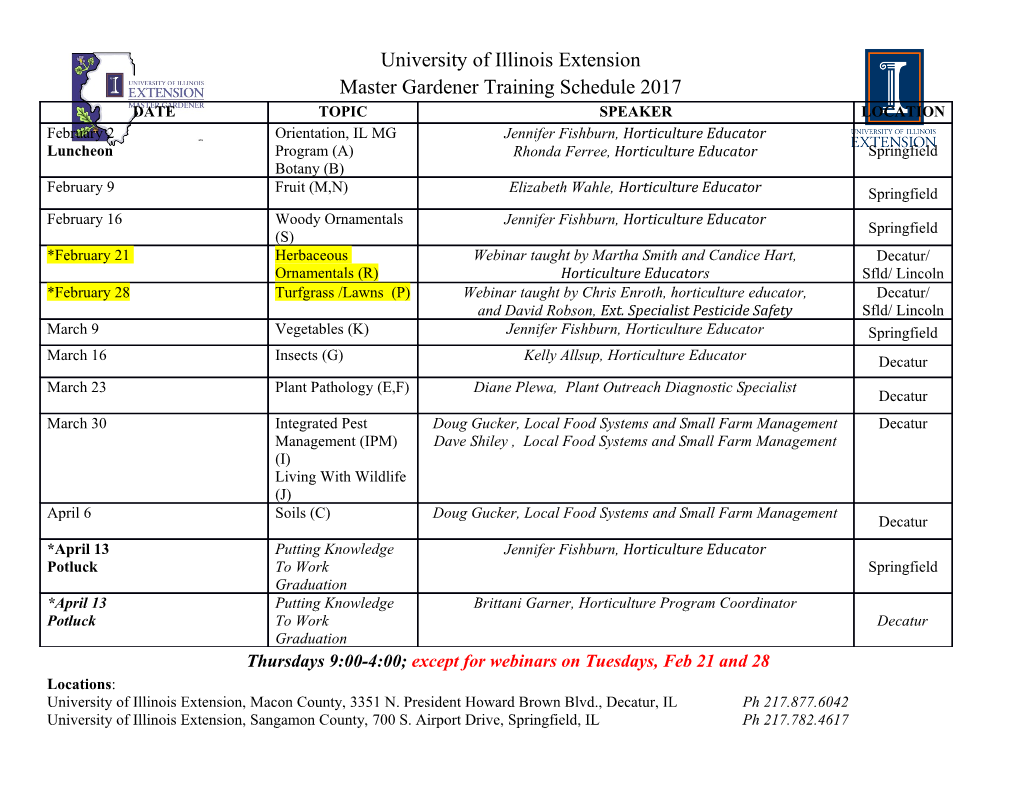
FROM OUR PEER REVIEW ... “Chris Richardson targets critical design issues for lightweight Java enterprise applications using POJOs with fantastic in-depth exam- ples. This book extends Martin Fowler’s great book, Enterprise Architecture Patterns, as well as the more recent Domain-Driven Design by Eric Evans, by providing practical design guidance and useful examples. It also addresses the architecture and design issues asso- ciated with Spring and Hibernate development, whereas Man- ning’s companion ‘in Action’ books focus primarily on the Spring and Hibernate technologies. “This is a powerful book for architects, senior developers, and consultants. It uniquely combines best practices and design wisdom to integrate domain-driven design and test-driven development for object-oriented Java enterprise applications using lightweight Spring, Hibernate, and JDO technologies. “The table of contents reflects the important topics that most architects and enterprise developers face every day. There is signifi- cant need for a book like this, which shows how to address many common and complex design issues with real-world examples. The content in this book is unique and not really available elsewhere.” DOUG WARREN Software Architect Countrywide Financial “POJOs in Action fills a void: the need for a practical explanation of the techniques used at various levels for the successful building of J2EE projects. This book can be compared with the less enterprise- oriented and more abstract J2EE Development without EJB by Rod Johnson, but Richardson offers a step-by-step guide to a successful J2EE project. The explanations of the various alternatives available for each step provide the main thrust of this book. Also, the various ‘When to use it’ paragraphs are helpful in making choices. “The ‘lightweight J2EE’ point of view is very under-represented in books and this one is the most didactic J2EE-light book I have read.” OLIVIER JOLLY J2EE Architect Interface SI “POJOs in Action provides good coverage of the current EJB 3.0 and POJO discussions in the developer community. The book is easy to read and has lots of good examples. It provides a complete discus- sion of the subject matter, from the basic data definitions to the implications on the client-side: I haven’t seen another book that takes this approach, so it definitely fills a niche. “The author describes some technologies as being unsuitable for most situations, but sticks to his guns and maintains the philosophy of providing the user with a choice, describing each possible solu- tion in depth, despite previous assertions that a particular solution may be sub-optimal. This reflects the realities in a developer’s world, where we are often forced to use technologies that we might not have chosen ourselves: this support is A Good Thing. “Compared to Martin Fowler’s Enterprise Architecture Patterns, which provides a generalized description of the enterprise, this book attempts to present the solutions to the situations Fowler describes. While much of the information can be found elsewhere, including the websites for the technologies as well as Fowler’s book, the combination of focused information and the explicit samples makes POJOs in Action much more than the sum of its parts. It isn’t merely a duplication of what’s available elsewhere: it carefully explains the technologies with plenty of sample code, in a consistent style.” BRENDAN MURRAY Senior Software Architect IBM POJOs in Action DEVELOPING ENTERPRISE APPLICATIONS WITH LIGHTWEIGHT FRAMEWORKS CHRIS RICHARDSON MANNING Greenwich (74° w. long.) For online information and ordering of this and other Manning books, please visit www.manning.com. The publisher offers discounts on this book when ordered in quantity. For more information, please contact: Special Sales Department Manning Publications Co. 209 Bruce Park Avenue Fax:(203) 661-9018 Greenwich, CT 06830 email: [email protected] ©2006 by Chris Richardson. All rights reserved. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form or by means electronic, mechanical, photocopying, or otherwise, without prior written permission of the publisher. Many of the designations used by manufacturers and sellers to distinguish their products are claimed as trademarks. Where those designations appear in the book, and Manning Publications was aware of a trademark claim, the designations have been printed in initial caps or all caps. Recognizing the importance of preserving what has been written, it is Manning’s policy to have the books they publish printed on acid-free paper, and we exert our best efforts to that end. Manning Publications Co.Copyeditor:Liz Welch 209 Bruce Park Avenue Typesetter: Gordan Salinovic Greenwich, CT 06830 Cover designer: Leslie Haimes ISBN 1932394583 Printed in the United States of America 1 2 3 4 5 6 7 8 9 10 – VHG – 10 09 08 07 06 05 To my mum, my dad, and my grandparents Thank you for everything mmmmmmmmmmmmmmmmmmmmmmmmmmm—C. R. brief contents PART 1 OVERVIEW OF POJOS AND LIGHTWEIGHT FFFFFFFFFFFFFFFFRAMEWORKS .............................................1 Chapter 1 ■ Developing with POJOs: faster and easier 3 Chapter 2 ■ J2EE design decisions 31 PART 2 A SIMPLER, FASTER APPROACH................... 59 Chapter 3 ■ Using the Domain Model pattern 61 Chapter 4 ■ Overview of persisting a domain model 95 Chapter 5 ■ Persisting a domain model with JDO 2.0 149 Chapter 6 ■ Persisting a domain model with Hibernate 3 195 Chapter 7 ■ Encapsulating the business logic with a POJO façade 243 PART 3 VARIATIONS ........................................... 287 Chapter 8 ■ Using an exposed domain model 289 Chapter 9 ■ Using the Transaction Script pattern 317 Chapter 10 ■ Implementing POJOs with EJB 3 360 vii viii BRIEF CONTENTS PART 4 DEALING WITH DATABASES AND CCCCCCCCCCCCCONCURRENCY .......................................405 Chapter 11 ■ Implementing dynamic paged queries 407 Chapter 12 ■ Database transactions and concurrency 451 Chapter 13 ■ Using offline locking patterns 488 contents preface xix acknowledgments xxi about this book xxiii about the title xxx about the cover illustration xxxi PART 1 OVERVIEW OF POJOS AND LLLLLLLLLLLIGHTWEIGHT FRAMEWORKS ............................1 Developing with POJOs: faster and easier 3 1 1.1 The disillusionment with EJBs 5 A brief history of EJBs 5 ■ A typical EJB 2 application architecture 6 The problems with EJBs 7 ■ EJB 3 is a step in the right direction 11 1.2 Developing with POJOs 12 Using an object-oriented design 14 ■ Using POJOs 15 ■ Persisting POJOs 16 ■ Eliminating DTOs 18 ■ Making POJOs transactional 19 ■ Configuring applications with Spring 25 Deploying a POJO application 27 ■ POJO design summary 28 1.3 Summary 30 ix x CONTENTS J2EE design decisions 31 2 2.1 Business logic and database access decisions 32 2.2 Decision 1: organizing the business logic 35 Using a procedural design 35 ■ Using an object-oriented design 36 Table Module pattern 37 2.3 Decision 2: encapsulating the business logic 37 EJB session façade 38 ■ POJO façade 39 Exposed Domain Model pattern 40 2.4 Decision 3: accessing the database 41 What’s wrong with using JDBC directly? 41 Using iBATIS 42 ■ Using a persistence framework 43 2.5 Decision 4: handling concurrency in database transactions 44 Isolated database transactions 44 ■ Optimistic locking 45 Pessimistic locking 45 2.6 Decision 5: handling concurrency in long transactions 46 Optimistic Offline Lock pattern 46 Pessimistic Offline Lock pattern 47 2.7 Making design decisions on a project 48 Overview of the example application 48 ■ Making high-level design decisions 51 ■ Making use case–level decisions 53 2.8 Summary 58 PART 2 A SIMPLER, FASTER APPROACH ........................ 59 Using the Domain Model pattern 61 3 3.1 Understanding the Domain Model pattern 62 Where the domain model fits into the overall architecture 63 An example domain model 64 ■ Roles in the domain model 66 3.2 Developing a domain model 68 Identifying classes, attributes, and relationships 69 Adding behavior to the domain model 69 CONTENTS xi 3.3 Implementing a domain model: an example 80 Implementing a domain service method 80 ■ Implementing a domain entity method 87 ■ Summary of the design 92 3.4 Summary 93 Overview of persisting a domain model 95 4 4.1 Mapping an object model to a database 96 Mapping classes 97 ■ Mapping object relationships 99 ■ Mapping inheritance 103 ■ Managing object lifecycles 107 Persistent object identity 107 4.2 Overview of ORM frameworks 108 Why you don’t want to persist objects yourself 109 ■ The key features of an ORM framework 109 ■ Benefits and drawbacks of using an ORM framework 114 4.3 Overview of JDO and Hibernate 117 Declarative mapping between the object model and the schema 117 API for creating, reading, updating, and deleting objects 118 Query language 119 ■ Support for transactions 120 ■ Lazy and eager loading 121 ■ Object caching 121 ■ Detached objects 124 Hibernate vs. JDO 124 4.4 Designing repositories with Spring 125 Implementing JDO and Hibernate repositories 125 ■ Using the Spring ORM classes 126 ■ Making repositories easier to test 129 4.5 Testing a persistent domain model 132 Object/relational testing strategies 133 ■ Testing against the database 135 ■ Testing without the database 138 Overview of ORMUnit 140 4.6 Performance tuning JDO and Hibernate 141 Without any tuning 141 ■ Configuring eager loading 142 Using a process-level cache 145 ■ Using the query cache 145 4.7 The example schema 146 4.8 Summary 148 Persisting a domain model with JDO
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages594 Page
-
File Size-