Chapter 1: Is Management: Issues And Challenges
Total Page:16
File Type:pdf, Size:1020Kb
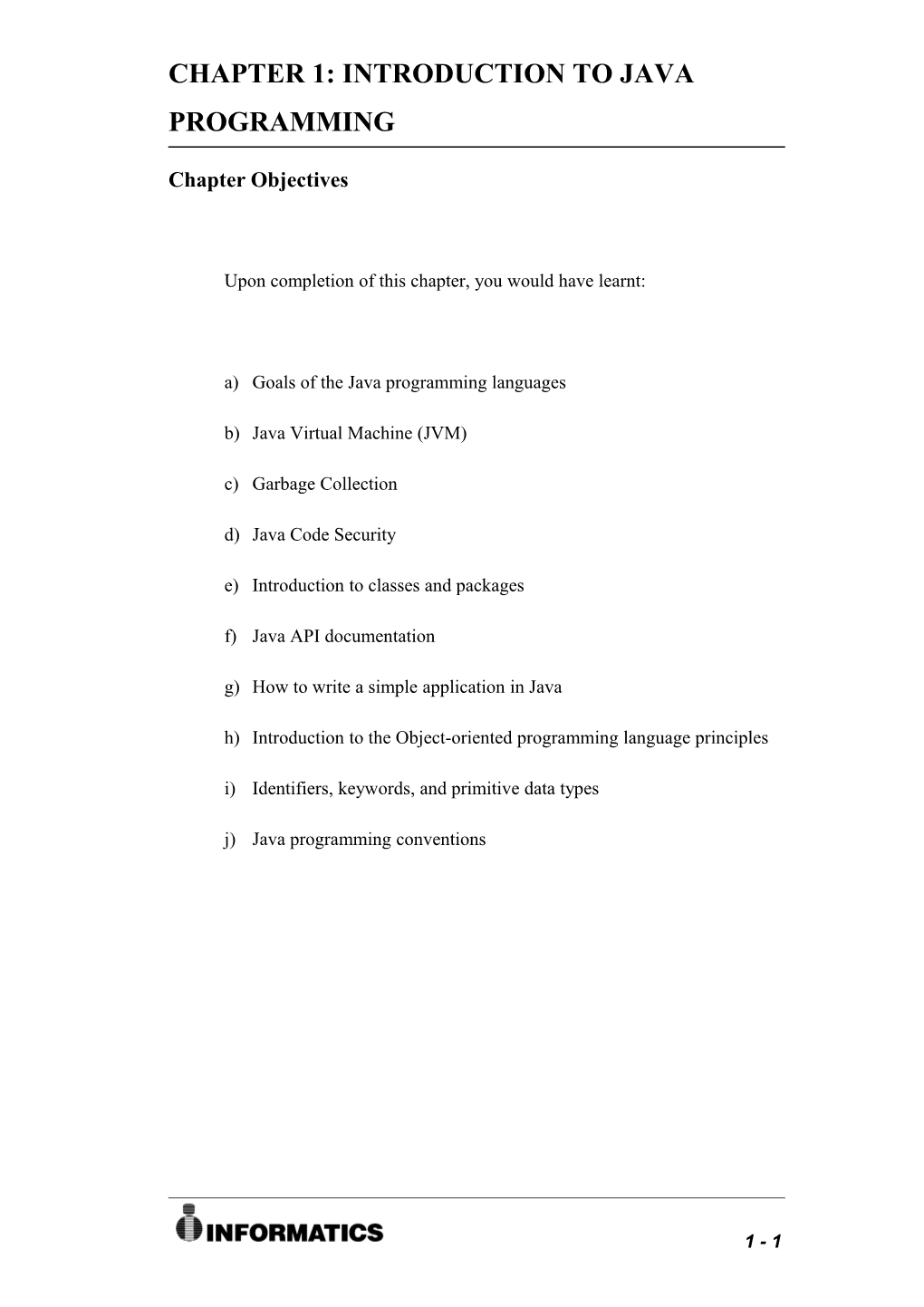
CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
Chapter Objectives
Upon completion of this chapter, you would have learnt:
a) Goals of the Java programming languages
b) Java Virtual Machine (JVM)
c) Garbage Collection
d) Java Code Security
e) Introduction to classes and packages
f) Java API documentation
g) How to write a simple application in Java
h) Introduction to the Object-oriented programming language principles
i) Identifiers, keywords, and primitive data types
j) Java programming conventions
1 - 1 CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
1.0 Introduction to Java Programming Language
Java is a general purpose object-oriented programming language developed by SUN Microsystems.
Java is a programming language, a development, an application and a deployment environment.
used for developing both applets and application
Applet - program written in Java that resides on WWW servers, is downloaded by a browser to a client’s system, and run by that browser - usually small in size to minimize download time and is invoked by a HTML web page
Application - stand alone program that does not require any Web browser to execute.
1.1 Why Java is popular?
Simple Object-oriented Robust Secure Distributed Architectural Neutral Portable Fast Multithreaded
1 - 2 CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
2.0 Introduction to Classes and Package
The .java source file can contain 3 ‘top-level’ elements :
- a package declaration - any number of import statements - class and interface definition
These items must appear in this order
A class is a module that provides functionality (JDK comes with standard class library ) A class is a template from which objects with similar characteristics can be created A class instance is an actual object A package is a collection of related classes and interface
3.0 Introduction to Object-oriented programming language principles
ENCAPSULATION
The mechanism that binds together code and data it manipulates, and keeps both safe from outside interference or misuse
In Java, the basis of encapsulation is the class
INHERITANCE
The process by which one object acquires the properties of another object. A subclass inherits all the variables and methods of a superclass. POLYMORPHISMS
A feature that allows one interface to be used for a general class of actions, i.e, the ability of an object to take many forms or identities. (“one interface, multiple methods” ).
1 - 3 CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
4.0 A Basic Java Application
Program filename : HelloCS214.java
// Sample application class HelloCS214 { public static void main ( String args[] ) { System.out.println (“Hello CS214 !”); } }
Description :
HelloCS214.java : This is the name of the source file.
//Sample application : This is a comment. The contents of a comment are ignored by the compiler. class HelloCS214 : The keyword class declares that a new class is being defined and HelloCS214 is the name of the class.
{ } : The entire class definition, including all its members, will be between { and }. public : This keyword is an access specifier, which allows the main() method to be accessed by code outside this class. static : This keyword allows main() to be called without having to create a particular instance of the class. This is necessary since main() is called by the Java interpreter before any objects are made. void : This keyword tells the compiler that the main() does not return a value. main() : This is a method called when a Java application begins. The second { and } indicates the main()’s block.
1 - 4 CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
Take note : main() method is simply a starting place for the interpreter. A program may have one or more classes, but only one of which will need to have a main() to get things started. For Java applets, you won’t use main() at all because the Web browser uses a different means of starting the execution of applets.
String args[ ] : This declares a parameter named args, which is an array of instances of the class String. args receives any command-line arguments present when the program is executed.
System.out.println(“Hello CS214 !”) : This statement will display the string “Hello CS214 !” followed by a new line on the screen. The println() displays the string which is passed to it. System is the predefined class that provides access to the system, and out is the output stream that is connected to the console.
5.0 Identifiers and Keywords
Identifiers are names given to a variable, class or method
You can use letters, digits, dollar signs, and underscores in an identifier, but the first character MUST be a letter, an underscore( _ ), or a dollar sign ($)
Java identifiers are case sensitive : Java treats upper- and lower-case letters as different characters, so, for example, Hello is not the same identifier as HELLO or hello.
You cannot use a space or other symbols in an identifier
Java identifiers have no maximum length. You can use any number of characters to make up an identifier name.
1 - 5 CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
Example of identifiers :
Unacceptable to the compiler Acceptable to the Compiler Poor style Good style student ID s1 studentID student-Name sn student_Name **LATEFEE** LATEFEE LATE_FEE class Class JavaClass 20Students S20 get20Students
Java keywords : there are 48 reserved keywords defined in the Java language. These keywords cannot be used as names for a variable, class, or method.
abstract do implements private throw boolean double import protected throws break else instanceof public transient byte extends int return true case false interface short try catch final long static void char finally native super volatile class float new switch while continue for null synchronized default if package this
1 - 6 CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
10.0 Primitive Data Types
The Java programming language defines 8 primitive types :
Logical : boolean Textual : char Integral : byte, short, int, long Floating : double, float
Logical : boolean
The boolean data type has 2 literals : true and false For example : boolean truth = true;
- this statement declares the variable truth as boolean type and assigns it a value of true
Textual : char
Represents a 16-bit Unicode character Must have its literal enclosed in single quotes For example :
‘a’ ‘\t’ tab ‘\u????’ a specific Unicode character is replaced with exactly 4 hexadecimal digits
String is not a primitive data type, it is a class. String has its literal enclosed in double quotes. For example, String greeting = “Happy Birthday!”
1 - 7 CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
Example :
class CharSample { public static void main ( String args [ ] ) { char c; c = ‘X’; System.out.println(“The value of c is ” + c ); c--; System.out.println(“Now the value of c is ” + c ); } } // end of class CharSample
Identify the output.
Integral
Each of the integral data types have the following range
Size Type Range 8 bits byte -27 .. 27 - 1 ( -128 to 127) 16 bits short -215 .. 215 - 1 ( -32,768 to 32,767 ) 32 bits int -231 .. 231 - 1 ( -2,147,483,648 to 2,147,483,647 ) 64 bits long -263 .. 263 - 1 ( -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 )
has a default int. An integer is whole number, such as 5, 11, or –22. class IntegerSample { defines long, by using the letter ‘L’ or ‘l’ public static void main ( String args [ ] ) { int a; long b; a = 200000; b = 1000 * 25 * 60 * 60; Example : System.out.println(“ a = ” + a + “ b = ” + b ); } 1 - 8} // end of class IntegerSample Identify the output. CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
Floating
Floating point literal includes either a decimal point . Default is double. Floating point data types have the following ranges :
Size Type Range 32 bits float 1.40129846432481707e-45 to 3.40282346638528860e+30 64 bits double 4.94065645841246544e-324 to 1.79769313486231570e+308
For example :
class FloatingSample { public static void main ( String args [ ] ) { double d, dd; d = 1.2; dd = 3.45; System.out.print( “The output of ” + d + “ * ” + dd ); System.out.println( “ is” + (d * dd) ); } } // end of class FloatingSample Identify the output.
11.0 Java Programming Conventions
1 - 9 CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
Three permissible styles of comment in a Java Technology program are
1. // comment on one line
2. /* comment on one or more lines */
3. /** documenting comment */
A statement is a single line of code terminated by a semicolon(;) eg. total = a + b + c ;
A block is a collection of statements bounded by opening and closing braces eg. { x = x + 1; y = y + 2; } A block can be used in a class definition public class Date { int day; int month; int year; } Block statements can be nested
Any amount of whitespace is allowed in a Java program
Classes - name should be nouns, in mixed case, with the first letter of each word capitalized. - eg. class AccountBook Interfaces - names should be capitalized.
1 - 10 CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
- eg. interface Account
Methods - names should be verbs, in mixed case, with the first letter in lowercase - eg. addAccount( )
Variables - should be in mixed case with a lowercase first letter
Constants - should be all uppercase with the words separated by underscore
Control structures - use braces ({ } ) around all statements, even single statement, when they are part of a control statement
12.0 Programming Exercises
1 - 11 CS214 CHAPTER 1: INTRODUCTION TO JAVA PROGRAMMING
1. Write a simple program, called MyParticulars, to display the following details :
Class : CS214 Lecturer : < put your lecturer’s name here > ------Name : < put your name here > Age : < put your age here > Height : < put your height here >
2. Examine the following code. Correct errors, if any, and identify its output.
Class TraceOutput { public static void main ( String argument[] ){ System.out.println( “I’m tracing.. ); System.out.println( 20 + 5 ) System.out.print( “ should be ” + ( 20 + 5 ) ); } } / end of class TraceOutput
.
1 - 12