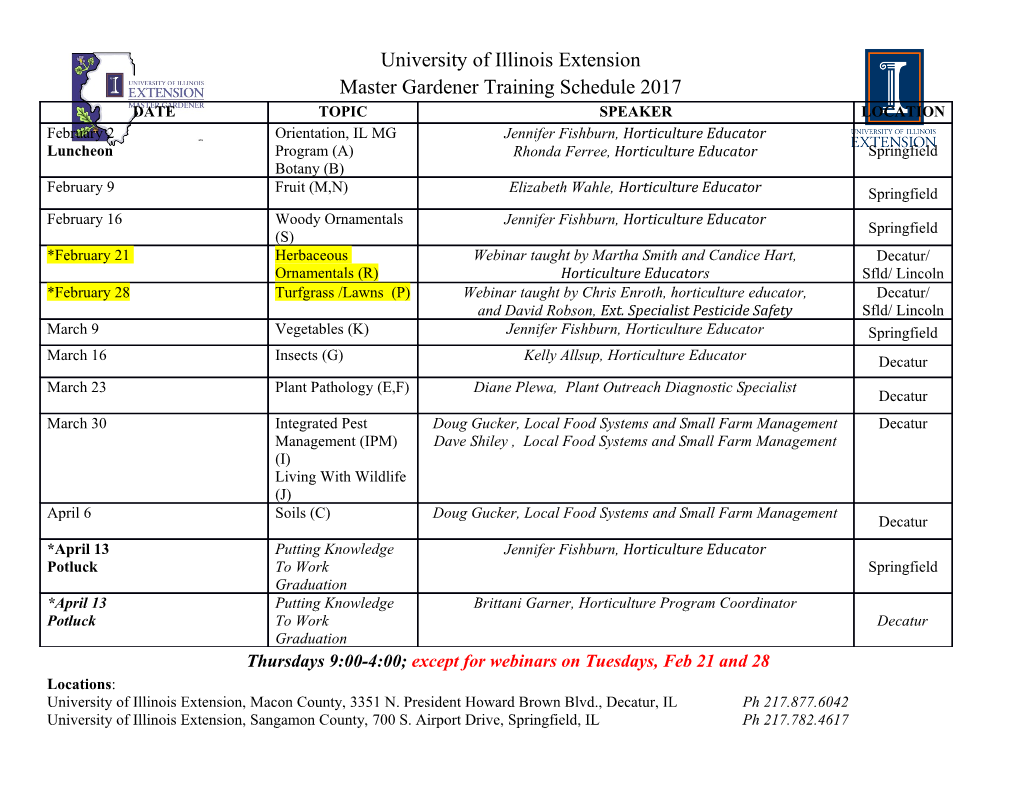
Object Oriented Programming Introduction Object Oriented Programming Introduction to Computer Science II Definition Christopher M. Bourke Objected-Oriented Programming is a programming paradigm that uses [email protected] objects (data structures which have data fields and methods) which interact together within a computer program. Object Oriented Programming Object Oriented Programming Key Ideas Keep in mind When talking about OOP, keep in mind: I Modularity of design I Definitions are often blurred, authors/contexts may differ slightly I Encapsulation of data into Objects I Languages can have some, all, or none OOP features I Abstraction of an object’s behavior and representation I Programs can ignore or utilize some subset of features I Hierarchical organization of objects with subtypes inheriting behavior and state I Non-OOP languages can support some (or all!) OOP features without being “OOP” I Polymorphism ability of objects to have multiple forms in different contexts at different times I Debate still going as to “best” practices, how to do OOP, etc. I Understanding some concepts requires understanding other concepts Object Oriented Programming MotivationI Advantages Consider the evolution of computer languages I Facilitates modeling of real-world agents & problems I Machine Code Assembly Imperative Language I Supports modularity & decomposition → → I Clear demarcation of responsibilities and functionality I Each iteration still forces you to think in terms of a computer’s structure I Separates interface (the what) from implementation (the how) I Other Programming language paradigms force models/problems into I Promotes code reuse their paradigm I Bugs, side-effects, etc. can be localized, less and more predictable testing I Example: LISP assumes that all problems involve lists I Example: C assumes all problems are procedural (algorithmic) MotivationII Contrasting with Other Paradigms I In general, problems are forced into a language’s paradigm I Involves some “translation” or pigeonholing I Most modern programming languages support some OOP features I Good for domain-specific problems or just number-crunching, bad for I Features back-added due to demand or advantages that OOP provides generality I OOP vs. Declarative paradigm I Opposite of what it should be: computers should be serving our needs I OOP vs. Imperative paradigm I Languages should model our world and problems Versus Declarative Versus Imperative Declarative Paradigm Imperative Paradigm I “Declares” behavior of a program I Specifies a specific process (algorithm) that changes a program’s state I Specifies logic of computation, not control flow I Built from one or more procedures (methods, functions) I Functional, avoids state (Lisp, Scheme, Haskell) I Procedural & Structured paradigms (state changes are local or I Logic programming parameterized), not encapsulated I Domain languages: Regular Expressions, HTML, Excel, SQL I Examples: FORTRAN, C I Not necessarily Turing-complete History of OOPI History of OOPII I First compilers simply translated C++ syntax to pure C I Paradigms have “evolved” to OOP I 1983: Brad Cox & Tom Love develop Objective-C I MIT 50s - 60s: LISP “atoms” I Inspired to add OOP features to C from Smalltalk I 1960: Sketchpad (first CAD, revolutionary GUI) by Ivan Sutherland, I 1990s: OOP came to dominate programming methodologies introduced initial concepts (especially due to GUI development) I 1967: Simula 67 by Dahl & Nygaard (bank simulation; agents: I Languages updated to include OOP features tellers, customers, accounts, transactions; physical modeling) I 1995: Java! I 1972: Smalltalk by Alan Kay I 1995 by James Gosling, Sun Microsystems I Based on Plato’s Theory of Forms I “simple, object-oriented and familiar” I Allegory of the Cave I “robust & secure” I “A form is an objective ’blueprint’ of perfection” (an archetype) I “architecture-neutral and portable” I No perfect circle, just shadows, representations of it verything has a I “high performance” quintessence, an archetype, an apotheosis I “interpreted, threaded, dynamic” I 1983: Bjarne Stoustrup develops C++ I Inspired to add OOP features to C from Simula Continued RefinementsI Continued RefinementsII I Design Patterns - a general reusable solution to a common and reoccurring problem in software design (also called idioms) I Anti-patterns and “smells” I Modeling tools (UML – Unified Modeling Language) I Design-by-contract frameworks I Integrated Development Environments (IDEs) I Development of libraries, frameworks I Development of methodologies, best practices, etc. Figure : UML Diagram Example Objects Objects Abstract Data Types I Basis for Objects: Abstract Data Types (ADTs) Definition I Mathematical model for classes of data structures with similar An object is a general entity characterized by the following. behavior or semantics I Purely theoretical entities I Identity – An aspect that distinguishes it from other objects I Generalization applicable to areas other than OOP I State – properties (attributes, elements, data, fields) I Algebraic structures, AI, algorithms, etc. I Behavior – describes an object’s interface (methods, routines, I Examples: stacks, queues, maps, strings, and their operations functions) ObjectsI ObjectsII Key Concepts Key Concepts Message sending Complex Data Types I Objects provide services I Collection of multiple pieces of information I Client code (user) can access these services by sending messages I Mixed types I Example: a signal to execute one of its methods I Varying visibility I A signal to turn a Light object on() or off() I Facilitated by an externally available interface Objects III ObjectsIV Key Concepts Key Concepts Construction Object Design I General approach: decompose an entity to the point where it is I Objects are constructed rather than assigned simple enough to implement or a suitable object already exists I Construction may be specified through constructors I Keep it simple I Assignment through references I Semantics may dictate design I Contrast with primitive types I Avoid the God-Object anti-pattern ObjectsV 4 Main Principles Key Concepts Examples I An animal is a general type Object Oriented Programming involves 4 main principles I Dogs, Cats, Snakes, Lizards are all animals I How can we further develop a taxonomy? 1. Abstraction I Should the following be incorporated? 2. Encapsulation I Pets? 3. Inheritance I Gender? 4. Polymorphism I Strict is-a relationship I Examples: vehicle, automobile, truck, car, boat plane AbstractionI AbstractionII Definition Already familiar with Procedural Abstraction: the implementation of a process is codified into a reusable piece of code inside a function. Abstraction is the mechanism by which objects are given their Example: a square root function may be implemented using various representation and implementation details are hidden. algorithms. Abstraction is apparent in many areas: I Taylor series I Babylonian method I Application layers (database, middle-tier, front-end) I Some other interpolation method I System Layers I Protocol layers (OSI networking model) Abstraction III AbstractionIV Example: A sorting function I Abstraction in OOP takes this further: methods implementations are abstracted, but so are the internal representations of the objects I Selection sort? Quick sort? Other? I Objects are defined with a representation similar to is meaning I Temporary space? (semantics) while hiding implementation details I How does it swap? I Defines the conceptual boundaries of an object, distinguishing between objects In the end: who cares? I Allows the client (programmer, user) to deal with objects based on Procedural abstraction relieves us of the need to worry about their public interface without worrying about details implementation details I It just works! Abstraction in languages Abstraction Example 1 Date/Time A date/time stamp object may be defined to represent a particular point in time (with functionality for formatting, comparing and operating with durations, intervals, other date/time instances). I Abstraction of objects is achieved through its publicly available How could such an object be represented? interface I Interface specifies how you can use the object I A collection of individual numbers (year, month, day, etc.) I Specifies which methods may be signaled by client code I A single numerical value (time from a particular epoch) I May specify a contract I ISO 8601-formatted string (ex: 2012-05-04T12:20:34.000343+01:00) I In conjunction with inheritance: behavior of subtypes may change, be enhanced, etc. Abstraction relieves us of the need to worry about such details I Representation is internal to the object I Consistency and changes are handled by the object I We only interact with its interface Abstraction Example 2 Example 3 Strings Most languages support strings, but may use different representations I A null-terminated character array I A length-prefixed character array A Graph has vertices (nodes) and edges; may be represented as: I Dynamic character array I matrix I ASCII or Unicode? I adjacency list Representing a string as an object relieves us of the need to worry about I maps these details I Contrast with procedural language (C): memory management, consistency, expectations must be handled manually I Instead of worrying about details: just use it! EncapsulationI EncapsulationII Definition I A form of information hiding I In contrast to abstraction which is implementation hiding Encapsulation is a mechanism for restricting access to (some of) an object’s components and for bundling
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages15 Page
-
File Size-