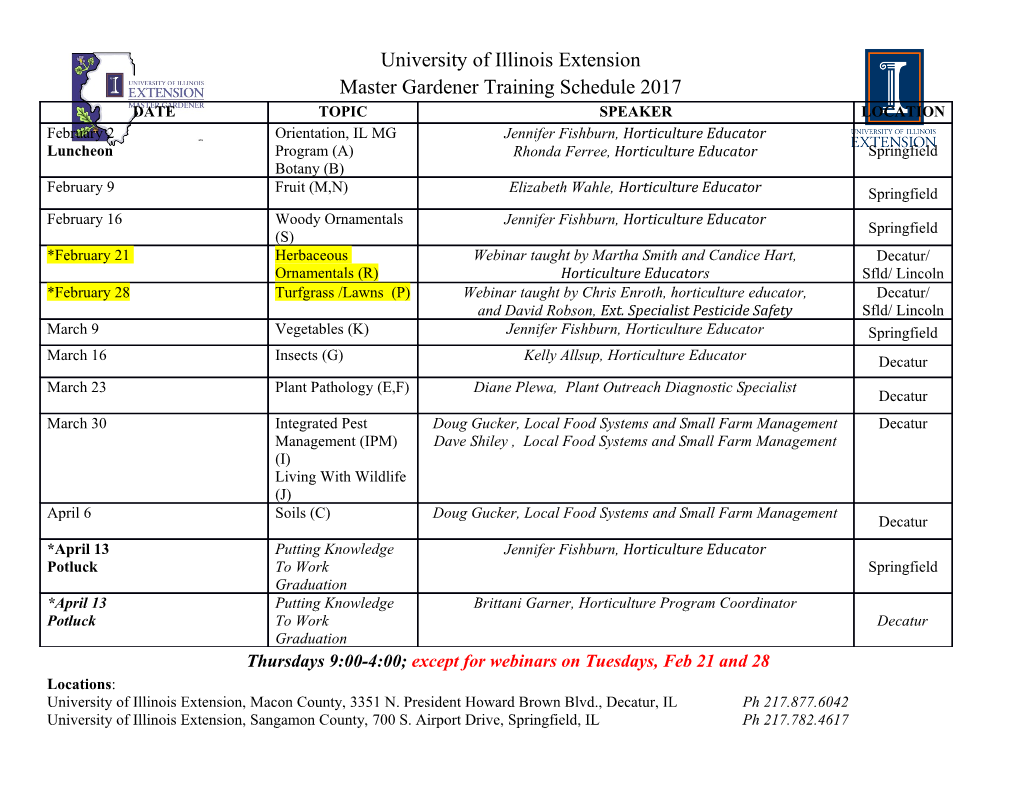
I/O Package Overview Computer Science and Engineering The Ohio State University Package java.io IO Core concept: streams Computer Science and Engineering College of Engineering The Ohio State University Ordered sequences of data that have a source (for input) or a destination (for output) Two major flavors: Lecture 21 Byte streams 8 bits, data-based Input streams and output streams Character streams 16 bits, text-based Readers and writers See Java API documentation for details Byte Streams Example 1: Counting Characters Computer Science and Engineering The Ohio State University Computer Science and Engineering The Ohio State University Two abstract base classes: InputStream and OutputStream import java.io.*; InputStream (for reading bytes) defines: class CountBytes { An abstract method for reading 1 byte at a time public abstract int read() public static void main(String[] args) Returns next byte value (0-255) or -1 if end-of-stream throws IOException { encountered Concrete input stream overrides this method to provide useful InputStream in = new FileInputStream(args[0]); functionality int total = 0; Methods to read an array of bytes or skip a number of bytes OutputStream (for writing bytes) defines: while (in.read() != -1) { An abstract method for writing 1 byte at a time total++; public abstract void write(int b) } Upper 24 bits are ignored Methods to write bytes from a specified byte array in.close(); Close the stream after reading/writing System.out.println(total + ” bytes”); public void close() Frees up limited operating system resources } All of these methods can throw IOException } Standard Streams Example 2: Console Streams Computer Science and Engineering The Ohio State University Computer Science and Engineering The Ohio State University Three standard streams for console IO import java.io.*; class TranslateBytes { System.in public static void main(String[] args) Input from keyboard throws IOException { System.out byte from = (byte)args[0].charAt(0); Output to console byte to = (byte)args[1].charAt(0); System.err int x; Output to error (console by default) while((x = System.in.read()) != -1) These streams are byte streams! System.out.write(x == from ? to : x); } System.in is an InputStream, the others are } PrintStreams (inherit from OutputStream) Would be more logical for these to be character If you run “java TranslateBytes b B” and enter text streams not byte streams, but they predate the bigboy via the keyboard the output will be: BigBoy inclusion of character streams in Java 1 Character Streams Converting Byte/Character Streams Computer Science and Engineering The Ohio State University Computer Science and Engineering The Ohio State University Two abstract base classes: Reader and Writer Translation supported by conversion streams: InputStreamReader and OutputStreamWriter Similar methods to byte stream counterparts Subclasses of Reader and Writer respectively Reader abstract class defines: InputStreamReader public int read() public InputStreamReader(InputStream in) Returns value in range 0..65535 (or -1) public InputStreamReader(InputStream in, String encoding) An encoding is a standard map of characters to bits (eg UTF-16) public int read(char[] cbuf) public int read() Returns number of characters read Reads bytes from associated InputStream and converts them to public void skip(int n) characters using the appropriate encoding for that stream OutputStreamWriter Writer abstract class defines: public OutputStreamWriter(OutputStream out) public void write(int c) public OutputStreamWriter(OutputStream out, String enc) public void write(char[] cbuf) public void write(int c) public abstract void flush() Converts argument to bytes using the appropriate encoding and writes these bytes to its associated OutputStream Ensures previous writes have been sent to destination Closing the conversion stream also closes the associated byte Useful for buffered streams stream – may not always desirable Both classes define: public void close() Working with Files Efficient IO Computer Science and Engineering The Ohio State University Computer Science and Engineering The Ohio State University A file can be identified in one of three ways Buffering greatly improves IO performance A String object (file name) Example: BufferedReader for character input A File object streams A FileDescriptor object public BufferedReader(Reader in) Sequential-Access file: read/write at end of stream only The buffered stream “wraps” the unbuffered FileInputStream, FileOutputStream, FileReader, stream FileWriter Example declarations of BufferedReaders Each file stream type has three constructors An InputStreamReader inside a BufferedReader Random-Access file: read/write at a specified location Reader r = new InputStreamReader(System.in); RandomAccessFile BufferedReader in = new BufferedReader(r); A file pointer is used to guide the starting position A FileReader inside a BufferedReader Not a subclass of any of the four basic IO classes Reader fr = new FileReader(“fileName”); (InputStream, OutputStream, Reader, or Writer) BufferedReader in = new BufferedReader(fr); Supports both input and output Then you can invoke in.readLine() to read from Supports both bytes and characters the stream line by line Example: A Random Access File The File Class Computer Science and Engineering The Ohio State University Computer Science and Engineering The Ohio State University class Filecopy { Useful for retrieving information about a file or a public static void main(String args[]) { RandomAccessFile fh1 = null; directory RandomAccessFile fh2 = null; Represents a path, not necessarily an underlying file try { Does not open/close files or provide file-processing fh1 = new RandomAccessFile(args[0], “r”); capabilities fh2 = new RandomAccessFile(args[1], “rw”); } catch (FileNotFoundException e) { Three constructors System.out.println(“File not found”); public File(String name) System.exit(100); public File(String pathToName, String name) } public File(File directory, String name) try { Main methods int bufsize = (int) (fh1.length())/2; byte[] buffer1 = new byte[bufsize]; boolean canRead() / boolean canWrite() fh1.readFully(buffer1, 0, bufsize); boolean exists() fh2.write(buffer1, 0, bufsize); } catch (IOException e) { boolean isFile() / boolean isDirectory() System.out.println("IO error occurred!"); String getAbsolutePath() / String getPath() System.exit(200); String getParent() } } String getName() } long length() long lastModified() 2 Example Computer Science and Engineering The Ohio State University public class EfficientReader { public static void main (String[] args) { try { Reader fr = new FileReader(args[0]); BufferedReader br = new BufferedReader(fr) String line = br.readLine(); while (line != null) { System.out.println("Read a line:"); System.out.println(line); line = br.readLine(); } br.close(); } catch(FileNotFoundException e) { System.out.println(“File not found: ” + args[0]); } catch(IOException e) { System.out.println(“Can’t read from file: ” + args[0]); } } } 3.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages3 Page
-
File Size-