Evaluating the Effectiveness of Pointer Alias Analyses
Total Page:16
File Type:pdf, Size:1020Kb
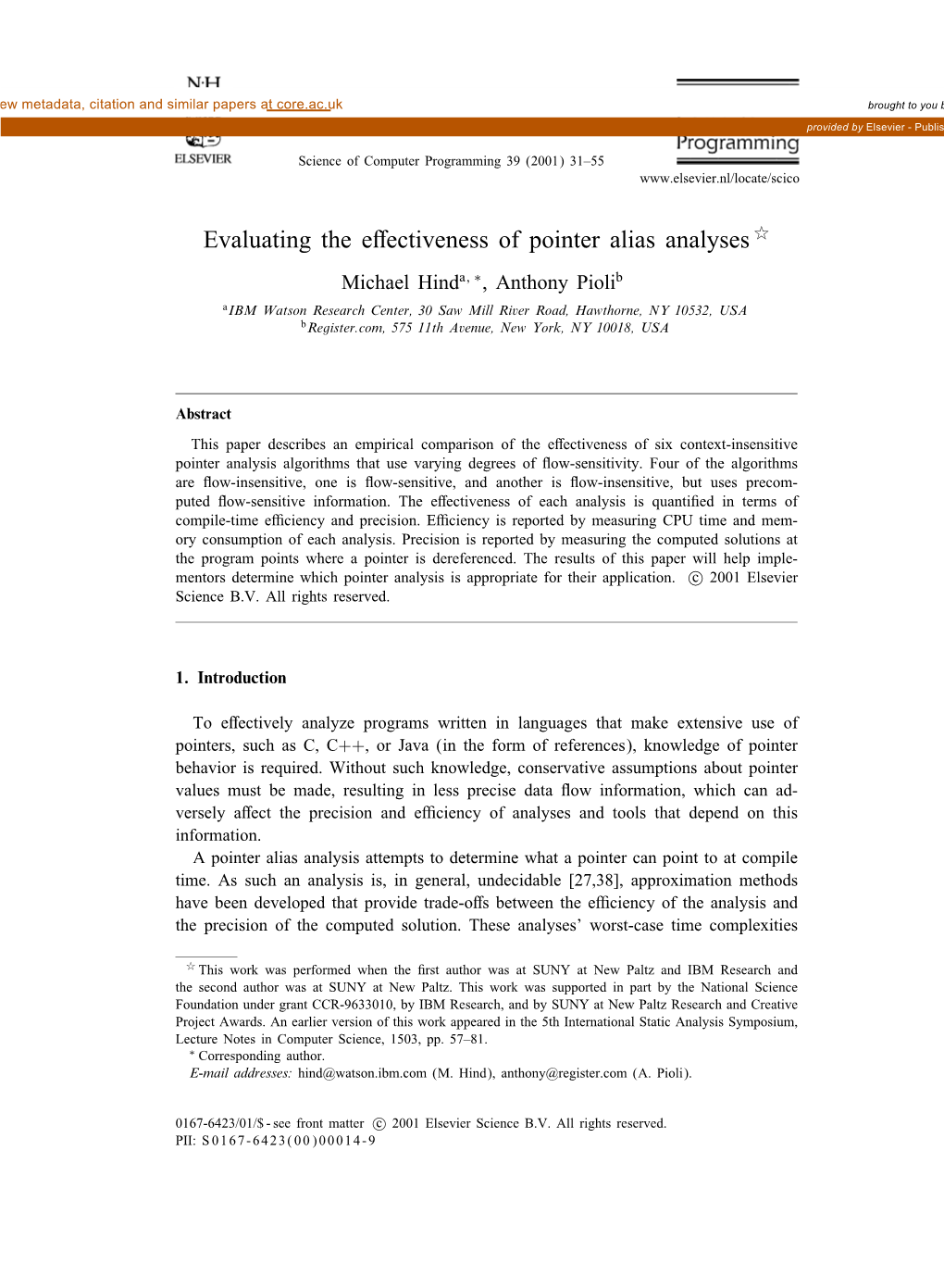
Load more
Recommended publications
-
Using the GNU Compiler Collection (GCC)
Using the GNU Compiler Collection (GCC) Using the GNU Compiler Collection by Richard M. Stallman and the GCC Developer Community Last updated 23 May 2004 for GCC 3.4.6 For GCC Version 3.4.6 Published by: GNU Press Website: www.gnupress.org a division of the General: [email protected] Free Software Foundation Orders: [email protected] 59 Temple Place Suite 330 Tel 617-542-5942 Boston, MA 02111-1307 USA Fax 617-542-2652 Last printed October 2003 for GCC 3.3.1. Printed copies are available for $45 each. Copyright c 1988, 1989, 1992, 1993, 1994, 1995, 1996, 1997, 1998, 1999, 2000, 2001, 2002, 2003, 2004 Free Software Foundation, Inc. Permission is granted to copy, distribute and/or modify this document under the terms of the GNU Free Documentation License, Version 1.2 or any later version published by the Free Software Foundation; with the Invariant Sections being \GNU General Public License" and \Funding Free Software", the Front-Cover texts being (a) (see below), and with the Back-Cover Texts being (b) (see below). A copy of the license is included in the section entitled \GNU Free Documentation License". (a) The FSF's Front-Cover Text is: A GNU Manual (b) The FSF's Back-Cover Text is: You have freedom to copy and modify this GNU Manual, like GNU software. Copies published by the Free Software Foundation raise funds for GNU development. i Short Contents Introduction ...................................... 1 1 Programming Languages Supported by GCC ............ 3 2 Language Standards Supported by GCC ............... 5 3 GCC Command Options ......................... -
Memory Tagging and How It Improves C/C++ Memory Safety Kostya Serebryany, Evgenii Stepanov, Aleksey Shlyapnikov, Vlad Tsyrklevich, Dmitry Vyukov Google February 2018
Memory Tagging and how it improves C/C++ memory safety Kostya Serebryany, Evgenii Stepanov, Aleksey Shlyapnikov, Vlad Tsyrklevich, Dmitry Vyukov Google February 2018 Introduction 2 Memory Safety in C/C++ 2 AddressSanitizer 2 Memory Tagging 3 SPARC ADI 4 AArch64 HWASAN 4 Compiler And Run-time Support 5 Overhead 5 RAM 5 CPU 6 Code Size 8 Usage Modes 8 Testing 9 Always-on Bug Detection In Production 9 Sampling In Production 10 Security Hardening 11 Strengths 11 Weaknesses 12 Legacy Code 12 Kernel 12 Uninitialized Memory 13 Possible Improvements 13 Precision Of Buffer Overflow Detection 13 Probability Of Bug Detection 14 Conclusion 14 Introduction Memory safety in C and C++ remains largely unresolved. A technique usually called “memory tagging” may dramatically improve the situation if implemented in hardware with reasonable overhead. This paper describes two existing implementations of memory tagging: one is the full hardware implementation in SPARC; the other is a partially hardware-assisted compiler-based tool for AArch64. We describe the basic idea, evaluate the two implementations, and explain how they improve memory safety. This paper is intended to initiate a wider discussion of memory tagging and to motivate the CPU and OS vendors to add support for it in the near future. Memory Safety in C/C++ C and C++ are well known for their performance and flexibility, but perhaps even more for their extreme memory unsafety. This year we are celebrating the 30th anniversary of the Morris Worm, one of the first known exploitations of a memory safety bug, and the problem is still not solved. -
Statically Detecting Likely Buffer Overflow Vulnerabilities
Statically Detecting Likely Buffer Overflow Vulnerabilities David Larochelle [email protected] University of Virginia, Department of Computer Science David Evans [email protected] University of Virginia, Department of Computer Science Abstract Buffer overflow attacks may be today’s single most important security threat. This paper presents a new approach to mitigating buffer overflow vulnerabilities by detecting likely vulnerabilities through an analysis of the program source code. Our approach exploits information provided in semantic comments and uses lightweight and efficient static analyses. This paper describes an implementation of our approach that extends the LCLint annotation-assisted static checking tool. Our tool is as fast as a compiler and nearly as easy to use. We present experience using our approach to detect buffer overflow vulnerabilities in two security-sensitive programs. 1. Introduction ed a prototype tool that does this by extending LCLint [Evans96]. Our work differs from other work on static detection of buffer overflows in three key ways: (1) we Buffer overflow attacks are an important and persistent exploit semantic comments added to source code to security problem. Buffer overflows account for enable local checking of interprocedural properties; (2) approximately half of all security vulnerabilities we focus on lightweight static checking techniques that [CWPBW00, WFBA00]. Richard Pethia of CERT have good performance and scalability characteristics, identified buffer overflow attacks as the single most im- but sacrifice soundness and completeness; and (3) we portant security problem at a recent software introduce loop heuristics, a simple approach for engineering conference [Pethia00]; Brian Snow of the efficiently analyzing many loops found in typical NSA predicted that buffer overflow attacks would still programs. -
Aliasing Restrictions of C11 Formalized in Coq
Aliasing restrictions of C11 formalized in Coq Robbert Krebbers Radboud University Nijmegen December 11, 2013 @ CPP, Melbourne, Australia int f(int *p, int *q) { int x = *p; *q = 314; return x; } If p and q alias, the original value n of *p is returned n p q Optimizing x away is unsound: 314 would be returned Alias analysis: to determine whether pointers can alias Aliasing Aliasing: multiple pointers referring to the same object Optimizing x away is unsound: 314 would be returned Alias analysis: to determine whether pointers can alias Aliasing Aliasing: multiple pointers referring to the same object int f(int *p, int *q) { int x = *p; *q = 314; return x; } If p and q alias, the original value n of *p is returned n p q Alias analysis: to determine whether pointers can alias Aliasing Aliasing: multiple pointers referring to the same object int f(int *p, int *q) { int x = *p; *q = 314; return x *p; } If p and q alias, the original value n of *p is returned n p q Optimizing x away is unsound: 314 would be returned Aliasing Aliasing: multiple pointers referring to the same object int f(int *p, int *q) { int x = *p; *q = 314; return x; } If p and q alias, the original value n of *p is returned n p q Optimizing x away is unsound: 314 would be returned Alias analysis: to determine whether pointers can alias It can still be called with aliased pointers: x union { int x; float y; } u; y u.x = 271; return h(&u.x, &u.y); &u.x &u.y C89 allows p and q to be aliased, and thus requires it to return 271 C99/C11 allows type-based alias analysis: I A compiler -
Teach Yourself Perl 5 in 21 Days
Teach Yourself Perl 5 in 21 days David Till Table of Contents: Introduction ● Who Should Read This Book? ● Special Features of This Book ● Programming Examples ● End-of-Day Q& A and Workshop ● Conventions Used in This Book ● What You'll Learn in 21 Days Week 1 Week at a Glance ● Where You're Going Day 1 Getting Started ● What Is Perl? ● How Do I Find Perl? ❍ Where Do I Get Perl? ❍ Other Places to Get Perl ● A Sample Perl Program ● Running a Perl Program ❍ If Something Goes Wrong ● The First Line of Your Perl Program: How Comments Work ❍ Comments ● Line 2: Statements, Tokens, and <STDIN> ❍ Statements and Tokens ❍ Tokens and White Space ❍ What the Tokens Do: Reading from Standard Input ● Line 3: Writing to Standard Output ❍ Function Invocations and Arguments ● Error Messages ● Interpretive Languages Versus Compiled Languages ● Summary ● Q&A ● Workshop ❍ Quiz ❍ Exercises Day 2 Basic Operators and Control Flow ● Storing in Scalar Variables Assignment ❍ The Definition of a Scalar Variable ❍ Scalar Variable Syntax ❍ Assigning a Value to a Scalar Variable ● Performing Arithmetic ❍ Example of Miles-to-Kilometers Conversion ❍ The chop Library Function ● Expressions ❍ Assignments and Expressions ● Other Perl Operators ● Introduction to Conditional Statements ● The if Statement ❍ The Conditional Expression ❍ The Statement Block ❍ Testing for Equality Using == ❍ Other Comparison Operators ● Two-Way Branching Using if and else ● Multi-Way Branching Using elsif ● Writing Loops Using the while Statement ● Nesting Conditional Statements ● Looping Using -
User-Directed Loop-Transformations in Clang
User-Directed Loop-Transformations in Clang Michael Kruse Hal Finkel Argonne Leadership Computing Facility Argonne Leadership Computing Facility Argonne National Laboratory Argonne National Laboratory Argonne, USA Argonne, USA [email protected] hfi[email protected] Abstract—Directives for the compiler such as pragmas can Only #pragma unroll has broad support. #pragma ivdep made help programmers to separate an algorithm’s semantics from popular by icc and Cray to help vectorization is mimicked by its optimization. This keeps the code understandable and easier other compilers as well, but with different interpretations of to optimize for different platforms. Simple transformations such as loop unrolling are already implemented in most mainstream its meaning. However, no compiler allows applying multiple compilers. We recently submitted a proposal to add generalized transformations on a single loop systematically. loop transformations to the OpenMP standard. We are also In addition to straightforward trial-and-error execution time working on an implementation in LLVM/Clang/Polly to show its optimization, code transformation pragmas can be useful for feasibility and usefulness. The current prototype allows applying machine-learning assisted autotuning. The OpenMP approach patterns common to matrix-matrix multiplication optimizations. is to make the programmer responsible for the semantic Index Terms—OpenMP, Pragma, Loop Transformation, correctness of the transformation. This unfortunately makes it C/C++, Clang, LLVM, Polly hard for an autotuner which only measures the timing difference without understanding the code. Such an autotuner would I. MOTIVATION therefore likely suggest transformations that make the program Almost all processor time is spent in some kind of loop, and return wrong results or crash. -
Towards Restrict-Like Aliasing Semantics for C++ Abstract
Document Number: N3988 Date: 2014-05-23 Authors: Hal Finkel, [email protected] Hubert Tong, [email protected] Chandler Carruth, [email protected], Clark Nelson, [email protected], Daveed Vandevoode, [email protected], Michael Wong, [email protected] Project: Programming Language C++, EWG Reply to: [email protected] Revision: 1 Towards restrict-like aliasing semantics for C++ Abstract This paper is a follow-on to N3635 after a design discussion with many compiler implementers at Issaquah, and afterwards by telecon, and specifically proposes a new alias_set attribute as a way to partition type-based aliasing to enable more fine grain control, effectively giving C++ a more complete restrict-like aliasing syntax and semantics. Changes from previous papers: N3988: this paper updates with syntax and semantics N3635: Outlined initial idea on AliasGroup which became alias_set. The alternative of newType was rejected as too heavy weight of a solution. 1. Problem Domain There is no question that the restrict qualifier benefits compiler optimization in many ways, notably allowing improved code motion and elimination of loads and stores. Since the introduction of C99 restrict, it has been provided as a C++ extension in many compilers. But the feature is brittle in C++ without clear rules for C++ syntax and semantics. Now with the introduction of C++11, functors are being replaced with lambdas and users have begun asking how to use restrict in the presence of lambdas. Given the existing compiler support, we need to provide a solution with well-defined C++ semantics, before use of some common subset of C99 restrict becomes widely used in combination with C++11 constructs. -
Autotuning for Automatic Parallelization on Heterogeneous Systems
Autotuning for Automatic Parallelization on Heterogeneous Systems Zur Erlangung des akademischen Grades eines Doktors der Ingenieurwissenschaften von der KIT-Fakultät für Informatik des Karlsruher Instituts für Technologie (KIT) genehmigte Dissertation von Philip Pfaffe ___________________________________________________________________ ___________________________________________________________________ Tag der mündlichen Prüfung: 24.07.2019 1. Referent: Prof. Dr. Walter F. Tichy 2. Referent: Prof. Dr. Michael Philippsen Abstract To meet the surging demand for high-speed computation in an era of stagnat- ing increase in performance per processor, systems designers resort to aggregating many and even heterogeneous processors into single systems. Automatic paral- lelization tools relieve application developers of the tedious and error prone task of programming these heterogeneous systems. For these tools, there are two aspects to maximizing performance: Optimizing the execution on each parallel platform individually, and executing work on the available platforms cooperatively. To date, various approaches exist targeting either aspect. Automatic parallelization for simultaneous cooperative computation with optimized per-platform execution however remains an unsolved problem. This thesis presents the APHES framework to close that gap. The framework com- bines automatic parallelization with a novel technique for input-sensitive online autotuning. Its first component, a parallelizing polyhedral compiler, transforms implicitly data-parallel program parts for multiple platforms. Targeted platforms then automatically cooperate to process the work. During compilation, the code is instrumented to interact with libtuning, our new autotuner and second com- ponent of the framework. Tuning the work distribution and per-platform execu- tion maximizes overall performance. The autotuner enables always-on autotuning through a novel hybrid tuning method, combining a new efficient search technique and model-based prediction. -
Program Sequentially, Carefully, and Benefit from Compiler Advances For
Program Sequentially, Carefully, and Benefit from Compiler Advances for Parallel Heterogeneous Computing Mehdi Amini, Corinne Ancourt, Béatrice Creusillet, François Irigoin, Ronan Keryell To cite this version: Mehdi Amini, Corinne Ancourt, Béatrice Creusillet, François Irigoin, Ronan Keryell. Program Se- quentially, Carefully, and Benefit from Compiler Advances for Parallel Heterogeneous Computing. Magoulès Frédéric. Patterns for Parallel Programming on GPUs, Saxe-Coburg Publications, pp. 149- 169, 2012, 978-1-874672-57-9 10.4203/csets.34.6. hal-01526469 HAL Id: hal-01526469 https://hal-mines-paristech.archives-ouvertes.fr/hal-01526469 Submitted on 30 May 2017 HAL is a multi-disciplinary open access L’archive ouverte pluridisciplinaire HAL, est archive for the deposit and dissemination of sci- destinée au dépôt et à la diffusion de documents entific research documents, whether they are pub- scientifiques de niveau recherche, publiés ou non, lished or not. The documents may come from émanant des établissements d’enseignement et de teaching and research institutions in France or recherche français ou étrangers, des laboratoires abroad, or from public or private research centers. publics ou privés. Program Sequentially, Carefully, and Benefit from Compiler Advances for Parallel Heterogeneous Computing Mehdi Amini1;2 Corinne Ancourt1 B´eatriceCreusillet2 Fran¸coisIrigoin1 Ronan Keryell2 | 1MINES ParisTech/CRI, firstname.lastname @mines-paristech.fr 2SILKAN, firstname.lastname @silkan.com September 26th 2012 Abstract The current microarchitecture trend leads toward heterogeneity. This evolution is driven by the end of Moore's law and the frequency wall due to the power wall. Moreover, with the spreading of smartphone, some constraints from the mobile world drive the design of most new architectures. -
Alias-Free Parameters in C Using Multibodies Medhat Assaad Iowa State University
Computer Science Technical Reports Computer Science 7-2001 Alias-free parameters in C using multibodies Medhat Assaad Iowa State University Follow this and additional works at: http://lib.dr.iastate.edu/cs_techreports Part of the Programming Languages and Compilers Commons, and the Theory and Algorithms Commons Recommended Citation Assaad, Medhat, "Alias-free parameters in C using multibodies" (2001). Computer Science Technical Reports. 301. http://lib.dr.iastate.edu/cs_techreports/301 This Article is brought to you for free and open access by the Computer Science at Iowa State University Digital Repository. It has been accepted for inclusion in Computer Science Technical Reports by an authorized administrator of Iowa State University Digital Repository. For more information, please contact [email protected]. Alias-free parameters in C using multibodies Abstract Aliasing may cause problems for both optimization and reasoning about programs. Since static alias analysis is expensive, and sometimes, even impossible, compilers tend to be conservative. This usually leads to missing some optimization opportunities. Furthermore, when trying to understand code, one must consider all possible aliasing patterns. This can make reasonning about code difficult and non-trivial. We have implemented an approach for having alias-free parameters in C. This approach allows aliasing between the arguments at the call site, but guarantees that there will be no aliasing between arguments and between arguments and globals inside procedure bodies. This is done by having multiple bodies, up to one for each aliasing pattern. Procedure calls will be dispatched to the body that matches the aliasing pattern at the call site. By having alias-free parameters in the bodies, good optimization can be achieved. -
Aliasing Contracts, a Novel, Dynamically-Checked Alias Protection Scheme for Object- Oriented Programming Languages
UCAM-CL-TR-880 Technical Report ISSN 1476-2986 Number 880 Computer Laboratory Access contracts: a dynamic approach to object-oriented access protection Janina Voigt February 2016 15 JJ Thomson Avenue Cambridge CB3 0FD United Kingdom phone +44 1223 763500 http://www.cl.cam.ac.uk/ c 2016 Janina Voigt This technical report is based on a dissertation submitted May 2014 by the author for the degree of Doctor of Philosophy to the University of Cambridge, Trinity College. Technical reports published by the University of Cambridge Computer Laboratory are freely available via the Internet: http://www.cl.cam.ac.uk/techreports/ ISSN 1476-2986 Abstract In object-oriented (OO) programming, variables do not contain objects directly but ad- dresses of objects on the heap. Thus, several variables can point to the same object; we call this aliasing. Aliasing is a central feature of OO programming that enables efficient sharing of objects across a system. This is essential for the implementation of many programming idioms, such as iterators. On the other hand, aliasing reduces modularity and encapsulation, making programs difficult to understand, debug and maintain. Much research has been done on controlling aliasing. Alias protection schemes (such as Clarke et al.’s influential ownership types) limit which references can exist, thus guar- anteeing the protection of encapsulated objects. Unfortunately, existing schemes are significantly restrictive and consequently have not been widely adopted by software de- velopers. This thesis makes three contributions to the area of alias protection. Firstly, it pro- poses aliasing contracts, a novel, dynamically-checked alias protection scheme for object- oriented programming languages. -
Flexible Alias Protection
Flexible Alias Protection James Noble1, Jan Vitek2, and John Potter1 1 Microsoft Research Institute, Macquarie University, Sydney kjx,[email protected] 2 Object Systems Group, Universit´e de Gen`eve, Geneva. [email protected] Abstract. Aliasing is endemic in object oriented programming. Because an object can be modified via any alias, object oriented programs are hard to understand, maintain, and analyse. Flexible alias protection is a conceptual model of inter-object relationships which limits the visibility of changes via aliases, allowing objects to be aliased but mitigating the undesirable effects of aliasing. Flexible alias protection can be checked statically using programmer supplied aliasing modes and imposes no run- time overhead. Using flexible alias protection, programs can incorporate mutable objects, immutable values, and updatable collections of shared objects, in a natural object oriented programming style, while avoiding the problems caused by aliasing. 1 Introduction I am who I am; I will be who I will be. Object identity is the foundation of object oriented programming. Objects are useful for modelling application domain abstractions precisely because an ob- ject's identity always remains the same during the execution of a program | even if an object's state or behaviour changes, the object is always the same object, so it always represents the same phenomenon in the application domain [30]. Object identity causes practical problems for object oriented programming. In general, these problems all reduce to the presence of aliasing | that a par- ticular object can be referred to by any number of other objects [20]. Problems arise because objects' state can change, while their identity remains the same.