Meta-Programming
Total Page:16
File Type:pdf, Size:1020Kb
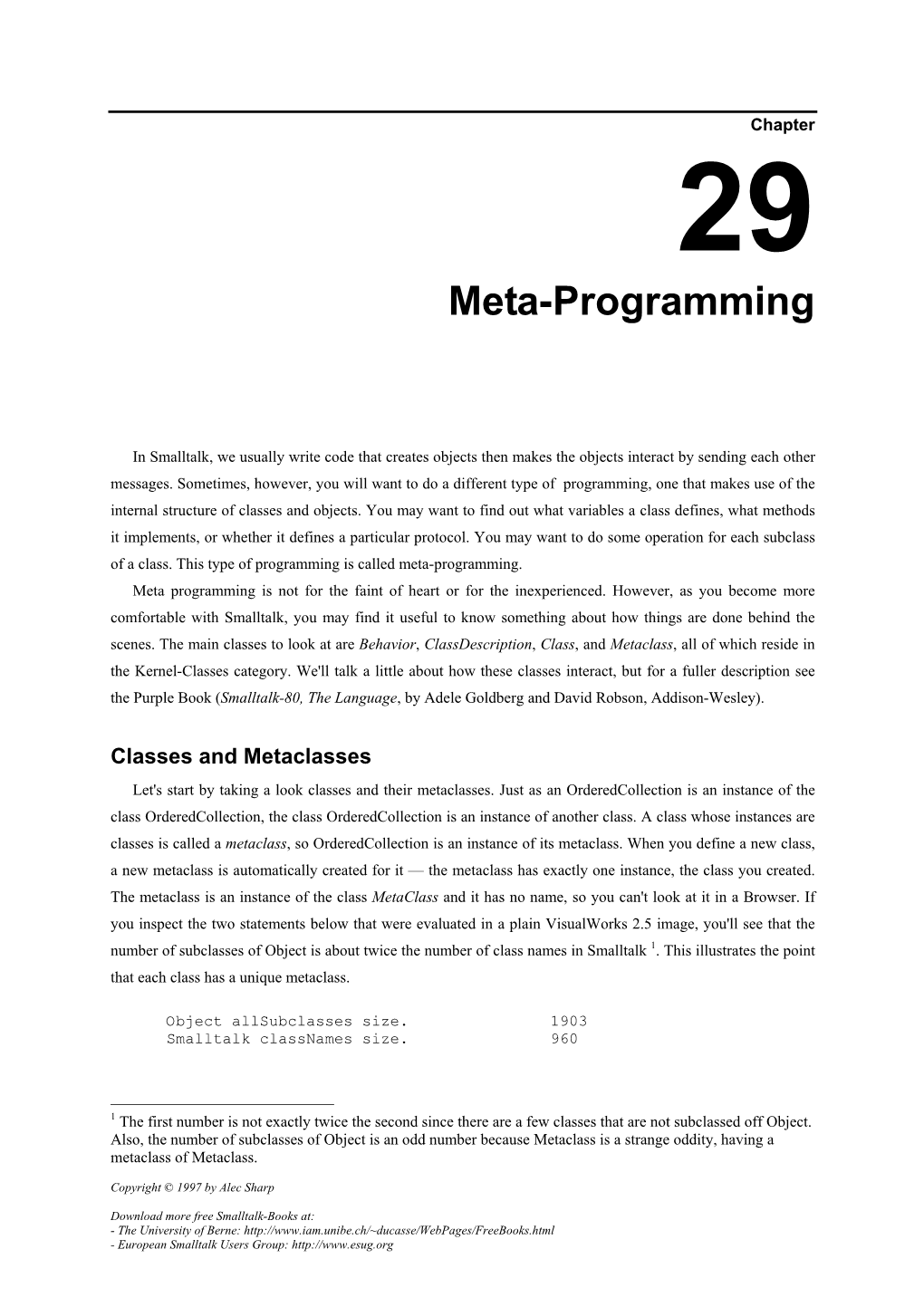
Load more
Recommended publications
-
CS 61A Streams Summer 2019 1 Streams
CS 61A Streams Summer 2019 Discussion 10: August 6, 2019 1 Streams In Python, we can use iterators to represent infinite sequences (for example, the generator for all natural numbers). However, Scheme does not support iterators. Let's see what happens when we try to use a Scheme list to represent an infinite sequence of natural numbers: scm> (define (naturals n) (cons n (naturals (+ n 1)))) naturals scm> (naturals 0) Error: maximum recursion depth exceeded Because cons is a regular procedure and both its operands must be evaluted before the pair is constructed, we cannot create an infinite sequence of integers using a Scheme list. Instead, our Scheme interpreter supports streams, which are lazy Scheme lists. The first element is represented explicitly, but the rest of the stream's elements are computed only when needed. Computing a value only when it's needed is also known as lazy evaluation. scm> (define (naturals n) (cons-stream n (naturals (+ n 1)))) naturals scm> (define nat (naturals 0)) nat scm> (car nat) 0 scm> (cdr nat) #[promise (not forced)] scm> (car (cdr-stream nat)) 1 scm> (car (cdr-stream (cdr-stream nat))) 2 We use the special form cons-stream to create a stream: (cons-stream <operand1> <operand2>) cons-stream is a special form because the second operand is not evaluated when evaluating the expression. To evaluate this expression, Scheme does the following: 1. Evaluate the first operand. 2. Construct a promise containing the second operand. 3. Return a pair containing the value of the first operand and the promise. 2 Streams To actually get the rest of the stream, we must call cdr-stream on it to force the promise to be evaluated. -
Chapter 2 Basics of Scanning And
Chapter 2 Basics of Scanning and Conventional Programming in Java In this chapter, we will introduce you to an initial set of Java features, the equivalent of which you should have seen in your CS-1 class; the separation of problem, representation, algorithm and program – four concepts you have probably seen in your CS-1 class; style rules with which you are probably familiar, and scanning - a general class of problems we see in both computer science and other fields. Each chapter is associated with an animating recorded PowerPoint presentation and a YouTube video created from the presentation. It is meant to be a transcript of the associated presentation that contains little graphics and thus can be read even on a small device. You should refer to the associated material if you feel the need for a different instruction medium. Also associated with each chapter is hyperlinked code examples presented here. References to previously presented code modules are links that can be traversed to remind you of the details. The resources for this chapter are: PowerPoint Presentation YouTube Video Code Examples Algorithms and Representation Four concepts we explicitly or implicitly encounter while programming are problems, representations, algorithms and programs. Programs, of course, are instructions executed by the computer. Problems are what we try to solve when we write programs. Usually we do not go directly from problems to programs. Two intermediate steps are creating algorithms and identifying representations. Algorithms are sequences of steps to solve problems. So are programs. Thus, all programs are algorithms but the reverse is not true. -
Objects and Classes in Python Documentation Release 0.1
Objects and classes in Python Documentation Release 0.1 Jonathan Fine Sep 27, 2017 Contents 1 Decorators 2 1.1 The decorator syntax.........................................2 1.2 Bound methods............................................3 1.3 staticmethod() .........................................3 1.4 classmethod() ..........................................3 1.5 The call() decorator.......................................4 1.6 Nesting decorators..........................................4 1.7 Class decorators before Python 2.6.................................5 2 Constructing classes 6 2.1 The empty class...........................................6 3 dict_from_class() 8 3.1 The __dict__ of the empty class...................................8 3.2 Is the doc-string part of the body?..................................9 3.3 Definition of dict_from_class() ...............................9 4 property_from_class() 10 4.1 About properties........................................... 10 4.2 Definition of property_from_class() ............................ 11 4.3 Using property_from_class() ................................ 11 4.4 Unwanted keys............................................ 11 5 Deconstructing classes 13 6 type(name, bases, dict) 14 6.1 Constructing the empty class..................................... 14 6.2 Constructing any class........................................ 15 6.3 Specifying __doc__, __name__ and __module__.......................... 15 7 Subclassing int 16 7.1 Mutable and immutable types.................................... 16 7.2 -
File I/O Stream Byte Stream
File I/O In Java, we can read data from files and also write data in files. We do this using streams. Java has many input and output streams that are used to read and write data. Same as a continuous flow of water is called water stream, in the same way input and output flow of data is called stream. Stream Java provides many input and output stream classes which are used to read and write. Streams are of two types. Byte Stream Character Stream Let's look at the two streams one by one. Byte Stream It is used in the input and output of byte. We do this with the help of different Byte stream classes. Two most commonly used Byte stream classes are FileInputStream and FileOutputStream. Some of the Byte stream classes are listed below. Byte Stream Description BufferedInputStream handles buffered input stream BufferedOutputStrea handles buffered output stream m FileInputStream used to read from a file FileOutputStream used to write to a file InputStream Abstract class that describe input stream OutputStream Abstract class that describe output stream Byte Stream Classes are in divided in two groups - InputStream Classes - These classes are subclasses of an abstract class, InputStream and they are used to read bytes from a source(file, memory or console). OutputStream Classes - These classes are subclasses of an abstract class, OutputStream and they are used to write bytes to a destination(file, memory or console). InputStream InputStream class is a base class of all the classes that are used to read bytes from a file, memory or console. -
Csci 658-01: Software Language Engineering Python 3 Reflexive
CSci 658-01: Software Language Engineering Python 3 Reflexive Metaprogramming Chapter 3 H. Conrad Cunningham 4 May 2018 Contents 3 Decorators and Metaclasses 2 3.1 Basic Function-Level Debugging . .2 3.1.1 Motivating example . .2 3.1.2 Abstraction Principle, staying DRY . .3 3.1.3 Function decorators . .3 3.1.4 Constructing a debug decorator . .4 3.1.5 Using the debug decorator . .6 3.1.6 Case study review . .7 3.1.7 Variations . .7 3.1.7.1 Logging . .7 3.1.7.2 Optional disable . .8 3.2 Extended Function-Level Debugging . .8 3.2.1 Motivating example . .8 3.2.2 Decorators with arguments . .9 3.2.3 Prefix decorator . .9 3.2.4 Reformulated prefix decorator . 10 3.3 Class-Level Debugging . 12 3.3.1 Motivating example . 12 3.3.2 Class-level debugger . 12 3.3.3 Variation: Attribute access debugging . 14 3.4 Class Hierarchy Debugging . 16 3.4.1 Motivating example . 16 3.4.2 Review of objects and types . 17 3.4.3 Class definition process . 18 3.4.4 Changing the metaclass . 20 3.4.5 Debugging using a metaclass . 21 3.4.6 Why metaclasses? . 22 3.5 Chapter Summary . 23 1 3.6 Exercises . 23 3.7 Acknowledgements . 23 3.8 References . 24 3.9 Terms and Concepts . 24 Copyright (C) 2018, H. Conrad Cunningham Professor of Computer and Information Science University of Mississippi 211 Weir Hall P.O. Box 1848 University, MS 38677 (662) 915-5358 Note: This chapter adapts David Beazley’s debugly example presentation from his Python 3 Metaprogramming tutorial at PyCon’2013 [Beazley 2013a]. -
The Use of UML for Software Requirements Expression and Management
The Use of UML for Software Requirements Expression and Management Alex Murray Ken Clark Jet Propulsion Laboratory Jet Propulsion Laboratory California Institute of Technology California Institute of Technology Pasadena, CA 91109 Pasadena, CA 91109 818-354-0111 818-393-6258 [email protected] [email protected] Abstract— It is common practice to write English-language 1. INTRODUCTION ”shall” statements to embody detailed software requirements in aerospace software applications. This paper explores the This work is being performed as part of the engineering of use of the UML language as a replacement for the English the flight software for the Laser Ranging Interferometer (LRI) language for this purpose. Among the advantages offered by the of the Gravity Recovery and Climate Experiment (GRACE) Unified Modeling Language (UML) is a high degree of clarity Follow-On (F-O) mission. However, rather than use the real and precision in the expression of domain concepts as well as project’s model to illustrate our approach in this paper, we architecture and design. Can this quality of UML be exploited have developed a separate, ”toy” model for use here, because for the definition of software requirements? this makes it easier to avoid getting bogged down in project details, and instead to focus on the technical approach to While expressing logical behavior, interface characteristics, requirements expression and management that is the subject timeliness constraints, and other constraints on software using UML is commonly done and relatively straight-forward, achiev- of this paper. ing the additional aspects of the expression and management of software requirements that stakeholders expect, especially There is one exception to this choice: we will describe our traceability, is far less so. -
Metaclasses: Generative C++
Metaclasses: Generative C++ Document Number: P0707 R3 Date: 2018-02-11 Reply-to: Herb Sutter ([email protected]) Audience: SG7, EWG Contents 1 Overview .............................................................................................................................................................2 2 Language: Metaclasses .......................................................................................................................................7 3 Library: Example metaclasses .......................................................................................................................... 18 4 Applying metaclasses: Qt moc and C++/WinRT .............................................................................................. 35 5 Alternatives for sourcedefinition transform syntax .................................................................................... 41 6 Alternatives for applying the transform .......................................................................................................... 43 7 FAQs ................................................................................................................................................................. 46 8 Revision history ............................................................................................................................................... 51 Major changes in R3: Switched to function-style declaration syntax per SG7 direction in Albuquerque (old: $class M new: constexpr void M(meta::type target, -
C++ Input/Output: Streams 4
C++ Input/Output: Streams 4. Input/Output 1 The basic data type for I/O in C++ is the stream. C++ incorporates a complex hierarchy of stream types. The most basic stream types are the standard input/output streams: istream cin built-in input stream variable; by default hooked to keyboard ostream cout built-in output stream variable; by default hooked to console header file: <iostream> C++ also supports all the input/output mechanisms that the C language included. However, C++ streams provide all the input/output capabilities of C, with substantial improvements. We will exclusively use streams for input and output of data. Computer Science Dept Va Tech August, 2001 Intro Programming in C++ ©1995-2001 Barnette ND & McQuain WD C++ Streams are Objects 4. Input/Output 2 The input and output streams, cin and cout are actually C++ objects. Briefly: class: a C++ construct that allows a collection of variables, constants, and functions to be grouped together logically under a single name object: a variable of a type that is a class (also often called an instance of the class) For example, istream is actually a type name for a class. cin is the name of a variable of type istream. So, we would say that cin is an instance or an object of the class istream. An instance of a class will usually have a number of associated functions (called member functions) that you can use to perform operations on that object or to obtain information about it. The following slides will present a few of the basic stream member functions, and show how to go about using member functions. -
Meta-Class Features for Large-Scale Object Categorization on a Budget
Meta-Class Features for Large-Scale Object Categorization on a Budget Alessandro Bergamo Lorenzo Torresani Dartmouth College Hanover, NH, U.S.A. faleb, [email protected] Abstract cation accuracy over a predefined set of classes, and without consideration of the computational costs of the recognition. In this paper we introduce a novel image descriptor en- We believe that these two assumptions do not meet the abling accurate object categorization even with linear mod- requirements of modern applications of large-scale object els. Akin to the popular attribute descriptors, our feature categorization. For example, test-recognition efficiency is a vector comprises the outputs of a set of classifiers evaluated fundamental requirement to be able to scale object classi- on the image. However, unlike traditional attributes which fication to Web photo repositories, such as Flickr, which represent hand-selected object classes and predefined vi- are growing at rates of several millions new photos per sual properties, our features are learned automatically and day. Furthermore, while a fixed set of object classifiers can correspond to “abstract” categories, which we name meta- be used to annotate pictures with a set of predefined tags, classes. Each meta-class is a super-category obtained by the interactive nature of searching and browsing large im- grouping a set of object classes such that, collectively, they age collections calls for the ability to allow users to define are easy to distinguish from other sets of categories. By us- their own personal query categories to be recognized and ing “learnability” of the meta-classes as criterion for fea- retrieved from the database, ideally in real-time. -
Subtyping, Declaratively an Exercise in Mixed Induction and Coinduction
Subtyping, Declaratively An Exercise in Mixed Induction and Coinduction Nils Anders Danielsson and Thorsten Altenkirch University of Nottingham Abstract. It is natural to present subtyping for recursive types coin- ductively. However, Gapeyev, Levin and Pierce have noted that there is a problem with coinductive definitions of non-trivial transitive inference systems: they cannot be \declarative"|as opposed to \algorithmic" or syntax-directed|because coinductive inference systems with an explicit rule of transitivity are trivial. We propose a solution to this problem. By using mixed induction and coinduction we define an inference system for subtyping which combines the advantages of coinduction with the convenience of an explicit rule of transitivity. The definition uses coinduction for the structural rules, and induction for the rule of transitivity. We also discuss under what condi- tions this technique can be used when defining other inference systems. The developments presented in the paper have been mechanised using Agda, a dependently typed programming language and proof assistant. 1 Introduction Coinduction and corecursion are useful techniques for defining and reasoning about things which are potentially infinite, including streams and other (poten- tially) infinite data types (Coquand 1994; Gim´enez1996; Turner 2004), process congruences (Milner 1990), congruences for functional programs (Gordon 1999), closures (Milner and Tofte 1991), semantics for divergence of programs (Cousot and Cousot 1992; Hughes and Moran 1995; Leroy and Grall 2009; Nakata and Uustalu 2009), and subtyping relations for recursive types (Brandt and Henglein 1998; Gapeyev et al. 2002). However, the use of coinduction can lead to values which are \too infinite”. For instance, a non-trivial binary relation defined as a coinductive inference sys- tem cannot include the rule of transitivity, because a coinductive reading of transitivity would imply that every element is related to every other (to see this, build an infinite derivation consisting solely of uses of transitivity). -
Metaclass Functions: Generative C++
Metaclass functions: Generative C++ Document Number: P0707 R4 Date: 2019-06-16 Reply-to: Herb Sutter ([email protected]) Audience: SG7, EWG Contents 1 Overview .............................................................................................................................................................2 2 Language: “Metaclass” functions .......................................................................................................................6 3 Library: Example metaclasses .......................................................................................................................... 18 4 Applying metaclasses: Qt moc and C++/WinRT .............................................................................................. 35 5 FAQs ................................................................................................................................................................. 41 R4: • Updated notes in §1.3 to track the current prototype, which now also has consteval support. • Added using metaclass function in the class body. Abstract The only way to make a language more powerful, but also make its programs simpler, is by abstraction: adding well-chosen abstractions that let programmers replace manual code patterns with saying directly what they mean. There are two major categories: Elevate coding patterns/idioms into new abstractions built into the language. For example, in current C++, range-for lets programmers directly declare “for each” loops with compiler support and enforcement. -
Middlesex University Research Repository
Middlesex University Research Repository An open access repository of Middlesex University research http://eprints.mdx.ac.uk Clark, Tony (1997) Metaclasses and reflection in smalltalk. Technical Report. University of Bradford. Available from Middlesex University’s Research Repository at http://eprints.mdx.ac.uk/6181/ Copyright: Middlesex University Research Repository makes the University’s research available electronically. Copyright and moral rights to this thesis/research project are retained by the author and/or other copyright owners. The work is supplied on the understanding that any use for commercial gain is strictly forbidden. A copy may be downloaded for personal, non-commercial, research or study without prior permission and without charge. Any use of the thesis/research project for private study or research must be properly acknowledged with reference to the work’s full bibliographic details. This thesis/research project may not be reproduced in any format or medium, or extensive quotations taken from it, or its content changed in any way, without first obtaining permission in writing from the copyright holder(s). If you believe that any material held in the repository infringes copyright law, please contact the Repository Team at Middlesex University via the following email address: [email protected] The item will be removed from the repository while any claim is being investigated. Metaclasses and Reection in Smalltalk A N Clark Department of Computing University of Bradford Bradford West Yorkshire BD DP UK email ANClarkcompbradacuk