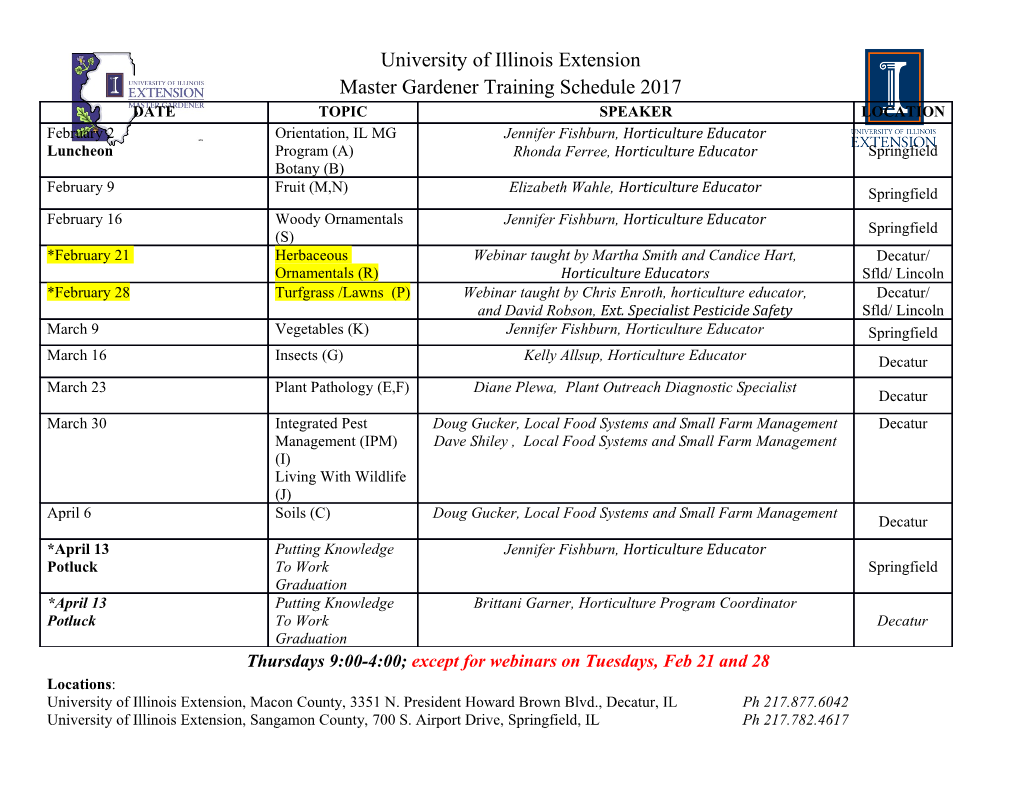
Announcements (1/23/09) CSE 326: Data Structures • HW #2 due now • HW #3 out today, due at beginning of class next Binary Search Trees Friday. • Project 2A due next Wed. night. Steve Seitz Winter 2009 • Read Chapter 4 1 2 ADTs Seen So Far The Dictionary ADT • seitz •Data: Steve • Stack •Priority Queue Seitz –a set of insert(seitz, ….) CSE 592 –Push –Insert (key, value) pairs –Pop – DeleteMin •ericm6 Eric • Operations: McCambridge – Insert (key, value) CSE 218 •Queue find(ericm6) – Find (key) • ericm6 • soyoung – Enqueue Then there is decreaseKey… Eric, McCambridge,… – Remove (key) Soyoung Shin – Dequeue CSE 002 •… The Dictionary ADT is also 3 called the “Map ADT” 4 A Modest Few Uses Implementations insert find delete •Sets • Unsorted Linked-list • Dictionaries • Networks : Router tables • Operating systems : Page tables • Unsorted array • Compilers : Symbol tables • Sorted array Probably the most widely used ADT! 5 6 Binary Trees Binary Tree: Representation • Binary tree is A – a root left right – left subtree (maybe empty) A pointerpointer A – right subtree (maybe empty) B C B C B C • Representation: left right left right pointerpointer pointerpointer D E F D E F Data left right G H D E F pointer pointer left right left right left right pointerpointer pointerpointer pointerpointer I J 7 8 Tree Traversals Inorder Traversal void traverse(BNode t){ A traversal is an order for if (t != NULL) visiting all the nodes of a tree + traverse (t.left); process t.element; Three types: * 5 traverse (t.right); • Pre-order: Root, left subtree, right subtree 2 4 } • In-order: Left subtree, root, right subtree } (an expression tree) • Post-order: Left subtree, right subtree, root 9 10 Binary Tree: Special Cases Binary Tree: Some Numbers… Recall: height of a tree = longest path from root to leaf. A A A A For binary tree of height h: – max # of leaves: B C B C B C B – max # of nodes: D E F D E F G D E F G C Complete Tree Perfect Tree “List” Tree – min # of leaves: H I Full Tree – min # of nodes: 11 12 Binary Tree: Some Numbers… Binary Search Tree Data Structure Recall: depth of a node = path length from node to root. • Structural property – each node has ≤ 2children Consider the space of all possible binary trees of N nodes. – result: 8 • Sum up the depths of every node in that forest and divide by • storage is small the number of nodes. • operations are simple • This is the average depth over all nodes over all binary trees of 5 11 size N. How big is it? • Order property – all keys in left subtree smaller 2 6 10 12 than root’s key What would the average depth be for a well-balanced tree? – all keys in right subtree larger than root’s key 4 7 9 14 – result: easy to find any given key 13 13 14 Example and Counter-Example Find in BST, Recursive Node Find(Object key, 12 Node root) { 5 8 if (root == NULL) return NULL; 5 15 4 8 5 11 if (key < root.key) 2 9 20 return Find(key, root.left); 1 7 11 2 7 6 10 18 else if (key > root.key) 7 10 17 30 return Find(key, root.right); 3 4 15 20 else return root; Runtime: } BINARY SEARCH TREES? 21 15 16 Find in BST, Iterative Bonus: FindMin/FindMax Node Find(Object key, Node root) { • Find minimum 12 12 while (root != NULL && root.key != key) { if (key < root.key) 5 15 5 15 root = root.left; • Find maximum else 2 9 20 2 9 20 root = root.right; } 7 10 17 30 7 10 17 30 return root; } Runtime: 17 18 Insert in BST BuildTree for BST 12 • Suppose keys 1, 2, 3, 4, 5, 6, 7, 8, 9 are inserted into Insert(13) an initially empty BST. 5 15 Insert(8) Insert(31) If inserted in given order, what is the tree? What big-O 2 9 20 runtime for this kind of sorted input? 7 10 17 30 Insertions happen only at the leaves – easy! If inserted in reverse order, Runtime: what is the tree? What big-O runtime for this kind of 19 sorted input? 20 BuildTree for BST Deletion in BST • Suppose keys 1, 2, 3, 4, 5, 6, 7, 8, 9 are inserted into 12 an initially empty BST. 5 15 – If inserted median first, then left median, right median, etc., what is the tree? What is the big-O runtime for this kind of sorted input? 2 9 20 7 10 17 30 Why might deletion be harder than insertion? 21 22 Deletion Deletion – The Leaf Case • Removing an item disrupts the tree structure. • Basic idea: find the node that is to be removed. 12 Then “fix” the tree so that it is still a binary search Delete(17) tree. 5 15 • Three cases: – node has no children (leaf node) 2 9 20 – node has one child – node has two children 7 10 17 30 23 24 Deletion – The One Child Case Deletion – The Two Child Case 12 Delete(15) 12 Delete(5) 5 20 5 15 2 9 30 2 9 20 7 10 7 10 30 What can we replace 5 with? 25 26 Deletion – The Two Child Case Finally… Idea: Replace the deleted node with a value guaranteed to be between the two child subtrees 12 7 replaces 5 Options: 7 20 • succ from right subtree: findMin(t.right) • pred from left subtree : findMax(t.left) 2 9 30 10 Now delete the original node containing succ or pred • Leaf or one child case – easy! Original node containing 7 gets deleted 27 28 Balanced BST Observations • BST: the shallower the better! • For a BST with n nodes – Average depth (averaged over all possible insertion orderings) is O(log n) – Worst case maximum depth is O(n) • Simple cases such as insert(1, 2, 3, ..., n) lead to the worst case scenario Solution: Require a Balance Condition that 1. ensures depth is O(log n) – strong enough! 2. is easy to maintain – not too strong! 29.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages8 Page
-
File Size-