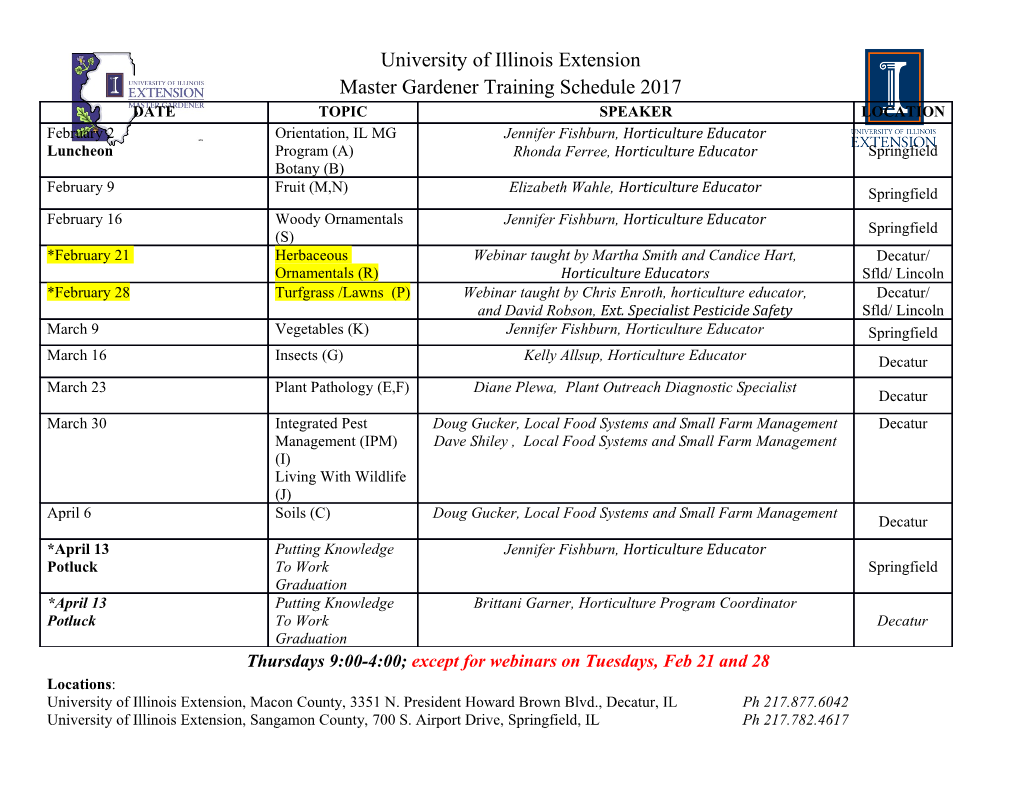
WritingWriting XMLXML withwith JavaJava • You don’t need to know any special APIs like DOM or SAX or JDOM. All you have to know is how to System.out.println(). If you want to store your XML document in a file, you can use the FileOutputStream class ApplicationApplication DevelopmentDevelopment instead. withwith XMLXML andand JavaJava • We are going to develop a program that writes Fibonacci numbers into an XML document. • the principles of XML we will use here Lecture 3 will be much more broadly applicable Writing XML with Java to other, more complex systems. Introduction to Parsing • The key idea is that data arrives from some source, is encoded in XML, and is then output. Where the input comes from, whether an algorithm, a file, a network socket, user input, or some other source, really doesn’t concern us here. Kosmas Kosmopoulos Application development with XML and Java Kosmas Kosmopoulos Application development with XML and Java FibonacciFibonacci NumbersNumbers FibonacciFibonacci NumbersNumbers • As far as we know, the Fibonacci series • It’s very easy to calculate the was first discovered by Leonardo of Pisa Fibonacci sequence by computer. A around 1200 C.E. Leonardo “How many simple for loop will do. For example, pairs of rabbits are born in one year from this code fragment prints the first 40 one pair?” To solve his problem, Fibonacci numbers: Leonardo estimated that rabbits have a int low = 1; one month gestation period, and can first int high = 1; mate at the age of one month, so that for (int i = 1; i <= 40; i++) { each female rabbit has its first litter at two System.out.println(low); int temp = high; months. He made the simplifying high = high+low; assumption that each litter consisted of low = temp; } exactly one male and one female. • However, the Fibonacci numbers do • The rabbits aren’t so important, but the grow very large very quickly, and math is. Each integer in the series is exceed the bounds of an int shortly formed by the sum of the two previous before the fiftieth generation. integers. The first two integers in the Consequently, it’s better to use the series are 1 and 1.The Fibonacci series turns up in some very unexpected places java.math.BigInteger class instead including decimal expansions of π. Kosmas Kosmopoulos Application development with XML and Java Kosmas Kosmopoulos Application development with XML and Java FibonacciFibonacci NumbersNumbers ProducingProducing XMLXML documentdocument import java.math.BigInteger; <?xml version="1.0"?> public class FibonacciNumbers <Fibonacci_Numbers> { <fibonacci>1</fibonacci> public static void main(String[] <fibonacci>1</fibonacci> args) { <fibonacci>2</fibonacci> BigInteger low = BigInteger.ONE; <fibonacci>3</fibonacci> BigInteger high = BigInteger.ONE; <fibonacci>5</fibonacci> for (int i = 1; i <= 10; i++) <fibonacci>8</fibonacci> { <fibonacci>13</fibonacci> System.out.println(low); <fibonacci>21</fibonacci> BigInteger temp = high; <fibonacci>34</fibonacci> high = high.add(low); <fibonacci>55</fibonacci> low = temp; </Fibonacci_Numbers> } } } • To produce this, just add string • When run, this program produces the first ten Fibonacci numbers: literals for the <fibonacci> and </fibonacci> tags inside the print java FibonacciNumbers statements, as well as a few extra print 1 1 2 3 5 8 13 21 34 55 statements to produce the XML declaration and the root element start- and end-tags. XML documents are just text, and you can output them any way you’d output any other text document. Kosmas Kosmopoulos Application development with XML and Java Kosmas Kosmopoulos Application development with XML and Java JavaJava ExampleExample XMLXML--RPCRPC import java.math.BigInteger; • An XML-RPC request is just an XML public class FibonacciXML document sent over a network socket. { • We will write a very simple XML-RPC public static void main(String[] args) { client for a particular service. BigInteger low = BigInteger.ONE; – We will just ask the user for the data to BigInteger high = BigInteger.ONE; send to the server, wrap it up in some XML, and write it onto a URL object System.out.println("<?xml pointing at the server. version=\"1.0\"?>"); System.out.println("<Fibonacci_Number – The response will come back in XML as s>"); well. Since we haven’t yet learned how to read XML documents, Response will be for (int i = 0; i < 10; i++) { a text to System.out. Later we’ll pay more System.out.print(" <fibonacci>"); attention to the response, and provide a System.out.print(low); nicer user interface. System.out.println("</fibonacci>"); • The specific XML-RPC service we’re BigInteger temp = high; going to talk to is a Fibonacci high = high.add(low); generator. The request passes an int to low = temp; the server. The server responds with } the value of that Fibonacci number. System.out.println("</Fibonacci_Numbe rs>"); } } Kosmas Kosmopoulos Application development with XML and Java Kosmas Kosmopoulos Application development with XML and Java ExampleExample ExampleExample • This request document asks for the • The client needs to read an integer input by value of the 23rd Fibonacci number: the user, wrap that integer in the XML-RPC <?xml version="1.0"?> envelope, then send that document to the <methodCall> server using HTTP POST. <methodName>calculateFibonacci • In Java the simplest way to post a document </methodName> is through the <params> java.net.HttpURLConnection class. You <param> get an instance of this class by calling a URL <value><int>23</int></value> object’s openConnection() method, and </param> then casting the resulting URLConnection </params> HttpURLConnection </methodCall> object to . • Once you have an HttpURLConnection, And here’s the response: you set its protected doOutput field to true <?xml version="1.0"?> using the setDoOutput() method and its <methodResponse> <params> request method to POST using the <param> setRequestMethod() method. <value><double>28657</double>< • Then you grab hold of an output stream /value> using getOutputStream() and proceed to </param> </params> write your request body on that. </methodResponse> Kosmas Kosmopoulos Application development with XML and Java Kosmas Kosmopoulos Application development with XML and Java XMLXML ParsersParsers WhatWhat isis anan XMLXML ParserParser What is a Parser? The parser is a software library (a Java class) that reads the XML document and checks it Defining Parser Responsibilities for well-formedness. Client applications use Evaluating Parsers method calls defined in the parser API to receive or request information the parser Validation retrieves from the XML document. – The parser shields the client application from Validating v. Nonvalidating Parsers all the complex and not particularly relevant XML Interfaces details of XML including: – Transcoding the document to Unicode Object v. Tree Based Interfaces – Assembling the different parts of a document Interface Standards: DOM, SAX divided into multiple entities. – Resolving character references Java XML Parsers – Understanding CDATA sections – Checking hundreds of well-formedness constraints – Maintaining a list of the namespaces in-scope on each element. – Validating the document against its DTD or schema – Associating unparsed entities with particular URLs and notations – Assigning types to attributes Kosmas Kosmopoulos Application development with XML and Java Kosmas Kosmopoulos Application development with XML and Java TheThe BigBig PicturePicture Java Application XML XML Or Parser Document Servlet WhatWhat isis anan XMLXML Parser?Parser? An XML Parser enables your Java application or Servlet to more easily access XML Data. Kosmas Kosmopoulos Application development with XML and Java Kosmas Kosmopoulos Application development with XML and Java DefiningDefining ParserParser ThreeThree WhyWhy useuse anan XMLXML Parser?Parser? ResponsibilitiesResponsibilities 1. Retrieve and Read an XML If your application is going to use document XML, you could write your own For example, the file may reside on the local file system or on another web site. parser. The parser takes care of all the But, it makes more sense to use a necessary network connections and/or file connections. pre-built XML parser. This helps simplify your work, as you This enables you to do build your do not need to worry about creating application much more quickly. your own network connections. 2. Ensure that the document adheres to specific standards. Parser Evaluation Does the document match the DTD? When evaluating which XML Is the document well-formed? Parser to use, there are two very 3. Make the document contents important questions to ask: available to your application. Is the Parser validating or non- The parser will parse the XML validating? document, and make this data available to your application. What interface does the parser provide to the XML document? Kosmas Kosmopoulos Application development with XML and Java Kosmas Kosmopoulos Application development with XML and Java XMLXML ValidationValidation PerformancePerformance andand MemoryMemory Validating Parser Questions: Which parser will have better performance? a parser that verifies that the XML Which parser will take up less memory? document adheres to the DTD. Validating parsers: Non-Validating Parser more useful a parser that does not check the slower DTD. take up more memory Lots of parsers provide an option Non-validating parsers: less useful to turn validation on or off. faster take up less memory Therefore, when high performance and low-memory are the most important criteria,
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages23 Page
-
File Size-