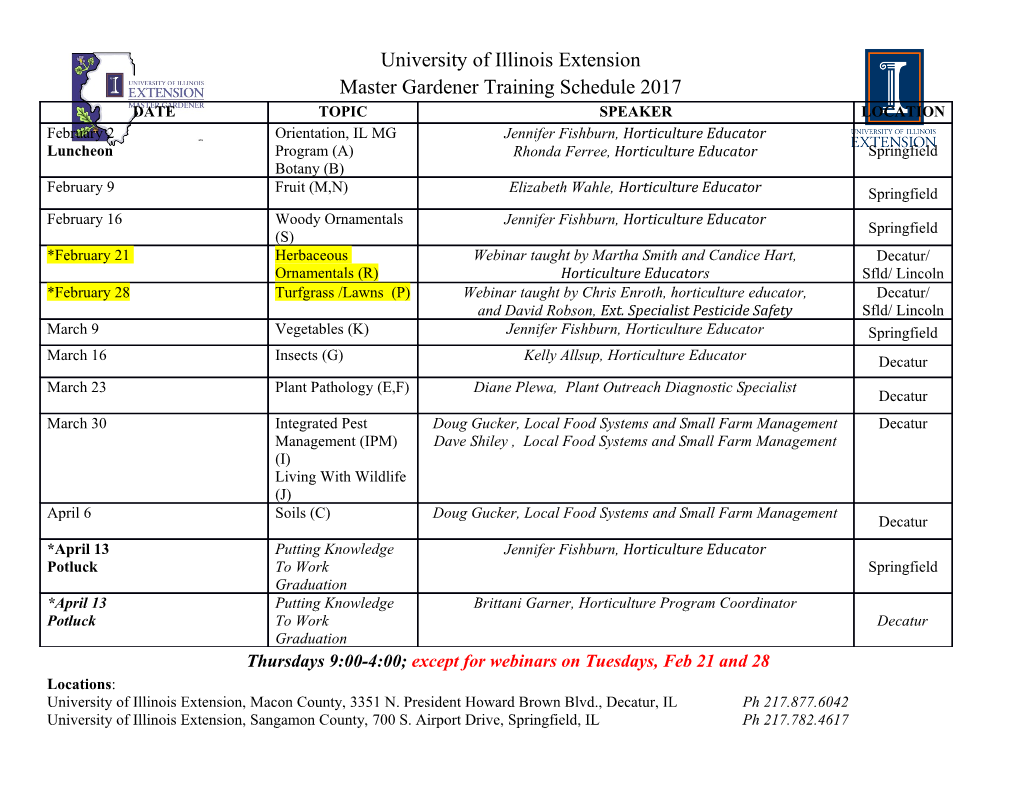
CH09 Computer Arithmetic Arithmetic & Logic Unit 4CPU combines of ALU and Control Unit, this chapter • Does the calculations discusses ALU • Everything else in the computer is there to service this • The Arithmetic and Logic Unit (ALU) unit • Number Systems • Handles integers • Integer Representation • May handle floating point (real) numbers • Integer Arithmetic • May be separate FPU (maths co-processor) • Floating-Point Representation • May be on chip separate FPU (486DX +) • Floating-Point Arithmetic TECH Computer Science CH08 ALU Inputs and Outputs Number Systems 4ALU does calculations with binary numbers • Decimal number system 4Uses 10 digits (0,1,2,3,4,5,6,7,8,9) 4In decimal system, a number 84, e.g., means 84 = (8x10) + 3 44728 = (4x1000)+(7x100)+(2x10)+8 4Base or radix of 10: each digit in the number is multiplied by 10 raised to a power corresponding to that digit’s position 4E.g. 83 = (8x101)+ (3x100) 44728 = (4x103)+(7x102)+(2x101)+(8x100) Decimal number system… Binary Number System • Fractional values, e.g. • Uses only two digits, 0 and 1 4472.83=(4x102)+(7x101)+(2x100)+(8x10-1)+(3x10-2) • It is base or radix of 2 4In general, for the decimal representation of • Each digit has a value depending on its position: X = {… x x x x x x …} 2 1 0. -1 -2 -3 1 0 4 102 = (1x2 )+(0x2 ) = 210 i 4 11 = (1x21)+(1x20) = 3 X = ∑ i xi10 2 10 2 1 0 4 1002 = (1x2 )+ (0x2 )+(0x2 ) = 410 3 2 1 0 41001.1012 = (1x2 )+(0x2 )+ (0x2 )+(1x2 ) -1 -2 -3 +(1x2 )+(0x2 )+(1x2 ) = 9.62510 1 Decimal to Binary conversion Decimal to Binary conversion, e.g. // • Integer and fractional parts are handled separately, • e.g. 11.81 to 1011.11001 (approx) 4Integer part is handled by repeating division by 2 411/2 = 5 remainder 1 4Factional part is handled by repeating multiplication by 45/2 = 2 remainder 1 2 42/2 = 1 remainder 0 • E.g. convert decimal 11.81 to binary 41/2 = 0 remainder 1 4Integer part 11 4Binary number 1011 4Factional part .81 4.81x2 = 1.62 integral part 1 4.62x2 = 1.24 integral part 1 4.24x2 = 0.48 integral part 0 4.48x2 = 0.96 integral part 0 4.96x2 = 1.92 integral part 1 4Binary number .11001 (approximate) Hexadecimal Notation: Integer Representation (storage) 4 command ground between computer and Human • Only have 0 & 1 to represent everything • Use 16 digits, (0,1,3,…9,A,B,C,D,E,F) • Positive numbers stored in binary 1 o • 1A16 = (116 x 16 )+(A16 x 16 ) 4e.g. 41=00101001 1 0 = (110 x 16 )+(1010 x 16 )=2610 • No minus sign • Convert group of four binary digits to/from one • No period hexadecimal digit, • How to represent negative number 40000=0; 0001=1; 0010=2; 0011=3; 0100=4; 0101=5; 4Sign-Magnitude 0110=6; 0111=7; 1000=8; 1001=9; 1010=A; 1011=B; 1100=C; 1101=D; 1110=E; 1111=F; 4Two’s compliment • e.g. 41101 1110 0001. 1110 1101 = DE1.DE Sign-Magnitude Two’s Compliment (representation) • Left most bit is sign bit • +3 = 00000011 • 0 means positive • +2 = 00000010 • 1 means negative • +1 = 00000001 • +18 = 00010010 • +0 = 00000000 • -18 = 10010010 • -1 = 11111111 • Problems • -2 = 11111110 4Need to consider both sign and magnitude in arithmetic • -3 = 11111101 4Two representations of zero (+0 and -0) 2 Geometric Depiction of Twos Benefits Complement Integers • One representation of zero • Arithmetic works easily (see later) • Negating is fairly easy (2’s compliment operation) 43 = 00000011 4Boolean complement gives 11111100 4Add 1 to LSB 11111101 Range of Numbers Conversion Between Lengths • 8 bit 2s compliment • Positive number pack with leading zeros 4+127 = 01111111 = 27 -1 • +18 = 00010010 7 4 -128 = 10000000 = -2 • +18 = 00000000 00010010 • 16 bit 2s compliment • Negative numbers pack with leading ones 4+32767 = 011111111 11111111 = 215 -1 • -18 = 10010010 4 -32768 = 100000000 00000000 = -215 • -18 = 11111111 10010010 • i.e. pack with MSB (sign bit) Integer Arithmetic: Negation Negation Special Case 1 4Take Boolean complement of each bit, I.e. each 1 to 0, • 0 = 00000000 and each 0 to 1. • Bitwise not 11111111 4Add 1 to the result • Add 1 to LSB +1 4E.g. +3 = 011 4Bitwise complement = 100 • Result 1 00000000 4Add 1 • Overflow is ignored, so: 4= 101 • - 0 = 0 OK! 4= -3 3 Negation Special Case 2 Addition and Subtraction • -128 = 10000000 • Normal binary addition • bitwise not 01111111 • 0011 0101 1100 • Add 1 to LSB +1 • +0100 +0100 +1111 • Result 10000000 • -------- ---------- ------------ • 0111 1001 = overflow 11011 • So: • Monitor sign bit for overflow (sign bit change as • -(-128) = -128 NO OK! adding two positive numbers or two negative • Monitor MSB (sign bit) numbers.) • It should change during negation • Subtraction: Take twos compliment of subtrahend • >> There is no representation of +128 in this case. (no then add to minuend +2n) 4i.e. a - b = a + (-b) • So we only need addition and complement circuits Hardware for Addition and Subtraction Multiplication • Complex • Work out partial product for each digit • Take care with place value (column) • Add partial products Multiplication Example Unsigned Binary Multiplication • (unsigned numbers e.g.) • 1011 Multiplicand (11 dec) • x 1101 Multiplier (13 dec) • 1011 Partial products • 0000 Note: if multiplier bit is 1 copy • 1011 multiplicand (place value) • 1011 otherwise zero • 10001111 Product (143 dec) • Note: need double length result 4 Flowchart for Execution of Example Unsigned Binary Multiplication Multiplying Negative Numbers Booth’s Algorithm • The previous method does not work! • Solution 1 4Convert to positive if required 4Multiply as above 4If signs of the original two numbers were different, negate answer • Solution 2 4Booth’s algorithm Example of Booth’s Algorithm Division • More complex than multiplication • However, can utilize most of the same hardware. • Based on long division 5 Flowchart for Division of Unsigned Binary Integers Unsigned Binary division 00001101 Quotient Divisor 1011 10010011 Dividend 1011 Partial 001110 1011 Remainders 001111 1011 Remainder 100 Real Numbers Floating Point • Numbers with fractions • Could be done in pure binary 4 0 -1 -3 41001.1010 = 2 + 2 +2 + 2 =9.625 Biased Significand or Mantissa Exponent • Where is the binary point? bit Sign • Fixed? exponent 4Very limited • +/- .significand x 2 • Moving? • Point is actually fixed between sign bit and body of 4How do you show where it is? mantissa • Exponent indicates place value (point position) Floating Point Examples Signs for Floating Point • Exponent is in excess or biased notation 4e.g. Excess (bias) 127 means 48 bit exponent field 4Pure value range 0-255 4Subtract 127 to get correct value 4Range -127 to +128 • The relative magnitudes (order) of the numbers do not change. 4Can be treated as integers for comparison. 6 Normalization // FP Ranges • FP numbers are usually normalized • For a 32 bit number • i.e. exponent is adjusted so that leading bit (MSB) of 48 bit exponent mantissa is 1 4+/- 2256 ≈ 1.5 x 1077 • Since it is always 1 there is no need to store it • Accuracy • (c.f. Scientific notation where numbers are 4The effect of changing lsb of mantissa normalized to give a single digit before the decimal 423 bit mantissa 2-23 ≈ 1.2 x 10-7 point 4About 6 decimal places • e.g. 3.123 x 103) Expressible Numbers IEEE 754 • Standard for floating point storage • 32 and 64 bit standards • 8 and 11 bit exponent respectively • Extended formats (both mantissa and exponent) for intermediate results • Representation: sign, exponent, faction 40: 0, 0, 0 4-0: 1, 0, 0 4Plus infinity: 0, all 1s, 0 4Minus infinity: 1, all 1s, 0 4NaN; 0 or 1, all 1s, =! 0 FP Arithmetic +/- FP Arithmetic x/÷ • Check for zeros • Check for zero • Align significands (adjusting exponents) • Add/subtract exponents • Add or subtract significands • Multiply/divide significands (watch sign) • Normalize result • Normalize • Round • All intermediate results should be in double length storage 7 Floating Floating Point Point Multiplication Division Exercises • Read CH 8, IEEE 754 on IEEE Web site • Email to: [email protected] • Class notes (slides) online at: www.laTech.edu/~choi 8.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages8 Page
-
File Size-