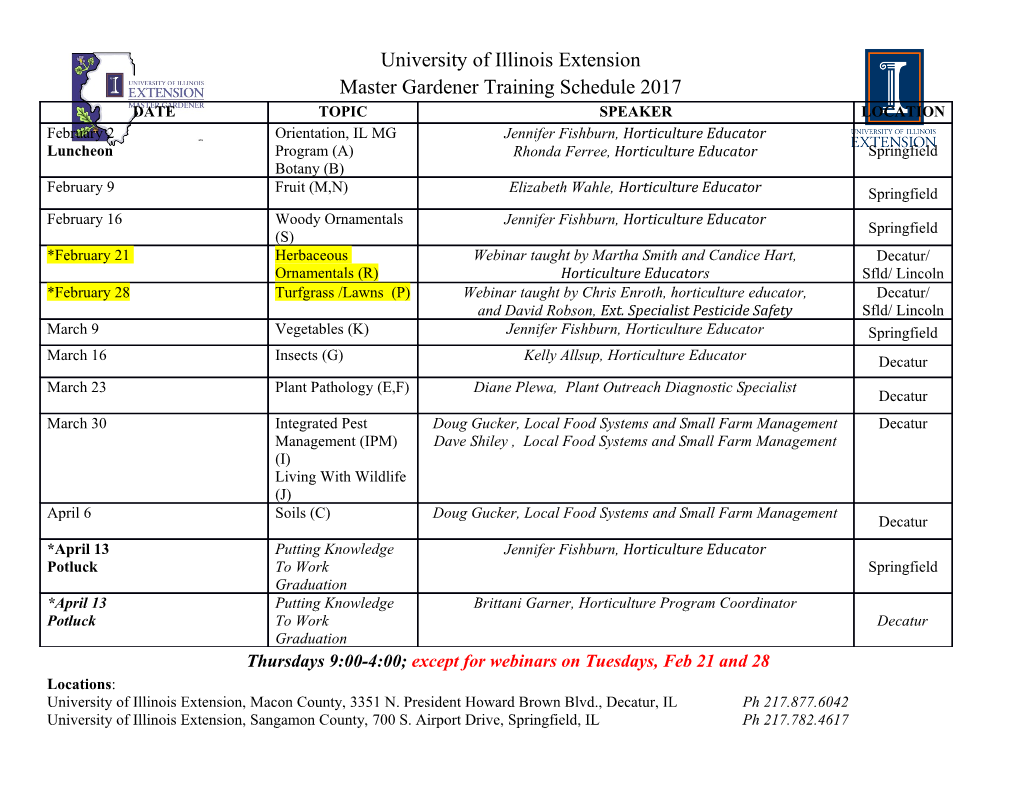
1 New Arithmetic Algorithms for Hereditarily Binary Natural Numbers Paul Tarau Deptartment of Computer Science and Engineering University of North Texas [email protected] Abstract—Hereditarily binary numbers are a tree-based num- paper and discusses future work. The Appendix wraps our ber representation derived from a bijection between natural arithmetic operations as instances of Haskell’s number and numbers and iterated applications of two simple functions order classes and provides the function definitions from [1] corresponding to bijective base 2 numbers. This paper describes several new arithmetic algorithms on hereditarily binary num- referenced in this paper. bers that, while within constant factors from their traditional We have adopted a literate programming style, i.e., the counterparts for their average case behavior, make tractable code contained in the paper forms a Haskell module, avail- important computations that are impossible with traditional able at http://www.cse.unt.edu/∼tarau/research/2014/hbinx.hs. number representations. It imports code described in detail in the paper [1], from file Keywords-hereditary numbering systems, compressed number ∼ representations, arithmetic computations with giant numbers, http://www.cse.unt.edu/ tarau/research/2014/hbin.hs. A Scala compact representation of large prime numbers package implementing the same tree-based computations is available from http://code.google.com/p/giant-numbers/. We hope that this will encourage the reader to experiment inter- I. INTRODUCTION actively and validate the technical correctness of our claims. This paper is a sequel to [1]1 where we have introduced a tree based number representation, called hereditarily binary II. HEREDITARILY BINARY NUMBERS numbers. We will summarize, following [1], the basic concepts behind In [1] we present specialized algorithms for basic arithmetic hereditarily binary numbers. Through the paper, we denote N operations (addition, subtraction, comparison, double, half, the set of natural numbers and N+ the set of strictly positive exponent of 2 and bitsize), that favor numbers with relatively natural numbers. few blocks of contiguous 0 and 1 bijective base-2 digits, for which dramatic complexity reductions result even when A. Bijective base-2 numbers operating on very large, “towers-of-exponents” numbers. Natural numbers can be seen as represented by iterated This paper covers several new arithmetic operations on applications of the functions o(x) = 2x+1 and i(x) = 2x+2 hereditarily binary numbers and some specialized arithmetic corresponding to the so called bijective base-2 representation algorithms that are used in number theory and cryptography, (defined for an arbitrary base in [3] pp. 90-92 as “m-adic” like modular operations and Miller-Rabin and Lucas-Lehmer numbers). Each n 2 can be seen as a unique composition primality tests. N of these functions. We can make this precise as follows: In addition, we perform a performance evaluation confirm- Definition 1: We call bijective base-2 representation of n 2 ing our theoretical complexity estimates of [1] and illustrate the unique sequence of applications of functions o and i to compact representations of some record-holder large prime N 0 that evaluates to n. numbers, while emphasizing that hereditarily binary numbers With this representation, one obtains ; 1 = o(0); 2 = i(0); 3 = replace bitsize with a measure of structural complexity as the o(o(0)); 4 = i(o(0)); 5 = o(i(0)) etc. Clearly: key parameter deciding tractability of arithmetic operations. The paper is organized as follows. Section II overviews i(x) = o(x) + 1 (1) basic definitions for hereditarily binary numbers and sum- marizes some of their properties, following [1]. Section III B. Efficient arithmetic with iterated functions on and in describes new arithmetic algorithms for hereditarily binary Several arithmetic identities are proven in [1] and used to numbers. Section IV compares the performance of several express efficient “one block of on or in operations at a time” algorithms operating on hereditarily binary numbers with algorithms for various arithmetic operations. Among them, their conventional counterparts. Section V illustrates compact we recall the following two, showing the connection of our tree-representations of some record-holder number-theoretical iterated function applications with “left shift/multiplication by entities like Mersenne, Fermat, Proth and Woodall primes. a power of 2” operations. Section VI discusses related work. Section VII concludes the 1An extended draft version of [1] is available at the arxiv repository [2]. on(k) = 2n(k + 1) − 1 (2) 2 in(k) = 2n(k + 2) − 2 (3) III. ARITHMETIC OPERATIONS WORKING ONE ok OR ik BLOCK AT A TIME In particular In [1] we provide algorithms for the basic arithmetic op- on(0) = 2n − 1 (4) erations of addition (add) subtraction (sub), exponent of 2 (exp2), multiplication by a power of 2, division by a power of 2 (leftshiftBy, rightshiftBy) arithmetic compari- in(0) = 2n+1 − 2 (5) son (cmp) and computation of the size of the equivalent binary representation of a number, bitsize. We will describe in this section algorithms for several other arithmetic operations, C. Hereditarily binary numbers as a data type optimized for working one block of ok or ik applications at First we define a data type for our tree represented natural a time. numbers, that we call hereditarily binary numbers to empha- size that binary rather than unary encoding is recursively used General multiplication in their representation. Like in the case of add and sub operations in [1] we can Definition 2: The data type T of the set of hereditarily binary numbers is defined in [1] by the Haskell declaration: derive a multiplication algorithm based on several arithmetic data T = E | V T [T] | W T [T] identities involving exponents of 2 and iterated applications of deriving (Eq,Read,Show) the functions o and i. corresponding to the recursive data type equation T = 1 + T × Proposition 1: The following holds: T∗ + T × T∗. on(a)om(b) = on+m(ab + a + b) − on(a) − om(b) (7) The intuition behind the type T is the following: • The term E (empty leaf) corresponds to zero Proof: By (2), we can expand and then reduce: n m n m • the term V x xs counts the number x+1 of o applica- o (a)o (b) = (2 (a + 1) − 1)(2 (b + 1) − 1) = n+m n m tions followed by an alternation of similar counts of i 2 (a + 1)(b + 1) − (2 (a + 1) + 2 (b + 1)) + 1 = n+m n m and o applications xs 2 (a+1)(b+1)−1−(2 (a+1)−1+2 (b+1)−1+2)+2 = n+m n m • the term W x xs counts the number x+1 of i applica- o (ab + a + b + 1) − (o (a) + o (b)) − 2 + 2 = n+m n m tions followed by an alternation of similar counts of o o (ab + a + b) − o (a) − o (b) and i applications xs Proposition 2: n m n+m n+1 m+1 In [1] the bijection between N and T is provided by the i (a)i (b) = i (ab+2(a+b+1))+2−i (a)−i (b) function n : T ! N and its inverse t : N ! T. (8) Definition 3: The function n : T ! N shown in equation Proof: By (3), we can expand and then reduce: (6) defines the unique natural number associated to a term of in(a)im(b) = (2n(a + 2) − 2)(2m(b + 2) − 2) = type T. 2n+m(a + 2)(b + 2) − (2n+1(a + 2) − 2 + 2m+1(b + 2) − 2) = n+m n+1 m+1 This bijection ensures that hereditarily binary numbers provide 2 (a + 2)(b + 2) − i (a) − i (b) = n+m n+1 m+1 a canonical representation of natural numbers and the equality 2 (a + 2)(b + 2) − 2 − (i (a) + i (b)) + 2 = n+m n+1 m+1 relation on type T can be derived by structural induction. 2 (ab + 2a + 2b + 2 + 2) − 2 − (i (a) + i (b)) + 2 = n+m n+1 m+1 The following examples show the workings of the bijection i (ab + 2a + 2b + 2) − (i (a) + i (b)) + 2 = n+m n+1 m+1 n and illustrate that “structural complexity”, defined in [1] i (ab + 2(a + b + 1)) + 2 − i (a) − i (b) as the size of the tree representation without the root, is The corresponding Haskell code starts with the obvious base bounded by the bitsize of a number and favors numbers in cases: the neighborhood of towers of exponents of 2. mul _ E = E 16 22 − 1 ! V (V (V (V (V E[])[])[])[])[] mul E _ = E 20+1−1+1 22 −1+1−1+1 ! 22 −1+1 − 1 When both terms represent odd numbers we apply the identity 20 ! W E [E,E,E] (7): ! (((20+1 − 1 + 2)20+1 − 2 + 1)20+1 − 1 + 2)20+1 − 2 mul x y j o_ x && o_ y = r2 where (n,a) = osplit x In [1] basic arithmetic operations are introduced with com- (m,b) = osplit y plexity parameterized by the size of the tree representation of p1 = add (mul a b) (add a b) their operands rather than their bitsize. p2 = otimes (add (s n) (s m)) p1 r1 = sub p2 x After defining constant average time successor and prede- r2 = sub r1 y cessor functions s and s’, constant average time definitions of o and i are given in [1], as well as for the corresponding Note that the function osplit defined in [1] is used to inverse operations o’ and i’, that can be seen as “un- separate the first block of o applications. The next two cases applying” a single instance of o or i, and “recognizers” e_ are reduced to the previous one by using the identity i = s◦o.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages11 Page
-
File Size-