Learning the Bash Shell Other Resources from O’Reilly
Total Page:16
File Type:pdf, Size:1020Kb
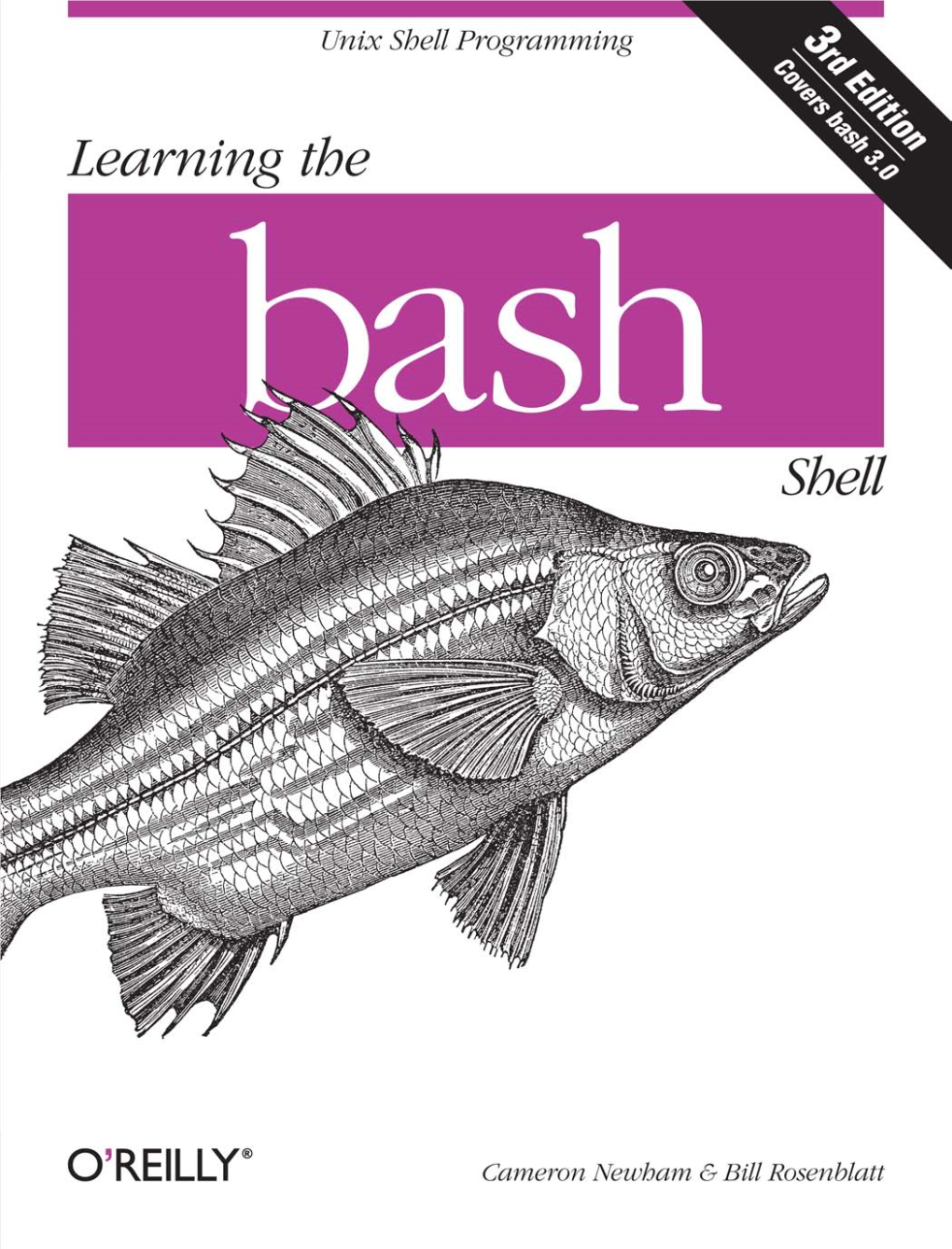
Load more
Recommended publications
-
Linksys Viewer Cab File
Linksys viewer cab file We would like to show you a description here but the site won’t allow us. The first step is to download the cab file from your Linksys camera. You will want to place this in the same directory as your html document. We would like to show you a description here but the site won’t allow us. Jun 27, 2012 · This video is in response to the search traffic my blog continually gets on how to download these Linksys IP Camera Cab files. Four years ago I posted. Download Linksys Ml Viewer Cab related programs and software, check Linksys Ml Viewer Cab latest version. Download the latest Linksys WVC80N device drivers (Official and Certified). Linksys WVC80N drivers updated daily. Download Now. Jul 23, 2015 · Cam Viewer for Linksys cameras. 820. Cam Viewer Mobi Business. Everyone. Offers in-app purchases. Viewer for Linksys IP Cameras . … ineed linksys viewer . 0 Kudos Message 5 of 9 (8,550 Views) Reply. kawal. Posts. download for viewer & recorder utility. Options. Mark as New; Bookmark; Subscribe; File Name: External File. iWATCH DVR is a H.264 DVR remote viewer. It can be viewed in. Submit a problem report for Viewer for Linksys Cams. Learn about, buy and get support for the many home networking products we manufacture, including wireless routers, range extenders and network cameras. Linksys Driver Update How To.. Once the file(s) are updated the Linksys WUSB54GC V1 can. Requires knowledge of command line and installshield cab file viewer. How To Embed a Linksys Wireless-G Internet Home Monitoring Camera. -
Storebackup 3.5.1
StoreBackup 3.5.1 https://savannah.nongnu.org/projects/storebackup January 27, 2020 1 Super Quick Start StoreBackup is a very space efficient disk-to-disk backup suite for GNU/Linux and other unixoid systems. Additional details and help are provided in later sections of this document. In these brief quick start steps we make certain simplifying assumptions. If you are OK with that, then proceed as follows: 1. Download the source from https://download.savannah.nongnu.org/releases/storebackup/ 2. Unpack it (using tar -jxvf) into /opt (it will make the folder /opt/storeBackup.)1 3. Create symbolic links. In a terminal, run these 2 commands (the 2nd line ends with: space,dot): # cd /usr/local/bin # ln -s /opt/storeBackup/bin/* . 4. Run your first backup with this command (substituting your actual username in the command):2 storeBackup.pl --sourceDir /home/your_username --backupDir /tmp/my_master_backup This may take a while. Open a second shell and see what happens in the backup directory. You have now backed up your home directory to /tmp/my master backup. For more details, please continue reading; especially see installation, section 2 and storeBackup.pl, section 6.2. If the above steps gave you any challenges, don’t worry. This document will cover everything from storeBackup installation to NFS server settings in much more detail. See storeBackup’s Top Features on the next page 1You need root permissions to install storeBackup at /opt/storeBackup and to follow the next steps. You can also unpack and run storeBackup from a place where you do not have root permissions. -
Revista Espirito Livre
SACIX TerminaislevesomLinux http://revista.espiritolivre.org | #002 | Mio 2009 TCOSPROJECT Montando Thin Clientsno Linux DÚVIDA CRUEL... LEVEZA, Qual a melhor distribuiÇÃo Linux? ESTABILIDADE E PERFORMANCE As distribuiÇÕes Linux dÃo um show quando o assunto É isso! ENTREVISTA ISCA ANZOL REDE DOTPROJECT Robert Shingledeker Cuidado, muito uidado Gereniamento de fala sobre o TinyCore, omertospesdores... projetosomSoftware seu novo projeto Livre COMLICENÇA Revist EspÍrito Livre | Mio 2009 | http://revista.espiritolivre.org |02 EDITORIAL / EXPEDIENTE Chegamos para ficar! EXPEDIENTE DiretorGerl JoÃo Fernando Costa Júnior Aqui estamos nÓs, depois do turbilhÃo e euforia quanto ao lançmento da ediÇÃo nÚmero um, retornamos om um ediÇÃo ainda mis reheada de Editor muita informÇÃo par voê, leitor. AtÉ o presente momento a primeir ediÇÃo JoÃo Fernando Costa Júnior teve 8173 downloads, um mr que a meu ver, par um publicaÇÃo om foo espeífio, meree menÇÃo. Etudo isso grçsa voê, que estÁ a nosler neste momento e que possivelmente teve de posse da ediÇÃo de nÚmero um. Revisão Se ainda nÃo leu, orr atrásda sua! Mrelo Tonieto Nesta ediÇÃo, voê enontrrá um assunto que rende muito pano pr Arte e DiagrmÇÃo mnga: distribuiÇÕes leves e om propÓsitos espeífios, trzendo um JoÃo Fernando Costa Júnior novidade: 2 entrevistas, a internaional, omRobert Shingledeker, riadordo Damn Smll Linuxe misreentemente o TinyCore, ambasdistribuiÇÕesbem Capa focadas no objetivo de ter um distribuiÇÃo leve, funional e de alta Nilton Pessnha performne em omputadores om baixos requisitos de hardware. A entrevista naional É om FlÁvio de Oliveir, responsável pela distribuiÇÃo Contribuirmnesta ediÇÃo GoblinX Linux, um distro bacana e desolada, que trz um visual bem Aéio Pires moderno e igualmente leve. -
Compute Issue 064 1985 Sep.Pdf
Word Search: Puzzle Program Inside For Commodore, Atari, Apple, IBM, & Tl ~~it~mber 1985 Issue 64 I Vol. 7, No. 9 Canada M/i3 g II ISSN 0194-357X The Leading Magazine Of Home, Educational, And Recreational Computing Programs Inside: I .I.--~- Atari Animation All About C64 · IBM Batch Files 1962 71fc 2IJ ~ump JSearcli 19~ 1 For ·commodore, Atari, Apple, IBM, And Tl Easy Apple Screen Editing Enhanced BASIC Line Editor For Apple ~II+, lie 09 0 107 COMPU TER ROOM With Commodore 128's instead of Apple llc's, these kids would be on computers instead of in line. Meet the Commodore 128.™The That adds a built-in extra cost for it less versatile. It also doesn't new personal computer that's al a feature your classroom doesn't have the Commodore 128's ex ready destined to be at the head really need. There's even more to panded keyboard that offers of its class. It not only outsmarts the Commodore 128 than being more commands for easier pro the Apple ~ Ile in price, it comes able to put more students on com gramming and more varied out way ahead in performance. puters for less money. use of graphics and text. Or a A lower price is welcome numeric keypad that's a real news to any tight school budget. There's more intelligence. necessity when using a computer But it's not the only way the 128 As your students grow smarter, in math or science classes. saves you money. You only need so does the Commodore 128. -
Special Characters A
453 Index ■ ~/Library/Safari/WebpageIcons.db file, Special Characters 112 $(pwd) command, 89–90 ~/Library/Saved Searches directory, 105 $PWD variable, 90 ~/Library/Services directory, 422–423 % (Execute As AppleScript) menu option, ~/Library/Workflow/Applications/Folder 379 Actions folder, 424 ~/ directory, 6, 231 ~/Library/Workflows/Applications/Image ~/bin directory, 6, 64, 291 Capture folder, 426 ~/Documents directory, 281, 290 ~/Movies directory, 323, 348 ~/Documents/Knox directory, 255 ~/Music directory, 108, 323 ~/Downloads option, 221, 225 ~/Music/Automatically Add To iTunes ~/Downloads/Convert For iPhone folder, folder, 424 423–424 ~/Pictures directory, 281 ~/Downloads/MacUpdate ~/.s3conf directory, 291 Desktop/MacUpdate Desktop ~/ted directory, 231 2010-02-20 directory, 16 ~/Templates directory, 60 ~/Downloads/To Read folder, 425 ~/Templates folder, 62 ~/Dropbox directory, 278–282 Torrent program, 236 ~/Library folder, 28 1Password, 31, 135, 239–250 ~/Library/Application 1Password extension button, 247–248 Support/Evom/ffmpeg directory, 1Password.agilekeychain file, 249 338 1PasswordAnywhere tool, 249 ~/Library/Application 1Password.html file, 250 Support/Fluid/SSB/[Your 2D Black option, 52 SSB]/Userstyles/ directory, 190 2D With Transparency Effect option, 52 ~/Library/Application Support/TypeIt4Me/ 2-dimensional, Dock, 52 directory, 376 7digital Music Store extension, 332 ~/Library/Caches/com.apple.Safari/Webp age Previews directory, 115 ~/Library/Internet Plug-Ins directory, 137 ■A ~/Library/LaunchAgents directory, 429, 432 -
Google Overview Created by Phil Wane
Google Overview Created by Phil Wane PDF generated using the open source mwlib toolkit. See http://code.pediapress.com/ for more information. PDF generated at: Tue, 30 Nov 2010 15:03:55 UTC Contents Articles Google 1 Criticism of Google 20 AdWords 33 AdSense 39 List of Google products 44 Blogger (service) 60 Google Earth 64 YouTube 85 Web search engine 99 User:Moonglum/ITEC30011 105 References Article Sources and Contributors 106 Image Sources, Licenses and Contributors 112 Article Licenses License 114 Google 1 Google [1] [2] Type Public (NASDAQ: GOOG , FWB: GGQ1 ) Industry Internet, Computer software [3] [4] Founded Menlo Park, California (September 4, 1998) Founder(s) Sergey M. Brin Lawrence E. Page Headquarters 1600 Amphitheatre Parkway, Mountain View, California, United States Area served Worldwide Key people Eric E. Schmidt (Chairman & CEO) Sergey M. Brin (Technology President) Lawrence E. Page (Products President) Products See list of Google products. [5] [6] Revenue US$23.651 billion (2009) [5] [6] Operating income US$8.312 billion (2009) [5] [6] Profit US$6.520 billion (2009) [5] [6] Total assets US$40.497 billion (2009) [6] Total equity US$36.004 billion (2009) [7] Employees 23,331 (2010) Subsidiaries YouTube, DoubleClick, On2 Technologies, GrandCentral, Picnik, Aardvark, AdMob [8] Website Google.com Google Inc. is a multinational public corporation invested in Internet search, cloud computing, and advertising technologies. Google hosts and develops a number of Internet-based services and products,[9] and generates profit primarily from advertising through its AdWords program.[5] [10] The company was founded by Larry Page and Sergey Brin, often dubbed the "Google Guys",[11] [12] [13] while the two were attending Stanford University as Ph.D. -
Dakota, a Multilevel Parallel Object-Oriented Framework For
SAND2014-4633 Unlimited Release July 2014 Updated May 15, 2019 Dakota, A Multilevel Parallel Object-Oriented Framework for Design Optimization, Parameter Estimation, Uncertainty Quantification, and Sensitivity Analysis: Version 6.10 User’s Manual Brian M. Adams, Michael S. Eldred, Gianluca Geraci, Russell W. Hooper, John D. Jakeman, Kathryn A. Maupin, Jason A. Monschke, Ahmad A. Rushdi, J. Adam Stephens, Laura P. Swiler, Timothy M. Wildey Optimization and Uncertainty Quantification Department William J. Bohnhoff Radiation Effects Theory Department Keith R. Dalbey Software Simulation and Analysis Department Mohamed S. Ebeida Discrete Math and Optimization Department John P. Eddy Mathematical Analysis and Decision Sciences Department Patricia D. Hough, Mohammad Khalil Quantitative Modeling and Analysis Department Kenneth T. Hu W76-1 System Life Extension Department Elliott M. Ridgway, Dena M. Vigil Software Engineering and Research Department Justin G. Winokur V&V, UQ, Credibility Processes Department Sandia National Laboratories P.O. Box 5800 Albuquerque, New Mexico 87185 4 Abstract The Dakota toolkit provides a flexible and extensible interface between simulation codes and iterative analysis methods. Dakota contains algorithms for optimization with gradient and nongradient-based methods; uncertainty quantification with sampling, reliability, and stochastic expansion methods; parameter estimation with nonlinear least squares methods; and sensitivity/variance analysis with design of experiments and parameter study methods. These capabilities may be used on their own or as components within advanced strategies such as surrogate- based optimization, mixed integer nonlinear programming, or optimization under uncertainty. By employing object-oriented design to implement abstractions of the key components required for iterative systems analyses, the Dakota toolkit provides a flexible and extensible problem-solving environment for design and performance analysis of computational models on high performance computers. -
Practical-Computing
REVIEWS Canon AS -100C BBC graphics packages Orion ,.J 1PrOVICA41AS:"E"E-Ticx. '1/474..1 .0.0c 4X Sy.u...:_-,4a.sosirN3 Stec o. Cromemco System One MicroCentre introduce Cromemco's newSystem One computer, available with an integral 5 megabyteWinchester hard disk, at a new low price. The System One supports thefull range of Cromemco interfacecards, including high resolution colourgraphics, and software packages. The choice of operating systemsincludes CDOS, CP/M and CROMIX Cromemco's answer toUnix. Call MicroCentrefor Q Cromemco 30 Dundas Street MicroCentre Ltd Britain's independent Edinburgh EH3 6JN Cromemco importer (Complete Micro Systems) Tel: 031-556 7354 Circle No. 101 PRACTICAL COMPUTING MAY 1983 DATABASED 1842ATARI BOOKS 128CHARITY *a BY THE DOZEN >NEWS How the National Association for Twelve books that set out to tell you SOFTWARE NEWS Gifted Children uses a database to what Atari doesn't tell you. 21New packages and other keep in touch with its members announcements. around the country. >FEATURES 2.2HARDWARE NEWS %IP What's happening in the >REV I EWS INTERVIEW personal -computer world. ihi MARTIN HEALEY 7aCANON AS -100C Strong views on operating systems IBM PC NEWS WI NEW FROM JAPAN and the future of the British micro 29"Big Blue" launches The editor tried out the latest IBM - from the man who designed the a go -faster version of the PC; plus PC work -alike. Find out why he Orion. more newly announced software. cried when they came and took it away. ROBOT PING-PONG 3.2DIARY 99John Billingsley of Li Forthcoming events, 8/TEXET'S LOW -PRICE Micromouse fame introduces a exhibitions and conferences. -
Guardian User's Guide
Guardian User’s Guide Abstract This guide provides basic information about the programs and utilities that are used most often in the Guardian environment by general system or application users. It also provides more detailed procedures for system operations, management, and maintenance tasks useful to both beginning and experienced Compaq NonStop™ Kernel operating system users. Product Version N.A. Supported Releases This manual supports G06.05 and all subsequent G-series releases, and D48.00 and all subsequent D-series releases, until otherwise indicated in a new edition. Part Number Published 425266-001 August 2000 Document History Part Number Product Version Published 425266-001 N.A. August 2000 421957-001 N.A. June 1999 142477 N.A. December 1998 117897 N.A. July 1995 089808 N.A. January 1993 Ordering Information For manual ordering information: domestic U.S. customers, call 1-800-243-6886; international customers, contact your local sales representative. Document Disclaimer Information contained in a manual is subject to change without notice. Please check with your authorized representative to make sure you have the most recent information. Export Statement Export of the information contained in this manual may require authorization from the U.S. Department of Commerce. Examples Examples and sample programs are for illustration only and may not be suited for your particular purpose. The inclusion of examples and sample programs in the documentation does not warrant, guarantee, or make any representations regarding the use or the results of the use of any examples or sample programs in any documentation. You should verify the applicability of any example or sample program before placing the software into productive use. -
A Model of Consumer Eservice Adoption Within Dubai
School of Information Systems A model of consumer eService adoption within Dubai Gregory Phillip Kelaart-Courtney This thesis is presented for the Degree of Doctor of Philosophy of Curtin University of Technology March 2010 DECLARATION To the best of my knowledge and belief this thesis contains no material previously published by any other person except where due acknowledgment has been made. This thesis contains no material which has been accepted for the award of any other degree or diploma in any university. Signature: …………………………………………. Date: ………………………... i ABSTRACT This study will create a model to determine the level of adoption of eServices (consisting of eCommerce and eBanking) within Dubai, an Emirate within the United Arab Emirates (UAE), and how this adoption has been influenced by an individual’s exposure to an ‘Emerging and Developing Economy’ and/or an ‘Advanced Economy’. The study investigates the ‘enablers’ and ‘inhibitors’ for individuals belonging to the Emerging and Developing Economies, Advanced Economies and a hybrid of the two. Lastly, for those individuals who do partake in eServices the researcher also identifies similar trends and differences in usage patterns between Dubai and Advanced Economy countries and regions such as the United States (US), Canada, United Kingdom (UK) and Western Europe. This research utilises a positivist methodological approach in the collection and analyses of the primary data vital to achieving the aforementioned aims surrounding the adoption of eServices within Dubai. Two anonymous research instruments, online questionnaires, were distributed to potential respondents, firstly in 2005, and then a more advanced version in 2008. Both research instruments were distributed throughout Dubai via social networking sites, expatriate website groups, multi-national companies’ HR departments, Dubai Government organisations and several Emirati only and mixed nationality universities, to both faculty and students. -
Χρήση Του Λειτουργικού Συστήµατος Unix/Linux
ΕΛΛΗΝΙΚΗ ∆ΗΜΟΚΡΑΤΙΑ ΥΠΟΥΡΓΕΙΟ ΕΣΩΤΕΡΙΚΩΝ, ∆ΗΜΟΣΙΑΣ ∆ΙΟΙΚΗΣΗΣ ΚΑΙ ΑΠΟΚΕΝΤΡΩΣΗΣ ΙΝΣΤΙΤΟΥΤΟ ΕΠΙΜΟΡΦΩΣΗΣ ΤΟΜΕΑΣ ΝΕΩΝ ΤΕΧΝΟΛΟΓΙΩΝ Επιστηµονικός Υπεύθυνος: Ηλίας Μαραγκός ΕΚΠΑΙ∆ΕΥΤΙΚΟ ΥΛΙΚΟ Χρήση του Λειτουργικού Συστήµατος Unix/Linux Συντάκτης: Μανόλης Παπαστεφανάκης Χρήση του Λειτουργικού Συστήµατος Unix/Linux Εκπαιδευτικές σηµειώσεις Χρήση Λειτουργικού Συστήµατος Unix/Linux – i Πρόλογος Το παρόν κείµενο αποτελεί εκπαιδευτικό υλικό για το σεµινάριο «Χρήση του Λειτουργικού Συστήµατος Unix/Linux», το οποίο εντάσσεται σε ένα κύκλο σεµιναρίων του Ινστιτούτου Επιµόρφωσης του Εθνικού Κέντρου ∆ηµόσιας ∆ιοίκησης και Αυτοδιοίκησης. ∆εδοµένης της έκτασης του υλικού κάθε σεµιναρίου και του αλληλεπιδραστικού τρόπου διεξαγωγής των σεµιναρίων, καθώς και της ευρείας χρήσης εργαστηριακών ασκήσεων, ως µέθοδος συγγραφής αυτών των εκπαιδευτικών σηµειώσεων προκρίθηκε η σύντοµη αναφορά σε όλα τα θέµατα που αναπτύσσονται στα σεµινάρια και η «ανάπτυξή» τους από τον κάθε χρήστη ξεχωριστά. Η επιλογή αυτή έγινε για δύο λόγους: α) Η έκταση του υλικού. Η ύλη που καλύπτεται σε κάθε ένα από τα σεµινάρια του Unix/Linux είναι συµπυκνωµένη και καλύπτει υλικό ενός εξαµηνιαίου εργαστηριακού µαθήµατος, συγκερασµένου µε την εµπειρία της χρήσης του Unix/Linux σε πραγµατικό περιβάλλον παραγωγής. β) Το πλήθος των σχετικών βιβλίων. Τα βιβλία που αναφέρονται στο Unix/Linux είναι εξαιρετικά πολυάριθµα και καθένα απ’ αυτά χρησιµοποιεί ξεχωριστή µεθοδολογία. Το πρόβληµα, λοιπόν, είναι ποιο βιβλίο ταιριάζει σε όλους τους χρήστες. ∆υστυχώς, δεν υπάρχει άλλη απάντηση, παρά «όποιο ταιριάζει στον κάθε χρήστη». Προκειµένου να αντιµετωπιστούν τα ζητήµατα που τέθηκαν παραπάνω και να τηρηθεί σε όλο το εύρος των σεµιναρίων µία µόνο µεθοδολογία συγγραφής, προτιµήθηκε η συγγραφή των εκπαιδευτικών σηµειώσεων µε τέτοιο τρόπο, ώστε αυτές να συµπληρώνονται από κάθε εκπαιδευόµενο µε το υλικό που εκείνος κρίνει απαραίτητο. -
Ddpv3bestpractiseguide
DDPV3BestPractiseGuide November 27, 2013 1 Contents 1 Introduction 4 2 Best practises in setting up the ethernet network in combina- tion with the DDP 4 2.1 Each ethernet port on the DDP must be a unique subnet . 4 2.2 Autosensing or not . 4 2.3 A 1GbE infrastructure . 5 2.4 An all 10 GbE infrastructure . 5 2.5 1 GbE switch with 10 GbE uplink . 5 2.6 Flow Control, Bandwidth Limiting, Traffic Control and Switch buffer Overrun . 6 2.7 Subnet and VLAN . 6 2.8 Teaming, Bonding . 7 2.9 Firewall Protection . 7 3 Using DDP with Avid Applications except DS 7 3.1 Avid Facts To Know . 7 3.1.1 One Avid MediaFiles directory per DDP volume or fold- ervolume . 8 3.1.2 Not enough Drive Letters . 8 3.1.3 Do not use these characters with Avid . 8 3.2 Lock files and bins . 8 3.3 Sharing Methods . 9 3.3.1 Shared Bins . 9 3.3.2 Shared Bins and Projects . 9 3.3.3 Shared Bin and Project Limitations . 10 3.3.4 Shared Bin Lock Icon Limitation . 11 3.3.5 Naming Conventions . 11 3.3.6 Shared Bins and Windows Explorer . 12 3.4 Time Synchronization . 12 3.5 User Access Control with Avid edit applications and DDP . 12 3.6 Avid Editors and AMA material on Windows . 12 3.6.1 One large DDP volume and foldervolumes for all projects with a foldervolume for AMA material . 13 3.7 Using the DDP in combination with Avid Storage .