Four Useful VBA Utilities for SAS® Programmers David Franklin, Theprogrammerscabin.Com, Litchfield, NH
Total Page:16
File Type:pdf, Size:1020Kb
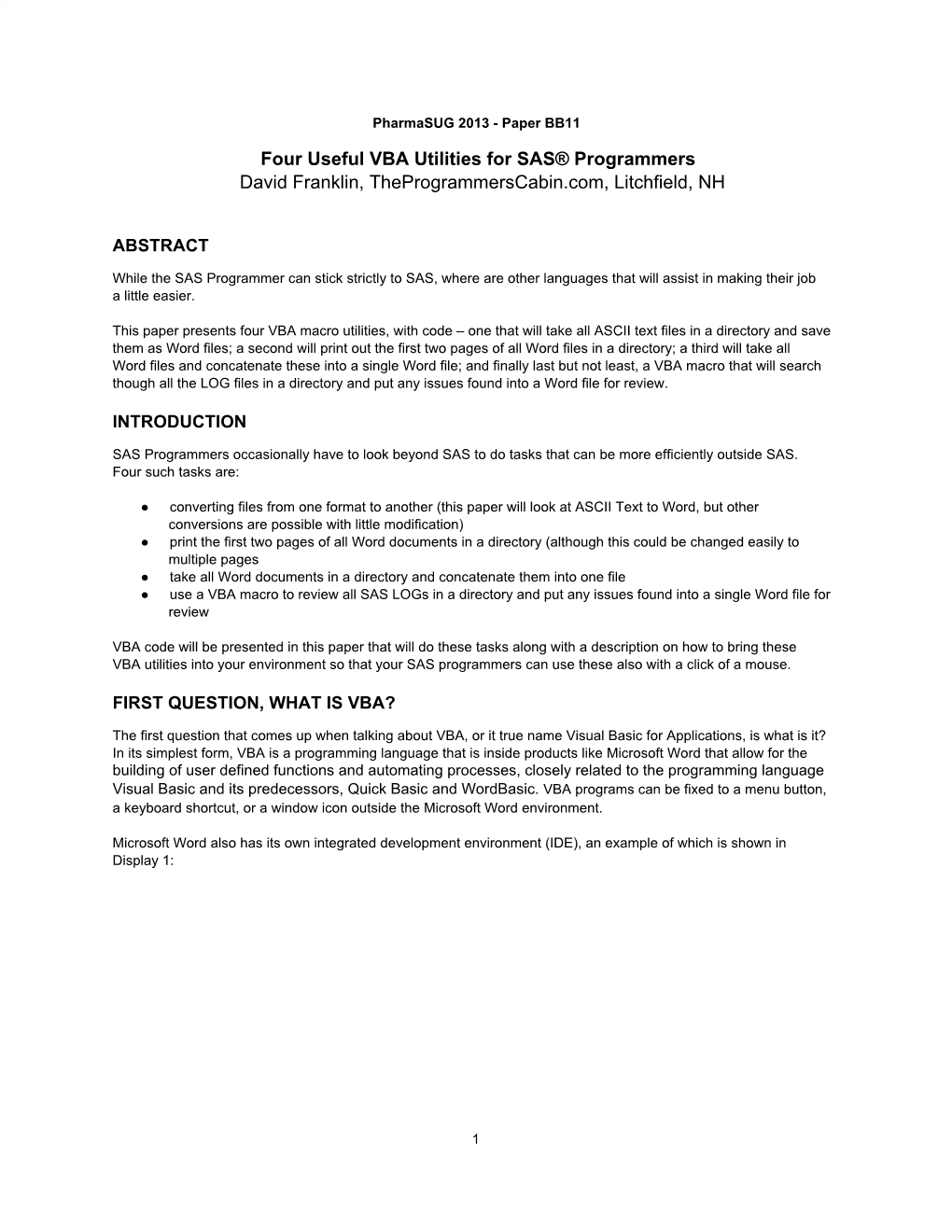
Load more
Recommended publications
-
Liste Von Programmiersprachen
www.sf-ag.com Liste von Programmiersprachen A (1) A (21) AMOS BASIC (2) A# (22) AMPL (3) A+ (23) Angel Script (4) ABAP (24) ANSYS Parametric Design Language (5) Action (25) APL (6) Action Script (26) App Inventor (7) Action Oberon (27) Applied Type System (8) ACUCOBOL (28) Apple Script (9) Ada (29) Arden-Syntax (10) ADbasic (30) ARLA (11) Adenine (31) ASIC (12) Agilent VEE (32) Atlas Transformatikon Language (13) AIMMS (33) Autocoder (14) Aldor (34) Auto Hotkey (15) Alef (35) Autolt (16) Aleph (36) AutoLISP (17) ALGOL (ALGOL 60, ALGOL W, ALGOL 68) (37) Automatically Programmed Tools (APT) (18) Alice (38) Avenue (19) AML (39) awk (awk, gawk, mawk, nawk) (20) Amiga BASIC B (1) B (9) Bean Shell (2) B-0 (10) Befunge (3) BANCStar (11) Beta (Programmiersprache) (4) BASIC, siehe auch Liste der BASIC-Dialekte (12) BLISS (Programmiersprache) (5) Basic Calculator (13) Blitz Basic (6) Batch (14) Boo (7) Bash (15) Brainfuck, Branfuck2D (8) Basic Combined Programming Language (BCPL) Stichworte: Hochsprachenliste Letzte Änderung: 27.07.2016 / TS C:\Users\Goose\Downloads\Softwareentwicklung\Hochsprachenliste.doc Seite 1 von 7 www.sf-ag.com C (1) C (20) Cluster (2) C++ (21) Co-array Fortran (3) C-- (22) COBOL (4) C# (23) Cobra (5) C/AL (24) Coffee Script (6) Caml, siehe Objective CAML (25) COMAL (7) Ceylon (26) Cω (8) C for graphics (27) COMIT (9) Chef (28) Common Lisp (10) CHILL (29) Component Pascal (11) Chuck (Programmiersprache) (30) Comskee (12) CL (31) CONZEPT 16 (13) Clarion (32) CPL (14) Clean (33) CURL (15) Clipper (34) Curry (16) CLIPS (35) -
Microsoft Word 1 Microsoft Word
Microsoft Word 1 Microsoft Word Microsoft Office Word 2007 in Windows Vista Developer(s) Microsoft Stable release 12.0.6425.1000 (2007 SP2) / April 28, 2009 Operating system Microsoft Windows Type Word processor License Proprietary EULA [1] Website Microsoft Word Windows Microsoft Word 2008 in Mac OS X 10.5. Developer(s) Microsoft Stable release 12.2.1 Build 090605 (2008) / August 6, 2009 Operating system Mac OS X Type Word processor License Proprietary EULA [2] Website Microsoft Word Mac Microsoft Word is Microsoft's word processing software. It was first released in 1983 under the name Multi-Tool Word for Xenix systems.[3] [4] [5] Versions were later written for several other platforms including IBM PCs running DOS (1983), the Apple Macintosh (1984), SCO UNIX, OS/2 and Microsoft Windows (1989). It is a component of the Microsoft Office system; however, it is also sold as a standalone product and included in Microsoft Microsoft Word 2 Works Suite. Beginning with the 2003 version, the branding was revised to emphasize Word's identity as a component within the Office suite; Microsoft began calling it Microsoft Office Word instead of merely Microsoft Word. The latest releases are Word 2007 for Windows and Word 2008 for Mac OS X, while Word 2007 can also be run emulated on Linux[6] . There are commercially available add-ins that expand the functionality of Microsoft Word. History Word 1981 to 1989 Concepts and ideas of Word were brought from Bravo, the original GUI writing word processor developed at Xerox PARC.[7] [8] On February 1, 1983, development on what was originally named Multi-Tool Word began. -
C:\Andrzej\PDF\ABC Nagrywania P³yt CD\1 Strona.Cdr
IDZ DO PRZYK£ADOWY ROZDZIA£ SPIS TREFCI Wielka encyklopedia komputerów KATALOG KSI¥¯EK Autor: Alan Freedman KATALOG ONLINE T³umaczenie: Micha³ Dadan, Pawe³ Gonera, Pawe³ Koronkiewicz, Rados³aw Meryk, Piotr Pilch ZAMÓW DRUKOWANY KATALOG ISBN: 83-7361-136-3 Tytu³ orygina³u: ComputerDesktop Encyclopedia Format: B5, stron: 1118 TWÓJ KOSZYK DODAJ DO KOSZYKA Wspó³czesna informatyka to nie tylko komputery i oprogramowanie. To setki technologii, narzêdzi i urz¹dzeñ umo¿liwiaj¹cych wykorzystywanie komputerów CENNIK I INFORMACJE w ró¿nych dziedzinach ¿ycia, jak: poligrafia, projektowanie, tworzenie aplikacji, sieci komputerowe, gry, kinowe efekty specjalne i wiele innych. Rozwój technologii ZAMÓW INFORMACJE komputerowych, trwaj¹cy stosunkowo krótko, wniós³ do naszego ¿ycia wiele nowych O NOWOFCIACH mo¿liwoYci. „Wielka encyklopedia komputerów” to kompletne kompendium wiedzy na temat ZAMÓW CENNIK wspó³czesnej informatyki. Jest lektur¹ obowi¹zkow¹ dla ka¿dego, kto chce rozumieæ dynamiczny rozwój elektroniki i technologii informatycznych. Opisuje wszystkie zagadnienia zwi¹zane ze wspó³czesn¹ informatyk¹; przedstawia zarówno jej historiê, CZYTELNIA jak i trendy rozwoju. Zawiera informacje o firmach, których produkty zrewolucjonizowa³y FRAGMENTY KSI¥¯EK ONLINE wspó³czesny Ywiat, oraz opisy technologii, sprzêtu i oprogramowania. Ka¿dy, niezale¿nie od stopnia zaawansowania swojej wiedzy, znajdzie w niej wyczerpuj¹ce wyjaYnienia interesuj¹cych go terminów z ró¿nych bran¿ dzisiejszej informatyki. • Komunikacja pomiêdzy systemami informatycznymi i sieci komputerowe • Grafika komputerowa i technologie multimedialne • Internet, WWW, poczta elektroniczna, grupy dyskusyjne • Komputery osobiste — PC i Macintosh • Komputery typu mainframe i stacje robocze • Tworzenie oprogramowania i systemów komputerowych • Poligrafia i reklama • Komputerowe wspomaganie projektowania • Wirusy komputerowe Wydawnictwo Helion JeYli szukasz ]ród³a informacji o technologiach informatycznych, chcesz poznaæ ul. -
User Guide Disclaimer
HostAccess User Guide Disclaimer Every effort has been made to ensure that the information contained within this publication is accurate and up-to-date. However, Rogue Wave Software, Inc. does not accept liability for any errors or omissions. Rogue Wave Software, Inc. continuously develops its products and services, and therefore reserves the right to alter the information within this publication without notice. Any changes will be included in subsequent editions of this publication. As the computing industry lacks consistent standards, Rogue Wave Software, Inc. cannot guarantee that its products will be compatible with any combination of systems you choose to use them with. While we may be able to help, you must determine for yourself the compatibility in any particular instance of Rogue Wave Software, Inc. products and your hardware/software environment. Rogue Wave Software, Inc. acknowledges that certain proprietary programs, products or services may be mentioned within this publication. These programs, products or services are distributed under Trademarks or Registered Trademarks of their vendors and/or distributors in the relevant country. Your right to copy this publication, in either hard-copy (paper) or soft-copy (electronic) format, is limited by copyright law. You must obtain prior authorisation from Rogue Wave Software, Inc. before copying, adapting or making compilations of this publication. HostAccess is a trademark of Quovadx Ltd in the United Kingdom and is a registered trademark in the USA. Microsoft is a registered trademark and Windows is a trademark of the Microsoft Corporation. Other brands and their products are trademarks or registered trademarks of their respected holders and should be noted as such. -
Virus Bulletin, November 1996
ISSN 0956-9979 NOVEMBER 1996 THE INTERNATIONAL PUBLICATION ON COMPUTER VIRUS PREVENTION, RECOGNITION AND REMOVAL Editor: Ian Whalley CONTENTS Assistant Editor: Megan Skinner EDITORIAL Technical Editor: Jakub Kaminski When the going gets tough, the tough play dirty 2 Consulting Editors: VIRUS PREVALENCE TABLE 3 Richard Ford, Command Software, USA Edward Wilding, Network Security, UK NEWS 1. Sophos Wins 3i Competition 3 2. MicroWazzuSoft…3 3. Takeover for Cheyenne 3 IN THIS ISSUE: IBM PC VIRUSES (UPDATE) 4 • With a macro here, a macro there. The Word macro CONFERENCE REPORT virus phenomenon began with Concept, and is now moving VB ’96: Brighton Rock 6 apace. This edition of VB contains two macro virus analy- ses: NPad, which is spreading rapidly in the wild, and VIRUS ANALYSES Outlaw, which features polymorphism. See p.8 and p.12. 1. NPad: Escape from Indonesia 8 2. Batch Sketches 9 • Let the Word go forth. Word and Excel’s internal file 3. Unsnared and (not so) Dangerous 10 formats have been, until recently, something in which few 4. Outlaw: The Changing Face of Macro Viruses 12 were interested – macro viruses, however, have changed all this. Andrew Krukow discusses the risks; see p.14. FEATURE • A new Trend? Trend Micro Devices PC-cillin has been ‘In the Beginning was the Word…’ 14 completely revamped in recent years: our reviewer takes a PRODUCT REVIEWS look at the new DOS and Windows version on p.18. 1. PC-cillin 95 18 2. ViruSafe LAN 21 END NOTES & NEWS 24 VIRUS BULLETIN ©1996 Virus Bulletin Ltd, The Pentagon, Abingdon, Oxfordshire, OX14 3YP, England. -
SUGI 25: Using Sasr Software and Visual Basic for Applications To
Applications Development Paper 21-25 Using SAS® Software and Visual Basic for Applications to Automate Tasks in Microsoft Word: An Alternative to Dynamic Data Exchange Mark Stetz, Amgen, Inc., Thousand Oaks, CA ABSTRACT DDE AND THE HARRIS DESIGN Using Dynamic Data Exchange (DDE) to drive Microsoft While DDE implementation is simple, the mixture of SAS Office applications such as Word and Excel has long and WordBasic syntax can result in confusing, difficult to been a technique utilized by SAS programmers. While maintain code. The following shows a simple DATA step alternatives exist (e.g., generating RTF code, OLE for populating a Word document using DDE and Automation), the use of DDE in conjunction with the native WordBasic (assuming Word is currently running): scripting languages of these applications has been appealing since implementation is simple and the macro filename cmds dde 'winword|system' ; recording capabilities of Office applications make script generation nearly automatic. Harris (SUGI 24 data _null_ ; Proceedings, 1999) described a particularly elegant file cmds ; design for using the SAS System and DDE to populate Microsoft Word documents. put '[Insert "Hello World"]' ; put '[FileSaveAs ' Now that the more sophisticated Visual Basic for '.Name = "c:\My Documents\Hello", ' Applications (VBA) is the common development '.Format = 0, ' environment among all Office applications, however, DDE '.AddToMru=0]' ; only works with Office application legacy macro put '[FileClose]' ; languages. Employing Harris’ design, this paper run ; describes a technique to simulate the ease of use of the DDE methodology while taking full advantage of VBA to Harris suggested a design to simplify the coding of such automate Microsoft Word. -
An Introduction to S and the Hmisc and Design Libraries
An Introduction to S and The Hmisc and Design Libraries Carlos Alzola, MS Frank Harrell, PhD Statistical Consultant Professor of Biostatistics 401 Glyndon Street SE Department of Biostatistics Vienna, Va 22180 Vanderbilt University School of Medicine [email protected] S-2323 Medical Center North Nashville, Tn 37232 [email protected] http://biostat.mc.vanderbilt.edu/RS September 24, 2006 ii Updates to this document may be obtained from biostat.mc.vanderbilt.edu/RS/sintro.pdf. Contents 1 Introduction 1 1.1 S, S-Plus, R, and Source References ........................... 1 1.1.1 R ........................................... 4 1.2 Starting S .......................................... 4 1.2.1 UNIX/Linux .................................... 4 1.2.2 Windows ...................................... 5 1.3 Commands vs. GUIs .................................... 7 1.4 Basic S Commands ..................................... 7 1.5 Methods for Entering and Saving S Commands ..................... 9 1.5.1 Specifying System File Names in S ........................ 11 1.6 Differences Between S and SAS .............................. 11 1.7 A Comparison of UNIX/Linux and Windows for Running S .............. 18 1.8 System Requirements ................................... 19 1.9 Some Useful System Tools ................................. 19 2 Objects, Getting Help, Functions, Attributes, and Libraries 25 2.1 Objects ........................................... 25 2.2 Getting Help ........................................ 25 2.3 Functions ......................................... -
Hostaccess Developer's Guide
HostAccess Developer’s Guide Disclaimer Every effort has been made to ensure that the information contained within this publication is accurate and up-to-date. However, Rogue Wave Software, Inc. does not accept liability for any errors or omissions. Rogue Wave Software, Inc. continuously develops its products and services. We therefore reserve the right to alter the information within this publication without notice. Any changes will be included in subsequent editions of this publication. As the computing industry lacks consistent standards, Rogue Wave Software, Inc. cannot guarantee that its products will be compatible with any combination of systems you choose to use them with. While we may be able to help, you must determine for yourself the compatibility in any particular instance of Rogue Wave Software, Inc. products and your hardware/software environment. Rogue Wave Software, Inc. acknowledges that certain proprietary programs, products or services may be mentioned within this publication. These programs, products or services are distributed under Trademarks or Registered Trademarks of their vendors and/or distributors in the relevant country. Your right to copy this publication, in either hard-copy (paper) or soft-copy (electronic) format, is limited by copyright law. You must obtain prior authorization from Rogue Wave Software, Inc. before copying, adapting or making compilations of this publication. HostAccess is a trademark of Quovadx Ltd in the United Kingdom and is a registered trademark in the USA. Microsoft is a registered trademark and Windows is a trademark of the Microsoft Corporation. Other brands and their products are trademarks or registered trademarks of their respected holders and should be noted as such. -
034-29: Let SAS Tell Microsoft Word to Collate
SUGI 29 Applications Development Paper 034-29 Let SAS® Tell Microsoft Word® to Collate Haiping Luo, Department of Veterans Affairs, Washington, DC ABSTRACT This paper describes the process of developing an application to collate and print Microsoft(MS) Word documents with SAS output tables and charts by region. The user's need was to customize Word documents using SAS generated values, collate the Word document with SAS created tables and charts for individual regions selected by the SAS code, then print and mail the set of Word and SAS files to individual regions. After comparing different possible solutions, the author chose to use SAS sending WordBasic scripts and Word macro names through Dynamic Data Exchange (DDE) links to automate the process. In the resulting application, SAS code was written to prepare data, to send WordBasic scripts, to invoke VBA subroutines, and to loop through regions. Word was driven by SAS to customize Word documents using SAS provided values and to print both Word and SAS output files. This paper presents the design of the application, explains the code of an example, and discusses the problems encountered during the development process. The code of the example application is included in the appendices. The example application was tested in a Windows 2000 platform. PC SAS 8.2 with Base SAS and SAS/Graph installed. The version of MS Office was 2000. INTRODUCTION My office regularly needs to mail letters associated by SAS output tables and charts to about 160 hospitals. Those hospitals are usually selected by SAS programs. The manual process was to customize the letter in MS Word with hospital address, attach the tables and graphics created by SAS for a specific hospital, then put the letter and SAS output into the envelope to send to that specific hospital. -
Wordbasic for Writers
A Book Proposal for WordBasic for Writers SAMPLE Steven Radecki XXX Shadow Dance Drive San Jose, California 95110 (408) 123-4567 SAMPLE The contents of this proposal are copyright © 1994 by Steven Radecki. All rights reserved. All product names mentioned in this proposal are the property of their respective copyright and trademark owners. Table of Contents About This Proposal ......................................................................................................... 1 About This Book ................................................................................................................ 2 About the Author ............................................................................................................... 4 Chapter Outline ................................................................................................................. 6 Understanding the Basics of WordBasic .................................................................................................. 6 Using the Macro Recorder ........................................................................................................................ 6 Automating Repetitive Tasks ................................................................................................................... 6 Using the Macro Dialog Editor ................................................................................................................ 6 Customizing Your Word for Windows Environment .............................................................................. -
How to Use Word.Pdf
The Word MVP Site Search Tips Tutorials Word HomeWord:mac Word General TroubleshootTutorialsAbout UsContact ● Light Relief Word Tutorials ❍ Humor! Literature Recommended books on how to use Word ❍ Book reviews and recommendations Links to other sites ❍ Links to other sites Light relief ● Word Tutorials ❍ Bend Word to Your Will (written for Word for the How to Use Word Macintosh, this article has Bend Word to Your Will (written for Word for the Macintosh, this broad applicability to all versions of Word). article has broad applicability to all versions of Word). ❍ How to save yourself hours How to save yourself hours by using Outline View properly by using Outline View properly What do all those funny marks, like the dots between the words in ❍ What do all those funny my document, and the square bullets in the left margin, mean? marks, like the dots between the words in my document, Typographical Tips from Microsoft Publisher and the square bullets in the left margin, mean? Some of the most useful Word keyboard shortcuts ❍ Typographical Tips from Word commands, and their descriptions, default shortcuts and Microsoft Publisher menu assignments ❍ Some of the most useful Word keyboard shortcuts How can I insert special characters, such as dingbats and accented letters, in my document? ❍ Word commands, and their descriptions, default Setting tabs shortcuts and menu assignments Ruler of all you survey: How to make the best use of Word's rulers ❍ How can I insert special characters, such as dingbats The strait and narrow – using columns http://word.mvps.org/Tutorials/index.htm -
Petik Archiver 1.0
PetiK Archiver 1.0 17/05/2009 After 7 years to stop coding virus/worms, I decided to assemble all my works. It is sorted by date like this : YYYYMMDD (where Y is the year, M the month and D the day) and the name of the works. In the begining you can see my old website page. Then my works. Newt, my not finish works and some articles. Best reading. PetiK Homepage (last update : July 9th 2002) EMAIL : [email protected] NEW : FORUM FOR ALL VXERS : CLICK HERE PLEASE SIGN MY GUESTBOOK : CLICK HERE 2002: July 9th : GOOD BYE TO ALL VXERS. I LEAVE THE VX-SCENE. I HOPE MY WORKS LIKE YOU AND WILL HELP YOU IN YOUR VX-LIFE. IF YOU WANT TO CONTACT ME, PLEASE WRITE IN THE GUESTBOOK. Special Thanx to : alc0paul, Benny/29A, Bumblebee, Vecna, Mandragore, ZeMacroKiller98and the greatest coder group : 29A July 7th : Add some new descriptions of AV (from Trend Micro and McAfee) July 3rd : Add the binary of my last Worm coded with alc0paul : VB.Brigada.Worm July 2nd : Add a new link : Second Part To Hell June 29th : Add my new tool : PetiK’s VBS Hex Convert and add my last full spread VBS worm : VBS.Hatred June 26th : Add W32/HTML.Dilan June 24th : Add VBS.Park June 22nd : I finish my new worm : VB.DocTor.Worm June 20th : PETIKVX EZINE #2 REALIZED : DOWNLOAD IT and add a new tool : CryptoText and my last worm : VB.Mars.Worm June 19th : Add VBS.Cachemire. Add my new article VBS/HTML Multi-Infection.