1 Introduction
Total Page:16
File Type:pdf, Size:1020Kb
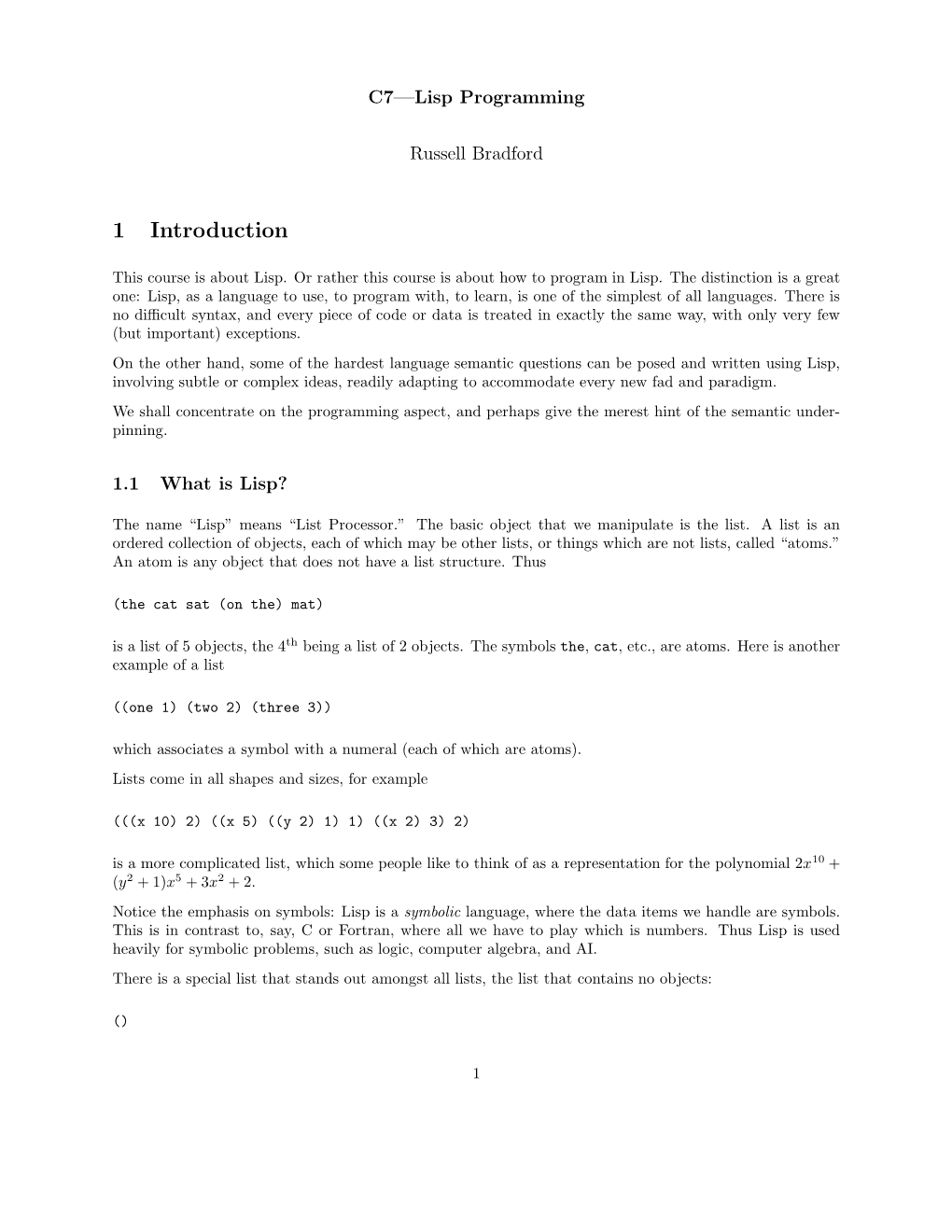
Load more
Recommended publications
-
Introduction to Programming in Lisp
Introduction to Programming in Lisp Supplementary handout for 4th Year AI lectures · D W Murray · Hilary 1991 1 Background There are two widely used languages for AI, viz. Lisp and Prolog. The latter is the language for Logic Programming, but much of the remainder of the work is programmed in Lisp. Lisp is the general language for AI because it allows us to manipulate symbols and ideas in a commonsense manner. Lisp is an acronym for List Processing, a reference to the basic syntax of the language and aim of the language. The earliest list processing language was in fact IPL developed in the mid 1950’s by Simon, Newell and Shaw. Lisp itself was conceived by John McCarthy and students in the late 1950’s for use in the newly-named field of artificial intelligence. It caught on quickly in MIT’s AI Project, was implemented on the IBM 704 and by 1962 to spread through other AI groups. AI is still the largest application area for the language, but the removal of many of the flaws of early versions of the language have resulted in its gaining somewhat wider acceptance. One snag with Lisp is that although it started out as a very pure language based on mathematic logic, practical pressures mean that it has grown. There were many dialects which threaten the unity of the language, but recently there was a concerted effort to develop a more standard Lisp, viz. Common Lisp. Other Lisps you may hear of are FranzLisp, MacLisp, InterLisp, Cambridge Lisp, Le Lisp, ... Some good things about Lisp are: • Lisp is an early example of an interpreted language (though it can be compiled). -
High-Level Language Features Not Found in Ordinary LISP. the GLISP
DOCUMENT RESUME ED 232 860 SE 042 634 AUTHOR Novak, Gordon S., Jr. TITLE GLISP User's Manual. Revised. INSTITUTION Stanford Univ., Calif. Dept. of Computer Science. SPONS AGENCY Advanced Research Projects Agency (DOD), Washington, D.C.; National Science Foundation, Washington, D.C. PUB DATE 23 Nov 82 CONTRACT MDA-903-80-c-007 GRANT SED-7912803 NOTE 43p.; For related documents, see SE 042 630-635. PUB TYPE Guides General (050) Reference Materials General (130) EDRS PRICE MF01/PCO2 Plus Postage. DESCRIPTORS *Computer Programs; *Computer Science; Guides; *Programing; *Programing Languages; *Resource Materials IDENTIFIERS *GLISP Programing Language; National Science Foundation ABSTRACT GLISP is a LISP-based language which provides high-level language features not found in ordinary LISP. The GLISP language is implemented by means of a compiler which accepts GLISP as input and produces ordinary LISP as output. This output can be further compiled to machine code by the LISP compiler. GLISP is available for several ISP dialects, including Interlisp, Maclisp, UCI Lisp, ELISP, Franz Lisp, and Portable Standard Lisp. The goal of GLISP is to allow structured objects to be referenced in a convenient, succinct language and to allow the structures of objects to be changed without changing the code which references the objects. GLISP provides both PASCAL-like and English-like syntaxes; much of the power and brevity of GLISP derive from the compiler features necessary to support the relatively informal, English-like language constructs. Provided in this manual is the documentation necessary for using GLISP. The documentation is presented in the following sections: introduction; object descriptions; reference to objects; GLISP program syntax; messages; context rules and reference; GLISP and knowledge representation languages; obtaining and using GLISP; GLISP hacks (some ways of doing things in GLISP which might not be entirely obvious at first glance); and examples of GLISP object declarations and programs. -
Implementation Notes
IMPLEMENTATION NOTES XEROX 3102464 lyric Release June 1987 XEROX COMMON LISP IMPLEMENTATION NOTES 3102464 Lyric Release June 1987 The information in this document is subject to change without notice and should not be construed as a commitment by Xerox Corporation. While every effort has been made to ensure the accuracy of this document, Xerox Corporation assumes no responsibility for any errors that may appear. Copyright @ 1987 by Xerox Corporation. Xerox Common Lisp is a trademark. All rights reserved. "Copyright protection claimed includes all forms and matters of copyrightable material and information now allowed by statutory or judicial law or hereinafter granted, including, without limitation, material generated from the software programs which are displayed on the screen, such as icons, screen display looks, etc. " This manual is set in Modern typeface with text written and formatted on Xerox Artificial Intelligence workstations. Xerox laser printers were used to produce text masters. PREFACE The Xerox Common Lisp Implementation Notes cover several aspects of the Lyric release. In these notes you will find: • An explanation of how Xerox Common Lisp extends the Common Lisp standard. For example, in Xerox Common Lisp the Common Lisp array-constructing function make-array has additional keyword arguments that enhance its functionality. • An explanation of how several ambiguities in Steele's Common Lisp: the Language were resolved. • A description of additional features that provide far more than extensions to Common Lisp. How the Implementation Notes are Organized . These notes are intended to accompany the Guy L. Steele book, Common Lisp: the Language which represents the current standard for Co~mon Lisp. -
PUB DAM Oct 67 CONTRACT N00014-83-6-0148; N00014-83-K-0655 NOTE 63P
DOCUMENT RESUME ED 290 438 IR 012 986 AUTHOR Cunningham, Robert E.; And Others TITLE Chips: A Tool for Developing Software Interfaces Interactively. INSTITUTION Pittsburgh Univ., Pa. Learning Research and Development Center. SPANS AGENCY Office of Naval Research, Arlington, Va. REPORT NO TR-LEP-4 PUB DAM Oct 67 CONTRACT N00014-83-6-0148; N00014-83-K-0655 NOTE 63p. PUB TYPE Reports - Research/Technical (143) EDRS PRICE MF01/PC03 Plus Postage. DESCRIPTORS *Computer Graphics; *Man Machine Systems; Menu Driven Software; Programing; *Programing Languages IDENTIF7ERS Direct Manipulation Interface' Interface Design Theory; *Learning Research and Development Center; LISP Programing Language; Object Oriented Programing ABSTRACT This report provides a detailed description of Chips, an interactive tool for developing software employing graphical/computer interfaces on Xerox Lisp machines. It is noted that Chips, which is implemented as a collection of customizable classes, provides the programmer with a rich graphical interface for the creation of rich graphical interfaces, and the end-user with classes for modeling the graphical relationships of objects on the screen and maintaining constraints between them. This description of the system is divided into five main sections: () the introduction, which provides background material and a general description of the system; (2) a brief overview of the report; (3) detailed explanations of the major features of Chips;(4) an in-depth discussion of the interactive aspects of the Chips development environment; and (5) an example session using Chips to develop and modify a small portion of an interface. Appended materials include descriptions of four programming techniques that have been sound useful in the development of Chips; descriptions of several systems developed at the Learning Research and Development Centel tsing Chips; and a glossary of key terms used in the report. -
The Evolution of Lisp
1 The Evolution of Lisp Guy L. Steele Jr. Richard P. Gabriel Thinking Machines Corporation Lucid, Inc. 245 First Street 707 Laurel Street Cambridge, Massachusetts 02142 Menlo Park, California 94025 Phone: (617) 234-2860 Phone: (415) 329-8400 FAX: (617) 243-4444 FAX: (415) 329-8480 E-mail: [email protected] E-mail: [email protected] Abstract Lisp is the world’s greatest programming language—or so its proponents think. The structure of Lisp makes it easy to extend the language or even to implement entirely new dialects without starting from scratch. Overall, the evolution of Lisp has been guided more by institutional rivalry, one-upsmanship, and the glee born of technical cleverness that is characteristic of the “hacker culture” than by sober assessments of technical requirements. Nevertheless this process has eventually produced both an industrial- strength programming language, messy but powerful, and a technically pure dialect, small but powerful, that is suitable for use by programming-language theoreticians. We pick up where McCarthy’s paper in the first HOPL conference left off. We trace the development chronologically from the era of the PDP-6, through the heyday of Interlisp and MacLisp, past the ascension and decline of special purpose Lisp machines, to the present era of standardization activities. We then examine the technical evolution of a few representative language features, including both some notable successes and some notable failures, that illuminate design issues that distinguish Lisp from other programming languages. We also discuss the use of Lisp as a laboratory for designing other programming languages. We conclude with some reflections on the forces that have driven the evolution of Lisp. -
Balancing the Eulisp Metaobject Protocol
Balancing the EuLisp Metaob ject Proto col x y x z Harry Bretthauer and Harley Davis and Jurgen Kopp and Keith Playford x German National Research Center for Computer Science GMD PO Box W Sankt Augustin FRG y ILOG SA avenue Gallieni Gentilly France z Department of Mathematical Sciences University of Bath Bath BA AY UK techniques which can b e used to solve them Op en questions Abstract and unsolved problems are presented to direct future work The challenge for the metaob ject proto col designer is to bal One of the main problems is to nd a b etter balance ance the conicting demands of eciency simplicity and b etween expressiveness and ease of use on the one hand extensibili ty It is imp ossible to know all desired extensions and eciency on the other in advance some of them will require greater functionality Since the authors of this pap er and other memb ers while others require greater eciency In addition the pro of the EuLisp committee have b een engaged in the design to col itself must b e suciently simple that it can b e fully and implementation of an ob ject system with a metaob ject do cumented and understo o d by those who need to use it proto col for EuLisp Padget and Nuyens intended This pap er presents a metaob ject proto col for EuLisp to correct some of the p erceived aws in CLOS to sim which provides expressiveness by a multileveled proto col plify it without losing any of its p ower and to provide the and achieves eciency by static semantics for predened means to more easily implement it eciently The current metaob -
Allegro CL User Guide
Allegro CL User Guide Volume 1 (of 2) version 4.3 March, 1996 Copyright and other notices: This is revision 6 of this manual. This manual has Franz Inc. document number D-U-00-000-01-60320-1-6. Copyright 1985-1996 by Franz Inc. All rights reserved. No part of this pub- lication may be reproduced, stored in a retrieval system, or transmitted, in any form or by any means electronic, mechanical, by photocopying or recording, or otherwise, without the prior and explicit written permission of Franz incorpo- rated. Restricted rights legend: Use, duplication, and disclosure by the United States Government are subject to Restricted Rights for Commercial Software devel- oped at private expense as specified in DOD FAR 52.227-7013 (c) (1) (ii). Allegro CL and Allegro Composer are registered trademarks of Franz Inc. Allegro Common Windows, Allegro Presto, Allegro Runtime, and Allegro Matrix are trademarks of Franz inc. Unix is a trademark of AT&T. The Allegro CL software as provided may contain material copyright Xerox Corp. and the Open Systems Foundation. All such material is used and distrib- uted with permission. Other, uncopyrighted material originally developed at MIT and at CMU is also included. Appendix B is a reproduction of chapters 5 and 6 of The Art of the Metaobject Protocol by G. Kiczales, J. des Rivieres, and D. Bobrow. All this material is used with permission and we thank the authors and their publishers for letting us reproduce their material. Contents Volume 1 Preface 1 Introduction 1.1 The language 1-1 1.2 History 1-1 1.3 Format -
1960 1970 1980 1990 Japan California………………… UT IL in PA NJ NY Massachusetts………… Europe Lisp 1.5 TX
Japan UT IL PA NY Massachusetts………… Europe California………………… TX IN NJ Lisp 1.5 1960 1970 1980 1990 Direct Relationships Lisp 1.5 LISP Family Graph Lisp 1.5 LISP 1.5 Family Lisp 1.5 Basic PDP-1 LISP M-460 LISP 7090 LISP 1.5 BBN PDP-1 LISP 7094 LISP 1.5 Stanford PDP-1 LISP SDS-940 LISP Q-32 LISP PDP-6 LISP LISP 2 MLISP MIT PDP-1 LISP Stanford LISP 1.6 Cambridge LISP Standard LISP UCI-LISP MacLisp Family PDP-6 LISP MacLisp Multics MacLisp CMU SAIL MacLisp MacLisp VLISP Franz Lisp Machine Lisp LISP S-1 Lisp NIL Spice Lisp Zetalisp IBM Lisp Family 7090 LISP 1.5 Lisp360 Lisp370 Interlisp Illinois SAIL MacLisp VM LISP Interlisp Family SDS-940 LISP BBN-LISP Interlisp 370 Spaghetti stacks Interlisp LOOPS Common LOOPS Lisp Machines Spice Lisp Machine Lisp Interlisp Lisp (MIT CONS) (Xerox (BBN Jericho) (PERQ) (MIT CADR) Alto, Dorado, (LMI Lambda) Dolphin, Dandelion) Lisp Zetalisp TAO (3600) (ELIS) Machine Lisp (TI Explorer) Common Lisp Family APL APL\360 ECL Interlisp ARPA S-1 Lisp Scheme Meeting Spice at SRI, Lisp April 1991 PSL KCL NIL CLTL1 Zetalisp X3J13 CLTL2 CLOS ISLISP X3J13 Scheme Extended Family COMIT Algol 60 SNOBOL METEOR CONVERT Simula 67 Planner LOGO DAISY, Muddle Scheme 311, Microplanner Scheme 84 Conniver PLASMA (actors) Scheme Revised Scheme T 2 CLTL1 Revised Scheme Revised2 Scheme 3 CScheme Revised Scheme MacScheme PC Scheme Chez Scheme IEEE Revised4 Scheme Scheme Object-Oriented Influence Simula 67 Smalltalk-71 LOGO Smalltalk-72 CLU PLASMA (actors) Flavors Common Objects LOOPS ObjectLisp CommonLOOPS EuLisp New Flavors CLTL2 CLOS Dylan X3J13 ISLISP Lambda Calculus Church, 1941 λ Influence Lisp 1.5 Landin (SECD, ISWIM) Evans (PAL) Reynolds (Definitional Interpreters) Lisp370 Scheme HOPE ML Standard ML Standard ML of New Jersey Haskell FORTRAN Influence FORTRAN FLPL Lisp 1.5 S-1 Lisp Lisp Machine Lisp CLTL1 Revised3 Zetalisp Scheme X3J13 CLTL2 IEEE Scheme X3J13 John McCarthy Lisp 1.5 Danny Bobrow Richard Gabriel Guy Steele Dave Moon Jon L White. -
Lisp: Program Is Data
LISP: PROGRAM IS DATA A HISTORICAL PERSPECTIVE ON MACLISP Jon L White Laboratory for Computer Science, M.I.T.* ABSTRACT For over 10 years, MACLISP has supported a variety of projects at M.I.T.'s Artificial Intelligence Laboratory, and the Laboratory for Computer Science (formerly Project MAC). During this time, there has been a continuing development of the MACLISP system, spurred in great measure by the needs of MACSYMAdevelopment. Herein are reported, in amosiac, historical style, the major features of the system. For each feature discussed, an attempt will be made to mention the year of initial development, andthe names of persons or projectsprimarily responsible for requiring, needing, or suggestingsuch features. INTRODUCTION In 1964,Greenblatt and others participated in thecheck-out phase of DigitalEquipment Corporation's new computer, the PDP-6. This machine had a number of innovative features that were thought to be ideal for the development of a list processing system, and thus it was very appropriate that thefirst working program actually run on thePDP-6 was anancestor of thecurrent MACLISP. This earlyLISP was patterned after the existing PDP-1 LISP (see reference l), and was produced by using the text editor and a mini-assembler on the PDP-1. That first PDP-6 finally found its way into M.I.T.'s ProjectMAC for use by theArtificial lntelligence group (the A.1. grouplater became the M.I.T. Artificial Intelligence Laboratory, and Project MAC became the Laboratory for Computer Science). By 1968, the PDP-6 wasrunning the Incompatible Time-sharing system, and was soon supplanted by the PDP-IO.Today, the KL-I 0, anadvanced version of thePDP-10, supports a variety of time sharing systems, most of which are capable of running a MACLISP. -
Published with the Approbation of the Board of Trustees
JOHNS HOPKINS UNIVERSITY CIRCULARS Published with the approbation of the Board of Trustees VOL. V.—No. 46.] BALTIMORE, JANUARY, 1886. [PRICE, 10 CENTS. RECENT PUBLICATIONS. Photograph of the Normal Solar Spectrum. Made Reproduction in Phototype of a Syriac Manuscript by PROFESSOR H. A. ROWLAND. (Baltimore, Johns Hopkins University, 1886). (Williams MS.) with the Antilegomena Epistles. Edited by IsAAC H. HALL. (Baltimore, Johns Hopkins University, 1886). [Froma letter to Science, New York, December 18, 1885]. The photographic map of the spectrum, upon which Professor Rowland [Froman article by I. H. Hall in the Journal of the Society of Biblical Literature and has expended so much hard work during the past three years, is nearly Exegesis for 1885, with additions]. ready for publication. The map is issued in a series of seven plates, cover- In September last (1884) I announced in The Independent the discovery ing the region from wave-length 3100 to 5790. Each plate is three feet of a manuscript of the Acts and Epistles, among which occur also the long and one foot wide, and contains two strips of the spectrum, except Epistles that were antilegomena among the Syrians; namely the Second plate No. 2, which contains three. Most of the plates are on a scalethree Epistle of Peter, the Second and Third Epistles of John, and the Epistle of times that of Angstrdm’s map, and in definition are more than equal to any Jude, in the version usually printed with our Peshitto New Testaments. It map yet published, at least to wave-length 5325. The 1474 line is widely is well known that the printed copies of these Epistles in that version all double, as also are b rest upon one manuscriptonly, in the Bodleian Library at Oxford, England, 3 and b4, while E may be recognized as double by the from which they were first published by Edward Pococke (Leyden, Elzevirs) expert. -
Ccured Type-Safe Retrofitting of Legacy Code
CCured Type-Safe Retrofitting of Legacy Code George C. Necula Scott McPeak Westley Weimer University of California, Berkeley {necula, smcpeak, weimer}@cs, berkeley, edu Abstract While in lhe 1970s sacrificing lype safely for flexibilily and perfbrmance mighl have been a sensible language design In lhis paper we propose a scheme lhai combines type in- choice, today lhere are more and more siiuaiions in which ference and run-time checking to make exisiing C programs type safety is jusi as imporiani as, if noi more importani type saI~. }V~ describe lhe CCured type sysiem, which ex- lhan, performance. Errors like array oui-of:bounds accesses lends lhat of C by separaiing poinier types according to lead boih lo painful debugging sessions chasing inadverieni lheir usage. This type sysiem allows boih poiniers whose memory updaies and lo malicious atiacks exploiiing buft>r usage can be verified siaiically to be type saI>, and poiniers overrun errors in security-criiical code. (Almost g0% of re- whose sai>ty musi be checked ai run lime. }V~ prove a type ceni CERT advisories resuli fi'om security violaiions of this soundness resuli and lhen we preseni a surprisingly simple kind [29].) Type safety is desirable for isolaiing program type inference algoriihm lhai is able lo infer lhe appropriaie componenis in a large or exiensible sysiem, wiihoui lhe loss poinier kinds for exisiing C programs. of performance of separaie address spaces. II is also valu- Our experience wiih lhe CCured sysiem shows lhai lhe able for inter-operaiion wiih sysiems wriiien in type-saf> inference is very eft~ciive for many C programs, as ii is able languages (such as type-saf> Java naiive meihods, for exam- io infer ihai most or all of the pointers are statically ver- pie). -
The Embeddable Common Lisp
ACM Lisp Pointers 8(1), 1995, 30-41 The Embeddable Common Lisp Giuseppe Attardi Dipartimento di Informatica, Universit`adi Pisa Corso Italia 40, I-56125 Pisa, Italy net: [email protected] Abstract The Embeddable Common Lisp is an implementation of Common Lisp designed for being embeddable within C based applications. ECL uses standard C calling conventions for Lisp compiled functions, which allows C programs to easily call Lisp functions and viceversa. No foreign function interface is required: data can be exchanged between C and Lisp with no need for conversion. ECL is based on a Common Runtime Support (CRS) which provides basic facilities for memory management, dynamic loading and dumping of binary images, support for multiple threads of execution. The CRS is built into a library that can be linked with the code of the application. ECL is modular: main modules are the program development tools (top level, debugger, trace, stepper), the compiler, and CLOS. A native implementation of CLOS is available in ECL: one can configure ECL with or without CLOS. A runtime version of ECL can be built with just the modules which are required by the application. 1 Introduction As applications become more elaborate, the facilities required to build them grow in number and sophistica- tion. Each facility is accessed through a specific package, quite complex itself, like in the cases of: modeling, simulation, graphics, hypertext facilities, data base management, numerical analysis, deductive capabilities, concurrent programming, heuristic search, symbolic manipulation, language analysis, special device control. Reusability is quite a significant issue: once a package has been developed, tested and debugged, it is un- desirable having to rewrite it in a different language just because the application is based in such other language.