Space-Based Partitioning Data Structures and Their Algorithms
Total Page:16
File Type:pdf, Size:1020Kb
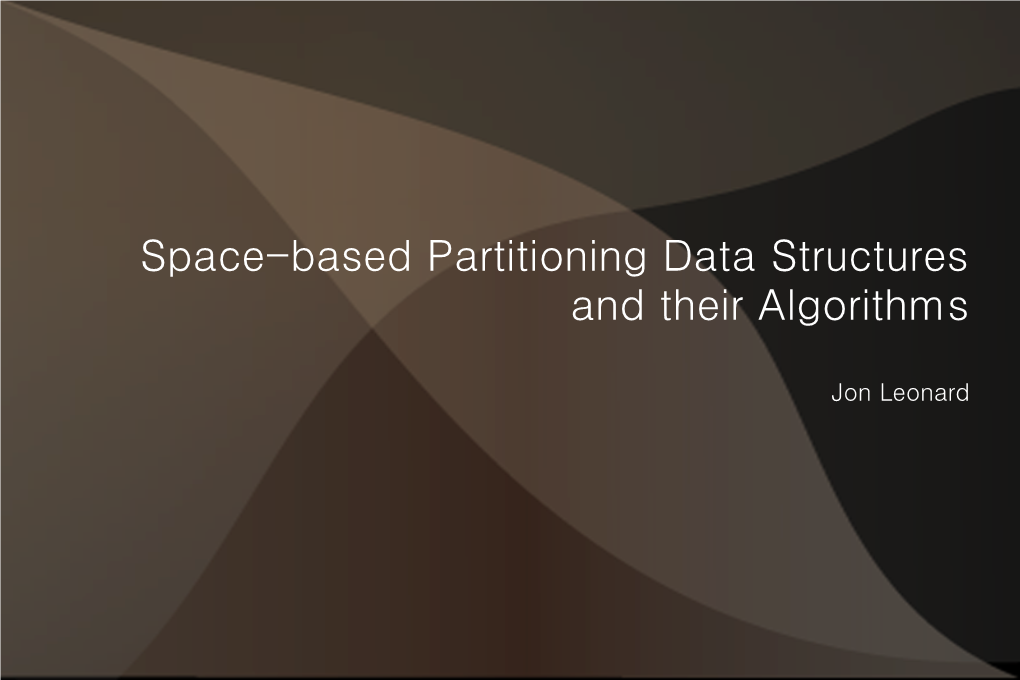
Load more
Recommended publications
-
Efficient Evaluation of Radial Queries Using the Target Tree
Efficient Evaluation of Radial Queries using the Target Tree Michael D. Morse Jignesh M. Patel William I. Grosky Department of Electrical Engineering and Computer Science Dept. of Computer Science University of Michigan, University of Michigan-Dearborn {mmorse, jignesh}@eecs.umich.edu [email protected] Abstract from a number of chosen points on the surface of the brain and end in the tumor. In this paper, we propose a novel indexing structure, We use this problem to motivate the efficient called the target tree, which is designed to efficiently evaluation of a new type of spatial query, which we call a answer a new type of spatial query, called a radial query. radial query, which will allow for the efficient evaluation A radial query seeks to find all objects in the spatial data of surgical trajectories in brain surgeries. The radial set that intersect with line segments emanating from a query is represented by a line in multi-dimensional space. single, designated target point. Many existing and The results of the query are all objects in the data set that emerging biomedical applications use radial queries, intersect the line. An example of such a query is shown in including surgical planning in neurosurgery. Traditional Figure 1. The data set contains four spatial objects, A, B, spatial indexing structures such as the R*-tree and C, and D. The radial query is the line emanating from the quadtree perform poorly on such radial queries. A target origin (the target point), and the result of this query is the tree uses a regular hierarchical decomposition of space set that contains the objects A and B. -
The Skip Quadtree: a Simple Dynamic Data Structure for Multidimensional Data
The Skip Quadtree: A Simple Dynamic Data Structure for Multidimensional Data David Eppstein† Michael T. Goodrich† Jonathan Z. Sun† Abstract We present a new multi-dimensional data structure, which we call the skip quadtree (for point data in R2) or the skip octree (for point data in Rd , with constant d > 2). Our data structure combines the best features of two well-known data structures, in that it has the well-defined “box”-shaped regions of region quadtrees and the logarithmic-height search and update hierarchical structure of skip lists. Indeed, the bottom level of our structure is exactly a region quadtree (or octree for higher dimensional data). We describe efficient algorithms for inserting and deleting points in a skip quadtree, as well as fast methods for performing point location and approximate range queries. 1 Introduction Data structures for multidimensional point data are of significant interest in the computational geometry, computer graphics, and scientific data visualization literatures. They allow point data to be stored and searched efficiently, for example to perform range queries to report (possibly approximately) the points that are contained in a given query region. We are interested in this paper in data structures for multidimensional point sets that are dynamic, in that they allow for fast point insertion and deletion, as well as efficient, in that they use linear space and allow for fast query times. Related Previous Work. Linear-space multidimensional data structures typically are defined by hierar- chical subdivisions of space, which give rise to tree-based search structures. That is, a hierarchy is defined by associating with each node v in a tree T a region R(v) in Rd such that the children of v are associated with subregions of R(v) defined by some kind of “cutting” action on R(v). -
Principles of Computer Game Design and Implementation
Principles of Computer Game Design and Implementation Lecture 14 We already knew • Collision detection – high-level view – Uniform grid 2 Outline for today • Collision detection – high level view – Other data structures 3 Non-Uniform Grids • Locating objects becomes harder • Cannot use coordinates to identify cells Idea: choose the cell size • Use trees and navigate depending on what is put them to locate the cell. there • Ideal for static objects 4 Quad- and Octrees Quadtree: 2D space partitioning • Divide the 2D plane into 4 (equal size) quadrants – Recursively subdivide the quadrants – Until a termination condition is met 5 Quad- and Octrees Octree: 3D space partitioning • Divide the 3D volume into 8 (equal size) parts – Recursively subdivide the parts – Until a termination condition is met 6 Termination Conditions • Max level reached • Cell size is small enough • Number of objects in any sell is small 7 k-d Trees k-dimensional trees • 2-dimentional k-d tree – Divide the 2D volume into 2 2 parts vertically • Divide each half into 2 parts 3 horizontally – Divide each half into 2 parts 1 vertically » Divide each half into 2 parts 1 horizontally • Divide each half …. 2 3 8 k-d Trees vs (Quad-) Octrees • For collision detection k-d trees can be used where (quad-) octrees are used • k-d Trees give more flexibility • k-d Trees support other functions – Location of points – Closest neighbour • k-d Trees require more computational resources 9 Grid vs Trees • Grid is faster • Trees are more accurate • Combinations can be used Cell to tree Grid to tree 10 Binary Space Partitioning • BSP tree: recursively partition tree w.r.t. -
Computation of Potentially Visible Set for Occluded Three-Dimensional Environments
Computation of Potentially Visible Set for Occluded Three-Dimensional Environments Derek Carr Advisor: Prof. William Ames Boston College Computer Science Department May 2004 Page 1 Abstract This thesis deals with the problem of visibility culling in interactive three- dimensional environments. Included in this thesis is a discussion surrounding the issues involved in both constructing and rendering three-dimensional environments. A renderer must sort the objects in a three-dimensional scene in order to draw the scene correctly. The Binary Space Partitioning (BSP) algorithm can sort objects in three- dimensional space using a tree based data structure. This thesis introduces the BSP algorithm in its original context before discussing its other uses in three-dimensional rendering algorithms. Constructive Solid Geometry (CSG) is an efficient interactive modeling technique that enables an artist to create complex three-dimensional environments by performing Boolean set operations on convex volumes. After providing a general overview of CSG, this thesis describes an efficient algorithm for computing CSG exp ression trees via the use of a BSP tree. When rendering a three-dimensional environment, only a subset of objects in the environment is visible to the user. We refer to this subset of objects as the Potentially Visible Set (PVS). This thesis presents an algorithm that divides an environment into a network of convex cellular volumes connected by invisible portal regions. A renderer can then utilize this network of cells and portals to compute a PVS via a depth first traversal of the scene graph in real-time. Finally, this thesis discusses how a simulation engine might exploit this data structure to provide dynamic collision detection against the scene graph. -
Introduction to Computer Graphics Farhana Bandukwala, Phd Lecture 17: Hidden Surface Removal – Object Space Algorithms Outline
Introduction to Computer Graphics Farhana Bandukwala, PhD Lecture 17: Hidden surface removal – Object Space Algorithms Outline • BSP Trees • Octrees BSP Trees · Binary space partitioning trees · Steps: 1. Group scene objects into clusters (clusters of polygons) 2. Find plane, if possible that wholly separates clusters 3. Clusters on same side of plane as viewpoint can obscure clusters on other side 4. Each cluster can be recursively subdivided P1 P1 back A front D P2 P2 3 1 front back front back P2 B D C A B C 3,1,2 3,2,1 1,2,3 2,1,3 2 BSP Trees,contd · Suppose the tree was built with polygons: 1. Pick 1 polygon (arbitrary) as root 2. Its plane is used to partition space into front/back 3. All intersecting polygons are split into two 4. Recursively pick polygons from sub-spaces to partition space further 5. Algorithm terminates when each node has 1 polygon only P1 P1 back P6 front P3 P2 back front back P5 P2 P4 P3 P4 P6 P5 BSP Trees,contd · Traverse tree in order: 1. Start from root node 2. If view point in front: a) Draw polygons in back branch b) Then root polygon c) Then polygons in front branch 3. If view point in back: a) Draw polygons in front branch b) Then root polygon c) Then polygons in back branch 4. Recursively perform front/back test and traverse child nodes P1 P1 back P6 front P3 P2 back front back P5 P2 P4 P3 P4 P6 P5 Octrees · Consider splitting volume into 8 octants · Build tree containing polygons in each octant · If an octant has more than minimum number of polygons, split again 4 5 4 5 4 5 1 0 5 0 0 1 5 5 0 1 1 1 7 0 1 0 2 3 5 7 7 3 3 2 3 2 3 Octrees, contd · Best suited for orthographic projection · Display polygons in octant farthest from viewer first · Then those nodes that share a border with this octant · Recursively display neighboring octant · Last display closest octant Octree, example y 2 4 Viewpoint 8 11 6 12 114 12 14 9 6 10 13 1 5 3 5 x z Octants labeled in the order drawn given the viewpoint shown here. -
Hierarchical Bounding Volumes Grids Octrees BSP Trees Hierarchical
Spatial Data Structures HHiieerraarrcchhiiccaall BBoouunnddiinngg VVoolluummeess GGrriiddss OOccttrreeeess BBSSPP TTrreeeess 11/7/02 Speeding Up Computations • Ray Tracing – Spend a lot of time doing ray object intersection tests • Hidden Surface Removal – painters algorithm – Sorting polygons front to back • Collision between objects – Quickly determine if two objects collide Spatial data-structures n2 computations 3 Speeding Up Computations • Ray Tracing – Spend a lot of time doing ray object intersection tests • Hidden Surface Removal – painters algorithm – Sorting polygons front to back • Collision between objects – Quickly determine if two objects collide Spatial data-structures n2 computations 3 Speeding Up Computations • Ray Tracing – Spend a lot of time doing ray object intersection tests • Hidden Surface Removal – painters algorithm – Sorting polygons front to back • Collision between objects – Quickly determine if two objects collide Spatial data-structures n2 computations 3 Speeding Up Computations • Ray Tracing – Spend a lot of time doing ray object intersection tests • Hidden Surface Removal – painters algorithm – Sorting polygons front to back • Collision between objects – Quickly determine if two objects collide Spatial data-structures n2 computations 3 Speeding Up Computations • Ray Tracing – Spend a lot of time doing ray object intersection tests • Hidden Surface Removal – painters algorithm – Sorting polygons front to back • Collision between objects – Quickly determine if two objects collide Spatial data-structures n2 computations 3 Spatial Data Structures • We’ll look at – Hierarchical bounding volumes – Grids – Octrees – K-d trees and BSP trees • Good data structures can give speed up ray tracing by 10x or 100x 4 Bounding Volumes • Wrap things that are hard to check for intersection in things that are easy to check – Example: wrap a complicated polygonal mesh in a box – Ray can’t hit the real object unless it hits the box – Adds some overhead, but generally pays for itself. -
Chapter 2: the Game Core
Lecture Notes Managing and Mining Multiplayer Online Games Summer Term 2018 Chapter 2: The Game Core Script © 2012-2018 Matthias Schubert http://www.dbs.ifi.lmu.de/cms/VO_Managing_Massive_Multiplayer_Online_Games 1 Chapter Overview • Modelling the state of a game (Game State) • Modelling time (turn- and tick-system) • Handling actions • Interaction with other game components • Spatial management and distributing the game state 2 Internal Representation of games ID Type PosX PosY Health … 412 Knight 1023 2142 98 … 232 Soldier 1139 2035 20 … 245 Cleric 1200 2100 40 … … user view Game State Good Design: strict separation of data and display (Model-View-Controller Pattern) • MMO-Server: Managing the game state / no visualization necessary • MMO-Client: only parts of the game state but need for I/O and visualization. supports the implementation of various clients for the same game 3 Game State All data representing the current state of the game • object, attribute, relationship, … ( compare ER or UML models ) • models all alterable information • lists all game entities • contains all attributes of game entities • information concerning the whole game not necessarily in the Game State: • static information • environmental models/maps • preset attributes of game entities 4 Game Entities Game entities = objects in the game examples for game entities: • units in a RTS-Game • squares or figures in a board game • characters in a RPG • items • environmental objects (chests, doors, ...) 5 Attributes and Relationships Properties of a game entity that -
Spatial Partitioning and Functional Shape Matched Deformation
SPATIAL PARTITIONING AND FUNCTIONAL SHAPE MATCHED DEFORMATION ALGORITHM FOR INTERACTIVE HAPTIC MODELING A dissertation presented to the faculty of the Russ College of Engineering and Technology of Ohio University In partial fulfillment of the requirements for the degree Doctor of Philosophy Wei Ji November 2008 © 2008 Wei Ji. All Rights Reserved. 2 This dissertation titled SPATIAL PARTITIONING AND FUNCTIONAL SHAPE MATCHED DEFORMATION ALGORITHM FOR INTERACTIVE HAPTIC MODELING by WEI JI has been approved for the School of Electrical Engineering and Computer Science and the Russ College of Engineering and Technology by Robert L. Williams II Professor of Mechanical Engineering Dennis Irwin Dean, Russ College of Engineering and Technology 3 Abstract JI, WEI, Ph.D., November 2008, Computer Science SPATIAL PARTITIONING AND FUNCTIONAL SHAPE MATCHED DEFORMATION ALGORITHM FOR INTERACTIVE HAPTIC MODELING (161 pp.) Director of Dissertation: Robert L. Williams II This dissertation focuses on the fast rendering algorithms on real-time 3D modeling. To improve the computational efficiency, binary space partitioning (BSP) and octree are implemented on the haptic model. Their features are addressed in detail. Three methods of triangle assignment with the splitting plane are discussed. The solutions for optimizing collision detection (CD) are presented and compared. The complexities of these partition methods are discussed, and recommendation is made. Then the deformable haptic model is presented. In this research, for the haptic modeling, the deformation calculation is only related to the graphics process because the haptic rendering is invisible and non-deformable rendering saves much computation cost. To achieve the fast deformation computation in the interactive simulation, the functional shape matched deformation (FSMD) algorithm is proposed for the tangential and normal components of the surface deformation. -
Analyzing Performance of Bounding Volume
ANALYZING PERFORMANCE OF BOUNDING VOLUME HIERARCHIES FOR RAY TRACING by KEVIN W. BEICK A THESIS Presented to the Department of Computer and Information Science and the Robert D. Clark Honors College in partial fulfillment of the requirements for the degree of Bachelor of Arts December 2014 Acknowledgements I would like to thank Professor Childs for providing guidance and encouraging me to achieve my full potential as well as for being flexible and accommodating despite having many other duties throughout this process that started well over a year ago. I would also like to thank Matthew Larsen for providing me with some starter code, sharing his knowledge and directing me towards useful resources and Professor Hopkins for her willingness to serve as my CHC Representative on such short notice. iii Table of Contents Introduction 1 Computer Graphics 1 Rasterization 2 Ray Tracing 3 Bounding Volume Hierarchies 6 Related Works 9 Experiment Overview 14 BVH Variants 14 Test Cases 15 Analyzing Performance 16 Construction Algorithms 17 Results and Analysis 20 Summary and Conclusion 25 Data Tables 27 Primary Rays - Ray Trace Depth: 1, Opacity: 0.0 28 Secondary Rays - Ray Trace Depth: 2, Opacity: 0.0 29 Secondary Rays - Ray Trace Depth: 1, Opacity: 0.5 30 References 31 iv List of Figures Figure 1: A simple illustration of ray tracing’s intersection detection. 4 Figure 2: An example of a bounding volume hierarchy, using rectangles as bounding volumes. 7 Figure 3: A rendering of one of the five input geometries processed during the ray tracing tests. 15 Figure 4: A graph of BVH build times. -
Collision Detection Using Hierarchical Grid Spatial Partitioning on the GPU
Collision Detection Using Hierarchical Grid Spatial Partitioning on the GPU by Ryan R. Kroiss B.S., University of Wisconsin - Madison, 2009 A thesis submitted to the Faculty of the Graduate School of the University of Colorado in partial fulfillment of the requirements for the degree of Master of Science Department of Computer Science 2013 This thesis entitled: Collision Detection Using Hierarchical Grid Spatial Partitioning on the GPU written by Ryan R. Kroiss has been approved for the Department of Computer Science Prof. Willem Schre¨uder Prof. Ken Anderson Prof. Roger King Date The final copy of this thesis has been examined by the signatories, and we find that both the content and the form meet acceptable presentation standards of scholarly work in the above mentioned discipline. iii Kroiss, Ryan R. (M.S., Computer Science) Collision Detection Using Hierarchical Grid Spatial Partitioning on the GPU Thesis directed by Prof. Willem Schre¨uder Collision detection is a popular topic in computer graphics. Any physics-based application (e.g. surgical simulations, robotics, video games, computer animations, etc.) necessitates some degree of collision detection and handling. Many techniques exist for performing collision detection. Until recently, the majority of them rely on CPU-based approaches. The increasing accessibilty of general purpose GPU (GPGPU) programming has marshalled in new possibilities for computing collision detection in virtual environments. However, GPGPU programming is certainly not a silver bullet. There are a variety of challenges to recognize and overcome, in particular, as it relates to collision detection. This thesis will explore existing methods for performing collision detection and present a novel GPU-based approach to a common technique - hierarchical grid spatial partitioning. -
Ray Collection Bounding Volume Hierarchy
University of Central Florida STARS Electronic Theses and Dissertations, 2004-2019 2011 Ray Collection Bounding Volume Hierarchy Kris Krishna Rivera University of Central Florida Part of the Computer Sciences Commons, and the Engineering Commons Find similar works at: https://stars.library.ucf.edu/etd University of Central Florida Libraries http://library.ucf.edu This Masters Thesis (Open Access) is brought to you for free and open access by STARS. It has been accepted for inclusion in Electronic Theses and Dissertations, 2004-2019 by an authorized administrator of STARS. For more information, please contact [email protected]. STARS Citation Rivera, Kris Krishna, "Ray Collection Bounding Volume Hierarchy" (2011). Electronic Theses and Dissertations, 2004-2019. 1707. https://stars.library.ucf.edu/etd/1707 RAY COLLECTION BOUNDING VOLUME HIERARCHY by KRIS KRISHNA RIVERA B.S. University of Central Florida, 2007 A thesis submitted in partial fulfillment of the requirements for the degree of Master of Science in the Department of Electrical Engineering & Computer Science in the College of Engineering & Computer Science at the University of Central Florida Orlando, Florida Fall Term 2011 Major Professor: Sumanta Pattanaik ABSTRACT This thesis presents Ray Collection BVH, an improvement over a current day Ray Tracing acceleration structure to both build and perform the steps necessary to efficiently render dynamic scenes. Bounding Volume Hierarchy (BVH) is a commonly used acceleration structure, which aides in rendering complex scenes in 3D space using Ray Tracing by breaking the scene of triangles into a simple hierarchical structure. The algorithm this thesis explores was developed in an attempt at accelerating the process of both constructing this structure, and also using it to render these complex scenes more efficiently. -
A Tutorial on Binary Space Partitioning Trees
See discussions, stats, and author profiles for this publication at: https://www.researchgate.net/publication/238348725 A Tutorial on Binary Space Partitioning Trees Article · January 2005 CITATIONS READS 7 3,639 1 author: Bruce F. Naylor University of Texas at Austin 27 PUBLICATIONS 1,416 CITATIONS SEE PROFILE Some of the authors of this publication are also working on these related projects: Augmented Reality - model based tracking View project Use of machine learning to discern covert cognitive correlates View project All content following this page was uploaded by Bruce F. Naylor on 13 August 2015. The user has requested enhancement of the downloaded file. A Tutorial on Binary Space Partitioning Trees Bruce F. Naylor Spatial Labs Inc. I. Introduction In most applications involving computation with 3D geometric models, manipulating objects and generating images of objects are crucial operations. Performing these operations requires determining for every frame of an animation the spatial relations between objects: how they might intersect each other, and how they may occlude each other. However, the objects, rather than being monolithic, are most often comprised of many pieces, such as by many polygons forming the faces of polyhedra. The number of pieces may be any where from t h e 100's to the 1,000,000's. To compute spatial relations between n polygons by brute force entails comparing every pair of polygons, and so would require O(n2). For large scenes comprised of 105 polygons, this would mean 1010 operations, which is much more than necessary. The number of operations can be substantially reduced to anywhere from O(n log2 n) when the objects interpenetrate (and so in our example reduced to ~106), to as little a s constant time, O(1), when they are somewhat separated from each other.