Optimizing and Evaluating Transient Gradual Typing
Total Page:16
File Type:pdf, Size:1020Kb
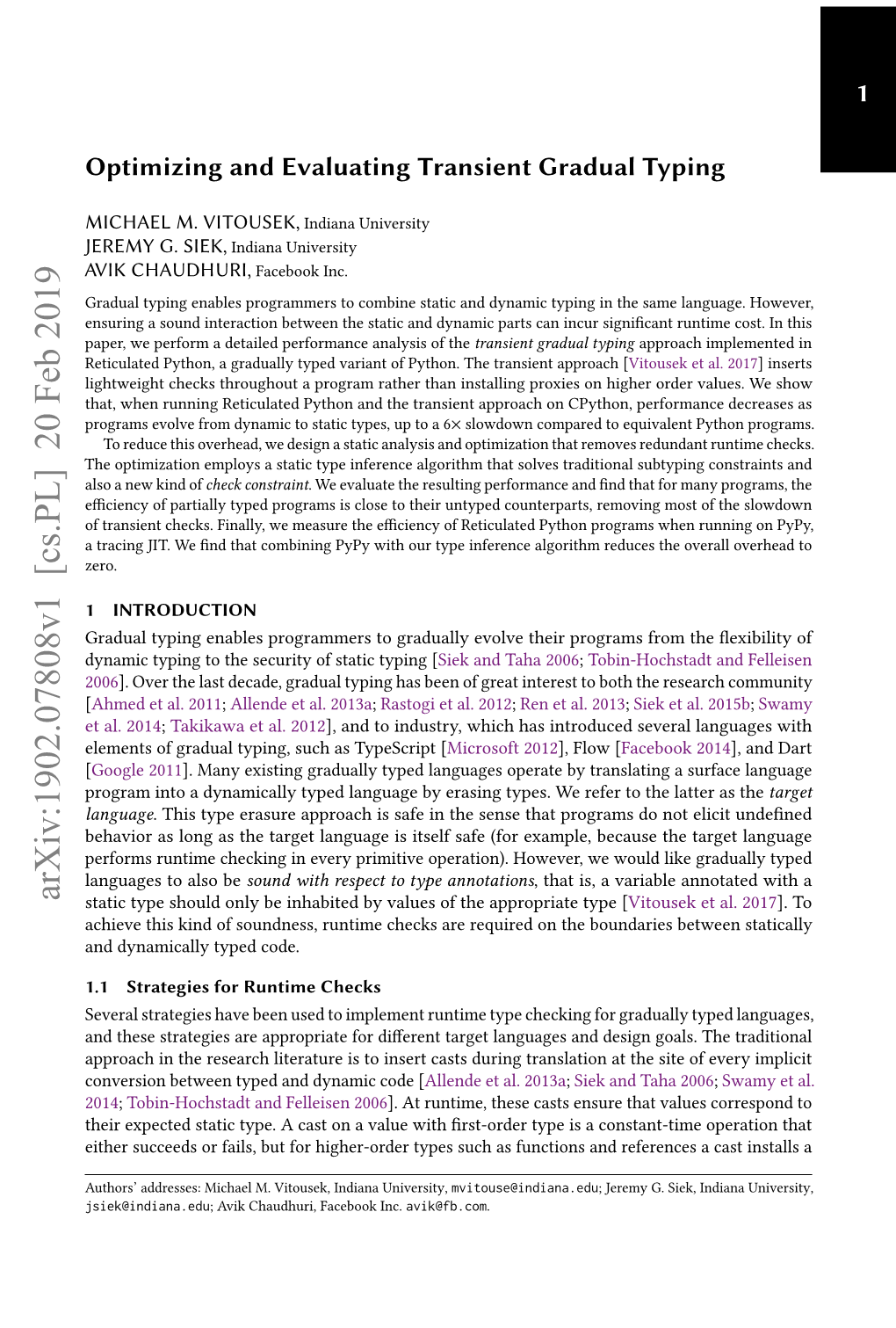
Load more
Recommended publications
-
Gradual Typing with Inference Jeremy Siek University of Colorado at Boulder
Gradual Typing with Inference Jeremy Siek University of Colorado at Boulder joint work with Manish Vachharajani Overview • Motivation • Background • Gradual Typing • Unification-based inference • Exploring the Solution Space • Type system (specification) • Inference algorithm (implementation) Why Gradual Typing? • Static and dynamic type systems have complimentary strengths. • Static typing provides full-coverage error checking, efficient execution, and machine-checked documentation. • Dynamic typing enables rapid development and fast adaption to changing requirements. • Why not have both in the same language? Java Python Goals for gradual typing Treat programs without type annotations as • dynamically typed. Programmers may incrementally add type • annotations to gradually increase static checking. Annotate all parameters and the type system • catches all type errors. 4 Goals for gradual typing Treat programs without type annotations as • dynamically typed. Programmers may incrementally add type • annotations to gradually increase static checking. Annotate all parameters and the type system • catches all type errors. dynamic static 4 Goals for gradual typing Treat programs without type annotations as • dynamically typed. Programmers may incrementally add type • annotations to gradually increase static checking. Annotate all parameters and the type system • catches all type errors. dynamic gradual static 4 The Gradual Type System • Classify dynamically typed expressions with the type ‘?’ • Allow implicit coercions to ? and from ? with any other type • Extend coercions to compound types using a new consistency relation 5 Coercions to and from ‘?’ (λa:int. (λx. x + 1) a) 1 Parameters with no type annotation are given the dynamic type ‘?’. 6 Coercions to and from ‘?’ ? (λa:int. (λx. x + 1) a) 1 Parameters with no type annotation are given the dynamic type ‘?’. -
Principal Type Schemes for Gradual Programs with Updates and Corrections Since Publication (Latest: May 16, 2017)
Principal Type Schemes for Gradual Programs With updates and corrections since publication (Latest: May 16, 2017) Ronald Garcia ∗ Matteo Cimini y Software Practices Lab Indiana University Department of Computer Science [email protected] University of British Columbia [email protected] Abstract to Siek and Taha (2006) has been used to integrate dynamic checks Gradual typing is a discipline for integrating dynamic checking into into a variety of type structures, including object-oriented (Siek a static type system. Since its introduction in functional languages, and Taha 2007), substructural (Wolff et al. 2011), and ownership it has been adapted to a variety of type systems, including object- types (Sergey and Clarke 2012). The key technical pillars of grad- oriented, security, and substructural. This work studies its applica- ual typing are the unknown type, ?, the consistency relation among tion to implicitly typed languages based on type inference. Siek gradual types, and support for the entire spectrum between purely and Vachharajani designed a gradual type inference system and dynamic and purely static checking. A gradual type checker rejects algorithm that infers gradual types but still rejects ill-typed static type inconsistencies in programs, accepts statically safe code, and programs. However, the type system requires local reasoning about instruments code that could plausibly be safe with runtime checks. type substitutions, an imperative inference algorithm, and a subtle This paper applies the gradual typing approach to implicitly correctness statement. typed languages, like Standard ML, OCaml, and Haskell, where This paper introduces a new approach to gradual type inference, a type inference (a.k.a. type reconstruction) procedure is integral to type checking. -
Gradual Typing for a More Pure Javascript
! Gradual Typing for a More Pure JavaScript Bachelor’s Thesis in Computer Science and Engineering JAKOB ERLANDSSON ERIK NYGREN OSKAR VIGREN ANTON WESTBERG Department of Computer Science and Engineering CHALMERS TEKNISKA HÖGSKOLA GÖTEBORGS UNIVERSITET Göteborg, Sverige 2019 Abstract Dynamically typed languages have surged in popularity in recent years, owing to their flexibility and ease of use. However, for projects of a certain size dynamic typing can cause problems of maintainability as refactoring becomes increas- ingly difficult. One proposed solution is the use of gradual type systems, where static type annotations are optional. This results in providing the best of both worlds. The purpose of this project is to create a gradual type system on top of JavaScript. Another goal is to explore the possibility of making guarantees about function purity and immutability using the type system. The types and their relations are defined and a basic type checker is implemented to confirm the ideas. Extending type systems to be aware of side effects makes it easier to write safer software. It is concluded that all of this is possible and reasonable to do in JavaScript. Sammanfattning Dynamiskt typade programmeringsspråk har ökat kraftigt i popularitet de se- naste åren tack vare deras flexibilitet och användbarhet. För projekt av en viss storlek kan dock dynamisk typning skapa underhållsproblem då omstrukture- ring av kod blir allt svårare. En föreslagen lösning är användande av så kallad gradvis typning där statiskt typad annotering är frivillig, vilket i teorin fångar det bästa av två världar. Syftet med det här projektet är att skapa ett gradvist typsystem ovanpå Javascript. -
Reconciling Noninterference and Gradual Typing
Reconciling noninterference and gradual typing Arthur Azevedo de Amorim Matt Fredrikson Limin Jia Carnegie Mellon University Carnegie Mellon University Carnegie Mellon University Abstract let f x = One of the standard correctness criteria for gradual typing is let b (* : Bool<S> *) = true in the dynamic gradual guarantee, which ensures that loosening let y = ref b in type annotations in a program does not affect its behavior in let z = ref b in arbitrary ways. Though natural, prior work has pointed out if x then y := false else (); that the guarantee does not hold of any gradual type system if !y then z := false else (); !z for information-flow control. Toro et al.’s GSLRef language, for example, had to abandon it to validate noninterference. We show that we can solve this conflict by avoiding a fea- f (<S>true) ture of prior proposals: type-guided classification, or the use of type ascription to classify data. Gradual languages require Figure 1. Prototypical failure of the DGG due to NSU checks. run-time secrecy labels to enforce security dynamically; if The program throws an error when run, but successfully type ascription merely checks these labels without modifying terminates if we uncomment the type annotation Bool<S>. them (that is, without classifying data), it cannot violate the dynamic gradual guarantee. We demonstrate this idea with GLIO, a gradual type system based on the LIO library that Sadly, the guarantee does not hold in any existing gradual enforces both the gradual guarantee and noninterference, language for information-flow control (IFC) [33]. featuring higher-order functions, general references, coarse- The goal of this paper is to remedy the situation for IFC lan- grained information-flow control, security subtyping and guages without giving up on noninterference. -
Safe & Efficient Gradual Typing for Typescript
Safe & Efficient Gradual Typing for TypeScript (MSR-TR-2014-99) Aseem Rastogi ∗ Nikhil Swamy Cedric´ Fournet Gavin Bierman ∗ Panagiotis Vekris University of Maryland, College Park MSR Oracle Labs UC San Diego [email protected] fnswamy, [email protected] [email protected] [email protected] Abstract without either rejecting most programs or requiring extensive an- Current proposals for adding gradual typing to JavaScript, such notations (perhaps using a PhD-level type system). as Closure, TypeScript and Dart, forgo soundness to deal with Gradual type systems set out to fix this problem in a principled issues of scale, code reuse, and popular programming patterns. manner, and have led to popular proposals for JavaScript, notably We show how to address these issues in practice while retain- Closure, TypeScript and Dart (although the latter is strictly speak- ing soundness. We design and implement a new gradual type sys- ing not JavaScript but a variant with some features of JavaScript re- tem, prototyped for expediency as a ‘Safe’ compilation mode for moved). These proposals bring substantial benefits to the working TypeScript.1Our compiler achieves soundness by enforcing stricter programmer, usually taken for granted in typed languages, such as static checks and embedding residual runtime checks in compiled a convenient notation for documenting code; API exploration; code code. It emits plain JavaScript that runs on stock virtual machines. completion; refactoring; and diagnostics of basic type errors. Inter- Our main theorem is a simulation that ensures that the checks in- estingly, to be usable at scale, all these proposals are intentionally troduced by Safe TypeScript (1) catch any dynamic type error, and unsound: typeful programs may be easier to write and maintain, but (2) do not alter the semantics of type-safe TypeScript code. -
The Dynamic Practice and Static Theory of Gradual Typing Michael Greenberg Pomona College, Claremont, CA, USA [email protected]
The Dynamic Practice and Static Theory of Gradual Typing Michael Greenberg Pomona College, Claremont, CA, USA http://www.cs.pomona.edu/~michael/ [email protected] Abstract We can tease apart the research on gradual types into two ‘lineages’: a pragmatic, implementation- oriented dynamic-first lineage and a formal, type-theoretic, static-first lineage. The dynamic-first lineage’s focus is on taming particular idioms—‘pre-existing conditions’ in untyped programming languages. The static-first lineage’s focus is on interoperation and individual type system features, rather than the collection of features found in any particular language. Both appear in programming languages research under the name “gradual typing”, and they are in active conversation with each other. What are these two lineages? What challenges and opportunities await the static-first lineage? What progress has been made so far? 2012 ACM Subject Classification Social and professional topics → History of programming lan- guages; Software and its engineering → Language features Keywords and phrases dynamic typing, gradual typing, static typing, implementation, theory, challenge problems Acknowledgements I thank Sam Tobin-Hochstadt and David Van Horn for their hearty if dubious encouragment. Conversations with Sam, Ron Garcia, Matthias Felleisen, Robby Findler, and Spencer Florence improved the argumentation. Stephanie Weirich helped me with Haskell programming; Richard Eisenberg wrote the program in Figure 3. Ross Tate, Neel Krishnaswami, Ron, and Éric Tanter provided helpful references I had overlooked. Ben Greenman provided helpful feedback. The anonymous SNAPL reviewers provided insightful comments as well as the CDuce code in Section 3.4.3. Finally, I thank Stephen Chong and Harvard Computer Science for hosting me while on sabbatical. -
Refined Criteria for Gradual Typing
Refined Criteria for Gradual Typing∗ Jeremy G. Siek1, Michael M. Vitousek1, Matteo Cimini1, and John Tang Boyland2 1 Indiana University – Bloomington, School of Informatics and Computing 150 S. Woodlawn Ave. Bloomington, IN 47405, USA [email protected] 2 University of Wisconsin – Milwaukee, Department of EECS PO Box 784, Milwaukee WI 53201, USA [email protected] Abstract Siek and Taha [2006] coined the term gradual typing to describe a theory for integrating static and dynamic typing within a single language that 1) puts the programmer in control of which regions of code are statically or dynamically typed and 2) enables the gradual evolution of code between the two typing disciplines. Since 2006, the term gradual typing has become quite popular but its meaning has become diluted to encompass anything related to the integration of static and dynamic typing. This dilution is partly the fault of the original paper, which provided an incomplete formal characterization of what it means to be gradually typed. In this paper we draw a crisp line in the sand that includes a new formal property, named the gradual guarantee, that relates the behavior of programs that differ only with respect to their type annotations. We argue that the gradual guarantee provides important guidance for designers of gradually typed languages. We survey the gradual typing literature, critiquing designs in light of the gradual guarantee. We also report on a mechanized proof that the gradual guarantee holds for the Gradually Typed Lambda Calculus. 1998 ACM Subject Classification F.3.3 Studies of Program Constructs – Type structure Keywords and phrases gradual typing, type systems, semantics, dynamic languages Digital Object Identifier 10.4230/LIPIcs.SNAPL.2015.274 1 Introduction Statically and dynamically typed languages have complementary strengths. -
Gradual Session Types
JFP 29, e17, 56 pages, 2019. c The Author(s) 2019. This is an Open Access article, distributed under 1 the terms of the Creative Commons Attribution licence (http://creativecommons.org/licenses/by/4.0/), which permits unrestricted re-use, distribution, and reproduction in any medium, provided the original work is properly cited. doi:10.1017/S0956796819000169 Gradual session types ATSUSHI IGARASHI Kyoto University, Japan (e-mail: [email protected]) PETER THIEMANN University of Freiburg, Germany (e-mail: [email protected]) YUYA TSUDA Kyoto University, Japan (e-mail: [email protected]) VASCO T. VASCONCELOS LASIGE, Department of Informatics, Faculty of Sciences, University of Lisbon, Portugal (e-mail: [email protected]) PHILIP WADLER University of Edinburgh, Scotland (e-mail: [email protected]) Abstract Session types are a rich type discipline, based on linear types, that lifts the sort of safety claims that come with type systems to communications. However, web-based applications and microser- vices are often written in a mix of languages, with type disciplines in a spectrum between static and dynamic typing. Gradual session types address this mixed setting by providing a framework which grants seamless transition between statically typed handling of sessions and any required degree of dynamic typing. We propose Gradual GV as a gradually typed extension of the functional session type system GV. Following a standard framework of gradual typing, Gradual GV consists of an external language, which relaxes the type system of GV using dynamic types; an internal language with casts, for which operational semantics is given; and a cast-insertion translation from the former to the latter. -
Gradual Typing for Objects Benjamin Chung, Paley Li, Francesco Zappa Nardelli, Jan Vitek
KafKa: Gradual Typing for Objects Benjamin Chung, Paley Li, Francesco Zappa Nardelli, Jan Vitek To cite this version: Benjamin Chung, Paley Li, Francesco Zappa Nardelli, Jan Vitek. KafKa: Gradual Typing for Objects. ECOOP 2018 - 2018 European Conference on Object-Oriented Programming, Jul 2018, Amsterdam, Netherlands. hal-01882148 HAL Id: hal-01882148 https://hal.inria.fr/hal-01882148 Submitted on 26 Sep 2018 HAL is a multi-disciplinary open access L’archive ouverte pluridisciplinaire HAL, est archive for the deposit and dissemination of sci- destinée au dépôt et à la diffusion de documents entific research documents, whether they are pub- scientifiques de niveau recherche, publiés ou non, lished or not. The documents may come from émanant des établissements d’enseignement et de teaching and research institutions in France or recherche français ou étrangers, des laboratoires abroad, or from public or private research centers. publics ou privés. KafKa: Gradual Typing for Objects Benjamin Chung Northeastern University, Boston, MA, USA Paley Li Czech Technical University, Prague, Czech Republic, and Northeastern University, Boston, MA, USA Francesco Zappa Nardelli Inria, Paris, France, and Northeastern University, Boston, MA, USA Jan Vitek Czech Technical University, Prague, Czech Republic, and Northeastern University, Boston, MA, USA Abstract A wide range of gradual type systems have been proposed, providing many languages with the ability to mix typed and untyped code. However, hiding under language details, these gradual type systems embody fundamentally different ideas of what it means to be well-typed. In this paper, we show that four of the most common gradual type systems provide distinct guarantees, and we give a formal framework for comparing gradual type systems for object- oriented languages. -
Reconciling Noninterference and Gradual Typing Full Version with Definitions and Proofs
Reconciling noninterference and gradual typing Full Version with Definitions and Proofs Arthur Azevedo de Amorim Matt Fredrikson Limin Jia Carnegie Mellon University Carnegie Mellon University Carnegie Mellon University Abstract let f x = One of the standard correctness criteria for gradual typing is let b (* : Bool<S> *) = true in the dynamic gradual guarantee, which ensures that loosening let y = ref b in type annotations in a program does not affect its behavior in let z = ref b in arbitrary ways. Though natural, prior work has pointed out if x then y := false else (); that the guarantee does not hold of any gradual type system if !y then z := false else (); !z for information-flow control. Toro et al.’s GSLRef language, for example, had to abandon it to validate noninterference. We show that we can solve this conflict by avoiding a fea- f (<S>true) ture of prior proposals: type-guided classification, or the use of type ascription to classify data. Gradual languages require Figure 1. Prototypical failure of the DGG due to NSU checks. run-time secrecy labels to enforce security dynamically; if The program throws an error when run, but successfully type ascription merely checks these labels without modifying terminates if we uncomment the type annotation Bool<S>. them (that is, without classifying data), it cannot violate the dynamic gradual guarantee. We demonstrate this idea with for more than basic type safety. It had to be abandoned in a GLIO, a gradual type system based on the LIO library that gradual variant of System F to enforce parametricity [33],1 enforces both the gradual guarantee and noninterference, and in the GSLRef language [32] to enforce noninterference. -
Gradual Typing for Functional Languages
Gradual Typing for Functional Languages Jeremy G. Siek Walid Taha University of Colorado Rice University [email protected] [email protected] Abstract ling the degree of static checking by annotating function parameters with types, or not. We use the term gradual typing for type systems Static and dynamic type systems have well-known strengths and that provide this capability. Languages that support gradual typing weaknesses, and each is better suited for different programming to a large degree include Cecil [8], Boo [10], extensions to Visual tasks. There have been many efforts to integrate static and dynamic Basic.NET and C# proposed by Meijer and Drayton [26], and ex- typing and thereby combine the benefits of both typing disciplines tensions to Java proposed by Gray et al. [17], and the Bigloo [6, 36] in the same language. The flexibility of static typing can be im- dialect of Scheme [24]. The purpose of this paper is to provide a proved by adding a type Dynamic and a typecase form. The safety type-theoretic foundation for languages such as these with gradual and performance of dynamic typing can be improved by adding typing. optional type annotations or by performing type inference (as in soft typing). However, there has been little formal work on type There are numerous other ways to combine static and dynamic typ- systems that allow a programmer-controlled migration between dy- ing that fall outside the scope of gradual typing. Many dynamically namic and static typing. Thatte proposed Quasi-Static Typing, but typed languages have optional type annotations that are used to im- it does not statically catch all type errors in completely annotated prove run-time performance but not to increase the amount of static programs. -
Gradual Typing for First-Class Classes∗
Gradual Typing for First-Class Classes ∗ Asumu Takikawa T. Stephen Strickland Christos Dimoulas Sam Tobin-Hochstadt Matthias Felleisen PLT, Northeastern University asumu, sstrickl, chrdimo, samth, matthias @ccs.neu.edu f g Abstract 1. Untyped Object-Oriented Style Dynamic type-checking and object-oriented programming The popularity of untyped programming languages such as often go hand-in-hand; scripting languages such as Python, Python, Ruby, or JavaScript has stimulated work on com- Ruby, and JavaScript all embrace object-oriented (OO) pro- bining static and dynamic type-checking. The idea is now gramming. When scripts written in such languages grow and popularly called gradual typing [27]. At this point, gradual evolve into large programs, the lack of a static type disci- typing is available for functional programming languages pline reduces maintainability. A programmer may thus wish such as Racket [33, 34], for object-oriented languages such to migrate parts of such scripts to a sister language with a as Ruby [12] or Thorn [38], and for Visual Basic [23] on the static type system. Unfortunately, existing type systems nei- .NET platform. Proposals for gradual typing also exist for ther support the flexible OO composition mechanisms found JavaScript [19] and Perl [31]. Formal models have validated in scripting languages nor accommodate sound interopera- soundness for gradual type systems, allowing seamless in- tion with untyped code. teroperation between sister languages [22, 27, 32]. In this paper, we present the design of a gradual typing system that supports sound interaction between statically- (define drracket-frame% and dynamically-typed units of class-based code. The type (size-pref-mixin system uses row polymorphism for classes and thus supports (searchable-text-mixin mixin-based OO composition.