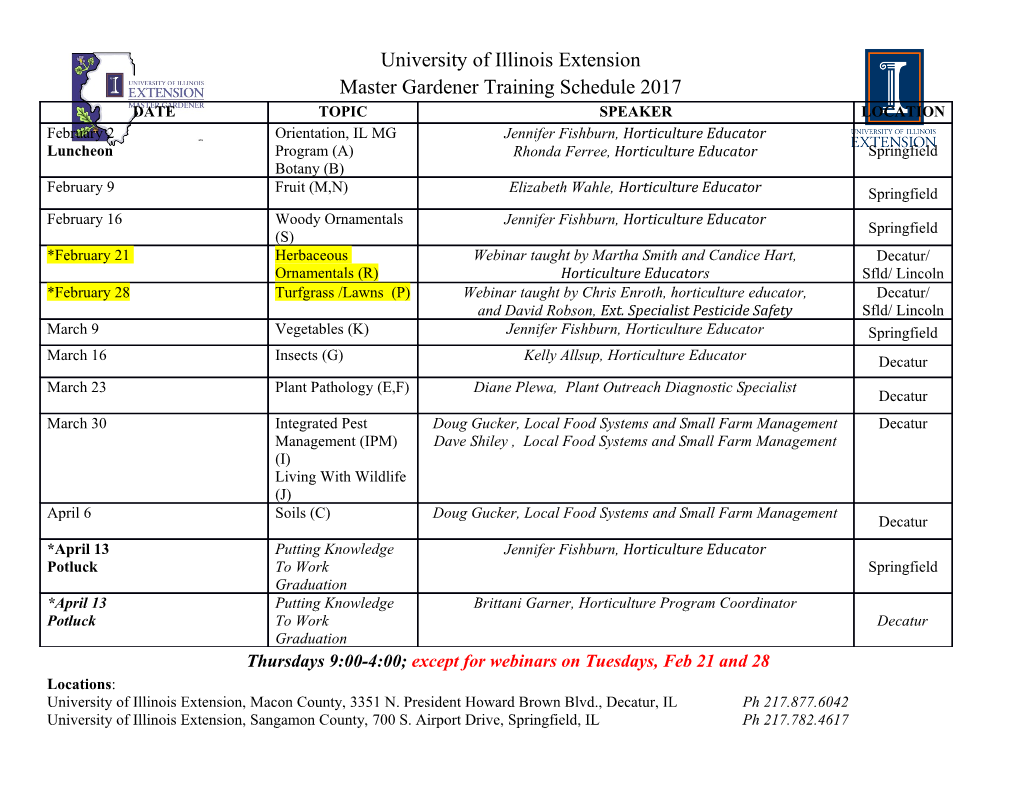
Power Set of a set and Relations 1 Power Set (1) • Definition: The power set of a set S, denoted P(S), is the set of all subsets of S. • Examples – Let A={a,b,c}, P(A)={,{a},{b},{c},{a,b},{b,c},{a,c},{a,b,c}} – Let A={{a,b},c}, P(A)={,{{a,b}},{c},{{a,b},c}} • Note: the empty set and the set itself are always elements of the power set. Power Set (2) • The power set is a fundamental combinatorial object useful when considering all possible combinations of elements of a set • Fact: Let S be a set such that |S|=n, then |P(S)| = 2n Proof of |P(A)|=2^|A| • Proof: ( By induction) – Base case holds: |A|=0, then A={} and P(A)={}. – Induction Hypothesis: Assume to be true for all sets of cardinality n-1, i.e. |A|=n-1 implies |P(A)|=2^{n-1}. – For |A|=n • Let A={a_1,a_2,…,a_n}. Any subset not containing a_n is a subset of B=A\{a_n}. How many subsets of A are there not containing a_n? This is nothing but the set of all subsets of B. The number is 2^{n-1} by induction hypothesis. 4 Proof of |P(A)|=2^|A| Contd… • How to get a subset of A containing a_n? – Take a subset S of B. – Let S’=S {a_n}. – Now S’ is a subset of A containing a_n. • Can any subset of A containing a_n be obtained in this way? – Answer is Yes!! – Let S’ be any subset of A containing a_n. Let S=S’\{a_n}. Now S is a subset of A not containing a_n. 5 Proof of |P(A)|=2^|A| Contd… • Let X_1 be the set of all subsets of A not containing a_n • Let X_2 be the set of all subsets of A containg a_n. • Now P(A)= X_1 X_2 and X_1 X_2 =. • Also S S’=S{a_n} is a bijection. – This implies |S|=|S’|=2^{n-1} by induction hypothesis • So |P(A))|= |S|+|S’|= 2^{n-1} +2^{n-1}=2^{n}. 6 How to generate all subsets of a Set of n elements? 7 Computer Representation of Sets (1) • There really aren’t ways to represent infinite sets by a computer since a computer has a finite amount of memory • If we assume that the universal set U is finite, then we can easily and effectively represent sets by bit vectors • Specifically, we force an ordering on the objects, say: U={a1, a2,…,an} • For a set AU, a bit vector can be defined as, for i=1,2,…,n – bi=0 if ai A – bi=1 if ai A Computer Representation of Sets (2) • Examples – Let U={0,1,2,3,4,5,6,7} and A={0,1,6,7} – The bit vector representing A is: 1100 0011 – How is the empty set represented? – How is U represented? • Set operations become trivial when sets are represented by bit vectors – Union is obtained by making the bit-wise OR – Intersection is obtained by making the bit-wise AND BITWISE OPERATOR (IN C) Operators Meaning of operators & Bitwise AND | Bitwise OR ^ Bitwise exclusive OR ~ Bitwise complement << Shift left >> Shift right Computer Representation of Sets (3) • Let U={0,1,2,3,4,5,6,7}, A={0,1,6,7}, B={0,4,5} • What is the bit-vector representation of B? • Compute, bit-wise, the bit-vector representation of AB • Compute, bit-wise, the bit-vector representation of AB • What sets do these bit vectors represent? Computer Representation of Sets … • Array representations: – U={1,2,….n} – S can be represented by an array A such that A[i]=1 if i S else A[i]=0. • Disjoint Set operations: – Union, j=Find(i) if I in S_j. 12 Programming Question • Using bit vector, we can represent sets of cardinality equal to the size of the vector • What if we want to represent an arbitrary sized set in a computer (i.e., that we do not know a priori the size of the set)? • What data structure could we use? • Tree representation of disjoint Set: To be discussed after Graphs are introduced. Relations 14 Relations and Their Properties – To represent relationships between elements of sets • Ordered pairs: binary relations • Definition 1: Let A and B be sets. A binary relation from A to B is a subset of AB. – a R b: (a,b)R • a is related to b by R – a R b: (a,b)R – Ex.1-3 • Functions as relations – Relations are generalization of functions FIGURE 1 (8.1) FIGURE 1 Displaying the Ordered Pairs in the Relation R from Example 3. Relations on a Set • Definition 2: a relation on the set A is a relation from A to A. – How many relations are there on a set with n elements? ( Such questions will be discussed in Counting and advanced counting) FIGURE 2 Displaying the Ordered Pairs in the Relation R from Example 4. Properties of Relations • Definition 3: A relation R on a set A is called reflexive if (a,a)R for every element aA. – a((a,a)R) • Definition 4: A relation R on a set A is called symmetric if (b,a)R whenever (a,b)R, for all a,bA. A relation R on a set A such that for all a,bA, if (a,b)R and (b,a)R, then a=b is called antisymmetric. – ab((a,b)R →(b,a)R) – ab(((a,b)R (b,a)R) →(a=b)) – Not opposites • Definition 5: A relation R on a set A is called transitive if whenever (a,b)R and (b,c)R, then (a,c)R, for all a,b,cA. – abc(((a,b)R (b,c)R) → (a,c)R) Combining Relations • Two relations can be combined in any way two sets can be combined • Definition 6: R: relation from A to B, S: relation from B to C. The composite of R and S is the relation consisting of ordered pairs (a,c), where aA, cC, and there exists an element bB such that (a,b)R and (b,c)S. (denoted by S○R) • Definition 7: Let R be a relation on the set A. The powers Rn, n=1,2,3,…, are defined recursively by R1=R, and Rn+1=Rn ○R. • Theorem 1: The relation R on a set A is transitive iff Rn R for n=1,2,3,… – Proof. Representing Relations • To represent binary relations – Ordered pairs – Tables – Zero-one matrices – Directed graphs Representing Relations Using Matrices • Suppose that R is a relation from A={a1,a2,…,am} to B={b1,b2,…,bn}. R can be represented by the matrix MR=[mij], where mij=1 if (ai,bj)R, or 0 if (ai,bj)R. FIGURE 1 The Zero-One Matrix for a Reflexive Relation. FIGURE 2 (8.3) FIGURE 2 The Zero-One Matrices for Symmetric and Antisymmetric Relations. Representing Relations Using Digraphs • Definition 1: A directed graph (digraph) consists of a set V of vertices (or nodes) and a set E of ordered pairs of elements of V called edges (or arcs). The vertex a is called the initial vertex of the edge (a,b), and the vertex b is called the terminal vertex of this edge. FIGURE 3 The Directed Graph. FIGURE 4 (8.3) FIGURE 4 The Directed Graph of the Relations R. FIGURE 5 (8.3) FIGURE 5 The Directed Graph of the Relations R. FIGURE 6 (8.3) FIGURE 6 The Directed Graph of the Relations R and S. Closures of Relations • Ex. Telephone line connections • Let R be a relation on a set A. R may or may not have some property P. If there is a relation S with property P containing R such that S is a subset of every relation with property P containing R, then S is called the closure of R with respect to P. – S: the smallest relation that contains R Closures • Reflexive closure – Ex: R={(1,1),(1,2),(2,1),(3,2)} on the set A={1,2,3} • The smallest relation contains R? – The reflexive closure of R=R∆, where ∆={(a,a)|aA} • Symmetric closure – The symmetric closure of R=RR-1, where R-1 ={(b,a)|(a,b)R} • Transitive closure Paths in Directed Graphs • Definition 1: A path from a to b in the directed graph G is a sequence of edges (x0,x1), (x1,x2), …,(xn-1,xn) in G, where n is a nonnegative integer, and x0=a and xn = b. – The path denoted by x0,x1,…,xn-1, xn has length n. – A path of length n >=1 that begins and ends at the same vertex is called a circuit or cycle. – There is a path from a to b in R if there is a sequence of elements a, x1, x2, …, xn-1, b with (a,x1)R, (x1,x2)R, …, and (xn-1,b)R. FIGURE 1 A Directed Graph. • Theorem 1: Let R be a relation on a set A. There is a path of length n, where n is a positive integer, from a to b iff (a,b)Rn. – Proof. (by mathematical induction) Transitive Closures • Definition 2: Let R be a relation on a set A. The connectivity relation R* consists of pairs (a,b) such that there is a path of length at least one from a to b in R. n – R*=n=1..R . • Theorem 2: The transitive closure of a relation R equals the connectivity relation R*.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages75 Page
-
File Size-