Development of a Rubik's Cube Solving Application For
Total Page:16
File Type:pdf, Size:1020Kb
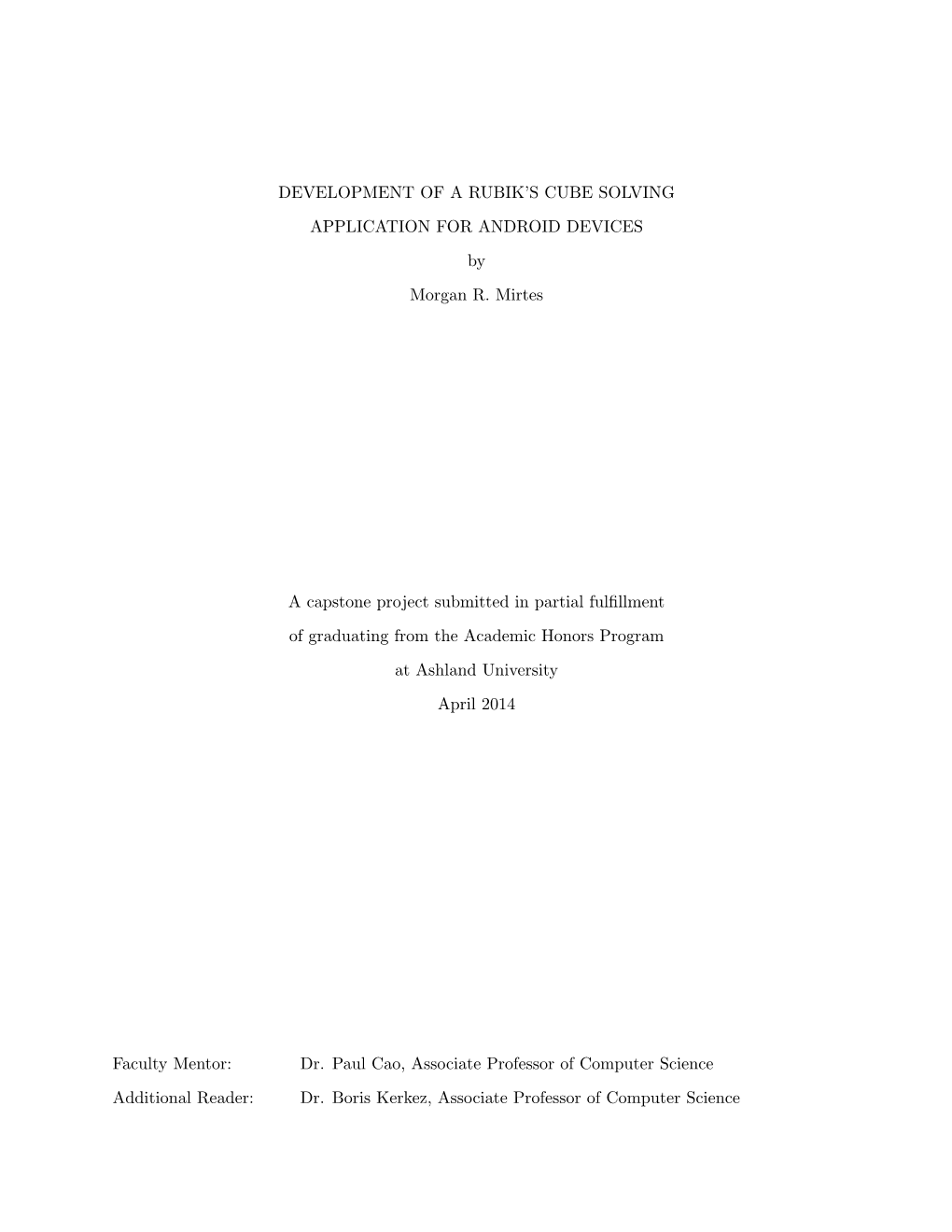
Load more
Recommended publications
-
Benchmarking Beginner Algorithms for Rubik's Cube
DEGREE PROJECT, IN COMPUTER SCIENCE , FIRST LEVEL STOCKHOLM, SWEDEN 2015 Benchmarking Beginner Algorithms for Rubik's cube ANDREAS NILSSON, ANTON SPÅNG KTH ROYAL INSTITUTE OF TECHNOLOGY CSC SCHOOL Supervisor: Michael Schliephake Examiner: Örjan Ekeberg Abstract Over the years different algorithms have been developed to step-by-step solve parts of the Rubik’s cube until fi- nally reaching the unique solution. This thesis explores two commonly known beginner algorithms for solving Rubik’s cube to find how they differ in solving speed and amount of moves. The algorithms were implemented and run on a large amount of scrambled cubes to collect data. The re- sults showed that Layer-by-layer with daisy algorithm had a lower average amount of moves than the Dedmore al- gorithm. The main difference in amount of moves lies in the steps that solve the last layer of the cube. The Layer- by-layer with daisy algorithm uses only one-seventh of the time-consuming operations that Dedmore algorithm uses, which concludes that it is more suitable for speedcubing. Sammanfattning Över åren har ett antal olika algoritmer utvecklats för att steg-för-steg lösa delar av Rubik’s kub för att till sist kom- ma fram till den unika lösningen. Denna rapport utforskar två allmänt kända nybörjaralgoritmer för att lösa Rubik’s kub, för att finna hur dem skiljer sig åt i tid samt antal operationer för att nå lösningen. Algoritmerna implemen- terades och kördes på ett stort antal blandade kuber för att samla data. Resultatet visar att Lager-för-lager med daisy algoritmen hade ett lägre genomsnittligt antal förflyttning- ar jämfört med Dedmore algoritmen. -
Solving Megaminx Puzzle with Group Theory
Solving Megaminx puzzle With Group Theory Student Gerald Jiarong Xu Deerfield Academy 7 Boyden lane Deerfield MA 01342 Phone: (917) 868-6058 Email: [email protected] Mentor David Xianfeng Gu Instructor Department of Computer Science Department of Applied Mathematics State University of New York at Stony Brook Phone: (203) 676-1648 Email: [email protected] Solving Megaminx Puzzle with Group Theory Abstract Megaminx is a type of combination puzzle, generalized from the conventional Rubik’s cube. Although the recipes for manually solving megaminx are known, the structure of the group of all megaminx moves remains unclear, further the algorithms for solving megaminx blindfolded are unknown. First, this work proves the structure of the megaminx group: semidirect product of a orientation twisting sub- group and a position permutation subgroup, the former subgroup is decomposed further into the product of multiplicative groups of integers modulo 2 or 3, the later subgroup is the product of alternating groups. Second, the work gives the sufficient and necessary conditions for a configuration to be solvable. Third, the work shows a constructive algorithm to solve the megaminx, which is suitable for the blindfolded competition. Contributions 1. prove the structure of the megaminx move group, theorem 3.12; 2. give sufficient and necessary conditions for a configuration to be solvable, theorem 3.8; 3. construct an algorithm to solve megaminx, corollary 3.4. Solving Megaminx Puzzle with Group Theory Gerald Xua aDeerfield Academy Abstract Megaminx is a type of combination puzzle, generalized from the conventional Rubik’s cube. Although the recipes for manually solving megaminx are known, the structure of the group of all megaminx moves remains unclear, further the algorithms for solving megaminx blindfolded are unknown. -
O. Deventer 'From Computer Operations to Mechanical Puzzles'
Case Study 9 From Computer Operations to Mechanical Puzzles M. Oskar van Deventer, Freelance Mechanical Puzzle Designer Igor Kriz, Department of Mathematics, University of Michigan This article is about transforming a virtual puzzle implemented by computer operations into a physical puzzle. We describe why a puzzle motivated by a mathematical idea of the sporadic simple Mathieu group M12 is an interesting concept. We then describe how it was turned into two different physical puzzles called Topsy Turvy and Number Planet. Finally, we will show one version of a practical algorithmic solution to the puzzle, and describe challenges that remain. 1 Introduction ones are called sporadic simple groups [5]. The word ‘simple’ here refers to the fact that they are ver since E. Rubik invented his famous cube building blocks for finite groups, just as prime E in 1974, a boom of twisty puzzles began. The numbers are basic building blocks of natural num- puzzles which emerged are too numerous to list bers. However, it may be a misnomer to call those here (for some information, see [1, 2]).1 How- groups simple, since they are typically extremely ever, after an algorithm by David Singmaster complicated. for Rubik’s cube was published in 1981 [3], it Could one make a twisty puzzle based on a soon became clear that only small modifications sporadic simple group, which would then require of the strategy for finding the algorithm for Ru- a completely novel strategy, different in essential bik’s cube also produce algorithms for the other ways from Singmaster’s algorithm? The problem twisty puzzles. -
Read Book Logic Puzzles to Bend Your Brain Ebook
LOGIC PUZZLES TO BEND YOUR BRAIN PDF, EPUB, EBOOK Kurt Smith | 72 pages | 02 May 2002 | Sterling Juvenile | 9780806980126 | English | New York, United States Logic Puzzles to Bend Your Brain PDF Book Hanayama cast puzzles are the world's finest brain teasers. Memory Support. If you make it moving, you will feel the sense of doing a slide puzzle. Crafted by Little 10 Robot. Bryan and his wife Krista currently reside in Lebanon, Ohio with their 5 children. Palavras e frases frequentes acres answers answers on page apples average balls bases baskets Berries birdie Blue Bobcats bones bowl Buffaloes carpet chose cinnamon roll Cities Clark clues contains corn dogs Cranberries Dale's Dalton double bogey drank Drive drove earned Favorite feet fewer figure five four Fractions free throws gallon glasses of cider Grandpa Green guppies half Height hogs Hole hour inches Joey Jones kind kitten knock kookaburra leaves liters lives lost match miles minutes month needs ordered paid Panthers person pieces pizza played player points pounds Ranch Rockets Room Sale sandwich scoops scored sheep Sit-ups slices Smith soccer soft drink sold Spartans Street taller tire took traveled trip truck twice varsity walked week weighs Willy Yard. Connecting with Nature: The Benefits To see what your friends thought of this book, please sign up. Javascript is not enabled in your browser. Open Preview See a Problem? How do we get around that? Happy Neuron was created by a team of French neuroscientists specifically to work the brain. More than brain-jogging puzzles give you a crash course in cryptography, while the messages reward you with the comedy of Jay Leno, Elayne Boosler, and Jerry Seinfeld, as well as the wit and wisdom of Hillary Clinton, Stephen King, The accident itself was witnessed by six bystanders. -
Preface Preface Preface
CubeRoot Preface Preface Preface The Rubiks cube is a Combination puzzle in the shape of a cube that is cut two times along each of three axes. It is invented in 1974 by Ernő Rubik, which is the best-selling toy in history. Since 2003, WCA (World Cube Association), the official ruling and organizing entity for speedsolving Rubiks Cube and other puzzles, has organized competitions worldwide and recognize world records. From around 2007 the cubing community has seen the development of speedcubes made in China like Qiyi, GAN, Moyu, Yuxin. This puzzle consists of 6 faces, each with 9 colored facets. From another aspect, it has 6 fixed centers, 12 edges, 8 corners, and a total of 43,252,003,274,489,856,000 positions. A solved cube has all facets on each face with the same color. The cube move F,, BU , DLR ,, rotates the front, back, upper, down, left, right face 90 degree turn clockwise, respectively. A letter followed by a prime () symbol denotes a 90 degree turn counterclockwise. A letter followed by a 2 denotes a double turn of that face. CFOP method (Cross – F2L – OLL – PLL), the most commonly used speedsolving method for 3x3, heavily relies on algorithms, pattern recognition and muscle memory compared to more intuitive methods such as Roux, ZZ or Petrus method. It was first developed in the early 1980s combining innovations by a number of cubers. Jessica Fridrich popularized it by publishing it online in 1997. Its average number of moves is 56. Gods Number is either used to refer to the diameter of the group of the puzzle (the furthest distance two states can be from each other) or to the furthest distance any position can be from solved. -
Algorithms for Solving the Rubik's Cube
DEGREE PROJECT, IN COMPUTER SCIENCE , FIRST LEVEL STOCKHOLM, SWEDEN 2015 Algorithms for solving the Rubik's cube A STUDY OF HOW TO SOLVE THE RUBIK’S CUBE USING TWO FAMOUS APPROACHES: THE THISTLEWAITE’S ALGORITHM AND THE IDA* ALGORITHM. HARPREET KAUR KTH ROYAL INSTITUTE OF TECHNOLOGY CSC SCHOOL Algorithms for solving the Rubik's cube A study of how to solve the Rubik's cube using two popular approaches: the Thistlewaite's algorithm and the IDA* algorithm. Harpreet Happy Kaur ([email protected]) DD143X Degree Project in Computer Science, first level Supervisor: Arvind Kumar Examiner : Orjan¨ Ekeberg CSC, KTH May 27, 2015 Abstract There are different computational algorithms for solving the Rubik's cube, such as Thistlewaite's algorithm, Kociemba's algorithm and IDA* search algorithm. This thesis evaluates the efficiency of two algorithms by analyzing time, performance and how many moves are required to solve the Rubik's cube. The results show that the Thistlewaite's algorithm is less efficient than the IDA* algorithm based on time and performance. The paper attempts to answer which algorithm are more efficient for solving the Rubik's cube.It is important to mention that this report could not prove which algorithm is most efficient while solving the whole cube due to limited data, literature studies and authors are used as an argument to prove that the Korf's algorithm is more efficient. Abstract Det finns ett antal olika algoritmer f¨oratt l¨osaRubik's kuben , s˚asom Thistlewaite algoritm , Kociemba algoritm och IDA* algoritm. Denna rap- port utforskar effektiviteten av tv˚aoptimala algoritmer genom att analysera tid , prestanda och hur m˚angaoperationer som kr¨avsf¨oratt l¨osaRubik's kuben. -
Group Theory and the Rubik's Cube
Group Theory and the Rubik's Cube by Lindsey Daniels A project submitted to the Department of Mathematical Sciences in conformity with the requirements for Math 4301 (Honours Seminar) Lakehead University Thunder Bay, Ontario, Canada copyright c (2014) Lindsey Daniels Abstract The Rubik's Cube is a well known puzzle that has remarkable group theory properties. The objective of this project is to understand how the Rubik's Cube operates as a group and explicitly construct the Rubik's Cube Group. i Acknowledgements I would like to thank my supervisors Dr. Adam Van Tuyl and Dr. Greg Lee for their expertise and patience while preparing this project. ii Contents Abstract i Acknowledgements ii Chapter 1. Introduction 1 Chapter 2. Groups 3 1. Preliminaries 3 2. Types of Groups 4 3. Isomorphisms 6 Chapter 3. Constructing Groups 9 1. Direct Products 9 2. Semi-Direct Products 10 3. Wreath Products 10 Chapter 4. The Rubik's Cube Group 12 1. Singmaster Notation 12 2. The Rubik's Cube Group 12 3. Fundamental Theorems of Cube Theory 16 4. Applications of the Legal Rubik's Cube Group 20 Chapter 5. Concluding Remarks 22 Chapter 6. Appendix 23 Bibliography 24 iii CHAPTER 1 Introduction In 1974, Ern¨oRubik invented the popular three dimensional combination puzzle known as the Rubik's Cube. The cube was first launched to the public in May of 1980 and quickly gained popularity. Since its launch, 350 million cubes have been sold, becoming one of the best selling puzzles [2]. By 1982, the cube had become part of the Oxford English Dictionary and a household name [5]. -
Read Ebook \\ Mechanical Toys / 4XGV1KFZXPM0
KC5LEBOZV84C « eBook > Mechanical toys Mech anical toys Filesize: 2.96 MB Reviews Very beneficial to all category of folks. I really could comprehended every little thing out of this created e publication. I found out this book from my dad and i encouraged this ebook to discover. (Maia O'Hara) DISCLAIMER | DMCA M3XMACUOUKBF / Kindle ^ Mechanical toys MECHANICAL TOYS To get Mechanical toys eBook, make sure you follow the web link beneath and save the file or gain access to other information that are highly relevant to MECHANICAL TOYS ebook. Reference Series Books LLC Okt 2012, 2012. Taschenbuch. Book Condition: Neu. 247x190x10 mm. Neuware - Source: Wikipedia. Pages: 78. Chapters: Mechanical puzzles, Soma cube, Tower of Hanoi, Flexagon, Rubik's Cube, Jigsaw puzzle, Peg solitaire, Combination puzzle, Speedcubing, Toy train, Rubik's Revenge, Fieen puzzle, Professor's Cube, Klotski, Square One, V-Cube 6, V-Cube 7, Pyramorphix, Megaminx, Rubik's Magic, Dogic, Cymbal- banging monkey toy, Helicopter Cube, Pyraminx, Rubik's Snake, Impossible bottle, Pocket Cube, Snake cube, Pyraminx Crystal, Happy Cube, Rush Hour, Impossiball, Skewb Ultimate, BrainTwist, Rubik's Clock, Mechanical toy, Sliding puzzle, Disentanglement puzzle, Secret decoder ring, Equilibrium, Rubik's Magic: Master Edition, Sudoku Cube, Rubik's 360, Puzzle jug, Alexander's Star, Minus Cube, Puzzle ring, Wind-up toy, Skewb Diamond, Baguenaudier, Nintendo tumbler puzzle, Burr puzzle, Egg of Columbus, Magnet Space Wheel, Eastsheen, Hoppers, Snapper Puzzle, Puzzle globe, Think-a-Dot, Rubik's Triamid, Puzzle box, Missing Link, Pyramid puzzle, Diabolical cube, Lock puzzle, Moo box, Yoshimoto Cube, Human knot, Fuddling cup, Mechanical bank, Puzzle jewelry, Gridlock, Libelle. -
Using Group Theory for Solving Rubik's Cube
Submitted by Paul Kainberger Submitted at Institut f¨ur Algebra Supervisor Assoz. Univ.-Prof. Dipl.-Ing. Dr. Erhard Aichinger November 2018 Using Group Theory for solving Rubik's Cube Bachelor Thesis to obtain the academic degree of Bachelor of Science in the Bachelor's Program Technische Mathematik JOHANNES KEPLER UNIVERSITY LINZ Altenbergerstraße 69 4040 Linz, Osterreich¨ www.jku.at DVR 0093696 1 Statutory Declaration 1 Statutory Declaration I declare that I have authored this thesis independently, that I have not used other than the declared sources / resources, and that I have explicitly marked all material which has been quoted either literally or by content from the used sources. .......................................................... Date Paul Kainberger iii 2 Acknowledgement 2 Acknowledgement I want to thank my supervisor Assoz. Univ.-Prof. Dipl.-Ing. Dr. Erhard Aichinger for his assistance and support on my bachelor thesis. Furthermore, I am grateful for my family always supporting me during my studies. iv 3 Abstract 3 Abstract We consider Rubik's Cube mathematically using algebraic group theory. We will see that the 6 possible rotations on the cube generate a group. With the help of the computer algebra software GAP we will be able to calculate a guidance to solve the combination puzzle without using any solving techniques made for humans. Finally, we want to compare the quality of GAP's solutions with those of a beginner's algorithm for humans. v 4 Kurzfassung 4 Kurzfassung Wir betrachten Rubiks Zauberw¨urfelmathematisch mittels algebraischer Gruppenthe- orie. Mithilfe des Computeralgebrasystems GAP m¨ochtenwir eine Anleitung entwick- eln, um einen beliebig verdrehten Zauberw¨urfell¨osenzu k¨onnen.Dabei verwenden wir keinerlei L¨osungsalgorithmen f¨urMenschen. -
Permutation Groups and Puzzle Tile Configurations of Instant Insanity II Amanda N
East Tennessee State University Digital Commons @ East Tennessee State University Electronic Theses and Dissertations Student Works 5-2014 Permutation Groups and Puzzle Tile Configurations of Instant Insanity II Amanda N. Justus East Tennessee State University Follow this and additional works at: https://dc.etsu.edu/etd Part of the Algebra Commons, Discrete Mathematics and Combinatorics Commons, and the Set Theory Commons Recommended Citation Justus, Amanda N., "Permutation Groups and Puzzle Tile Configurations of Instant Insanity II" (2014). Electronic Theses and Dissertations. Paper 2337. https://dc.etsu.edu/etd/2337 This Thesis - Open Access is brought to you for free and open access by the Student Works at Digital Commons @ East Tennessee State University. It has been accepted for inclusion in Electronic Theses and Dissertations by an authorized administrator of Digital Commons @ East Tennessee State University. For more information, please contact [email protected]. Permutation Groups and Puzzle Tile Configurations of Instant Insanity II A thesis presented to the faculty of the Department of Mathematics East Tennessee State University In partial fulfillment of the requirements for the degree Master of Science in Mathematical Sciences by Amanda Justus May 2014 Robert A. Beeler, Ph.D., Chair Robert Gardner, Ph.D. Debra Knisley, Ph.D. Rick Norwood, Ph.D. Keywords: algebra, group theory, combinatorics ABSTRACT Permutation Groups and Puzzle Tile Configurations of Instant Insanity II by Amanda Justus The manufacturer claims that there is only one solution to the puzzle Instant Insanity II. However, a recent paper shows that there are two solutions. Our goal is to find ways in which we only have one solution. -
Benchmarking Rubik's Revenge Algorithms
2013-04-12 Stockholm Benchmarking Rubik’s Revenge algorithms Paolo Brolin Echeverria - [email protected] - 0735 44 10 70 Joakim Westermark - [email protected] - 0762 06 61 50 KTH, CSC - DD143X Degree Project in Computer Science, First Level Supervisor: Johan Boye Abstract This Bachelor thesis paper investigates 2 different methods used to solve the Rubik’s Cube 4x4x4 puzzle. The analyzed methods are Reduction and Big Cube method. We have implemented the cube and the two solvers in Python. Through a series of tests we have concluded that the Big Cube method has a better average move count as well as a low standard deviation in comparison to the Reduction method. However the reduction method has a lower minimum move count and consists of fewer algorithms. The best approach would be to combine both methods to form an optimal solution. Sammanfattning Denna kandidatexamensuppsats undersöker två olika metoder som används för att lösa Rubiks Kub 4x4x4. Metoderna som analyseras är Reduction och Big Cube. Vi har implementerat kuben samt de bägge lösarna I Python. Genom en serie tester har vi kommit fram till att Big Cube har ett lägre genomsnittligt rotationsantal samt lägre standardavvikelse än Reduction. Reduction- metoden har däremot ett lägre minimumvärde på antalet rotationer och består av färre algoritmer. Det bästa tillvägagångssättet vore att kombinera de båda lösningarna. i Table of Contents Abstract .................................................................................................................................................... -
6042 – a Case in Which the Court De
Date December 19, 2001 Court Tokyo High Court, Case number 2000 (Ne) 6042 13th Civil Division – A case in which the court determined that the configuration of rotating cube combination puzzles (generally called "Rubik's Cube") can be regarded as an "indication of goods or business" referred to in Article 2, paragraph (1), item (i) or item (ii) of the Unfair Competition Prevention Act. References: Article 2, paragraph (1), items (i) and (ii) of the Unfair Competition Prevention Act Summary of the Judgment 1. In this case, the plaintiff in the first instance [i] alleged against Defendant RUN'A in the first instance that, since the configuration of rotating cube combination puzzles (the "plaintiff's goods") manufactured and sold by the plaintiff in the first instance may be regarded as an "indication of goods or business" referred to in Article 2, paragraph (1), item (i) or item (ii) of the Unfair Competition Prevention Act, the act of Defendant RUN'A in the first instance of importing and selling its rotating cube combination puzzles (the "defendants' goods") constitutes an act of unfair competition, thereby seeking an injunction against said act and payment of damages; [ii] alleged against Defendant RUN'A in the first instance that, since the mark (the "defendant's mark") that Defendant RUN'A in the first instance affixed to the defendants' goods is similar to a registered trademark of the plaintiff in the first instance, the use of the defendant's mark constitutes infringement of the trademark right of the plaintiff in the first instance, thereby seeking an injunction against said use and payment of damages; and also [iii] alleged against Defendant P in the first instance, who is the representative of Defendant RUN'A in the first instance, that, regarding the act of unfair competition and the infringement of the trademark right as mentioned above, Defendant P in the first instance committed a willful or negligent act in the course of carrying out his/her job duties, thereby seeking payment of damages under Article 266-3, paragraph (1) of the Commercial Code.