Dynamic Dispatch and Duck Typing
Total Page:16
File Type:pdf, Size:1020Kb
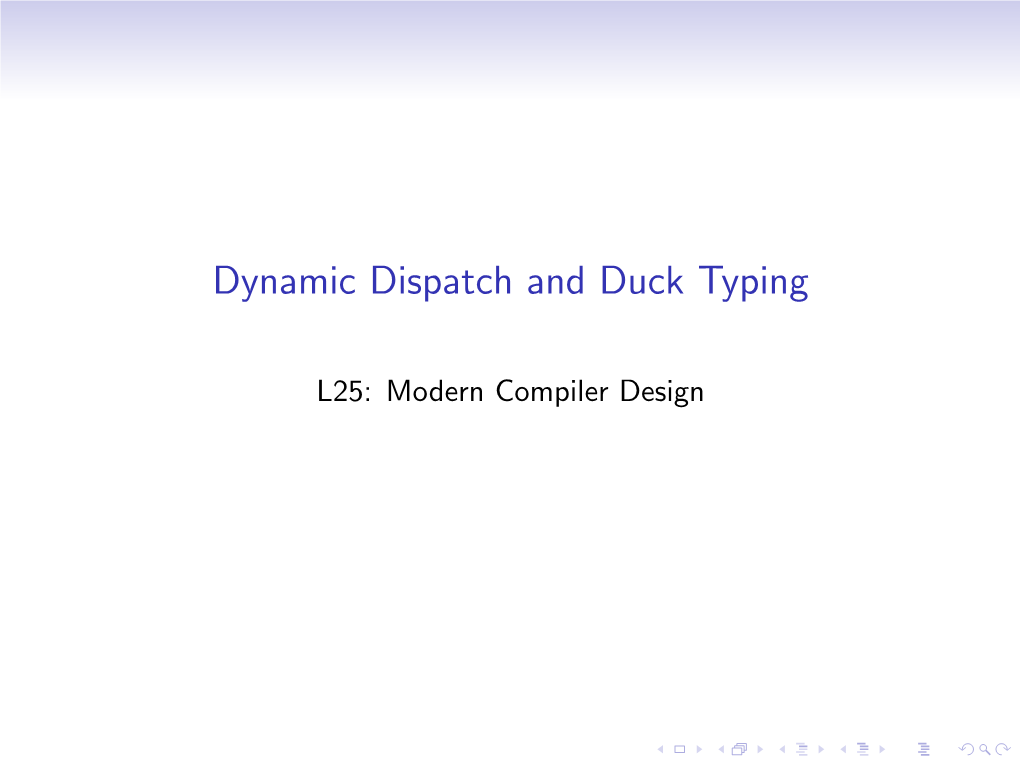
Load more
Recommended publications
-
MANNING Greenwich (74° W
Object Oriented Perl Object Oriented Perl DAMIAN CONWAY MANNING Greenwich (74° w. long.) For electronic browsing and ordering of this and other Manning books, visit http://www.manning.com. The publisher offers discounts on this book when ordered in quantity. For more information, please contact: Special Sales Department Manning Publications Co. 32 Lafayette Place Fax: (203) 661-9018 Greenwich, CT 06830 email: [email protected] ©2000 by Manning Publications Co. All rights reserved. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form or by means electronic, mechanical, photocopying, or otherwise, without prior written permission of the publisher. Many of the designations used by manufacturers and sellers to distinguish their products are claimed as trademarks. Where those designations appear in the book, and Manning Publications was aware of a trademark claim, the designations have been printed in initial caps or all caps. Recognizing the importance of preserving what has been written, it is Manning’s policy to have the books we publish printed on acid-free paper, and we exert our best efforts to that end. Library of Congress Cataloging-in-Publication Data Conway, Damian, 1964- Object oriented Perl / Damian Conway. p. cm. includes bibliographical references. ISBN 1-884777-79-1 (alk. paper) 1. Object-oriented programming (Computer science) 2. Perl (Computer program language) I. Title. QA76.64.C639 1999 005.13'3--dc21 99-27793 CIP Manning Publications Co. Copyeditor: Adrianne Harun 32 Lafayette -
Safe, Fast and Easy: Towards Scalable Scripting Languages
Safe, Fast and Easy: Towards Scalable Scripting Languages by Pottayil Harisanker Menon A dissertation submitted to The Johns Hopkins University in conformity with the requirements for the degree of Doctor of Philosophy. Baltimore, Maryland Feb, 2017 ⃝c Pottayil Harisanker Menon 2017 All rights reserved Abstract Scripting languages are immensely popular in many domains. They are char- acterized by a number of features that make it easy to develop small applications quickly - flexible data structures, simple syntax and intuitive semantics. However they are less attractive at scale: scripting languages are harder to debug, difficult to refactor and suffers performance penalties. Many research projects have tackled the issue of safety and performance for existing scripting languages with mixed results: the considerable flexibility offered by their semantics also makes them significantly harder to analyze and optimize. Previous research from our lab has led to the design of a typed scripting language built specifically to be flexible without losing static analyzability. Inthis dissertation, we present a framework to exploit this analyzability, with the aim of producing a more efficient implementation Our approach centers around the concept of adaptive tags: specialized tags attached to values that represent how it is used in the current program. Our frame- work abstractly tracks the flow of deep structural types in the program, and thuscan ii ABSTRACT efficiently tag them at runtime. Adaptive tags allow us to tackle key issuesatthe heart of performance problems of scripting languages: the framework is capable of performing efficient dispatch in the presence of flexible structures. iii Acknowledgments At the very outset, I would like to express my gratitude and appreciation to my advisor Prof. -
Comp 411 Principles of Programming Languages Lecture 19 Semantics of OO Languages
Comp 411 Principles of Programming Languages Lecture 19 Semantics of OO Languages Corky Cartwright Mar 10-19, 2021 Overview I • In OO languages, OO data values (except for designated non-OO types) are special records [structures] (finite mappings from names to values). In OO parlance, the components of record are called members. • Some members of an object may be functions called methods. Methods take this (the object in question) as an implicit parameter. Some OO languages like Java also support static methods that do not depend on this; these methods have no implicit parameters. In efficient OO language implementations, method members are shared since they are the same for all instances of a class, but this sharing is an optimization in statically typed OO languages since the collection of methods in a class is immutable during program evaluation (computation). • A method (instance method in Java) can only be invoked on an object (the receiver, an implicit parameter). Additional parameters are optional, depending on whether the method expects them. This invocation process is called dynamic dispatch because the executed code is literally extracted from the object: the code invoked at a call site depends on the value of the receiver, which can change with each execution of the call. • A language with objects is OO if it supports dynamic dispatch (discussed in more detail in Overview II & III) and inheritance, an explicit taxonomy for classifying objects based on their members and class names where superclass/parent methods are inherited unless overridden. • In single inheritance, this taxonomy forms a tree; • In multiple inheritance, it forms a rooted DAG (directed acyclic graph) where the root class is the universal class (Object in Java). -
Comparative Studies of Programming Languages; Course Lecture Notes
Comparative Studies of Programming Languages, COMP6411 Lecture Notes, Revision 1.9 Joey Paquet Serguei A. Mokhov (Eds.) August 5, 2010 arXiv:1007.2123v6 [cs.PL] 4 Aug 2010 2 Preface Lecture notes for the Comparative Studies of Programming Languages course, COMP6411, taught at the Department of Computer Science and Software Engineering, Faculty of Engineering and Computer Science, Concordia University, Montreal, QC, Canada. These notes include a compiled book of primarily related articles from the Wikipedia, the Free Encyclopedia [24], as well as Comparative Programming Languages book [7] and other resources, including our own. The original notes were compiled by Dr. Paquet [14] 3 4 Contents 1 Brief History and Genealogy of Programming Languages 7 1.1 Introduction . 7 1.1.1 Subreferences . 7 1.2 History . 7 1.2.1 Pre-computer era . 7 1.2.2 Subreferences . 8 1.2.3 Early computer era . 8 1.2.4 Subreferences . 8 1.2.5 Modern/Structured programming languages . 9 1.3 References . 19 2 Programming Paradigms 21 2.1 Introduction . 21 2.2 History . 21 2.2.1 Low-level: binary, assembly . 21 2.2.2 Procedural programming . 22 2.2.3 Object-oriented programming . 23 2.2.4 Declarative programming . 27 3 Program Evaluation 33 3.1 Program analysis and translation phases . 33 3.1.1 Front end . 33 3.1.2 Back end . 34 3.2 Compilation vs. interpretation . 34 3.2.1 Compilation . 34 3.2.2 Interpretation . 36 3.2.3 Subreferences . 37 3.3 Type System . 38 3.3.1 Type checking . 38 3.4 Memory management . -
Dynamic Dispatch
CS 3110 Lecture 24: Dynamic Dispatch Prof. Clarkson Spring 2015 Today’s music: "Te Core" by Eric Clapton Review Current topic: functional vs. object-oriented programming Today: • Continue encoding objects in OCaml • Te core of OOP – dynamic dispatch – sigma calculus Review: key features of OOP 1. Encapsulation 2. Subtyping 3. Inheritance 4. Dynamic dispatch Review: Counters class Counter {! protected int x = 0;! public int get() { return x; }! public void inc() { x++; }! }! Review: Objects • Type of object is record of functions !type counter = {! !get : unit -> int;! !inc : unit -> unit;! !} • Let-binding hides internal state (with closure) !let x = ref 0 in {! !get = (fun () -> !x);! !inc = (fun () -> x := !x+1);! !}! Review: Classes • Representation type for internal state: !type counter_rep = {! !!x : int ref;! !}! • Class is a function from representation type to object: !let counter_class (r:counter_rep) = {! !!get = (fun () -> !(r.x));! !!inc = (fun () -> (r.x := !(r.x) + 1));! !}! • Constructor uses class function to make a new object: !let new_counter () =! !!let r = {x = ref 0} in! ! !counter_class r !! Review: Inheritance • Subclass creates an object of the superclass with the same internal state as its own – Bind resulting parent object to super • Subclass creates a new object with same internal state • Subclass copies (inherits) any implementations it wants from superclass 4. DYNAMIC DISPATCH This class SetCounter {! protected int x = 0;! public int get() { return x; }! public void set(int i) { x = i; }! public void inc() -
Static Analyses for Stratego Programs
Static analyses for Stratego programs BSc project report June 30, 2011 Author name Vlad A. Vergu Author student number 1195549 Faculty Technical Informatics - ST track Company Delft Technical University Department Software Engineering Commission Ir. Bernard Sodoyer Dr. Eelco Visser 2 I Preface For the successful completion of their Bachelor of Science, students at the faculty of Computer Science of the Technical University of Delft are required to carry out a software engineering project. The present report is the conclusion of this Bachelor of Science project for the student Vlad A. Vergu. The project has been carried out within the Software Language Design and Engineering Group of the Computer Science faculty, under the direct supervision of Dr. Eelco Visser of the aforementioned department and Ir. Bernard Sodoyer. I would like to thank Dr. Eelco Visser for his past and ongoing involvment and support in my educational process and in particular for the many opportunities for interesting and challenging projects. I further want to thank Ir. Bernard Sodoyer for his support with this project and his patience with my sometimes unconvential way of working. Vlad Vergu Delft; June 30, 2011 II Summary Model driven software development is gaining momentum in the software engi- neering world. One approach to model driven software development is the design and development of domain-specific languages allowing programmers and users to spend more time on their core business and less on addressing non problem- specific issues. Language workbenches and support languages and compilers are necessary for supporting development of these domain-specific languages. One such workbench is the Spoofax Language Workbench. -
Blossom: a Language Built to Grow
Macalester College DigitalCommons@Macalester College Mathematics, Statistics, and Computer Science Honors Projects Mathematics, Statistics, and Computer Science 4-26-2016 Blossom: A Language Built to Grow Jeffrey Lyman Macalester College Follow this and additional works at: https://digitalcommons.macalester.edu/mathcs_honors Part of the Computer Sciences Commons, Mathematics Commons, and the Statistics and Probability Commons Recommended Citation Lyman, Jeffrey, "Blossom: A Language Built to Grow" (2016). Mathematics, Statistics, and Computer Science Honors Projects. 45. https://digitalcommons.macalester.edu/mathcs_honors/45 This Honors Project - Open Access is brought to you for free and open access by the Mathematics, Statistics, and Computer Science at DigitalCommons@Macalester College. It has been accepted for inclusion in Mathematics, Statistics, and Computer Science Honors Projects by an authorized administrator of DigitalCommons@Macalester College. For more information, please contact [email protected]. In Memory of Daniel Schanus Macalester College Department of Mathematics, Statistics, and Computer Science Blossom A Language Built to Grow Jeffrey Lyman April 26, 2016 Advisor Libby Shoop Readers Paul Cantrell, Brett Jackson, Libby Shoop Contents 1 Introduction 4 1.1 Blossom . .4 2 Theoretic Basis 6 2.1 The Potential of Types . .6 2.2 Type basics . .6 2.3 Subtyping . .7 2.4 Duck Typing . .8 2.5 Hindley Milner Typing . .9 2.6 Typeclasses . 10 2.7 Type Level Operators . 11 2.8 Dependent types . 11 2.9 Hoare Types . 12 2.10 Success Types . 13 2.11 Gradual Typing . 14 2.12 Synthesis . 14 3 Language Description 16 3.1 Design goals . 16 3.2 Type System . 17 3.3 Hello World . -
Specialising Dynamic Techniques for Implementing the Ruby Programming Language
SPECIALISING DYNAMIC TECHNIQUES FOR IMPLEMENTING THE RUBY PROGRAMMING LANGUAGE A thesis submitted to the University of Manchester for the degree of Doctor of Philosophy in the Faculty of Engineering and Physical Sciences 2015 By Chris Seaton School of Computer Science This published copy of the thesis contains a couple of minor typographical corrections from the version deposited in the University of Manchester Library. [email protected] chrisseaton.com/phd 2 Contents List of Listings7 List of Tables9 List of Figures 11 Abstract 15 Declaration 17 Copyright 19 Acknowledgements 21 1 Introduction 23 1.1 Dynamic Programming Languages.................. 23 1.2 Idiomatic Ruby............................ 25 1.3 Research Questions.......................... 27 1.4 Implementation Work......................... 27 1.5 Contributions............................. 28 1.6 Publications.............................. 29 1.7 Thesis Structure............................ 31 2 Characteristics of Dynamic Languages 35 2.1 Ruby.................................. 35 2.2 Ruby on Rails............................. 36 2.3 Case Study: Idiomatic Ruby..................... 37 2.4 Summary............................... 49 3 3 Implementation of Dynamic Languages 51 3.1 Foundational Techniques....................... 51 3.2 Applied Techniques.......................... 59 3.3 Implementations of Ruby....................... 65 3.4 Parallelism and Concurrency..................... 72 3.5 Summary............................... 73 4 Evaluation Methodology 75 4.1 Evaluation Philosophy -
BEGINNING OBJECT-ORIENTED PROGRAMMING with JAVASCRIPT Build up Your Javascript Skills and Embrace PRODUCT Object-Oriented Development for the Modern Web INFORMATION
BEGINNING OBJECT-ORIENTED PROGRAMMING WITH JAVASCRIPT Build up your JavaScript skills and embrace PRODUCT object-oriented development for the modern web INFORMATION FORMAT DURATION PUBLISHED ON Instructor-Led Training 3 Days 22nd November, 2017 DESCRIPTION OUTLINE JavaScript has now become a universal development Diving into Objects and OOP Principles language. Whilst offering great benefits, the complexity Creating and Managing Object Literals can be overwhelming. Defining Object Constructors Using Object Prototypes and Classes In this course we show attendees how they can write Checking Abstraction and Modeling Support robust and efficient code with JavaScript, in order to Analyzing OOP Principles in JavaScript create scalable and maintainable web applications that help developers and businesses stay competitive. Working with Encapsulation and Information Hiding Setting up Strategies for Encapsulation LEARNING OUTCOMES Using the Meta-Closure Approach By completing this course, you will: Using Property Descriptors Implementing Information Hiding in Classes • Cover the new object-oriented features introduced as a part of ECMAScript 2015 Inheriting and Creating Mixins • Build web applications that promote scalability, Implementing Objects, Inheritance, and Prototypes maintainability and usability Using and Controlling Class Inheritance • Learn about key principles like object inheritance and Implementing Multiple Inheritance JavaScript mixins Creating and Using Mixins • Discover how to skilfully develop asynchronous code Defining Contracts with Duck Typing within larger JS applications Managing Dynamic Typing • Complete a variety of hands-on activities to build Defining Contracts and Interfaces up your experience of real-world challenges and Implementing Duck Typing problems Comparing Duck Typing and Polymorphism WHO SHOULD ATTEND Advanced Object Creation Mastering Patterns, Object Creation, and Singletons This course is for existing developers who are new to Implementing an Object Factory object-oriented programming in the JavaScript language. -
Subclassing and Dynamic Dispatch
Subclassing and Dynamic Dispatch 6.170 Lecture 3 This lecture is about dynamic dispatch: how a call o.m() may actually invoke the code of different methods, all with the same name m, depending on the runtime type of the receiver object o. To explain how this happens, we show how one class can be defined as a subclass of another, and can override some of its methods. This is called subclassing or inheritance, and it is a central feature of object oriented languages. At the same time, it’s arguably a rather dangerous and overrused feature, for reasons that we’ll discuss later when we look at subtyping. The notion of dynamic dispatch is broader than the notion of inheritance. You can write a Java program with no inheritance in which dynamic dispatch still occurs. This is because Java has built- in support for specifications. You can declare an object to satisfy a kind of partial specification called an interface, and you can provide many implementations of the same interface. This is actually a more important and fundamental notion than subclassing, and it will be discussed in our lecture on specification. 1 Bank Transaction Code Here is the code for a class representing a bank account: class Account { String name; Vector transv; int balance; Account (String n) { transv = new Vector (); balance = 0; name = n; } boolean checkTrans (Trans t) { return (balance + t.amount >= 0); } void post (Trans t) { transv.add (t); balance += t.amount; } } and a class representing a transaction: class Trans { int amount; 1 Date date; ... } 2 Extending a Class by Inheritance Suppose we want to implement a new kind of account that allows overdrafts. -
4500/6500 Programming Languages What Are Types? Different Definitions
What are Types? ! Denotational: Collection of values from domain CSCI: 4500/6500 Programming » integers, doubles, characters ! Abstraction: Collection of operations that can be Languages applied to entities of that type ! Structural: Internal structure of a bunch of data, described down to the level of a small set of Types fundamental types ! Implementers: Equivalence classes 1 Maria Hybinette, UGA Maria Hybinette, UGA 2 Different Definitions: Types versus Typeless What are types good for? ! Documentation: Type for specific purposes documents 1. "Typed" or not depends on how the interpretation of the program data representations is determined » Example: Person, BankAccount, Window, Dictionary » When an operator is applied to data objects, the ! Safety: Values are used consistent with their types. interpretation of their values could be determined either by the operator or by the data objects themselves » Limit valid set of operation » Languages in which the interpretation of a data object is » Type Checking: Prevents meaningless operations (or determined by the operator applied to it are called catch enough information to be useful) typeless languages; ! Efficiency: Exploit additional information » those in which the interpretation is determined by the » Example: a type may dictate alignment at at a multiple data object itself are called typed languages. of 4, the compiler may be able to use more efficient 2. Sometimes it just means that the object does not need machine code instructions to be explicitly declared (Visual Basic, Jscript -
Topic 9: Type Checking • Readings: • Chapter 13.5, 13.6 and 13.7
Recommended Exercises and Readings • From Haskell: The craft of functional programming (3rd Ed.) • Exercises: • 13.17, 13.18, 13.19, 13.20, 13.21, 13.22 Topic 9: Type Checking • Readings: • Chapter 13.5, 13.6 and 13.7 1 2 Type systems Static vs. Dynamic Typing • There are (at least) three different ways that we can compare type • Static typing systems • The type of a variable is known at compile time • Static vs. dynamic typing • It is explicitly stated by the programmer… • Strong vs. weak typing • Or it can be inferred from context • Explicit vs. implicit typing • Type checking can be performed without running the program • Used by Haskell • Also used by C, C++, C#, Java, Fortran, Pascal, … 3 4 Static vs. Dynamic Typing Static vs. Dynamic Typing • Dynamic typing • Static vs. Dynamic typing is a question of timing… • The type of a variable is not known until the program is running • When is type checking performed? • Typically determined from the value that is actually stored in it • At compile time (before the program runs)? Static • Program can crash at runtime due to incorrect types • As the program is running? Dynamic • Permits downcasting, dynamic dispatch, reflection, … • Used by Python, Javascript, PHP, Lisp, Ruby, … 5 6 Static vs. Dynamic Typing Static vs. Dynamic Typing • Some languages do a mixture of both of static and dynamic typing • Advantages of static typing • Most type checking in Java is performed at compile time… • … but downcasting is permitted, and can’t be verified at compile time • Generated code includes a runtime type check • A ClassCastException is thrown if the downcast wasn’t valid • Advantages of dynamic typing • Most type checking in C++ is performed at compile time.