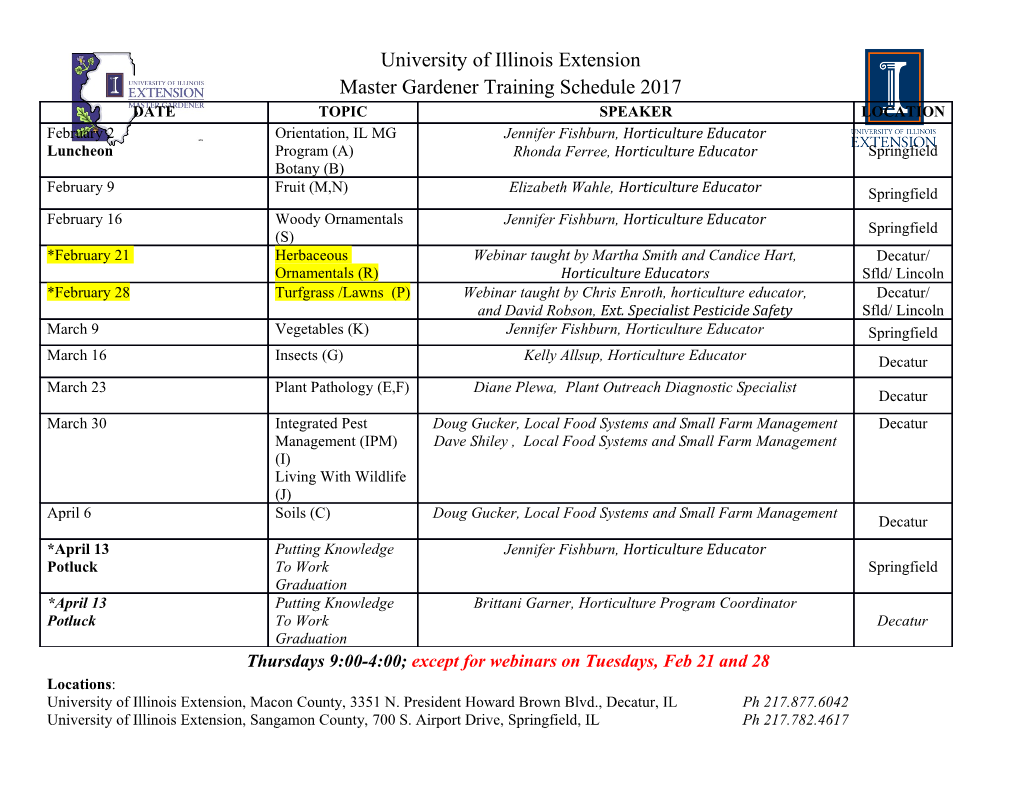
Computer Programming Decision Making (2) Loops Topics • The Conditional Execution of C Statements (review) • Making a Decision (review) • If Statement (review) • Switch-case • ? operator • Repeating Statements • while loop • Examples Conditional Execution of Statements (Review from last week) A Statement defines an action to be carried out. However, you may want to carry out the action on certain conditions. e.g. If b is not zero then compute a/b if D is not negative then find its square root Conditions A conditional expression is executed only if the given condition is true. A condition is expressed as a logical expression. e.g. Verify if the value in variable X is even or not The value in variable X is even if it is divisible by 2 so the condition will be: X%2==0 Decision Making To carry out a statement conditionally, a decision should be made. Decision making in C is done by if-else statement if( condition ) [The statements carried out when condition is true] else [The statements carried out when condition is false] Example Read an integer and check if it is positive and divisible by 5 or not. Print a proper message in each case #include<stdio.h> void main() { int num; scanf(“%d”,&num); if( num % 5 ==0 && num >= 0 ) printf(“The condition was true\n”); else printf(“The condition was false\n”); } Decision Making using Switch-Case If an integer or a character variable is tested against several constant values we can use switch-case statement for decision making. Example: The example below can be written with switch-case int num; scanf(“%d”,&num); if(num == 10) printf(“Excellent \n”); else if(num == 9) printf(“Very good \n”); else if(num == 8) printf(“Good \n”); else if(num == 7) printf(“Average \n”); else printf(“Weak \n”); When Can We Use switch-case The variable should be integer or character Compare for equality only ( only ==, we cannot use !=,>, >=, <, or <= with switch-case) The condition is not a compound condition (AND, OR are not used to combine simple logical expressions ) Switch-Case Statements The structure of a switch-case statement is as below: switch(variable) { case value1: [statements if variable==value1]; break; // exit switch-case case value2: [statements if variable==value2]; break; // exit switch-case ………… default: [ statements if variable is not equal to any value given is cases] } Example #include<stdio.h> void main() { int grade; scanf(“%d”,&grade); switch(grade) { case 10: printf(“Excellent\n”); break; case 9: printf(“Very Good\n”); break; case 8: printf(“Good\n”); break; case 7: printf(“Average\n”); break; default: printf(“Weak\n”); } } Break Statement Break statement makes the program exit the switch-case statement. Default part is the last part of switch-case, so we do not need a break in default part. If a case does not have a break statement, the next case is also executed. This can be used to OR two cases. Example Using switch-case statement write a program to read an integer number greater than 1 and less than 10 and do the followings: If the number is 2,4, 6, or 8 print “EVEN” If the number is 3, 5, or 7 print “PRIME” If the number is 9 print “ODD” Otherwise print “Out of Range” #include<stdio.h> void main() { int num; scanf(“%d”,&num); switch(num) { case 2: case 4: case 6: case 8: printf(“EVEN\n”); break; case 9: printf(“ODD\n”); break; case 3: case 5: case 7: printf(“PRIME\n”); break; default: printf(“Out of Range\n”); } } Default Default is executed when the variable does not have any of the values in case parts In fact it can be assumed as “if non of the above values then..” Default part of switch-case statements is optional (same as else in if statements) ? Operator ? Operator is a short form for if statements Syntax: Condition? True part : false part True or false parts return a value, so it can be used in assignment statements Example: int large, a,b; scanf(“%d%d”,&a,&b); large = a>b ? a : b; Nested ? operators ? Operator can be nested in another ? Statement The syntax then will be: Condition ? [Nested ?] : false part Condition ? True part : [Nested ?] Condition ? [Nested ?] : [Nested ?] Example Read three integer numbers and choose the largest one. int large, a, b, c; scanf(“%d%d%d”,&a,&b,&c); large = a>b && a>c ? a : b>c ? b : c ; printf(“ The largest number was %d\n”, large); Repeating Statements In many cases same operations are repeated for different data. For example, finding average of a student is repeated for other students too. In these cases, we can use a statement called a loop The statements inside a loop are repeated until its condition becomes false. C uses three types of loops: While loop Do-while loop (will be discussed next week) For loop (will be discussed next week) While Loop While loop repeats a group of C statements until its condition becomes false While loop has the following syntax: while(condition) [statements]; Condition is a logical expression Statements inside the while loop are called body of while loop If more than one statement is written inside body of a while loop, they should be put in {} Example 1 Write a program to print all positive even integers less than 100. The output should be like: 2 4 6 ….. 96 98 #include<stdio.h> void main() { int count; count = 2; while( count < 100 ) { printf(“%d\n”, count); count = count + 2; } } Example 2 Re-write the calculator example to find the results until -1 is entered as the value of both operands. (e.g. ends when -1 / -1 is entered) Calculator This example is a simple calculator which can perform four operations: +, - , * , / The program reads two numbers and a character. The character defines the operation. The character can be ‘*’ , ‘-’, ‘/’, or ‘+’ The program carries out the operation and prints the result #include<stdio.h> void main() { float num1, num2, result; char op; printf(“Enter the expression:\n”); scanf(“%f%c%f”,&num1,&op,&num2); while( num1 != -1 || num2 != -1 ) { if( op == ‘+’ ) { result = num1+num2; printf(“ The result is %f\n”, result); } else if( op == ‘-’ ) { result = num1-num2; printf(“ The result is %f\n”, result); } else if( op == ‘*’ ) { result = num1*num2; printf(“ The result is %f\n”, result); } else if( op == ‘/’ ) { if( num2 != 0 ) { result = num1/num2; printf(“ The result is %f\n”, result); } else printf(“Oops !!! Division by zero\n”); } else /* if the operator is not one of the 4 valid operators*/ printf(“ Invalid operator entered!!! \n”); /* read next expression */ printf(“Enter the expression:\n”); scanf(“%f%c%f”,&num1,&op,&num2); } /* end of while loop is here */ } Example 3 Write a C program to read three grades of each student and find his/her average. The first grade is midterm exam grade, the second grade is final exam grade, and the third grade is assignment grade. The weight of each grade is: Midterm 30% Assignment 25% Final 45% Repeat finding average for 20 students #include<stdio.h> void main() { float midterm, assignment, final, avg; int count; count = 0; while(count < 20) { printf(“Enter the midterm, assignment, and final grade \n”); scanf(“%f%f%f”, &midterm, &assignment, &final); avg=midterm *0.3+assignmenrt*0.25+final*0.45; printf(“The average is %6.2f\n”,avg); count=count+1; } } Example 4 Write a C program to read a positive integer and find sum of all positive integers up to and including that number. Hint: Make sure that the number is positive #include<stdio.h> void main() { int num, sum; int count; count = 1; sum = 0; printf(“Enter a positive integer \n”); scanf(“%d”, &num); if( num < 0 ) printf(“ You entered a negative number\n”); else { while(count <= num) { sum=sum+count; count=count+1; } printf(“The sum is %d \n”,sum); } } Summary Some statements in a C program are executed conditionally If the condition is a simple logical statement testing an integer or character variable using equality (==), switch- case statements can be used If parts of a program are repeated with different values, we can use a loop while loop repeats executing the statements until the condition becomes false Questions?.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages30 Page
-
File Size-