Hierarchical Histogram-Based Median Filter for Gpus
Total Page:16
File Type:pdf, Size:1020Kb
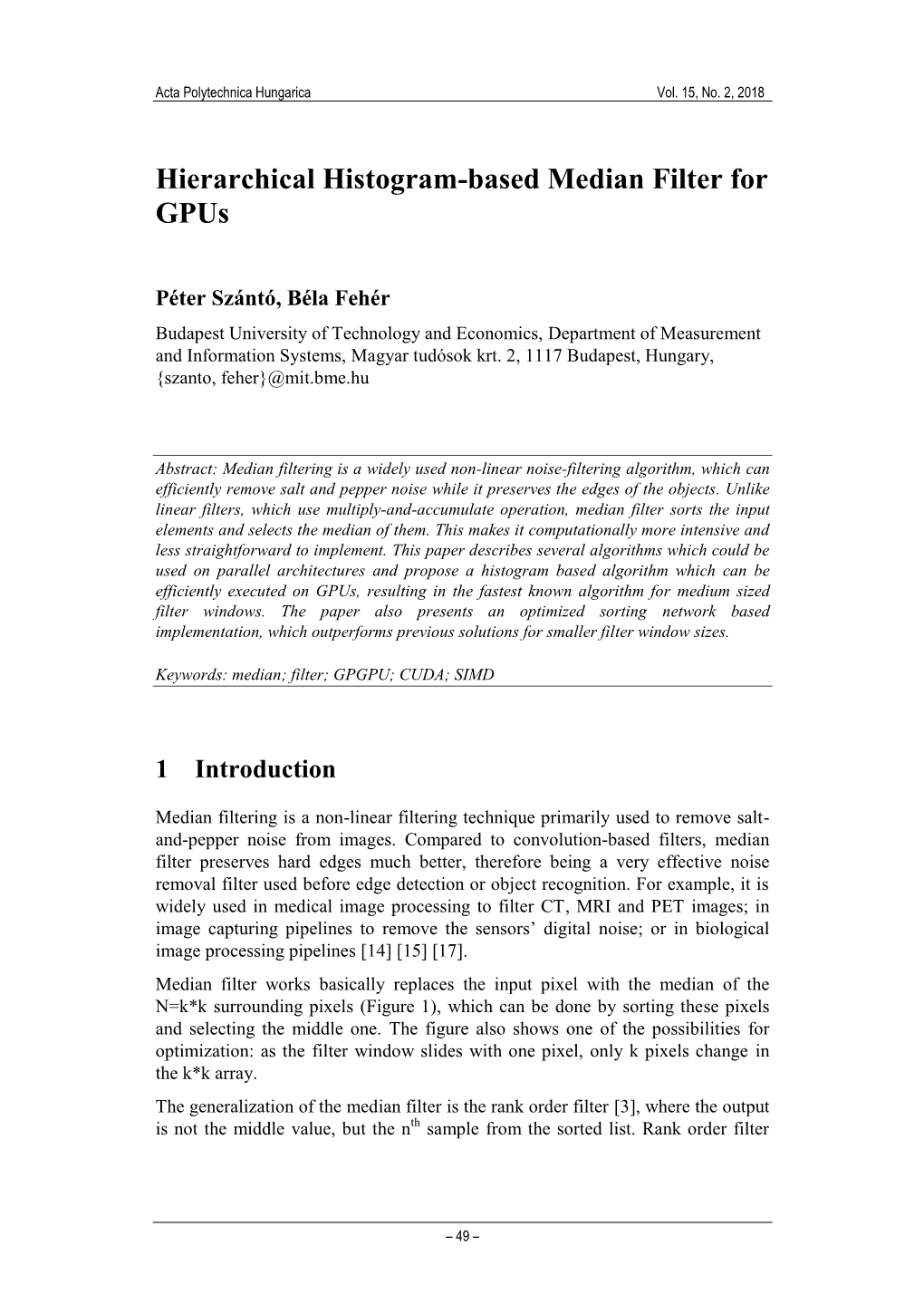
Load more
Recommended publications
-
An Evolutionary Approach for Sorting Algorithms
ORIENTAL JOURNAL OF ISSN: 0974-6471 COMPUTER SCIENCE & TECHNOLOGY December 2014, An International Open Free Access, Peer Reviewed Research Journal Vol. 7, No. (3): Published By: Oriental Scientific Publishing Co., India. Pgs. 369-376 www.computerscijournal.org Root to Fruit (2): An Evolutionary Approach for Sorting Algorithms PRAMOD KADAM AND Sachin KADAM BVDU, IMED, Pune, India. (Received: November 10, 2014; Accepted: December 20, 2014) ABstract This paper continues the earlier thought of evolutionary study of sorting problem and sorting algorithms (Root to Fruit (1): An Evolutionary Study of Sorting Problem) [1]and concluded with the chronological list of early pioneers of sorting problem or algorithms. Latter in the study graphical method has been used to present an evolution of sorting problem and sorting algorithm on the time line. Key words: Evolutionary study of sorting, History of sorting Early Sorting algorithms, list of inventors for sorting. IntroDUCTION name and their contribution may skipped from the study. Therefore readers have all the rights to In spite of plentiful literature and research extent this study with the valid proofs. Ultimately in sorting algorithmic domain there is mess our objective behind this research is very much found in documentation as far as credential clear, that to provide strength to the evolutionary concern2. Perhaps this problem found due to lack study of sorting algorithms and shift towards a good of coordination and unavailability of common knowledge base to preserve work of our forebear platform or knowledge base in the same domain. for upcoming generation. Otherwise coming Evolutionary study of sorting algorithm or sorting generation could receive hardly information about problem is foundation of futuristic knowledge sorting problems and syllabi may restrict with some base for sorting problem domain1. -
Bitonic Sorting Algorithm: a Review
International Journal of Computer Applications (0975 – 8887) Volume 113 – No. 13, March 2015 Bitonic Sorting Algorithm: A Review Megha Jain Sanjay Kumar V.K Patle S.O.S In CS & IT, S.O.S In CS & IT, S.O.S In CS & IT, PT. Ravi Shankar Shukla PT. Ravi Shankar Shukla PT. Ravi Shankar Shukla University, Raipur (C.G), India University, Raipur (C.G), India University, Raipur (C.G), India ABSTRACT 2.1 Bitonic Sequence The Batcher`s bitonic sorting algorithm is a parallel sorting A bitonic sequence of n elements ranges from x0,……,xn-1 algorithm, which is used for sorting the numbers in modern with characteristics (a) Existence of the index i, where 0 ≤ i ≤ parallel machines. There are various parallel sorting n-1, such that there exist monotonically increasing sequence algorithms such as radix sort, bitonic sort, etc. It is one of the from x0 to xi, and monotonically decreasing sequence from xi efficient parallel sorting algorithm because of load balancing to xn-1.(b) Existence of the cyclic shift of indices by which property. It is widely used in various scientific and characterics satisfy [10]. After applying the above engineering applications. However, Various researches have characteristics bitonic sequence occur. The bitonic split worked on a bitonic sorting algorithm in order to improve up operation is applied for producing two bitonic sequences on the performance of original batcher`s bitonic sorting which merge and sort operation is applied as shown in Fig 1. algorithm. In this paper, tried to review the contribution made by these researchers. 3. -
Finalabc.Pdf
A. Department goals and objectives are linked to the university and college mission and strategic priorities, and to their strategy for improving their position within the discipline. (1 page maximum) 1. What is the department’s mission and is it clearly aligned with the university and college mission and direction? The department strives to offer quality undergraduate and graduate courses / programs; places a high priority on quality research and effective teaching; seeks to attract highly- qualified students; and strives to attract and nurture high-caliber faculty. This mission is consistent with university goals of ensuring student success, enhancing academic excellence, expanding breakthrough research, and developing / recognizing our people. 2. How does the department’s mission relate to curriculum; enrollments; faculty teaching; research/professional/creative activity and outreach? Is it aligned with the college’s strategic priorities? The departmental mission aligns with university and college strategic priorities, although the department is now in financial jeopardy under KSU’s new RCM budget model due to developmental growth in faculty numbers under state direction to grow a doctoral program. 3. How does the department contribute to university-wide curricular needs through general education and service instruction? The department was limited to a single Liberal Education Course (CS 10051 Introduction to Computer Science), but may have an opportunity to offer more courses under the new Kent Core curriculum. However, unlike many universities, KSU does not have a university-wide computing requirement, and does not have a large number of engineering majors taking CS courses. 4. How does the department promote diversity? The department has an active NSF S-STEM grant for broadening participation, and a diverse set of faculty, two of whom are women, and outreach activities to women and other minority students in computing. -
Evaluation of Sorting Algorithms, Mathematical and Empirical Analysis of Sorting Algorithms
International Journal of Scientific & Engineering Research Volume 8, Issue 5, May-2017 86 ISSN 2229-5518 Evaluation of Sorting Algorithms, Mathematical and Empirical Analysis of sorting Algorithms Sapram Choudaiah P Chandu Chowdary M Kavitha ABSTRACT:Sorting is an important data structure in many real life applications. A number of sorting algorithms are in existence till date. This paper continues the earlier thought of evolutionary study of sorting problem and sorting algorithms concluded with the chronological list of early pioneers of sorting problem or algorithms. Latter in the study graphical method has been used to present an evolution of sorting problem and sorting algorithm on the time line. An extensive analysis has been done compared with the traditional mathematical methods of ―Bubble Sort, Selection Sort, Insertion Sort, Merge Sort, Quick Sort. Observations have been obtained on comparing with the existing approaches of All Sorts. An “Empirical Analysis” consists of rigorous complexity analysis by various sorting algorithms, in which comparison and real swapping of all the variables are calculatedAll algorithms were tested on random data of various ranges from small to large. It is an attempt to compare the performance of various sorting algorithm, with the aim of comparing their speed when sorting an integer inputs.The empirical data obtained by using the program reveals that Quick sort algorithm is fastest and Bubble sort is slowest. Keywords: Bubble Sort, Insertion sort, Quick Sort, Merge Sort, Selection Sort, Heap Sort,CPU Time. Introduction In spite of plentiful literature and research in more dimension to student for thinking4. Whereas, sorting algorithmic domain there is mess found in this thinking become a mark of respect to all our documentation as far as credential concern2. -
36 Algorithms.Txt 4/4/2010 Zhan and Noon Tested Algorithms For
36_Algorithms.txt 4/4/2010 Zhan and Noon tested algorithms for solving this problem, finding approximate or double bucket modifications to one algorithm and Pallattino's algorithm with two queues were fastest on actual data. Johnson's algorithm solves this problem in the sparse case faster than the cubic Floyd's algorithm, both of which solve this for all pairs. Another approach uses dynamic programming, and checks all edges n times; that algorithm also detects negative cost cycles, and is named for Bellman and Ford. A Fibonacci heap is used to speed up a greedy approach, which works only for positive-weight edges, while if an admissible heuristic is available, the A* algorithm can be used. For 10 points, identify this problem in graph theory, most famously solved by Dijkstra's algorithm, which asks for a fast route between two nodes. Answer: shortest path algorithms [accept with any of the following modifying the answer: all-pairs, single-source, or single-source single-destination] Along with a binary search tree, one of these structures is used to store future beach-line-changing events in Fortune's algorithm for generating Voronoi diagrams. The ROAM algorithm uses a pair of these data structures to store triangles that can be either split or merged. A meldable heap is a version of this structure implemented using a binomial heap, which gives it a "merge" operation, and the “fringe” structure in the A* algorithm is one of these. They're used on network routing protocols to ensure real-time traffic is forwarded first, and they optionally implement the PeekAtNext operation. -
LEARNING NEURAL NETWORKS THAT CAN SORT a Thesis
LEARNING NEURAL NETWORKS THAT CAN SORT A Thesis Presented to The Academic Faculty By Arnab Dey In Partial Fulfillment of the Requirements for the Degree Bachelor of Science in the College of Computing Georgia Institute of Technology Dec 2020 © Arnab Dey 2020 LEARNING NEURAL NETWORKS THAT CAN SORT Thesis committee: Dr. Richard Vuduc Dr. Jeffrey Young College of Computing College of Computing Georgia Institute of Technology Georgia Institute of Technology ACKNOWLEDGMENTS I would like to express my appreciation to Professor Vuduc for introducing me to this research project. I have learned a lot from all our stimulating discussions, and I am truly grateful for all of the guidance. I would like to especially acknowledge Srinivas Eswar for mentoring me. Without all of his insightful advice and constant encouragement throughout the past couple of semesters, I could not have completed this work. I would also like to thank Dr. Jeffrey Young for providing valuable insight while I was working on this thesis. Lastly, I would like to convey my gratitude to my friends and family for the support and encouragement that I have received from the very beginning. iii TABLE OF CONTENTS Acknowledgments................................... iii List of Tables...................................... vii List of Figures..................................... viii Chapter 1: Introduction and Background...................... 1 1.1 Parallel Sorting ................................ 1 1.2 Neural Networks ............................... 3 1.3 Reinforcement Learning ........................... 4 1.4 Goal...................................... 6 Chapter 2: Literature Review............................ 7 2.1 Sorting Networks............................... 7 2.2 Neural Networks ............................... 8 2.3 Sorting using Neural Networks........................ 9 2.4 Reinforcement Learning ........................... 10 2.5 Sorting using Reinforcement Learning.................... 11 Chapter 3: Neural Networks............................ -
Estimation of Execution Time and Speedup for Bitonic Sorting in Sequential and Parallel Enviroment
International Journal of Computer Applications (0975 – 8887) Volume 122 – No.19, July 2015 Estimation of Execution Time and Speedup for Bitonic Sorting in Sequential and Parallel Enviroment Megha Jain Sanjay Kumar V.K Patle S.o.S In CS & IT, S.o.S In CS & IT, S.o.S In CS & IT, Pt. Ravi Shankar Shukla Pt. Ravi Shankar Shukla Pt. Ravi Shankar Shukla University, Raipur (C.G), India University, Raipur (C.G), India University, Raipur (C.G), India ABSTRACT 1.1 Parallel Algorithm The Batcher`s bitonic sorting algorithm is one of the best If there are N concurrent processes, if N=1 then the sequential parallel sorting algorithms, for sorting random numbers in algorithm runs on a uniprocessor machine, and if N>1 then modern parallel machines. Load balancing property of bitonic parallel algorithm runs on parallel machines. Parallel sorting algorithm makes it unique among other parallel algorithm is opposed of sequential algorithm. However, sorting algorithms. Contribution of bitonic sorting algorithm parallel algorithm can be executed on different processors by can be seen in various scientific and engineering applications. using parallel algorithmic techniques. Techniques such as Research on a bitonic sorting algorithm has been reported by divide and conquer, randomization, parallel pointer various researchers in order to improve up the performance of techniques etc. initial batcher`s bitonic sorting algorithm. In this paper, time estimation of bitonic sort algorithm is done in both sequential 1.2 Parallel Sorting Algorithm as well as in parallel domain. Parallel Sorting Algorithms are used in parallel computers for sorting the numbers. Sorting in parallel machine is achieved Keywords by dividing and computing data separately among processors. -
Hierarchical Histogram-Based Median Filter for Gpus
Acta Polytechnica Hungarica Vol. 15, No. 2, 2018 Hierarchical Histogram-based Median Filter for GPUs Péter Szántó, Béla Fehér Budapest University of Technology and Economics, Department of Measurement and Information Systems, Magyar tudósok krt. 2, 1117 Budapest, Hungary, {szanto, feher}@mit.bme.hu Abstract: Median filtering is a widely used non-linear noise-filtering algorithm, which can efficiently remove salt and pepper noise while it preserves the edges of the objects. Unlike linear filters, which use multiply-and-accumulate operation, median filter sorts the input elements and selects the median of them. This makes it computationally more intensive and less straightforward to implement. This paper describes several algorithms which could be used on parallel architectures and propose a histogram based algorithm which can be efficiently executed on GPUs, resulting in the fastest known algorithm for medium sized filter windows. The paper also presents an optimized sorting network based implementation, which outperforms previous solutions for smaller filter window sizes. Keywords: median; filter; GPGPU; CUDA; SIMD 1 Introduction Median filtering is a non-linear filtering technique primarily used to remove salt- and-pepper noise from images. Compared to convolution-based filters, median filter preserves hard edges much better, therefore being a very effective noise removal filter used before edge detection or object recognition. For example, it is widely used in medical image processing to filter CT, MRI and PET images; in image capturing pipelines to remove the sensors’ digital noise; or in biological image processing pipelines [14] [15] [17]. Median filter works basically replaces the input pixel with the median of the N=k*k surrounding pixels (Figure 1), which can be done by sorting these pixels and selecting the middle one. -
On the Practicality of Data-Oblivious Sorting Kris Vestergaard Ebbesen, 20094539
On the Practicality of Data-Oblivious Sorting Kris Vestergaard Ebbesen, 20094539 Master’s Thesis, Computer Science April 2015 Advisor: Gerth Stølting Brodal ii Abstract In this thesis various data-oblivious sorting algorithms are discussed with a focus on practical and concise descriptions promoting ease of implementation. Furthermore, general performance concerns of data-oblivious sorting algo- rithms are explored, along with demonstrations of both SSE and CUDA imple- mentation variants of major data-oblivious sorting algorithms. This is further expanded by presenting a technique for automatic vectorization of general sort- ing networks. Finally, several data-oblivious sorting algorithms are compared experimen- tally based on several parameters, many of which have a direct and measurable effect on real-life performance of the aforementioned algorithms. Along with these findings, we also present actual performance measurements for optimiza- tion techniques applied to the data-oblivious sorting algorithms, including both SSE, CUDA and OpenMP, alongside algorithm-specific optimizations. iii iv Resumé I dette speciale diskuteres forskellige data-oblivious sorteringsalgoritmer, med fokus på korte, praktiske beskrivelser for at fremme letheden ved deres imple- mentation. Derudover udforskes generelle performance-problemer for data-oblivious sor- teringsalgoritmer sammen med præsentationer af SSE og CUDA implementa- tioner af vigtige data-oblivious sorteringsalgoritmer. Dette udvides yderligere med præsentationen af en teknik til automatisk vektorisering af generelle sor- teringsnetværk. Endeligt sammenlignes flere data-oblivious sorteringsalgoritmer baseret på varierende parametre, hvoraf mange har en direkte og målbar effekt på den prak- tiske udførselstid. Sammen med disse resultater præsenteres også målinger for forskellige optimeringsteknikker for data-oblivious sorteringsalgoritmer inklusiv SSE, CUDA og OpenMP, sammen med algoritmespecifikke optimeringer. -
Priority-Based Task Management in a GPGPU Megakernel
Priority-Based Task Management in a GPGPU Megakernel Bernhard Kerbl∗ Supervised by: Markus Steinberger† Institute for Computer Graphics and Vision Graz University of Technology Graz / Austria Abstract architecture and associated compilers for industry stan- dard programming languages. With respect to certain re- In this paper, we examine the challenges of implement- strictions, the architecture enables programmers to run ing priority-based task management with respect to user- general purpose computations on compatible devices in defined preferential attributes on a graphics processing parallel. Newer models of GPUs supporting these features unit (GPU). Previous approaches usually rely on constant are therefore often referred to as general purpose graph- synchronization with the CPU to determine the proper ics processing units (GPGPU). Several hundred Stream chronological sequence for execution. We transfer the re- Processors (SP), also referred to as thread blocks, which sponsibility for evaluating and arranging planned tasks to are grouped in clusters called Streaming Multiprocessors the GPU where they are continuously processed in a per- (SM) can be instructed to execute commands at the same sistent kernel. By implementing a dynamic work queue time – the programmer simply specifies the number of nec- with segments of variable size, we introduce possibili- essary threads when launching a GPGPU kernel. The com- ties for gradually improving the overall order and iden- bined capacities of these SPs have produced a significant tify necessary meta data required to avoid write-read con- gap in raw speed between high-end GPUs and CPUs [12]. flicts in a massively parallel environment. We employ an However, using the available resources to their full poten- autonomous controller module to allow queue manage- tial is not an easy task. -
Técnicas De Ordenação Para Simulação Física Baseada Em
TECNICAS´ DE ORDENAC¸ AO˜ PARA SIMULAC¸ AO˜ FISICA´ BASEADA EM PARTICULAS´ Rubens Carlos Silva Oliveira Dissertac¸ao˜ de Mestrado apresentada ao Programa de Pos-graduac¸´ ao˜ em Engenharia de Sistemas e Computac¸ao,˜ COPPE, da Universidade Federal do Rio de Janeiro, como parte dos requisitos necessarios´ a` obtenc¸ao˜ do t´ıtulo de Mestre em Engenharia de Sistemas e Computac¸ao.˜ Orientador: Claudio Esperanc¸a Rio de Janeiro Setembro de 2012 TECNICAS´ DE ORDENAC¸ AO˜ PARA SIMULAC¸ AO˜ FISICA´ BASEADA EM PARTICULAS´ Rubens Carlos Silva Oliveira DISSERTAC¸ AO˜ SUBMETIDA AO CORPO DOCENTE DO INSTITUTO ALBERTO LUIZ COIMBRA DE POS-GRADUAC¸´ AO˜ E PESQUISA DE ENGENHARIA (COPPE) DA UNIVERSIDADE FEDERAL DO RIO DE JANEIRO COMO PARTE DOS REQUISITOS NECESSARIOS´ PARA A OBTENC¸ AO˜ DO GRAU DE MESTRE EM CIENCIASˆ EM ENGENHARIA DE SISTEMAS E COMPUTAC¸ AO.˜ Examinada por: Prof. Claudio Esperanc¸a, Ph.D. Prof. Ricardo Guerra Marroquim, D.Sc. Prof. Waldemar Celes Filho, Ph.D. RIO DE JANEIRO, RJ – BRASIL SETEMBRO DE 2012 Oliveira, Rubens Carlos Silva Tecnicas´ de Ordenac¸ao˜ para Simulac¸ao˜ F´ısica Baseada em Part´ıculas/Rubens Carlos Silva Oliveira. – Rio de Janeiro: UFRJ/COPPE, 2012. XIII, 87 p.: il.; 29, 7cm. Orientador: Claudio Esperanc¸a Dissertac¸ao˜ (mestrado) – UFRJ/COPPE/Programa de Engenharia de Sistemas e Computac¸ao,˜ 2012. Referenciasˆ Bibliograficas:´ p. 49 – 54. 1. Tecnicas´ de Ordenac¸ao.˜ 2. Simulac¸ao˜ F´ısica. 3. Part´ıculas. I. Esperanc¸a, Claudio. II. Universidade Federal do Rio de Janeiro, COPPE, Programa de Engenharia de Sistemas e Computac¸ao.˜ III. T´ıtulo. iii A` vida iv Agradecimentos Agradec¸o aos meus professores, que sao˜ todos referenciasˆ em inteligencia,ˆ razoabilidade e benevolencia.ˆ Em particular, ao professor Claudio Esperanc¸a, que, brilhante como e,´ soube ser um excelente orientador percebendo minhas limitac¸oes˜ e qualidades, oti- mizando, assim, meu rendimento no desenvolvimento desse trabalho.