Computer Science 477/677 Fall 2019 University of Nevada, Las Vegas Answers to Third Examination November 20, 2019
Total Page:16
File Type:pdf, Size:1020Kb
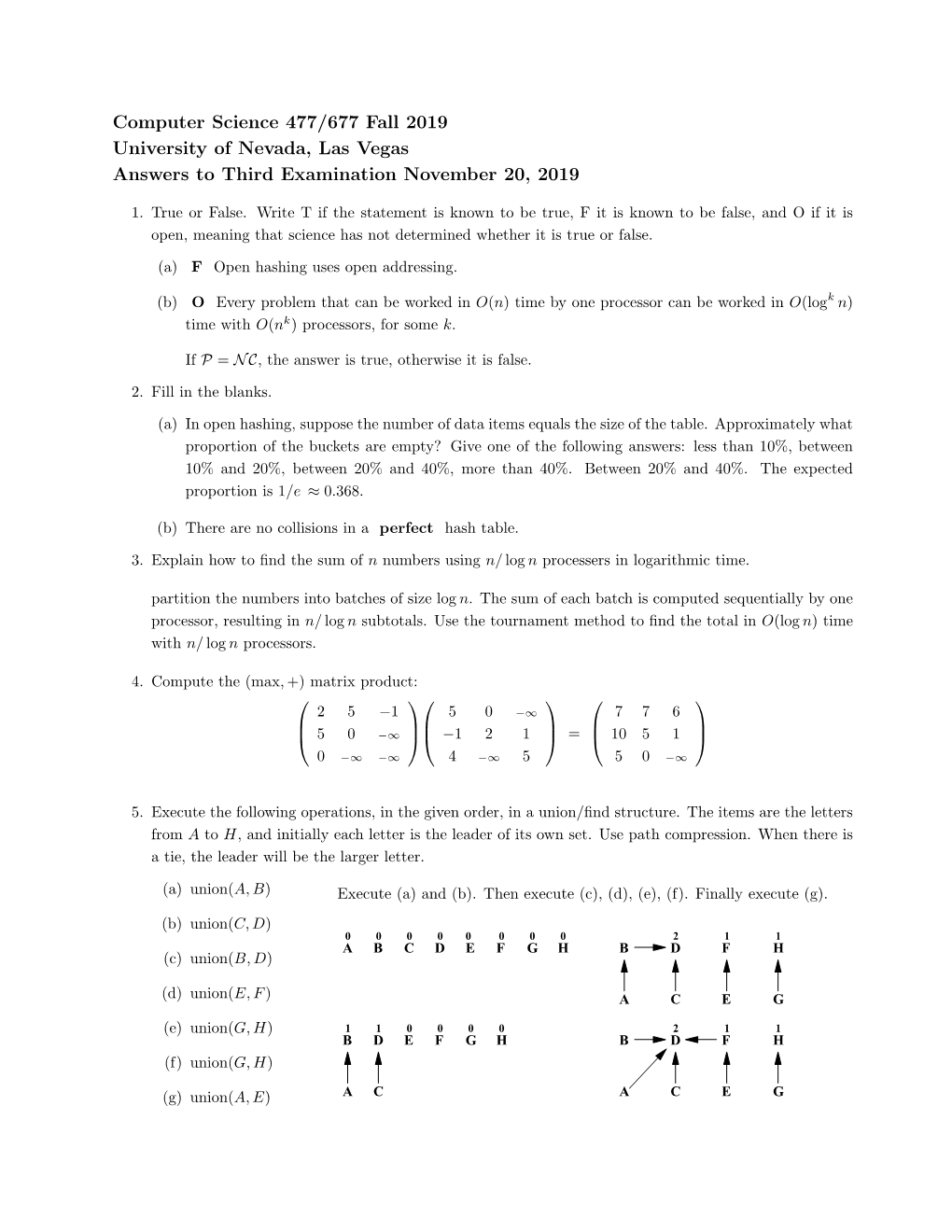
Load more
Recommended publications
-
Efficiently Mining Frequent Closed Partial Orders
Efficiently Mining Frequent Closed Partial Orders (Extended Abstract) Jian Pei1 Jian Liu2 Haixun Wang3 Ke Wang1 Philip S. Yu3 Jianyong Wang4 1 Simon Fraser Univ., Canada, fjpei, [email protected] 2 State Univ. of New York at Buffalo, USA, [email protected] 3 IBM T.J. Watson Research Center, USA, fhaixun, [email protected] 4 Tsinghua Univ., China, [email protected] 1 Introduction Account codes and explanation Account code Account type CHK Checking account Mining ordering information from sequence data is an MMK Money market important data mining task. Sequential pattern mining [1] RRSP Retirement Savings Plan can be regarded as mining frequent segments of total orders MORT Mortgage from sequence data. However, sequential patterns are often RESP Registered Education Savings Plan insufficient to concisely capture the general ordering infor- BROK Brokerage mation. Customer Records Example 1 (Motivation) Suppose MapleBank in Canada Cid Sequence of account opening wants to investigate whether there is some orders which cus- 1 CHK ! MMK ! RRSP ! MORT ! RESP ! BROK tomers often follow to open their accounts. A database DB 2 CHK ! RRSP ! MMK ! MORT ! RESP ! BROK in Table 1 about four customers’ sequences of opening ac- 3 MMK ! CHK ! BROK ! RESP ! RRSP counts in MapleBank is analyzed. 4 CHK ! MMK ! RRSP ! MORT ! BROK ! RESP Given a support threshold min sup, a sequential pattern is a sequence s which appears as subsequences of at least Table 1. A database DB of sequences of ac- min sup sequences. For example, let min sup = 3. The count opening. following four sequences are sequential patterns since they are subsequences of three sequences, 1, 2 and 4, in DB. -
Approximating Transitive Reductions for Directed Networks
Approximating Transitive Reductions for Directed Networks Piotr Berman1, Bhaskar DasGupta2, and Marek Karpinski3 1 Pennsylvania State University, University Park, PA 16802, USA [email protected] Research partially done while visiting Dept. of Computer Science, University of Bonn and supported by DFG grant Bo 56/174-1 2 University of Illinois at Chicago, Chicago, IL 60607-7053, USA [email protected] Supported by NSF grants DBI-0543365, IIS-0612044 and IIS-0346973 3 University of Bonn, 53117 Bonn, Germany [email protected] Supported in part by DFG grants, Procope grant 31022, and Hausdorff Center research grant EXC59-1 Abstract. We consider minimum equivalent digraph problem, its max- imum optimization variant and some non-trivial extensions of these two types of problems motivated by biological and social network appli- 3 cations. We provide 2 -approximation algorithms for all the minimiza- tion problems and 2-approximation algorithms for all the maximization problems using appropriate primal-dual polytopes. We also show lower bounds on the integrality gap of the polytope to provide some intuition on the final limit of such approaches. Furthermore, we provide APX- hardness result for all those problems even if the length of all simple cycles is bounded by 5. 1 Introduction Finding an equivalent digraph is a classical computational problem (cf. [13]). The statement of the basic problem is simple. For a digraph G = (V, E), we E use the notation u → v to indicate that E contains a path from u to v and E the transitive closure of E is the relation u → v over all pairs of vertices of V . -
Copyright © 1980, by the Author(S). All Rights Reserved
Copyright © 1980, by the author(s). All rights reserved. Permission to make digital or hard copies of all or part of this work for personal or classroom use is granted without fee provided that copies are not made or distributed for profit or commercial advantage and that copies bear this notice and the full citation on the first page. To copy otherwise, to republish, to post on servers or to redistribute to lists, requires prior specific permission. ON A CLASS OF ACYCLIC DIRECTED GRAPHS by J. L. Szwarcfiter Memorandum No. UCB/ERL M80/6 February 1980 ELECTRONICS RESEARCH LABORATORY College of Engineering University of California, Berkeley 94720 ON A CLASS OF ACYCLIC DIRECTED GRAPHS* Jayme L. Szwarcfiter** Universidade Federal do Rio de Janeiro COPPE, I. Mat. e NCE Caixa Postal 2324, CEP 20000 Rio de Janeiro, RJ Brasil • February 1980 Key Words: algorithm, depth first search, directed graphs, graphs, isomorphism, minimal chain decomposition, partially ordered sets, reducible graphs, series parallel graphs, transitive closure, transitive reduction, trees. CR Categories: 5.32 *This work has been supported by the Conselho Nacional de Desenvolvimento Cientifico e Tecnologico (CNPq), Brasil, processo 574/78. The preparation ot the manuscript has been supported by the National Science Foundation, grant MCS78-20054. **Present Address: University of California, Computer Science Division-EECS, Berkeley, CA 94720, USA. ABSTRACT A special class of acyclic digraphs has been considered. It contains those acyclic digraphs whose transitive reduction is a directed rooted tree. Alternative characterizations have also been given, including one by forbidden subgraph containment of its transitive closure. For digraphs belonging to the mentioned class, linear time algorithms have been described for the following problems: recognition, transitive reduction and closure, isomorphism, minimal chain decomposition, dimension of the induced poset. -
95-106 Parallel Algorithms for Transitive Reduction for Weighted Graphs
Math. Maced. Vol. 8 (2010) 95-106 PARALLEL ALGORITHMS FOR TRANSITIVE REDUCTION FOR WEIGHTED GRAPHS DRAGAN BOSNAˇ CKI,ˇ WILLEM LIGTENBERG, MAXIMILIAN ODENBRETT∗, ANTON WIJS∗, AND PETER HILBERS Dedicated to Academician Gor´gi´ Cuponaˇ Abstract. We present a generalization of transitive reduction for weighted graphs and give scalable polynomial algorithms for computing it based on the Floyd-Warshall algorithm for finding shortest paths in graphs. We also show how the algorithms can be optimized for memory efficiency and effectively parallelized to improve the run time. As a consequence, the algorithms can be tuned for modern general purpose graphics processors. Our prototype imple- mentations exhibit significant speedups of more than one order of magnitude compared to their sequential counterparts. Transitive reduction for weighted graphs was instigated by problems in reconstruction of genetic networks. The first experiments in that domain show also encouraging results both regarding run time and the quality of the reconstruction. 1. Introduction 0 The concept of transitive reduction for graphs was introduced in [1] and a similar concept was given previously in [8]. Transitive reduction is in a sense the opposite of transitive closure of a graph. In transitive closure a direct edge is added between two nodes i and j, if an indirect path, i.e., not including edge (i; j), exists between i and j. In contrast, the main intuition behind transitive reduction is that edges between nodes are removed if there are also indirect paths between i and j. In this paper we present an extension of the notion of transitive reduction to weighted graphs. -
LNCS 7034, Pp
Confluent Hasse Diagrams DavidEppsteinandJosephA.Simons Department of Computer Science, University of California, Irvine, USA Abstract. We show that a transitively reduced digraph has a confluent upward drawing if and only if its reachability relation has order dimen- sion at most two. In this case, we construct a confluent upward drawing with O(n2)features,inanO(n) × O(n)gridinO(n2)time.Forthe digraphs representing series-parallel partial orders we show how to con- struct a drawing with O(n)featuresinanO(n)×O(n)gridinO(n)time from a series-parallel decomposition of the partial order. Our drawings are optimal in the number of confluent junctions they use. 1 Introduction One of the most important aspects of a graph drawing is that it should be readable: it should convey the structure of the graph in a clear and concise way. Ease of understanding is difficult to quantify, so various proxies for it have been proposed, including the number of crossings and the total amount of ink required by the drawing [1,18]. Thus given two different ways to present information, we should choose the more succinct and crossing-free presentation. Confluent drawing [7,8,9,15,16] is a style of graph drawing in which multiple edges are combined into shared tracks, and two vertices are considered to be adjacent if a smooth path connects them in these tracks (Figure 1). This style was introduced to re- duce crossings, and in many cases it will also Fig. 1. Conventional and confluent improve the ink requirement by represent- drawings of K5,5 ing dense subgraphs concisely. -
An Improved Algorithm for Transitive Closure on Acyclic Digraphs
View metadata, citation and similar papers at core.ac.uk brought to you by CORE provided by Elsevier - Publisher Connector Theoretical Computer Science 58 (1988) 325-346 325 North-Holland AN IMPROVED ALGORITHM FOR TRANSITIVE CLOSURE ON ACYCLIC DIGRAPHS Klaus SIMON Fachbereich IO, Angewandte Mathematik und Informatik, CJniversitCt des Saarlandes, 6600 Saarbriicken, Fed. Rep. Germany Abstract. In [6] Goralcikova and Koubek describe an algorithm for finding the transitive closure of an acyclic digraph G with worst-case runtime O(n. e,,,), where n is the number of nodes and ered is the number of edges in the transitive reduction of G. We present an improvement on their algorithm which runs in worst-case time O(k. crud) and space O(n. k), where k is the width of a chain decomposition. For the expected values in the G,,.,, model of a random acyclic digraph with O<p<l we have F(k)=O(y), E(e,,,)=O(n,logn), O(n’) forlog’n/n~p<l. E(k, ercd) = 0( n2 log log n) otherwise, where “log” means the natural logarithm. 1. Introduction A directed graph G = ( V, E) consists of a vertex set V = {1,2,3,. , n} and an edge set E c VX V. Each element (u, w) of E is an edge and joins 2, to w. If G, = (V,, E,) and G, = (V,, E2) are directed graphs, G, is a subgraph of Gz if V, G V, and E, c EZ. The subgraph of G, induced by the subset V, of V, is the graph G, = (V,, E,), where E, is the set of all elements of E, which join pairs of elements of V, . -
Some NP-Complete Problems
Appendix A Some NP-Complete Problems To ask the hard question is simple. But what does it mean? What are we going to do? W.H. Auden In this appendix we present a brief list of NP-complete problems; we restrict ourselves to problems which either were mentioned before or are closely re- lated to subjects treated in the book. A much more extensive list can be found in Garey and Johnson [GarJo79]. Chinese postman (cf. Sect. 14.5) Let G =(V,A,E) be a mixed graph, where A is the set of directed edges and E the set of undirected edges of G. Moreover, let w be a nonnegative length function on A ∪ E,andc be a positive number. Does there exist a cycle of length at most c in G which contains each edge at least once and which uses the edges in A according to their given orientation? This problem was shown to be NP-complete by Papadimitriou [Pap76], even when G is a planar graph with maximal degree 3 and w(e) = 1 for all edges e. However, it is polynomial for graphs and digraphs; that is, if either A = ∅ or E = ∅. See Theorem 14.5.4 and Exercise 14.5.6. Chromatic index (cf. Sect. 9.3) Let G be a graph. Is it possible to color the edges of G with k colors, that is, does χ(G) ≤ k hold? Holyer [Hol81] proved that this problem is NP-complete for each k ≥ 3; this holds even for the special case where k =3 and G is 3-regular. -
Perturbation Graphs, Invariant Prediction and Causal Relations in Psychology
Perturbation graphs, invariant prediction and causal relations in psychology Lourens Waldorp, Jolanda Kossakowski, Han L. J. van der Maas University of Amsterdam, Nieuwe Achtergracht 129-B, 1018 NP, the Netherlands arXiv:2109.00404v1 [stat.ME] 1 Sep 2021 Abstract Networks (graphs) in psychology are often restricted to settings without interven- tions. Here we consider a framework borrowed from biology that involves multiple interventions from different contexts (observations and experiments) in a single analysis. The method is called perturbation graphs. In gene regulatory networks, the induced change in one gene is measured on all other genes in the analysis, thereby assessing possible causal relations. This is repeated for each gene in the analysis. A perturbation graph leads to the correct set of causes (not necessarily di- rect causes). Subsequent pruning of paths in the graph (called transitive reduction) should reveal direct causes. We show that transitive reduction will not in general lead to the correct underlying graph. There is however a close relation with an- other method, called invariant causal prediction. Invariant causal prediction can be considered as a generalisation of the perturbation graph method where including ad- ditional variables (and so conditioning on those variables) does reveal direct causes, and thereby replacing transitive reduction. We explain the basic ideas of pertur- bation graphs, transitive reduction and invariant causal prediction and investigate their connections. We conclude that perturbation graphs provide a promising new tool for experimental designs in psychology, and combined with invariant prediction make it possible to reveal direct causes instead of causal paths. As an illustration we apply the perturbation graphs and invariant causal prediction to a data set about attitudes on meat consumption. -
Arxiv:1112.1444V2 [Cs.DS] 12 Feb 2013 Rnprainntok N8,NG8,Admr Eety Trop AGG10]
ON THE COMPLEXITY OF STRONGLY CONNECTED COMPONENTS IN DIRECTED HYPERGRAPHS XAVIER ALLAMIGEON Abstract. We study the complexity of some algorithmic problems on directed hypergraphs and their strongly connected components (Sccs). The main con- tribution is an almost linear time algorithm computing the terminal strongly connected components (i.e. Sccs which do not reach any components but themselves). Almost linear here means that the complexity of the algorithm is linear in the size of the hypergraph up to a factor α(n), where α is the inverse of Ackermann function, and n is the number of vertices. Our motivation to study this problem arises from a recent application of directed hypergraphs to computational tropical geometry. We also discuss the problem of computing all Sccs. We establish a super- linear lower bound on the size of the transitive reduction of the reachability relation in directed hypergraphs, showing that it is combinatorially more com- plex than in directed graphs. Besides, we prove a linear time reduction from the well-studied problem of finding all minimal sets among a given family to the problem of computing the Sccs. Only subquadratic time algorithms are known for the former problem. These results strongly suggest that the prob- lem of computing the Sccs is harder in directed hypergraphs than in directed graphs. 1. Introduction Directed hypergraphs consist in a generalization of directed graphs, in which the tail and the head of the arcs are sets of vertices. Directed hypergraphs have a very large number of applications, since hyperarcs naturally provide a represen- tation of implication dependencies. Among others, they are used to solve several problems related to satisfiability in propositional logic, in particular on Horn for- arXiv:1112.1444v2 [cs.DS] 12 Feb 2013 mulas, see for instance [AI91, AFFG97, GP95, GGPR98, Pre03]. -
APPROXIMATING the MINIMUM EQUIVALENT DIGRAPH* SAMIR KHULLER T, BALAJI RAGHAVACHARI, and NEAL YOUNG Abstract
SIAM J. COMPUT. () 1995 Society for Industrial and Applied Mathematics Vol. 24, No. 4, pp, 859-872, August 1995 011 APPROXIMATING THE MINIMUM EQUIVALENT DIGRAPH* SAMIR KHULLER t, BALAJI RAGHAVACHARI, AND NEAL YOUNG Abstract. The minimum equivalent graph (MEG) problem is as follows: given a directed graph, find a smallest subset of the edges that maintains all teachability relations between nodes. This problem is NP-hard; this paper gives an approximation algorithm achieving a performance guarantee of about 1.64 in polynomial time. The algorithm achieves a performance guarantee of 1.75 in the time required for transitive closure. The heart of the MEG problem is the minimum strongly connected spanning subgraph (SCSS) problem--the MEG problem restricted to strongly connected digraphs. For the minimum SCSS problem, the paper gives a practical, nearly linear-time implementation achieving a performance guarantee of 1.75. The algorithm and its analysis are based on the simple idea of contracting long cycles. The analysis applies directly to 2-EXCHANCE, a general "local improvement" algorithm, showing that its performance guarantee is 1.75. Key words, directed graph, approximation algorithm, strong connectivity, local improvement AMS subject classifications. 68R10, 90C27, 90C35, 05C85, 68Q20 1. Introduction. Connectivity is fundamental to the study of graphs and graph algo- rithms. Recently, many approximation algorithms for finding minimum subgraphs that meet given connectivity requirements have been developed [1], [9], [11], [15], [16], [24]. These results provide practical approximation algorithms for NP-hard network-design problems via an increased understanding of connectivity properties. Until now, the techniques developed have been applicable only to undirected graphs. -
Transitive Closure and Transitive Reduction in Bidirected Graphs 3 Where X, X1,...,Y Are Vertices and E1,E2
TRANSITIVE CLOSURE AND TRANSITIVE REDUCTION IN BIDIRECTED GRAPHS OUAHIBA BESSOUF, ALGER, ABDELKADER KHELLADI, ALGER, AND THOMAS ZASLAVSKY, BINGHAMTON Abstract. In a bidirected graph an edge has a direction at each end, so bidirected graphs generalize directed graphs. We generalize the definitions of transitive closure and transitive reduction from directed graphs to bidirected graphs by introducing new notions of bipath and bicircuit that generalize di- rected paths and cycles. We show how transitive reduction is related to tran- sitive closure and to the matroids of the signed graph corresponding to the bidirected graph. 1. Introduction. Bidirected graphs are a generalization of undirected and directed graphs. Harary defined in 1954 the notion of signed graph. For any bidirected graph, we can asso- ciate a signed graph of which the bidirected graph is an orientation. Reciprocally, any signed graph can be associated to a bidirected graph in multiple ways, just as a graph can be associated to a directed graph. Transitive closure is well known. Transitive reduction in directed graphs was introduced by Aho, Garey, and Ullman [1]. The aim of this paper is to extend the concepts of transitive closure, which is denoted by Tr(Gτ ), and transitive reduction, which is denoted by R(Gτ ), to bidirected graphs. We seek to find definitions of transitive closure and transitive reduction for bidirected graphs through which the classical concepts would be a special case. We establish for bidirected graphs some properties of these concepts and a duality relationship between transitive closure and transitive reduction. 2. Bidirected Graphs We allow graphs to have loops and multiple edges. -
Some Directed Graph Algorithms and Their Application to Pointer Analysis
University of London Imperial College of Science, Technology and Medicine Department of Computing Some directed graph algorithms and their application to pointer analysis David J. Pearce February 2005 Submitted in partial fulfilment of the requirements for the degree of Doctor of Philosophy in Engineering of the University of London Abstract This thesis is focused on improving execution time and precision of scalable pointer analysis. Such an analysis statically determines the targets of all pointer variables in a program. We formulate the analysis as a directed graph problem, where the solution can be obtained by a computation similar, in many ways, to transitive closure. As with transitive closure, identifying strongly connected components and transitive edges offers significant gains. However, our problem differs as the computation can result in new edges being added to the graph and, hence, dynamic algorithms are needed to efficiently identify these structures. Thus, pointer analysis has often been likened to the dynamic transitive closure problem. Two new algorithms for dynamically maintaining the topological order of a directed graph are presented. The first is a unit change algorithm, meaning the solution must be recomputed immediately following an edge insertion. While this has a marginally inferior worse-case time bound, compared with a previous solution, it is far simpler to implement and has fewer restrictions. For these reasons, we find it to be faster in practice and provide an experimental study over random graphs to support this. Our second is a batch algorithm, meaning the solution can be updated after several insertions, and it is the first truly dynamic solution to obtain an optimal time bound of O(v + e + b) over a batch b of edge insertions.