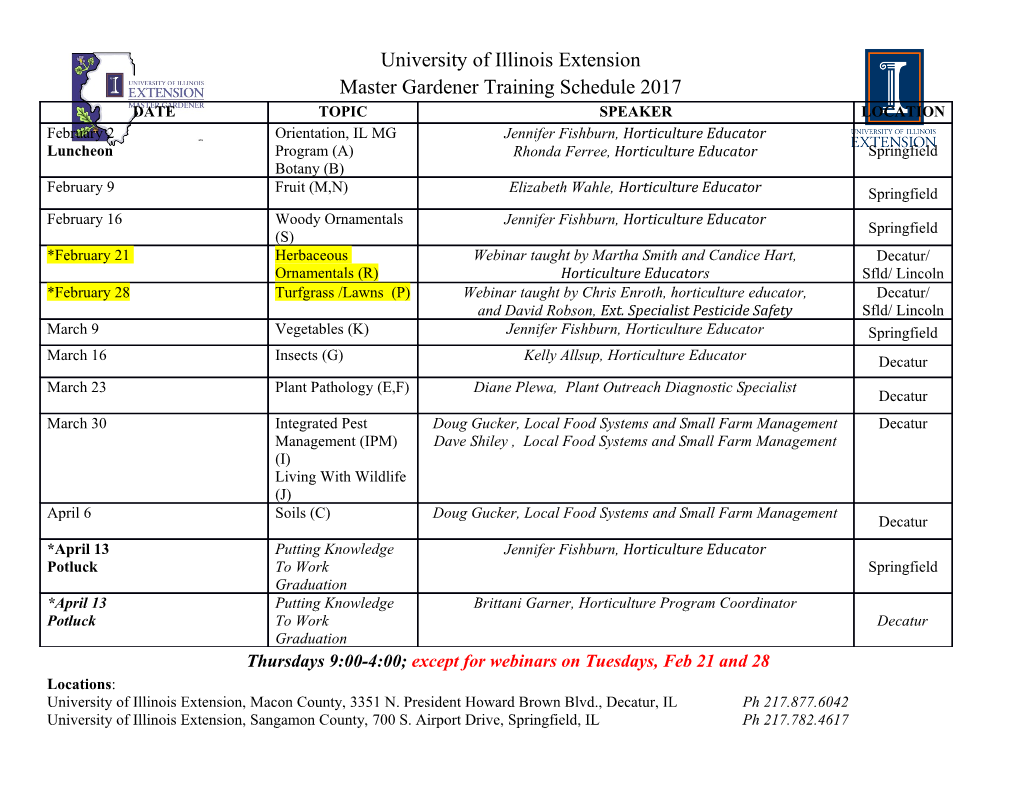
Supporting Precise Garbage Collection in Java Bytecode- to-C Ahead-of-Time Compiler for Embedded Systems Dong-Heon Jung Sung-Hwan Jaemok Lee Soo-Mook JongKuk Park Bae Moon School of Electrical Engineering and Computer Science Seoul National University, Seoul 151-742, Korea {clamp,uhehe99,seasoul,jaemok,smoon}@altair.snu.ac.kr Abstract digital TVs, and telematics [19]. The advantage of Java as an A Java bytecode-to-C ahead-of-time compiler (AOTC) can embedded platform is three folds. First, the use of a virtual improve the performance of a Java virtual machine (JVM) by machine allows a consistent runtime environment for a wide translating bytecode into C code, which is then compiled into range of devices that have different CPUs, OS, and hardware machine code via an existing C compiler. Although AOTC is (e.g., displays). Secondly, Java has an advantage in security such effective in embedded Java systems, a bytecode-to-C AOTC that it is extremely difficult for a malicious Java code to break could not easily employ precise garbage collection (GC) due to a down a whole system. Finally, it is much easier to develop difficulty in making a GC map, which keeps information on software contents with Java due to its sufficient, mature APIs where each root live object is located when GC occurs. This is and its language features that increase software stability such as one of the reasons why all previous JVMs using a bytecode-to-C garbage collection and exception handling. AOTC employed conservative GC, which can lead to poorer GC The advantage of platform independence is achieved by using performance with more frequent memory shortage, especially in the Java virtual machine (JVM), a program that executes Java’s embedded systems where memory is tight. compiled executable called bytecode [1]. The bytecode is a In this paper, we propose a way of allowing precise GC in a stack-based instruction set which can be executed by bytecode-to-C AOTC by generating additional C code which interpretation on any platform without porting the original collects GC map-equivalent information at runtime. In order to source code. Since this software-based execution is obviously reduce this runtime overhead, we also propose two optimization much slower than hardware execution, compilation techniques techniques which remove unnecessary C code. Our experimental for executing Java programs as native executables have been results on Sun’s CVM indicate that we can support precise GC used, such as just-in-time compiler (JITC) [15] and ahead-of- for bytecode-to-C AOTC with a relatively low overhead. time compiler (AOTC) [7]. JITC translates bytecode into machine code at runtime while AOTC translates before runtime. Categories and Subject Descriptors AOTC is more advantageous in embedded systems since it D3.3 [Programming Languages]: Language Constructs and obviates the runtime translation overhead and the memory Features; D.3.4 [Processors]: Compilers, Run-time overhead of JITC, which consume the limited computing power environments; D.4.7 [Operating Systems]: Organization and and memory space of embedded systems. Design-real-time and embedded systems; There are two approaches to AOTC. One is translating bytecode directly into machine code, referred to as bytecode-to- General Terms native [2-6]. The other approach is translating bytecode into C Design, Experimentation, Performance, Languages code first, which is then compiled into machine code by an Keywords existing compiler, referred to as bytecode-to-C (b-to-C) [7-10]. The approach of b-to-C allows faster development of a stable, Bytecode-to-C, ahead-of-time compiler, precise garbage powerful AOTC by resorting to full optimizations of an existing collection, Java virtual machine, J2ME CDC compiler and by using its debugging and profiling tools. It also 1. Introduction allows a portable AOTC that can work on different CPUs. Java has been employed popularly as a standard software There is one important issue in designing a b-to-C AOTC platform for many embedded systems, including mobile phones, related to garbage collection (GC) [11], though. A JVM that _________________________________________________________________ supports precise GC requires a GC map at each program point where GC can possibly occur, which is a data structure describing This research was supported in part by Samsung Electronics. where each root live object is located at that point. Since a b-to-C AOTC normally translates such locations that have root references into C variables, it is difficult to know where the C compiler Permission to make digital or hard copies of all or part of this work for places those C variables in the final machine code. All previous personal or classroom use is granted without fee provided that copies are not made or distributed for profit or commercial advantage and that JVMs using a b-to-C AOTC employed conservative GC [7, 9, 10], copies bear this notice and the full citation on the first page. To copy which is simpler to implement but can lead to poorer GC otherwise, or republish, to post on servers or to redistribute to lists, performance than precise GC. requires prior specific permission and/or a fee. CASES'06, October 23–25, 2006, Seoul, Korea. This paper proposes a method to support precise GC in a b-to- Copyright 2006 ACM 1-59593-543-6/06/0010...$5.00. C AOTC by generating C code that records references in a stack 35 frame whenever a reference-type C variable is updated. In order (a) Java Method (b) Bytecode to reduce the runtime overhead caused by the additional C code, public int max(int a, 0: iload_0 we also propose two optimization techniques. We evaluated the int b) { 1: iload_1 proposed technique on a CVM in Sun’s J2ME CDC environment return (a>=b)? a:b; 2: if_icmplt 9 which requires precise GC. We developed a b-to-C AOTC in this } 5: iload_0 environment and performed experiments with it. 6: goto 10 9: iload_1 The rest of this paper is organized as follows. Section 2 10:ireturn reviews precise GC and our b-to-C AOTC. Section 3 introduces the proposed solution to allow precise GC with a b-to-C AOTC. (c) Translated C Code Section 4 describes two optimization techniques to reduce the Int Java_java_lang_Math_max_II(JNIEnv overhead of our solution. Section 5 shows our experimental *env, int l0_int, int l1_int) results. A summary follows in Section 6. { int s0_int; 2. Precise GC and Bytecode-to-C AOTC int s1_int; In this section, we briefly review our b-to-C AOTC and issues in s0_int = l0_int; // 0: precise GC. Then, we discuss why it is difficult to support s1_int = l1_int; // 1: precise GC with a b-to-C AOTC. if(s0_int < s1_int) goto L9; // 2: 2.1 Overview of JVM and Our Bytecode-to-C s0_int = l0_int; // 5: goto L10; // 6: AOTC L9: s0_int = l1_int; // 9: The Java VM is a typed stack machine [14]. Each thread of L10: return s0_int; // 10: execution has its own Java stack where a new activation record } is pushed when a method is invoked and is popped when it Figure 0. An example of Java code and translated C code returns. An activation record includes state information, local variables and the operand stack. Method parameters are also 2.2 Precise GC local variables which are initialized to the actual parameters by As an object-oriented language, Java applications tend to the JVM. All computations are performed on the operand stack allocate objects at a high rate while the memory space of and temporary results are saved in local variables, so there are embedded systems is tight, so GC should reclaim garbage objects many pushes and pops between the local variables and the efficiently. Most GC techniques first trace all reachable objects on operand stack. a directed graph formed by program variables and heap-allocated In our b-to-C AOTC, each local variable is translated into a C objects where program variables constitute the root set of the variable (which we call a local C variable). Also, each stack slot graph [11]. In Java, the roots are located in the operand stack slots is also translated into a C variable (which we call a stack C and local variables of all methods in the call stack, whose types variable). Since the same stack slot can be pushed with are object references. So, GC traces all reachable objects starting differently-typed values during execution, a type name is from the root set and reclaims all unreachable objects. There are attached into a stack C variable name such that a stack slot can two approaches to tracing reachable objects: conservative and be translated into multiple C variables. For example, s0_ref is precise [12]. a C variable corresponding to a reference-type stack slot 0, while Conservative GC regards all word-size data items saved in the s0_int is a C variable corresponding to an integer-type stack JVM as references, which simplifies the identification of the root slot 0. set and makes the GC module easily portable [13]. However, non- Our AOTC first analyzes the bytecode and decides the C reference numeric values can be misunderstood as references, variables that need to be declared. It then translates each which might cause incomplete reclamation of garbage objects. bytecode one-by-one into corresponding C statements, with the Precise GC can reclaim all garbage objects completely by status of the operand stack being kept track of. For example, computing the root set precisely with a help from the JVM or from aload_1 which pushes a reference-type local variable 1 onto the compiler. The GC module is thus more complicated to the stack is translated into a C statement s0_ref = l1_ref; implement, yet there is no misunderstanding of numeric values as if the current stack pointer points to the zero_th slot when this references [12].
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages8 Page
-
File Size-