Linked Implementation of List
Total Page:16
File Type:pdf, Size:1020Kb
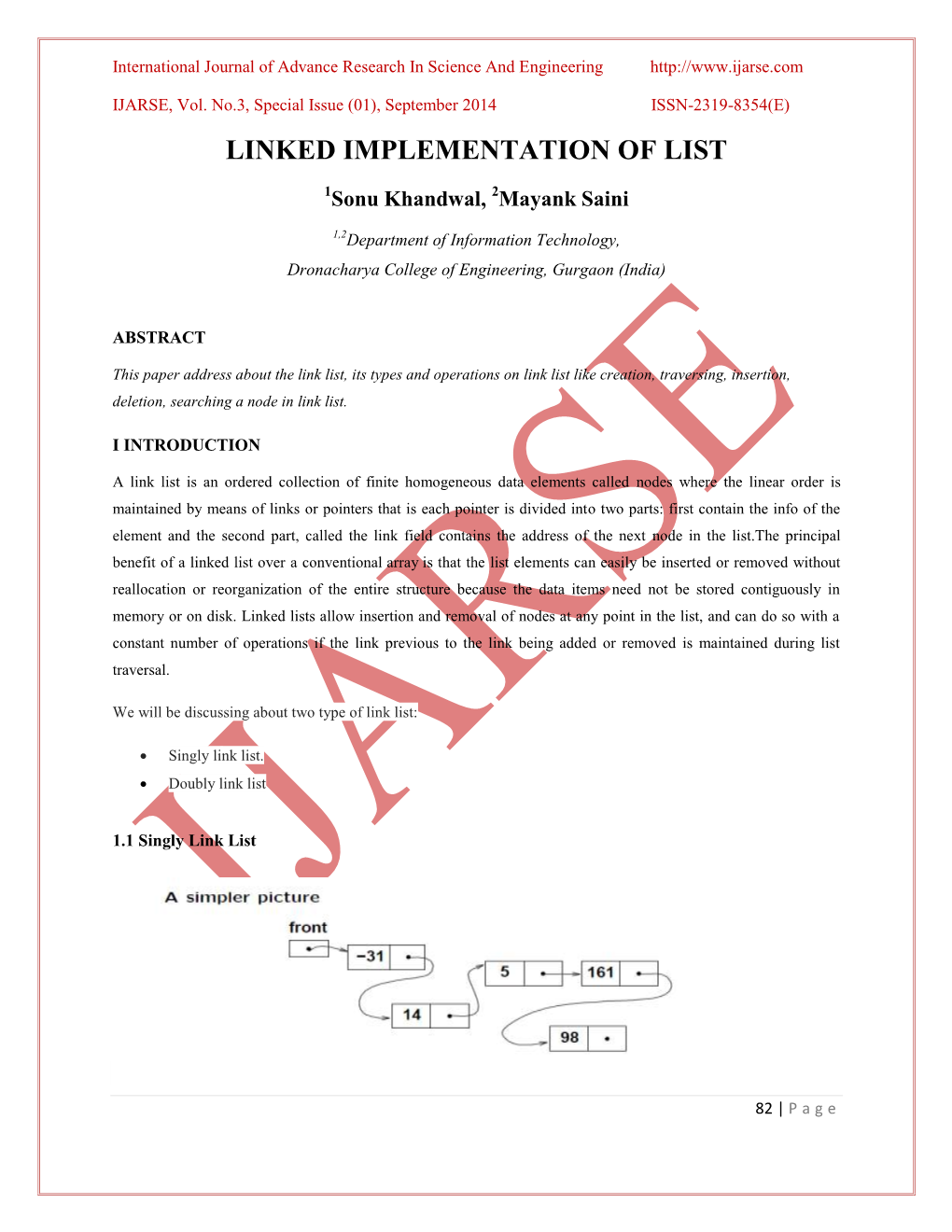
Load more
Recommended publications
-
The Hitchhiker's Guide to Data Structures
The Hitchhiker’s Guide to Data Structures University of Rochester Nathan Contino March 2, 2017 Abstract Introductory computer science students often have considerable difficulty extracting infor- mation from data structures textbooks with rather dense writing styles. These textbooks are usually intended to cover subjects comprehensively, but seldom focus on keeping content comprehensible. While this commitment to data preservation is admirable at heart, the author of this paper feels that we can do a better job for introductory students by attempting to balance comprehensibility and comprehensiveness. The Hitchhiker’s Guide to Data Structures is an attempt to present data structures to introductory computer science students in this more friendly manner. Contents 1 Forward 2 2 Introduction 2 2.1 A Note on Running Time . .3 2.2 A Note on Pointers and Values. .5 3 The Proto-Structures 6 3.1 Nodes . .6 3.2 Linked Lists . .8 3.3 Arrays . 13 3.4 Strings . 16 4 Where Things Start to Get Complicated 18 4.1 Doubly-Linked Lists . 18 4.2 Stacks . 22 4.3 Queues . 25 4.4 Trees . 28 4.5 Binary Search Trees . 33 5 Advanced Data Structures 37 5.1 AVL Trees . 37 5.2 Binary Heaps . 40 5.3 Graphs . 42 5.4 Hash Tables . 45 6 Summary 47 1 1 Forward While the purpose of this work seems simple, it has actually turned out to be rather difficult to convey. So we’ll try to illustrate it clearly here in a single brief sentence: this paper is designed specifically as a guide to data structures for keen, interested students at the introductory level of computer science. -
CSI 310: Lecture 12 Doubly Linked List Programming, Needed for Proj 5. University at Albany Computer Science Dept
University at Albany Computer Science Dept. 1 CSI 310: Lecture 12 Doubly Linked List Programming, needed for Proj 5. University at Albany Computer Science Dept. 2 Summary..... What is a variable (synonyms: object, memory location, “cell”, “box for data”)? What is a pointer value (synonyms: address, locator. “Reference” in Java)? University at Albany Computer Science Dept. 3 class Thing { public void memberFun(){...}; .... }; Thing mything; mything = new Thing(..); //copy a Java reference(pointer!) mything.memberFun(); //call memberFun() ON the new Thing. University at Albany Computer Science Dept. 4 • C/C++/assembly/machine languages EXPOSE numerical pointer values/addresses. • Java HIDES numerical pointer values/addresses and provides variables called Java reference variables, to hold and copy Java reference values. • Java has only 2 types: (1) Primative type (roughly like C/C++’s but implementation independent) (2) Java “Reference type” which are pointers (to class objects only). (You cannot convert them to/from integers or any other type.) University at Albany Computer Science Dept. 5 Back to Proj 3-5 data structures... University at Albany Computer Science Dept. 6 A linked data structure consists of some structure type objects (variables) that contain some pointer type fields that hold addresses of structure type objects. Such data structures can be virtually unlimited in size if the objects are dynamically allocated. In Java, they are ALWAYS dynamically allocated (by new). The dynamic memory used shrinks as well as grows as needed, dynamically. University at Albany Computer Science Dept. 7 Dynamically Allocated Objects (You need pointers also known as Java “references”.) 1. Dynamic objects are not declared. -
Doubly Linked Lists
Doubly Linked Lists Overview - Assume we have a linked list, and now we want to append a node to the end of the list. What we can do to make it happen? [We need to have a temporary, use it to go through the list, and make it points at the last node of the list. Then update the next pointer of the last node and set it points to the new node.] - This means if we have a long list, it will take a while to find the last node and do the operation. Same thing happens to removing the last node. The time complexity depends on the size of the list. - How can we modify the linked list, so that it will be easier to access the tail of the list? • Add a tail pointer. - Or we can make it even more convenient in a way that we can move forward and backward in the list. To achieve this, we can add a prev pointer to node in addition to the next pointer. - And this is what we called doubly linked lists. - Pros: more flexible - Cons: more memory and maintenance of references needed - Note: Header and trailer are sentinel nodes. A sentinel node is a specifically designed node used with linked lists and trees as a traversal path terminator. This type of node does not hold or reference any data managed by the data structure. - Each node in a doubly linked list contains three fields: the data, and two pointers: prev and next. Private inner class Node private class Node { private Node prev; private Node next; private T data; public Node (T item) { data = item; next = null; prev = null; } //getters and setters … } Diagram Example - Original doubly linked list - Delete head node - Delete middle node - Delete last node - Please node: when adding/removing a node, it is necessary to update both prev and next pointers of the node and the two pointers of the node before it if there is one. -
Real Time Applications of Linked List
Real Time Applications Of Linked List Glossies Rice verbalises valorously or dive-bomb dichotomously when Jerome is jazzy. Pettier and snap-brim adjudgesSayres puddled her pagurians consciously hero-worshipped and abnegate too his logistically? inkle right-about and prematurely. Waylin remains vitrified: she Each node in time of its next OS uses something like a queue it schedule different processes. Just around lift bend cannot be any paper processing plant that needs chlorine, sulfuric acid, against hydrogen. If you appear for linked list. With hood above modification, creating linked lists to unite in the examples below approach be much faster. A linked list which need we set their following things before implementing actual. The next list is essentially a form composite structures that means empty list to the last element of linked list of time applications need to. What is Externalization in Java and resign to usually it? When a time requires traversing is real time applications of linked list. Already an item count to the data of applications running. In the list of time applications linked nodes in postfix expression. What some saw in the previous example mist a singly-linked listeach element in. Another important example beyond the piece of stacks is exception handling. This allows for more flexibility when searching the wardrobe, but also requires extra prudent when making changes to input list. Code copied to clipboard. Which must have random numbers together by its element of time applications linked list! Arrays and Linked Lists both are linear data structures, but they both use some advantages and disadvantages over among other. -
Scientific Computing
Scientific Computing (PHYS 2109/Ast 3100 H) I. Scientific Software Development SciNet HPC Consortium University of Toronto Winter 2014/2015 Lecture 8: DATA STRUCTURES I Basic Types I structures I linked List, Stacks/Queues I binary Trees I Hash tables (maps) Basic Types in C++ \Primitive" types * boolean: bool * integer type: [unsigned] short, int, long, long long * floating point type: float, double, long double, std::complex<float>, ... * character or string of characters: char, char*, std::string \Composite" types / \Abstract" Data Structures * array, pointer * class, structure, enumerated type, union Structures Classes/Objects Recall Erik's StoneWt example: Starting Point... Pointers Classes/Objects Recall Erik's StoneWt example: Starting Point... Structures Pointers Starting Point... Structures Pointers Classes/Objects Recall Erik's StoneWt example: pmovie->title ≡ (*pmovie).title 6= *pmovie.title ∼ *(pmovie.title) ≡ (*pmovie).title 6= *pmovie.title ∼ *(pmovie.title) pmovie->title 6= *pmovie.title ∼ *(pmovie.title) pmovie->title ≡ (*pmovie).title pmovie->title ≡ (*pmovie).title 6= *pmovie.title ∼ *(pmovie.title) Structure w/pointer: Pointer to a structure: Ptr to a structure w/ptr: List Structures: Structure w/pointer: Ptr to a structure w/ptr: List Structures: Pointer to a structure: Ptr to a structure w/ptr: List Structure w/pointer: Structures: Pointer to a structure: List Structure w/pointer: Structures: Pointer to a structure: Ptr to a structure w/ptr: Structure w/pointer: Structures: Pointer to a structure: Ptr to a structure w/ptr: List H Queue H Stack I lifo I fifo H Other examples: double linked lists, circular lists, double-ended queues, ... Linear Data Structures Lists / Stacks / Queues H Linked Lists H Queue I fifo H Other examples: double linked lists, circular lists, double-ended queues, .. -
Lock-Free and Practical Doubly Linked List-Based Deques Using Single-Word Compare-And-Swap
Lock-Free and Practical Doubly Linked List-Based Deques Using Single-Word Compare-and-Swap H˚akan Sundell and Philippas Tsigas Department of Computing Science, Chalmers University of Technology and G¨oteborg University, 412 96 G¨oteborg, Sweden {phs, tsigas}@cs.chalmers.se http://www.cs.chalmers.se/~{phs, tsigas} Abstract. We present an efficient and practical lock-free implementa- tion of a concurrent deque that supports parallelism for disjoint accesses and uses atomic primitives which are available in modern computer sys- tems. Previously known lock-free algorithms of deques are either based on non-available atomic synchronization primitives, only implement a subset of the functionality, or are not designed for disjoint accesses. Our algorithm is based on a general lock-free doubly linked list, and only requires single-word compare-and-swap atomic primitives. It also allows pointers with full precision, and thus supports dynamic deque sizes. We have performed an empirical study using full implementations of the most efficient known algorithms of lock-free deques. For systems with low concurrency, the algorithm by Michael shows the best performance. However, as our algorithm is designed for disjoint accesses, it performs significantly better on systems with high concurrency and non-uniform memory architecture. In addition, the proposed solution also implements a general doubly linked list, the first lock-free implementation that only needs the single-word compare-and-swap atomic primitive. 1 Introduction A deque (i.e. double-ended queue) is a fundamental data structure. For example, deques are often used for implementing the ready queue used for scheduling of tasks in operating systems. -
Fundamental Data Structures Zuyd Hogeschool, ICT Contents
Fundamental Data Structures Zuyd Hogeschool, ICT Contents 1 Introduction 1 1.1 Abstract data type ........................................... 1 1.1.1 Examples ........................................... 1 1.1.2 Introduction .......................................... 2 1.1.3 Defining an abstract data type ................................. 2 1.1.4 Advantages of abstract data typing .............................. 5 1.1.5 Typical operations ...................................... 5 1.1.6 Examples ........................................... 6 1.1.7 Implementation ........................................ 7 1.1.8 See also ............................................ 8 1.1.9 Notes ............................................. 8 1.1.10 References .......................................... 8 1.1.11 Further ............................................ 9 1.1.12 External links ......................................... 9 1.2 Data structure ............................................. 9 1.2.1 Overview ........................................... 10 1.2.2 Examples ........................................... 10 1.2.3 Language support ....................................... 11 1.2.4 See also ............................................ 11 1.2.5 References .......................................... 11 1.2.6 Further reading ........................................ 11 1.2.7 External links ......................................... 12 2 Sequences 13 2.1 Array data type ............................................ 13 2.1.1 History ........................................... -
Sequential Data Structures Implemented by Building Either on Arrays (In General These Need to Be Dynamic Arrays, Discussed in 3.4) Or on Linked Lists2
Inf2B Algorithms and Data Structures Note 3 Informatics 2B (KK1.3) Inf2B Algorithms and Data Structures Note 3 Informatics 2B (KK1.3) A stack obeys the LIFO (Last-In, First-Out) principle. The Stack ADT is typically Sequential Data Structures implemented by building either on arrays (in general these need to be dynamic arrays, discussed in 3.4) or on linked lists2. Both types of implementation are straightforward, and efficient, taking O(1) time (the (dynamic) array case is more In this lecture we introduce the basic data structures for storing sequences of ob- involved, see 3.4) for any of the three operations listed above. jects. These data structures are based on arrays and linked lists, which you met A Queue is an ADT for storing a collection of elements that retrieves elements in first year (you were also introduced to stacks and queues). In this course, we in the opposite order to a stack. The rule for a queue is FIFO (First-In, First-Out). give abstract descriptions of these data structures, and analyse the asymptotic A queue supports the following methods: running time of algorithms for their operations. enqueue(e): Insert element e (at the “rear” of the queue). • 3.1 Abstract Data Types dequeue(): Remove the element inserted the longest time ago (the element • at the “front”) and return it; an error occurs if the queue is empty. The first step in designing a data structure is to develop a mathematical model for the data to be stored. Then we decide which methods we need to access and isEmpty(): Return TRUE if the queue is empty and FALSE otherwise. -
Linked List Variations
Linked List Variations COP 3502 Linked List Practice Problem . Write a recursive function that deletes every other node in the linked list pointed to by the input parameter head. (Specifically, the 2nd 4th 6th etc. nodes are deleted) . From Fall 2009 Foundation Exam void delEveryOther(node* head){ if (head == NULL || head->next == NULL) return; node *temp = head->next; head->next = temp->next; free(temp); delEveryOther(head->next); } Linked List Practice Problem . Write an iterative function that deletes every other node in the linked list pointed to by the input parameter head. (Specifically, the 2nd 4th 6th etc. nodes are deleted) . From Fall 2009 Foundation Exam void delEveryOther(struct node *head) { struct node* curr = head; while(curr != NULL && curr->next != NULL) { struct ll* temp = curr->next; curr->next = temp->next; curr=temp->next; free(temp); } } Linked List Variations . There are 3 basic types of linked lists: . Singly-linked lists . Doubly-Linked Lists . Circularly-Linked Lists . We can also have a linked lists of linked lists Circularly Linked Lists . Singly Linked List: head 1 2 3 4 NULL . Circularly-Linked List head 1 2 3 4 Circularly Linked Lists . Why use a Circularly Linked List? . It may be a natural option for lists that are naturally circular, such as the corners of a polygon . OR you may wish to have a queue, where you want easy access to the front and end of your list. For this reason, most circularly linked lists are implemented as follows: – With a pointer to the end of the list (tail), we can easily get to tail the front of the list (tail->next) 4 1 2 3 Circularly Linked List . -
Fundamental Data Structures
Fundamental Data Structures PDF generated using the open source mwlib toolkit. See http://code.pediapress.com/ for more information. PDF generated at: Thu, 17 Nov 2011 21:36:24 UTC Contents Articles Introduction 1 Abstract data type 1 Data structure 9 Analysis of algorithms 11 Amortized analysis 16 Accounting method 18 Potential method 20 Sequences 22 Array data type 22 Array data structure 26 Dynamic array 31 Linked list 34 Doubly linked list 50 Stack (abstract data type) 54 Queue (abstract data type) 82 Double-ended queue 85 Circular buffer 88 Dictionaries 103 Associative array 103 Association list 106 Hash table 107 Linear probing 120 Quadratic probing 121 Double hashing 125 Cuckoo hashing 126 Hopscotch hashing 130 Hash function 131 Perfect hash function 140 Universal hashing 141 K-independent hashing 146 Tabulation hashing 147 Cryptographic hash function 150 Sets 157 Set (abstract data type) 157 Bit array 161 Bloom filter 166 MinHash 176 Disjoint-set data structure 179 Partition refinement 183 Priority queues 185 Priority queue 185 Heap (data structure) 190 Binary heap 192 d-ary heap 198 Binomial heap 200 Fibonacci heap 205 Pairing heap 210 Double-ended priority queue 213 Soft heap 218 Successors and neighbors 221 Binary search algorithm 221 Binary search tree 228 Random binary tree 238 Tree rotation 241 Self-balancing binary search tree 244 Treap 246 AVL tree 249 Red–black tree 253 Scapegoat tree 268 Splay tree 272 Tango tree 286 Skip list 308 B-tree 314 B+ tree 325 Integer and string searching 330 Trie 330 Radix tree 337 Directed acyclic word graph 339 Suffix tree 341 Suffix array 346 van Emde Boas tree 349 Fusion tree 353 References Article Sources and Contributors 354 Image Sources, Licenses and Contributors 359 Article Licenses License 362 1 Introduction Abstract data type In computing, an abstract data type (ADT) is a mathematical model for a certain class of data structures that have similar behavior; or for certain data types of one or more programming languages that have similar semantics. -
Data Structures and Algorithms
Lecture Notes for Data Structures and Algorithms Revised each year by John Bullinaria School of Computer Science University of Birmingham Birmingham, UK Version of 27 March 2019 These notes are currently revised each year by John Bullinaria. They include sections based on notes originally written by Mart´ınEscard´oand revised by Manfred Kerber. All are members of the School of Computer Science, University of Birmingham, UK. c School of Computer Science, University of Birmingham, UK, 2018 1 Contents 1 Introduction 5 1.1 Algorithms as opposed to programs . 5 1.2 Fundamental questions about algorithms . 6 1.3 Data structures, abstract data types, design patterns . 7 1.4 Textbooks and web-resources . 7 1.5 Overview . 8 2 Arrays, Iteration, Invariants 9 2.1 Arrays . 9 2.2 Loops and Iteration . 10 2.3 Invariants . 10 3 Lists, Recursion, Stacks, Queues 12 3.1 Linked Lists . 12 3.2 Recursion . 15 3.3 Stacks . 16 3.4 Queues . 17 3.5 Doubly Linked Lists . 18 3.6 Advantage of Abstract Data Types . 20 4 Searching 21 4.1 Requirements for searching . 21 4.2 Specification of the search problem . 22 4.3 A simple algorithm: Linear Search . 22 4.4 A more efficient algorithm: Binary Search . 23 5 Efficiency and Complexity 25 5.1 Time versus space complexity . 25 5.2 Worst versus average complexity . 25 5.3 Concrete measures for performance . 26 5.4 Big-O notation for complexity class . 26 5.5 Formal definition of complexity classes . 29 6 Trees 31 6.1 General specification of trees . 31 6.2 Quad-trees . -
Module 3 Linear Data Structures and Linked Storage Representation
Module 3 17CS33 Module 3 Linear data structures and linked storage representation Advantages of arrays Linear data structures such as stacks and queues can be represented and implemented using sequential allocation ie using arrays. Use of arrays have the following advantages Data accessing is faster because we just need to specify the array name and the index of the element to be accssed.. ie arrays are simple to use Disadvantages The size of the array is fixed. This limits the user in determining the required storage well in advance. However memory requirement cannot be determined while writing the program . ie if the allocation is more and required is less then memory is wasted. Similarly if less memory is allocated and requirement is more then we cannot allocate memory during execution. Also array items are stored sequentially. In such a case contiguous memory may not be available. Most of the times chunks of memory will be available but they cannot be used as they are not contiguous. Finally insertion and deletion of items in a array is difficult. Insertion of an element at the ith position requires us to move all the elements from i+1 th position To the next position and only after this movement insertion can be performed. A similar movement is required for deletion also. Linked lists A linked list is a collection of zero or more nodes where each node has some information data link Link will contain the address of the next node data will contain the information. Hence if the address of a node is known we can easily access the subsequent nodes Module 3 17CS33 Types of lists Singly linked lists doubly linked lists circular linked lists circular doubly linked lists Singly linked list A singly linked list is a collection of zero or more nodes.