HYGIENIC MACROS for JAVASCRIPT a Dissertation Submitted in Partial Satisfaction of the Requirements for the Degree Of
Total Page:16
File Type:pdf, Size:1020Kb
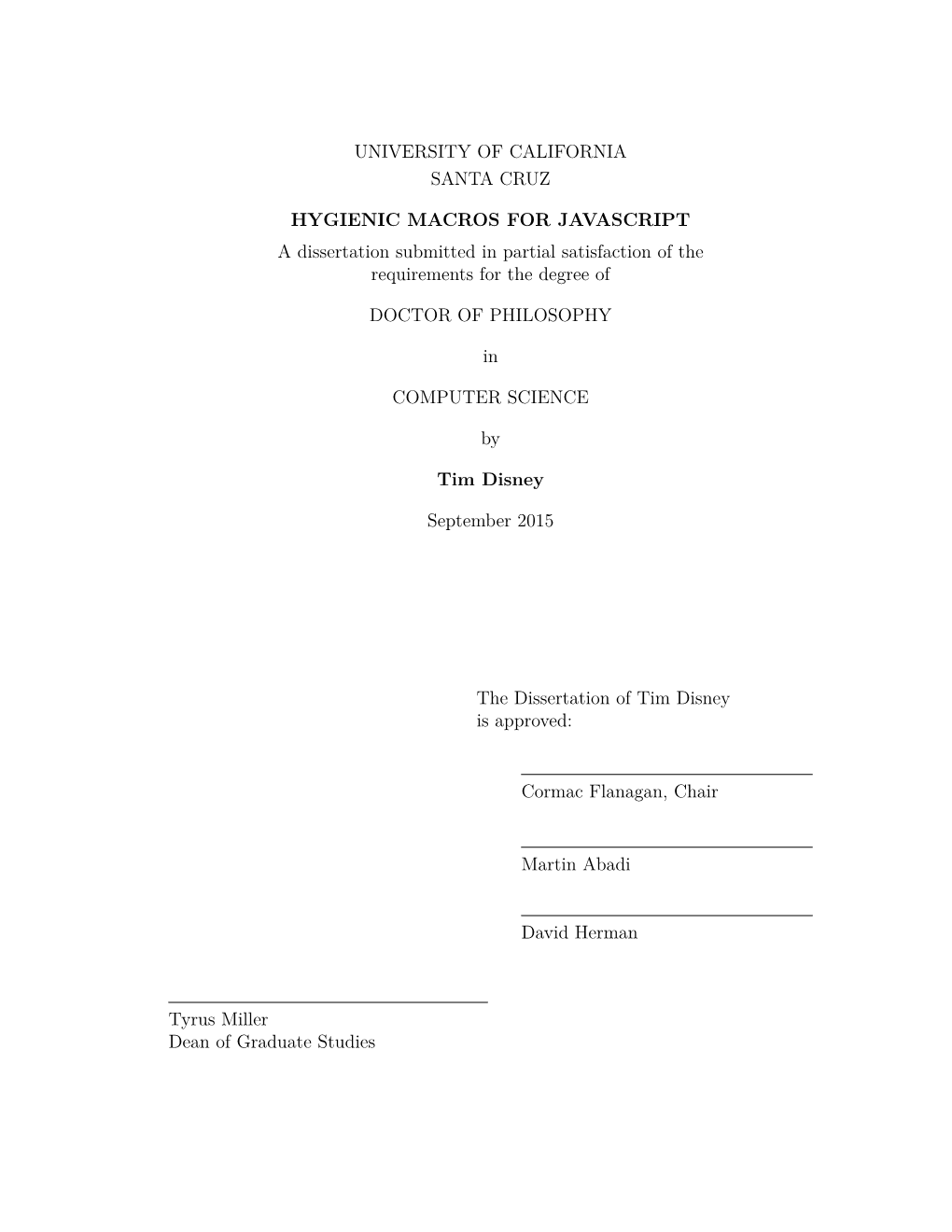
Load more
Recommended publications
-
Processing an Intermediate Representation Written in Lisp
Processing an Intermediate Representation Written in Lisp Ricardo Pena,˜ Santiago Saavedra, Jaime Sanchez-Hern´ andez´ Complutense University of Madrid, Spain∗ [email protected],[email protected],[email protected] In the context of designing the verification platform CAVI-ART, we arrived to the need of deciding a textual format for our intermediate representation of programs. After considering several options, we finally decided to use S-expressions for that textual representation, and Common Lisp for processing it in order to obtain the verification conditions. In this paper, we discuss the benefits of this decision. S-expressions are homoiconic, i.e. they can be both considered as data and as code. We exploit this duality, and extensively use the facilities of the Common Lisp environment to make different processing with these textual representations. In particular, using a common compilation scheme we show that program execution, and verification condition generation, can be seen as two instantiations of the same generic process. 1 Introduction Building a verification platform such as CAVI-ART [7] is a challenging endeavour from many points of view. Some of the tasks require intensive research which can make advance the state of the art, as it is for instance the case of automatic invariant synthesis. Some others are not so challenging, but require a lot of pondering and discussion before arriving at a decision, in order to ensure that all the requirements have been taken into account. A bad or a too quick decision may have a profound influence in the rest of the activities, by doing them either more difficult, or longer, or more inefficient. -
An Optimized R5RS Macro Expander
An Optimized R5RS Macro Expander Sean Reque A thesis submitted to the faculty of Brigham Young University in partial fulfillment of the requirements for the degree of Master of Science Jay McCarthy, Chair Eric Mercer Quinn Snell Department of Computer Science Brigham Young University February 2013 Copyright c 2013 Sean Reque All Rights Reserved ABSTRACT An Optimized R5RS Macro Expander Sean Reque Department of Computer Science, BYU Master of Science Macro systems allow programmers abstractions over the syntax of a programming language. This gives the programmer some of the same power posessed by a programming language designer, namely, the ability to extend the programming language to fit the needs of the programmer. The value of such systems has been demonstrated by their continued adoption in more languages and platforms. However, several barriers to widespread adoption of macro systems still exist. The language Racket [6] defines a small core of primitive language constructs, including a powerful macro system, upon which all other features are built. Because of this design, many features of other programming languages can be implemented through libraries, keeping the core language simple without sacrificing power or flexibility. However, slow macro expansion remains a lingering problem in the language's primary implementation, and in fact macro expansion currently dominates compile times for Racket modules and programs. Besides the typical problems associated with slow compile times, such as slower testing feedback, increased mental disruption during the programming process, and unscalable build times for large projects, slow macro expansion carries its own unique problems, such as poorer performance for IDEs and other software analysis tools. -
The Evolution of Lisp
1 The Evolution of Lisp Guy L. Steele Jr. Richard P. Gabriel Thinking Machines Corporation Lucid, Inc. 245 First Street 707 Laurel Street Cambridge, Massachusetts 02142 Menlo Park, California 94025 Phone: (617) 234-2860 Phone: (415) 329-8400 FAX: (617) 243-4444 FAX: (415) 329-8480 E-mail: [email protected] E-mail: [email protected] Abstract Lisp is the world’s greatest programming language—or so its proponents think. The structure of Lisp makes it easy to extend the language or even to implement entirely new dialects without starting from scratch. Overall, the evolution of Lisp has been guided more by institutional rivalry, one-upsmanship, and the glee born of technical cleverness that is characteristic of the “hacker culture” than by sober assessments of technical requirements. Nevertheless this process has eventually produced both an industrial- strength programming language, messy but powerful, and a technically pure dialect, small but powerful, that is suitable for use by programming-language theoreticians. We pick up where McCarthy’s paper in the first HOPL conference left off. We trace the development chronologically from the era of the PDP-6, through the heyday of Interlisp and MacLisp, past the ascension and decline of special purpose Lisp machines, to the present era of standardization activities. We then examine the technical evolution of a few representative language features, including both some notable successes and some notable failures, that illuminate design issues that distinguish Lisp from other programming languages. We also discuss the use of Lisp as a laboratory for designing other programming languages. We conclude with some reflections on the forces that have driven the evolution of Lisp. -
The Semantics of Syntax Applying Denotational Semantics to Hygienic Macro Systems
The Semantics of Syntax Applying Denotational Semantics to Hygienic Macro Systems Neelakantan R. Krishnaswami University of Birmingham <[email protected]> 1. Introduction body of a macro definition do not interfere with names oc- Typically, when semanticists hear the words “Scheme” or curring in the macro’s arguments. Consider this definition of and “Lisp”, what comes to mind is “untyped lambda calculus a short-circuiting operator: plus higher-order state and first-class control”. Given our (define-syntax and typical concerns, this seems to be the essence of Scheme: it (syntax-rules () is a dynamically typed applied lambda calculus that sup- ((and e1 e2) (let ((tmp e1)) ports mutable data and exposes first-class continuations to (if tmp the programmer. These features expose a complete com- e2 putational substrate to programmers so elegant that it can tmp))))) even be characterized mathematically; every monadically- representable effect can be implemented with state and first- In this definition, even if the variable tmp occurs freely class control [4]. in e2, it will not be in the scope of the variable definition However, these days even mundane languages like Java in the body of the and macro. As a result, it is important to support higher-order functions and state. So from the point interpret the body of the macro not merely as a piece of raw of view of a working programmer, the most distinctive fea- syntax, but as an alpha-equivalence class. ture of Scheme is something quite different – its support for 2.2 Open Recursion macros. The intuitive explanation is that a macro is a way of defining rewrites on abstract syntax trees. -
What Macros Are and How to Write Correct Ones
What Macros Are and How to Write Correct Ones Brian Goslinga University of Minnesota, Morris 600 E. 4th Street Morris, Minnesota 56267 [email protected] ABSTRACT such as Java and Scheme. There arise situations in most Macros are a powerful programming construct found in some high-level languages where code falls short of this ideal by programming languages. Macros can be thought of a way having redundancy and complexity not inherent to the prob- to define an abbreviation for some source code by providing lem being solved. Common abstraction mechanisms, such as a program that will take the abbreviated source code and functions and objects, are often unable to solve the problem return the unabbreviated version of it. In essence, macros [6]. Languages like Scheme provide macros to handle these enable the extension of the programming language by the situations. By using macros when appropriate, code can be programmer, thus allowing for compact programs. declarative, and thus easier to read, write, and understand. Although very powerful, macros can introduce subtle and Macros can be thought of as providing an abbreviation for hard to find errors in programs if the macro is not written common source code in a program. In English, an abbrevi- correctly. Some programming languages provide a hygienic ation (UMM) only makes sense if one knows how to expand macro system to ensure that macros written in that pro- it into what it stands for (University of Minnesota, Morris). gramming language do not result in these sort of errors. Likewise, macros undergo macro expansion to derive their In a programming language with a hygienic macro system, meaning. -
Types of Macros • Short for Macroinstruction
9/16/14 CS152 – Advanced Programming Language Principles Prof. Tom Austin, Fall 2014 For next class, install LaTeX Macros (pronounced "LAH-tech") What is a macro? Types of macros • Short for macroinstruction. • A rule or pattern that specifies how a Text substitution certain input sequence should be mapped to a replacement sequence. Procedural macros Syntactic macros Text Substitution Macros A Review of Compilers This type of macro works by expanding text. Fast, but limited power. Some examples: source Lexer/ tokens Parser code Tokenizer • C preprocessor • Embedded languages (PHP, Ruby's erb, etc.) Abstract are similar, but more powerful Compiler Interpreter Syntax Tree (AST) Machine code Commands 1 9/16/14 Some variants work at the token level, but the C preprocessor example concept is the same. #define PI 3.14159 expanded source Pre- code Lexer/ tokens code Parser processor Tokenizer #define SWAP(a,b) {int tmp=a;a=b;b=tmp;} int main(void) { Abstract Compiler Interpreter int x=4, y=5, diam=7, circum=diam*PI; Syntax Tree SWAP(x,y); (AST) } Machine code Commands int main(void) { Procedural macros int x=4, y=5, diam=7, circum=diam*PI; SWAP(x,y); Preprocessor Procedural macros execute preprocessor } statements at compilation time. • Allows macros to be written in a procedural language. int main(void) { int x=4, y=5, diam=7, • More powerful than text substitution… circum=diam*3.14159; • …but slower and more complex compiler. {int tmp=x;x=y;y=tmp;}; } Syntactic macros Macro expansion process • Work at the level of abstract syntax trees Abstract Abstract Macro • From the Lisp family (including Scheme) Syntax Tree Syntax Tree Expander – Why Lisp? Because Lisp programs are ASTs (AST) (AST) • Powerful, but expensive • Where our attention will be focused Essentially this is a source-to-source compiler 2 9/16/14 Many macro systems suffer from a problem with inadvertent variable capture. -
CSE341: Programming Languages Lecture 15 Macros
CSE341: Programming Languages Lecture 15 Macros Dan Grossman Winter 2013 What is a macro • A macro definition describes how to transform some new syntax into different syntax in the source language • A macro is one way to implement syntactic sugar – “Replace any syntax of the form e1 andalso e2 with if e1 then e2 else false” • A macro system is a language (or part of a larger language) for defining macros • Macro expansion is the process of rewriting the syntax for each macro use – Before a program is run (or even compiled) Winter 2013 CSE341: Programming Languages 2 Using Racket Macros • If you define a macro m in Racket, then m becomes a new special form: – Use (m …) gets expanded according to definition • Example definitions (actual definitions coming later): – Expand (my-if e1 then e2 else e3) to (if e1 e2 e3) – Expand (comment-out e1 e2) to e2 – Expand (my-delay e) to (mcons #f (lambda () e)) Winter 2013 CSE341: Programming Languages 3 Example uses It is like we added keywords to our language – Other keywords only keywords in uses of that macro – Syntax error if keywords misused – Rewriting (“expansion”) happens before execution (my-if x then y else z) ; (if x y z) (my-if x then y then z) ; syntax error (comment-out (car null) #f) (my-delay (begin (print "hi") (foo 15))) Winter 2013 CSE341: Programming Languages 4 Overuse Macros often deserve a bad reputation because they are often overused or used when functions would be better When in doubt, resist defining a macro? But they can be used well Winter 2013 CSE341: Programming Languages -
Writing Hygienic Macros in Scheme with Syntax-Case
Writing Hygienic Macros in Scheme with Syntax-Case R. Kent Dybvig Indiana University Computer Science Department Bloomington, IN 47405 [email protected] Copyright c 1992 R. Kent Dybvig August 1992 Abstract This article describes a pattern-based hygienic macro system for Scheme and provides numerous examples of its use. Macros defined using this system are automatically hygienic and referentially transparent. Unlike earlier hygienic macro systems, this system does not require \low level" macros to be written in a different style from \high level" macros. In particular, automatic hygiene, referential transparency, and the ability to use patterns extend to all macro definitions, and there is never any need to explicitly manipulate syntactic environments of any kind. The macro system also supplies a hygiene-preserving mechanism for controlled variable capture, allowing macros to introduce implicit identifier bindings or references. 1 Introduction This article describes a hygienic macro system for Scheme that is similar to the one documented in an appendix to the \Revised4 Report on the Algorithmic Language Scheme" [2], with several important differences. Most importantly, there is no practical distinction in this system between \high level" and \low level" macros. Although some macros will not take advantage of the more primitive operators, there is no penalty in terms of loss of automatic hygiene, referential trans- parency, or ease-of-use for those macros that do. In particular, the same pattern matching facilities that were previously available only to high-level macros can now be used in any context. There is never any need to completely rewrite a macro originally written in a high-level style simply because it now requires access to some more primitive feature. -
Using TEX's Language Within a Course About Functional
Jean-Michel Hufflen EUROTEX 2009 71 Using TEX’s Language within a Course about Functional Programming Abstract functions’ application, whereas imperative program- We are in charge of a teaching unit, entitled Advanced ming—the paradigm implemented within more ‘tra- Functional Programming, for 4th-year university ditional’ languages, such as C [16]—emphasises students in Computer Science. This unit is optional changes in state. Many universities include courses within the curriculum, so students attending it are about functional programming, examples being re- especially interested in programming. The main ported in [35]. Besides, such languages are some- language studied in this unit is Scheme, but an important part is devoted to general features, e.g., times taught as first programming languages, ac- lexical vs dynamic scoping, limited vs unlimited extent, cording to an approach comparable to [1, 8, 32] in call by value vs call by name or need, etc. As an the case of Scheme. alternative to other programming languages, TEX Let us remark some tools developed as part of allows us to show a language where dynamic and TEX’s galaxy have already met functional program- lexical scoping—\def vs \edef—coexist. In addition, ming: cl-bibtex [18], an extension of BIBTEX— we can show how dynamic scoping allows users to the bibliography processor [26] usually associated customise T X’s behaviour. Other commands related E with the LaTEX word processor [20]—is written us- to strategies are shown, too, e.g., \expandafter, ◦ ANSI2 OMMON ISP xındy \noexpand. More generally, TEX commands are ing C L [7]; , a multilin- related to macros in more classical programming gual index processor for documents written using languages, and we can both emphasise difficulty LaTEX [24, § 11.3] is based on COMMON LISP, too; related to macros and shown non-artificial examples. -
Macros That Work Together Compile-Time Bindings, Partial Expansion, and Definition Contexts
ZU064-05-FPR expmodel 27 March 2012 12:33 Under consideration for publication in J. Functional Programming 1 Macros that Work Together Compile-Time Bindings, Partial Expansion, and Definition Contexts Matthew Flatt1, Ryan Culpepper1, David Darais1, and Robert Bruce Findler2 1University of Utah 2Northwestern University Abstract Racket is a large language that is built mostly within itself. Unlike the usual approach taken by non-Lisp languages, the self-hosting of Racket is not a matter of bootstrapping one implementation through a previous implementation, but instead a matter of building a tower of languages and libraries via macros. The upper layers of the tower include a class system, a component system, pedagogic variants of Scheme, a statically typed dialect of Scheme, and more. The demands of this language- construction effort require a macro system that is substantially more expressive than previous macro systems. In particular, while conventional Scheme macro systems handle stand-alone syntactic forms adequately, they provide weak support for macros that share information or macros that use existing syntactic forms in new contexts. This paper describes and models features of the Racket macro system, including support for general compile-time bindings, sub-form expansion and analysis, and environment management. The presentation assumes a basic familiarity with Lisp-style macros, and it takes for granted the need for macros that respect lexical scope. The model, however, strips away the pattern and template system that is normally associated with Scheme macros, isolating a core that is simpler, can support pattern and template forms themselves as macros, and generalizes naturally to Racket’s other extensions. -
An Optimized R5RS Macro Expander
Brigham Young University BYU ScholarsArchive Theses and Dissertations 2013-02-05 An Optimized R5RS Macro Expander Sean P. Reque Brigham Young University - Provo Follow this and additional works at: https://scholarsarchive.byu.edu/etd Part of the Computer Sciences Commons BYU ScholarsArchive Citation Reque, Sean P., "An Optimized R5RS Macro Expander" (2013). Theses and Dissertations. 3509. https://scholarsarchive.byu.edu/etd/3509 This Thesis is brought to you for free and open access by BYU ScholarsArchive. It has been accepted for inclusion in Theses and Dissertations by an authorized administrator of BYU ScholarsArchive. For more information, please contact [email protected], [email protected]. An Optimized R5RS Macro Expander Sean Reque A thesis submitted to the faculty of Brigham Young University in partial fulfillment of the requirements for the degree of Master of Science Jay McCarthy, Chair Eric Mercer Quinn Snell Department of Computer Science Brigham Young University February 2013 Copyright c 2013 Sean Reque All Rights Reserved ABSTRACT An Optimized R5RS Macro Expander Sean Reque Department of Computer Science, BYU Master of Science Macro systems allow programmers abstractions over the syntax of a programming language. This gives the programmer some of the same power posessed by a programming language designer, namely, the ability to extend the programming language to fit the needs of the programmer. The value of such systems has been demonstrated by their continued adoption in more languages and platforms. However, several barriers to widespread adoption of macro systems still exist. The language Racket [6] defines a small core of primitive language constructs, including a powerful macro system, upon which all other features are built. -
Beyond Notations: Hygienic Macro Expansion for Theorem Proving Languages
Beyond Notations: Hygienic Macro Expansion for Theorem Proving Languages Sebastian Ullrich1 and Leonardo de Moura2 1 Karlsruhe Institute of Technology, Germany [email protected] 2 Microsoft Research, USA [email protected] Abstract. In interactive theorem provers (ITPs), extensible syntax is not only crucial to lower the cognitive burden of manipulating complex mathematical objects, but plays a critical role in developing reusable abstractions in libraries. Most ITPs support such extensions in the form of restrictive “syntax sugar” substitutions and other ad hoc mechanisms, which are too rudimentary to support many desirable abstractions. As a result, libraries are littered with unnecessary redundancy. Tactic lan- guages in these systems are plagued by a seemingly unrelated issue: accidental name capture, which often produces unexpected and counterin- tuitive behavior. We take ideas from the Scheme family of programming languages and solve these two problems simultaneously by proposing a novel hygienic macro system custom-built for ITPs. We further describe how our approach can be extended to cover type-directed macro expan- sion resulting in a single, uniform system offering multiple abstraction levels that range from supporting simplest syntax sugars to elaboration of formerly baked-in syntax. We have implemented our new macro system and integrated it into the upcoming version (v4) of the Lean theorem prover. Despite its expressivity, the macro system is simple enough that it can easily be integrated into other systems. 1 Introduction Mixfix notation systems have become an established part of many modern ITPs for attaching terse and familiar syntax to functions and predicates of arbitrary arity. _`_:_= Typing Agda Notation"Ctx ` E:T" := (Typing CtxET).