CSE 167 (WI 2021) Homework 0 Homework 0 Is Here to Make Sure That You Can Compile and Run Modern Opengl (Required for Hws 1 and 2)
Total Page:16
File Type:pdf, Size:1020Kb
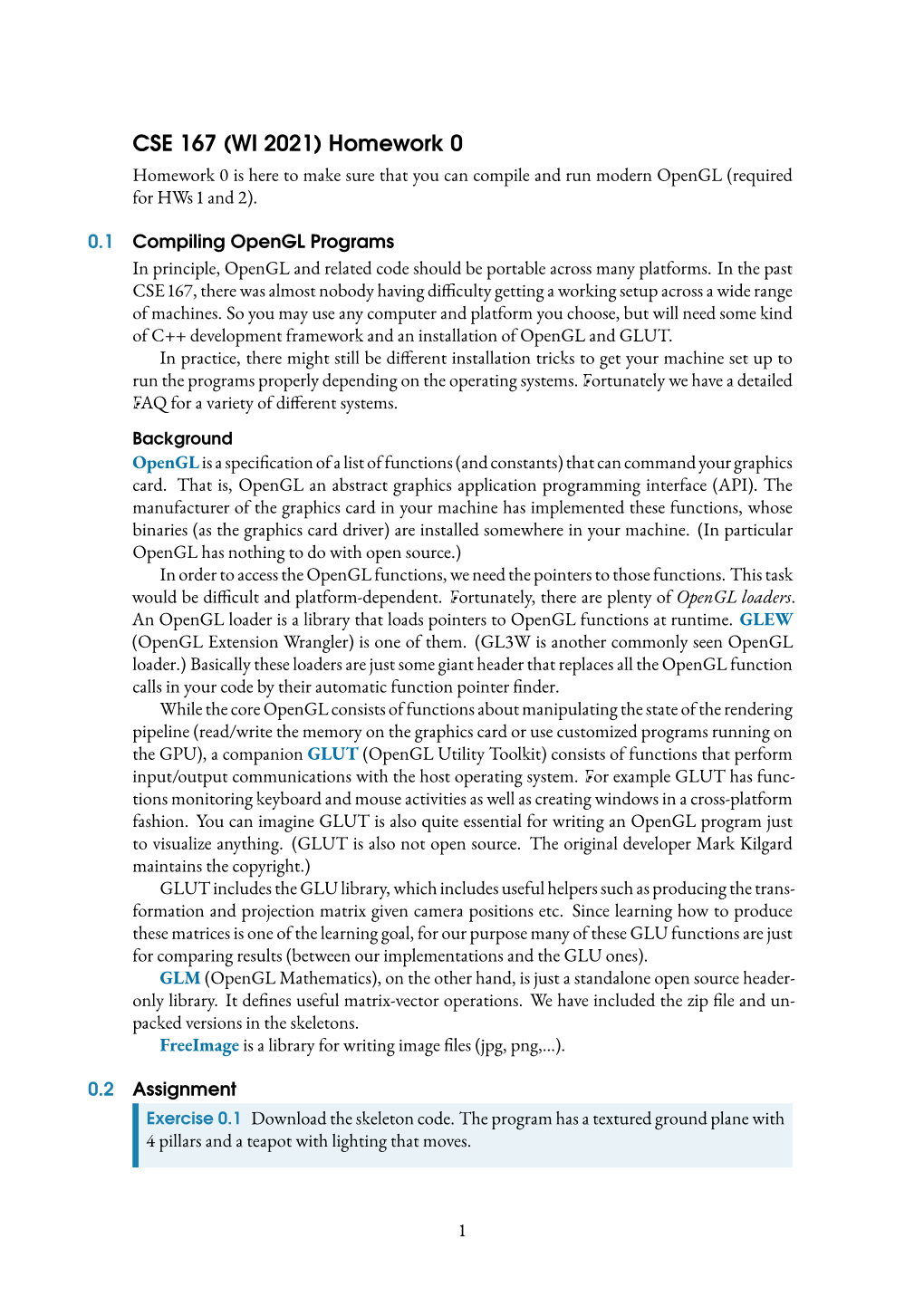
Load more
Recommended publications
-
Advanced Computer Graphics Spring 2011
CSCI 4830/7000 Advanced Computer Graphics Spring 2011 Instructor ● Willem A (Vlakkies) Schreüder ● Email: [email protected] – Begin subject with 4830 or 7000 – Resend email not answered promptly ● Office Hours: – Before and after Class – By appointment ● Weekday Contact Hours: 6:30am - 9:00pm Course Objectives ● Explore advanced topics in Computer Graphics – Pipeline Programming (Shaders) – Embedded System (OpenGL ES) – GPU Programming (OpenCL) – Ray Tracing – Special topics ● Particle systems ● Assignments: Practical OpenGL – Building useful applications Course Organization and Grading ● Class participation (50% grade) – First hour: Discussion/Show and tell ● Weekly homework assignments ● Volunteers and/or round robin – Second hour: Introduction of next topic ● Semester project (50% grade) – Build a significant application in OpenGL – 15 minute presentation last class periods ● No formal tests or final Assumptions ● You need to be fluent in C – Examples are in C (or simple C++) – You can do assignments in any language ● I may need help getting it to work on my system ● You need to be comfortable with OpenGL – CSCI 4229/5229 or equivalent – You need a working OpenGL environment Grading ● Satisfactory complete all assignments => A – The goal is to impress your friends ● Assignments must be submitted on time unless prior arrangements are made – Due by Thursday morning – Grace period until Thursday noon ● Assignments must be completed individually – Stealing ideas are encouraged – Code reuse with attribution is permitted ● Class attendance HIGHLY encouraged Code Reuse ● Code from the internet or class examples may be used – You take responsibility for any bugs in the code – Make the code your own ● Understand it ● Format it consistently – Improve upon what you found – Credit the source ● The assignment is a minimum requirement Text ● OpenGL Shading Language (3ed) – Randi J. -
Opengl Basics What Is Opengl?
CS312 OpenGL basics What is openGL? A low-level graphics library specification. A small set of geometric primitives: Points Lines Geometric primitives Polygons Images Image primitives Bitmaps OpenGL Libraries OpenGL core library OpenGL32 on Windows GL/Mesa on most unix/linux systems OpenGL Utility Library (GLU) Provides functionality in OpenGL core but avoids having to rewrite code GL is window system independent Extra libraries are needed to connect GL to the OS GLX – X windows, Unix AGL – Apple Macintosh WGL – Microsoft Windows GLUT OpenGL Utility Toolkit (GLUT) Provides functionality common to all window systems Open a window Get input from mouse and keyboard Menus Event-driven Code is portable but GLUT is minimal Software Organization application program OpenGL Motif widget or similar GLUT GLX, AGL or WGL GLU X, Win32, Mac O/S GL software and/or hardware OpenGL Architecture Immediate Mode Geometric pipeline Per Vertex Polynomial Operations & Evaluator Primitive Assembly Display Per Fragment Frame Rasterization CPU List Operations Buffer Texture Memory Pixel Operations OpenGL State OpenGL is a state machine OpenGL functions are of two types Primitive generating Can cause output if primitive is visible How vertices are processed and appearance of primitive are controlled by the state State changing Transformation functions Attribute functions Typical GL Program Structure Configure and open a window Initialize GL state Register callback functions Render Resize Events Enter infinite event processing loop Render/Display Draw simple geometric primitives Change states (how GL draws these primitives) How they are lit or colored How they are mapped from the user's two- or three-dimensional model space to the two- dimensional screen. -
IT Acronyms.Docx
List of computing and IT abbreviations /.—Slashdot 1GL—First-Generation Programming Language 1NF—First Normal Form 10B2—10BASE-2 10B5—10BASE-5 10B-F—10BASE-F 10B-FB—10BASE-FB 10B-FL—10BASE-FL 10B-FP—10BASE-FP 10B-T—10BASE-T 100B-FX—100BASE-FX 100B-T—100BASE-T 100B-TX—100BASE-TX 100BVG—100BASE-VG 286—Intel 80286 processor 2B1Q—2 Binary 1 Quaternary 2GL—Second-Generation Programming Language 2NF—Second Normal Form 3GL—Third-Generation Programming Language 3NF—Third Normal Form 386—Intel 80386 processor 1 486—Intel 80486 processor 4B5BLF—4 Byte 5 Byte Local Fiber 4GL—Fourth-Generation Programming Language 4NF—Fourth Normal Form 5GL—Fifth-Generation Programming Language 5NF—Fifth Normal Form 6NF—Sixth Normal Form 8B10BLF—8 Byte 10 Byte Local Fiber A AAT—Average Access Time AA—Anti-Aliasing AAA—Authentication Authorization, Accounting AABB—Axis Aligned Bounding Box AAC—Advanced Audio Coding AAL—ATM Adaptation Layer AALC—ATM Adaptation Layer Connection AARP—AppleTalk Address Resolution Protocol ABCL—Actor-Based Concurrent Language ABI—Application Binary Interface ABM—Asynchronous Balanced Mode ABR—Area Border Router ABR—Auto Baud-Rate detection ABR—Available Bitrate 2 ABR—Average Bitrate AC—Acoustic Coupler AC—Alternating Current ACD—Automatic Call Distributor ACE—Advanced Computing Environment ACF NCP—Advanced Communications Function—Network Control Program ACID—Atomicity Consistency Isolation Durability ACK—ACKnowledgement ACK—Amsterdam Compiler Kit ACL—Access Control List ACL—Active Current -
Introduction to the Software Libraries Opengl: Open Graphics Library GLU: Opengl Utility Library Glut: Opengl Utility Toolkit
Introduction to the software libraries This course makes use of several popular libraries to help build sophisticated portable graphics applications with minimal effort. The following diagram gives an overview of the packages and how they interact. For the purposes of this course, one can think of the GLU and glut libraries as being part of the OpenGL library or the OpenGL API (application programmer's interface), eventhough this is not really the case. -provides a software interface to graphics hardware and OpenGL implements most of the graphics functionality. provides support for some additional operations and primitive GLU types, and is implemented using OpenGL function calls designed specifically to be used with OpenGL and it takes care of things like opening windows, redraw events, and keyboard and glut mouse input. It effectively hides all the windowing system dependencies for OpenGL. OpenGL: Open Graphics Library • standardized 3D graphics library (API) • available on many platforms, often with hardware support • derivative of the Silicon Graphics' GL library • all function calls have the gl prefix, e.g.: glScale3fv() • OpenGL resources on the Internet** GLU: OpenGL Utility Library • support for NURBS surfaces, quadric surfaces, surface trimming, ... • all function calls have the glu prefix, e.g.: gluOrtho2D() • comes with all OpenGL implementations, including Windows and Mesa, no separate installation required • typically involves no hardware acceleration • glu32.dll on Win95/Win98/WinNT, look in same directory as Opengl32.dll -
Opengl API Introduction Agenda
OpenGL API Introduction Agenda • Structure of Implementation • Paths and Setup • API, Data Types & Function Name Conventions • GLUT • Buffers & Colours 2 OpenGL: An API, Not a Language • OpenGL is not a programming language; it is an application programming interface (API). • An OpenGL application is a program written in some programming language (such as C or C++) that makes calls to one or more of the OpenGL libraries. • Does not mean program uses OpenGL exclusively to do drawing: • It might combine the best features of two different graphics packages. • Or it might use OpenGL for only a few specific tasks and environment- specific graphics (such as the Windows GDI) for others. 3 Implementations • Although OpenGL is a “standard” programming library, this library has many implementations and versions. • On Microsoft Windows the implementation is in the opengl32.dll dynamic link library, located in the Windows system directory. • The OpenGL library is usually accompanied by the OpenGL utility library (GLU), which on Windows is in glu32.dll,. • GLU is a set of utility functions that perform common tasks, such as special matrix calculations, or provide support for common types of curves and surfaces. • On Mac OS X, OpenGL and the GLU libraries are both included in the OpenGL Framework. • The steps for setting up your compiler tools to use the correct OpenGL headers and to link to the correct OpenGL libraries vary from tool to tool and from platform to platform. 4 Include Paths • On all platforms, the prototypes for all OpenGL functions, types, and macros #include<windows.h> are contained (by convention) in the #include<gl/gl.h> header file gl.h. -
NVIDIA's Opengl Functionality
NVIDIANVIDIA ’’ss OpenGLOpenGL FunctionalityFunctionality Session 2127 | Room A5 | Monday, September, 20th, 16:00 - 17:20 San Jose Convention Center, San Jose, California Mark J. Kilgard • Principal System Software Engineer – OpenGL driver – Cg (“C for graphics”) shading language • OpenGL Utility Toolkit (GLUT) implementer • Author of OpenGL for the X Window System • Co-author of Cg Tutorial Outline • OpenGL’s importance to NVIDIA • OpenGL 3.3 and 4.0 • OpenGL 4.1 • Loose ends: deprecation, Cg, further extensions OpenGL Leverage Cg Parallel Nsight SceniX CompleX OptiX Example of Hybrid Rendering with OptiX OpenGL (Rasterization) OptiX (Ray tracing) Parallel Nsight Provides OpenGL Profiling Configure Application Trace Settings Parallel Nsight Provides OpenGL Profiling Magnified trace options shows specific OpenGL (and Cg) tracing options Parallel Nsight Provides OpenGL Profiling Parallel Nsight Provides OpenGL Profiling Trace of mix of OpenGL and CUDA shows glFinish & OpenGL draw calls OpenGL In Every NVIDIA Business OpenGL on Quadro – World class OpenGL 4 drivers – 18 years of uninterrupted API compatibility – Workstation application certifications – Workstation application profiles – Display list optimizations – Fast antialiased lines – Largest memory configurations: 6 gigabytes – GPU affinity – Enhanced interop with CUDA and multi-GPU OpenGL – Advanced multi-GPU rendering – Overlays – Genlock – Unified Back Buffer for less framebuffer memory usage – Cross-platform • Windows XP, Vista, Win7, Linux, Mac, FreeBSD, Solaris – SLI Mosaic – -
Opengl Programming Gregor Miller Gregor{At}Ece.Ubc.Ca
Gregor Miller gregor{at}ece.ubc.ca OpenGL Programming Background Conventions Simple Program SGI & GL • Silicon Graphics revolutionized graphics in 1982 by implementing the pipeline in hardware • System was accessed through API called GL • Much simpler than before to implement interactive 3D applications OpenGL • Success of GL led to OpenGL (1992), a platform-independent API that was: • Easy to use • Level of abstraction high enough for simple implementation but low enough to be close to hardware to get better performance • Focus on rendering, no device contexts or any OS specific dependencies OpenGL Review • Originally controlled by Architecture Review Board (ARB) • Extensions named after this • ARB replaced by Khronos • Current version 4.3, previous versions stable • OpenGL ES 1.0 and 2.0 (Embedded Systems) in iPhone and PS3 OpenGL Libraries • Core library • OpenGL32.lib on Windows • libGL.a on *nix systems • Utility Library (GLU) • Provides higher-level functionality using the Core • Camera setup, etc. • OS GUI Drivers • GLX, WGL, AGL (useful for extensions) GLUT • OpenGL Utility Toolkit (GLUT) • Provides OS-level functionality in a cross-platform API • Open a window, set up graphics context • Get input from mouse and keyboard • Menus • Event-driven (common to GUIs) • Code is portable but options are limited in scope OpenGL Functions • Primitives • Points • Lines • Triangles / Quads / Polygons • Attributes • Colour / Texture / Transparency OpenGL Functions • Transformations • Viewing • Modelling • Control (GLUT) • Input (GLUT) • Query -
Advanced Computer Graphics Spring 2013
CSCI 4239/5239 Advanced Computer Graphics Spring 2013 Instructor ● Willem A (Vlakkies) Schreüder ● Email: [email protected] – Begin subject with 4830 or 7000 – Resend email not answered promptly ● Office Hours: – ECST 121 Thursday 4-5pm – Other times by appointment ● Weekday Contact Hours: 6:30am - 9:00pm Course Objectives ● Explore advanced topics in Computer Graphics – Pipeline Programming (Shaders) – Embedded System (OpenGL ES) – GPU Programming (CUDA&OpenCL) – Ray Tracing – Special topics ● Particle systems ● Assignments: Practical OpenGL – Building useful applications Course Organization and Grading ● Class participation (50% grade) – First hour: Discussion/Show and tell ● Weekly homework assignments ● Volunteers and/or round robin – Second hour: Introduction of next topic ● Semester project (50% grade) – Build a significant application in OpenGL – 10 minute presentation last class periods ● No formal tests or final Assumptions ● You need to be fluent in C/C++ – Examples are in C or simple C++ – You can do assignments in any language ● I may need help getting it to work on my system ● You need to be comfortable with OpenGL – CSCI 4229/5229 or equivalent – You need a working OpenGL environment Grading ● Satisfactory complete all assignments => A – The goal is to impress your friends ● Assignments must be submitted on time unless prior arrangements are made – Due by Thursday morning – Grace period until Thursday noon ● Assignments must be completed individually – Stealing ideas are encouraged – Code reuse with attribution is permitted -
Introduction to Opengl and GLUT What’S Opengl?
Introduction to OpenGL and GLUT What’s OpenGL? • An Application Programming Interface (API) • A low‐level graphics programming API – Contains over 250 functions – Developed by Silicon Graphics Inc. (SGI) in 1992 – Most recent version: 4.2, released on 08/08/2011 OpenGL Applications And more… Portability • Independent of display devices • Independent of window systems • Independent of operating systems • Device 1: (100, 50) Line(100, 50, 150, 80) (100 • Device 2: (150, 80) MoveTo(100, 50) LineTo(150, 80) OpenGL Basics • The main use: Rendering. Rendering is the process of generating an image from a model (or models in what collectively could be called a scene file), by means of computer programs. -----Wikipedia • OpenGL can render: – Geometric primitives – Bitmaps and images An Example void Display() { glClear(GL_COLOR_BUFFER_BITS); glColor4f(1, 1, 0, 1); glBegin(GL_POLYGON); glVertex2f(-0.5, -0.5); glVertex2f(-0.5, 0.5); glVertex2f( 0.5, 0.5); glVertex2f( 0.5, -0.5); glEnd(); glutSwapBuffers(); } Primitives • Primitives are specified as: glBegin(primType); // your primitive vertices here. // ... // ... glEnd(); • PrimType: – GL_POINTS, GL_LINES, GL_TRIANGLES, … Primitive Types An Example void Display() { glClear(GL_COLOR_BUFFER_BITS); (‐0.5, 0.5) (0.5, 0.5) glColor4f(1, 1, 0, 1); glBegin(GL_POLYGON); glVertex2f(-0.5, -0.5); glVertex2f(-0.5, 0.5); glVertex2f( 0.5, 0.5); glVertex2f( 0.5, -0.5); glEnd(); glutSwapBuffers(); (‐0.5, ‐0.5) (0.5, ‐0.5) } Vertices v stands for vectors glVertex2fv(x, y) glVertex2f(x, y) Number of dimensions/components Format 2: (x, y) b: byte 3: (x, y, z) or (r, g, b) ub: unsigned byte 4: (x, y, z, w) or (r, g, b, a) i: int ui: unsigned int f: float d: double An Example void Draw_Circle() { glColor4f(0, 0, 0, 1); int number = 20; //number of vertices float radius = 0.8f; //radius float twoPi = 2.0f * 3.14159f; glBegin(GL_POLGYON); for(int i = 0; i <number; i++) glVertex2f(radius*cosf(i*twoPi/number), radius*sinf(i*twoPi/number)); glEnd(); } Window‐Based Programming • Most modern operating systems are windows‐ based. -
Vector Graphic Reference Implementation for Embedded System
Vector Graphic Reference Implementation for Embedded System Sang-Yun Lee1 and Byung-Uk Choi2 1 Dept. of Electronical Telecommunication Engineering, Hanyang University, Seoul, Korea [email protected] 2 Division of Information and Communications, Hanyang University, Seoul, Korea [email protected] Abstract. We propose the reference implementation with software rendering of OpenVG for the scalable vector graphic hardware acceleration, which the Khronos group standardizes. We present the design scheme that enables EGL and OpenVG to be ported easily in an embedded environment. Moreover, we de- scribe the background of selection of an algorithm, and the mathematical func- tion adopted for the performance improvement, and we propose the optimum rendering method. We present displaying of vector image on a screen through the OpenVG implemented using software rendering method. And, we present the test result of the CTS which is compatibility test tool. And we show the perform- ance comparison against the Hybrid corp.'s reference implementation. Keywords: OpenVG, EGL, Scalable Vector Graphic, Embedded System, Soft- ware Rendering. 1 Introduction Recently, the demand for the applications using the vector graphics technology has increased [1]. Particularly, in areas such as SVG viewer, hand-held guidance service, E-Book reader, game, scalable user interface, and etc, the vector graphics technology is widely applied. OpenVG™ is a royalty-free, cross-platform API that provides a low-level hardware acceleration interface for vector graphics libraries such as Flash and SVG [2]. Currently in development, OpenVG is targeted primarily at handheld devices that require portable acceleration of high-quality vector graphics for compelling user inter- faces and text on small screen devices while enabling hardware acceleration to pro- vide fluidly interactive performance at very low power levels. -
Opengl Quick Start
A Graphics System • Application program – Puts all subsequent layers together into a piece of software that is of direct use to some human being • Framework – Successive layers over a core graphics engine to make it easier to use – User interfaces, image processing libraries, 3D scene specifiers live here • Graphics engine – Software specific to graphics rendering: pipelines, special algorithms (such as full-screen anti-aliasing) • Operating system – Manages the context of graphics applications: windows, full-screen mode, drag-and-drop – If properly done, is a cleanly separate layer from core operating system functions: processes, memory management, file systems, I/O • Device driver – Software specific to the video hardware – Interfaces with the operating system in a standard way – May also have hooks to a graphics framework (i.e. OpenGL) • Video hardware – Video memory, registers – Coprocessors, special functions – Interface to output devices • Input devices – Allows the user to affect software: physical activity such as movement travels through the layers of a system until something figures out what to do with it An OpenGL Graphics System • Application program – Written in C, C++, Java, or any other programming language for which OpenGL can be “exposed” • Framework – GLUT: OpenGL Utility Toolkit — platform-independent windowing framework specifically for OpenGL software, available on multiple platforms…results in portable OpenGL software – Platform-specific frameworks (xgl, wgl, agl) — binds OpenGL to APIs that are specific to an operating -
NVIDIA GPUS Mark Kilgard Principal System Software Engineer, NVIDIA
SG4121: OPENGL 4.5 UPDATE FOR NVIDIA GPUS Mark Kilgard Principal System Software Engineer, NVIDIA Piers Daniell Senior Graphics Software Engineer, NVIDIA Mark Kilgard • Principal System Software Engineer – OpenGL driver and API evolution – Cg (“C for graphics”) shading language – GPU-accelerated path rendering • OpenGL Utility Toolkit (GLUT) implementer • Author of OpenGL for the X Window System • Co-author of Cg Tutorial • Worked on OpenGL for 20+ years Piers Daniell • Senior Graphics Software Engineer • NVIDIA’s Khronos OpenGL representative – Since 2010 – Authored numerous OpenGL extension specifications now core • Leads OpenGL version updates – Since OpenGL 4.1 • 10+ years with NVIDIA NVIDIA’s OpenGL Leverage GeForce Programmable Graphics Tegra Debugging with Nsight Quadro OptiX Adobe Creative Cloud Single 3D API for Every Platform Windows OS X Linux Android Solaris FreeBSD Adobe Creative Cloud: GPU-accelerated Illustrator • 27 year old application – World’s leading graphics design application • 6 million users – Never used the GPU • Until this June 2014 • Adobe and NVIDIA worked to integrate NV_path_rendering into Illustrator CC 2014 OpenGL 4.x Evolution 2010 2011 2012 2013 2014 ??? OpenGL 4.4: Persistently mapped buffers, multi bind OpenGL 4.3: Compute shaders, SSBO, ES3 compatibility OpenGL 4.2: GLSL upgrades and shader image load store OpenGL 4.1: Shader mix-and-match, ES2 compatibility OpenGL 4.0: Tessellation . Major revision of OpenGL every year since OpenGL 3.0, 2008 . Maintained full backwards compatibility Big News: OpenGL 4.5 Released Today! . Direct State Access (DSA) finally! . Robustness . OpenGL ES 3.1 compatibility . Faster MakeCurrent . DirectX 11 features for porting and emulation . SubImage variant of GetTexImage .