Improving Java Server Performance with Interruptlets
Total Page:16
File Type:pdf, Size:1020Kb
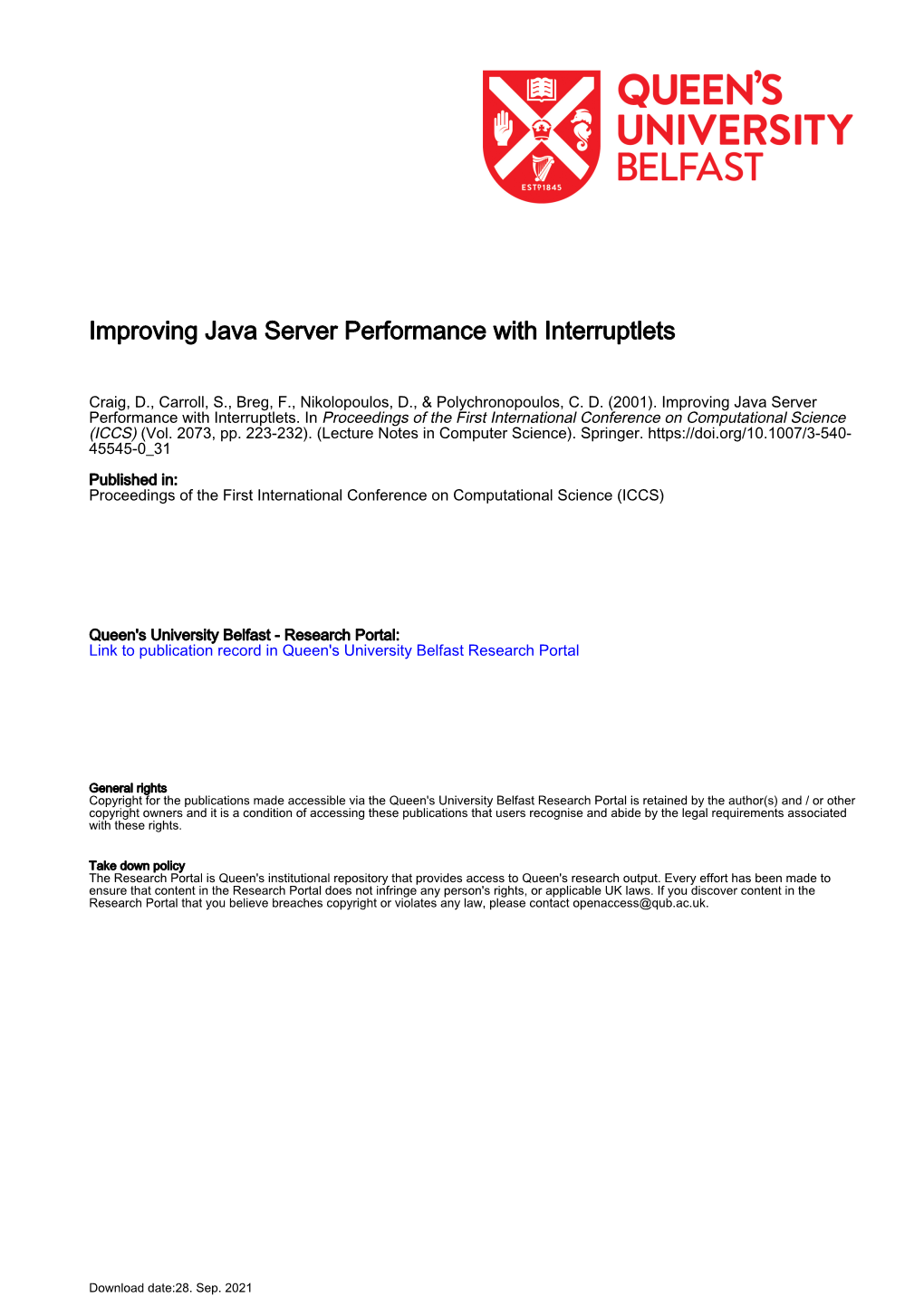
Load more
Recommended publications
-
Linux Performance Tools
Linux Performance Tools Brendan Gregg Senior Performance Architect Performance Engineering Team [email protected] @brendangregg This Tutorial • A tour of many Linux performance tools – To show you what can be done – With guidance for how to do it • This includes objectives, discussion, live demos – See the video of this tutorial Observability Benchmarking Tuning Stac Tuning • Massive AWS EC2 Linux cloud – 10s of thousands of cloud instances • FreeBSD for content delivery – ~33% of US Internet traffic at night • Over 50M subscribers – Recently launched in ANZ • Use Linux server tools as needed – After cloud monitoring (Atlas, etc.) and instance monitoring (Vector) tools Agenda • Methodologies • Tools • Tool Types: – Observability – Benchmarking – Tuning – Static • Profiling • Tracing Methodologies Methodologies • Objectives: – Recognize the Streetlight Anti-Method – Perform the Workload Characterization Method – Perform the USE Method – Learn how to start with the questions, before using tools – Be aware of other methodologies My system is slow… DEMO & DISCUSSION Methodologies • There are dozens of performance tools for Linux – Packages: sysstat, procps, coreutils, … – Commercial products • Methodologies can provide guidance for choosing and using tools effectively • A starting point, a process, and an ending point An#-Methodologies • The lack of a deliberate methodology… Street Light An<-Method 1. Pick observability tools that are: – Familiar – Found on the Internet – Found at random 2. Run tools 3. Look for obvious issues Drunk Man An<-Method • Tune things at random until the problem goes away Blame Someone Else An<-Method 1. Find a system or environment component you are not responsible for 2. Hypothesize that the issue is with that component 3. Redirect the issue to the responsible team 4. -
Why Open Source Software?
Why Open Source Software / Free Software (OSS/FS)? Look at the Numbers! David A. Wheeler http://www.dwheeler.com/contactme.html Revised as of November 7, 2004 This paper provides quantitative data that, in many cases, using open source software / free software is a reasonable or even superior approach to using their proprietary competition according to various measures. This paper’s goal is to show that you should consider using OSS/FS when acquiring software. This paper examines market share, reliability, performance, scalability, security, and total cost of ownership. It also has sections on non- quantitative issues, unnecessary fears, OSS/FS on the desktop, usage reports, governments and OSS/FS, other sites providing related information, and ends with some conclusions. An appendix gives more background information about OSS/FS. You can view this paper at http://www.dwheeler.com/oss_fs_why.html (HTML format). Palm PDA users may wish to use Plucker to view this. A short briefing based on this paper is also available in PDF and Open Office Impress formats (for the latter, use Open Office Impress). Old archived copies and a list of changes are also available. 1. Introduction Open Source Software / Free Software (OSS/FS) has risen to great prominence. Briefly, OSS/FS programs are programs whose licenses give users the freedom to run the program for any purpose, to study and modify the program, and to redistribute copies of either the original or modified program (without having to pay royalties to previous developers). This goal of this paper is to show that you should consider using OSS/FS when you’re looking for software, based on quantitative measures. -
Linux Certification Bible.Pdf
Turn in: .75 Board: 7.0625 .4375 VISIBLE SPINE = 1.75 .4375 Board: 7.0625 Turn in: .75 The only guide you need for Linux+ exam success . “This is the all-inclusive Linux+ guide you’ve been looking for.” You’re holding in your hands the most comprehensive and effective guide available for the CompTIA Linux+ 100% — Tim Sosbe, Editorial Director, Certification Magazine COMPREHENSIVE 100% exam. Trevor Kay delivers incisive, crystal-clear explanations of every Linux+ topic, highlighting exam- ONE HUNDRED PERCENT critical concepts and offering hands-on tips that can help you in your real-world career. Throughout, he COMPREHENSIVE Covers CompTIA Linux+ AUTHORITATIVE provides pre-tests, exam-style assessment questions, and scenario problems — everything you need to Exam XK0-001 WHAT YOU NEED master the material and pass the exam. ONE HUNDRED PERCENT Inside, you’ll find complete coverage Linux+ of Linux+ exam objectives Linux+ Master the • Get up to speed on Linux basics and understand the differences material for the between different Linux distributions CompTIA Linux+ • Tackle Linux installation, from planning to network configuration, Exam XK0-001 dual-boot systems, and upgrades Test your knowledge • Get the scoop on managing Linux disks, file systems, and with assessment processes; implementing security; and backing up your system Hundreds of unique, exam-like questions give you a random set of questions each questions and • Learn the ins and outs of configuring the X Window time you take the exam. scenario problems system and setting up a network • Find out how to establish users and groups, navigate Practice on the Linux file system, and use Linux system commands A customizable format enables state-of-the-art • Delve into troubleshooting techniques for the boot you to define test-preparation process, software, and networking your own software preferences • Get a handle on maintaining system hardware, from for question CPU and memory to peripherals presentation. -
Instant OS Updates Via Userspace Checkpoint-And
Instant OS Updates via Userspace Checkpoint-and-Restart Sanidhya Kashyap, Changwoo Min, Byoungyoung Lee, and Taesoo Kim, Georgia Institute of Technology; Pavel Emelyanov, CRIU and Odin, Inc. https://www.usenix.org/conference/atc16/technical-sessions/presentation/kashyap This paper is included in the Proceedings of the 2016 USENIX Annual Technical Conference (USENIX ATC ’16). June 22–24, 2016 • Denver, CO, USA 978-1-931971-30-0 Open access to the Proceedings of the 2016 USENIX Annual Technical Conference (USENIX ATC ’16) is sponsored by USENIX. Instant OS Updates via Userspace Checkpoint-and-Restart Sanidhya Kashyap Changwoo Min Byoungyoung Lee Taesoo Kim Pavel Emelyanov† Georgia Institute of Technology †CRIU & Odin, Inc. # errors # lines Abstract 50 1000K 40 100K In recent years, operating systems have become increas- 10K 30 1K 20 ingly complex and thus more prone to security and per- 100 formance issues. Accordingly, system updates to address 10 10 these issues have become more frequently available and 0 1 increasingly important. To complete such updates, users 3.13.0-x 3.16.0-x 3.19.0-x May 2014 must reboot their systems, resulting in unavoidable down- build/diff errors #layout errors Jun 2015 time and further loss of the states of running applications. #static local errors #num lines++ We present KUP, a practical OS update mechanism that Figure 1: Limitation of dynamic kernel hot-patching using employs a userspace checkpoint-and-restart mechanism, kpatch. Only two successful updates (3.13.0.32 34 and → which uses an optimized data structure for checkpoint- 3.19.0.20 21) out of 23 Ubuntu kernel package releases. -
How to Install Xenomai?
How to install Xenomai? This tutorial will detail Xenomai installation on a small Dell Optiplex 990 desktop having 16GB of RAM and an Intel Core i7-2600 CPU @ 3.40GHz × 8 cores. The machine has two 128GB Samsung SSD's. For the Ubuntu installation, 100GB of the first drive was dedicated to the operating system (mount point /) and the rest was left as swap space. On the second drive, 75GB was dedicated to user home directories (mount point /Volumes/<computer_name>) and the rest was left to backup space (mount point /Volumes/backup). 1 Step-by-step guide for Installing Linux 3.8.13 + Xenomai 2.6.3 (Ubuntu 12.04) 1.1 Prerequisites 1.2 Building a Xenomai-patched Linux kernel package 1.2.1 Downloading the sources/configuring xenomai 1.2.2 Kernel configuration and compilation 1.2.3 Testing for latency issues 1.2.4 Graphics drivers and Xenomai 1.2.4.1 NVIDIA GF119 1.2.4.2 Intel Onboard Graphics i915 1.3 Installing RT-NET 1.3.1 Downloading and installation 1.3.2 Network configuration 1.3.3 Running RTNet 1.3.4 Running RTNet at startup (optional) 1.3.5 Testing RTNet (on the SARCOS robot) 1.4 Installing usb4rt 1.4.1 Background 1.4.2 Installation 1.4.3 Configuration 1.4.4 Resolving IRQ sharing 1.4.5 Running usb4rt 1.4.6 Running usbrt at startup (optional) 1.4.7 Using usb4rt with more than one device 1.4.8 Testing usb4rt 1.4.9 Debugging usb4rt 1.5 Issue Log 1.6 Random Notes: 1.6.1 Reinstalling Ubuntu while preserving partitions 1.6.2 Solving the "invalid arch independent ELF magic" GRUB issue 1.6.3 SSH woes Step-by-step guide for Installing Linux 3.8.13 -
Speculative Use of Idle Resources Ph.D
Speculative Use of Idle Resources Ph.D. Dissertation Proposal Lars Eggert [email protected] Computer Science Department University of Southern California Los Angeles, CA 90089-0781 USA October 22, 2001 (Appendices added July 2, 2002) Abstract Even a fully loaded computer system, where the bottleneck resource is constantly busy, often has some idle capacities available on other resources. This proposal argues for using these idle ca- pacities speculatively, increasing system performance for correct predictions. In such a system, all resources will ideally be constantly loaded with either regular foreground tasks, or speculative idle-time tasks. The key contribution of this proposal is a model for non-interfering use of idle resource capacity, based on three principles: resource prioritization between regular foreground and idle-time use, preemptability of idle-time processing, and isolation of speculative side effects. Current operating systems fail to provide all three capabilities. Without new mechanisms, processing of speculative tasks can delay or even starve foreground processing, and result in a decreased foreground per- formance, instead of increasing it. Under the proposed model, speculative tasks only execute using otherwise idle resource capaci- ties; the model also shields foreground processing from the side effects of their presence in the system. Thus, speculation can no longer delay or interfere with foreground processing. Based on the model, a proof-of-concept design of network extensions for idle-time service can isolate fore- ground network packets from the presence of idle-time traffic to within 1-2% throughput. The remainder of this thesis will focus on idle-time support for the network file system (NFS). -
Developing a Solution for Multimedia Home Networking
Peng Liu Developing a Solution for Multimedia Home Networking School of Electrical Engineering Thesis submitted for examination for the degree of Master of Science in Technology. Espoo 20.5.2015 Thesis supervisor: Prof. Raimo A. Kantola Thesis advisor: PhD Mikko Välimäki aalto university abstract of the school of electrical engineering master’s thesis Author: Peng Liu Title: Developing a Solution for Multimedia Home Networking Date: 20.5.2015 Language: English Number of pages: 9+66 Department of Communications and Networking Professorship: Networking Technology Code: S-38 Supervisor: Prof. Raimo A. Kantola Advisor: PhD Mikko Välimäki In recent years, the rapid development of electronics and computer science has enabled home networking devices to become more affordable and more powerful. Several widely used multimedia-streaming solutions have become available in the market. However, as a result of their different technical designs, these standards naturally experience serious compatibility issues. Thus, end users can have several multimedia devices, with each one using a distinctive, unique protocol, making it challenging or even impossible sometimes to share media between those devices. These compatibility issues have motivated the need to determine the technological features common to the existing multimedia-streaming standards and to develop a more easy-to-use multimedia home networking solution. This thesis compares the modern solutions for multimedia home networking (MHN), including AirPlay, Miracast, Chromecast, and especially the Digital Liv- ing Network Alliance (DLNA) standard due to its wide adoption. By conducting research on the features and capabilities of these existing solutions, a suitable mo- bile solution for MHN, which takes advantage of AirPlay, Discovery and Launch (DIAL), and DLNA, is proposed for the Android platform. -
Why Open Source Software / Free Software (OSS/FS, FLOSS, Or FOSS)? Look at the Numbers!
Translations available: Czech | French | Japanese | Spanish Why Open Source Software / Free Software (OSS/FS, FLOSS, or FOSS)? Look at the Numbers! David A. Wheeler http://www.dwheeler.com/contactme.html Revised as of July 18, 2015 This paper (and its supporting database) provides quantitative data that, in many cases, using open source software / free software (abbreviated as OSS/FS, FLOSS, or FOSS) is a reasonable or even superior approach to using their proprietary competition according to various measures. This paper’s goal is to show that you should consider using OSS/FS when acquiring software. This paper examines popularity, reliability, performance, scalability, security, and total cost of ownership. It also has sections on non-quantitative issues, unnecessary fears, OSS/FS on the desktop, usage reports, governments and OSS/FS, other sites providing related information, and ends with some conclusions. An appendix gives more background information about OSS/FS. You can view this paper at http://www.dwheeler.com/oss_fs_why.html (HTML format). A short presentation (briefing) based on this paper is also available. Palm PDA users may wish to use Plucker to view this longer report. Old archived copies and a list of changes are also available. 1. Introduction Open Source Software / Free Software (aka OSS/FS), also described as Free/Libre and Open Source Software (FLOSS), has risen to great prominence. Briefly, FLOSS programs are programs whose licenses give users the freedom to run the program for any purpose, to study and modify the program, and to redistribute copies of either the original or modified program (without having to pay royalties to previous developers). -
The TUX Web Server
CITI Technical Report 00-8 An analysis of the TUX web server Chuck Lever, Sun-Netscape Alliance [email protected] Marius Aamodt Eriksen, Linux.com [email protected] Stephen P. Molloy, University of Michigan [email protected] ABSTRACT We report on a high-performance in-kernel web server for Linux known as the Threaded linUX http layer, or TUX, for short. TUX uses aggressive network layer data caching to accelerate static content delivery, and invokes CGI scripts directly from the kernel to accelerate dynamic content generation. We describe the TUX web server architecture, modifications included in the patch, and how they affect kernel operation and web server performance. November 16, 2000 Center for Information Technology Integration University of Michigan 519 West William Street Ann Arbor, MI 48103-4943 This document was written as part of the Linux Scalability Project. The work described in this paper was supported via grants from the Sun-Netscape Alliance, Intel, Dell, and IBM. For more information, see our home page. If you have comments or suggestions, email <[email protected]> Copyright © 2000 by the Regents of the University of Michigan, and by AOL-Netscape Inc. All rights reserved. Trademarked material referenced in this document is copyright by its respective owner. An analysis of the TUX web server Chuck Lever, Sun-Netscape Alliance [email protected] Marius Aamodt Eriksen, Linux.com [email protected] Stephen P. Molloy, University of Michigan [email protected] 1. Introduction • Driving the web server directly from the kernel’s networking layer to create a truly network event- As the demand for faster and more scalable web driven server service increases, system designers have discovered ways to improve web server performance and • Caching complete responses in the kernel’s scalability by integrating web server functionality networking layer to accelerate static content into operating systems. -
1 111111 111111111111111111111111111111 11111 1111 Iii 10.25.2011
TTAB KARL R. CANNON (Registration No. 36,468) BRETT J. DAVIS (Registration No. 46,655) CLAYTON, HOWARTH & CANNON, P.C. 6965 Union Park Center, Suite 400 Cottonwood Heights, Utah 84047 P.O. Box 1909 Sandy, Utah 84091-1909 Telephone: (801) 255-5335 Facsimile: (801) 255-5338 Attorneys for Connect Public Relations, Inc. Opposed Mark: CONNECT U.S. Trademark Application Serial Number: 77/714,693 Published: March 2, 2010 IN THE UNITED STATES PATENT AND TRADEMARK OFFICE BEFORE THE TRADEMARK TRIAL AND APPEAL BOARD CONNECT PUBLIC RELATIONS, INC., a Utah corporation, NOTICE OF WITHDRAWAL OF Opposer MOTION FOR PARTIAL SUMMARY JUDGMENT MAILED V. OCTOBER 17, 2011 DIGITALMOJO, INC., a California corporation, Opposition No. 91196299 Applicant. Opposer Connect Public Relations, Inc. ("ConnectPR" or "Opposer") hereby gives notice of the withdrawal of its Motion for Partial Summary Judgement ("Motion") having a certificate of deposit dated October 17, 2011. The reason for the withdrawal is that page 2 of the Motion Certificate of Deposit Under 37 C.F.R. § 2.197 I hereby certify that this correspondence is being deposited with the United States Postal Service ai.prst class mail, postage prepaid, in an envelope addressed to Trademark Trial and Appeal Board, P.O. Box 1451, Alexandria, Virginia 22313-1451, on the I day of Oc 2011. Karl R. annon Attorney Registration No. 36,468 Attorney for Applicant 1 111111 111111111111111111111111111111 11111 1111 III 10.25.2011 . Pat Ent 1 T,"101:171 118 inadvertently included a large blank portion when printed that caused the Motion to exceed the page limit set by the Board. -
Implementation and Evaluation of Transparent Fault-Tolerant Web Service with Kernel-Level Support
Proceedings of the IEEE International Conference on Computer Communications and Networks Miami, Florida, pp. 63-68, October 2002. Implementation and Evaluation of Transparent Fault-Tolerant Web Service with Kernel-Level Support Navid Aghdaie and Yuval Tamir Concurrent Systems Laboratory UCLA Computer Science Department Los Angeles, California 90095 {navid,tamir}@cs.ucla.edu AbstractÐMost of the techniques used for increasing the 1 2 availability of web services do not provide fault tolerance for requests being processed at the time of server failure. Other schemes require deterministic servers or changes to the web Client Web Server Back-end client. These limitations are unacceptable for many current and future applications of the Web. We have developed an efficient 4 3 implementation of a client-transparent mechanism for providing fault-tolerant web service that does not have the limitations Figure 1: If the web server fails before sending the client reply (step mentioned above. The scheme is based on a hot standby backup 4), the client can not determine whether the failure was before or server that maintains logs of requests and replies. The after the web server communication with the back-end (steps 2,3) implementation includes modifications to the Linux kernel and to web browser, are widely distributed and they are typically the Apache web server, using their respective module mechanisms. We describe the implementation and present an developed independently of the web service, it is critical that evaluation of the impact of the backup scheme in terms of any fault tolerance scheme used be transparent to the client. throughput, latency, and CPU processing cycles overhead. -
On the Design of Reliable and Scalable Networked Systems
On the Design of Reliable and Scalable Networked Systems Ph.D. Thesis Tomas Hruby VU University Amsterdam, 2014 This work was funded by European Research Council under ERC Advanced Grant 227874. Copyright © 2014 by Tomas Hruby. ISBN 978-90-5383-072-7 Printed by Wöhrmann Print Service. VRIJE UNIVERSITEIT ON THE DESIGN OF RELIABLE AND SCALABLE NETWORKED SYSTEMS ACADEMISCH PROEFSCHRIFT ter verkrijging van de graad Doctor aan de Vrije Universiteit Amsterdam, op gezag van de rector magnificus prof.dr. F.A. van der Duyn Schouten, in het openbaar te verdedigen ten overstaan van de promotiecommissie van de Faculteit der Exacte Wetenschappen op donderdag 10 april 2014 om 13.45 uur in de aula van de universiteit, De Boelelaan 1105 door TOMAS HRUBY geboren te Stod, Tsjechische Republiek promotors: prof.dr. Andrew S. Tanenbaum prof.dr. Herbert Bos Acknowledgements Tomas Hruby Amsterdam, The Netherlands, December 2013 v Contents Acknowledgements v Contents vii List of Figures xi List of Tables xiii Publications xv 1 Introduction 1 1.1 Overview of Operating System Architectures . 4 1.1.1 Monolithic Kernels . 4 1.1.2 Microkernels . 5 1.2 New Multicore Hardware . 7 1.3 Network Stack . 9 1.4 NEWTOS ............................... 11 1.5 Contributions . 12 1.6 Organization of the Dissertation . 12 2 Keep Net Working On a Dependable and Fast Networking Stack 15 2.1 Introduction . 16 2.2 Reliability, performance and multicore . 17 2.3 Rethinking the Internals . 19 2.3.1 IPC: What’s the kernel got to do with it? . 19 2.3.2 Asynchrony for Performance and Reliability .