C++ Object Oriented Multiple Inheritance Duplicated Member Method Parameterized Type Class and Function Templates
Total Page:16
File Type:pdf, Size:1020Kb
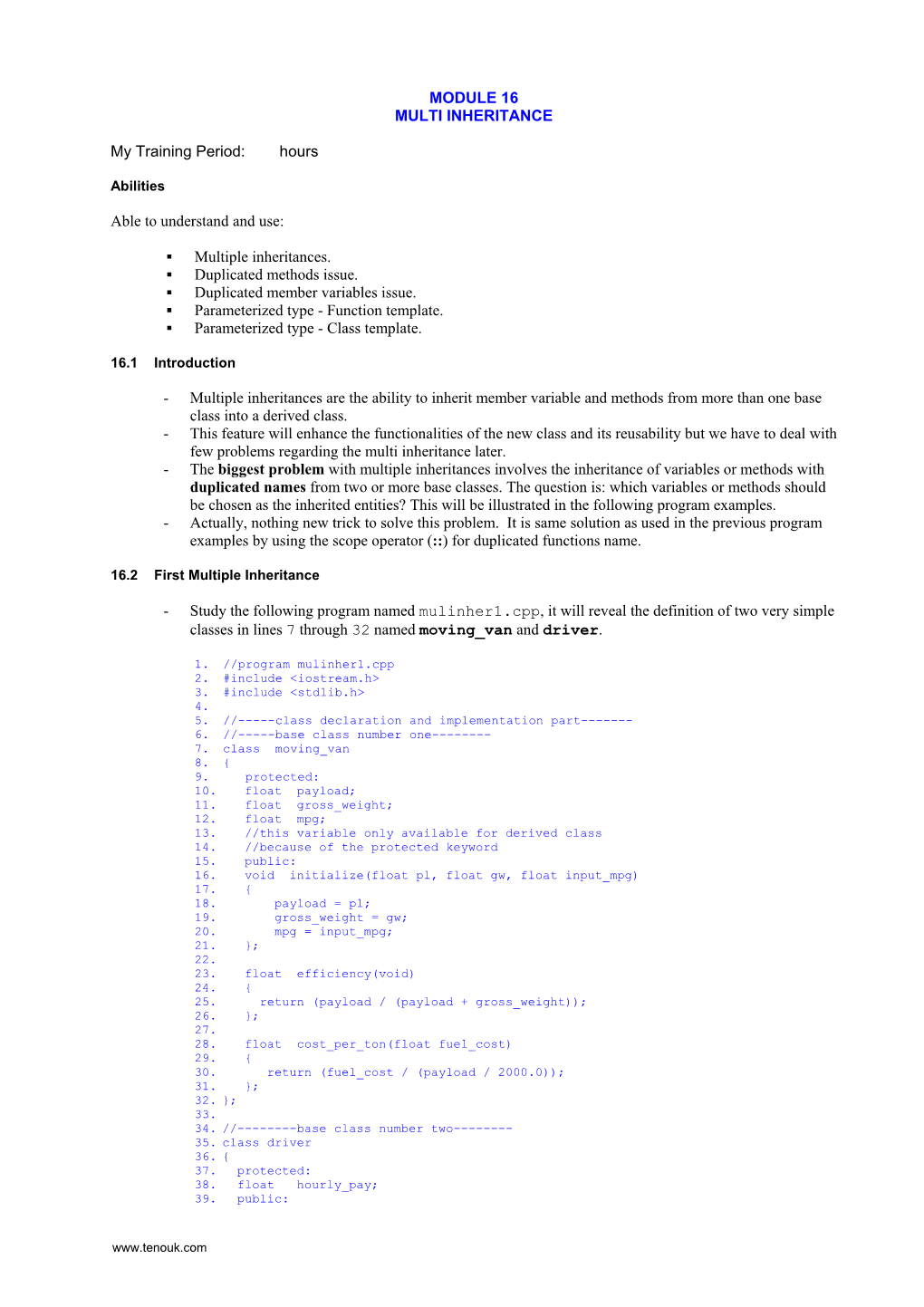
Load more
Recommended publications
-
MANNING Greenwich (74° W
Object Oriented Perl Object Oriented Perl DAMIAN CONWAY MANNING Greenwich (74° w. long.) For electronic browsing and ordering of this and other Manning books, visit http://www.manning.com. The publisher offers discounts on this book when ordered in quantity. For more information, please contact: Special Sales Department Manning Publications Co. 32 Lafayette Place Fax: (203) 661-9018 Greenwich, CT 06830 email: [email protected] ©2000 by Manning Publications Co. All rights reserved. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form or by means electronic, mechanical, photocopying, or otherwise, without prior written permission of the publisher. Many of the designations used by manufacturers and sellers to distinguish their products are claimed as trademarks. Where those designations appear in the book, and Manning Publications was aware of a trademark claim, the designations have been printed in initial caps or all caps. Recognizing the importance of preserving what has been written, it is Manning’s policy to have the books we publish printed on acid-free paper, and we exert our best efforts to that end. Library of Congress Cataloging-in-Publication Data Conway, Damian, 1964- Object oriented Perl / Damian Conway. p. cm. includes bibliographical references. ISBN 1-884777-79-1 (alk. paper) 1. Object-oriented programming (Computer science) 2. Perl (Computer program language) I. Title. QA76.64.C639 1999 005.13'3--dc21 99-27793 CIP Manning Publications Co. Copyeditor: Adrianne Harun 32 Lafayette -
A Comparative Study on the Effect of Multiple Inheritance Mechanism in Java, C++, and Python on Complexity and Reusability of Code
(IJACSA) International Journal of Advanced Computer Science and Applications, Vol. 8, No. 6, 2017 A Comparative Study on the Effect of Multiple Inheritance Mechanism in Java, C++, and Python on Complexity and Reusability of Code Fawzi Albalooshi Amjad Mahmood Department of Computer Science Department of Computer Science University of Bahrain University of Bahrain Kingdom of Bahrain Kingdom of Bahrain Abstract—Two of the fundamental uses of generalization in Booch, there are two problems associated with multiple object-oriented software development are the reusability of code inheritance and they are how to deal with name collisions from and better structuring of the description of objects. Multiple super classes, and how to handle repeated inheritance. He inheritance is one of the important features of object-oriented presents solutions to these two problems. Other researchers [4] methodologies which enables developers to combine concepts and suggest that there is a real need for multiple inheritance for increase the reusability of the resulting software. However, efficient object implementation. They justify their claim multiple inheritance is implemented differently in commonly referring to the lack of multiple subtyping in the ADA 95 used programming languages. In this paper, we use Chidamber revision which was considered as a deficiency that was and Kemerer (CK) metrics to study the complexity and rectified in the newer version [5]. It is clear that multiple reusability of multiple inheritance as implemented in Python, inheritance is a fundamental concept in object-orientation. The Java, and C++. The analysis of results suggests that out of the three languages investigated Python and C++ offer better ability to incorporate multiple inheritance in system design and reusability of software when using multiple inheritance, whereas implementation will better structure the description of objects Java has major deficiencies when implementing multiple modeling, their natural status and enabling further code reuse inheritance resulting in poor structure of objects. -
Mixins and Traits
◦ ◦◦◦ TECHNISCHE UNIVERSITAT¨ MUNCHEN¨ ◦◦◦◦ ◦ ◦ ◦◦◦ ◦◦◦◦ ¨ ¨ ◦ ◦◦ FAKULTAT FUR INFORMATIK Programming Languages Mixins and Traits Dr. Michael Petter Winter 2016/17 What advanced techiques are there besides multiple implementation inheritance? Outline Design Problems Cons of Implementation Inheritance 1 Inheritance vs Aggregation 1 2 (De-)Composition Problems Lack of finegrained Control 2 Inappropriate Hierarchies Inheritance in Detail A Focus on Traits 1 A Model for single inheritance 1 2 Inheritance Calculus with Separation of Composition and Inheritance Expressions Modeling 2 3 Modeling Mixins Trait Calculus Mixins in Languages Traits in Languages 1 (Virtual) Extension Methods 1 Simulating Mixins 2 Squeak 2 Native Mixins Reusability ≡ Inheritance? Codesharing in Object Oriented Systems is often inheritance-centric. Inheritance itself comes in different flavours: I single inheritance I multiple inheritance All flavours of inheritance tackle problems of decomposition and composition The Adventure Game Door ShortDoor LockedDoor canPass(Person p) canOpen(Person p) ? ShortLockedDoor canOpen(Person p) canPass(Person p) The Adventure Game Door <interface>Doorlike canPass(Person p) canOpen(Person p) Short canPass(Person p) Locked canOpen(Person p) ShortLockedDoor ! Aggregation & S.-Inheritance Door must explicitely provide canOpen(Person p) chaining canPass(Person p) Doorlike must anticipate wrappers ) Multiple Inheritance X The Wrapper FileStream SocketStream read() read() write() write() ? SynchRW acquireLock() releaseLock() ! Inappropriate Hierarchies -
Mixin-Based Programming in C++1
Mixin-Based Programming in C++1 Yannis Smaragdakis Don Batory College of Computing Department of Computer Sciences Georgia Institute of Technology The University of Texas at Austin Atlanta, GA 30332 Austin, Texas 78712 [email protected] [email protected] Abstract. Combinations of C++ features, like inheritance, templates, and class nesting, allow for the expression of powerful component patterns. In particular, research has demonstrated that, using C++ mixin classes, one can express lay- ered component-based designs concisely with efficient implementations. In this paper, we discuss pragmatic issues related to component-based programming using C++ mixins. We explain surprising interactions of C++ features and poli- cies that sometimes complicate mixin implementations, while other times enable additional functionality without extra effort. 1 Introduction Large software artifacts are arguably among the most complex products of human intellect. The complexity of software has led to implementation methodologies that divide a problem into manageable parts and compose the parts to form the final prod- uct. Several research efforts have argued that C++ templates (a powerful parameteriza- tion mechanism) can be used to perform this division elegantly. In particular, the work of VanHilst and Notkin [29][30][31] showed how one can implement collaboration-based (or role-based) designs using a certain templatized class pattern, known as a mixin class (or just mixin). Compared to other techniques (e.g., a straightforward use of application frameworks [17]) the VanHilst and Notkin method yields less redundancy and reusable components that reflect the structure of the design. At the same time, unnecessary dynamic binding can be eliminated, result- ing into more efficient implementations. -
Comp 411 Principles of Programming Languages Lecture 19 Semantics of OO Languages
Comp 411 Principles of Programming Languages Lecture 19 Semantics of OO Languages Corky Cartwright Mar 10-19, 2021 Overview I • In OO languages, OO data values (except for designated non-OO types) are special records [structures] (finite mappings from names to values). In OO parlance, the components of record are called members. • Some members of an object may be functions called methods. Methods take this (the object in question) as an implicit parameter. Some OO languages like Java also support static methods that do not depend on this; these methods have no implicit parameters. In efficient OO language implementations, method members are shared since they are the same for all instances of a class, but this sharing is an optimization in statically typed OO languages since the collection of methods in a class is immutable during program evaluation (computation). • A method (instance method in Java) can only be invoked on an object (the receiver, an implicit parameter). Additional parameters are optional, depending on whether the method expects them. This invocation process is called dynamic dispatch because the executed code is literally extracted from the object: the code invoked at a call site depends on the value of the receiver, which can change with each execution of the call. • A language with objects is OO if it supports dynamic dispatch (discussed in more detail in Overview II & III) and inheritance, an explicit taxonomy for classifying objects based on their members and class names where superclass/parent methods are inherited unless overridden. • In single inheritance, this taxonomy forms a tree; • In multiple inheritance, it forms a rooted DAG (directed acyclic graph) where the root class is the universal class (Object in Java). -
Multiple Inheritance and the Resolution of Inheritance Conflicts
JOURNAL OF OBJECT TECHNOLOGY Online at http://www.jot.fm. Published by ETH Zurich, Chair of Software Engineering ©JOT, 2005 Vol. 4, No.2, March-April 2005 The Theory of Classification Part 17: Multiple Inheritance and the Resolution of Inheritance Conflicts Anthony J H Simons, Department of Computer Science, University of Sheffield, Regent Court, 211 Portobello Street, Sheffield S1 4DP, UK 1 INTRODUCTION This is the seventeenth article in a regular series on object-oriented theory for non- specialists. Using a second-order λ-calculus model, we have previously modelled the notion of inheritance as a short-hand mechanism for defining subclasses by extending superclass definitions. Initially, we considered the inheritance of type [1] and implementation [2] separately, but later combined both of these in a model of typed inheritance [3]. By simplifying the short-hand inheritance expressions, we showed how these are equivalent to canonical class definitions. We also showed how classes derived by inheritance are type compatible with their superclass. Further aspects of inheritance have included method combination [4], mixin inheritance [5] and inheritance among generic classes [6]. Most recently, we re-examined the ⊕ inheritance operator [7], to show how extending a class definition (the intension of a class) has the effect of restricting the set of objects that belong to the class (the extension of the class). We also added a type constraint to the ⊕ operator, to restrict the types of fields that may legally be combined with a base class to yield a subclass. By varying the form of this constraint, we modelled the typing of inheritance in Java, Eiffel, C++ and Smalltalk. -
Multiple Inheritance
Multiple Inheritance [email protected] Text: Chapter11 and 21, Big C++ Multiple Inheritance • Inheritance discussed so far is Single Inheritance • If a class has only one super class, then it is Single Inheritance • C++, also support Multiple Inheritance , i.e., when a class has more than one parent class Multiple Inheritance • Some of examples are- – Faculty could be Alumnus and Employee in DIICTian scenario – Head-Engineering , needs to be Manager and Engineer both – A CustomerEmployee would be Employee (a Person too), and Customer (a Person too)– forms diamond inheritance Here is how we have multiple inheritance in C++ class C : public A, public B { } • In this case, C inherits from A and B both … “public” Example: Multiple Inheritance Consider Example given • What methods class C has? • What is their visibility in class C? • What data members class C has? • What is their visibility in class C? Example: Multiple Inheritance Issues in Multiple Inheritance : Name ambiguity Base classes A and B of C both has getX() method Issues in Multiple Inheritance : Diamond Inheritance • Class B1 and B2 inherits from L, and • D inherits from B1 and B2, both • Therefore, in D, L inherits twice • It brings in some issues, consider example on next slide • What data and function members C has? • What is their visibility? Issues in Multiple Inheritance : Diamond Inheritance • Class D has – two copies of data ax – Ambiguous method names getX(), getAX() • Two copies of same variable should be more critical Issues in multiple inheritance Virtual Inheritance • C++ addresses this issue by allowing such base (being inherited multiple times) class to be virtual base class • As a result all virtual occurrences of the class throughout the class hierarchy share one actual occurrence of it. -
Object Oriented Perl: an Introduction
Object Oriented Perl: An Introduction Object Oriented Perl: An Introduction Abram Hindle Department of Computer Science University of Victoria [email protected] July 13, 2004 Abram Hindle 1 Object Oriented Perl: An Introduction This Presentation • What am I going to cover? – OO Intro – Packages – References – Constructor – Attributes – Methods – Inheritance – Fun Stuff – Getting Help – Conclusions – References Abram Hindle 2 Object Oriented Perl: An Introduction OO Introduction • What are objects? – A combination of attributes and functions associated with those attributes. Objects can have state, identify and behaviors. You can execute actions associated with objects. e.g. A dog object could bark. Effectively objects are instances of Classes. • What are classes? – The definition or blueprint for an object. http://www.justpbinfo.com/techdocs/ooterms.asp Abram Hindle 3 Object Oriented Perl: An Introduction OO Introduction • Why use Object Oriented Programming – OOP helps us organize our code and split our problems up into smaller blocks. – OOP is the current dominant programming style. – OOP seems to be the best that we currently have in imperative programming. – With OOP we gain useful things like polymorphism, encapsulation and other big words/concepts. Abram Hindle 4 Object Oriented Perl: An Introduction Packages • What are Packages? – The main way code is organized in Perl is with packages. – Sample Package: ∗ package Name; use strict; #save us debugging time ...code... 1; # outdated, used for a return from a use. – More than 1 package can exist in a file. Usually packages are saved into .pm files like Name.pm Abram Hindle 5 Object Oriented Perl: An Introduction Packages • More Details... – Our Class Names will be the package name. -
An Exemplar Based Smalltalk
An Exemplar Based Smalltaik Will R. LaLonde, Dave A. Thomas and John R. Pugh School of Computer Science Carleton University Ottawa, Ontario, Canada KIS 5B6 Abstract Two varieties of object-oriented systems exist: one In this paper, we detail some of the Smalltalk deficiencies based on classes as in Smalltalk and another based on that are a direct result of its class based orientation and describe exemplars (or prototypicai objects) as in Act/l. By converting how a more general and flexible system is obtained by Smalltalk from a class based orientation to an exemplar base, changing its underlying organizational base from classes to independent instance hierarchies and class hierarchies can be exemplars. The work is relevant to the evolution of any provided. Decoupling the two hierarchies in this way enables object-oriented system including the Flavour system in Lisp the user's (logical) view of a data type to be separated from the [Weinreb 807, Loops [Bobrow 81], Trellis [O'Brien 857, and implementer's (physical) view. It permits the instances of a more conventional languages being retro-fitted with class to have a representation totally different from the object-oriented facilities; e.g., C [Cox 84], Pascal [Tesler 847, instances of a superclass. Additionally, it permits the notion of Forth [Duff84], and Prolog [Shapiro 83, Vaucber 867. multiple representations to be provided without the need to Exemplar based systems include Act/l[Lieberman 81, introduce specialized classes for each representation. In the Smallworld [Laffgl], Thinglab [Borning 827, and Actra context of multiple inheritance, it leads to a novel view of [Thomas 857. -
COMS 3101 Programming Languages: Perl Lecture 5
COMS 3101 Programming Languages: Perl Lecture 5 Fall 2013 Instructor: Ilia Vovsha http://www.cs.columbia.edu/~vovsha/coms3101/perl Lecture Outline Packages & Modules Concepts: Subroutine references SbliSymbolic references Garbage collection Saving structures Objects and Classes Next: More OOP, CPAN 5.2 Remarks Pattern matching “cage” can be any character: • m// or // is equivalent to m{} • s/// is equivalent to s{} {} • // are just customary quote characters for pattern matching behavior. In fact you could choose your own character instead of {} (e.g. m ! !) • Convenient if lots of slhlashes in the pattern ref function: returns type of reference (a string) • $rtype = ref($href); # returns “HASH” • $rtype = ref($aref); # returns “ARRAY” • if ( ref($href) eq “HASH” ) { … } 5.3 Packages & Modules Why do we need them? Package or module, what is the difference? ‘use’ vs. ‘require’ Importing from another package Pragmatic modules (ppgragmas) 5.4 Packages (purpose) sub parse_text { # code from one file … $count = $count++; … } sub normalize { # code from another file $count++; … } # Use both functions: parse_text(); normalize(); print “$count\n”; # Which $count? What is its value? 5.5 Packages (definition) Balanced code: abstraction + reuse Every chkhunk of code has its own namespace. In PlPerl, a namespace is called a package Independent of files: • Multiple packages in one file • Single package spanning multiple files • Most common: one package per file Best approach: one package per file where file name is package name -
Encapsulation, Inheritance, Poly Ion, Inheritance, Polymorphism
Encapsulation, Inheritance, Polymorphism • Data members • Methods Object Orientation PHP is an Object-Oriented programming language Fundamental feature of OO is the class PHP classes support Encapsulation Inheritance Polymorphism What is a Class? Classes Sophisticated ‘variable types’ Data variables (data members) and functions (methods) are wrapped up in a class. Collectively, data members and methods are referred to as class members. An instance of a class is known as an object. //defining a new class named Robot class Robot { //place class members here... } Instantiating a class using new Once a class has been created, any number of object instances of that class can be created. $dogRobot = new Robot(); To invoke methods: e.g. object->method() <?php .... $dogRobot = new Robot(); $dogRobot ->crawlWeb(); $dogRobot -> play(); echo $dogRobot ->talk(); ... ?> Defining classes <?php class Person { Data members private $strFirstname = “Napoleon"; private $strSurname = “Reyes"; function getFirstname() { return $this->strFirstname; } Methods function getSurname() { return $this->strSurname; } } // outside the class definition $obj = new Person; // an object of type Person echo "<p>Firstname: " . $obj->getFirstname() . "</p>"; echo "<p>Surname: " . $obj->getSurname() . "</p>"; ?> Example16-1.php Encapsulation Data members are normally set inaccessible from outside the class (as well as certain types of methods) protecting them from the rest of the script and other classes. This protection of class members is known as encapsulation. e.g. <?php .... $dogRobot = new Robot(); $dogRobot ->crawlWeb(); $dogRobot -> play(); echo $dogRobot ->talk(); ... ?> Inheritance New classes can be defined very similar to existing ones. All we need to do is specify the differences between the new class and the existing one. Data members and methods which are not defined as being private to a class are automatically accessible by the new class. -
What Is Object-Oriented Perl?
This is a series of extracts from Object Oriented Perl, a new book from Manning Publications that will be available in August 1999. For more information on this book, see http://www.manning.com/Conway/. What is object-oriented Perl? Object-oriented Perl is a small amount of additional syntax and semantics, added to the existing imperative features of the Perl programming language. Those extras allow regular Perl packages, variables, and subroutines to behave like classes, objects, and methods. It's also a small number of special variables, packages and modules, and a large number of new techniques, that together provide inheritance, data encapsulation, operator overloading, automated definition of commonly used methods, generic programming, multiply-dispatched polymorphism, and persistence. It's an idiosyncratic, no-nonsense, demystified approach to object-oriented programming, with a typically Perlish disregard for accepted rules and conventions. It draws inspiration (and sometimes syntax) from many different object-oriented predecessors, adapting their ideas to its own needs. It reuses and extends the functionality of existing Perl features, and in the process throws an entirely new slant on what they mean. In other words, it's everything that regular Perl is, only object-oriented. Using Perl makes object-oriented programming more enjoyable, and using object- oriented programming makes Perl more enjoyable too. Life is too short to endure the cultured bondage-and-discipline of Eiffel programming, or to wrestle the alligators that lurk in the muddy semantics of C++. Object-oriented Perl gives you all the power of those languages, with very few of their tribulations. And best of all, like regular Perl, it's fun! Before we look at object orientation in Perl, let’s talk about what object orientation is in general..