GTI More Complexity
Total Page:16
File Type:pdf, Size:1020Kb
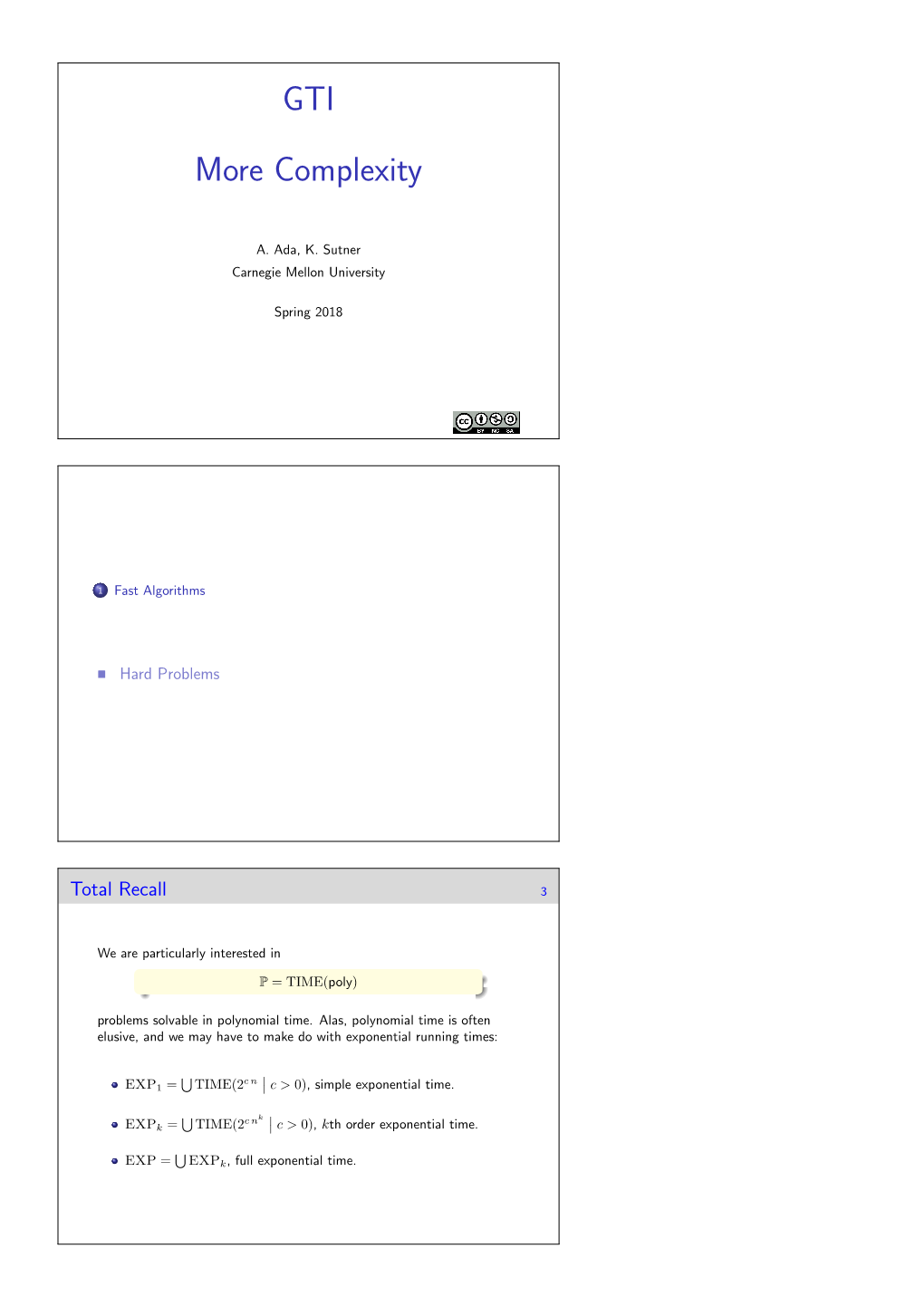
Load more
Recommended publications
-
Coq in a Hurry Yves Bertot
Coq in a Hurry Yves Bertot To cite this version: Yves Bertot. Coq in a Hurry. Types Summer School, also used at the University of Nice Goteborg, Nice, 2006. inria-00001173v2 HAL Id: inria-00001173 https://cel.archives-ouvertes.fr/inria-00001173v2 Submitted on 24 May 2006 (v2), last revised 19 Oct 2017 (v6) HAL is a multi-disciplinary open access L’archive ouverte pluridisciplinaire HAL, est archive for the deposit and dissemination of sci- destinée au dépôt et à la diffusion de documents entific research documents, whether they are pub- scientifiques de niveau recherche, publiés ou non, lished or not. The documents may come from émanant des établissements d’enseignement et de teaching and research institutions in France or recherche français ou étrangers, des laboratoires abroad, or from public or private research centers. publics ou privés. Coq in a Hurry Yves Bertot May 24, 2006 These notes provide a quick introduction to the Coq system and show how it can be used to define logical concepts and functions and reason about them. It is designed as a tutorial, so that readers can quickly start their own experiments, learning only a few of the capabilities of the system. A much more comprehensive study is provided in [1], which also provides an extensive collection of exercises to train on. 1 Expressions and logical formulas The Coq system provides a language in which one handles formulas, verify that they are well-formed, and prove them. Formulas may also contain functions and limited forms of computations are provided for these functions. The first thing you need to know is how you can check whether a formula is well- formed. -
Computation and Reflection in Coq And
Computation and reflection in Coq and HOL John Harrison Intel Corporation, visiting Katholieke Universiteit Nijmegen 20th August 2003 0 What is reflection? Stepping back from the straightforward use of a formal system and reasoning about it (‘reflecting upon it’). • Exploiting the syntax to prove general/meta theorems • Asserting consistency or soundness Similar concept in programming where programs can examine and modify their own syntax [Brian Cantwell Smith 1984]. The ‘reflection principle’ in ZF set theory is rather different. 1 Logical power of reflection The process of reflection may involve: • Adding new theorems that were not provable before • Adding a new, but conservative, rule to the logic • Making no extension of the logic [Giunchiglia and Smail 1989] use ‘reflection principles’ to refer to logic-strengthening rules only, but use ‘reflection’ to describe the process in all cases. 2 Reflection principles The classic reflection principle is an assertion of consistency. More generally, we can assert the ‘local reflection schema’: ⊢ Pr(pφq) ⇒ φ By Godel’s¨ Second Incompleteness Theorem, neither is provable in the original system, so this makes the system stronger. The addition of reflection principles can then be iterated transfinitely [Turing 1939], [Feferman 1962], [Franzen´ 2002]. 3 A conservative reflection rule Consider instead the following reflection rule: ⊢ Pr(pφq) ⊢ φ Assuming that the original logic is Σ-sound, this is a conservative extension. On the other hand, it does make some proofs much shorter. Whether it makes any interesting ones shorter is another matter. 4 Total internal reflection We can exploit the syntax-semantics connection without making any extensions to the logical system. -
A Complete Proof of Coq Modulo Theory
EPiC Series in Computing Volume 46, 2017, Pages 474–489 LPAR-21. 21st International Conference on Logic for Programming, Artificial Intelligence and Reasoning Coq without Type Casts: A Complete Proof of Coq Modulo Theory Jean-Pierre Jouannaud1;2 and Pierre-Yves Strub2 1 INRIA, Project Deducteam, Université Paris-Saclay, France 2 LIX, École Polytechnique, France Abstract Incorporating extensional equality into a dependent intensional type system such as the Calculus of Constructions provides with stronger type-checking capabilities and makes the proof development closer to intuition. Since strong forms of extensionality lead to undecidable type-checking, a good trade-off is to extend intensional equality with a decidable first-order theory T , as done in CoqMT, which uses matching modulo T for the weak and strong elimination rules, we call these rules T -elimination. So far, type-checking in CoqMT is known to be decidable in presence of a cumulative hierarchy of universes and weak T -elimination. Further, it has been shown by Wang with a formal proof in Coq that consistency is preserved in presence of weak and strong elimination rules, which actually implies consistency in presence of weak and strong T -elimination rules since T is already present in the conversion rule of the calculus. We justify here CoqMT’s type-checking algorithm by showing strong normalization as well as the Church-Rosser property of β-reductions augmented with CoqMT’s weak and strong T -elimination rules. This therefore concludes successfully the meta-theoretical study of CoqMT. Acknowledgments: to the referees for their careful reading. 1 Introduction Type checking for an extensional type theory being undecidable, as is well-known since the early days of Martin-Löf’s type theory [16], most proof assistants implement an intensional type theory. -
Calculi for Program Incorrectness and Arithmetic Philipp Rümmer
Thesis for the Degree of Doctor of Philosophy Calculi for Program Incorrectness and Arithmetic Philipp R¨ummer Department of Computer Science and Engineering Chalmers University of Technology and G¨oteborg University SE-412 96 G¨oteborg Sweden G¨oteborg, 2008 Calculi for Program Incorrectness and Arithmetic Philipp R¨ummer ISBN 978-91-628-7242-7 c Philipp R¨ummer, 2008 Technical Report no. 50D Department of Computer Science and Engineering Research Group: Formal Methods Department of Computer Science and Engineering Chalmers University of Technology and G¨oteborg University SE-412 96 G¨oteborg, Sweden Telephone +46 (0)31–772 1000 Printed at Chalmers, G¨oteborg, 2008 II Abstract This thesis is about the development and usage of deductive methods in two main areas: (i) the deductive dis-verification of programs, i.e., how techniques for deductive verification of programs can be used to detect program defects, and (ii) reasoning modulo integer arithmetic, i.e., how to prove the validity (and, in special cases, satisfiability) of first-order formulae that involve integer arithmetic. The areas of program verification and of testing are traditionally considered as complementary: the former searches for a formal proof of program correctness, while the latter searches for witnesses of program incorrectness. Nevertheless, de- ductive verification methods can discover bugs indirectly: the failure to prove the absence of bugs is interpreted as a sign for the incorrectness of the program. This approach is bound to produce “false positives” and bugs can be reported also for correct programs. To overcome this problem, I investigate how techniques that are normally used for verification can be used to directly prove the incorrectness of programs. -
Presburger Arithmetic: Cooper’S Algorithm Top- Ics in Em- Michael R
02917 Ad- 02917 Advanced Topics in Embedded Systems vanced Presburger Arithmetic: Cooper’s algorithm Top- ics in Em- Michael R. Hansen bed- ded Sys- tems Michael R. Hansen 1 DTU Informatics, Technical University of Denmark Presburger Arithmetic: Cooper’s algorithm MRH 17/06/2010 Introduction 02917 Ad- vanced Presburger Arithmetic (introduced by Mojzesz Presburger in 1929), is Top- the first-order theory of natural numbers with addition. ics in Em- Examples of formulas are: ∃x.2x = y and ∃x.∀y.x + y > z. bed- ded Unlike Peano Arithmetic, which also includes multiplication, Sys- Presburger Arithmetic is a decidable theory. tems Michael R. Hansen We shall consider the algorithm introduced by D.C Cooper in 1972. The presentation is based on: Chapter 7: Quantified Linear Arithmetic of The Calculus of Computation by Bradley and Manna. 2 DTU Informatics, Technical University of Denmark Presburger Arithmetic: Cooper’s algorithm MRH 17/06/2010 Introduction 02917 Ad- vanced Presburger Arithmetic (introduced by Mojzesz Presburger in 1929), is Top- the first-order theory of natural numbers with addition. ics in Em- Examples of formulas are: ∃x.2x = y and ∃x.∀y.x + y > z. bed- ded Unlike Peano Arithmetic, which also includes multiplication, Sys- Presburger Arithmetic is a decidable theory. tems Michael R. Hansen We shall consider the algorithm introduced by D.C Cooper in 1972. The presentation is based on: Chapter 7: Quantified Linear Arithmetic of The Calculus of Computation by Bradley and Manna. 3 DTU Informatics, Technical University of Denmark Presburger Arithmetic: Cooper’s algorithm MRH 17/06/2010 Introduction 02917 Ad- vanced Presburger Arithmetic (introduced by Mojzesz Presburger in 1929), is Top- the first-order theory of natural numbers with addition. -
Decidability and Decision Procedures –Some Historical Notes–
Satisfiability Checking Decidability and Decision Procedures –Some Historical Notes– Prof. Dr. Erika Ábrahám RWTH Aachen University Informatik 2 LuFG Theory of Hybrid Systems WS 19/20 Satisfiability Checking — Prof. Dr. Erika Ábrahám (RWTH Aachen University) WS 19/20 1 / 26 Propositional logic decidable SAT-solving Equational logic decidable SAT-encoding Equational logic with uninterpr. functions decidable SAT-encoding Linear real algebra (R with +) decidable Simplex Real algebra (R with + and ∗) decidable CAD virtual substitution Presburger arithmetic (N with +) decidable branch and bound, Omega test Peano arithmetic (N with + and ∗) undecidable - But actually what does it mean “decidable” or “undecidable”? FO theories and their decidability Some first-order theories: Logic decidability algorithm Satisfiability Checking — Prof. Dr. Erika Ábrahám (RWTH Aachen University) WS 19/20 2 / 26 decidable SAT-solving Equational logic decidable SAT-encoding Equational logic with uninterpr. functions decidable SAT-encoding Linear real algebra (R with +) decidable Simplex Real algebra (R with + and ∗) decidable CAD virtual substitution Presburger arithmetic (N with +) decidable branch and bound, Omega test Peano arithmetic (N with + and ∗) undecidable - But actually what does it mean “decidable” or “undecidable”? FO theories and their decidability Some first-order theories: Logic decidability algorithm Propositional logic Satisfiability Checking — Prof. Dr. Erika Ábrahám (RWTH Aachen University) WS 19/20 2 / 26 SAT-solving Equational logic decidable SAT-encoding Equational logic with uninterpr. functions decidable SAT-encoding Linear real algebra (R with +) decidable Simplex Real algebra (R with + and ∗) decidable CAD virtual substitution Presburger arithmetic (N with +) decidable branch and bound, Omega test Peano arithmetic (N with + and ∗) undecidable - But actually what does it mean “decidable” or “undecidable”? FO theories and their decidability Some first-order theories: Logic decidability algorithm Propositional logic decidable Satisfiability Checking — Prof. -
Presburger Arithmetic with Threshold Counting Quantifiers Is Easy
Presburger arithmetic with threshold counting quantifiers is easy Dmitry Chistikov ! Centre for Discrete Mathematics and its Applications (DIMAP) & Department of Computer Science, University of Warwick, Coventry, UK Christoph Haase ! Department of Computer Science, University of Oxford, Oxford, UK Alessio Mansutti ! Department of Computer Science, University of Oxford, Oxford, UK Abstract We give a quantifier elimination procedures for the extension of Presburger arithmetic with aunary threshold counting quantifier ∃≥cy that determines whether the number of different y satisfying some formula is at least c ∈ N, where c is given in binary. Using a standard quantifier elimination procedure for Presburger arithmetic, the resulting theory is easily seen to be decidable in 4ExpTime. Our main contribution is to develop a novel quantifier-elimination procedure for a more general counting quantifier that decides this theory in 3ExpTime, meaning that it is no harder to decide than standard Presburger arithmetic. As a side result, we obtain an improved quantifier elimination procedure for Presburger arithmetic with counting quantifiers as studied by Schweikardt [ACM Trans. Comput. Log., 6(3), pp. 634-671, 2005], and a 3ExpTime quantifier-elimination procedure for Presburger arithmetic extended with a generalised modulo counting quantifier. 2012 ACM Subject Classification Theory of computation → Logic Keywords and phrases Presburger arithmetic, counting quantifiers, quantifier elimination Funding This work is part of a project that has received funding from the European Research Council (ERC) under the European Union’s Horizon 2020 research and innovation programme (Grant agreement No. 852769, ARiAT). 1 Introduction Counting the number of solutions to an equation, or the number of elements in a set subject to constraints, is a fundamental problem in mathematics and computer science. -
A Decidable Fragment of Second Order Logic with Applications to Synthesis
A Decidable Fragment of Second Order Logic With Applications to Synthesis P. Madhusudan University of Illinois, Urbana Champaign, Urbana, IL, USA [email protected] Umang Mathur University of Illinois, Urbana Champaign, Urbana, IL, USA [email protected] https://orcid.org/0000-0002-7610-0660 Shambwaditya Saha University of Illinois, Urbana Champaign, Urbana, IL, USA [email protected] Mahesh Viswanathan University of Illinois, Urbana Champaign, Urbana, IL, USA [email protected] Abstract We propose a fragment of many-sorted second order logic called EQSMT and show that checking satisfiability of sentences in this fragment is decidable. EQSMT formulae have an ∃∗∀∗ quan- tifier prefix (over variables, functions and relations) making EQSMT conducive for modeling synthesis problems. Moreover, EQSMT allows reasoning using a combination of background the- ories provided that they have a decidable satisfiability problem for the ∃∗∀∗ FO-fragment (e.g., linear arithmetic). Our decision procedure reduces the satisfiability of EQSMT formulae to satis- fiability queries of ∃∗∀∗ formulae of each individual background theory, allowing us to use existing efficient SMT solvers supporting ∃∗∀∗ reasoning for these theories; hence our procedure can be seen as effectively quantified SMT (EQSMT ) reasoning. 2012 ACM Subject Classification Theory of computation → Logic → Logic and verification Keywords and phrases second order logic, synthesis, decidable fragment Digital Object Identifier 10.4230/LIPIcs.CSL.2018.30 Funding This work has been supported by NSF grants 1422798, 1329991, 1138994 and 1527395. 1 Introduction The goal of program synthesis is to automatically construct a program that satisfies a given arXiv:1712.05513v3 [cs.LO] 27 Sep 2018 specification. This problem has received a lot of attention from the research community in recent years [34, 4, 14]. -
Arithmetic, First-Order Logic, and Counting Quantifiers
Arithmetic, First-Order Logic, and Counting Quantifiers NICOLE SCHWEIKARDT Humboldt-Universitat¨ Berlin, Germany This paper gives a thorough overview of what is known about first-order logic with counting quantifiers and with arithmetic predicates. As a main theorem we show that Presburger arithmetic is closed under unary counting quantifiers. Precisely, this means that for every first-order formula '(y; ~z) over the signature f<; +g there is a first-order formula (x; ~z) which expresses over the structure hN; <; +i (respectively, over initial segments of this structure) that the variable x is interpreted exactly by the number of possible interpretations of the variable y for which the formula '(y; ~z) is satisfied. Applying this theorem, we obtain an easy proof of Ruhl's result that reachability (and similarly, connectivity) in finite graphs is not expressible in first-order logic with unary counting quantifiers and addition. Furthermore, the above result on Presburger arithmetic helps to show the failure of a particular version of the Crane Beach conjecture. Categories and Subject Descriptors: F.4.1 [Mathematical Logic and Formal Languages]: Mathematical Logic General Terms: Theory, Languages Additional Key Words and Phrases: counting quantifiers, first-order logic, Presburger arithmetic, quantifier elimination 1. INTRODUCTION In computational complexity theory the complexity of a problem is measured by the amount of time or space resources that are necessary for solving a problem on an (idealized) com- putational device such as a Turing machine. Fagin’s seminal work tied this computational complexity to the descriptive complexity, i.e., to the complexity (or, the richness) of a logic that is capable of describing the problem. -
Gödel's Incompleteness
Rose-Hulman Undergraduate Mathematics Journal Volume 11 Issue 1 Article 8 Gödel's Incompleteness Tyson Lipscomb Marshall University, [email protected] Follow this and additional works at: https://scholar.rose-hulman.edu/rhumj Recommended Citation Lipscomb, Tyson (2010) "Gödel's Incompleteness," Rose-Hulman Undergraduate Mathematics Journal: Vol. 11 : Iss. 1 , Article 8. Available at: https://scholar.rose-hulman.edu/rhumj/vol11/iss1/8 Gödel's Incompleteness Theorems: A Revolutionary View of the Nature of Mathematical Pursuits Tyson Lipscomb Marshall University March 11th, 2010 Abstract The work of the mathematician Kurt Gödel changed the face of mathematics forever. His famous incompleteness theorem proved that any formalized system of mathematics would always contain statements that were undecidable, showing that there are certain inherent limitations to the way many mathematicians studies mathematics. This paper provides a history of the mathematical developments that laid the foundation for Gödel's work, describes the unique method used by Gödel to prove his famous incompleteness theorem, and discusses the far- reaching mathematical implications thereof. 2 I. Introduction For thousands of years mathematics has been the one field of human thought wherein truths have been known with absolute certainty. In other fields of thought, such as science and philosophy, there has always been a level of imprecision or speculation. In mathematics, however, there are truths that we can know beyond a shadow of a doubt due to the methods of proof first devised by the ancient Greeks [10]. The unparallelled certainty of mathematics has throughout the past millennia led many to accept the notion that it would be possible to answer any mathematical question through expansive enough development of mathematical techniques [16]. -
Lecture 14 Rosser's Theorem, the Length of Proofs, Robinson's
Lecture 14 Rosser’s Theorem, the length of proofs, Robinson’s Arithmetic, and Church’s theorem Michael Beeson The hypotheses needed to prove incompleteness The question immediate arises whether the incompleteness of PA can be “fixed” by extending the theory. G¨odel’s original publication was about Russell and Whitehead’s theory in Principia Mathematica. First-order logic was not yet a standard concept– that really didn’t happen until the textbook of Hilbert-Bernays appeared in the last thirties, and because WWII arrived soon on the heels of Hilbert-Bernays, it was at least 1948 before the concept was widespread. G¨odel’s methods were new and confusing: first-order logic was ill-understood, G¨odel numbering was completely new, the diagonal method was confusing, the arithmetization of syntax was confusing. There were doubts about the generality of the result. Maybe it was a special property of Principia Mathematica? Then (as now) few people were familiar with that dense and difficult theory. Maybe we just “forgot some axioms”? An examination of the proof puts that idea to rest. Clearly the proof applies to any theory T such that ◮ T contains PA ◮ The proof predicate PrfT (k,x) (“k is a proof in T of the formula with G¨odel number x”) is recursive (so representable) ◮ the axioms of T are true in some model whose “integers” are isomorphic to the structure defined by N with standard successor, addition, and multiplication. It works for all the axiom systems we know ◮ In particular those conditions apply to the strongest axioms accepted by most mathematicians, for example Zermelo-Frankel set theory with the axiom of choice, ZFC. -
Deciding Presburger Arithmetic Using Reflection Master’S Internship Under T
Deciding Presburger arithmetic using reflection Master's internship under T. Altenkirch supervision University of Nottingham G. Allais May 12, 2011 Abstract The need to prove or disprove a formula of Presburger arithmetic is quite frequent in certified software development (constraints generated automatically) or when working on higher arithmetic (number theory). The fact that this theory is decidable and that Agda is now mature enough to be able to implement such a solver pushed us to try to tackle this problem. The numerous steps of Cooper's decision procedure makes it easy to introduce bugs in an unverified implementation. We will show how the use of reflection together with dependent types can allow us to develop a bug-free solver that is proved to be complete. In the following developments, k, m, n will refer to integers, x, y, z to variables ranging over the integers, P , Q, R to quantifier free propositions and Φ, Ψ to any formula of Presburger arithmetic. Part I Deciding Presburger arithmetic 1 Preliminaries 1.1 Definitions The main concern of this paper is to be able to deal automatically with formulas of an extension of Presburger arithmetic without free variable. The first-order theory on natural numbers with addition but without multiplication (Presburger arithmetic) is extended with the use of the canonical order on the integers and a couple notions that are only syntactic sugar on top of it: • We allow the use of multiplication when the multiplicand is an integer: k ∗ e is only a shortcut for sign(k):e + ··· + sign(k):e ; | {z } jkj times • We also allow the use of k div e which is just a shortcut for 9x; e = k ∗ x.