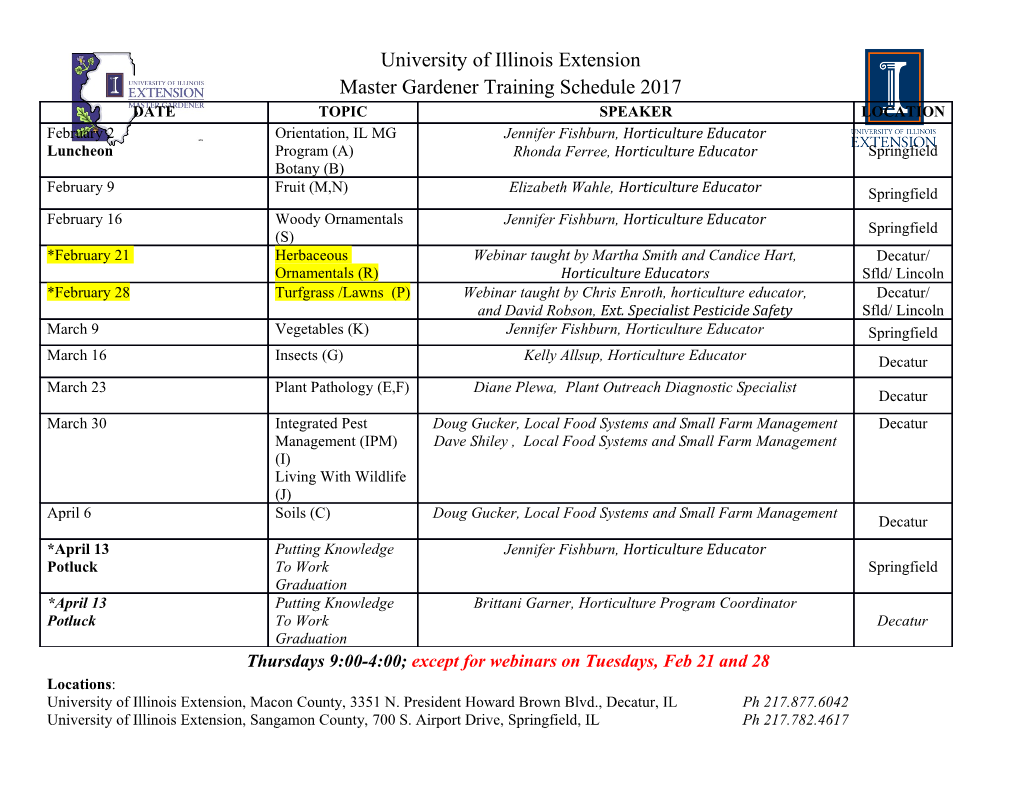
<p>CONCEPT EXERCISES</p><p>10.1 a. Show the effect of making the following insertions into an initially empty binary search tree:</p><p>30, 40, 20, 90, 10, 50, 70, 60, 80</p><p>30</p><p>20 40</p><p>10 90</p><p>50</p><p>70</p><p>60 80 </p><p> b. Find a different ordering of the above elements whose insertions would generate the same binary search tree as in part a.</p><p>30, 20, 40, 10, 90, 50, 70, 80, 60 10.2 Describe in English how to remove each of the following from a binary search tree:</p><p> a. an element with no children</p><p>Simply remove the element and remove the corresponding subtree-link from the element’s parent (if the element is not the root).</p><p> b. an element with one child</p><p>Replace the parent-element link and the element-child link with a parent-child link.</p><p> c. an element with two children</p><p>Copy the element’s immediate successor into element, and then remove that immediate successor (by part a or part b).</p><p>10.3 a. For any positive integer n, describe how to arrange the integers 1, 2, …, n so that when they are inserted into a BinarySearchTree object, the height of the tree will be linear in n. </p><p>Arrange the elements in increasing order, for example (or in decreasing order).</p><p> b. For any positive integer n, describe how to arrange the integers 1, 2, …, n so that when they are inserted into a BinarySearchTree object, the height of the tree will be logarithmic in n. </p><p>The first element should be n / 2. Then half of the remaining elements will be in the left subtree, and half in the right subtree. The next element in the arrangement can be n / 4. Then half of the remaining elements in the left subtree of the whole tree will be in the left subtree of n / 4. The next element in the arrangement can be 3n / 4. The arrangement is</p><p> n / 2, n / 4, 3n / 4, n / 8, 3n / 8, 5n / 8, 7n / 8, n / 16, 3n / 16, … c. For any positive integer n, describe how to arrange the integers 1, 2, …, n so that when they are inserted into an AVLTree object, the height of the tree will be logarithmic in n. </p><p>Any arrangement will do, because the height of an AVL tree is always logarithmic in n.</p><p> d. For any positive integer n, is it possible to arrange the integers 1, 2, …, n so that when they are inserted into an AVLTree object, the height of the tree will be linear in n? Explain.</p><p>No, because the height of an AVL tree is always logarithmic in n.</p><p>10.4 In each of the following binary search trees, perform a left rotation around 50.</p><p> a. 50</p><p>60</p><p>70</p><p>60</p><p>50 70</p><p> b. 30</p><p>20 50</p><p>40 80 70 100</p><p>30</p><p>20 80</p><p>50 100</p><p>40 70 </p><p> c. 30</p><p>20 50</p><p>40 80</p><p>45 70 100</p><p>60 75 30</p><p>20 80</p><p>50 100</p><p>40 70</p><p>45 60 75 </p><p>10.5 In each of the following binary search trees, perform a right rotation around 50.</p><p> a. 50</p><p>40</p><p>30</p><p>40</p><p>30 50 b. 60</p><p>50 70</p><p>40 55</p><p>30 45</p><p>60</p><p>40 70</p><p>30 50</p><p>45 55 c. 30</p><p>20 50</p><p>40 80</p><p>48 60 100 55 75</p><p>30</p><p>20 40</p><p>50</p><p>48 80</p><p>60 100</p><p>55 75</p><p>10.6 In the following binary search tree, perform a double rotation (a left rotation around 20 and then a right rotation around 50) to reduce the height to 2.</p><p>50</p><p>20 90</p><p>10 40</p><p>30 (after the left rotation around 20)</p><p>50</p><p>40 90</p><p>20 </p><p>10 30</p><p>(after the right rotation around 50)</p><p>40</p><p>20 50 </p><p>10 30 90</p><p>10.7 In the following binary search tree, perform a "double rotation" to reduce the height to 2:</p><p>50</p><p>20 80</p><p>70 100 60</p><p>(after the right rotation around 80)</p><p>50</p><p>20 70 </p><p>60 80</p><p>100</p><p>(after the left rotation around 50)</p><p>70</p><p>50 80 </p><p>20 60 100</p><p>10.9 Suppose we define maxh to be the maximum number of elements in an AVL tree of height h.</p><p> a. Calculate max3.</p><p>The maximum number of elements will be attained for a full AVL 3 tree. By the Binary Tree Theorem, max3 = 2 * 2 – 1 = 15.</p><p> b. Determine the formula for maxh for any h >= 0. Hint: Use the Binary Tree Theorem from Chapter 9.</p><p> h+1 maxh = 2 – 1 c. What is the maximum height of an AVL tree with 100 elements?</p><p>Since min9 = fib (12) – 1 = 143, the maximum height of an AVL tree with 100 elements must be less than 9. Since min8 = fib (11) – 1 = 88, the maximum height of an AVL tree with 100 elements is 8.</p><p>10.10 Show that the height of an AVL tree with 32 elements must be exactly</p><p>5. Hint: calculate max4 and min6.</p><p>Since max4 = 31, the maximum number of elements in any AVL tree of height 4 is 31. Since min6 = fib (9) – 1 = 33, the minimum number of elements in an AVL tree of height 6 is 33. So any AVL tree with 32 elements must have height 5.</p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages10 Page
-
File Size-