Common Lisp Recipes
Total Page:16
File Type:pdf, Size:1020Kb
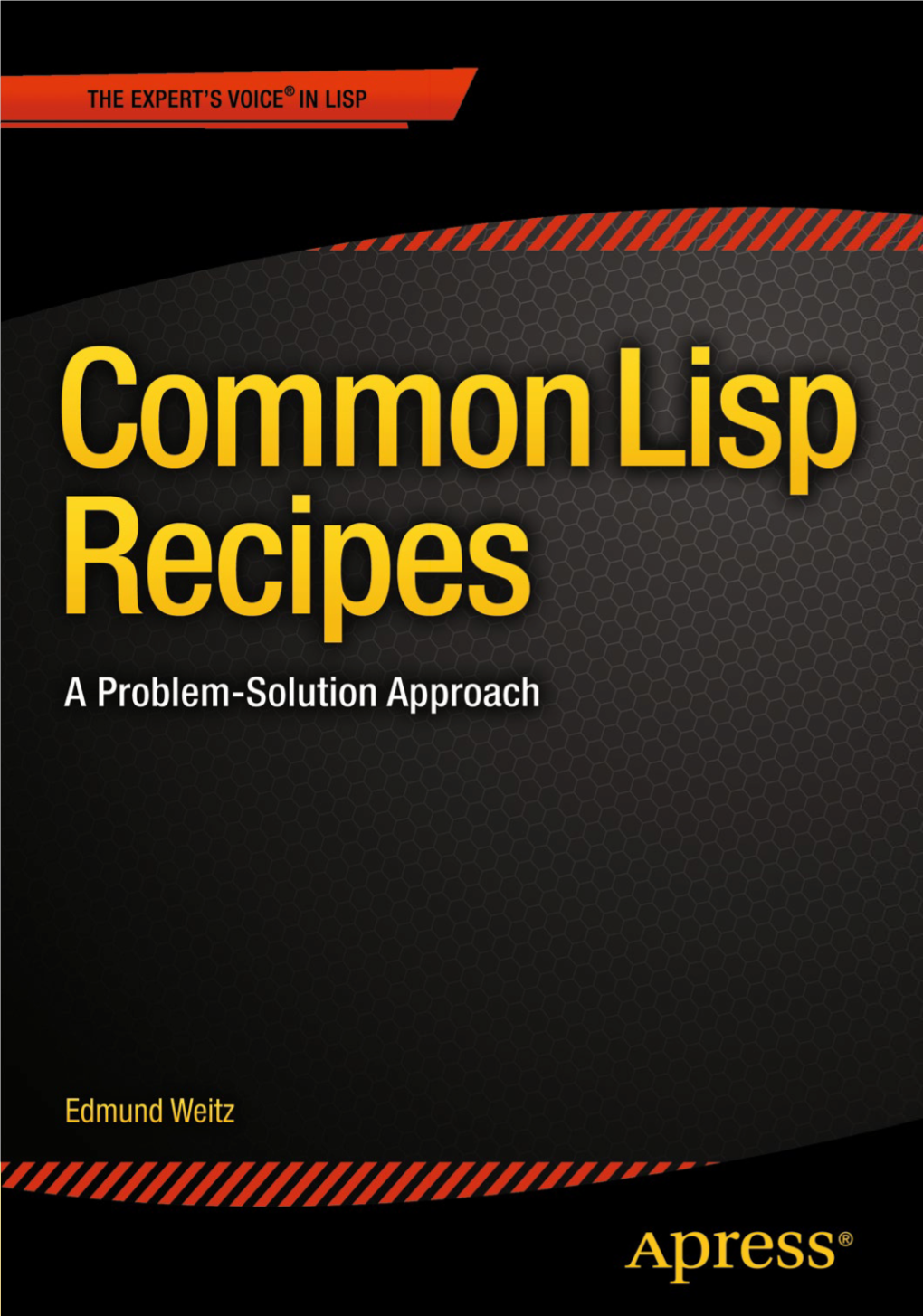
Load more
Recommended publications
-
Chapter 5 Names, Bindings, and Scopes
Chapter 5 Names, Bindings, and Scopes 5.1 Introduction 198 5.2 Names 199 5.3 Variables 200 5.4 The Concept of Binding 203 5.5 Scope 211 5.6 Scope and Lifetime 222 5.7 Referencing Environments 223 5.8 Named Constants 224 Summary • Review Questions • Problem Set • Programming Exercises 227 CMPS401 Class Notes (Chap05) Page 1 / 20 Dr. Kuo-pao Yang Chapter 5 Names, Bindings, and Scopes 5.1 Introduction 198 Imperative languages are abstractions of von Neumann architecture – Memory: stores both instructions and data – Processor: provides operations for modifying the contents of memory Variables are characterized by a collection of properties or attributes – The most important of which is type, a fundamental concept in programming languages – To design a type, must consider scope, lifetime, type checking, initialization, and type compatibility 5.2 Names 199 5.2.1 Design issues The following are the primary design issues for names: – Maximum length? – Are names case sensitive? – Are special words reserved words or keywords? 5.2.2 Name Forms A name is a string of characters used to identify some entity in a program. Length – If too short, they cannot be connotative – Language examples: . FORTRAN I: maximum 6 . COBOL: maximum 30 . C99: no limit but only the first 63 are significant; also, external names are limited to a maximum of 31 . C# and Java: no limit, and all characters are significant . C++: no limit, but implementers often impose a length limitation because they do not want the symbol table in which identifiers are stored during compilation to be too large and also to simplify the maintenance of that table. -
Ain Stn:Et:. Forma Camegie Ubwy, Ua Local
City of New Rochelle Dcparl!nent of Development TO: 1HRU: FROM: DATE: SUBJECT: ain Stn:et:. forma Camegie ubwy, u a Local Backgtowad: A request wu received by the Historical and Lo.odmarks Review Board (HLRB) from alocol resident in ]tml2(}' 2016 to nominate 662 Main Street, the former Camegie Library, u a locallandnwk. The request is attached for your reference. The libraty is a Nco-O.ssicol Rmval inotitutiosul building designed by Albett Randolph Rosa and built by prominent New Rocb~ muter buildet Mic;had Barnett. The building aerved as New Rocbclle'slibnty &omits opening in 1914 until1979 andu one of just three Camegic library buildings otilletanding in Westchester County. The building is ama1dy owned by Hagerdom Communications The HLRB held a public hearing on Much 9, 2015 and voted wtanimously in fiavor of the request. A copy of tbdt tesolution is attached for your refe%ellce. RccommettdatioD: It is t=>IDD>ellded that the Council designate tbia site a landmuk after holding a public meding; however it is also l'eCOtllnldlded that the City should not proceed with further landmatking until a city wide hi.otoric plan bas been completed to identify structun:s and sites of historic sigoilicance. Such a plan will p.rovide valuable info=ation and protection of important sttuctures, and msure future landmark applications can be ...essed u part of the City's ovc:nll fabric, not as single otand-olone cites. In accordance with the City code provisions, tbia proposal must be ref=ed to the Planning Baud for ito recotn~JV.fldation u to the proposed landmW:'s compatibility with the City's comptthenoive plan. -
An Implementation of Python for Racket
An Implementation of Python for Racket Pedro Palma Ramos António Menezes Leitão INESC-ID, Instituto Superior Técnico, INESC-ID, Instituto Superior Técnico, Universidade de Lisboa Universidade de Lisboa Rua Alves Redol 9 Rua Alves Redol 9 Lisboa, Portugal Lisboa, Portugal [email protected] [email protected] ABSTRACT Keywords Racket is a descendent of Scheme that is widely used as a Python; Racket; Language implementations; Compilers first language for teaching computer science. To this end, Racket provides DrRacket, a simple but pedagogic IDE. On the other hand, Python is becoming increasingly popular 1. INTRODUCTION in a variety of areas, most notably among novice program- The Racket programming language is a descendent of Scheme, mers. This paper presents an implementation of Python a language that is well-known for its use in introductory for Racket which allows programmers to use DrRacket with programming courses. Racket comes with DrRacket, a ped- Python code, as well as adding Python support for other Dr- agogic IDE [2], used in many schools around the world, as Racket based tools. Our implementation also allows Racket it provides a simple and straightforward interface aimed at programs to take advantage of Python libraries, thus signif- inexperienced programmers. Racket provides different lan- icantly enlarging the number of usable libraries in Racket. guage levels, each one supporting more advanced features, that are used in different phases of the courses, allowing Our proposed solution involves compiling Python code into students to benefit from a smoother learning curve. Fur- semantically equivalent Racket source code. For the run- thermore, Racket and DrRacket support the development of time implementation, we present two different strategies: additional programming languages [13]. -
A Concurrent PASCAL Compiler for Minicomputers
512 Appendix A DIFFERENCES BETWEEN UCSD'S PASCAL AND STANDARD PASCAL The PASCAL language used in this book contains most of the features described by K. Jensen and N. Wirth in PASCAL User Manual and Report, Springer Verlag, 1975. We refer to the PASCAL defined by Jensen and Wirth as "Standard" PASCAL, because of its widespread acceptance even though no international standard for the language has yet been established. The PASCAL used in this book has been implemented at University of California San Diego (UCSD) in a complete software system for use on a variety of small stand-alone microcomputers. This will be referred to as "UCSD PASCAL", which differs from the standard by a small number of omissions, a very small number of alterations, and several extensions. This appendix provides a very brief summary Of these differences. Only the PASCAL constructs used within this book will be mentioned herein. Documents are available from the author's group at UCSD describing UCSD PASCAL in detail. 1. CASE Statements Jensen & Wirth state that if there is no label equal to the value of the case statement selector, then the result of the case statement is undefined. UCSD PASCAL treats this situation by leaving the case statement normally with no action being taken. 2. Comments In UCSD PASCAL, a comment appears between the delimiting symbols "(*" and "*)". If the opening delimiter is followed immediately by a dollar sign, as in "(*$", then the remainder of the comment is treated as a directive to the compiler. The only compiler directive mentioned in this book is (*$G+*), which tells the compiler to allow the use of GOTO statements. -
The Machine That Builds Itself: How the Strengths of Lisp Family
Khomtchouk et al. OPINION NOTE The Machine that Builds Itself: How the Strengths of Lisp Family Languages Facilitate Building Complex and Flexible Bioinformatic Models Bohdan B. Khomtchouk1*, Edmund Weitz2 and Claes Wahlestedt1 *Correspondence: [email protected] Abstract 1Center for Therapeutic Innovation and Department of We address the need for expanding the presence of the Lisp family of Psychiatry and Behavioral programming languages in bioinformatics and computational biology research. Sciences, University of Miami Languages of this family, like Common Lisp, Scheme, or Clojure, facilitate the Miller School of Medicine, 1120 NW 14th ST, Miami, FL, USA creation of powerful and flexible software models that are required for complex 33136 and rapidly evolving domains like biology. We will point out several important key Full list of author information is features that distinguish languages of the Lisp family from other programming available at the end of the article languages and we will explain how these features can aid researchers in becoming more productive and creating better code. We will also show how these features make these languages ideal tools for artificial intelligence and machine learning applications. We will specifically stress the advantages of domain-specific languages (DSL): languages which are specialized to a particular area and thus not only facilitate easier research problem formulation, but also aid in the establishment of standards and best programming practices as applied to the specific research field at hand. DSLs are particularly easy to build in Common Lisp, the most comprehensive Lisp dialect, which is commonly referred to as the “programmable programming language.” We are convinced that Lisp grants programmers unprecedented power to build increasingly sophisticated artificial intelligence systems that may ultimately transform machine learning and AI research in bioinformatics and computational biology. -
Common Lispworks User Guide
LispWorks® for the Windows® Operating System Common LispWorks User Guide Version 5.1 Copyright and Trademarks Common LispWorks User Guide (Windows version) Version 5.1 February 2008 Copyright © 2008 by LispWorks Ltd. All Rights Reserved. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form or by any means, electronic, mechanical, photocopying, recording, or otherwise, without the prior written permission of LispWorks Ltd. The information in this publication is provided for information only, is subject to change without notice, and should not be construed as a commitment by LispWorks Ltd. LispWorks Ltd assumes no responsibility or liability for any errors or inaccuracies that may appear in this publication. The software described in this book is furnished under license and may only be used or copied in accordance with the terms of that license. LispWorks and KnowledgeWorks are registered trademarks of LispWorks Ltd. Adobe and PostScript are registered trademarks of Adobe Systems Incorporated. Other brand or product names are the registered trade- marks or trademarks of their respective holders. The code for walker.lisp and compute-combination-points is excerpted with permission from PCL, Copyright © 1985, 1986, 1987, 1988 Xerox Corporation. The XP Pretty Printer bears the following copyright notice, which applies to the parts of LispWorks derived therefrom: Copyright © 1989 by the Massachusetts Institute of Technology, Cambridge, Massachusetts. Permission to use, copy, modify, and distribute this software and its documentation for any purpose and without fee is hereby granted, pro- vided that this copyright and permission notice appear in all copies and supporting documentation, and that the name of M.I.T. -
IDE User Guide Version 7.1 Copyright and Trademarks Lispworks IDE User Guide (Unix Version) Version 7.1 September 2017 Copyright © 2017 by Lispworks Ltd
LispWorks® IDE User Guide Version 7.1 Copyright and Trademarks LispWorks IDE User Guide (Unix version) Version 7.1 September 2017 Copyright © 2017 by LispWorks Ltd. All Rights Reserved. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form or by any means, electronic, mechanical, photocopying, recording, or otherwise, without the prior written permission of LispWorks Ltd. The information in this publication is provided for information only, is subject to change without notice, and should not be construed as a commitment by LispWorks Ltd. LispWorks Ltd assumes no responsibility or liability for any errors or inaccuracies that may appear in this publication. The software described in this book is furnished under license and may only be used or copied in accordance with the terms of that license. LispWorks and KnowledgeWorks are registered trademarks of LispWorks Ltd. Adobe and PostScript are registered trademarks of Adobe Systems Incorporated. Other brand or product names are the registered trade- marks or trademarks of their respective holders. The code for walker.lisp and compute-combination-points is excerpted with permission from PCL, Copyright © 1985, 1986, 1987, 1988 Xerox Corporation. The XP Pretty Printer bears the following copyright notice, which applies to the parts of LispWorks derived therefrom: Copyright © 1989 by the Massachusetts Institute of Technology, Cambridge, Massachusetts. Permission to use, copy, modify, and distribute this software and its documentation for any purpose and without fee is hereby granted, pro- vided that this copyright and permission notice appear in all copies and supporting documentation, and that the name of M.I.T. -
Omnipresent and Low-Overhead Application Debugging
Omnipresent and low-overhead application debugging Robert Strandh [email protected] LaBRI, University of Bordeaux Talence, France ABSTRACT application programmers as opposed to system programmers. The state of the art in application debugging in free Common The difference, in the context of this paper, is that the tech- Lisp implementations leaves much to be desired. In many niques that we suggest are not adapted to debugging the cases, only a backtrace inspector is provided, allowing the system itself, such as the compiler. Instead, throughout this application programmer to examine the control stack when paper, we assume that, as far as the application programmer an unhandled error is signaled. Most such implementations do is concerned, the semantics of the code generated by the not allow the programmer to set breakpoints (unconditional compiler corresponds to that of the source code. or conditional), nor to step the program after it has stopped. In this paper, we are mainly concerned with Common Furthermore, even debugging tools such as tracing or man- Lisp [1] implementations distributed as so-called FLOSS, i.e., ually calling break are typically very limited in that they do \Free, Libre, and Open Source Software". While some such not allow the programmer to trace or break in important sys- implementations are excellent in terms of the quality of the tem functions such as make-instance or shared-initialize, code that the compiler generates, most leave much to be simply because these tools impact all callers, including those desired when it comes to debugging tools available to the of the system itself, such as the compiler. -
WESTFIELD LEADER Westfield Since 1890
WESTFIELD LEADER Westfield Since 1890 USPSMO2O Published \R, NO. 32 Snoot CUii Ponnt Paid 24 Pages—MCcnti •I WaifMd. N.j. WESTFIELD, NEW JERSEY, THURSDAY, MARCH 2, 1989 Every Thursday ents May Take Part in Cosmair Proposes Placing M h Cholesterol Screening LPG Tank Underground Beginning U< rch 13); Approximately two weeks life when lifetime eating habits Representative of a Clark- Emerson Thomas, a principal in proximity to other residences on third, fourth a:- iders in after the screening, Dr. Lasser are established, it is important to based hair care products the firm Thomas & Associates, Summit Court. Stephen Ed- the Westfield Schools, will send letters notifying parents modify these patterns in manufacturer seeking permis- which designed the tank, testified wards, attorney for Cosmair, with parental permission, will of their child's cholesterol level. childhood. sion to locate a liquid propane as to the safety of the tank, which said that if this is the case, take part in the Dietary Interven- Children with a cholesterol level "In this way we hope to gas (LPG) tank on residentially complies with all federal, state Cosmair will still place the tank tion Study in Children (DISC) be- above average will be invited forestall adult coronary disease, zoned Westfield land, have pro- and industry safety standards. underground. ing conducted by the University back for a series of clinic visits to particularly in families with such posed placing the LPG tank Gerard Stockard, vice presi- The hearing will be continued of Medicine and Dentistry of New determine if they are eligible to a history," he said. -
MOCL: an Efficient Opencl Implementation for the Matrix-2000
MOCL: An Eicient OpenCL Implementation for the Matrix-2000 Architecture ABSTRACT Since existing OpenCL frameworks mainly target CPUs and is paper presents the design and implementation of an Open GPUs, they are not directly applicable to Matrix-2000 [1, 2, 7, 14, 15, Computing Language (OpenCL) framework for the Matrix-2000 18, 20]. Providing an ecient OpenCL implementation for Matrix- many-core architecture. is architecture is designed to replace 2000 is unique in that the hardware architecture diers from a the Intel XeonPhi accelerators of the TianHe-2 supercomputer. We many-core GPU with a smaller number of cores and runs a light- share our experience and insights on how to design an eective weight operating system. To exploit the hardware, we have to OpenCL system for this new hardware accelerator. We propose a develop a compiler to translate kernels into target-specic bina- set of new analysis and optimizations to unlock the potential of ries and provide a runtime to manage task dispatching and data the hardware. We extensively evaluate our approach using a wide communication between the host and the accelerator. range of OpenCL benchmarks on a single and multiple computing is paper presents the design and implementation of MOCL, an nodes. We present our design choices and provide guidance how OpenCL programming interface for Matrix-2000. MOCL consists of to optimize code on the new Matrix-2000 architecture. two main components: a kernel compiler and a runtime. e kernel compiler is built upon the LLVM compiler infrastructure [19]. It KEYWORDS translates each OpenCL kernel to an executable binary to run on Matrix-2000. -
Praise for Practical Common Lisp
Praise for Practical Common Lisp “Finally, a Lisp book for the rest of us. If you want to learn how to write a factorial function, this is not your book. Seibel writes for the practical programmer, emphasizing the engineer/artist over the scientist and subtly and gracefully implying the power of the language while solving understandable real-world problems. “In most chapters, the reading of the chapter feels just like the experience of writing a program, starting with a little understanding and then having that understanding grow, like building the shoulders upon which you can then stand. When Seibel introduced macros as an aside while building a test frame- work, I was shocked at how such a simple example made me really ‘get’ them. Narrative context is extremely powerful, and the technical books that use it are a cut above. Congrats!” —Keith Irwin, Lisp programmer “While learning Lisp, one is often referred to the CL HyperSpec if they do not know what a particular function does; however, I found that I often did not ‘get it’ just by reading the HyperSpec. When I had a problem of this manner, I turned to Practical Common Lisp every single time—it is by far the most readable source on the subject that shows you how to program, not just tells you.” —Philip Haddad, Lisp programmer “With the IT world evolving at an ever-increasing pace, professionals need the most powerful tools available. This is why Common Lisp—the most powerful, flexible, and stable programming language ever—is seeing such a rise in popu- larity. -
The Evolution of Lisp
1 The Evolution of Lisp Guy L. Steele Jr. Richard P. Gabriel Thinking Machines Corporation Lucid, Inc. 245 First Street 707 Laurel Street Cambridge, Massachusetts 02142 Menlo Park, California 94025 Phone: (617) 234-2860 Phone: (415) 329-8400 FAX: (617) 243-4444 FAX: (415) 329-8480 E-mail: [email protected] E-mail: [email protected] Abstract Lisp is the world’s greatest programming language—or so its proponents think. The structure of Lisp makes it easy to extend the language or even to implement entirely new dialects without starting from scratch. Overall, the evolution of Lisp has been guided more by institutional rivalry, one-upsmanship, and the glee born of technical cleverness that is characteristic of the “hacker culture” than by sober assessments of technical requirements. Nevertheless this process has eventually produced both an industrial- strength programming language, messy but powerful, and a technically pure dialect, small but powerful, that is suitable for use by programming-language theoreticians. We pick up where McCarthy’s paper in the first HOPL conference left off. We trace the development chronologically from the era of the PDP-6, through the heyday of Interlisp and MacLisp, past the ascension and decline of special purpose Lisp machines, to the present era of standardization activities. We then examine the technical evolution of a few representative language features, including both some notable successes and some notable failures, that illuminate design issues that distinguish Lisp from other programming languages. We also discuss the use of Lisp as a laboratory for designing other programming languages. We conclude with some reflections on the forces that have driven the evolution of Lisp.