Game Programming Primer.Docx
Total Page:16
File Type:pdf, Size:1020Kb
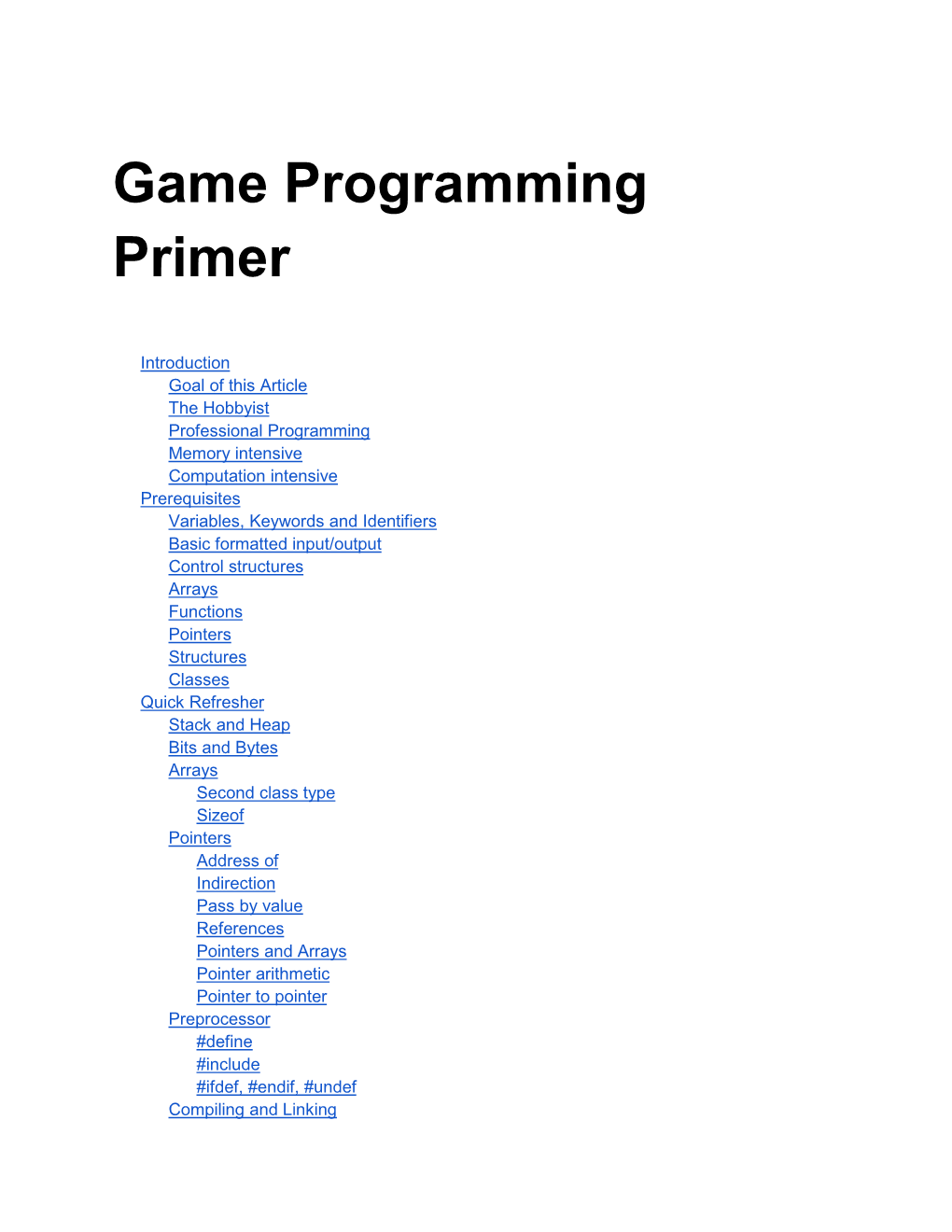
Load more
Recommended publications
-
Programming Documentation
Flying Samurai { Programming documentation Jan Beneˇs,Osk´arElek, Marek Hanes, J´anZahornadsk´y June 3, 2010 \We make war that we may live in peace." | Aristotle Contents 1 Introduction 1 2 About the project 2 2.1 Team..................................................2 2.2 Externists . .3 2.3 Review of specification . .5 2.4 Hardware requirements . .6 2.5 Comparison with similar software . .6 2.6 Timeline . .7 2.7 Future of the project . .8 2.8 Known bugs . .9 2.9 Some statistics . 10 3 Building the project 11 3.1 Setting up the environment . 11 3.2 Building from sources . 11 4 Programming documentation 12 4.1 Architecture . 13 4.2 Multi-threading model . 15 4.2.1 Threads and their purpose . 15 4.2.2 Synchronization . 15 4.2.3 Messages and heartbeat . 16 4.2.4 Reader and Writer . 16 4.2.5 Structures . 17 4.2.6 Swap and swap chain . 18 4.2.7 Messages in detail . 19 4.3 Menu ................................................. 21 4.3.1 Concepts . 21 4.3.2 Implementation . 21 4.3.3 Handlers and actions . 21 4.4 Game logic . 23 4.4.1 Logical entities . 23 4.4.2 Mission . 24 4.4.3 Career . 26 4.5 Graphics . 27 4.5.1 Scene graph . 28 4.5.2 Airplane meshes . 29 4.5.3 Terrain . 33 4.5.4 Static terrain geometry . 36 4.5.5 HUD and debugging graphics . 38 4.5.6 Special effects . 39 4.5.7 Camera . 40 4.5.8 GUID . 40 4.6 Physics . 41 4.6.1 Introduction . -
Multiplatformní GUI Toolkity GTK+ a Qt
Multiplatformní GUI toolkity GTK+ a Qt Jan Outrata KATEDRA INFORMATIKY UNIVERZITA PALACKÉHO V OLOMOUCI GUI toolkit (widget toolkit) (1) = programová knihovna (nebo kolekce knihoven) implementující prvky GUI = widgety (tlačítka, seznamy, menu, posuvník, bary, dialog, okno atd.) a umožňující tvorbu GUI (grafického uživatelského rozhraní) aplikace vlastní jednotný nebo nativní (pro platformu/systém) vzhled widgetů, možnost stylování nízkoúrovňové (Xt a Xlib v X Windows System a libwayland ve Waylandu na unixových systémech, GDI Windows API, Quartz a Carbon v Apple Mac OS) a vysokoúrovňové (MFC, WTL, WPF a Windows Forms v MS Windows, Cocoa v Apple Mac OS X, Motif/Lesstif, Xaw a XForms na unixových systémech) multiplatformní = pro více platforem (MS Windows, GNU/Linux, Apple Mac OS X, mobilní) nebo platformově nezávislé (Java) – aplikace může být také (většinou) událostmi řízené programování (event-driven programming) – toolkit v hlavní smyčce zachytává události (uživatelské od myši nebo klávesnice, od časovače, systému, aplikace samotné atd.) a umožňuje implementaci vlastních obsluh (even handler, callback function), objektově orientované programování (objekty = widgety aj.) – nevyžaduje OO programovací jazyk! Jan Outrata (Univerzita Palackého v Olomouci) Multiplatformní GUI toolkity duben 2015 1 / 10 GUI toolkit (widget toolkit) (2) language binding = API (aplikační programové rozhraní) toolkitu v jiném prog. jazyce než původní API a toolkit samotný GUI designer/builder = WYSIWYG nástroj pro tvorbu GUI s využitím toolkitu, hierarchicky skládáním prvků, z uloženého XML pak generuje kód nebo GUI vytvoří za běhu aplikace nekomerční (GNU (L)GPL, MIT, open source) i komerční licence např. GTK+ (C), Qt (C++), wxWidgets (C++), FLTK (C++), CEGUI (C++), Swing/JFC (Java), SWT (Java), JavaFX (Java), Tcl/Tk (Tcl), XUL (XML) aj. -
5G Simulation Framework
Master of Science Thesis in Electrical Engineering Department of Electrical Engineering, Linköping University, 2018 5G Simulation Framework Junior Asante and Joel Olsson Master of Science Thesis in Electrical Engineering 5G Simulation Framework Junior Asante and Joel Olsson LiTH-ISY-EX--18/5149--SE Supervisor: Ema Becirovic isy, Linköping University Henrik André-Jönsson Ericsson AB Examiner: Danyo Danev isy, Linköping University Division of Communication Systems Department of Electrical Engineering Linköping University SE-581 83 Linköping, Sweden Copyright © 2018 Junior Asante and Joel Olsson Abstract From the first generation, 1G, to the fourth generation, 4G, the development and technological advancements in telecommunications network systems have been remarkable. Faster and better connections have opened up for new markets, ideas and possibilities, to that extent that there now is a demand that surpasses the sup- ply. Despite all these advancements made in the mobile communications field most of the concept of how the technology works and its infrastructure has re- mained the same. This however, is about to change with the introduction of the fifth generation (5G) mobile communication. With the introduction of 5G much of the technology introduced will be differ- ent from that of previous generations. This change extends to include the entire infrastructure of the mobile communications system. With these major changes, many of the tools available today for telecommunications network evaluation do not really suffice to include the 5G network standard. For this reason, there is a need to develop a new kind of tool that will be able to include the changes brought by this new network standard. In this thesis a simulation framework adapted for the next generation telecom- munication standard 5G is set to be developed. -
Curriculum Vitae
Mikkel Kirkgaard Nielsen Tjaereborg Stationsvej 1, 1. sal • 6731 Tjaereborg, Denmark • +45 28139066 • [email protected] Updated 2021-03-23 Profile Software developer and architect with a hardware background, possessing relaxed attitude, analytical and communicative skills. Always striving for technical perfection but has a well developed commercial sense. Broad experience within the field of embedded software on numerous hardware platforms, operating systems and programming languages. Core competency lies in the intersection between hardware and software. Also experienced with development, operation and administration of back-end web-based server applications, solutions and deployments. Pitches generic, configurable and modular architectures in the pursuit of attaining flexible and reusable system components. Dedicated and fierce solution hunter when faced with a problem that needs to be solved. Advocating standardisation and cooperation on a technical level wherever possible. Firm believer in open and collaborative development as practioned in the FOSS (Free & Open Source Software) communities. Family status Single, living with my oldest daughter Two daughters born 2001+2005 Born 1977-01-17 Education Aalborg Universitet Esbjerg (Esbjerg, Denmark) B.Sc. E.E., Bachelor of Science in Electronic and Electrical Engineering, Digital Signal Processing (DSP) 1997 – 2001 Aarhus Akademi (Aarhus, Denmark) High School (stx, studenterkursus) 1996 – 1997 Esbjerg Gymnasium (Esbjerg, Denmark) High School (stx, gymnasium) 1993 – 1996 Blåbjerggårdskolen (Esbjerg, Denmark) Primary school, 0th–5th + 9th grade 1983 – 1989 1992 – 1993 Nuussuup Atuarfia (Nuuk, Greenland) Primary school, 5th-8th grade 1989 – 1992 Skills Embedded software development Serial based communication (RS232/RS485/TTL/SPI/I2C/CAN/USB/Modbus RTU) Device drivers (proprietary operating systems/Linux kernel) MCU interrupt routines Concurrent and realtime programming Generic middleware layers GUI programming (Ultimate++, Ogre, CEGUI, wxWidget) Mikkel Kirkgaard Nielsen Tjaereborg Stationsvej 1, 1. -
Panorama of GUI Toolkits on Haiku
Panorama of GUI toolkits on Haiku From ncurses to Qt5 François Revol [email protected] Haiku ● Free Software rewrite of BeOS ● An Operating System for the desktop ● A lot of POSIX – But we don't claim to be Unix® ● Some more funny things – Typed & indexable xattrs Native GUI ● InterfaceKit – C++ API (similar to Qt) – BMessage objects ● Multithreaded – 1 message loop / window + 1 app main thread ● Few bindings – yab (yabasic port) Toolkit Pros/Cons ✔ More apps, less work ✗ Never completely ✔ More potential users native ✔ More devs? ✗ No Scripting support ✗ hey Foo get Frame ✔ It can be done right of View … ✗ Screen reader… ✗ Less incentive for native apps Toolkit usage in Debian GNU/Linux ● for t in $(debtags cat | tr ' ,' '\n\n' | grep uitoolkit:: | sort | uniq); do echo -en "$t\t"; debtags search $t | wc -l; ● done Whatever TODO uitoolkit::athena 92 means uitoolkit::fltk 25 uitoolkit::glut 23 ● uitoolkit::gnustep 41 Probably not the best uitoolkit::gtk 2024 metric anyway uitoolkit::motif 53 uitoolkit::ncurses 757 uitoolkit::qt 965 uitoolkit::sdl 488 uitoolkit::tk 135 uitoolkit::TODO 52 uitoolkit::wxwidgets 117 uitoolkit::xlib 254 ncurses █████ ● So what, it's a toolkit � ● Works not too bad hdialog ████▒ ● Native implementation of [x]dialog ● Some missing features ● Shanty is a similar app (Zenity clone) SDL 1.2 █████ ● Of course! SDL 2 █████ ● What Else?™ nativefiledialog █████ ● Native file selectors for Win32, GTK+3, OSX ● Ported during GCI 2014 ● For use with SDL/SDL2/… LibreOffice (VCL) █▒▒▒▒ ● Visual Class Libraries ● LibreOffice / OpenOffice's own GUI toolkit – Is it used anywhere else? IUP █▒▒▒▒ ● Multi-platform GUI toolkit – GTK+, Motif and Windows – “Bindings for C, Lua and LED – Uses native interface elements – Simplicity of its API” ● WIP port by Adrien Destugues (PulkoMandy) wxWidget ▒▒▒▒▒ ● Is this still in use? ● Oh, I need it for KiCAD! ● Port started long ago, nothing usable yet. -
CEGUI Unified Editor
Charles University in Prague Faculty of Mathematics and Physics BACHELOR THESIS Martin Preisler <[email protected]> Unified Editor for CEGUI Department of Software and Computer Science Education Supervisor of the bachelor thesis: Martin Babka Study programme: Computer Science Specialization: Programming Prague 2012 Many thanks to my supervisor, Mgr. Martin Babka, for helpful advices and mentoring through the development. I would also like to thank contributors who provided feedback, bugfixes and valuable features. I declare that I carried out this bachelor thesis independently, and only with the cited sources, literature and other professional sources. I understand that my work relates to the rights and obliga- tions under the Act No. 121/2000 Coll., the Copyright Act, as amended, in particular the fact that the Charles University in Prague has the right to conclude a license agreement on the use of this work as a school work pursuant to Section 60 paragraph 1 of the Copyright Act. In date TITLE: Unified Editor for CEGUI AUTHOR: Martin Preisler DEPARTMENT: Katedra teoretické informatiky a matematické logiky SUPERVISOR: Mgr. Martin Babka ABSTRACT: This thesis presents a free software GUI application called the CEGUI Unified Editor. The application is mainly writ- ten in Python and is licensed under GPLv3. Its purpose is to create and modify assets of graphical interfaces made with the CEGUI library. Features include project management, imageset editing and layout editing. Data for older versions of CEGUI are transparently converted using compatibility layers. Big empha- sis is put on ease of use, collaboration between multiple content authors and portability. KEYWORDS: CEGUI, editor, imageset, layout, GUI design NÁZEV PRÁCE: Unified Editor pro CEGUI AUTOR: Martin Preisler KATEDRA: Katedra teoretické informatiky a matematické logiky VEDOUCÍ BAKALÁRSKɡ PRÁCE: Mgr. -
Interactive Visualization of Graphs on Multiple Levels of Detail Using a Direct-Touch Interactive Graphics Device
Interactive Visualization of Graphs on Multiple Levels of Detail Using a Direct-Touch Interactive Graphics Device Fajran Iman Rusadi 7th August 2009 Student number: 5781574 E-mail address: [email protected] Grid Computing | Master's Thesis Supervisor: Dr. R.G. Belleman ii Interactive Visualization of Graphs on Multiple Levels of Detail Using a Direct-Touch Interactive Graphics Device Master's Thesis (Afstudeerscriptie) written by Fajran Iman Rusadi under the supervision of Dr. R.G. Belleman, and submitted in partial fulfilment of the requirements for the degree of MSc in Grid Computing at the Universiteit van Amsterdam. Date of the public defence: Members of the Thesis Committee: 26th August 2009 Dr. R.G. Belleman Prof. C.R. Jesshope, PhD Dr. I. Bethke ii Abstract Graph visualization deals with the issues of graph representation and layout: how to draw the nodes and edges and where to place the nodes or bend the edges. These issues become more complex as the size of the graph increases. Graph simplification can be used to handle the problem. Recursive graph clustering is one of the simpli- fication method that works by transforming a graph into a hierarchical graphs. This kind of graphs can be visualized using a multiple levels of detail approach that allows users to not only see the overview of the graph but also see the more detailed view version. Interactions from users are needed to enable that exploration. A multi-touch table is used as an interaction device to help create an intuitive and easy to use user interface that can support effective visualization and interaction. -
Towards Left Duff S Mdbg Holt Winters Gai Incl Tax Drupal Fapi Icici
jimportneoneo_clienterrorentitynotfoundrelatedtonoeneo_j_sdn neo_j_traversalcyperneo_jclientpy_neo_neo_jneo_jphpgraphesrelsjshelltraverserwritebatchtransactioneventhandlerbatchinsertereverymangraphenedbgraphdatabaseserviceneo_j_communityjconfigurationjserverstartnodenotintransactionexceptionrest_graphdbneographytransactionfailureexceptionrelationshipentityneo_j_ogmsdnwrappingneoserverbootstrappergraphrepositoryneo_j_graphdbnodeentityembeddedgraphdatabaseneo_jtemplate neo_j_spatialcypher_neo_jneo_j_cyphercypher_querynoe_jcypherneo_jrestclientpy_neoallshortestpathscypher_querieslinkuriousneoclipseexecutionresultbatch_importerwebadmingraphdatabasetimetreegraphawarerelatedtoviacypherqueryrecorelationshiptypespringrestgraphdatabaseflockdbneomodelneo_j_rbshortpathpersistable withindistancegraphdbneo_jneo_j_webadminmiddle_ground_betweenanormcypher materialised handaling hinted finds_nothingbulbsbulbflowrexprorexster cayleygremlintitandborient_dbaurelius tinkerpoptitan_cassandratitan_graph_dbtitan_graphorientdbtitan rexter enough_ram arangotinkerpop_gremlinpyorientlinkset arangodb_graphfoxxodocumentarangodborientjssails_orientdborientgraphexectedbaasbox spark_javarddrddsunpersist asigned aql fetchplanoriento bsonobjectpyspark_rddrddmatrixfactorizationmodelresultiterablemlibpushdownlineage transforamtionspark_rddpairrddreducebykeymappartitionstakeorderedrowmatrixpair_rddblockmanagerlinearregressionwithsgddstreamsencouter fieldtypes spark_dataframejavarddgroupbykeyorg_apache_spark_rddlabeledpointdatabricksaggregatebykeyjavasparkcontextsaveastextfilejavapairdstreamcombinebykeysparkcontext_textfilejavadstreammappartitionswithindexupdatestatebykeyreducebykeyandwindowrepartitioning -
Dynamically Registering C++ Exception Handlers: Centralized Handling of C++ Exceptions in Application Framework Libraries
Dynamically Registering C++ Exception Handlers: Centralized Handling of C++ Exceptions in Application Framework Libraries by Nathaniel H. Daly A thesis submitted in partial fulfillment of the requirements for the degree of Bachelor of Science (Honors Computer Science) in the University of Michigan 2013 Thesis Committee: Professor David Kieras, Advisor Professor David Paoletti, Second Reader Abstract The C++ exceptions mechanism enables modularized programs to separate the reporting of exceptional conditions from the handling of those exceptional condi- tions into the locations where those actions can best be performed. It is often the case in such modular applications that the module capable of detecting an excep- tional situation cannot know how it should be handled, and so C++ exceptions allow the module that invoked the operation to react to the condition. Libraries developed separately from the applications using them are a perfect example of modularized code, and thus a perfect candidate for using C++ exceptions, how- ever many of the application framework library paradigms that have become commonplace do not provide adequate exception support to allow for good pro- gramming style with regard to catching and handling exceptions. In these librar- ies, there often exist multiple callback-style entry points that invoke application- specific operations, all of which require exactly identical exception handling, yet there is no way to collect this code together. Instead, the exception handling code must be duplicated across each entry point. In this paper, I will explore the best solutions to this problem currently provided by such frameworks; I will propose an additional solution to this problem: a method for allowing a program to regis- ter exception-handler operations templated by exception type; and finally, I will identify any impacts that the newly released C++11 standard and the currently evolving C++14 standard have on the problem. -
Title in 14 Point Arial Bold Centered
Criação de elementos interativos 2D para API’s gráficas utilizando CEGUI Daniel. M. Tortelli Jorge. L. Salvi Maikon. C. Santos Jacques. D. Brancher Universidade Regional Integrada do Alto Uruguai e das Missões – Campus de Erechim, Brasil Figura 1: Imagem utilizando elementos gráficos interativos da CEGUI. Abstract Em aplicações gráficas interativas, como por The objective of this paper is present the interactive exemplo jogos de computador, é necessário que os creation process stages of the elements 2D (widgets) elementos interativos sejam originais e o mais for graphical API's 3D, as OpenGL, Ogre 3D. The intuitivos possível para o usuário, o que influencia given emphasis is referring to the interface elements, diretamente na qualidade da jogabilidade. indispensable for the user-system interaction created in a computational game. The functioning of CEGUI Durante o processo de criação de um jogo, uma das library for creation of GUI is shown (Graphical User etapas necessárias é o desenvolvimento de um sistema Interface). de GUI. API’s gráficas como OpenGL e Ogre3D não dispõe desse recurso nativo, necessitando que ele seja Keywords: widgets, CEGUI, Ogre 3D, Graphical User criado e incorporado como parte do motor do jogo. Interface, interatividade Entretanto, o desenvolvimento de um sistema de Authors’ contact: uma GUI consome um tempo considerável no projeto {daniel.beka, maikoncs}@gmail.com de um jogo. Uma solução alternativa para quem faz {j_brancher, jlsalvi}@hotmail.com uso dessas API’s para criação de aplicações gráficas interativas é utilizar sistemas prontos de GUI, como o Sistema de GUI denominado CEGUI. 1. Introdução O objetivo deste artigo é apresentar as etapas de A interface com o usuário é uma parte importante para criação de um sistema de GUI utilizando a biblioteca qualquer tipo de aplicação digital, principalmente para CEGUI. -
CEGUI Unified Editor Developer Manual
CEGUI Unified Editor Developer Manual Martin Preisler July 13, 2014 ☛ ✟ This document has been laid out for a computer screen viewing and thus may be unsuitable for printing. LYX sources are available in doc/developer-manual-src in the source tarball if you wish to relayout. ✡ ✠ 1 Contents 1 Prerequisites 4 1.1 Knowledgerequirements . .................. 4 1.2 Gettingthesourcecode. ................... 4 1.2.1 BranchesandTags ............................... .............. 4 1.3 Starting without installation . ....................... 4 2 Directory structure 5 2.1 Topdirectory.................................... ................. 5 2.1.1 maintenancescript .... ... .... .... .... ... .... ... ................ 5 2.1.2 perform-pylint ................................ ............... 5 2.1.3 setup.py ...................................... ............. 5 2.1.4 cx_Freezer.py ................................. ............... 5 2.1.5 copyrightrelated .............................. ................ 5 2.2 bindirectory.................................... ................. 5 2.2.1 ceed-gui ...................................... ............. 5 2.2.2 ceed-mic...................................... ............. 5 2.2.3 ceed-migrate .................................. .............. 6 2.2.4 runwrapper.sh ................................. .............. 6 2.3 builddirectory.................................. .................. 6 2.4 ceeddirectory ................................... ................. 6 2.4.1 actionsubpackage .............................. .............. -
Game Engine Fundamentals
Game Engine Fundamentals Thanos Tsakiris Research Associate, CERTH/ITI What is a Game Engine? Above all else … NOT RESTRICTED TO GAME DEVELOPMENT! Game = Simulation A game engine is a framework comprised of a collection of different tools, utilities and interfaces that hide the lowlow--levellevel details of the various tasks that make up the game. A game engine is the core software of a game or a simulation and is used to describe a set of code used to develop the game. Everything you see on the screen and interact with in aa game world or simulation environment is powered by the game enenginegine It allows the abstraction of the details of doing common gameor simulation relatedtasks (e.g. rendering, physics, input), so that developers can focus on the aspectsthat make their games or simulations unique. Popular Game Engines Commercial ID Tech 4 (Quake 4, Doom3) Steam (Half(Half--LifeLife 2, Left4Dead) CryEngine, CryEngine 2 (Far Cry) Unreal Engine LithTech (F.E.A.R) Open Source OGRE Delta3D ID Tech 3 Irrlicht Platforms Most Game Engines are developed with portability in mind Target platforms: PC Wide range of CPUs Wide range of graphics cards Wide range of audio cards Wide range of memory Wide range of I/O devices Wide range of operating systems (Apple OSX, Linux) DirectX, OpenGL graphics subsystems Platforms Other platforms: VR Installations (CAVE, HMDs etc) Cell phones, PDAs, etc. Game Consoles: Sony PS1, PS2, PS3, PSP Nintendo Wii, DS Microsoft Xbox, Xbox 360 Classic consoles Arcade Location based entertainment