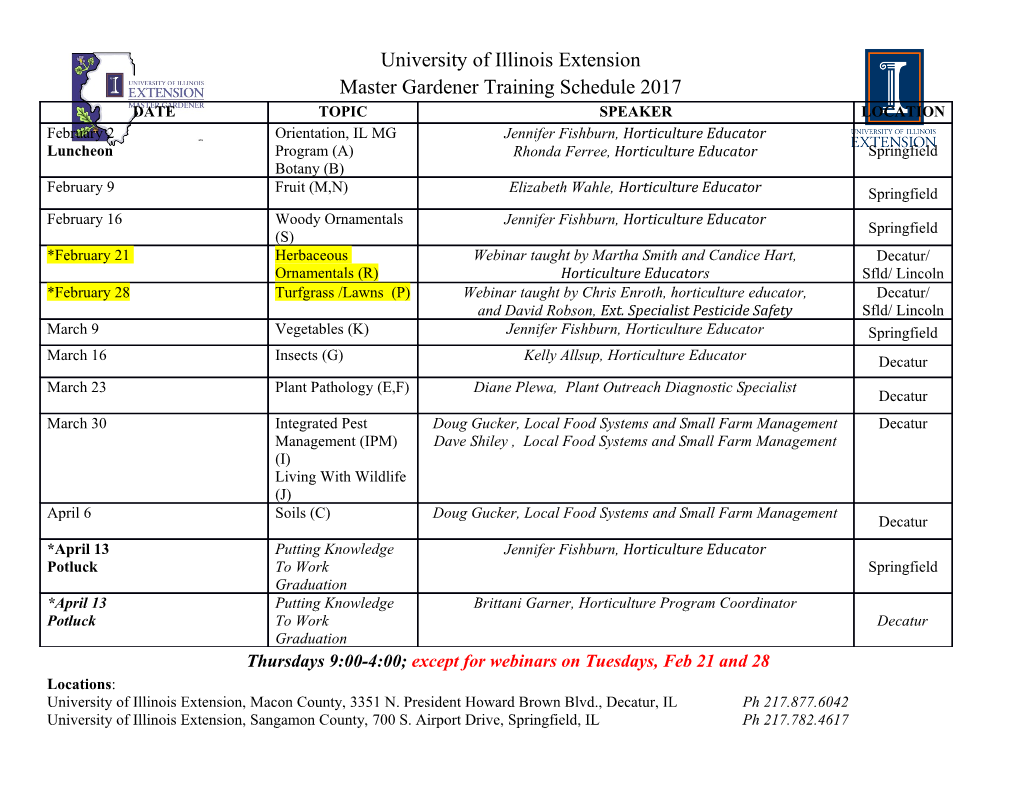
L07: Circuit Building Blocks I CSE369, Winter 2017 Intro to Digital Design Circuit Building Blocks I Instructor: Justin Hsia L07: Circuit Building Blocks I CSE369, Winter 2017 Practice: String Recognizer FSM Recognize the string 101 with the following behavior . Input: 1 0 0 1 0 1 0 1 1 0 0 1 0 . Output: 0 0 0 0 0 1 0 1 0 0 0 0 0 State diagram to implementation: 2 L07: Circuit Building Blocks I CSE369, Winter 2017 Administrivia Lab 7 – Useful Components . Modifying Lab 6 game to implement common circuit elements • Counter, Shift Registers covered later in lecture . Build a tunable computer opponent . Bonus points for smaller resource usage Quiz 2 is next week in lecture . First 25 minutes, worth 11% of your course grade . On Lectures 4‐6: Sequential Logic, Timing, FSMs, and Verilog . Au16 Quiz 2 (+ solutions) on website: Files Quizzes 3 L07: Circuit Building Blocks I CSE369, Winter 2017 Motivating Example Problem: Implement a simple pocket calculator Need: . Display: Seven segment displays . Inputs: Switches . Math: Arithmetic & Logic Unit (ALU) 4 L07: Circuit Building Blocks I CSE369, Winter 2017 Data Multiplexor Multiplexor (“MUX”) is a selector . Direct one of many ( = s) ‐bit wide inputs onto output . Called a ‐bit, ‐to‐1 MUX Example: ‐bit 2‐to‐1 MUX . Input S ( bits wide) selects between two inputs of bits each This input is passed to output if selector bits inputs match shown value 5 L07: Circuit Building Blocks I CSE369, Winter 2017 Review: Implementing a 1‐bit 2‐to‐1 MUX Schematic: Boolean Algebra: sabc Truth Table: 0 0 00 0 0 10 Circuit Diagram: 0 1 01 0 1 11 100 0 101 1 110 0 111 1 6 L07: Circuit Building Blocks I CSE369, Winter 2017 1‐bit 4‐to‐1 MUX Schematic: Truth Table: How many rows? 26 Boolean Expression: e = s1’s0’a + s1’s0b + s1s0’c + s1s0d 7 L07: Circuit Building Blocks I CSE369, Winter 2017 1‐bit 4‐to‐1 MUX Can we leverage what we’ve previously built? . Alternative hierarchical approach: 8 L07: Circuit Building Blocks I CSE369, Winter 2017 Multiplexers in General Logic Implement with a 8:1 MUX 0 1 2 3 4 8:1 5 MUX 6 7 S0 S1 S2 9 L07: Circuit Building Blocks I CSE369, Winter 2017 Review: Unsigned Integers Unsigned values follow the standard base 2 system . In bits, represent integers to Add and subtract using the normal “carry” and “borrow” rules, just in binary 63 00111111 64 01000000 +8 +00001000 -8 -00001000 71 01000111 56 00111000 10 L07: Circuit Building Blocks I CSE369, Winter 2017 Review: Two’s Complement (Signed) b has weight 2 , other bits have usual weights 2 bw‐1 bw‐2 . b0 Properties: . In bits, represent integers to . Positive number encodings match –1 + 0 unsigned numbers –2 1111 0000 + 1 –3 1110 0001 + 2 . Single zero (encoding = all zeros) 1101 0010 –4 + 3 1100 0011 Negation procedure: Two’s Complement . Take the bitwise complement –5 1011 0100 + 4 and then add one 1010 0101 –6 + 5 ( ~x + 1 == -x ) 1001 0110 –7 1000 0111 + 6 –8 + 7 11 L07: Circuit Building Blocks I CSE369, Winter 2017 Addition and Subtraction in Hardware The same bit manipulations work for both unsigned and two’s complement numbers! . Perform subtraction via adding the negated 2nd operand: 4‐bit examples: Two’s Un Two’s Un 0010 +2 2 1000 -8 8 +1100 -412 +0100 +4 4 0110 +6 6 1111 -115 -0010 +2 2 -1110 -214 12 L07: Circuit Building Blocks I CSE369, Winter 2017 Half Adder (1 bit) Carry Sum Carry‐out bit a0 b0 c1 s0 0000 0101 1001 1110 13 L07: Circuit Building Blocks I CSE369, Winter 2017 Full Adder (1 bit) Possible carry‐in c1 Carry‐in Carry‐out ci ai bi ci+1 si 00000 00101 01001 01110 10001 10110 11010 11111 14 L07: Circuit Building Blocks I CSE369, Winter 2017 Multi‐Bit Adder ( bits) Chain 1‐bit adders by connecting CarryOuti to CarryIni1: b0 + + + 15 L07: Circuit Building Blocks I CSE369, Winter 2017 Subtraction? Can we use our multi‐bit adder to do subtraction? . Flip the bits and add 1? • X⊕1X • CarryIn0 (using full adder in all positions) b0 + + + 16 L07: Circuit Building Blocks I CSE369, Winter 2017 Multi‐bit Adder/Subtractor ⊕1̅ Add 1 (flips the bits) +++ This signal is only high when you perform subtraction 17 L07: Circuit Building Blocks I CSE369, Winter 2017 Detecting Arithmetic Overflow Overflow: When a calculation produces a result that can’t be represented in the current encoding scheme . Integer range limited by fixed width . Can occur in both the positive and negative directions Signed Overflow . Result of add/sub is > TMax or < TMin . ( ) + ( ) = ( ) or ( ) + ( ) = ( ) 18 L07: Circuit Building Blocks I CSE369, Winter 2017 Signed Overflow Examples Two’s Two’s 0101 +5 1001 -7 +0011 +3 +1110 -2 Two’s Two’s 0101 +5 1101 -3 +0010 +2 +1011 -5 19 L07: Circuit Building Blocks I CSE369, Winter 2017 Multi‐bit Adder/Subtractor with Overflow +++ 20 L07: Circuit Building Blocks I CSE369, Winter 2017 Arithmetic and Logic Unit (ALU) Most processors contain a special logic block called the “Arithmetic and Logic Unit” (ALU) . Here’s an easy one that does ADD, SUB, bitwise AND, and bitwise OR (for 32‐bit numbers) Schematic: when S=00, R = A+B when S=01, R = A–B when S=10, R = A&B when S=11, R = A|B L07: Circuit Building Blocks I CSE369, Winter 2017 Simple ALU Schematic Notice that 3 values are ALWAYS calculated in parallel, but only 1 makes it to the Result L07: Circuit Building Blocks I CSE369, Winter 2017 1‐bit Adders in Verilog What’s wrong with this? module halfadd1 (s, a, b); output reg s; . Truncation! input a, b; always @(*) begin s = a + b; end endmodule Fixed: module halfadd2 (c, s, a, b); output reg c, s; . Use of {sig, …, sig} input a, b; for concatenation always @(*) begin {c, s} = a + b; end endmodule 23 L07: Circuit Building Blocks I CSE369, Winter 2017 Ripple‐Carry Adder in Verilog module fulladd (cout, s, cin, a, b); output reg cout, s; input cin, a, b; always @(*) begin {cout, s} = cin + a + b; end endmodule Chain full adders? module add2 (cout, s, cin, a, b); output cout; output [1:0] s; input cin; input [1:0] a, b; wire c1; fulladder b1 (cout, s[1], c1, a[1], b[1]); fulladder b0 (c1, s[0], cin, a[0], b[0]); endmodule 24 L07: Circuit Building Blocks I CSE369, Winter 2017 Add/Sub in Verilog (parameterized) Variable‐width add/sub (with overflow, carry) module addN #(parameter N=32) (OF, CF, S, sub, A, B); output reg OF, CF; output reg [N-1:0] S; input sub; input [N-1:0] A, B; reg [N-1:0] D; // possibly flipped B always @(*) begin D = B ^ {N{sub}}; // replication operator {CF, S} = A + D + sub; OF = CF ^ S[N-1]; end endmodule . Here using OF = overflow flag, CF = carry flag • From condition flags in x86‐64 processors . {n{m}} is replication operator – repeats value m, n times 25 L07: Circuit Building Blocks I CSE369, Winter 2017 Add/Sub in Verilog (parameterized) module addN_testbench (); parameter N = 4; reg sub; reg [N-1:0] A, B; wire OF, CF; wire [N-1:0] S; addN #(.N(N)) dut (.OF, .CF, .S, .sub, .A, .B); initial begin #100; sub = 0; A = 4'b0101; B = 4'b0010; // 5 + 2 #100; sub = 0; A = 4'b1101; B = 4'b1011; // -3 + -5 #100; sub = 0; A = 4'b0101; B = 4'b0011; // 5 + 3 #100; sub = 0; A = 4'b1001; B = 4'b1110; // -7 + -2 #100; sub = 1; A = 4'b0101; B = 4'b1110; // 5 -(-2) #100; sub = 1; A = 4'b1101; B = 4'b0101; // -3 - 5 #100; sub = 1; A = 4'b0101; B = 4'b1101; // 5 -(-3) #100; sub = 1; A = 4'b1001; B = 4'b0010; // -7 - 2 #100; end endmodule 26.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages26 Page
-
File Size-