Chapter 4, Adding Character to Your Game, Shows How to Load and Convert Models
Total Page:16
File Type:pdf, Size:1020Kb
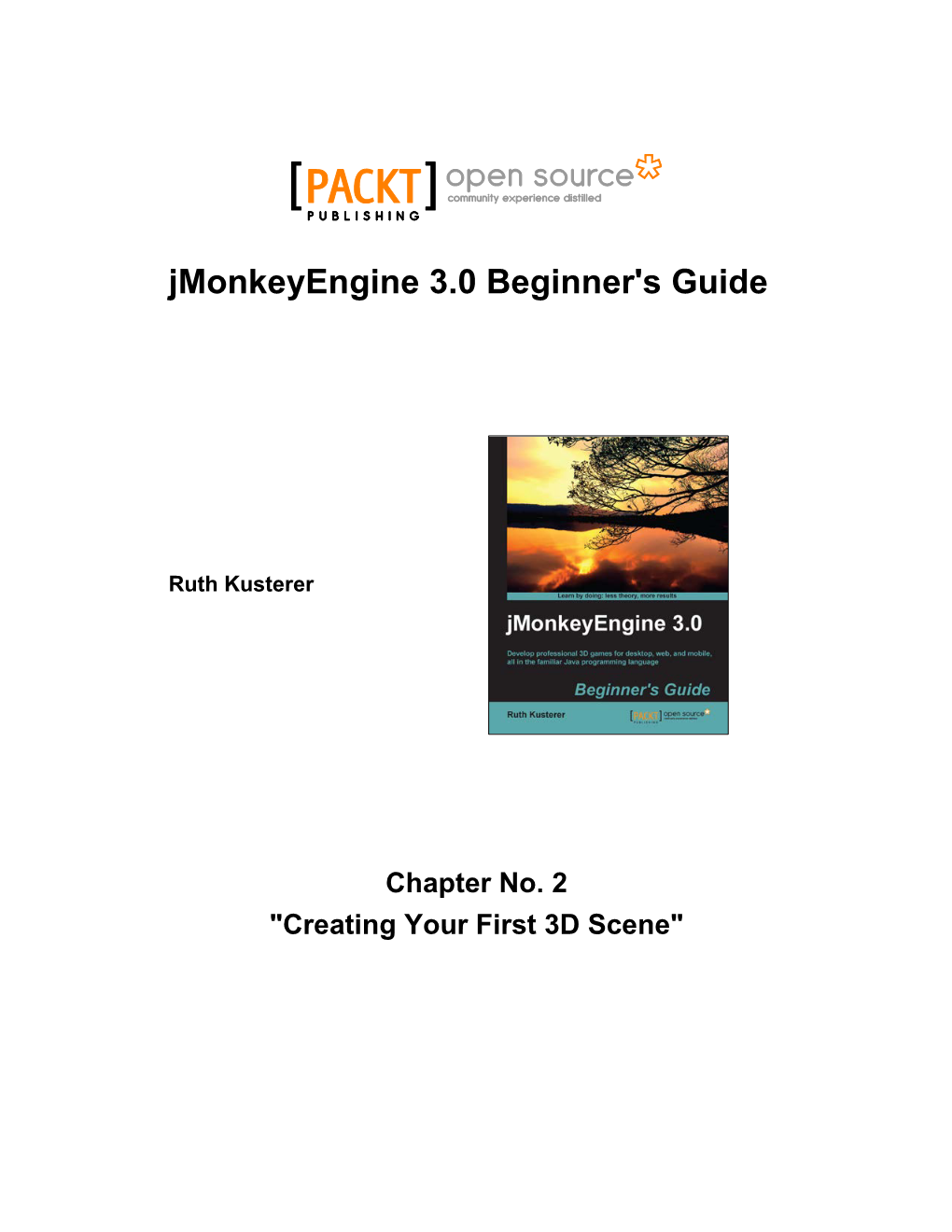
Load more
Recommended publications
-
Nethomura Deployment Platform and Networking Middleware Is Still Evolving
Networking Middleware and Online-Deployment Mechanisms for Java-based Games Chris Carter1, Dr Abdennour El Rhalibi1, Prof. Madjid Merabti1, Dr Marc Price2 (1) School of Computing & Mathematical Sciences, Liverpool John Moores University, Byrom Street, Liverpool, L3 3AF, UK [email protected]; [email protected]; [email protected] (2) BBC Research and Development. Kingswood Warren, Tadworth, Surrey, KT20 6NP, UK [email protected] Abstract. Currently, web-based online gaming applications are predominately utilising Adobe Flash or Java Applets as their core technologies. These games are often casual, two- dimensional games and do not utilise the specialist graphics hardware which has proliferated across modern PCs and Consoles. Multi-user online game play in these titles is often either non-existent or extremely limited. Computer games applications which grace the current generation of consoles and personal computers are designed to utilise the increasingly impressive hardware power at their disposal. However, these are commonly distributed using a physical medium or deployed through custom, proprietary networking mechanisms and rely upon platform-specific networking APIs to facilitate multi-user online game play. In order to unify the concepts of these disparate styles of gaming, this paper presents two interconnected systems which are implemented using Java Web Start and JXTA P2P technologies, providing a platform-independent framework capable of deploying hardware accelerated cross-platform, cross-browser online-enabled Java games, as part of the Homura Project. Keywords: Web Technologies, Distributed Systems, Java, Homura, NetHomura, Java Monkey Engine, jME, Java Web Start, Deployment, JXTA, Peer to Peer Networking, P2P Games. -
Advanced 3D Visualization for Simulation Using Game Technology
Proceedings of the 2011 Winter Simulation Conference S. Jain, R. R. Creasey, J. Himmelspach, K. P. White, and M. Fu, eds. ADVANCED 3D VISUALIZATION FOR SIMULATION USING GAME TECHNOLOGY Jonatan L. Bijl Csaba A. Boer TBA B.V. Karrepad 2a 2623 AP Delft, Netherlands ABSTRACT 3D visualization is becoming increasingly popular for discrete event simulation. However, the 3D visu- alization of many of the commercial off the shelf simulation packages is not up to date with the quickly developing area of computer graphics. In this paper we present an advanced 3D visualization tool, which uses game technology. The tool is especially fit for discrete event simulation, it is easily configurable, and it can be kept up to date with modern computer graphics techniques. The tool has been used in several container terminal simulation projects. From a survey under simulation experts and our experience with the visualization tool, we concluded that realism is important for some of the purposes of visualization, and the use of game technology can help to achieve this goal. 1 INTRODUCTION Simulation is the process of designing a model of a concrete system and conducting experiments with this model in order to understand the behavior of the concrete system and/or evaluate various strategies for the operation of the system (Shannon 1975). Although the simulation experiment can produce a large amount of data, the visualization of a simulated system provides a more complete understanding of its behavior. In the visualization, key elements of the system are represented on the screen by icons that dynamically change position, color and shape as the simulation evolves through time (Law and Kelton 2000). -
Topics from Graphics 1
Topics from Graphics 1 Image taken from http://www.felixgers.de/teaching/game/game-modules-talk/GameEngineAndModules.html 1 Synthetic Camera Model Images copied from http://www.cs.unm.edu/~angel/BOOK/INTERACTIVE_COMPUTER_GRAPHICS/SIXTH_EDITION/ART/ 2 Camera Specification Six degrees of freedom Position of center of lens (COP) Orientation Lens – focal length Film size (h,w) Orientation of film plane Images copied from http://www.cs.unm.edu/~angel/BOOK/INTERACTIVE_COMPUTER_GRAPHICS/SIXTH_EDITION/ART/ 3 LookAt Images copied from http://www.cs.unm.edu/~angel/BOOK/INTERACTIVE_COMPUTER_GRAPHICS/SIXTH_EDITION/ART/ 4 Perspective Frustum: a truncated pyramid Images copied from http://www.cs.unm.edu/~angel/BOOK/INTERACTIVE_COMPUTER_GRAPHICS/SIXTH_EDITION/ART/ 5 and slightly modified Using Field of View front plane fovy – angle in up direction aspect = w/h Images copied from http://www.cs.unm.edu/~angel/BOOK/INTERACTIVE_COMPUTER_GRAPHICS/SIXTH_EDITION/ART/ 6 Clipping Just as a real camera cannot “see” the whole world, the virtual camera can only see part of the world or object space Objects that are not within this view volume are said to be clipped out of the scene Images copied from http://www.cs.unm.edu/~angel/BOOK/INTERACTIVE_COMPUTER_GRAPHICS/SIXTH_EDITION/ART/ 7 Projection Projection: from 3D objects to 2D image Perspective projections: all projectors meet at the center of projection Parallel (orthogonal) projection: projectors are parallel, center of projection is replaced by a direction of projection 8 Orthogonal Viewing Images copied from http://www.cs.unm.edu/~angel/BOOK/INTERACTIVE_COMPUTER_GRAPHICS/SIXTH_EDITION/ART/ 9 and slightly modified Texture Mapping Image copied from http://blog.tartiflop.com/2008/11/first-steps-in-away3d-part-3-texture-mapping/ 10 Mapping a Texture A function f(u,v)=(x ,y ,z ) defines (u,v) (u,v) (u,v) the object position of each pixel in the texture, see this. -
Introduction to Jmonkeyengine 3
Introduction to jMonkeyEngine What is jMonkeyEngine? A jME Application Scene graphs Coordinate systems What is jMonkeyEngine? • jME is a game engine made for developers who want to create 3D games and other visualisation applications following modern technology standards • Uses Java and is platform independent. Can deploy to Windows, Mac, Linux, Android and iOS. • OpenSource, non-profit, New BSD License TWi Feb 15 Features of jMonkeyEngine • Has integrated tools to make it easier to create games and applications – Physics integration – Special effects (pre/post processing, particles) – Terrain-, Vegetation-, Water-systems++ – Graphical User Interface – Networking TWi Feb 15 Showcase • http://www.youtube.com/watch? v=eRC9FDin5dA&feature=player_embedded • http://jmonkeyengine.org/showcase/ TWi Feb 15 TWi Feb 15 TWi Feb 15 Why use a high level API? • Faster development process • Not necessary to reinvent the wheel • Provides abstraction from the low level: – Think Objects…. Not vertices – Think content… not rendering process. • Not necessary to tell when to draw, just tell what to draw – Retained mode • This does not mean you do not need to understand what is going on underneath • This is a programming course TWi Feb 15 What does jME do? • Uses OpenGL, and features a modern shader based architecture (GLSL) • Organises your scene with a scene graph data structure • Transformations and mathematics • jME performs rendering optimisations – View frustum culling – State sorting – Batching • jME is single threaded • jME is NOT thread safe. Only modify -
LJMU Research Online
CORE Metadata, citation and similar papers at core.ac.uk Provided by LJMU Research Online LJMU Research Online Tang, SOT and Hanneghan, M State-of-the-Art Model Driven Game Development: A Survey of Technological Solutions for Game-Based Learning http://researchonline.ljmu.ac.uk/205/ Article Citation (please note it is advisable to refer to the publisher’s version if you intend to cite from this work) Tang, SOT and Hanneghan, M (2011) State-of-the-Art Model Driven Game Development: A Survey of Technological Solutions for Game-Based Learning. Journal of Interactive Learning Research, 22 (4). pp. 551-605. ISSN 1093-023x LJMU has developed LJMU Research Online for users to access the research output of the University more effectively. Copyright © and Moral Rights for the papers on this site are retained by the individual authors and/or other copyright owners. Users may download and/or print one copy of any article(s) in LJMU Research Online to facilitate their private study or for non-commercial research. You may not engage in further distribution of the material or use it for any profit-making activities or any commercial gain. The version presented here may differ from the published version or from the version of the record. Please see the repository URL above for details on accessing the published version and note that access may require a subscription. For more information please contact [email protected] http://researchonline.ljmu.ac.uk/ State of the Art Model Driven Game Development: A Survey of Technological Solutions for Game-Based Learning Stephen Tang* and Martin Hanneghan Liverpool John Moores University, James Parsons Building, Byrom Street, Liverpool, L3 3AF, United Kingdom * Corresponding author. -
Creación De Un Motor De Videojuegos 2D Multiplataforma De Código Abierto
Trabajo Fin de Grado JAGE (JustAnotherGameEngine): Creación de un motor de videojuegos 2D mu ti! ata"orma de código abierto Autor#es Rub%n Tomás Gracia Director#es Eduardo 'ena (ieto Grado en )ngenier*a )nformática Escue a de )ngenier*a + Ar,uitectura 2-./ Repositorio de la Universidad de Zaragoza – Zaguan http://zaguan.unizar.es Para mi abuelo Juan y mi tío abuelo Mateo, por ser quienes más influyeron en mi interés por los videojuegos Agradecimientos A mi fami ia, !or nunca rechazar mi afición !or os videojuegos2 A mi novia, !or apo+arme siem!re + a+udarme a seguir trabajando. A mis amigos, !or a+udarme en as !ruebas de !royecto. JAGE (JustAnotherGameEngine): !reación de un motor de videojuegos $% multiplataforma de código abierto Resumen 3oy en d*a os videojuegos est&n más !resentes en a vida diaria de o ,ue nunca han estado. 4on mucho más accesib es !ara a gente con !ocos medios0 Asimismo, a e5tensión de a )nformática ha !ropiciado e hecho de ,ue mucha gente ha+a !odido adoptar a !rogramación como hobb+2 Esto ha desencadenado un estal ido en e mundo de os videojuegos0 de gente ,ue !rograma sus !ropios videojuegos con bajo (o ning6n) !resu!uesto !ara uego !ub icar os en )nternet: conocidos como juegos indie0 est&n ahora en su mejor momento. Todo esto ha evado a cabo una retroalimentación !or a ,ue cada ve1 os videojuegos están más ace!tados0 + m&s gente ,uiere hacer su !ropio juego. 'uchas !ersonas no ,uiere un videojuego comercial0 solo e5! otar su ado art*stico en un mundo ,ue disfrutan0 + !or e o cada ve1 han surgido m&s herramientas -
Visual Representation of 3D Language Constructs Specified By
Visual Representation of 3D Language Constructs Specified by Generic Depictions Jan Wolter University of Paderborn Department of Computer Science F¨urstenallee11, 33102 Paderborn, Germany [email protected] Abstract Several modeling domains make use of three-dimensional representations, e.g., the \ball-and-stick" models of molecules. Our generator framework DEViL3D supports the design and implementation of visual 3D languages for such modeling purposes. The front-end of a language implementation generated by DEViL3D is a dedicated 3D graphical structure editor, which is used to con- struct programs in that domain. DEViL3D supports the language designer to describe the visual appearance of the constructs of the particular language in terms of generic 3D depictions. Their parameters specify where substructures are embedded, and how the graphic adapts to space requirements of nested con- structs. The 3D editor used for such specifications is generated by DEViL3D, too. In this paper, we briefly introduce the research field of 3D visual lan- guages and report about our generator framework and the role that generic depictions play in the specification process for 3D languages. Our results show that our approach is suitable for a wide range of 3D languages. We emphasize this suitability by presenting requirements on the visual appearance for different languages. Key words. three-dimensional depictions, visual languages, visual program- ming, automated generation, 3D interaction techniques. Contents 1 Introduction 2 2 DEViL3D 3 3 Generic depictions 5 3.1 Application of Generic Depictions . .7 3.2 The 3D Editor for Generic Depictions . .8 3.3 Code-generation . 11 arXiv:1311.5126v1 [cs.PL] 20 Nov 2013 4 Range of application 12 5 Related Work 15 6 Conclusion 15 This paper is a extended version of my paper Specifying Generic Depictions of Language Con- structs for 3D Visual Languages, which I presented at the VL/HCC 2013: http://dx.doi.org/10. -
Game Design and Development Course Taught with Alice
GAME DESIGN AND DEVELOPMENT COURSE TAUGHT WITH ALICE Karen Villaverde, Daniel Jaramillo New Mexico State University 1290 Frenger Mall SH 123, Las Cruces, NM 88003 575-646-3723 [email protected] , [email protected] ABSTRACT In this paper we describe our very positive experience in teaching a game design and development course with Alice as a 3D game development platform. We describe why Alice was chosen as our 3D game development platform, what material was covered, how the course was conducted, the quality of the students’ game projects, features of Alice that students liked for game development, problems that students had when using Alice, and the evaluation of the course. 1. INTRODUCTION We always wanted to teach a game design and development course that would cover the theory behind game design, the use of a 3D game development platform, and also give students enough time to develop two complete games. However, the very steep learning curve of most 3D game development platforms prevented us from doing everything we wanted to do in one semester. Then we decided to give Alice [5] an opportunity. In the spring of 2009, we taught a game design and development course using Alice as our 3D game development platform. The course was fourteen weeks long and was taught in the Computer Science department of New Mexico State University. This was the first time that a game design and development course was taught in our department. The course prerequisite was a data structures and algorithms course. The course was extremely popular and filled up completely on the first day of registration. -
Introduction to Jmonkeyengine
Introduction to jMonkeyEngine What is jMonkeyEngine? Scene graphs A jME Application Coordinate systems What is jMonkeyEngine? • jME is a game engine made for developers who want to create 3D games and other visualisation applications following modern technology standards • Uses Java and is platform independent. Can deploy to windows, mac, linux and android. • OpenSource, non-profit, New BSD License • http://www.youtube.com/watch? v=eRC9FDin5dA&feature=player_embedded 26/09/2013 TWi Sept 13 What is jMonkeyEngine? • Has integrated tools to make it easier to create games and applications – Physics – Special effects (pre/post processing, particles) – Terrain-, Vegetation-, Water-systems++ – Graphical User Interface – Networking 26/09/2013 TWi Sept 13 Why use a high level API? • Faster development process • Not necessary to reinvent the wheel • Provides abstraction from the low level: – Think Objects…. Not vertices – Think content… not rendering process. 26/09/2013 TWi Sept 13 What does jME do? • jME performs rendering optimisation – View frustum culling – Batching – State sorting • Achieves high performance by rendering via OpenGL • Uses a modern shader based architecture • Helps organize your 3D scenes, transformations • jME is single threaded • jME is NOT thread safe. Only modify the scenegraph from the rendering thread. 26/09/2013 TWi Sept 13 Applications of jME • Games • Education • Scientific visualisation • Information visualisation • Geographic Information Systems (GIS) • Computer-aided design(CAD) • Animation 26/09/2013 TWi Sept 13 Getting -
Serious Games: the Confluence of Virtual Reality, Simulation & Modeling, and Immersive Education
SABBATICAL PROJECT REPORT – PART II Serious Games: The Confluence of Virtual Reality, Simulation & Modeling, and Immersive Education Dr. Robert H. Seidman, Ph.D. Professor of Computer Information Technology August 31, 2009 APPENDICES I. Game journals & magazines ..……………………………….……………………………………………. A-2 Scholarly journals - print ……………………………..…………………………………………………… A-2 Scholarly journals – online only ………………………..……………………………………………... A-5 Trade or non-scholarly publications – print ……………………………..…………………….…. A-7 Trade or non-scholarly publications/websites – online …………………………….………. A-11 II. Game conferences & proceedings ..…………………………………………………..…….……..….. A-12 Serious games ……………………………………………………………………………..……………….. A-12 Simulation ………………………………………………………………………………………………………. A-14 Immersive education ……………………………………………………………..………………………. A-16 Proceedings ……………………………………………………………………………………………………. A-18 III. Virtual worlds …………………………………………………………………….………………………..….. A-25 Social virtual worlds …………………………………………………………………………………..…... A-25 Closed social virtual worlds …………………………………………….…………………………… A-37 Educational virtual worlds …………………………………………….…………………………….….. A-38 MMOGs (Massively Multiplayer Online Games) …………………………………………….… A-39 Closed MMOGs ……………………………………………………………………………………….…. A-46 Virtual world building platforms ………………………………………………………………….…. A-47 IV. Game engines ………………………………………………………..………………………………………..… A-51 V. Bibliography ………………………………………….…………………………………………………….…… A-66 Miscellaneous organizations and projects ………………………………………………….…… A-164 Dr. Seidman – August -
Guide D'accompagnement
Interfaces graphiques 3D orientés Web Travail de diplôme réalisé en vue de l’obtention du diplôme HES par : Renaud SAUVAIN Conseiller au travail de diplôme : Peter DAEHNE, Professeur HES Genève, 5 septembre 2008 Haute École de Gestion de Genève (HEG-GE) Filière Informatique de Gestion Déclaration Ce travail de diplôme est réalisé dans le cadre de l’examen final de la Haute école de gestion de Genève, en vue de l’obtention du titre de bachelor of science. L’étudiant accepte, le cas échéant, la clause de confidentialité. L'utilisation des conclusions et recommandations formulées dans le travail de diplôme, sans préjuger de leur valeur, n'engage ni la responsabilité de l'auteur, ni celle du conseiller au travail de diplôme, du juré et de la HEG. « J’atteste avoir réalisé seul(e ) le présent travail, sans avoir utilisé des sources autres que celles citées dans la bibliographie. » Fait à Genève, le 05.09.08 Renaud Sauvain Remerciements Ce travail de diplôme ayant été mené à bien avec le soutien de quelques personnes, je veux ici leur adresser mes meilleurs remerciements. Je voudrais notamment citer les personnes et entreprises ayant donné de leurs temps pour répondre à mes questions concernant l'utilisation des interfaces 3D orientés Web, dont : Benjamin Pugliese (Iomedia), Vincent Greset (Innovagency), Luc St-Arnaud (Optaros) et M Wong (W3 Media). Un grand merci à Romain Sauvain et Christine Bovet pour leur soutien et conseils avisés, ainsi qu'à M Peter Daehne qui m'a suivi tout au long de ce projet. Interfaces graphiques 3D orienté Web SAUVAIN, Renaud i Sommaire Représentant le meilleur de la technologie informatique, démonstration de la puissance de calcul des ordinateurs dernier cri, depuis toujours associé à une atmosphère futuriste et passionnante, l'affichage en trois dimensions a depuis des années envahi le contenu vidéo ludique de nos ordinateurs. -
Download Simulation Software for Free to Help Them to Learn How to Create Electrical Power Networks
Project Number: DC MQP 1102 Empowerment Simulation System April 23, 2012 A Major Qualifying Project Report: Submitted to the Faculty of WORCESTER POLYTECHNIC INSTITUTE in partial fulfillment of the requirements for the Degree of Bachelor of Science in Interactive Media and Game Development By: Timothy Kolek Advised by: Professor David Cyganski Abstract Empowerment is a new game being developed by WPI and FIRST, inspired by the FIRST Robotics Competition, aimed at educating high school students about Energy. The project goal was creation of a sandbox for game physics and GUI exploration. It allows a player to configure electrical power networks using 3D models of various (and adjustable) types of generators, consumers, transmission towers and interconnecting power lines. The simulator demonstrates the automatic solution for power distribution, a core component of game state, based upon system parameters and player choices. The simulator was built upon the JMonkey 3 game engine in Java, yielding an operating system agnostic, open source implementation as a basis for the future game system implementation. 2 Table of Contents Abstract ......................................................................................................................................................... 2 Table of Contents .......................................................................................................................................... 3 Table of Figures ............................................................................................................................................