Graph Algorithms in the Language of Sparse Linear Algebra
Total Page:16
File Type:pdf, Size:1020Kb
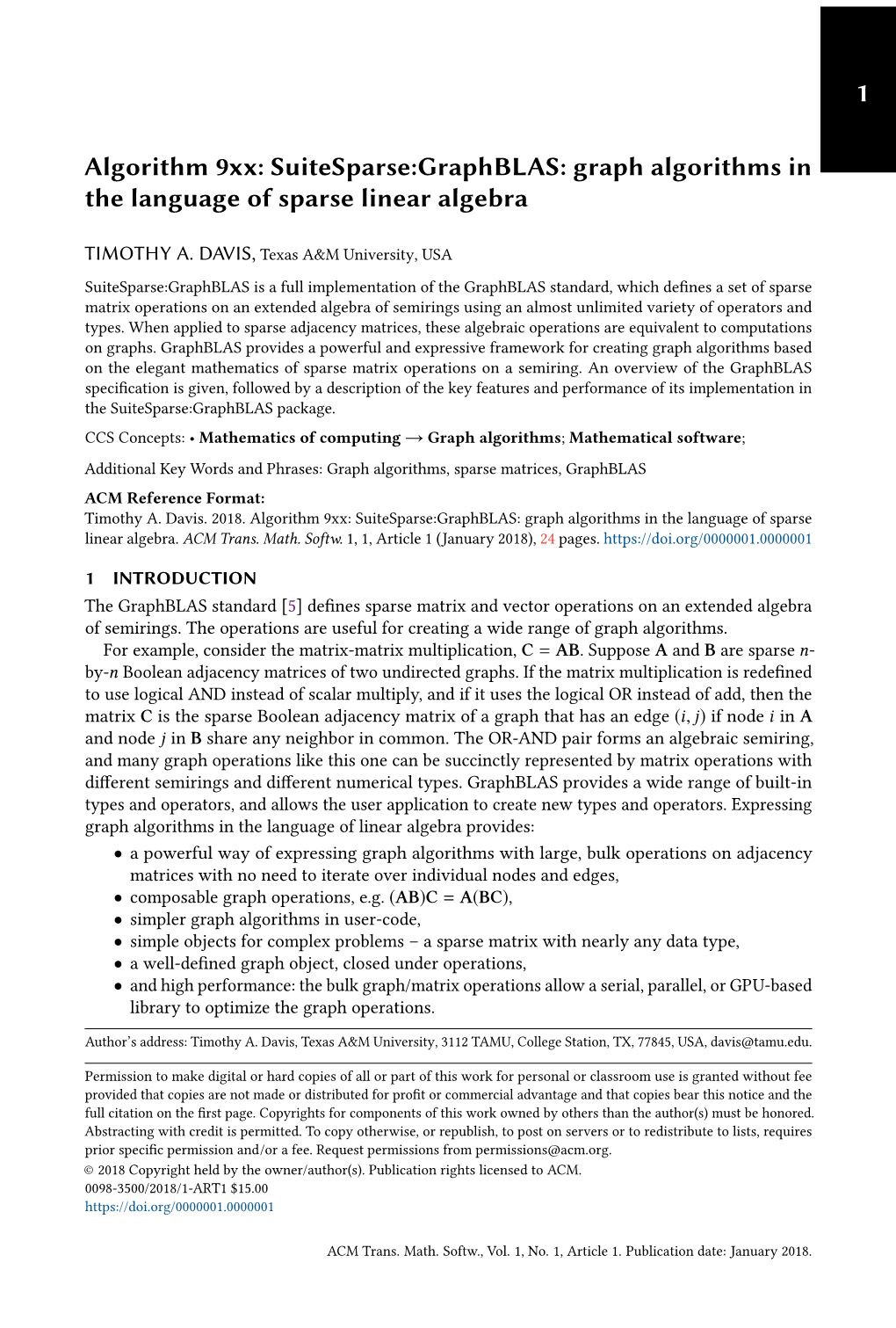
Load more
Recommended publications
-
Package 'Popbio'
Package ‘popbio’ February 1, 2020 Version 2.7 Author Chris Stubben, Brook Milligan, Patrick Nantel Maintainer Chris Stubben <[email protected]> Title Construction and Analysis of Matrix Population Models License GPL-3 Encoding UTF-8 LazyData true Suggests quadprog Description Construct and analyze projection matrix models from a demography study of marked individu- als classified by age or stage. The package covers methods described in Matrix Population Mod- els by Caswell (2001) and Quantitative Conservation Biology by Morris and Doak (2002). RoxygenNote 6.1.1 NeedsCompilation no Repository CRAN Date/Publication 2020-02-01 20:10:02 UTC R topics documented: 01.Introduction . .3 02.Caswell . .3 03.Morris . .5 aq.census . .6 aq.matrix . .7 aq.trans . .8 betaval............................................9 boot.transitions . 11 calathea . 13 countCDFxt . 14 damping.ratio . 15 eigen.analysis . 16 elasticity . 18 1 2 R topics documented: extCDF . 19 fundamental.matrix . 20 generation.time . 21 grizzly . 22 head2 . 24 hudcorrs . 25 hudmxdef . 25 hudsonia . 26 hudvrs . 27 image2 . 28 Kendall . 29 lambda . 31 lnorms . 32 logi.hist.plot . 33 LTRE ............................................ 34 matplot2 . 37 matrix2 . 38 mean.list . 39 monkeyflower . 40 multiresultm . 42 nematode . 43 net.reproductive.rate . 44 pfister.plot . 45 pop.projection . 46 projection.matrix . 48 QPmat . 50 reproductive.value . 51 resample . 52 secder . 53 sensitivity . 55 splitA . 56 stable.stage . 57 stage.vector.plot . 58 stoch.growth.rate . 59 stoch.projection . 60 stoch.quasi.ext . 62 stoch.sens . 63 stretchbetaval . 64 teasel . 65 test.census . 66 tortoise . 67 var2 ............................................. 68 varEst . 69 vitalsens . 70 vitalsim . 71 whale . 74 woodpecker . 74 Index 76 01.Introduction 3 01.Introduction Introduction to the popbio Package Description Popbio is a package for the construction and analysis of matrix population models. -
Workshop on Modern Applied Mathematics PK 2012 Kraków 23 — 24 November 2012
Workshop on Modern Applied Mathematics PK 2012 Kraków 23 — 24 November 2012 Warsztaty z Nowoczesnej Matematyki i jej Zastosowań Kraków 23 — 24 listopada 2012 Kraków 23 — 24 November 2012 3 Contents 1 Introduction 5 1.1 Organizing Committee . 6 1.2 Scientific Committee . 6 2 List of Participants 7 3 Abstracts 11 3.1 On residually finite groups Orest D. Artemovych . 11 3.2 Some properties of Bernstein-Durrmeyer operators Magdalena Baczyńska . 12 3.3 Some Applications of Gröbner Bases Katarzyna Bolek . 14 3.4 Criteria for normality via C0-semigroups and moment sequences Dariusz Cichoń . 15 3.5 Picard–Fuchs operator for certain one parameter families of Calabi–Yau threefolds Sławomir Cynk . 16 3.6 Inference with Missing data Christiana Drake . 17 3.7 An outer measure on a commutative ring Dariusz Dudzik . 18 3.8 Resampling methods for weakly dependent sequences Elżbieta Gajecka-Mirek . 18 3.9 Graphs with path systems of length k in a hamiltonian cycle Grzegorz Gancarzewicz . 19 3.10 Hausdorff gaps and automorphisms of P(N)=fin Magdalena Grzech . 20 3.11 Functional Regression Models with Structured Penalties Jarosław Harezlak, Madan Kundu . 21 3.12 Approximation of function of two variables from exponential weight spaces Monika Herzog . 22 3.13 The existence of a weak solution of the semilinear first-order differential equation in a Banach space Mariusz Jużyniec . 25 3.14 Bayesian inference for cyclostationary time series Oskar Knapik . 26 4 Workshop on Modern Applied Mathematics PK 2012 3.15 Hausdorff limit in o-minimal structures Beata Kocel–Cynk . 27 3.16 Resampling methods for nonstationary time series Jacek Leśkow . -
Projects and Publications of the Applied Mathematics Division
NATIONAL BUREAU OF STANDARDS REPORT 4546 PROJECTS and PUBLICATIONS of the APPLIED MATHEMATICS DIVISION A Quarterly Report October through December 1 955 U. S. DEPARTMENT OF COMMERCE NATIONAL BUREAU OF STANDARDS FOR OFFICIAL DISTRIBUTION U. S. DEPARTMENT OF COMMERCE Sinclair Weeks, Secretary NATIONAL BUREAU OF STANDARDS A. V. Astin, Director THE NATIONAL BUREAU OF STANDARDS The 6cope of activities of the National Bureau of Standards is suggested in the following listing of the divisions and sections engaged in technical work. In general, each section is engaged in specialized research, development, and engineering in the field indicated by its title. A brief description of the activities, and of the resultant reports and publications, appears on the inside of the back cover of this report. Electricity and Electronics. Resistance and Reactance. Electron Tubes. Electrical Instru- ments. Magnetic Measurements. Process Technology. Engineering Electronics. Electronic Instrumentation. Electrochemistry. Optics and Metrology. Photometry and Colorimetry. Optical Instruments. Photographic Technology. Length. Engineering Metrology. Heat and Power. Temperature Measurements. Thermodynamics. Cryogenic Physics. Engines and Lubrication. Engine Fuels. Atomic and Radiation Physics. Spectroscopy. Radiometry. Mass Spectrometry. Solid State Physics. Electron Physics. Atomic Physics. Nuclear Physics. Radioactivity. X-rays. Betatron. Nucleonic Instrumentation. Radiological Equipment. AEC Radiation Instruments. Chemistry. Organic Coatings. Surface Chemistry. Organic -
Projects and Publications of the Applied Mathematics Division
NATIONAL BUREAU OF STANDARDS REPORT 4658 PROJECTS and PUBLICATIONS of the APPLIED MATHEMATICS DIVISION A Quarterly Report January through March, 1956 U. S. DEPARTMENT OF COMMERCE NATIONAL BUREAU OF STANDARDS FOR OFFICIAL DISTRIBUTION U. S. DEPARTMENT OF COMMERCE Sinclair Weeks, Secretary NATIONAL BUREAU OF STANDARDS A. V. Astin, Director THE NATIONAL BUREAU OF STANDARDS The scope of activities of the National Bureau of Standards is suggested in the following listing of the divisions and sections engaged in technical work. In general, each section is engaged in specialized research, development, and engineering in the field indicated by its title. A brief description of the activities, and of the resultant reports and publications, appears on the inside of the back cover of this report. Electricity and Electronics. Resistance and Reactance. Electron Tubes. Electrical Instru- ments. Magnetic Measurements. Process Technology. Engineering Electronics. Electronic Instrumentation. Electrochemistry. Optics and Metrology. Photometry and Colorimetry. Optical Instruments. Photographic Technology. Length. Engineering Metrology. Heat and Power. Temperature Measurements. Thermodynamics. Cryogenic Physics. Engines and Lubrication. Engine Fuels. Atomic and Radiation Physics. Spectroscopy. Radiometry. Mass Spectrometry. Solid State Physics. Electron Physics. Atomic Physics. Nuclear Physics. Radioactivity. X-rays. Betatron. Nucleonic Instrumentation. Radiological Equipment. AEC Radiation Instruments. Chemistry. Organic Coatings. Surface Chemistry. Organic -
Package 'Matrix'
Package ‘Matrix’ March 8, 2019 Version 1.2-16 Date 2019-03-04 Priority recommended Title Sparse and Dense Matrix Classes and Methods Contact Doug and Martin <[email protected]> Maintainer Martin Maechler <[email protected]> Description A rich hierarchy of matrix classes, including triangular, symmetric, and diagonal matrices, both dense and sparse and with pattern, logical and numeric entries. Numerous methods for and operations on these matrices, using 'LAPACK' and 'SuiteSparse' libraries. Depends R (>= 3.2.0) Imports methods, graphics, grid, stats, utils, lattice Suggests expm, MASS Enhances MatrixModels, graph, SparseM, sfsmisc Encoding UTF-8 LazyData no LazyDataNote not possible, since we use data/*.R *and* our classes BuildResaveData no License GPL (>= 2) | file LICENCE URL http://Matrix.R-forge.R-project.org/ BugReports https://r-forge.r-project.org/tracker/?group_id=61 Author Douglas Bates [aut], Martin Maechler [aut, cre] (<https://orcid.org/0000-0002-8685-9910>), Timothy A. Davis [ctb] (SuiteSparse and 'cs' C libraries, notably CHOLMOD, AMD; collaborators listed in dir(pattern = '^[A-Z]+[.]txt$', full.names=TRUE, system.file('doc', 'SuiteSparse', package='Matrix'))), Jens Oehlschlägel [ctb] (initial nearPD()), Jason Riedy [ctb] (condest() and onenormest() for octave, Copyright: Regents of the University of California), R Core Team [ctb] (base R matrix implementation) 1 2 R topics documented: Repository CRAN Repository/R-Forge/Project matrix Repository/R-Forge/Revision 3291 Repository/R-Forge/DateTimeStamp 2019-03-04 11:00:53 Date/Publication 2019-03-08 15:33:45 UTC NeedsCompilation yes R topics documented: abIndex-class . .4 abIseq . .6 all-methods . .7 all.equal-methods . -
ESD.342 Network Representations Lecture 4
Basic Network Properties and How to Calculate Them • Data input • Number of nodes • Number of edges • Symmetry • Degree sequence • Average nodal degree • Degree distribution • Clustering coefficient • Average hop distance (simplified average distance) • Connectedness • Finding and characterizing connected clusters 2/16/2011 Network properties © Daniel E Whitney 1997-2006 1 Matlab Preliminaries • In Matlab everything is an array •So – a = [ 2 5 28 777] – for i = a – do something –end • Will “do something” for i = the values in array a • [a;b;c] yields a column containing a b c • [a b c] yields a row containing a b c 2/16/2011 Network properties © Daniel E Whitney 1997-2006 2 Matrix Representation of Networks »A=[0 1 0 1;1 0 1 1;0 1 0 0;1 1 0 0] 1 2 A = 0 1 0 1 1 3 4 0 1 1 0 1 0 0 1 1 0 0 » A is called the adjacency matrix A(i, j) = 1 if there is an arc from i to j A( j,i) = 1 if there is also an arc from j to i In this case A is symmetric and the graph is undirected 2/16/2011 Network properties © Daniel E Whitney 1997-2006 3 Directed, Undirected, Simple • Directed graphs have one-way arcs or links • Undirected graphs have two-way edges or links • Mixed graphs have some of each • Simple graphs have no multiple links between nodes and no self-loops 2/16/2011 Network properties © Daniel E Whitney 1997-2006 4 Basic Facts About Undirected Graphs • Let n be the number of nodes and m be the number of edges •Thenaverage nodal degree is < k >= 2m /n •TheDegree sequence is a list of the nodes and their respective degrees n • The sum of these degrees -
Solution of the Incompressible Navier- Stokes Equations on Unstructured Meshes
Solution of the Incompressible Navier- Stokes Equations on Unstructured Meshes by David J. Charlesworth Submitted for the Degree o f Doctor o f Philosophy The Department o f Mechanical Engineering University College London August 2003 UMI Number: U6028B6 All rights reserved INFORMATION TO ALL USERS The quality of this reproduction is dependent upon the quality of the copy submitted. In the unlikely event that the author did not send a complete manuscript and there are missing pages, these will be noted. Also, if material had to be removed, a note will indicate the deletion. Dissertation Publishing UMI U602836 Published by ProQuest LLC 2014. Copyright in the Dissertation held by the Author. Microform Edition © ProQuest LLC. All rights reserved. This work is protected against unauthorized copying under Title 17, United States Code. ProQuest LLC 789 East Eisenhower Parkway P.O. Box 1346 Ann Arbor, Ml 48106-1346 ABSTRACT Since Patankar first developed the SIMPLE (Semi Implicit Method for Pressure Linked Equations) algorithm, the incompressible Navier-Stokes equations have been solved using a variety of pressure-based methods. Over the last twenty years these methods have been refined and developed however the majority of this work has been based around the use of structured grids to mesh the fluid domain of interest. Unstructured grids offer considerable advantages over structured meshes when a fluid in a complex domain is being modelled. By using triangles in two dimensions and tetrahedrons in three dimensions it is possible to mesh many problems for which it would be impossible to use structured grids. In addition to this, unstructured grids allow meshes to be generated with relatively little effort, in comparison to structured grids, and therefore shorten the time taken to model a particular problem. -
Manifold Learning with Tensorial Network Laplacians
East Tennessee State University Digital Commons @ East Tennessee State University Electronic Theses and Dissertations Student Works 8-2021 Manifold Learning with Tensorial Network Laplacians Scott Sanders East Tennessee State University Follow this and additional works at: https://dc.etsu.edu/etd Part of the Data Science Commons, Geometry and Topology Commons, and the Other Applied Mathematics Commons Recommended Citation Sanders, Scott, "Manifold Learning with Tensorial Network Laplacians" (2021). Electronic Theses and Dissertations. Paper 3965. https://dc.etsu.edu/etd/3965 This Thesis - unrestricted is brought to you for free and open access by the Student Works at Digital Commons @ East Tennessee State University. It has been accepted for inclusion in Electronic Theses and Dissertations by an authorized administrator of Digital Commons @ East Tennessee State University. For more information, please contact [email protected]. Manifold Learning with Tensorial Network Laplacians A thesis presented to the faculty of the Department of Mathematics and Statistics East Tennessee State University In partial fulfillment of the requirements for the degree Master of Science in Mathematical Sciences by Scott Sanders August 2021 Jeff Knisley, Ph.D., Chair Michele Joyner, Ph.D. Nicole Lewis, Ph.D. Keywords: Tensors, Laplacian matrix, networks, data science, Poisson. ABSTRACT Manifold Learning with Tensorial Network Laplacians by Scott Sanders The interdisciplinary field of machine learning studies algorithms in which functional- ity is dependent on data sets. This data is often treated as a matrix, and a variety of mathematical methods have been developed to glean information from this data struc- ture such as matrix decomposition. The Laplacian matrix, for example, is commonly used to reconstruct networks, and the eigenpairs of this matrix are used in matrix decomposition. -
Author Index ______
______AUTHOR INDEX ______ a space; Homology group; Infinite Arellano, E. Ramirez de see: Ramirez de form in logarithms; Siegel theorem; dimensional space; Local decompo Arellano, E. Transcendental number sition; Metrizable space; Pontryagin __A __ Argand, J .R. see: Arithmetic; Complex Ball, R. see: Helical calculus duality; Projection spectrum; Suslin number; Imaginary number Balusabramanian, R. see: Waring prob theorem; Topological space; Width Aristotle see: Modal logic; Space-time lem Abel, N.H. see: Abel differential Alexander, J.W. see: Alexander dual Arkhangel'skil, A.V. see: Feathering Banach, S. see: Area; Banach indica equation; Abel-Goncharov prob ity; Alexander invariants; Duality; Arno I'd, V.I. see: Composite function; trix; Banach-Mazur functional; Ba lem; Abel problem; Abel theorem; Homology group; Jordan theorem; Quasi-periodic motion nach space; Banach-Steinhaus the Abel transformation; Abelian group; Knot theory; Manifold; Pontryagin Arsenin, V. Ya. see: Descriptive set the orem; Completely-continuous oper Abelian integral; Abelian variety; duality; Reaction-diffusion equation; ory ator; Contracting-mapping princi Algebra; Algebraic equation; Alge Topology of imbeddings Artin, E. see: Abstract algebraic ge ple; Franklin system; Hahn-Banach braic function; Algebraic geometry; Alexandrov, A.B. see: Boundary prop ometry; Algebra; Algebraic number theorem; Lacunary system; Linear Binomial series; Convergence, types erties of analytic functions theory; Alternative rings and alge operator; Luzin N -property; Non of; Elliptic function; Finite group; Alling, N.L. see: Surreal numbers bras; Commutative algebra; Con differentiable function; Nuclear op Galois theory; Group; Interpola Almgren, F.J. see: Plateau problem, gruence modulo a prime number; erator; Open-mapping theorem; Or tion; Inversion of an elliptic integral; multi-dimensional Divisorial ideal; Foundations of ge thogonal series; Tonelli plane vari Jacobi elliptic functions; Liouville Alvarez-Gaume, L. -
Matrices and Graphs of Relations [The Gist of Sec
Matrices and Graphs of Relations [the gist of Sec. 7.2 of Grimaldi] If A = n and B = p, and the elements are ordered and labeled (A = a ;a ;:::;a| | , etc.),| then| any relation from A to B (i.e., a subset of A B) can { 1 2 n} R × be represented by a matrix with n rows and p columns: Mjk , the element in row j and column k, equals 1 if aj bk and 0 otherwise. Such a matrix is somewhat less inscrutable than a long list ofR ordered pairs. Example: Let A = B = 1; 2; 3; 4 . The relation { } = (1; 2); (1; 3); (1; 4); (2; 3); (3; 1); (3; 4) R { } has the matrix 0111 0 00101 : B 1001C @ 0000A Boolean arithmetic Recall the operation tables for logical AND and OR: 0 1 0 1 —–—–—∧| | —–—–—∨| | 0 0 0 0 0 1 —–—–—| | —–—–—| | 1 0 1 1 1 1 | | | | Let’s compare this with arithmetical TIMES and PLUS: 0 1 + 0 1 —–—–—×| | —–—–—| | 0 0 0 0 0 1 —–—–—| | —–—–—| | 1 0 1 1 1 2 | | | | (If the addition is modulo 2, then the 2 in the crucial corner becomes 0.) We see that the logical operations are identical to the arithmetical ones, except that “1+1” must be redefined to be 1, not 2 or 0. Finally, notice that the logical relation of implication is equivalent to “less than or equal” in this numerical representation: 0 1 −→— –—–— | | 0 1 1 — –—–—| | 1 0 1 | | 1 Now recall (or learn, from Appendix 2) that multiplication of matrices is defined by (MN) = M N ik X ij jk j (i.e., each element of the product is calculated by taking the “dot product” of a row of M with a column of N; the number of columns of M must be equal to the number of rows of N for this to make sense). -
MATLAB Programming © COPYRIGHT 1984 - 2005 by the Mathworks, Inc
MATLAB® The Language of Technical Computing Programming Version 7 How to Contact The MathWorks: www.mathworks.com Web comp.soft-sys.matlab Newsgroup [email protected] Technical support [email protected] Product enhancement suggestions [email protected] Bug reports [email protected] Documentation error reports [email protected] Order status, license renewals, passcodes [email protected] Sales, pricing, and general information 508-647-7000 Phone 508-647-7001 Fax The MathWorks, Inc. Mail 3 Apple Hill Drive Natick, MA 01760-2098 For contact information about worldwide offices, see the MathWorks Web site. MATLAB Programming © COPYRIGHT 1984 - 2005 by The MathWorks, Inc. The software described in this document is furnished under a license agreement. The software may be used or copied only under the terms of the license agreement. No part of this manual may be photocopied or repro- duced in any form without prior written consent from The MathWorks, Inc. FEDERAL ACQUISITION: This provision applies to all acquisitions of the Program and Documentation by, for, or through the federal government of the United States. By accepting delivery of the Program or Documentation, the government hereby agrees that this software or documentation qualifies as commercial computer software or commercial computer software documentation as such terms are used or defined in FAR 12.212, DFARS Part 227.72, and DFARS 252.227-7014. Accordingly, the terms and conditions of this Agreement and only those rights specified in this Agreement, shall pertain to and govern the use, modification, reproduction, release, performance, display, and disclosure of the Program and Documentation by the federal government (or other entity acquiring for or through the federal government) and shall supersede any conflicting contractual terms or conditions. -
I. Two-Valued Classical Propositional Logic C2 8 1
Published by Luniver Press Beckington, Frome BA11 6TT, UK www.luniver.com Alexander S. Karpenko Lukasiewicz Logics and Prime Numbers (Luniver Press, Beckington, 2006). First Edition 2006 Copyright © 2006 Luniver Press . All rights reserved . British Library Cataloguing in Publication Data A catalog record is available from the British Library. Library of Congress Cataloguing in Publication Data A catalog record is available from the Library of Congress. All rights reserve d. This book, or parts thereof, may not be reproduced in a form or by any means , electronic or mechanical, including photocopying , recording or by any information storage and retrieval system, without permission in writing from the copyright holder. ISBN-13: 978-0-955 1170-3-9 ISBN-10: 0-955 1170-3-6 While every attempt is made to ensure that the information in this publicatic is correct. no liability can be accepted by the authors or publishers for loss. damage or injury caused by any errors in, or omission from, the informatior given . Alexander S. Karpenko Russian Academy of Sciences, Institute of Philosophy, Department of Logic ŁUKASIEWICZ'S LOGICS AND PRIME NUMBERS CONTENTS Introduction 4 I. Two-valued classical propositional logic C2 8 1. Logical connectives. Truth-tables .......................................... 8 2. The laws of classical propositional logic ................................. 10 3. Functional completeness ......................................................... 12 3.1. Sheffer stroke ........................................................................ 13 4. Axiomatization. Adequacy ……………………………… …. 17 II. Łukasiewicz’s three-valued logic Ł3 17 1. Logical fatalism………………………………………………. 17 2. Truth-tables. Axiomatization ………………………………… 19 3. Differences between Ł3 and C2 ………………………………. 21 4. An embedding C2 into Ł3 and three-valued isomorphs of C2… 23 III. Łukasiewicz’s finite-valued logics 27 1.