Coppin State College
Total Page:16
File Type:pdf, Size:1020Kb
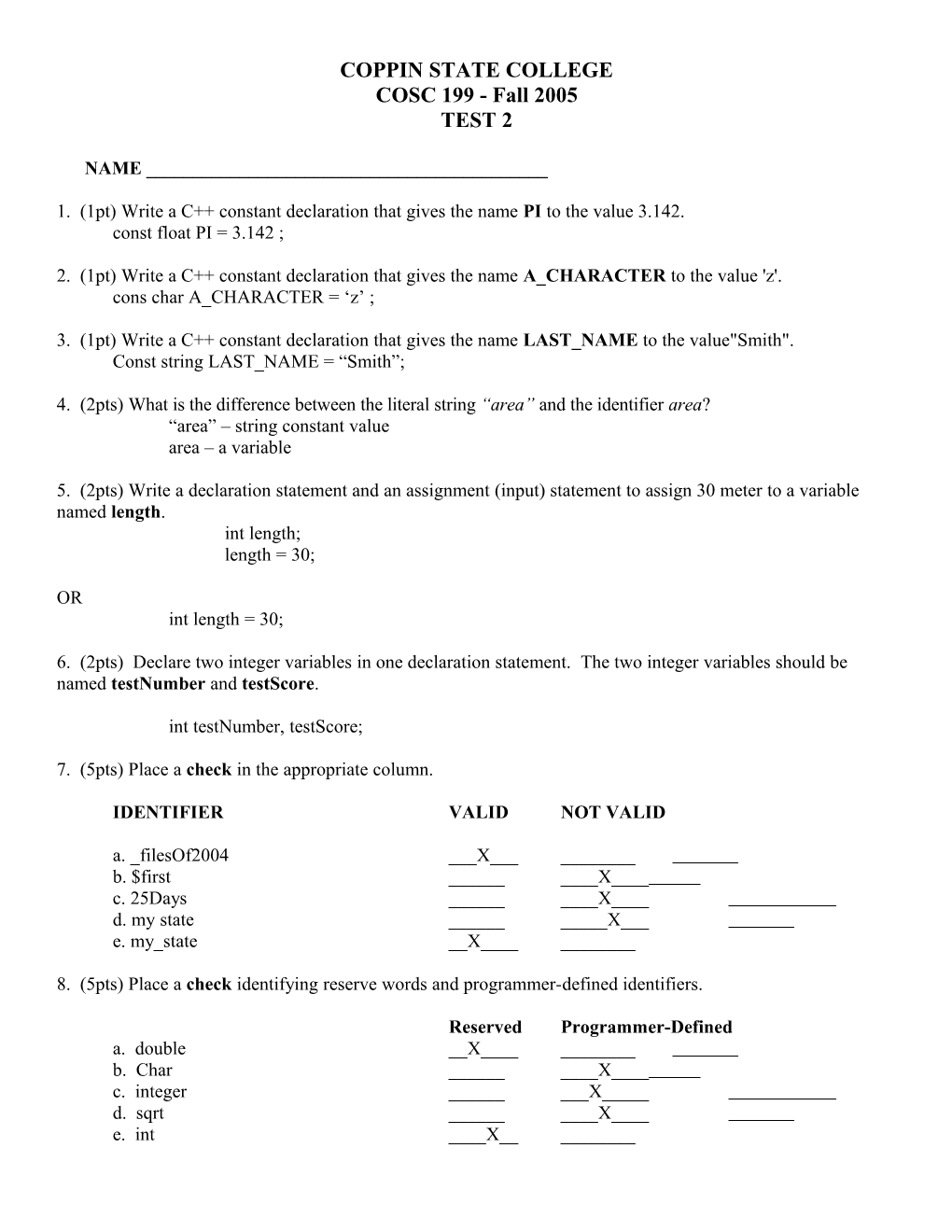
COPPIN STATE COLLEGE COSC 199 - Fall 2005 TEST 2
NAME ______
1. (1pt) Write a C++ constant declaration that gives the name PI to the value 3.142. const float PI = 3.142 ;
2. (1pt) Write a C++ constant declaration that gives the name A_CHARACTER to the value 'z'. cons char A_CHARACTER = ‘z’ ;
3. (1pt) Write a C++ constant declaration that gives the name LAST_NAME to the value"Smith". Const string LAST_NAME = “Smith”;
4. (2pts) What is the difference between the literal string “area” and the identifier area? “area” – string constant value area – a variable
5. (2pts) Write a declaration statement and an assignment (input) statement to assign 30 meter to a variable named length. int length; length = 30;
OR int length = 30;
6. (2pts) Declare two integer variables in one declaration statement. The two integer variables should be named testNumber and testScore.
int testNumber, testScore;
7. (5pts) Place a check in the appropriate column.
IDENTIFIER VALID NOT VALID
a. _filesOf2004 ___X______b. $first ______X____ c. 25Days ______X____ d. my state ______X___ e. my_state __X______
8. (5pts) Place a check identifying reserve words and programmer-defined identifiers.
Reserved Programmer-Defined a. double __X______b. Char ______X____ c. integer ______X_____ d. sqrt ______X____ e. int ____X______9. You want to divide 21 by 4.
(a) (1pt) How do you write the expression in C++ if you want the result to be the floating- point value 5.25? _____21 / 4.0 or 21.0 / 4.0, or 21.0 / 4______
(b) (1pt) How do you write the expression in C++ if you want only the integer quotient? ____21 / 4______
(c) (1pt) How do you write the expression in C++ if you want only the remainder? _____21 % 4 ____
10. (1pt) Among the C++ operators +, *, /, %, <, &&, ||, ! which one(s) has or have the lowest precedence?
A) && B) || C) *, /, and % D) < E) ! F. +
11. (1pt) Among the C++ operators unary -, +, -, *, /, %, and =, which one(s) has or have the highest precedence?
A) +, - B) = C) *, /, and % D) % E) / F) +
12. (2pts) The value of the C++ expression 9 / 5 * 2 is:
A) 3.6 B) 0.9 C) 0 D) 2 E) none
13. (2pts) The value of the C++ expression 5 / 9 * 2 is:
A) 0.27… B) 1.11… C) 0 D) 2 E) none
14. (2pts) Given that x is a int variable and num is an float variable containing the value 10.5, what will x contain after execution of the following statement: x = num + 2;
A) 12.5 B) 10.5 C) 12 D) nothing; a compile-time error occurs
15. (2pts) Given that x is a float variable containing the value 80.9 and num is an int variable, what will num contain after execution of the following statement: num = x + 2.9;
A) 83.8 B) 84.0 C) 83 D) 83.0 E) nothing; a compile-time error occurs
16. (2pts) The value of the C++ expression 13 + 21 % 4 - 2 is:
A) 0 B) 12 C) 16 D) 14 E) none of the above
17. (2pts) Given that x is a float variable and num is an int variable containing the value 38, what will x contain after execution of the following statement: x = num / 4 + 3.0;
A) 12.5 B) 13 C) 12 D) 12.0 E) nothing; a compile-time error occurs
18. (5pts) If originally x = 5, y = 4, and z = 2, what is the value of the following expression? Solve step-by- step. y * (x + y) % 3 / z + 10 = 10
19. (2pts) Write a valid C++ expression for the following algebraic expression:
x ---- - 32 _x / (y * z) - 32 yz
20. (2pts) Write a valid C++ expression for the following algebraic expression:
xy ------______x * y / (y – 32)______y - 32
21. (2pts) Write a valid C++ expression for the following algebraic expression:
x + 32 ------(x – 2y) ______(x + 32) / (y – 32) – (x – 2 * y) y - 32
22. A.(5pts) Write the following mathematical equation to a C++ equivalent expression. Use sqrt(x) and pow(x, y) functions where appropriate.
3ijk + k9 result = ------Answer: 7ik - 5j + k result = (3 * I * j * k + pow (k, 9)) / (7 * I * k – 5 * sqrt(j = k))
B. (2pts) In the above expression, identify input variables and output variables.
Input variable(s): _____i , j, k______
Output variable(s): _____result ______
C. (2pts) If you have to write a C++ program to solve the above equation, what is or are the header file(s) to be included as Preprocessor Directive Statements?
23. (2pts) Write an output statement that prints your first name.
cout << “Joe”;
24. (2pts) Given the constant declaration: const int CONSTANT = 95; Which of the following is not a valid use of CONSTANT? A. cout << CONSTANT * 3; B. CONSTANT = 24; C. cin >> CONSTANT; D. A and C E. B and C above
25. (10pts) the following program is to convert temperatures in Celsius from Fahrenheit temperatures. In the following program, two types of blanks are there: Comment types (inactive statements) and C++ codes (active statements). Fill up the blanks appropriately.
// ___Preprocessor Directives______#include
int main() { // Declaration Statements float celsius; // __output______variable. float fahrenheit; // ___input______variable
// _____Input______Statements cout << "Please enter the temperature: ; cin >> ___fahrenheit______;
// ______?Process statement______celsius = 5.0 / 9.0 * (fahrenheit - 32.0) ;
// Output Statements cout<< "Given Temperature in degree Fah = " << __ fahrenheit __ << endl << "Equivalent Temperature in degree ____Celsius______is " << ____ celsius _____ << endl ;
// Terminate the main function and return to Operating System return 0; } B. (2pts) Write the OUTPUT of the program if you enter the value of the input variable, fahrenheit = 32.0 Given Temperature in degree Fah = 32.0 Equivalent Temperature in degree Celsius is 0.0
26. (10pts) Trace the following program:
Statements 1. #include
27. The following C++ program has several syntax errors: (Note line numbers are not included in the program)
1. #include
A. (5pts) Identify the line numbers (all lines are essential to solve the problem) that are not correctly written:
__1, 5, 7, 8, 9, 10, 11, 12, 13______
B. (5pts) Write only those lines in correct syntax below:
#include
NOTE: Line 11 is a logical error and not a syntax error. Fin why? C. (5pts) After the syntax corrections, suppose you entered the value of radius as 3.0 in Line #8. Write the correct output of the program.
The Area of the Circle is201.09for the radius = 8
28. (2pts) What is the output of the following C++ Code?
x = 5; if ( x > 10 ) { cout << “Line 1” << endl; cout << “Line 2” << endl; } else cout << “Line 3” << endl; cout << “Line 4” << endl;
OUTPUT: Line 3 Line 4
29. (2pts) What is the output of the following C++ Code?
p = 1; if ( p >= 0 ) cout << “Hi” << endl; else cout << “Bye” << endl; cout << “Thank you” << endl;
OUTPUT: HI Thank you
30. (2pts) What is the output of the following C++ Code for the input data 35 30 36 24 cin >> a >> b >> c >> d; if (a > b | | a > c && c != d ) 35> 30 || 35 > 36 && 36 != 24 T || F && T T || F = T m = a; else if (b > d) m = b; else m = c; cout << m << endl;
OUTPUT: 35
31. (2pts) After execution of the following code, what will be the value of angle if the input value is 4?
cin >> angle; if (angle == 5) angle = angle + 10; else if (angle > 2) angle = angle + 5;
Value of angle is _____9 ______
32. (2pts) After execution of the following code, what will be the value of angle if the input value is 0?
cin >> angle; if (angle == 5) angle = angle + 10; else if (angle > 2) angle = angle + 5;
Value of angle is ____0 ______
32. (2pts) What is the output of the following C++ code fragment?
int1 = 5; cin >> int2; // Assume user types 6 if ((int1 <= 5) || (int2 > 6)) int3 = int1 / int2; else int3 = int1 % int2; cout << int1 << int2 << int3;
OUTPUT: 560