Su20-Quiz1-Solutions
Total Page:16
File Type:pdf, Size:1020Kb
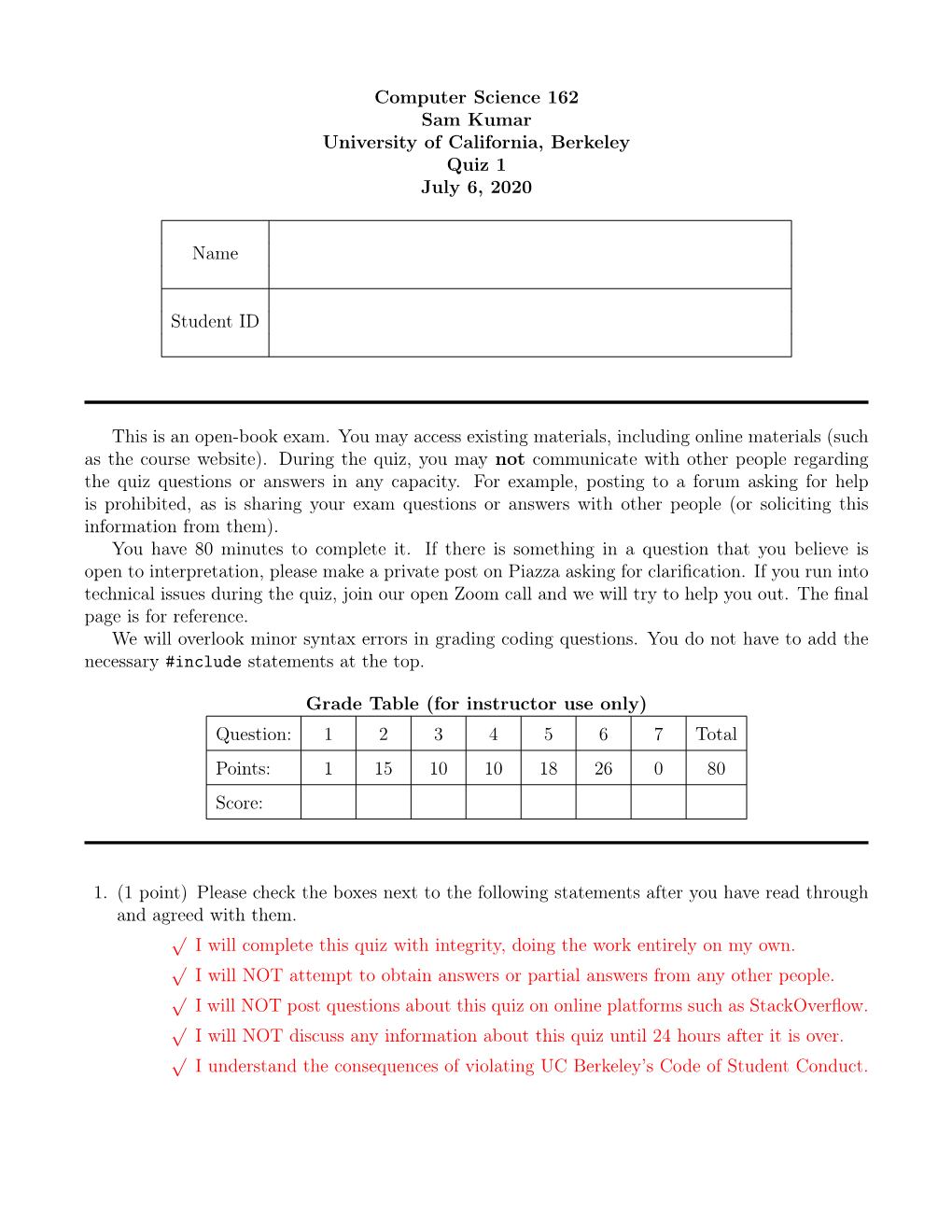
Load more
Recommended publications
-
Operating Systems (Undergrad): Spring 2020 Midterm Exam
New York University CSCI-UA.202: Operating Systems (Undergrad): Spring 2020 Midterm Exam • This exam is 75 minutes. • The answer sheet is available here: – Section 001 (Aurojit Panda) – Section 003 (Michael Walfish) The answer sheet has the hand-in instructions and the video upload instructions. • There are 14 problems in this booklet. Many can be answered quickly. Some may be harder than others, and some earn more points than others. You may want to skim all questions before starting. • This exam is closed book and notes. You may not use electronics: phones, tablets, calculators, laptops, etc. You may refer to ONE two-sided 8.5x11” sheet with 10 point or larger Times New Roman font, 1 inch or larger margins, and a maximum of 55 lines per side. • Do not waste time on arithmetic. Write answers in powers of 2 if necessary. • If you find a question unclear or ambiguous, be sure to write any assumptions you make. • Follow the instructions: if they ask you to justify something, explain your reasoning and any important assumptions. Write brief, precise answers. Rambling brain dumps will not work and will waste time. Think before you start writing so that you can answer crisply. Be neat. If we can’t understand your answer, we can’t give you credit! • If the questions impose a sentence limit, we will not read past that limit. In addition, a response that includes the correct answer, along with irrelevant or incorrect content, will lose points. • Don’t linger. If you know the answer, give it, and move on. -
Automatic Collection of Leaked Resources
IEICE TRANS. INF. & SYST., VOL.E96–D, NO.1 JANUARY 2013 28 PAPER Resco: Automatic Collection of Leaked Resources Ziying DAI†a), Student Member, Xiaoguang MAO†b), Nonmember, Yan LEI†, Student Member, Xiaomin WAN†, and Kerong BEN††, Nonmembers SUMMARY A garbage collector relieves programmers from manual rent approaches either strive to detect and fix these leaks memory management and improves productivity and program reliability. or provide new language features to simplify resource man- However, there are many other finite system resources that programmers agement [2], [3], [5]–[8]. Although these approaches can must manage by themselves, such as sockets and database connections. Growing resource leaks can lead to performance degradation and even achieve success to some extent, there are some escaped re- program crashes. This paper presents the automatic resource collection source leaks that manifest themselves after programs have approach called Resco (RESource COllector) to tolerate non-memory re- been deployed. We also note that similar to memory leaks, source leaks. Resco prevents performance degradation and crashes due to leaks of other resources are not necessarily a problem. A resource leaks by two steps. First, it utilizes monitors to count resource ff consumption and request resource collections independently of memory few or a small amount of leaked resources will neither a ect usage when resource limits are about to be violated. Second, it responds program behavior nor performance. Only when accumu- to a resource collection request by safely releasing leaked resources. We lated leaks exhaust all available resources or lead to large ex- implement Resco based on a Java Virtual Machine for Java programs. -
State-Taint Analysis for Detecting Resource Bugs
JID:SCICO AID:2114 /FLA [m3G; v1.218; Prn:10/07/2017; 11:30] P.1(1-17) Science of Computer Programming ••• (••••) •••–••• Contents lists available at ScienceDirect Science of Computer Programming www.elsevier.com/locate/scico State-taint analysis for detecting resource bugs ∗ Zhiwu Xu a, Cheng Wen a, Shengchao Qin b,a, a College of Computer Science and Software Engineering, Shenzhen University, China b School of Computing, Teesside University, UK a r t i c l e i n f o a b s t r a c t Article history: To ensure that a program uses its resources in an appropriate manner is vital for program Received 3 January 2017 correctness. A number of solutions have been proposed to check that programs meet such Received in revised form 20 June 2017 a property on resource usage. But many of them are sophisticated to use for resource Accepted 25 June 2017 bug detection in practice and do not take into account the expectation that a resource Available online xxxx should be used once it is opened or required. This open-but-not-used problem can cause Keywords: resource starvation in some cases, for example, smartphones or other mobile devices where Resource bug resources are not only scarce but also energy-hungry, hence inappropriate resource usage Static analysis can not only cause the system to run out of resources but also lead to much shorter battery Energy leak life between battery recharge. That is the so-call energy leak problem. Android In this paper, we propose a static analysis called state-taint analysis to detect resource bugs. -
Debug Your Threading and Memory Errors
Kevin O’Leary Intel SPDK, PMDK, Intel® Performance Analyzers Virtual Forum 01 Intel Inspector overview 02 Intel Inspector features 03 Summary SPDK, PMDK, Intel® Performance Analyzers Virtual Forum 2 Intel® Trace Analyzer Cluster N Scalable Tune MPI & Collector (ITAC) ? Intel APS Intel MPI Tuner Y Memory Effective Y Bandwidth N threading Vectorize Sensitive ? Intel® Inspector ? N Find any Y correctness errors Optimize Thread in your threads and bandwidth memory! Intel® Intel® Intel® VTune™ Profiler Advisor VTune™ Profiler SPDK, PMDK, Intel® Performance Analyzers Virtual Forum 3 Size and complexity of Correctness tools find defects applications is growing during development prior to shipment Reworking defects is 40%-50% Reduce time, effort, and of total project effort cost to repair Find errors earlier when they are less expensive to fix SPDK, PMDK, Intel® Performance Analyzers Virtual Forum 4 Debugger Breakpoints Correctness Tools Increase ROI By 12%-21%1 ▪ Errors found earlier are less expensive to fix ▪ Several studies, ROI% varies, but earlier is cheaper Diagnosing Some Errors Can Take Months ▪ Races & deadlocks not easily reproduced ▪ Memory errors can be hard to find without a tool Intel® Inspector dramatically sped up Debugger Integration Speeds Diagnosis our ability to track down difficult to isolate threading errors before our ▪ Breakpoint set just before the problem packages are released to the field. ▪ Examine variables & threads with the debugger Peter von Kaenel, Director, Diagnose in hours instead of months Software Development, -
Unit-Iv Process,Signals and File Locking
UNIT-IV PROCESS, SIGNALS AND FILE LOCKING III-I R14 - 14BT50502 PROCESS: When you execute a program on your UNIX system, the system creates a special environment for that program. This environment contains everything needed for the system to run the program as if no other program were running on the system. Whenever you issue a command in UNIX, it creates, or starts, a new process. When you tried out the ls command to list directory contents, you started a process. A process, in simple terms, is an instance of a running program. When you start a process (run a command), there are two ways you can run it − Foreground Processes Background Processes FOREGROUND PROCESSES: By default, every process that you start runs in the foreground. It gets its input from the keyboard and sends its output to the screen. Example: $ls ch*.doc When a program is running in foreground and taking much time, we cannot run any other commands (start any other processes) because prompt would not be available until program finishes its processing and comes out. BACKGROUND PROCESSES: A background process runs without being connected to your keyboard. If the background process requires any keyboard input, it waits. The advantage of running a process in the background is that you can run other commands; you do not have to wait until it completes to start another! The simplest way to start a background process is to add an ampersand (&) at the end of the command. Example: $ls ch*.doc & PROCESS IDENTIFIERS: The process identifier – normally referred to as the process ID or just PID, is a number used by most operating system kernels such as that of UNIX, Mac OS X or Microsoft Windows — to uniquely identify an active process. -
State-Taint Analysis for Detecting Resource Bugs
State-Taint Analysis for Detecting Resource Bugs Zhiwu Xu1 Dongxiao Fan1 Shengchao Qin2,1 1 College of Computer Science and Software Engineering, Shenzhen University, China 2 School of Computing, Teesside University, UK Email: [email protected], [email protected], [email protected] Abstract—To verify whether a program uses resources in it unused/unattended, in other cases this may lead to a valid manner is vital for program correctness. A number of more severe problems. For instance, when some resource solutions have been proposed to ensure such a property for is very limited and is not released timely, this issue can resource usage. But most of them are sophisticated to use for resource bugs detection in practice and do not concern about lead to a major problem, causing resource starvation the issue that an opened resource should be used. This open- and severe system slowdown or instability. Specifically, but-not-used problem can cause resource starvation in some for mobile devices, such as tablets and smartphones, case as well. In particular, resources of smartphones are not which have become hugely popular, the resource is only scarce but also energy-hungry. The misuse of resources rather limited, specially power energy (i.e. the battery could not only cause the system to run out of resources but also lead to a shorter battery life. That is the so-call energy capacity). Most resources of mobile devices are not only leak problem. scarce but also energy-hungry, such as GPS, WiFi, camera, Aiming to provide a lightweight method and to detect and so on. -
Processes, Address Spaces, and Context Switches
Processes, Address Spaces, and Context Switches Chester Rebeiro IIT Madras Executing Apps (Process) • Process – A program in execution – Most important abstraction in an OS – Comprises of $gcc hello.c • Code from ELF In the • Data user space • Stack of process ELF • Heap Executable Process • State in the OS In the kernel (a.out) $./a.out • Kernel stack space – State contains : registers, list of open files, related processes, etc. 2 Program ≠ Process Program Process code + static and global data Dynamic instantiation of code + data + heap + stack + process state One program can create several A process is unique isolated entity processes 3 Process Address Space • Virtual Address Map MAX_SIZE – All memory a process can Stack address – Large contiguous array of addresses from 0 to Heap MAX_SIZE Data Text (instructions) 0 4 Process Address Space • Each process has a different address space • This is achieved by the use of virtual memory • Ie. 0 to MAX_SIZE are virtual memory addresses MAX_SIZE Stack Stack MAX_SIZE Heap Heap Data Data Process A Process B Process A Text Text Process B Page Table (instructions) (instructions) Page Table 0 0 5 Virtual Address Mapping Process A Process B Stack Stack Heap Heap Process A Process B Data Page Page Data Table Table Text Text (instructions) (instructions) Virtual Memory Physical Memory Virtual Memory 6 Advantages of Virtual Address Map • Isolation (private address space) – One process cannot access another process’ memory • Relocatable – Data and code within the process is relocatable • Size – Processes can be much larger than physical memory 7 Kernel can access User Process can access Address Map in xv6 Process DeviceMemory (instructions) Text + Data, Text Kernel Stack Heap Data Text (0x80000000) KERNBASE 0xFE000000 0 • every process address space address process every mapped kernel into Entire – – kernelspace Easyaccess userof datafrom duringsystem calls) usercode kernelto code(ie. -
Lecture 3 : Process Management
Lecture 3 : Process Management INSTRUCTOR : DR. BIBHAS GHOSHAL ([email protected]) 1 Lecture Outline What is Process? Process States Process Creation Process Address Space Creating a process by Cloning Process Termination Zombie Process Orphans Process Process Address Map in xv6 2 Process Program in Execution Process Comprises of: #include<stdio.h> . Code int main(){ . Data In User space of process Char str[] = “Hello World!\n”; . Stack Printf(“%s”,str); . Heap } . State in OS . $ gcc hello.c Kernel stack In Kernel space of process ELF Process Executable(a.out) $ ./a.out Program Process . Code + static & global data . Dynamic instantiation of code + data + heap + stack + process . One program can create several state processes . A process is unique isolated entity 3 Data Structure of Process Stack Recursive calls Attributes of a Process . Process Id Heap Dynamic allocation of data structures . Program counter . Static Variable Process State . & Once created, it will be there for lifetime Priority . Global Variable General Purpose Register . List of open files Executable a.out . List of open devices . Protection While executing a process, you are restricted to process boundary or else segmentation fault will occur. Heap grows upwards while Stack grows downwards. 4 Process State Process Scheduled Exit NEW READY RUN Terminate ◦ Created I/O Request over I/O Request WAIT Running: In the running state, a process is running on a processor. This means it is executing instructions. Ready: In the ready state, a process is ready to run but for some reason the OS has chosen not to run it at this given moment. Wait: In the blocked state, a process has performed some kind of operation that makes it not ready to run until some other event takes place. -
Lightweight and Modular Resource Leak Verification
Lightweight and Modular Resource Leak Verification Martin Kellogg∗ Narges Shadab∗ Manu Sridharan Michael D. Ernst University of Washington, University of California, University of California, University of Washington, Seattle, USA Riverside, USA Riverside, USA Seattle, USA [email protected] [email protected] [email protected] [email protected] ABSTRACT 1 INTRODUCTION A resource leak occurs when a program allocates a resource, such as A resource leak occurs when some finite resource managed by the a socket or file handle, but fails to deallocate it. Resource leaks cause programmer is not explicitly disposed of. In an unmanaged lan- resource starvation, slowdowns, and crashes. Previous techniques to guage like C, that explicit resource might be memory; in a managed prevent resource leaks are either unsound, imprecise, inapplicable language like Java, it might be a file descriptor, a socket, or a data- to existing code, slow, or a combination of these. base connection. Resource leaks continue to cause severe failures, Static detection of resource leaks requires checking that de- even in modern, heavily-used Java applications [15]. This state- allocation methods are always invoked on relevant objects before of-the-practice does not differ much from two decades ago [35]. they become unreachable. Our key insight is that leak detection can Microsoft engineers consider resource leaks to be one of the most be reduced to an accumulation problem, a class of typestate prob- significant development challenges22 [ ]. Preventing resource leaks lems amenable to sound and modular checking without the need remains an urgent, difficult, open problem. for a heavyweight, whole-program alias analysis. -
Downloaded from Some Repair a Leak) That Consistently Produces Correct and Safe Chat Room
JOURNAL OF LATEX CLASS FILES, VOL. 14, NO. 8, AUGUST 2015 1 Automated Repair of Resource Leaks in Android Applications Bhargav Nagaraja Bhatt and Carlo A. Furia Abstract—Resource leaks—a program does not release resources it previously acquired—are a common kind of bug in Android applications. Even with the help of existing techniques to automatically detect leaks, writing a leak-free program remains tricky. One of the reasons is Android’s event-driven programming model, which complicates the understanding of an application’s overall control flow. In this paper, we present PLUMBDROID: a technique to automatically detect and fix resource leaks in Android applications. PLUMBDROID builds a succinct abstraction of an app’s control flow, and uses it to find execution traces that may leak a resource. The information built during detection also enables automatically building a fix—consisting of release operations performed at appropriate locations—that removes the leak and does not otherwise affect the application’s usage of the resource. An empirical evaluation on resource leaks from the DROIDLEAKS curated collection demonstrates that PLUMBDROID’s approach is scalable, precise, and produces correct fixes for a variety of resource leak bugs: PLUMBDROID automatically found and repaired 50 leaks that affect 9 widely used resources of the Android system, including all those collected by DROIDLEAKS for those resources; on average, it took just 2 minutes to detect and repair a leak. PLUMBDROID also compares favorably to Relda2/RelFix—the only other fully automated approach to repair Android resource leaks—since it usually detects more leaks with higher precision and producing smaller fixes. -
Executing Apps (Process)
Processes Chester Rebeiro IIT Madras Executing Apps (Process) • Process – A program in execution – Most important abstraction in an OS – Comprises of $gcc hello.c • Code • Data from ELF In the user space • Stack of process ELF • Heap Executable Process • State in the OS In the kernel (a.out) $./a.out • Kernel stack space – State contains : registers, list of open files, related processes, etc. 2 Program ≠ Process Program Process code + static and global data Dynamic instantiation of code + data + heap + stack + process state One program can create several A process is unique isolated entity processes 3 Process Address Space • Virtual Address Map MAX_SIZE – All memory a process can Stack address – Large contiguous array of addresses from 0 to Heap MAX_SIZE Data Text (instructions) 0 4 Process Address Space • Each process has a different address space • This is achieved by the use of virtual memory • Ie. 0 to MAX_SIZE are virtual memory addresses MAX_SIZE Stack Stack MAX_SIZE Heap Heap Data Data Process A Process B Process A Process B Page Table Text Text (instructions) (instructions) Page Table 0 0 5 Virtual Address Mapping Process A Process B Stack Stack Heap Heap Process A Process B Data Page Page Data Table Table Text Text (instructions) (instructions) Virtual Memory Physical Memory Virtual Memory 6 Advantages of Virtual Address Map • Isolation (private address space) – One process cannot access another process’ memory • Relocatable – Data and code within the process is relocatable • Size – Processes can be much larger than physical memory 7 Process Address Map in xv6 0xFE000000 • Entire kernel mapped into every process address space Kernel Text + Data, – This allows easy switching from DeviceMemory user code to kernel code (ie. -
Specification and Analysis of Resource Utilization Policies for Human-Intensive Systems
University of Massachusetts Amherst ScholarWorks@UMass Amherst Doctoral Dissertations Dissertations and Theses November 2016 Specification and Analysis of Resource Utilization Policies for Human-Intensive Systems Seung Yeob Shin University of Massachusetts - Amherst Follow this and additional works at: https://scholarworks.umass.edu/dissertations_2 Part of the Software Engineering Commons Recommended Citation Shin, Seung Yeob, "Specification and Analysis of Resource Utilization Policies for Human-Intensive Systems" (2016). Doctoral Dissertations. 805. https://scholarworks.umass.edu/dissertations_2/805 This Open Access Dissertation is brought to you for free and open access by the Dissertations and Theses at ScholarWorks@UMass Amherst. It has been accepted for inclusion in Doctoral Dissertations by an authorized administrator of ScholarWorks@UMass Amherst. For more information, please contact [email protected]. SPECIFICATION AND ANALYSIS OF RESOURCE UTILIZATION POLICIES FOR HUMAN-INTENSIVE SYSTEMS A Dissertation Presented by SEUNG YEOB SHIN Submitted to the Graduate School of the University of Massachusetts Amherst in partial fulfillment of the requirements for the degree of DOCTOR OF PHILOSOPHY September 2016 Computer Science c Copyright by Seung Yeob Shin 2016 All Rights Reserved SPECIFICATION AND ANALYSIS OF RESOURCE UTILIZATION POLICIES FOR HUMAN-INTENSIVE SYSTEMS A Dissertation Presented by SEUNG YEOB SHIN Approved as to style and content by: Leon J. Osterweil, Co-chair Yuriy Brun, Co-chair Lori A. Clarke, Member George S. Avrunin, Member Hari Balasubramanian, Member James Allan, Chair of the Faculty College of Information and Computer Sciences DEDICATION To my family ACKNOWLEDGMENTS First and foremost, I would like to express my sincere gratitude to my advisors: Leon J. Osterweil and Yuriy Brun.