Concurrent Programming in Harmony
Total Page:16
File Type:pdf, Size:1020Kb
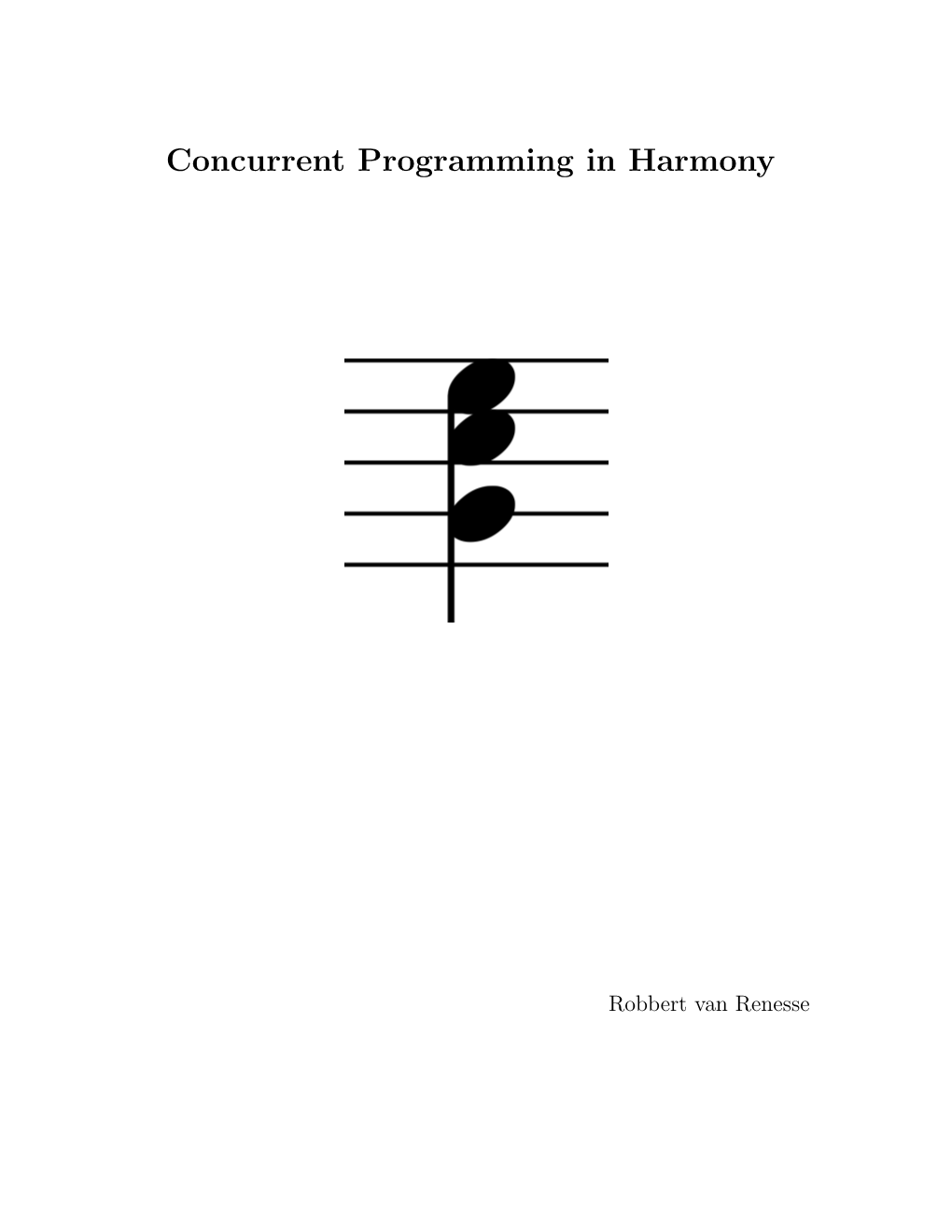
Load more
Recommended publications
-
Edsger W. Dijkstra: a Commemoration
Edsger W. Dijkstra: a Commemoration Krzysztof R. Apt1 and Tony Hoare2 (editors) 1 CWI, Amsterdam, The Netherlands and MIMUW, University of Warsaw, Poland 2 Department of Computer Science and Technology, University of Cambridge and Microsoft Research Ltd, Cambridge, UK Abstract This article is a multiauthored portrait of Edsger Wybe Dijkstra that consists of testimo- nials written by several friends, colleagues, and students of his. It provides unique insights into his personality, working style and habits, and his influence on other computer scientists, as a researcher, teacher, and mentor. Contents Preface 3 Tony Hoare 4 Donald Knuth 9 Christian Lengauer 11 K. Mani Chandy 13 Eric C.R. Hehner 15 Mark Scheevel 17 Krzysztof R. Apt 18 arXiv:2104.03392v1 [cs.GL] 7 Apr 2021 Niklaus Wirth 20 Lex Bijlsma 23 Manfred Broy 24 David Gries 26 Ted Herman 28 Alain J. Martin 29 J Strother Moore 31 Vladimir Lifschitz 33 Wim H. Hesselink 34 1 Hamilton Richards 36 Ken Calvert 38 David Naumann 40 David Turner 42 J.R. Rao 44 Jayadev Misra 47 Rajeev Joshi 50 Maarten van Emden 52 Two Tuesday Afternoon Clubs 54 2 Preface Edsger Dijkstra was perhaps the best known, and certainly the most discussed, computer scientist of the seventies and eighties. We both knew Dijkstra |though each of us in different ways| and we both were aware that his influence on computer science was not limited to his pioneering software projects and research articles. He interacted with his colleagues by way of numerous discussions, extensive letter correspondence, and hundreds of so-called EWD reports that he used to send to a select group of researchers. -
Glossary of Technical and Scientific Terms (French / English
Glossary of Technical and Scientific Terms (French / English) Version 11 (Alphabetical sorting) Jean-Luc JOULIN October 12, 2015 Foreword Aim of this handbook This glossary is made to help for translation of technical words english-french. This document is not made to be exhaustive but aim to understand and remember the translation of some words used in various technical domains. Words are sorted alphabetically. This glossary is distributed under the licence Creative Common (BY NC ND) and can be freely redistributed. For further informations about the licence Creative Com- mon (BY NC ND), browse the site: http://creativecommons.org If you are interested by updates of this glossary, send me an email to be on my diffusion list : • [email protected] • [email protected] Warning Translations given in this glossary are for information only and their use is under the responsability of the reader. If you see a mistake in this document, thank in advance to report it to me so i can correct it in further versions of this document. Notes Sorting of words Words are sorted by alphabetical order and are associated to their main category. Difference of terms Some words are different between the "British" and the "American" english. This glossary show the most used terms and are generally coming from the "British" english Words coming from the "American" english are indicated with the suffix : US . Difference of spelling There are some difference of spelling in accordance to the country, companies, universities, ... Words finishing by -ise, -isation and -yse can also be written with -ize, Jean-Luc JOULIN1 Glossary of Technical and Scientific Terms -ization and -yze. -
Apache Harmony Project Tim Ellison Geir Magnusson Jr
The Apache Harmony Project Tim Ellison Geir Magnusson Jr. Apache Harmony Project http://harmony.apache.org TS-7820 2007 JavaOneSM Conference | Session TS-7820 | Goal of This Talk In the next 45 minutes you will... Learn about the motivations, current status, and future plans of the Apache Harmony project 2007 JavaOneSM Conference | Session TS-7820 | 2 Agenda Project History Development Model Modularity VM Interface How Are We Doing? Relevance in the Age of OpenJDK Summary 2007 JavaOneSM Conference | Session TS-7820 | 3 Agenda Project History Development Model Modularity VM Interface How Are We Doing? Relevance in the Age of OpenJDK Summary 2007 JavaOneSM Conference | Session TS-7820 | 4 Apache Harmony In the Beginning May 2005—founded in the Apache Incubator Primary Goals 1. Compatible, independent implementation of Java™ Platform, Standard Edition (Java SE platform) under the Apache License 2. Community-developed, modular architecture allowing sharing and independent innovation 3. Protect IP rights of ecosystem 2007 JavaOneSM Conference | Session TS-7820 | 5 Apache Harmony Early history: 2005 Broad community discussion • Technical issues • Legal and IP issues • Project governance issues Goal: Consolidation and Consensus 2007 JavaOneSM Conference | Session TS-7820 | 6 Early History Early history: 2005/2006 Initial Code Contributions • Three Virtual machines ● JCHEVM, BootVM, DRLVM • Class Libraries ● Core classes, VM interface, test cases ● Security, beans, regex, Swing, AWT ● RMI and math 2007 JavaOneSM Conference | Session TS-7820 | -
Lecture 10: Fault Tolerance Fault Tolerant Concurrent Computing
02/12/2014 Lecture 10: Fault Tolerance Fault Tolerant Concurrent Computing • The main principles of fault tolerant programming are: – Fault Detection - Knowing that a fault exists – Fault Recovery - having atomic instructions that can be rolled back in the event of a failure being detected. • System’s viewpoint it is quite possible that the fault is in the program that is attempting the recovery. • Attempting to recover from a non-existent fault can be as disastrous as a fault occurring. CA463 Lecture Notes (Martin Crane 2014) 26 1 02/12/2014 Fault Tolerant Concurrent Computing (cont’d) • Have seen replication used for tasks to allow a program to recover from a fault causing a task to abruptly terminate. • The same principle is also used at the system level to build fault tolerant systems. • Critical systems are replicated, and system action is based on a majority vote of the replicated sub systems. • This redundancy allows the system to successfully continue operating when several sub systems develop faults. CA463 Lecture Notes (Martin Crane 2014) 27 Types of Failures in Concurrent Systems • Initially dead processes ( benign fault ) – A subset of the processes never even start • Crash model ( benign fault ) – Process executes as per its local algorithm until a certain point where it stops indefinitely – Never restarts • Byzantine behaviour (malign fault ) – Algorithm may execute any possible local algorithm – May arbitrarily send/receive messages CA463 Lecture Notes (Martin Crane 2014) 28 2 02/12/2014 A Hierarchy of Failure Types • Dead process – This is a special case of crashed process – Case when the crashed process crashes before it starts executing • Crashed process – This is a special case of Byzantine process – Case when the Byzantine process crashes, and then keeps staying in that state for all future transitions CA463 Lecture Notes (Martin Crane 2014) 29 Types of Fault Tolerance Algorithms • Robust algorithms – Correct processes should continue behaving thus, despite failures. -
Actor-Based Concurrency by Srinivas Panchapakesan
Concurrency in Java and Actor- based concurrency using Scala By, Srinivas Panchapakesan Concurrency Concurrent computing is a form of computing in which programs are designed as collections of interacting computational processes that may be executed in parallel. Concurrent programs can be executed sequentially on a single processor by interleaving the execution steps of each computational process, or executed in parallel by assigning each computational process to one of a set of processors that may be close or distributed across a network. The main challenges in designing concurrent programs are ensuring the correct sequencing of the interactions or communications between different computational processes, and coordinating access to resources that are shared among processes. Advantages of Concurrency Almost every computer nowadays has several CPU's or several cores within one CPU. The ability to leverage theses multi-cores can be the key for a successful high-volume application. Increased application throughput - parallel execution of a concurrent program allows the number of tasks completed in certain time period to increase. High responsiveness for input/output-intensive applications mostly wait for input or output operations to complete. Concurrent programming allows the time that would be spent waiting to be used for another task. More appropriate program structure - some problems and problem domains are well-suited to representation as concurrent tasks or processes. Process vs Threads Process: A process runs independently and isolated of other processes. It cannot directly access shared data in other processes. The resources of the process are allocated to it via the operating system, e.g. memory and CPU time. Threads: Threads are so called lightweight processes which have their own call stack but an access shared data. -
Mastering Concurrent Computing Through Sequential Thinking
review articles DOI:10.1145/3363823 we do not have good tools to build ef- A 50-year history of concurrency. ficient, scalable, and reliable concur- rent systems. BY SERGIO RAJSBAUM AND MICHEL RAYNAL Concurrency was once a specialized discipline for experts, but today the chal- lenge is for the entire information tech- nology community because of two dis- ruptive phenomena: the development of Mastering networking communications, and the end of the ability to increase processors speed at an exponential rate. Increases in performance come through concur- Concurrent rency, as in multicore architectures. Concurrency is also critical to achieve fault-tolerant, distributed services, as in global databases, cloud computing, and Computing blockchain applications. Concurrent computing through sequen- tial thinking. Right from the start in the 1960s, the main way of dealing with con- through currency has been by reduction to se- quential reasoning. Transforming problems in the concurrent domain into simpler problems in the sequential Sequential domain, yields benefits for specifying, implementing, and verifying concur- rent programs. It is a two-sided strategy, together with a bridge connecting the Thinking two sides. First, a sequential specificationof an object (or service) that can be ac- key insights ˽ A main way of dealing with the enormous challenges of building concurrent systems is by reduction to sequential I must appeal to the patience of the wondering readers, thinking. Over more than 50 years, more sophisticated techniques have been suffering as I am from the sequential nature of human developed to build complex systems in communication. this way. 12 ˽ The strategy starts by designing —E.W. -
Concurrent Computing
Concurrent Computing CSCI 201 Principles of Software Development Jeffrey Miller, Ph.D. [email protected] Outline • Threads • Multi-Threaded Code • CPU Scheduling • Program USC CSCI 201L Thread Overview ▪ Looking at the Windows taskbar, you will notice more than one process running concurrently ▪ Looking at the Windows task manager, you’ll see a number of additional processes running ▪ But how many processes are actually running at any one time? • Multiple processors, multiple cores, and hyper-threading will determine the actual number of processes running • Threads USC CSCI 201L 3/22 Redundant Hardware Multiple Processors Single-Core CPU ALU Cache Registers Multiple Cores ALU 1 Registers 1 Cache Hyper-threading has firmware logic that appears to the OS ALU 2 to contain more than one core when in fact only one physical core exists Registers 2 4/22 Programs vs Processes vs Threads ▪ Programs › An executable file residing in memory, typically in secondary storage ▪ Processes › An executing instance of a program › Sometimes this is referred to as a Task ▪ Threads › Any section of code within a process that can execute at what appears to be simultaneously › Shares the same resources allotted to the process by the OS kernel USC CSCI 201L 5/22 Concurrent Programming ▪ Multi-Threaded Programming › Multiple sections of a process or multiple processes executing at what appears to be simultaneously in a single core › The purpose of multi-threaded programming is to add functionality to your program ▪ Parallel Programming › Multiple sections of -
Download Distributed Systems Free Ebook
DISTRIBUTED SYSTEMS DOWNLOAD FREE BOOK Maarten van Steen, Andrew S Tanenbaum | 596 pages | 01 Feb 2017 | Createspace Independent Publishing Platform | 9781543057386 | English | United States Distributed Systems - The Complete Guide The hope is that together, the system can maximize resources and information while preventing failures, as if one system fails, it won't affect the availability of the service. Banker's algorithm Dijkstra's algorithm DJP algorithm Prim's algorithm Dijkstra-Scholten algorithm Dekker's algorithm generalization Smoothsort Shunting-yard algorithm Distributed Systems marking algorithm Concurrent algorithms Distributed Systems algorithms Deadlock prevention algorithms Mutual exclusion algorithms Self-stabilizing Distributed Systems. Learn to code for free. For the first time computers would be able to send messages to other systems with a local IP address. The messages passed between machines contain forms of data that the systems want to share like databases, objects, and Distributed Systems. Also known as distributed computing and distributed databases, a distributed system is a collection of independent components located on different machines that share messages with each other in order to achieve common goals. To prevent infinite loops, running the code requires some amount of Ether. As mentioned in many places, one of which this great articleyou cannot have consistency and availability without partition tolerance. Because it works in batches jobs a problem arises where if your job fails — Distributed Systems need to restart the whole thing. While in a voting system an attacker need only add nodes to the network which is Distributed Systems, as free access to the network is a design targetin a CPU power based scheme an attacker faces a physical limitation: getting access to more and more powerful hardware. -
Automated Distributed Implementation of Component-Based Models with Priorities Borzoo Bonakdarpour, Marius Bozga, Jean Quilbeuf
Automated Distributed Implementation of Component-Based Models with Priorities Borzoo Bonakdarpour, Marius Bozga, Jean Quilbeuf To cite this version: Borzoo Bonakdarpour, Marius Bozga, Jean Quilbeuf. Automated Distributed Implementation of Component-Based Models with Priorities. 1th International Conference on Embedded Software, EM- SOFT 2011, Oct 2011, Taipei, Taiwan. pp.59-68, 10.1145/2038642.2038654. hal-00722405 HAL Id: hal-00722405 https://hal.archives-ouvertes.fr/hal-00722405 Submitted on 1 Aug 2012 HAL is a multi-disciplinary open access L’archive ouverte pluridisciplinaire HAL, est archive for the deposit and dissemination of sci- destinée au dépôt et à la diffusion de documents entific research documents, whether they are pub- scientifiques de niveau recherche, publiés ou non, lished or not. The documents may come from émanant des établissements d’enseignement et de teaching and research institutions in France or recherche français ou étrangers, des laboratoires abroad, or from public or private research centers. publics ou privés. Automated Distributed Implementation of Component-based Models with Priorities∗ Borzoo Bonakdarpour Marius Bozga Jean Quilbeuf School of Computer Science VERIMAG, Centre Équation VERIMAG, Centre Équation University of Waterloo 2 avenue de Vignate 2 avenue de Vignate 200 University Avenue West 38610, GIÈRES, France 38610, GIÈRES, France Waterloo, Canada, N2L3G1 [email protected] [email protected] [email protected] ABSTRACT and Meanings of Programs]: Specifying and Verifying In this paper, we introduce a novel model-based approach for and Reasoning about Programs—Logic of programs; I.2.2 constructing correct distributed implementation of [Artificial Intelligence ]: Automatic Programming—Pro- component-based models constrained by priorities. -
Empirical Study of Concurrent Programming Paradigms
UNLV Theses, Dissertations, Professional Papers, and Capstones May 2016 Empirical Study of Concurrent Programming Paradigms Patrick M. Daleiden University of Nevada, Las Vegas Follow this and additional works at: https://digitalscholarship.unlv.edu/thesesdissertations Part of the Computer Sciences Commons Repository Citation Daleiden, Patrick M., "Empirical Study of Concurrent Programming Paradigms" (2016). UNLV Theses, Dissertations, Professional Papers, and Capstones. 2660. http://dx.doi.org/10.34917/9112055 This Thesis is protected by copyright and/or related rights. It has been brought to you by Digital Scholarship@UNLV with permission from the rights-holder(s). You are free to use this Thesis in any way that is permitted by the copyright and related rights legislation that applies to your use. For other uses you need to obtain permission from the rights-holder(s) directly, unless additional rights are indicated by a Creative Commons license in the record and/ or on the work itself. This Thesis has been accepted for inclusion in UNLV Theses, Dissertations, Professional Papers, and Capstones by an authorized administrator of Digital Scholarship@UNLV. For more information, please contact [email protected]. EMPIRICAL STUDY OF CONCURRENT PROGRAMMING PARADIGMS By Patrick M. Daleiden Bachelor of Arts (B.A.) in Economics University of Notre Dame, Notre Dame, IN 1990 Master of Business Administration (M.B.A.) University of Chicago, Chicago, IL 1993 A thesis submitted in partial fulfillment of the requirements for the Master of Science in Computer Science Department of Computer Science Howard R. Hughes College of Engineering The Graduate College University of Nevada, Las Vegas May 2016 c Patrick M. -
Mobile OS: Analysis of Customers’ Values
Mobile OS: analysis of customers’ values Georgii Shamugiia Bachelor's Thesis Business Administration 2020 Mobile OS 01.11.2020 Author(s) Georgii Shamugiia Degree programme Bachelor of Business Administration SAMPO18 Report/thesis title Number of pages Mobile OS: analysis of customer's values and appendix pages 14 + 47 The rapid development of the mobile industry since the start of new millennium led to much more extensive usage of mobile devices than desktop computers. Fast-developing technol- ogies of wireless networking led to the excessive need of a device, which can search any info on the web conveniently, be a decent communicational tool and be able to adapt to dif- ferent needs of customers. The device which fully fulfils this need is a smartphone, which is being widely used today by the majority of the global population. In this thesis, the author digests the tools, which allows smartphones to work appropriately, give consumers a pleasuring experience while using them and run all the operations and data stored on them. These tools are mobile op- erating systems – platforms, which allow all those things and even more. In this research, the author investigates the historical development of mobile operating systems, which put a mark in the history of the industry. By digesting three cases of Nokia, Blackberry and Mi- crosoft, the author explains what were the selling points, that succeeded and managed to popularise each mobile operating system globally among consumers and gadget manufac- turers and what were the reasons that caused their global downfall. A separate chapter of this research is dedicated to a survey regarding customer values and customer opinions about mobile devices and mobile operating systems. -
60 Years of Mastering Concurrent Computing Through Sequential Thinking Sergio Rajsbaum, Michel Raynal
60 Years of Mastering Concurrent Computing through Sequential Thinking Sergio Rajsbaum, Michel Raynal To cite this version: Sergio Rajsbaum, Michel Raynal. 60 Years of Mastering Concurrent Computing through Sequential Thinking. ACM SIGACT News, Association for Computing Machinery (ACM), 2020, 51 (2), pp.59-88. 10.1145/3406678.3406690. hal-03162635 HAL Id: hal-03162635 https://hal.archives-ouvertes.fr/hal-03162635 Submitted on 17 Jun 2021 HAL is a multi-disciplinary open access L’archive ouverte pluridisciplinaire HAL, est archive for the deposit and dissemination of sci- destinée au dépôt et à la diffusion de documents entific research documents, whether they are pub- scientifiques de niveau recherche, publiés ou non, lished or not. The documents may come from émanant des établissements d’enseignement et de teaching and research institutions in France or recherche français ou étrangers, des laboratoires abroad, or from public or private research centers. publics ou privés. 60 Years of Mastering Concurrent Computing through Sequential Thinking ∗ Sergio Rajsbaumy, Michel Raynal?;◦ yInstituto de Matemáticas, UNAM, Mexico ?Univ Rennes IRISA, 35042 Rennes, France ◦Department of Computing, Hong Kong Polytechnic University [email protected] [email protected] Abstract Modern computing systems are highly concurrent. Threads run concurrently in shared-memory multi-core systems, and programs run in different servers communicating by sending messages to each other. Concurrent programming is hard because it requires to cope with many possible, unpre- dictable behaviors of the processes, and the communication media. The article argues that right from the start in 1960’s, the main way of dealing with concurrency has been by reduction to sequential reasoning.