Embeddedsparkandada-Web.Pdf
Total Page:16
File Type:pdf, Size:1020Kb
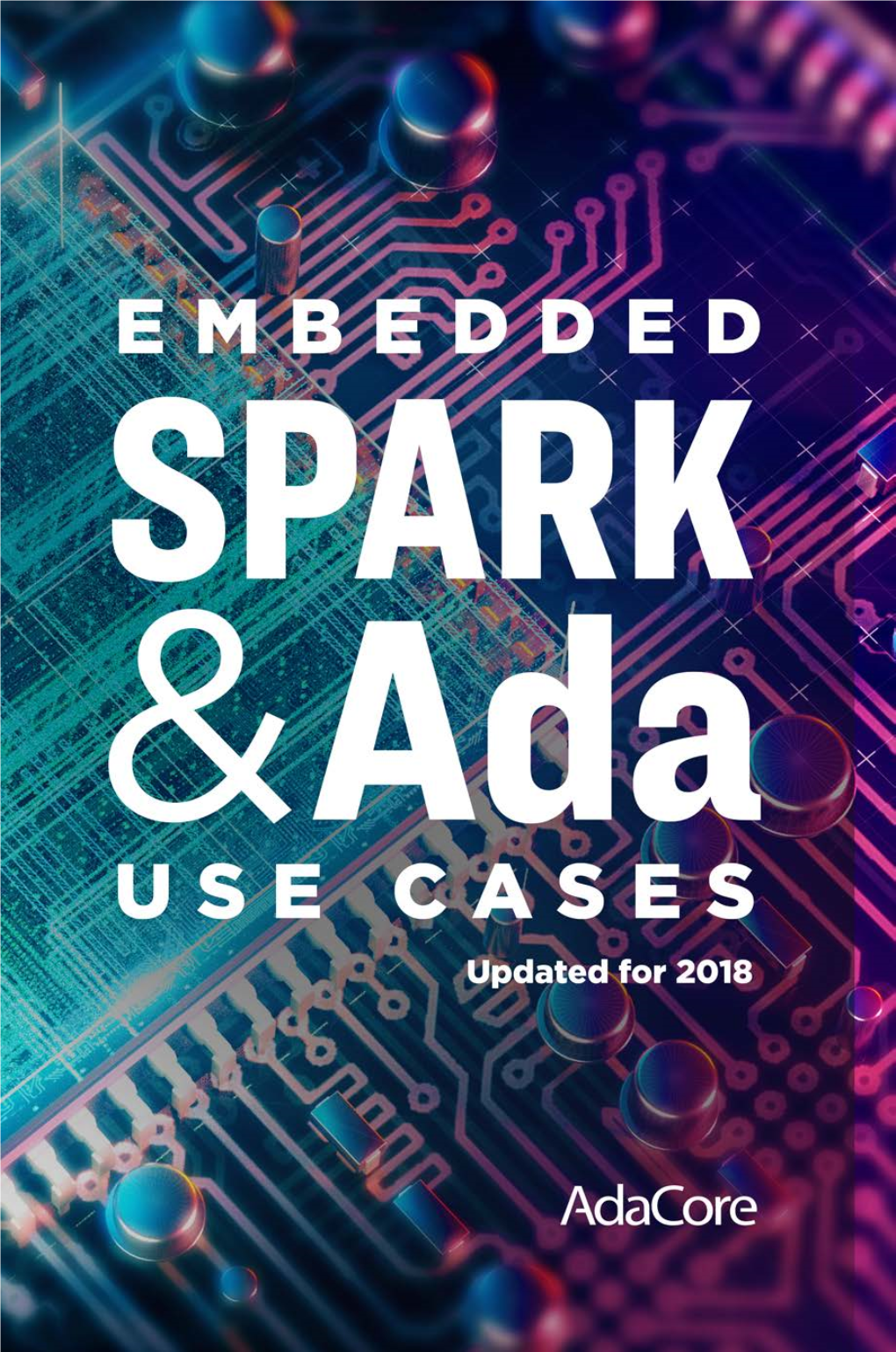
Load more
Recommended publications
-
The BBC Micro:Bit Inside And
The micro:bit… inside and out Joe Finney [email protected] School of Computing and Communications Lancaster University UK [email protected] School of Computing and Communications: InfoLab21 What computers used to look like… (I had one of these!) Commodore 64 ( I had one of these) What computers used to look like… (I didn’t have one of these – but martin did!) Sinclair ZX Spectrum (I didn’t have one of these) And then there was this one too! BBC Model B (Delivered free to every school in the UK in the 1980s) Circa January 2015 Micro:bit Legend Howard Baker “We have some prototypes…” BBC R&D by Michael Sparks early 2015… http://www.bbc.co.uk/rd/blog/2015-07-prototyping-the-bbc-microbit Delivered by a wide partnership More Prototyping… The micro:bit SB1… And More Prototyping… Micro:bits of Legend… In the most unlikely places… Micro:bit SB2 Proof of Concept… In the most unlikely places… Micro:Bit Font is called “Pendolino” for a reason… In the most unlikely places… Micro:bit device drivers largely written at the car park at Cockerham Junior Football Club… A whirlwind of events… But not without its problems… We delivered 22nd March 2016… DEMO: Coding a micro:bit with MakeCode Inside the micro:bit… . 25 LED matrix screen . Light sensor . User definable buttons . 17 Digital input/output . 6 Analog input . 3 PWM output . 3 Touch sensitive . I2C, SPI, UART Inside the micro:bit… . 16MHz ARM Cortex M0 . 16KB RAM, 256K FLASH . USB Storage/Serial/Debug . 3 axis accelerometer . -
Scratch, Blocky & Co., Blocksysteme Zum Programmieren
Notizen Lothar Griess • 26. Mai 2018 Scratch, Blocky & Co., Blocksysteme zum Programmieren Scratch-Alternativen, … https://wiki.scratch.mit.edu/wiki/Alternatives_to_Scratch HTML5 als Grundlage wär besser, die Zukunft von Flash ist unklar. Liste der Modifikationen ... https://scratch-dach.info/wiki/Liste_der_Modifikationen Enchanting (Scratch Modifikation) ... https://scratch-dach.info/wiki/Enchanting_(Scratch_Modifikation) Beetle Blocks ist wie Scratch oder BlocksCAD für 3D-Grafiken... https://scratch-dach.info/wiki/Beetle_Blocks Beetle Blocks GitHub Repository ... https://github.com/ericrosenbaum/BeetleBlocks Beetle Blocks (3D-Design), … http://beetleblocks.com/ Mod Share … z.B.: Supported Scratch-Modifications … https://wiki.scratch.mit.edu/wiki/Mod_Share Scratch … https://wiki.scratch.mit.edu/wiki/Scratch Snap … https://wiki.scratch.mit.edu/wiki/Snap_(Scratch_Modification) Bingo … https://wiki.scratch.mit.edu/wiki/Bingo_(Scratch_Modification) Panther … https://wiki.scratch.mit.edu/wiki/Panther_(Scratch_Modification) Insanity … https://wiki.scratch.mit.edu/wiki/Insanity_(Scratch_Modification) … weitere: Stack, Kitcat, Ghost, Streak • • • Blockly is used by hundreds of projects, most of them educational: ... https://developers.google.com/blockly/ Blockly, RoboBlockly, ... https://code.org/learn Google Education, 1 Stunde, ... https://hourofcode.com/blockly Got PCs with slow (or non-existent) internet access? Download the Blockly tutorials that were the precursor of the Code.org tutorials - a single 3MB ZIP file can be loaded onto any computer or used off a memory stick Blockly Games … https://blockly-games.appspot.com/ App Inventor … http://appinventor.mit.edu/explore/ Code (div.) … https://code.org/ Ozo Blockly (Mini-Roboter) - Ozobot Bit robot using the OzoBlockly editor. … http://ozoblockly.com/ micro:bit (Raspberrs Pi, MicroPython) … http://microbit.org/ BlocklyProp … www.parallax.com/product/program-blocklyprop wonder workshop (dash-Roboter) … www.makewonder.de Robertar, NEPO (div.) … https://lab.open-roberta.org// Made w/ Code (div. -
PDF Tutorial
www.adeept.com Catalogue About Micro bit ............................................................................................................................ - 1 - Project 1 Neopixel ................................................................................................................ - 10 - Rainbow ........................................................................................................................... - 10 - Makecode ................................................................................................................ - 10 - MU microPython .................................................................................................... - 18 - LED Rotate ...................................................................................................................... - 25 - Makecode ................................................................................................................ - 25 - Mu microPython: .................................................................................................. - 27 - Neopixel ........................................................................................................................... - 28 - Makecode ................................................................................................................ - 28 - Mu microPython .................................................................................................... - 28 - Project 2 Motor ..................................................................................................................... -
The Road to ARM an Unfinished Tale
The Road to ARM An unfinished tale Javier Guerra - 2018-09-07 Lua Workshop ‘18 - Kaunas, Lithuania 1 The beginning Acorn Archimedes (1987) - 32 bit ARM-2 - 8MHz - 512KB RAM - 256 colors - 8-channel sound (mono output) 2 Today - Mobile (and laptops) - Raspberry - Embedded - Server 3 Mobile 4 Education Raspberry PI 3: - 4-core ARMv8 1.2GHz - 1GB RAM - WiFi, Bluetooth, Ethernet, USB, HDMI, camera port Raspberry Pi Zero: - 1-core ARMv6 BBC Micro:bit 1GHz - 5 x 4 cm - 512MB RAM - ARM Cortex-M0, 16MHz - 1 µUSB, miniHDMI - 256KB Flash / 16KB RAM - WiFI/Bluetooth (W) - USB, Bluetooth, buttons, LED - Camera port, matrix, accelerometer, compass 40-pin GPIO - 20 GPIO pins edge connector 5 Embedded 6 Embedded 7 Embedded peripherals STM32F030 : - Cortex-M0 - 48 MHz - 16 KB Flash / 4KB SRAM - 5 timers, PWM - SPI, I²C, USART, 15 GPIO pins - 12-bit A/D 8 SERVERS! Qualcomm Centriq 10nm - ARMv8 64-bit 2.5GHz - 46 cores/socket - LLC : 1.25MB/core - 120W 9 CF situation - LuaJIT - Go - C - C++ - Rust - Python - eBPF - JavaScript 10 Docker + qemu-user $ docker run --rm -it stretch-arm64/master:latest root@d955deefbaa4:/# uname -a Linux d955deefbaa4 4.15.0-26-generic #28-Ubuntu SMP Wed Jul 4 16:24:29 UTC 2018 aarch64 GNU/Linux root@d955deefbaa4:/# lscpu Architecture: aarch64 CPU op-mode(s): 32-bit, 64-bit Byte Order: Little Endian CPU(s): 8 On-line CPU(s) list: 0-7 Thread(s) per core: 2 Core(s) per socket: 4 Socket(s): 1 NUMA node(s): 1 Vendor ID: GenuineIntel CPU family: 6 Model: 142 Model name: Intel(R) Core(TM) i7-8550U CPU @ 1.80GHz 11 Stepping: 10 CPU -
BBC Micro:Bit Lesson #0 Created by Carter Nelson
BBC micro:bit Lesson #0 Created by Carter Nelson Last updated on 2018-08-22 04:02:28 PM UTC Guide Contents Guide Contents 2 Intro 3 Lesson Parts 5 Required: 5 Take a Tour! 6 Microcontroller 8 Battery Jack & Supply 9 JST PH 2-Pin Cable - Female Connector 100mm 9 Choosing Battery Power Supply 10 USB Connection 11 Status LED 12 Reset Button 13 Bluetooth Antenna 14 Compass 15 Accelerometer 16 Edge Connector 17 Buttons 19 LED Matrix 20 Pads 21 Let's Code 23 It's Like a USB Thumb Drive! 23 JavaScript Blocks Editor 24 Hello Heart Example 25 Python Editor 31 What Next? 34 © Adafruit Industries https://learn.adafruit.com/bbc-micro-bit-lesson-number-0 Page 2 of 34 Intro So what is this unassuming little board? It's name is the BBC micro:bit (https://adafru.it/yEK), and it is a little computer you can write programs for. It has various gadgets like buttons and lights that you can use to do all kinds of fun stuff. There's even a compass! In this guide we'll briefly go over the main features of the BBC micro:bit, just so you have an idea what's going on. The main web site is at www.microbit.org (https://adafru.it/yEL) where you can go to find more information. The BBC part of the name stands for British Broadcasting Corporation (https://adafru.it/yEM). There's a lot of history (https://adafru.it/yEN) to why the BBC would get involved with making a little computer. -
A Just-In-Time Compiler for the BBC Micro:Bit
A Just-In-Time compiler for the BBC micro:bit Thomas Denney Supervisor: Alex Rogers Word count: 9,989 Trinity 2018 Abstract Cheap, low-powered programmable hardware, such as the BBC micro:bit, has lead to a renaissance of computer science education in schools; however many programming environments used by stu- dents either require the transfer of source code to be interpreted on the device, or the transfer of a much larger, compiled binary. We demonstrate the use of a stack-based intermediate bytecode for programs that can be Just-in- Time (JIT) compiled on a BBC micro:bit to support a broad range of programming languages on a limited device, reduce the transfer requirement, and improve performance by avoiding interpreta- tion. The use of a JIT compiler on such a low-powered device demonstrates the possibility of software updates to sensors or Internet of Things (IoT) devices in environments with extremely limited network connectivity and power. Acknowledgements I am indebted to my supervisor, Professor Alex Rogers. His expert guidance ensured that the project was a success, particularly when it came to performing quantatitve performance measures of my JIT compiler. Our off-topic discussions during our meetings were also an absolute pleasure. I’d also like to thank Steve Hodges and James Scott at Microsoft Research, without whom I wouldn’t have been exposed to embedded programming or the micro:bit. Along with Professor Gavin Lowe, they have been amongst the best mentors that I could have hoped for. Finally, I also owe a debt of gratitude to my friends and family, especially whilst I maintained a quirky — at best — sleep schedule working on this project. -
Quick Start Guide for Teachers
PREVIEW QUICK START GUIDE FOR TEACHERS • Tips and advice for getting to Hi there. grips with the BBC micro:bit • Step-by-step coding Wanna code? challenges with clear solutions • Guidance on creating and sharing your own programs Supported by Foreword Computational thinking is the thought processes involved in formulating a problem and expressing its solution(s) in a way that a computer – human or machine – can carry out effectively. In 2006, I began to advocate that everyone, regardless of profession, of career, or of age, can benefit from learning how to think computationally[1]. With the BBC micro:bit, the BBC and its partners, including Microsoft, catalyze our realization of this dream. Children can learn how to think computationally by first formulating a problem and conceptualizing a solution. Then, by expressing their solution using a code editor, such as Microsoft Touch Develop, and by compiling and running their program on the BBC micro:bit, they can see their code come alive! I commend the BBC and the UK for their leadership in the Make It Digital initiative. Teaching children at an early age the fundamentals of computing helps provide them with the programming skills and the computational thinking skills they will need to function in the 21st century workforce. Programming the BBC micro:bit will teach children basic coding concepts, such as variables, types, procedures, iteration, and conditionals. Solving problems with the BBC micro:bit will expose children to computational thinking skills, such as abstraction, decomposition, pattern matching, algorithm design, and data representation. Students knowledgeable with these skills will be in high demand by all industrial, government, and academic sectors, not just information technology. -
BBC Micro:Bit Connectivity with TI-Nspire™ CX II-T Family Products
FAQ BBC micro:bit connectivity with TI-Nspire™ CX II-T family products Overview In February 2021, version 5.3 calculator operating system (OS) and desktop software updates were released for TI-Nspire™ CX II-T family products. This update introduces OS and software enhancements that enable USB communication with a third-party microcontroller board called the BBC micro:bit. With this update, Python programming with the micro:bit board can be enabled by a module that is available for download from the Texas Instruments (TI) website and installed on the TI-Nspire™ CX II-T graphing calculator and software. What is BBC micro:bit? The BBC micro:bit is a pocket-sized microcontroller board that helps students learn how software and hardware work together. Per their site: “It has an LED light display, buttons, sensors and many input/output features that, when programmed, let it interact with you and your world.” (Front) (Back) (Battery) Globally, there are over four million micro:bit boards distributed across 60 countries with many hardware, content and education partners. While the original micro:bit board is pictured above, there was a new micro:bit version 2, or V2, introduced and made available in November 2020. Key updates with the V2 board include a built-in speaker, a built-in microphone, a capacitive touch sensor, and more memory on board. For a comprehensive overview of the V2 board, visit microbit.org/new-microbit/. The platform bar is a trademark of Texas Instruments. ©2021 Texas Instruments A typical micro:bit use scenario involves coding on a computer with drag-and-drop block code, Python code, or other coding languages, then “flashing” the resulting program onto the micro:bit board which is then disconnected and run while the micro:bit board is untethered from the computer but powered with the connected battery. -
Updating Daplink Firmware and Using REPL with Tera Term
APPENDIX A Updating DAPLink Firmware and Using REPL with Tera Term This appendix explains how to update the DAPLink firmware on the micro:bit by using maintenance mode. It also shows you how to use REPL (Read-Evaluate-Print-Loop) with Tera Term, a serial terminal program. DAPLink Firmware The firmware on the micro:bit is stored inside a separate interface chip called the KL26. This firmware is known as DAPLink. It is the responsibility of this firmware to connect to the USB port and to allow you to drag and drop .hex files that are then programmed into the application processor. If you want to determine what firmware is loaded in the KL26 interface chip, plug it in and open it in your file manager. Then look inside the details.txt file (see Figure A-1). © Pradeeka Seneviratne 2018 207 P. Seneviratne, Beginning BBC micro:bit, https://doi.org/10.1007/978-1-4842-3360-3 APPENDIX A UpdaTinG DAPLinK FiRMWaRe and UsinG REPL With TeRa TeRM Figure A-1. The details.txt file contains firmware information Updating DAPLink Firmware You can download the latest DAPLink firmware from https://github. com/ARMmbed/DAPLink/tags. At the time of this writing, it was version 0244 (https://github.com/ARMmbed/DAPLink/releases/tag/0244). Note You should only update the firmware when there is a new DAPLink version available. The following steps explain how to update the DAPLink firmware on micro:bit. 1. First, bring your micro:bit into maintenance mode. (Read the “Maintenance Mode” section to learn how to bring your micro:bit into maintenance mode.) 208 APPENDIX A UpdaTinG DAPLinK FiRMWaRe and UsinG REPL With TeRa TeRM 2. -
Makecode and CODAL: Intuitive and Efficient Embedded Systems Programming for Education
MakeCode and CODAL: Intuitive and Efficient Embedded Systems Programming for Education James Devine Joe Finney Peli de Halleux Lancaster University, UK Lancaster University, UK Microsoft, USA [email protected] [email protected] [email protected] Michał Moskal Thomas Ball Steve Hodges Microsoft, USA Microsoft, USA Microsoft, UK [email protected] [email protected] [email protected] Abstract Across the globe, it is now commonplace for educators to engage in the making (design and development) of embed- ded systems in the classroom to motivate and excite their students. This new domain brings its own set of unique re- quirements. Historically, embedded systems development requires knowledge of low-level programming languages, lo- Figure 1. Example projects undertaken within education: cal installation of compilation toolchains, device drivers, and Rishworth School sent a micro:bit to space [19] (left); The applications. For students and educators, these requirements micro:bit placed in a custom-built rocket car for teleme- can introduce insurmountable barriers. try [20, 21] (right). We present the motivation, requirements, implementation, and evaluation of a new programming platform that enables novice users to create software for embedded systems. The 1 Introduction platform has two major components: 1) Microsoft MakeCode Recent years have witnessed expansive growth in the popu- (www.makecode.com), a web app that encapsulates an entire larity and ubiquity of embedded systems. This growth can beginner IDE for microcontrollers; and 2) CODAL, an effi- be primarily attributed to the emergence of new application cient component-oriented C++ runtime for microcontrollers. domains ranging from wearables, to home automation, indus- We show how MakeCode and CODAL provide an accessible, trial automation, and smart grids ś a phenomenon broadly cross-platform, installation-free programming experience referred to as the Internet of Things (IoT). -
BBC Micro:Bit Micropython Documentation Release 1.0.1
BBC micro:bit MicroPython Documentation Release 1.0.1 Multiple authors Sep 20, 2021 Tutorials 1 Introduction 3 1.1 Hello, World!...............................................3 1.2 Images..................................................4 1.3 Buttons..................................................9 1.4 Input/Output............................................... 12 1.5 Music................................................... 16 1.6 Random.................................................. 19 1.7 Movement................................................ 21 1.8 Gestures................................................. 23 1.9 Direction................................................. 24 1.10 Storage.................................................. 25 1.11 Speech.................................................. 29 1.12 Network................................................. 36 1.13 Radio................................................... 42 1.14 Next Steps................................................ 45 2 micro:bit Micropython API 47 2.1 The microbit module........................................... 47 3 Microbit Module 53 3.1 Functions................................................. 53 3.2 Attributes................................................. 53 3.3 Classes.................................................. 59 3.4 Modules................................................. 63 4 Audio 75 4.1 Functions................................................. 75 4.2 Classes.................................................. 75 4.3 Using audio............................................... -
This Is a Cute Programmable Robot, You Can Control It with Micro:Bit. The
This is a cute programmable robot, you can control it with micro:bit. The body is assembled, so just plug the micro:bit and then play with it, which is great for you to choose as your entry-level robot. The robot comes with various sensors, such as ultrasonic sensor, touch sensor, sound sensor, buzzer, RGB LED and etc for you to learn about electronics, robot and programming at the same time. There are two ways to program the robot. You can use Microsoft Makecode, an easy way to learn programming. Or, you can use Micropython , which gives you python access to control the robot. The BBC micro:bit is a pocket-sized micro-computer that can be used for all sorts of cool creations, from robots to musical instruments, the possibilities are endless. It can be coded from any web browser in Blocks, Javascript, Python, Scratch, and etc. The micro:bit has 25 individually-programmable LEDs, these 5x5 red LEDs forms a very small section of a screen, it displays information like words, numbers, and pictures through a combination of ON and OFF LEDs. There are two buttons on the front of the micro:bit - button A and button B, you can detect when these buttons are pressed, allowing you to trigger code on the device. You can detect events such as a single press, a double press, and a long press by programming. The LED screen works as a basic light sensor by reversing the LEDs of the screen to become an input, which allows you to detect the ambient light.