Research Report On
Total Page:16
File Type:pdf, Size:1020Kb
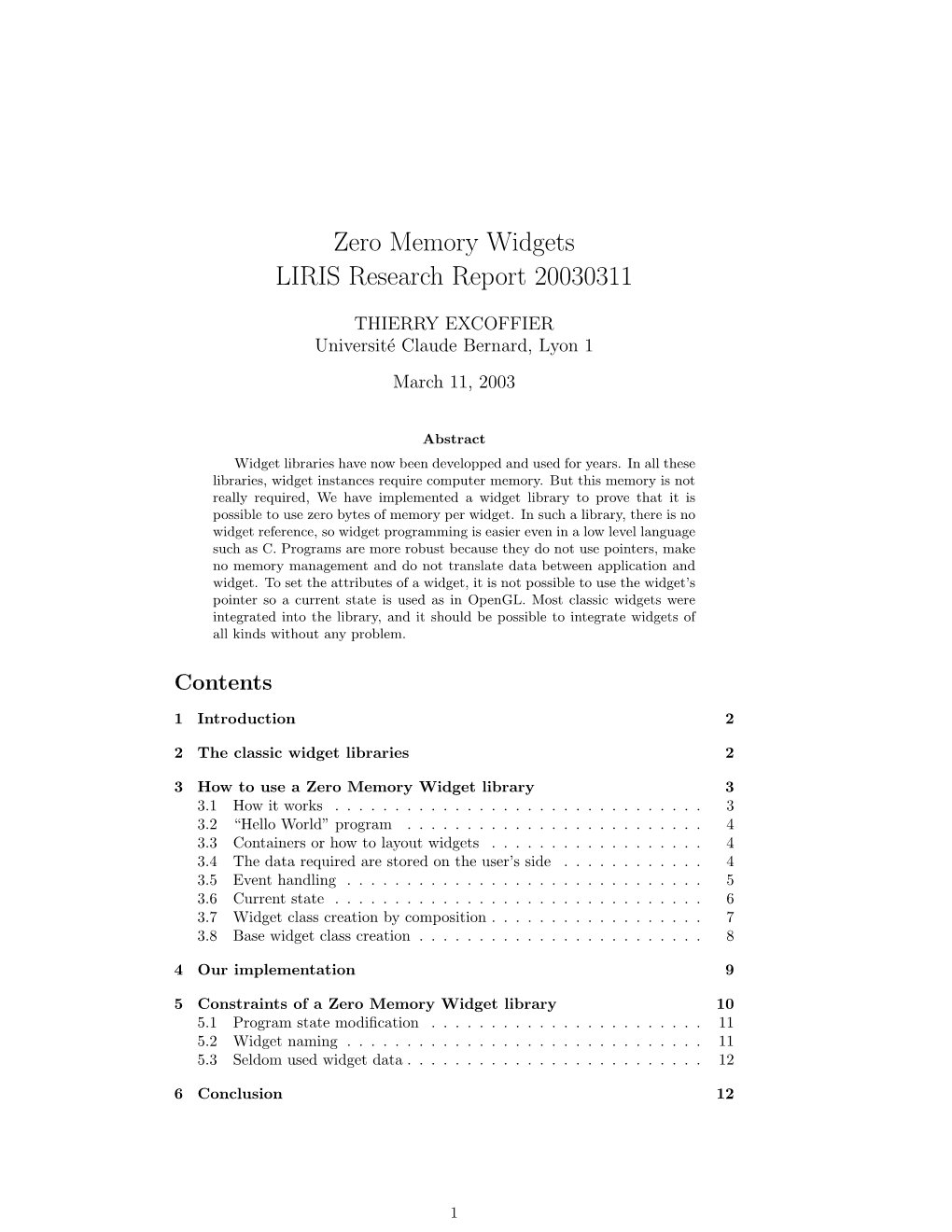
Load more
Recommended publications
-
Original File Was Cvddn.Tex
Paul Bowman Durao email: [email protected] Phone: (301) 412-6312 or SKYPE: paul.durao Maryland / Washington DC / Remote Senior Software Architect / Engineer / Software Developer 30+ years of experience full life-cycle software development Exceptionally well-qualified senior software architect engineer with extensive programming skills. Subject matter expert in applying methodologies, processes, and procedures in the execution of full life-cycle approach. There is no substitute for a proper engineering degree, first-rate hands on experience, and sophisticated problem solving methodology, but it’s not for everyone, and that’s where I shine and come through for the team. Flexible Working Classifications 1. 1099 Contract Worker 2. Corp to Corp Contract Worker 3. Statutory Employee 4. At Will Employee 5. Contract ± Sub Contract W-2 Employee 6. Regular W-2 Employee 7. Remote and work at home preferred 8. Willing to travel part, or full time. Technical Qualifications Languages: C, C++, Java, JavaScript, ( Angular Js, DOJO, JQuery), Html, CSS, Ruby, XML, Perl, Python, lisp, Assembly (ARM/Intel), SQL; Operating Systems: Linux, Unix, BSD, OSX. iOS, Motif, Embedded; Database: PL/SQL, Oracle; Library, Middleware and API’s: Java EE, ROR; Additional skills: Data Modeling; MVC; UML, TDD, O/R mapping; Miscellaneous: 508 Compliance (web accessibility). Career Highlights Work Chronology – Independent consulting, and some full time, sometimes there is a mix. I have done consulting on a full/long time basis too, for NASA and IBM for example, as my skill level has improved relative to the market. I have tended to do more independent contracting work as my skill level, comprehension, and abilities are just different, and more specialized, and therefore higher in fidelity, and in quality (Linux, UNIX, OSX, iOS 8 / xcode 6, development centric, all things UNIX related). -
Review: Classic Mac OS the X Window System ("X") X
Review: Classic Mac OS The X Window System ("X") • Designed for the user, not the developer • Asente, Reid (Stanford): W window system for V OS, (1982) • First commercially successful GUI system • W moved BWS&GEL to remote machine, replaced local library calls with • Technically few advances synch. communication • One address space, one process, “no” OS • Simplified porting to new architectures, but slow under Unix • But revolutionary approach to UI consistency (HI Guidelines) • MIT: X as improvement over W (1984) • Macintosh Toolbox • Asynchronous calls: much-improved performance • Pascal procedures grouped into Managers, ROM+RAM • Application = client, calls X Library (Xlib) which packages and sends GEL • Extended as technology advanced (color, multiprocessing,...), but calls to the X Server and receiving events using the X Protocol. architecture was showing its age by late 90s • Similar to Andrew, but window manager separate • Inspiration for other GUIs, esp. MS Windows • X10 first public release, X11 cross-platform redesigned Jan Borchers 1 media computing group Jan Borchers 2 media computing group X: Architecture X Server • X11 ISO standard, but limited since static protocol Application • X server process combines GEL and BWS • X is close to Widget Set • Responsible for one keyboard (one EL), but n physical screens UITK our 4-layer WM Xt Intrinsics (GLs) architecture • One machine can run several servers Xlib model Xlib • Applications (with UITK) and WM are clients Network • GEL: Direct drawing, raster model, rectangular clipp. X Server BWS+GEL • X-Server layers: Device-dependent X (DDX), device-independent X (DIX) HW • BWS can optionally buffer output regions Jan Borchers 3 media computing group Jan Borchers 4 media computing group Typical Xlib application (pseudocode) X Protocol #include Xlib.h, Xutil.h Display *d; int screen; GC gc; Window w; XEvent e; main () { • Between X server process and X clients (incl. -
A Bibliography of O'reilly & Associates and O
A Bibliography of O'Reilly & Associates and O'Reilly Media. Inc. Publishers Nelson H. F. Beebe University of Utah Department of Mathematics, 110 LCB 155 S 1400 E RM 233 Salt Lake City, UT 84112-0090 USA Tel: +1 801 581 5254 FAX: +1 801 581 4148 E-mail: [email protected], [email protected], [email protected] (Internet) WWW URL: http://www.math.utah.edu/~beebe/ 08 February 2021 Version 3.67 Title word cross-reference #70 [1263, 1264]. #70-059 [1263]. #70-068 [1264]. 2 [949]. 2 + 2 = 5986 [1456]. 3 [1149, 1570]. *# [1221]. .Mac [1940]. .NET [1860, 22, 186, 342, 441, 503, 591, 714, 716, 721, 730, 753, 786, 998, 1034, 1037, 1038, 1043, 1049, 1089, 1090, 1091, 1119, 1256, 1468, 1858, 1859, 1863, 1899, 1900, 1901, 1917, 1997, 2029]. '05 [461, 1532]. 08 [1541]. 1 [1414]. 1.0 [1009]. 1.1 [59]. 1.2 [1582]. 1000 [1511]. 1000D [1073]. 10g [711, 710]. 10th [2109]. 11 [1385]. 1 2 2 [53, 209, 269, 581, 2134, 919, 940, 1515, 1521, 1530, 2023, 2045]. 2.0 [2, 55, 203, 394, 666, 941, 1000, 1044, 1239, 1276, 1504, 1744, 1801, 2073]. 2.1 [501]. 2.2 [201]. 2000 [38, 202, 604, 610, 669, 927, 986, 1087, 1266, 1358, 1359, 1656, 1751, 1781, 1874, 1959, 2069]. 2001 [96]. 2003 [70, 71, 72, 73, 74, 279, 353, 364, 365, 789, 790, 856, 987, 1146, 1960, 2026]. 2003-2013 [1746]. 2004 [1195]. 2005 [84, 151, 755, 756, 1001, 1041, 1042, 1119, 1122, 1467, 2120, 2018, 2056]. 2006 [152, 153]. 2007 [618, 726, 727, 728, 1123, 1125, 1126, 1127, 2122, 1973, 1974, 2030]. -
X Toolkit Intrinsics – C Language Interface
X Toolkit Intrinsics – C Language Interface X Window System Joel McCormack, Digital Equipment Corporation Paul Asente, Digital Equipment Corporation Ralph R. Swick, Digital Equipment Corporation X Toolkit Intrinsics – C Language Interface: X Window System by Joel McCormack, Paul Asente, and Ralph R. Swick X Version 11, Release 7.7 X Toolkit Intrinsics Version 1.2.0 XWindow System is a trademark of X Consortium, Inc. Copyright © 1985, 1986, 1987, 1988, 1991, 1994 X Consortium Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the “Software”), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE X CONSORTIUM BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. Except as contained in this notice, the name of the X Consortium shall not be used in advertising or otherwise to promote the sale, use or other dealings in this Software without prior written authorization from the X Consortium. -
A Distinct Approach for X/Motif Application Gui Test Automation
IJRET: International Journal of Research in Engineering and Technology eISSN: 2319-1163 | pISSN: 2321-7308 A DISTINCT APPROACH FOR X/MOTIF APPLICATION GUI TEST AUTOMATION K.V. Maruthi Prasad1 1ISRO Satellite centre, HAL Airport road, Bangalore-17, India Abstract This paper titled “A distinct approach for X/Motif application GUI test automation” presents the research results of the innovative approach applied on X/Motif applications under test automation. It is the excerpts of the results obtained on X/Motif GUI software test automation without record & playback technique. This approach is based on virtualisation of mouse button and key board key events using “XSendEvent” Xlib routine. It also presents the details about the software that has been developed for X/Motif GUI application testing automated through a tester input file of identified keywords with the necessary input as test cases. The paper identifies the limitations and future plans for the expansion of the work. Keywords: X/Motif, test automation, XSendEvent, record & playback, GUI. ----------------------------------------------------------------------***---------------------------------------------------------------------- 1. INTRODUCTION Test automation can enable some testing tasks to be performed more efficiently than by testing manually. Automation of ISRO (Indian Space Research Organisation) is the premier testing makes the effort involved in performing regression government institute involved in space research and tests at minimal. GUI based applications test automation development activities. ISRO has been known for it’s allows the tester to run more tests in less time and also to accomplishments in nation building through science & execute them more often. Automation of GUI based technological innovations in space field. GEOSCHEMACS application testing enables us to execute test cases of input (Geostationary Earth Orbit SpaCecraft HEalth Monitoring entry with greater accuracy, run difficult or impossible test Analysis and Control Software) is the in-house developed end cases to do manually. -
Athena Widget Set - C Language Interface X Consortium Standard
Athena Widget Set - C Language Interface X Consortium Standard Chris Peterson Athena Widget Set - C Language Interface: X Consortium Standard by Chris Peterson X Version 11, Release 6.4 libXaw 1.0.7 Copyright © 1985 X Consortium Copyright © 1986 X Consortium Copyright © 1987 X Consortium Copyright © 1988 X Consortium Copyright © 1989 X Consortium Copyright © 1991 X Consortium Copyright © 1994 X Consortium Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated docu- mentation files (the “Software”), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE X CONSORTIUM BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. Except as contained in this notice, the name of the X Consortium shall not be used in advertising or otherwise to promote the sale, use or other dealings in this Software without prior written authorization from the X Consortium. -
An Introduction to X Window Application Development
Calhoun: The NPS Institutional Archive Theses and Dissertations Thesis Collection 1992-03 An introduction to X Window application development. Rust, David Michael Monterey, California. Naval Postgraduate School http://hdl.handle.net/10945/23933 FV KNOX LIBRARY •Ud SCHOOL SivAL POSTGRADUATE SoNTEHEV.CAUFORN.AG^^ CURITY CLASSIFICATION OF THIS PAGE REPORT DOCUMENTATION PAGE 1b. RESTRICTIVE MARKINGS REPORT SECURITY CLASSIFICATION UNCLASSIFIED a SECURITY CLASSIPICAT I6M AUTH6RITV 3. bisTRiBuTi6N7AVAlLABiUTY 6P REP5RT Approved for public release; b. dECLAssIFICATIoN/doWNgRADINg SCHEDULE distribution is unlimited PERFORMING ORGANIZATION REP6RT NUM&ER(S) 5. M6niT6Ring6Rgani2aTi6n rep6rT numbER(S) *. NAME 6F PERFORMING 6R6ANIZATI6N 6b OFFICE SYMBOL 7a. NAME 6E M6NIT6R1NG ORGANIZATION vdministrative Sciences Department (if applicable) Naval Postgraduate School Javal Postgraduate School AS :. ADDRESS (City, State, and ZIP Code) 7b. ADDRESS (City, State, and ZIP Code) /lonterey, CA 93943-5000 Monterey, CA 93943-5000 NAME OF FUNDING/SPONSORING 8b. OFFICE SYMBOL g PROCURE M E N T I NST RUMEN T ID E N T I FI CATION NUMBE R ORGANIZATION (if applicable) 6. ADDRESS (City, State, and ZIP Code) 10. SOURCE OF FUNDING NUMBERS PROGRAM 1 PR6JECT TTa^R" WoRkUNiT ELEMENT NO. NO. NO. ACCESSION NO. 1 . TITLE (Include Security Classification) ^N INTRODUCTION TO X WINDOW APPLICATION DEVELOPMENT (U) 2 PERSONAL AUTHOR(S) lust, David M. 3a. type of report 13b. time covered 14. DATE OF REPORT (Year, Month, Day) 16. PAGE COUNT Master's Thesis from 10/90 to 03/92 1992, March, 23 70 supplementary notation 6 The views expressed in this ^sis ^ those of the author md do not reflect the official policy or position of the Department of Defense or the United States Government. -
Portuguese-HOWTO
Portuguese−HOWTO Portuguese−HOWTO Table of Contents Linux Portuguese−HOWTO..............................................................................................................................1 Configurações do Linux para a Língua Portuguesa.................................................................................1 Carlos A. M. dos Santos < [email protected]>....................................................................1 CPMet/UFPEL −− Pelotas, RS, Brasil.......................................................................................1 1.Introdução.............................................................................................................................................1 2.Informações gerais................................................................................................................................1 3.Configuração do console (modo texto).................................................................................................1 4.Biblioteca de funções libc e aplicativos GNU......................................................................................2 5.Configuração do X................................................................................................................................2 6.Configuração dos vários programas .....................................................................................................2 7.Ficheiros necessários............................................................................................................................2 -
Motif Reference Manual for Openmotif 2.3
THE DEFINITIVE GUIDES TO THE X WINDOW SYSTEM VOLUME SIX B Motif Reference Manual for OpenMotif 2.3 Open Source Edition Based upon the manual writted by Antony Fountain and Paula Ferguson, O’Reilly and Associates Updated and Published by Peter Winston and the Technical Staff of Integrated Computer Solutions, Inc. Motif Reference Manual, Open Source Edition for OpenMotif version 2.3 Copyright ©2005 by Integrated Computer Solutions, Inc. This material may be distributed only subject to the terms and conditions set forth in the Open Publication License, v1.0 or later (the latest version is presently available at http://www.opencontent.org/openpub/). December 2001 Copyright ©1993, 2000, 2001 by O’Reilly & Associates, Inc. and Antony Fountain. This material may be distributed only subject to the terms and conditions set forth in the Open Publication License, v1.0 or later (the latest version is presently available at http://www.opencontent.org/openpub/). This is an updated version of the Motif Reference Manual, Open Source Edition for Motif 2.1, published by Imperial Software Technology in December 2001. The source files for this version of the document are available at http://www.motifzone.org. The source files for the Open Source Edition can be found at http://www.ist.co.uk/motif/motif_refs.html. A description of the modifications is contained in the Preface to the Open Source Edition. The Open Source Edition is a modified version of the Motif Reference Manual, Second Edition, published by O’Reilly & Associates in February 2000. The source files for the Second Edition can be found at http://www.oreilly.com/openbook/motif/. -
Motif Programming Manual 1 Preface
Motif Programming Manual 1 Preface...........................................................................................................................................................................1 1.1 The Plot..........................................................................................................................................................1 1.2 Assumptions...................................................................................................................................................2 1.3 How This Book Is Organized........................................................................................................................3 1.4 Related Documents........................................................................................................................................5 1.5 Conventions Used in This Book....................................................................................................................6 1.6 Obtaining Motif.............................................................................................................................................6 1.7 Obtaining the Example Programs..................................................................................................................7 1.7.1 FTP.................................................................................................................................................7 1.7.2 FTPMAIL......................................................................................................................................7 -
Debian Policy Manual Release 4.6.0.1
Debian Policy Manual Release 4.6.0.1 The Debian Policy Mailing List Aug 18, 2021 CONTENTS 1 About this manual 3 1.1 Scope ................................................... 3 1.2 New versions of this document ...................................... 4 1.3 Authors and Maintainers ......................................... 4 1.3.1 Early history ........................................... 4 1.3.2 Current process ......................................... 4 1.3.3 Improvements .......................................... 5 1.4 Related documents ............................................ 5 1.5 Definitions ................................................ 5 1.6 Translations ................................................ 6 2 The Debian Archive 7 2.1 The Debian Free Software Guidelines .................................. 7 2.2 Archive areas ............................................... 8 2.2.1 The main archive area ...................................... 8 2.2.2 The contrib archive area ..................................... 8 2.2.3 The non-free archive area .................................... 9 2.3 Copyright considerations ......................................... 9 2.4 Sections .................................................. 10 2.5 Priorities ................................................. 10 3 Binary packages 13 3.1 The package name ............................................ 13 3.1.1 Packages with potentially offensive content ........................... 13 3.2 The version of a package ......................................... 14 3.2.1 -
Athena Widget Set — C Language Interface Xwindowsystem Xversion 11, Release 6.7
Athena Widget Set — C Language Interface XWindowSystem XVersion 11, Release 6.7 Chris D. Peterson formerly MIT X Consortium XWindowSystem is a trademark of The Open Group. Copyright © 1985, 1986, 1987, 1988, 1989, 1991, 1994 X Consortium Permission is hereby granted, free of charge, to anyperson obtaining a copyofthis software and associated documenta- tion files (the ‘‘Software’’), to deal in the Software without restriction, including without limitation the rights to use, copy, modify,merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Soft- ware. THE SOFTWARE IS PROVIDED ‘‘ASIS’’, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOTLIMITED TOTHE WARRANTIES OF MERCHANTABILITY,FITNESS FOR A PARTIC- ULAR PURPOSE AND NONINFRINGEMENT.INNOEVENT SHALL THE X CONSORTIUM BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,WHETHER IN AN ACTION OF CONTRACT,TORTOROTH- ERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. Except as contained in this notice, the name of the X Consortium shall not be used in advertising or otherwise to pro- mote the sale, use or other dealings in this Software without prior written authorization from the X Consortium. Copyright © 1985, 1986, 1987, 1988, 1989, 1991 Digital Equipment Corporation, Maynard, Massachusetts. Permission to use, copy, modify and distribute this documentation for anypurpose and without fee is hereby granted, provided that the above copyright notice appears in all copies and that both that copyright notice and this permission notice appear in supporting documentation, and that the name of Digital not be used in in advertising or publicity per- taining to distribution of the software without specific, written prior permission.