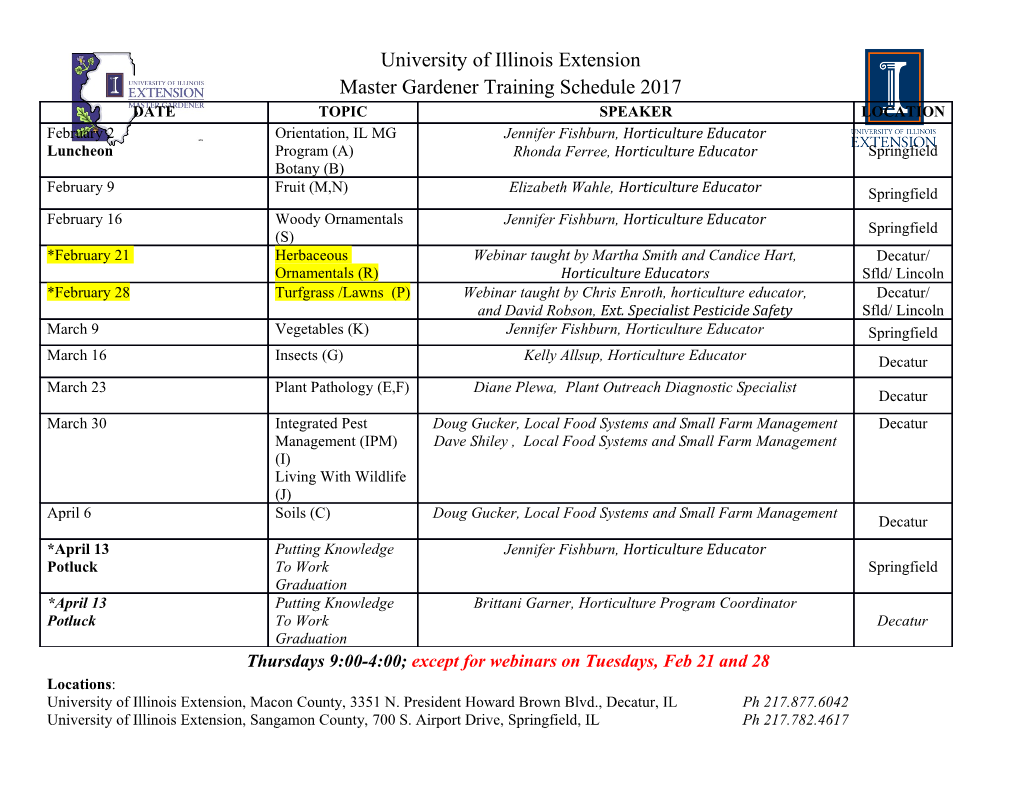
1. Introduction Overview The Eye4Software GPS Toolkit, is a software component that helps you developing your own GPS applications, including Track and Trace, Navigation, Positioning, Hydrographic Survey, construction and much more. The core of the toolkit is a single "GpsCtl32.dll" (32bit) or "GpsCtl64.dll" (64bit) file. The toolkit offers the following features: Supports up to 256 serial ports, including RS232, Bluetooth and USB serial ports; Supports Garmin GPS devices connected by USB (Garmin PVT protocol); Decodes the most common used GPS NMEA0183 sentences like: GGA, GLL, GSA, GSV, RMC, RME, RMZ, VTG and ZDA; Outputs latitude and longitude as both decimal and (user defined) string representations; Conversion between units, like km/h, mph, feet, knots, meters; Conversion between geodetic map datums and reference ellipsoids; Conversion between latitude / longitude and map projections; Convert coordinates between NAD83 and NAD27 using NADCON correction files; Convert coordinates between NAD83 and NAD83(HARN) using HARN/HPGN correction files; Convert coordinates between different map datums using NTv2 correction files; Distance and Azimuth calculation using Vincenty's formula; Built-in support for more than 350 map datums and 3500 map grids. When the toolkit is used to convert coordinates, the following map projections are supported: Lambert Conformal Conic (LCC) 1SP projection; Lambert Conformal Conic (LCC) 1SP projection (West Orientated); Lambert Conformal Conic (LCC) 2SP projection; Lambert Conformal Conic (LCC) 2SP projection (Belgium); Lambert Equal Area Conic projection; Transverse Mercator projection; Transverse Mercator projection (South Orientated); Oblique Mercator projection; Hotine Oblique Mercator projection; Swiss Oblique Mercator projection; Oblique Stereographic projection; Polar Stereographic projection; Albers Equal Area Conic projection; Cassini Soldner projection; Mercator projection 1SP; Mercator projection 2SP; Polyconic projection; Gauss Kruger projection; Universal Transverse Mercator (UTM) projection; Mollweide projection; Eckert IV projection; Eckert VI projection; Krovak projection; Cylindrical Equal Area Projection; Sinusoidal Projection; Miller Projection; Bonne Projection; Gall stereographic projection; Robinson projection; Equirectangular projection; Loximuthal projection; Plate Carree projection; Van der Grinten I projection; Winkel I projection Ortographic projection; Gnomonic projection; Hammer-Aitoff projection; Quartic authalic projection; Azimuthal Equidistant projection. Supported programming languages The toolkit can be used with every programming language which supports the use of ActiveX / COM objects. Some development environments that include ActiveX are: Microsoft Visual C++; Microsoft Visual Basic; Microsoft Visual C# .Net; Microsoft Visual Basic .Net; Microsoft VBScript; Microsoft Visual Basic for Applications (MS Excel, MS Access, MS Word); Microsoft ASP; Microsoft ASP .NET; Borland C++ Builder; Borland Delphi; PHP; Coldfusion; Powerbuilder. Requirements The toolkit can be used to write applications or scripts for the following platforms: Server Platforms: Windows 2000 Server; Windows 2003 Server; Windows 2008 Server; Windows 2003 R2 Server; Windows 2008 R2 Server. Desktop Platforms: Windows 2000 Professional; Windows XP; Windows Vista; Windows 7; Windows 8. Supported GPS hardware You can use all GPS devices equipped with a NMEA0183 port, or Garmin GPS devices equipped with an USB connector. If you do not have such a device, you also use a GPS or NMEA0183 simulator to get started with your project. All NMEA0183 versions are currently supported. NOTE: When using a Garmin GPS device, connected using an USB cable, no virtual serial port driver is needed, the GPS Toolkit communicates directly with Garmin's USB driver using Garmin's PVT protocol. Installation There are 2 ways to install this software on your computer: Automatic Installation To use the automatic installation, just download the installer from our website. The setup wizard will guide you through the installation process. After installation has been completed, all components and code samples are installed on your computer. Manual Installation When you want to include the product in your installer, or you want to test the component on a pc without using the setup wizard, just copy the core of the toolkit, the "GpsCtl32.dll" or GpsCtl64.dll (depending on the target platform) to the target computer, and register the control with the REGSVR32 command like this (replace the path with the path to your copy of the file): REGSVR32 C:\Program Files\Eye4Software\Gps Toolkit\Program\GpsCtl32.dll or for a x64 platform: REGSVR32 C:\Program Files\Eye4Software\Gps Toolkit\Program\GpsCtl64.dll When you encounter any troubles registering the component, make sure you have Administrator rights and/or UAC is turned off. 2. Getting Started This section of the manual describes how to use the component in the various programming environments. If your programming language is not listed, please contact our support department. Microsoft Visual C++ First, you have to create a new project. The toolkit can be used with both GUI and Console projects. Make sure the component is installed and registered on your development PC. For installation on a development PC, the automatic installation is recommended. In order to use the GPS toolkit from Visual C++, you have to copy some include files to your new project folder. These files are included in the Visual C++ sample included with the product: "GpsCtrl.h", "GpsCtrl_i.c" and "GpsConstantsX.h". In the source file you are going to use the component, include the following lines of code: #include "GpsCtrl.h" #include "GpsCtrl_i.c" #include "GpsConstantsX.h" Declare the component in your code like this: IGps * m_pGps = NULL; IGpsUtilities * m_pUtilities = NULL; IGpsProjection * m_pGpsProjection = NULL; IGpsDatumParameters * m_pGpsDatumParameters = NULL; IGpsGridParameters * m_pGpsDatumParameters = NULL; Because we are going to use an ActiveX object, we have to initialize COM like this: CoInitialize ( NULL ); Now you should be able to create an instance of the object(s), always make sure that you check the m_pGps pointer before using it, if its value is NULL, most probably the component isn't registered on the system. CoCreateInstance ( CLSID_Gps, NULL, CLSCTX_INPROC_SERVER, IID_IGps, ( void ** ) &m_pGps ); CoCreateInstance ( CLSID_GpsUtilities, NULL, CLSCTX_INPROC_SERVER, IID_IGpsUtilities, ( void ** ) &m_pGpsUtilities ); CoCreateInstance ( CLSID_GpsProjection, NULL, CLSCTX_INPROC_SERVER, IID_IGpsProjection, ( void ** ) &m_pGpsProjection ); CoCreateInstance ( CLSID_GpsDatumParameters, NULL , CLSCTX_INPROC_SERVER, IID_IGpsDatumParameters, ( void ** ) &m_pGpsDatumParameters ); CoCreateInstance ( CLSID_GpsGridParameters, NULL, CLSCTX_INPROC_SERVER, IID_IGpsGridParameters, ( void ** ), &m_pGpsGridParameters ); Visual Basic First you have to create a new project. The toolkit can be used with both GUI and Console projects, for a GUI object, just select the "Standard EXE" project option. Make sure the component is installed and registered on your development PC. For installation on a development PC, the automatic installation is recommended. In order to use the GPS Toolkit from Visual Basic, you have to add a reference to the ActiveX control. You can do this by selecting the "References..." option from the "Project" menu, and check the checkbox in front of the "Eye4Software GPS Toolkit" and click "OK". Declare the component in your code like this: Private objGps As Gps Private objGpsUtilities As GpsUtilities Private objGpsProjection As GpsProjection Private objGpsDatumParameters As GpsDatumParameters Private objGpsGridPatameters As GpsGridParameters Private objGpsConstants As GpsConstants Now you should be able to create an instance of the object(s), always make sure that you check the objGps object (it should not be Null) before using it. Set objGps = CreateObject ("Eye4Software.Gps") Set objGpsUtilities = CreateObject ("Eye4Software.GpsUtilities") Set objGpsProjection = CreateObject ("Eye4Software.GpsProjection") Set objGpsDatumParameters = CreateObject ("Eye4Software.GpsDatumParameters") Set objGpsGridParameters = CreateObject ("Eye4Software.GpsGridParameters") Set objGpsConstants = CreateObject ("Eye4Software.GpsConstants") Visual Basic .NET First you have to create a new project. The toolkit can be used with both .NET Windows Forms and Console Application projects, for a Windows Forms object, just select the "Windows Application" project option. Make sure the component is installed and registered on your development PC. For installation on a development PC, the automatic installation is recommended. In order to use the GPS Toolkit from Visual Basic .NET, you have to add a reference to the ActiveX control. You can do this by selecting the "Add Reference..." option from the "Project" menu, and check the checkbox in front of the "Eye4Software GPS Toolkit 2.2" and click "OK". Declare the component in your code like this: Private objGps As Gps Private objGpsUtilities As GpsUtilities Private objGpsProjection As GpsProjection Private objGpsDatumParameters As GpsDatumParameters Private objGpsGridPatameters As GpsGridParameters Private objGpsConstants As GpsConstants Now you should be able to create an instance of the object(s), always make sure that you check the objGps object (it should not be Null) before using it. objGps = New Gps objGpsUtilities
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages111 Page
-
File Size-