Lab2 - Arithmetic And Built-In Functions
Total Page:16
File Type:pdf, Size:1020Kb
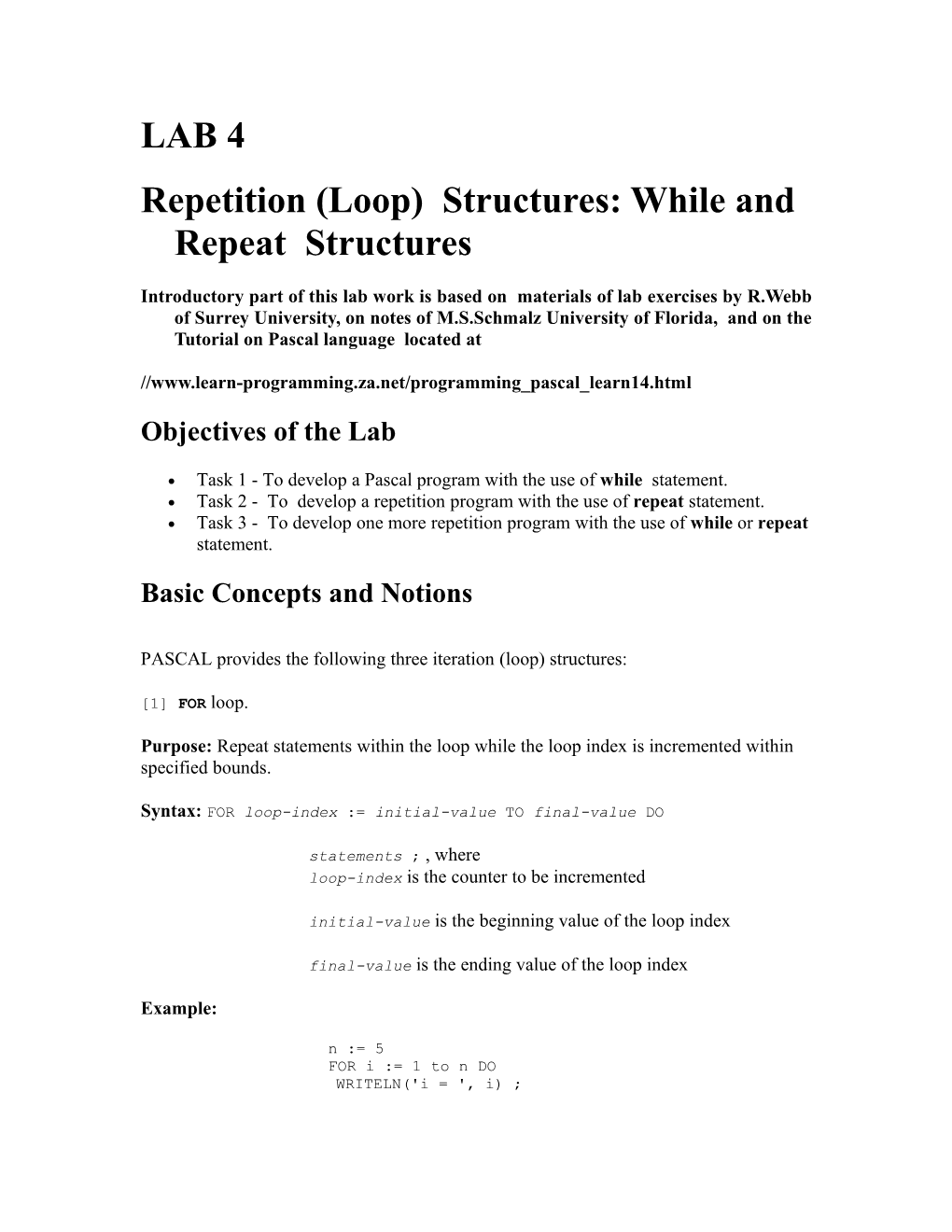
LAB 4 Repetition (Loop) Structures: While and Repeat Structures
Introductory part of this lab work is based on materials of lab exercises by R.Webb of Surrey University, on notes of M.S.Schmalz University of Florida, and on the Tutorial on Pascal language located at
//www.learn-programming.za.net/programming_pascal_learn14.html Objectives of the Lab
Task 1 - To develop a Pascal program with the use of while statement. Task 2 - To develop a repetition program with the use of repeat statement. Task 3 - To develop one more repetition program with the use of while or repeat statement. Basic Concepts and Notions
PASCAL provides the following three iteration (loop) structures:
[1] FOR loop.
Purpose: Repeat statements within the loop while the loop index is incremented within specified bounds.
Syntax: FOR loop-index := initial-value TO final-value DO
statements ; , where loop-index is the counter to be incremented
initial-value is the beginning value of the loop index
final-value is the ending value of the loop index
Example:
n := 5 FOR i := 1 to n DO WRITELN('i = ', i) ; In this, we have used a parameterized loop, whereby the variable n contains the loop index' final value.
[2] WHILE ... DO loop.
Purpose: The WHILE loop is also called a conditional loop, since it terminates based on a condition encoded in a logical predicate.
Syntax: WHILE predicate DO statements ; , where
predicate is the controlling condition.
Example:
x := 10; WHILE x > 5 DO BEGIN x := x – 2; WRITELN('x = ', x) END;
[3] REPEAT ... UNTIL loop.
Purpose: The REPEAT loop is another example of a conditional loop.
Syntax: REPEAT statements UNTIL predicate; , where
predicate is the controlling condition.
Example: (approximation of previous WHILE loop)
x := 10 REPEAT BEGIN x := x - 2 ; WRITELN('x = ', x); END; UNTIL x <= 5 ;
This lab work is focused on the use of while and repeat statements to implement loops. Thus, these two statements will be explained and illustrated with more details.
The while loop repeats while a condition is true. The condition is tested at the beginning of the loop. A while loop does not need a loop variable but if you want to use one then you must initialize its value before entering the loop. Example: program While_Loop; var i: Integer; begin i := 0; while i <= 10 begin i := i + 1; Writeln('Loop cycle', i:2); end; end.
The loop in this program is performed while condition I <= 10 is true. After the loop is finished, variable I has value 11 assigned in the last cycle.
The repeat loop statement is very similar to the while loop statement except the condition is tested at the end of the loop (post-test loop). If you want to execute multiple lines, then you don't need to put begin .. end keywords around the multiple lines. For instance, the following loop using while...
i := 1; while i <= 6 do begin writeln(i); i := i + 1; end; would be like this with a repeat loop...
i := 1; repeat writeln(i); i := i + 1 until i > 6;
Notice how the condition has changed slightly. For the while loop, it would do the loop while the condition is true, whereas the repeat loop does it until the condition remains not true. Break and Continue
The Break statement will exit a loop at any time. The following program will not print anything because it exits the loop before printing. program With_Break_of_Loop; var i: Integer; begin i := 0; repeat i := i + 1; Break; Writeln(i) until i = 10; end.
Note that, in this example, the loop will be performed (partially) only once!
The Continue statement will jump back to the beginning of a loop. The following program will also not print anything but unlike the Break example, it will count all the way to 10. That is, the loop is performed (partially) 10 times. program Loops; var i: Integer; begin i := 0; repeat i := i + 1; Continue; Writeln(i); until i = 10; end.
Task 1. Developing a Pascal program with the use of while statement.
Draw the flowchart, develop and run a program with a loop that uses the WHILE statement. The program should calculate the factorial of a positive integer N (that is, the value of N!). In doing this, the program should perform the following operations:
[1] Enter a positive integer and assign it to variable N.
[2] Check the value of N. It must be positive and not more than 15 to avoid integer overflow.
[3] Print value of N and its vactorial. Task 2. Developing a repetition program with the use of repeat statement.
Draw the flowchart, develop and run a program with a loop that uses the REPEAT statement. The program should calculate the factorial of a positive integer N (that is, the value of N!) and perform the same operations as the program in Task 1.
Task 3. Developing one more repetition program with the use of the WHILE or REPEAT statement.
Draw the flowchart, develop and run a program that uses the WHILE or REPEAT statement (on your choice). The program should output positive integer odd numbers that are divisible by M = 5 and not more than NMAX = 123. In doing this, the program should perform the following operations:
[1] Input values of M and N and check that they are positive.
[2] Perform a loop. In each cycle of the loop, the program should output an odd number divisible by 5 (in the given range).
Note. Use the function Odd() to determine whether the number is odd and the operator MOD to determine that the odd number is divisible by M.