Advanced Python
Total Page:16
File Type:pdf, Size:1020Kb
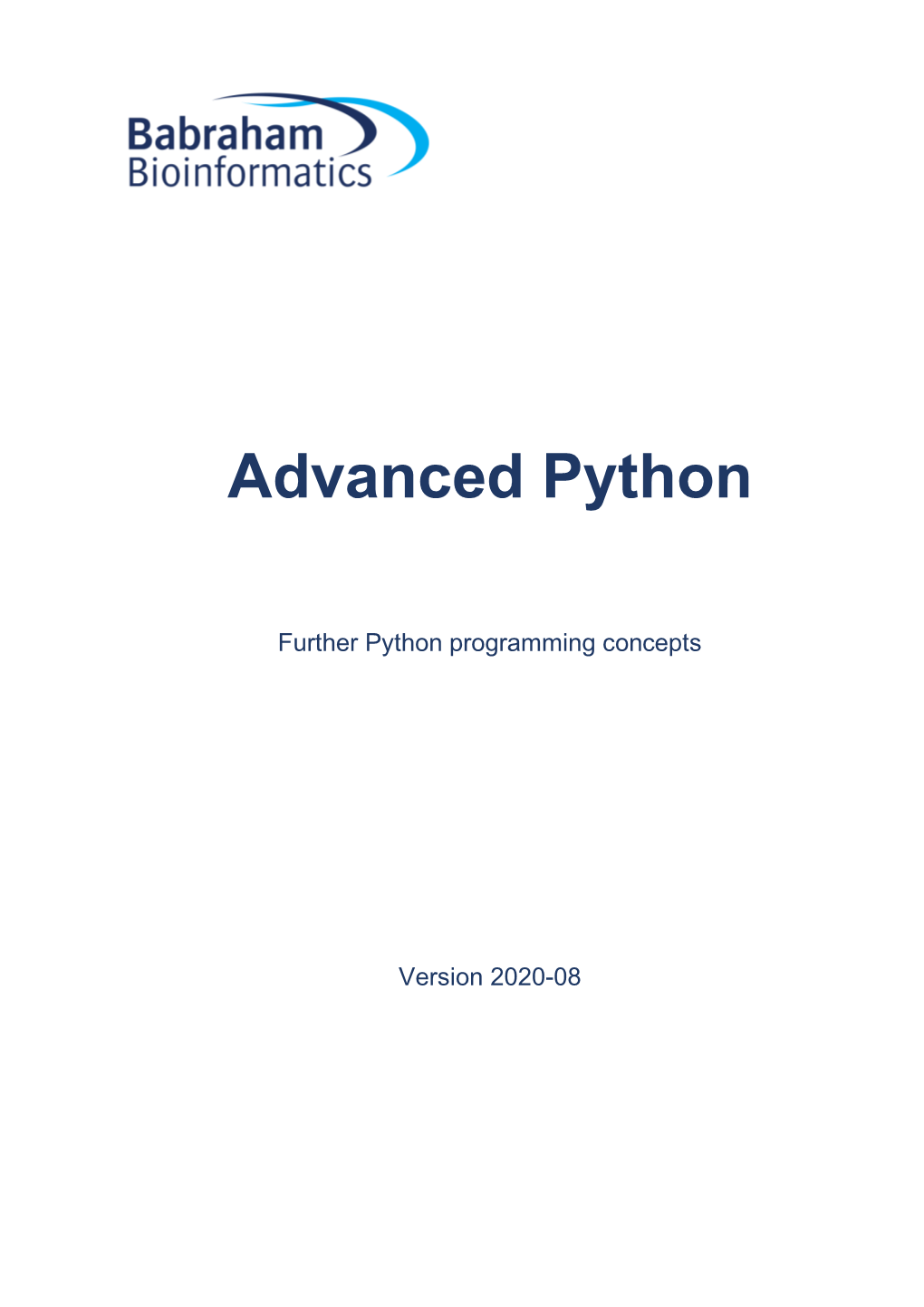
Load more
Recommended publications
-
SCIS Boardman Labs User Manual Version5
SCIS Common Use Labs in Boardman Hall USER MANUAL Note: The instructions in this manual assume students walking into the labs are first-time users of the labs and are complete novices in using the machines. Table of Contents Page I. Access to Boardman Hall SCIS Labs for Computer Science and New Media Students A. Services Provided 2 B. Gaining Access to Lab Rooms and Computers 3 II. SCIS Common Use Lab (Room 138 Boardman) A. Services Provided 3 B. Use of iMacs on Tables 4 C. Use of Large Table Monitor for Sharing Among Table Group 4 D. Use of Overhead Projector 5 E. Use of Laser Printer (8 ½ by 11” Prints) 6 F. Use of Large Format Plotter 7 G. Software Available on Computers in Room 138 7 H. Equipment Available for Checkout from Room 138 Lab Monitors 8 III. Combined Focus Ring and Stillwater Labs (Rooms 127 and 129 Boardman) A. Services Provided 8 B. Use of iMacs on Tables 9 C. Use of Cybertron PC for AI and VR D. Use of … E. Software Available on Computers in Rooms 127 and 129 F. Equipment Checkout Procedure G. Equipment Available for Checkout from Rooms 127 and 129 Lab Monitors IV. SCIS Student Lounge and Project Work Room (Room 137 Boardman) A. Services Provided B. Use of Large Screen by Students APPENDICES Appendix A. Laptop Computer Recommendations for Computer Science Students Appendix B. Laptop Computer Recommendations for New Media Students Appendix C. Convenient Web Resources for Development of Computer Code Note: On SCIS web site all of the above topics will link to anchors at the same topics below. -
Programming Environments
Programming Environments Presenter: Steve Baskauf [email protected] CodeGraf landing page • vanderbi.lt/codegraf What is an environment? vanderbi.lt/codegraf Coding environment • The definition of "environment" is a bit murky • We can consider an environment to include: • the value of defined variables • functions available to be used in our code • knowledge about position in file directory structure and other computer-wide parameters Accessing via the shell • Python example • R example Integrated development environment (IDE) What is an integrated development environment (IDE)? • An IDE is a graphical user interface (GUI) for developing code • An IDE includes: • a code editor • a shell • An IDE might include: • tools for examining the environment • formatting help and syntax checking • mechanisms for debugging code • a package manager Thonny example • Thonny is a simple Python IDE Spyder IDE for Python RStudio IDE for R Literate programming with Jupyter notebooks Literate programming • Programming paradigm for making code understandable to humans • Mix text, images, links with code. • Implementable in a primitive fashion with comments (#) • Implementable in a robust way with Jupyter notebooks and R Markdown Example: Jupyter notebooks • Formerly known as "iPython notebooks" (.ipynb file extension) • Now usable with Python, R, and other programming languages • Runnable in a browser when connected to a server • Viewable in GitHub (but not runnable) Functions Functions argument parameter • A function defines a block of code. • We pass arguments into functions: • functionName(argument1, argument2, ...) • It’s good to name functions by what they do. returned Example: value my_latte = make_latte(beans, milk, water) • Functions can be: • built-in • defined by you in your code • defined by somebody else in a module Image: Nykamp DQ, “Function machine f.” From Math Insight. -
Research Manual
MOBILE FORENSICS APPLICATION Research Manual Student: Connor Scanlan – C00226867 Supervisor: James Egan Cybercrime & IT Security – CW_KCCYB_B Institute of Technology Carlow Table of Contents Abstract .................................................................................................. 3 Introduction ............................................................................................. 3 Research ................................................................................................. 4 The need for better Mobile Forensics tools ......................................................... 4 Use of mobile phones to store and transmit sensitive information ........................... 4 Online transactions .................................................................................. 5 Law Enforcement and Criminals ................................................................... 5 Mobile Data as Evidence ............................................................................... 6 Definition of Digital Evidence ...................................................................... 6 Principles of Electronic Evidence .................................................................. 6 Framework of Mobile Forensics....................................................................... 8 Mobile Forensics System Structure ................................................................ 8 Mobile Forensics Processes ......................................................................... 9 Mobile Data Collection -
Instalación Wxpython Y Pyo En Thonny
Instalaci´on wxPython y pyo en Thonny Inform´aticaIII ISM - UNL [email protected] updated: 29 ago 2018 ´Indice 1. Introducci´on 2 2. Instalaci´onen Windows 7/8.1/10 (32 y 64 bits) 3 2.1. Reconocer arquitectura del Sistema Operativo . .3 2.2. Instalar paquetes de MS Visual C++ . .3 2.3. Instalar wxPython con Thonny .........................................4 2.4. Instalar pyo en Thonny . .5 2.5. Instalaci´onde wxFormBuilder .........................................6 3. Instalaci´onen Linux (Ubuntu 16.04 64 bits) 8 3.1. Prerrequisitos . .8 3.2. Instalar wxPython en Thonny .........................................8 3.3. Instalar pyo en Thonny . 10 3.4. Instalaci´onde wxFormBuilder ......................................... 12 4. Instalaci´onen MacOSX (Sierra 10.12 - 64 bits) 13 4.1. Instalar wxPython con Thonny ......................................... 13 4.2. Instalar pyo con Thonny ............................................ 14 4.3. Instalaci´onde wxFormBuilder ......................................... 16 1. Introducci´on En este documento se intentar´adar los pasos para la instalaci´onde wxPython y pyo en la interfaz Thonny. wxPython (https://wxpython.org) es un conjunto de librer´ıasgr´aficaspara Python que permite la interacci´on entre el usuario y componentes GUI (Graphical User Interface) de manera tal que se pueda programar ventanas, botones, listas, menu´es,facilitando as´ıla interacci´onentre el usuario y los programas. Por otro lado, pyo (http://ajaxsoundstudio.com/software/pyo) es un m´odulode Python dise~nadopor Olivier Belanger (PhD en Composici´onElectroac´usticadel Ajax Sound Studio, de Montreal, Canad´a)para procesamiento digital de se~nales(o DSP - Digital Signal Processing) que se utilizar´apara sintetizar, filtrar y generar efectos de audio utilizando Python. -
Python Intro #1
Python Intro #1 Python is a popular programming language. It was created by Guido van Rossum, and released in 1991. It is used for: • web development (server-side), • software development, • mathematics, • system scripting. What can Python do? • Python can be used on a server to create web applications. • Python can be used alongside software to create workflows. • Python can connect to database systems. It can also read and modify files. • Python can be used to handle big data and perform complex mathematics. • Python can be used for rapid prototyping, or for production-ready software development. Why Python? • Python works on different platforms (Windows, Mac, Linux, Raspberry Pi, etc). • Python has a simple syntax similar to the English language. • Python has syntax that allows developers to write programs with fewer lines than some other programming languages. • Python runs on an interpreter system, meaning that code can be executed as soon as it is written. This means that prototyping can be very quick. • Python can be treated in a procedural way, an object-orientated way or a functional way. Good to know • The most recent major version of Python is Python 3, which we shall be using in this tutorial. However, Python 2, although not being updated with anything other than security updates, is still quite popular. • In this tutorial Python will be written in a text editor. It is possible to write Python in an Integrated Development Environment, such as Thonny, Pycharm, Netbeans or Eclipse which are particularly useful when managing larger collections of Python files. • Python Syntax compared to other programming languages • Python was designed for readability, and has some similarities to the English language with influence from mathematics. -
Minecraft Pi DEVELOPED by D-LEARN & CCSDE Minecraft Pi Is a Version of Minecraft, with Minimal Features, Developed for Raspberry Pi
Reviving hands-on educational play for learning skills of tomorrow MODULE 1 PROJECT N° 2019-1-UK01-KA201-061466 Minecraft Pi DEVELOPED BY D-LEARN & CCSDE Minecraft Pi is a version of Minecraft, with minimal features, developed for Raspberry Pi. Pi edition is intended as an educational tool for novice programmers, allowing users to enjoy the game and learn programming at the same time. This resource presents the most important and practical guidelines for Minecraft Pi, such as how to control the player, manually build with blocks and use the Python interface to manipulate the world around you. It is meant for educational purposes and is considered a quick but all-inclusive manual for introducing a new player to Minecraft Pi. Once you follow this module you will be able to: • Access Minecraft Pi and create a new world • Navigate around Minecraft Pi • Know how to place and destroy a block, and navigate through different types of blocks in the in-game inventory • Connect Python to Minecraft Pi • Use the Python programming interface • Manipulate blocks using Python scripts • Make Minecraft interact with the physical world through the Raspberry Pi GPIO • Introduction to Minecraft Pi basic functions • Minecraft Pi elements and gameplay • Controlling Minecraft Pi with Python • Interaction of Minecraft Pi with the physical world through the Raspberry Pi’s GPIO: Connecting LEDs, buttons and switches Create electronic kits to interact with Minecraft Pi Once you have followed this topic you will be able to: • Know the basics before running Minecraft Pi • Run Minecraft Pi on your Play2Learn computer • Navigate around Minecraft Pi • Use the controls on your mouse and keyboard Playing Minecraft Pi for the first time: Your Play2Learn computer contains everything that you need to run Minecraft Pi in terms of software. -
2018Dec Buffalo Python Meetup
Tim Poulsen Software Engineer R&D Team ACV Labs RASPBERRY PI Overview & Examples Board components Basic setup Python dev Libraries Resources https://github.com/skypanther/PiLit https://www.timpoulsen.com/2018/pi-birdcam.html Camera controller board PyImageSearch blog www.pyimagesearch.com/2015/05/25/basic-motion-detection-and- tracking-with-python-and-opencv/ PI 3B+ Micro SD slot (back side) Power in (micro-USB) HDMI GPIO, I2C, serial CSI camera Stereo audio out Broadcom BCM2837B0, Cortex-A53 (ARMv8) 64-bit SoC @ 1.4GHz 1GB LPDDR2 SDRAM 2.4GHz and 5GHz IEEE Gigabit 802.11.b/g/n/ac 4 x USB 2.0 Ethernet Bluetooth 4.2, BLE OS OPTIONS • Raspbian (customized Debian distro) • NOOBs - an installer, not an OS • Third-party provided Ubuntu, Windows 10 IoT Core, RISC OS, etc. OS SETUP • Download OS “image” .img file • Copy to SD card • Insert in Pi and boot Etcher app - https://www.balena.io/etcher/ DEV ENVIRONMENT • Python 2.x / 3.x • Ruby • C • Wolfram language • C++ • SonicPi • Java • Git • Scratch IDEs Pre-installed: Installable: • Thonny (Python) • VS Code - https:// • Geany (multiple) code.headmelted.com/ • BlueJ (Java) • Spyder • Greenfoot (Java) • Ninja-IDE • Mathematica • • Node-RED Lazarus • Scratch • lots more… PYTHON LIBRARIES Pre-installed: Installable: • Any Python-only library • RPi.GPIO • Or, any that will compile for • GPIO Zero ARM • www.piwheels.org - • PiCamera precompiled packages • e.g. OpenCV, Tensorflow, WiringPi, etc. GPIO BASICS from gpiozero import LED from time import sleep red = LED(17) while True: red.on() sleep(1) red.off() sleep(1) GPIO / I2C / SERIAL https://pinout.xyz/ SERIAL OVER USB import serial # open serial port ser = serial.Serial('/dev/ttyUSB0') # write a string ser.write(b'hello') # close port ser.close() PICAMERA from picamera import PiCamera from time import sleep camera = PiCamera() camera.resolution = (1024, 768) camera.start_preview() # Camera warm-up time sleep(2) camera.capture('foo.jpg') THANKS! • timpoulsen.com • github.com/skypanther • @skypanther • www.linkedin.com/in/timpoulsen. -
What Is Python?
What is Python? Python is a popular programming language. It was created by Guido van Rossum, and released in 1991. It is used for: web development (server-side), software development, mathematics, system scripting. What can Python do? Python can be used on a server to create web applications. Python can be used alongside software to create workflows. Python can connect to database systems. It can also read and modify files. Python can be used to handle big data and perform complex mathematics. Python can be used for rapid prototyping, or for production-ready software development. Why Python? Python works on different platforms (Windows, Mac, Linux, Raspberry Pi, etc). Python has a simple syntax similar to the English language. Python has syntax that allows developers to write programs with fewer lines than some other programming languages. Python runs on an interpreter system, meaning that code can be executed as soon as it is written. This means that prototyping can be very quick. Python can be treated in a procedural way, an object-orientated way or a functional way. Good to know The most recent major version of Python is Python 3, which we shall be using in this tutorial. However, Python 2, although not being updated with anything other than security updates, is still quite popular. In this tutorial Python will be written in a text editor. It is possible to write Python in an Integrated Development Environment, such as Thonny, Pycharm, Netbeans or Eclipse which are particularly useful when managing larger collections of Python files. Python Syntax compared to other programming languages Python was designed for readability, and has some similarities to the English language with influence from mathematics. -
Raspberry Pi and Python Hans-Petter Halvorsen
Raspberry Pi and Python Hans-Petter Halvorsen https://www.halvorsen.blog Raspberry Pi and Python Raspberry Pi and Python Hans-Petter Halvorsen 2021 Raspberry Pi and Python c Hans-Petter Halvorsen January 4, 2021 1 Preface Python is a popular programming language, and it is one of the most used pro- gramming languages today. Python works on all the main platforms and operating systems used today, such Windows, macOS, and Linux. Python is a multi-purpose programming language, which can be use for simu- lation, creating web pages, communicate with database systems, etc. My Blog/Web Site [1]: https://www.halvorsen.blog Here you find lots of technical resources about Technology, Programming, Soft- ware Engineering, Automation and Control, Industrial IT, etc. Here you find my Web page with Python resources: https://www.halvorsen.blog/documents/programming/python/ These resources are a supplement to this textbook. Here you can download the software, download code examples, etc. This Textbook is written in LATEXusing Overleaf. LATEXis a document preparation system used for the communication and publi- cation of scientific documents. 2 For more information about LATEX: https://www.latex-project.org Overleaf is a web-bases LATEXsystem, meaning you can write your LATEXdocuments in your web browser, you co-work and share documents with others. For more information about Overleaf: https://www.overleaf.com Python Books You find other Python textbooks within different domains on my Python Web page: https://www.halvorsen.blog/documents/programming/python/ Python Books: • Python Programming - This is a textbook in Python Programming with lots of Practical Examples and Exercises. -
Bachelor Thesis of Liu (2017) [18]
Abstract of Thesis Zusammenfassung der Arbeit Abstract of Thesis Fachbereich: Electrical Engineering and Computer Science Department: : Studiengang: Information Technology University course: : Thema: Raspberry Pi Programming with Windows IoT using C# and Subject: Xamarin.Forms – Hardware connections with I²C Zusammenfassung: Abstract: The aim of this thesis is to investigate the possibilities and limitations of Raspberry Pi programming with Windows IoT operating system and Xamarin.Forms – a cross-platform development toolkit in C#. The thesis was conducted in May 2018 at Fachhochschule Lübeck. This topic is of interest because the Raspberry Pi, Windows IoT and Xamarin.Forms are new technologies for software development. This study did several programming experiments. These programs were connected to I²C devices to fulfil their tasks such as run time comparison to Python, converting Windows Forms applications to Xamarin.Forms applications, automation control program for temperature and balance, and remote control with Android smartphone. In addition, some C# external libraries like “Microcharts” for chart display and “MicroTimer” for high-performance timer were also investigated and used in the programs. The results show that Raspberry Pi with Windows IoT is capable of performing the automation control as expected, the “Microcharts” and “MicroTimer” can be easily implemented by Xamarin.Forms. Furthermore, the conversion of the old C# applications as well as the cross- platform programming in Xamarin.Forms is considerably convenient. Despite of its limitation of compatibility problems and lack of UI elements, the Xamarin.Forms with Raspberry Pi and Windows IoT has shown their potential and they may be a suitable platform for software development. This thesis may be useful in providing an alternative way of Raspberry Pi programming and cross-platform application development. -
Iostick Version 2021 SII/Abitur
IoStick Version 2021 SII/Abitur IoStick ist eine Sammlung von Programmen für den Informatikunterricht in der Schule. In Analogie zu den PortableApps sind alle Programme direkt vom USB-Stick lauffähig, eine Installation ist nicht mehr erforderlich. Dies gilt insbesondere für das Java Development Kit, das direkt auf dem IoStick installiert ist und von Java-Entwicklungsumgebungen genutzt werden kann. Neu in der Version 2021 Mit der Version 2021 wurde die Software – wenn verfügbar – auf 64 Bit umgestellt. In der Regel sind die Betriebssystemversionen von Windows 10 im schulischen Einsatz auch 64bittig. Sollte das Betriebssystem dies nicht unterstützen, so muss auf den IoStick 2020 ausgewichen werden. Außerdem ist nun die Installation in tieferliegende Ordnerstrukturen möglich. Installation/Einrichtung des Sticks Alle Dateien müssen unter Beibehaltung der Ordnerstruktur in ein Verzeichnis eines beschreibbaren Datenträgers entpackt werden. Dieser Vorgang kann in Abhängigkeit von der Geschwindigkeit des USB-Sticks zwischen 30 und 300 Minuten in Anspruch nehmen! Der Stick benötigt auf dem Datenträger einen Speicherplatz von ca. 2,2 GByte. Start und Startmenü des Sticks Das Startmenü des Sticks wird durch das Programm PStart geladen und im Systembereich neben der Uhr angezeigt. Außerdem wird das Tools Cafe gestartet. Dieses sorgt für die Assoziation von Dateitypen mit den Applikationen auf dem IoStick. Die Konfiguration erfolgt in der zugehörigen INI-Datei. Auswerfen des Sticks Das sichere Abmelden des Sticks sollte stets mit dem PStart-Menüeintrag „USB-Stick sicher auswerfen“ erfolgen. Damit werden alle ggf. noch geöffneten Dateien des Sticks geschlossen. Anpassung/Erweiterung/Reduzierungen des Sticks Für Erweiterungen sind die vorgefertigten Pakete von PortableApps besonders geeignet. Sie müssen nur in den Ordner IoStick installiert werden. -
All Installed Packages .PDF
Emperor-OS All Installed Package Editor: Hussein Seilany [email protected] www.Emperor-OS.com Emperor-has 5 package management system for installing, upgrading, configuring, and removing software. Also providing access over 60,000 software packages of Ubuntu. We per-installed several tools are available for interacting with Emperor's package management system. You can use simple command-line utilities to a graphical tools. Emperor-OS's package management system is derived from the same system used by the Ubuntu GNU/Linux distribution. We will show you the list of installed packages on Emperor-OS. The packages lists is long. Having a list of installed packages helps system administrators maintain, replicate, and reinstall Emperor-OS systems. Emperor-OS Linux systems install dependencies all the time, hence it is essential to know what is on the system. In this page you can find a specific package and python modules is installed, count installed packages and find out the version of an installed package. Download lists as PDF file or see them as html page in the following: Are you looking for all in one operating system? 70 Packages: Installed Special Packages 120 Tools: Installed Utility 260 Modules: Installed Python2 and 3 Modules 600Fonts: Installed Fonts 5 Desktops: Desktop Manager Packages 22Tools: Extra Development Tools 270 Themes: Installed Themes 40 Icons: Installed Icons 40Games: Installed Games 2533scanners: supports Scanners 2500 Cameras: supports Camera 4338Packages: All Installed Packages 2 [email protected] www.Emperor-OS.com The list installed packages: Emperor-OS Linux is an open source operating system with many utilities.