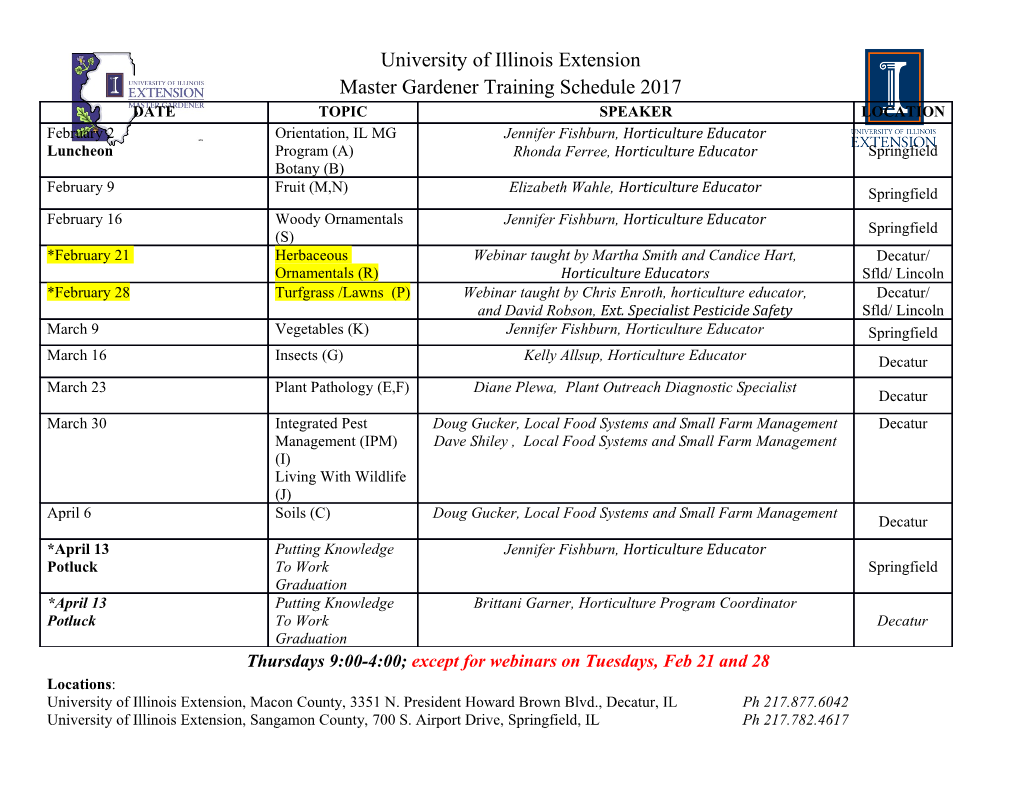
<p>NAME: ______CS1043 In Class Exercise</p><p>Make a class called Employee with instance variables name and salary. The Employee class should have one constructor that sets the name and salary. The Employee class should have a display method to print the current state of the instance variables (name it display). Make a class called Manager inherit from Employee. Add an instance variable, named department of type String to the Manager class. Include a constructor for the Manager class that sets the name, salary, and department. Supply an accessor method that prints the manager’s name, department, and salary (name it display).</p><p>Note: all instance variables (instance fields) must be declared as private. NAME:______CS1043 Inheritance and Polymorphism In-Class Exercise</p><p>Look at the main program on the next page. Trace the program and determine what is printed by each print statement. Use the BankAccount Class posted on the course website. /* Date: 10-05-06 Language Version: Java2 1.5.0_06 Course: CS1043 Section: 1 and 2 File Name: AcctExer.java Classes: AcctExer</p><p>Description: This program is an exercise for Inheritance and Polymorphism. Label each method call as either: 1. Polymorphic, 2. Inheritance, or 3. Overloading. */ public class AcctExer { public static void main( String [] args ) { TimeDepositAccount collegeFund = new TimeDepositAccount( 1, 4 );</p><p>CheckingAccount harrysChecking = new CheckingAccount( 1000. );</p><p> collegeFund.deposit( 5000.);</p><p> collegeFund.transfer( harrysChecking, 580. );</p><p> harrysChecking.withdraw( 500. ); harrysChecking.withdraw( 80. ); harrysChecking.withdraw( 10000. );</p><p> endOfMonth( collegeFund ); endOfMonth( harrysChecking );</p><p> printBalance( "the college fund", collegeFund ); printBalance( "Harry's checking", harrysChecking );</p><p>} // end main</p><p> public static void endOfMonth( SavingsAccount savings ) { savings.addInterest(); }</p><p> public static void endOfMonth( CheckingAccount checking ) { checking.deductFees(); }</p><p> public static void printBalance( String name, BankAccount account ) { System.out.printf("The balance of the %s account is $%.2f.\n", name, account.getBalance() ); }</p><p>} // end AcctExer </p>
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages4 Page
-
File Size-