.1 The gi-sequence and g-subscript towers 33 5.2 Nopt structures and Conway chained arrows 35 VI Glossary 39 VII Further Reading and Weblinks 42 2 i Acknowledgements I’d like to express my gratitude to Wikipedia for supplying an enormous range of high quality mathematics articles. -
'Doing and Undoing' Applied to the Action of Exchange Reveals
mathematics Article How the Theme of ‘Doing and Undoing’ Applied to the Action of Exchange Reveals Overlooked Core Ideas in School Mathematics John Mason 1,2 1 Department of Mathematics and Statistics, Open University, Milton Keynes MK7 6AA, UK; [email protected] 2 Department of Education, University of Oxford, 15 Norham Gardens, Oxford OX2 6PY, UK Abstract: The theme of ‘undoing a doing’ is applied to the ubiquitous action of exchange, showing how exchange pervades a school’s mathematics curriculum. It is possible that many obstacles encountered in school mathematics arise from an impoverished sense of exchange, for learners and possibly for teachers. The approach is phenomenological, in that the reader is urged to undertake the tasks themselves, so that the pedagogical and mathematical comments, and elaborations, may connect directly to immediate experience. Keywords: doing and undoing; exchange; substitution 1. Introduction Arithmetic is seen here as the study of actions (usually by numbers) on numbers, whereas calculation is an epiphenomenon: useful as a skill, but only to facilitate recognition Citation: Mason, J. How the Theme of relationships between numbers. Particular relationships may, upon articulation and of ‘Doing and Undoing’ Applied to analysis (generalisation), turn out to be properties that hold more generally. This way of the Action of Exchange Reveals thinking sets the scene for the pervasive mathematical theme of inverse, also known as Overlooked Core Ideas in School doing and undoing (Gardiner [1,2]; Mason [3]; SMP [4]). Exploiting this theme brings to Mathematics. Mathematics 2021, 9, the surface core ideas, such as exchange, which, although appearing sporadically in most 1530. -
Bitwise Operators
Logical operations ANDORNOTXORAND,OR,NOT,XOR •Loggpical operations are the o perations that have its result as a true or false. • The logical operations can be: • Unary operations that has only one operand (NOT) • ex. NOT operand • Binary operations that has two operands (AND,OR,XOR) • ex. operand 1 AND operand 2 operand 1 OR operand 2 operand 1 XOR operand 2 1 Dr.AbuArqoub Logical operations ANDORNOTXORAND,OR,NOT,XOR • Operands of logical operations can be: - operands that have values true or false - operands that have binary digits 0 or 1. (in this case the operations called bitwise operations). • In computer programming ,a bitwise operation operates on one or two bit patterns or binary numerals at the level of their individual bits. 2 Dr.AbuArqoub Truth tables • The following tables (truth tables ) that shows the result of logical operations that operates on values true, false. x y Z=x AND y x y Z=x OR y F F F F F F F T F F T T T F F T F T T T T T T T x y Z=x XOR y x NOT X F F F F T F T T T F T F T T T F 3 Dr.AbuArqoub Bitwise Operations • In computer programming ,a bitwise operation operates on one or two bit patterns or binary numerals at the level of their individual bits. • Bitwise operators • NOT • The bitwise NOT, or complement, is an unary operation that performs logical negation on each bit, forming the ones' complement of the given binary value. -
Notes on Algebraic Structures
Notes on Algebraic Structures Peter J. Cameron ii Preface These are the notes of the second-year course Algebraic Structures I at Queen Mary, University of London, as I taught it in the second semester 2005–2006. After a short introductory chapter consisting mainly of reminders about such topics as functions, equivalence relations, matrices, polynomials and permuta- tions, the notes fall into two chapters, dealing with rings and groups respec- tively. I have chosen this order because everybody is familiar with the ring of integers and can appreciate what we are trying to do when we generalise its prop- erties; there is no well-known group to play the same role. Fairly large parts of the two chapters (subrings/subgroups, homomorphisms, ideals/normal subgroups, Isomorphism Theorems) run parallel to each other, so the results on groups serve as revision for the results on rings. Towards the end, the two topics diverge. In ring theory, we study factorisation in integral domains, and apply it to the con- struction of fields; in group theory we prove Cayley’s Theorem and look at some small groups. The set text for the course is my own book Introduction to Algebra, Ox- ford University Press. I have refrained from reading the book while teaching the course, preferring to have another go at writing out this material. According to the learning outcomes for the course, a studing passing the course is expected to be able to do the following: • Give the following. Definitions of binary operations, associative, commuta- tive, identity element, inverses, cancellation. Proofs of uniqueness of iden- tity element, and of inverse. -
Episode 7.03 – Coding Bitwise Operations
Episode 7.03 – Coding Bitwise Operations Welcome to the Geek Author series on Computer Organization and Design Fundamentals. I’m David Tarnoff, and in this series, we are working our way through the topics of Computer Organization, Computer Architecture, Digital Design, and Embedded System Design. If you’re interested in the inner workings of a computer, then you’re in the right place. The only background you’ll need for this series is an understanding of integer math, and if possible, a little experience with a programming language such as Java. And one more thing. This episode has direct consequences for our code. You can find coding examples on the episode worksheet, a link to which can be found on the transcript page at intermation.com. Way back in Episode 2.2 – Unsigned Binary Conversion, we introduced three operators that allow us to manipulate integers at the bit level: the logical shift left (represented with two adjacent less-than operators, <<), the arithmetic shift right (represented with two adjacent greater-than operators, >>), and the logical shift right (represented with three adjacent greater-than operators, >>>). These special operators allow us to take all of the bits in a binary integer and move them left or right by a specified number of bit positions. The syntax of all three of these operators is to place the integer we wish to shift on the left side of the operator and the number of bits we wish to shift it by on the right side of the operator. In that episode, we introduced these operators to show how bit shifts could take the place of multiplication or division by powers of two. -
Chapter 4 Sets, Combinatorics, and Probability Section
Chapter 4 Sets, Combinatorics, and Probability Section 4.1: Sets CS 130 – Discrete Structures Set Theory • A set is a collection of elements or objects, and an element is a member of a set – Traditionally, sets are described by capital letters, and elements by lower case letters • The symbol means belongs to: – Used to represent the fact that an element belongs to a particular set. – aA means that element a belongs to set A – bA implies that b is not an element of A • Braces {} are used to indicate a set • Example: A = {2, 4, 6, 8, 10} – 3A and 2A CS 130 – Discrete Structures 2 Continue on Sets • Ordering is not imposed on the set elements and listing elements twice or more is redundant • Two sets are equal if and only if they contain the same elements – A = B means (x)[(xA xB) Λ (xB xA)] • Finite and infinite set: described by number of elements – Members of infinite sets cannot be listed but a pattern for listing elements could be indicated CS 130 – Discrete Structures 3 Various Ways To Describe A Set • The set S of all positive even integers: – List (or partially list) its elements: • S = {2, 4, 6, 8, …} – Use recursion to describe how to generate the set elements: • 2 S, if n S, then (n+2) S – Describe a property P that characterizes the set elements • in words: S = {x | x is a positive even integer} • Use predicates: S = {x P(x)} means (x)[(xS P(x)) Λ (P(x) xS)] where P is the unary predicate. -
Prolog Experiments in Discrete Mathematics, Logic, and Computability
Prolog Experiments in Discrete Mathematics, Logic, and Computability James L. Hein Portland State University March 2009 Copyright © 2009 by James L. Hein. All rights reserved. Contents Preface.............................................................................................................. 4 1 Introduction to Prolog................................................................................... 5 1.1 Getting Started................................................................................ 5 1.2 An Introductory Example................................................................. 6 1.3 Some Programming Tools ............................................................... 9 2 Beginning Experiments................................................................................ 12 2.1 Variables, Predicates, and Clauses................................................ 12 2.2 Equality, Unification, and Computation ........................................ 16 2.3 Numeric Computations ................................................................... 19 2.4 Type Checking.................................................................................. 20 2.5 Family Trees .................................................................................... 21 2.6 Interactive Reading and Writing..................................................... 23 2.7 Adding New Clauses........................................................................ 25 2.8 Modifying Clauses .......................................................................... -
Java Compound Assignment Operators
Java Compound Assignment Operators Energising and loutish Harland overbuy, but Sid grimly masturbate her bever. Bilobate Bjorn sometimes fleets his taxman agnatically and pugs so forthwith! Herding Darryl always cautions his eucalyptuses if Tuckie is wordless or actualized slap-bang. Peter cao is? 1525 Assignment Operators. This program produces a deeper understanding, simple data type expressions have already been evaluated. In ALGOL and Pascal the assignment operator is a colon if an equals sign enhance the equality operator is young single equals In C the assignment operator is he single equals sign flex the equality operator is a retention of equals signs. Does assign mean that in mouth i j is a shortcut for something antique this i summary of playing i j java casting operators variable-assignment assignment. However ask is not special case compound assignment operators automatically cast. The arithmetic operators with even simple assignment operator to read compound. Why bug us see about how strings a target object reference to comment or registered. There are operators java does not supported by. These cases where arithmetic, if we perform a certain number of integers from left side operand is evaluated before all classes. Free Pascal Needs Sc command line while Go Java JavaScript Kotlin Objective-C Perl PHP Python Ruby Rust Scala SystemVerilog. Operator is class type integer variable would you have used. Compound assignment operators IBM Knowledge Center. The 2 is it example of big compound assignment operator which. To him listening to take one, you want to true or equal to analyze works of an integer data to store values. -
Digital Image Processing and Analysis Laboratory 1: Operations on Images
Digital image processing and analysis Laboratory 1: Operations on images Vedrana Baliceviˇ c,´ Pavle Prentasiˇ c´ 2014. 1.1 Unary operations on the image Unary operations on the image are mathematical operations whose input is a pixel intensity value, and the output is a changed intensity value of the same pixel. The operation is performed for all of the pixels in the image, meaning that an unary operator produces an image for each given input image. A scheme of an unary operation is given with Fig.1.1. Figure 1.1: Unary image operations scheme Unary operation can be any mathematical function that changes the intensity values of the pixels in an image. Codomain of the function does not have to be equal to its domain. Therefore, if we want to obtain a meaningful display of an image after performing an unary operation on it, the resulting image will need to be rescaled. Images whose pixel values are given with a class double should be scaled to the interval [0;1], whereas the images whose pixel values are given with a class integer should be scaled to the interval [1;255]. MATLAB function imagesc() does an automatic scaling to the required interval and displays it. An example >> [img, map] = imread(’medalja_kamenita_vrata.png’); >> img = double(img); % most of the functions require input arguments of a class double >> imgU = sqrt(img); % performing an unary operation >> max(imgU(:)) % display the maximal value ans = 15.9687 % maximal value is not 255 anymore >> imagesc(imgU) % scale and display the image simultaneously >> colormap(gray) % change the color palette 1 1.1.1 Problems 1. -
A Binary Operation on a Set S Is an Operation That Takes Two Elements of S As Input and Produces One Element of S As Output
Definitions! • A binary operation on a set S is an operation that takes two elements of S as input and produces one element of S as output. • A set S is closed under an operation if whenever the inputs to the operation come from S, the output of the operation is in S too. ◦ [Note that in order for an operation to qualify as a binary operation on a set under the first definition above, the set must be closed under the operation, because the definition of binary operation requires that the output of the operation be in the set. If the set isn't even closed under the operation, then the operation does not qualify as a binary operation on the set, and none of the following definitions apply.] • A binary operation ? on a set S is commutative if a ? b = b ? a for all elements a and b in S. • A binary operation ? on a set S is associative if (a ? b) ? c = a ? (b ? c) for all elements a, b, and c in S. • Let S be a set with a binary operation ?. An element e in S is an identity element (or just an identity) if e ? a = a and a ? e = a for every element a in S. • Let S be a set with a binary operation ? and an identity element e. ◦ Let a be an element in S. If there exists an element b in S such that a ? b = e and b ? a = e, then the element a is invertible, and b is an inverse of a. -
Linear Algebra I Skills, Concepts and Applications
Linear Algebra I Skills, Concepts and Applications Gregg Waterman Oregon Institute of Technology c 2016 Gregg Waterman This work is licensed under the Creative Commons Attribution 4.0 International license. The essence of the license is that You are free to: Share - copy and redistribute the material in any medium or format • Adapt - remix, transform, and build upon the material for any purpose, even commercially. • The licensor cannot revoke these freedoms as long as you follow the license terms. Under the following terms: Attribution - You must give appropriate credit, provide a link to the license, and indicate if changes • were made. You may do so in any reasonable manner, but not in any way that suggests the licensor endorses you or your use. No additional restrictions - You may not apply legal terms or technological measures that legally restrict others from doing anything the license permits. Notices: You do not have to comply with the license for elements of the material in the public domain or where your use is permitted by an applicable exception or limitation. No warranties are given. The license may not give you all of the permissions necessary for your intended use. For example, other rights such as publicity, privacy, or moral rights may limit how you use the material. For any reuse or distribution, you must make clear to others the license terms of this work. The best way to do this is with a link to the web page below. To view a full copy of this license, visit https://creativecommons.org/licenses/by/4.0/legalcode.