Modular Exceptions a System for Handling Exceptions in a Modular Way
Total Page:16
File Type:pdf, Size:1020Kb
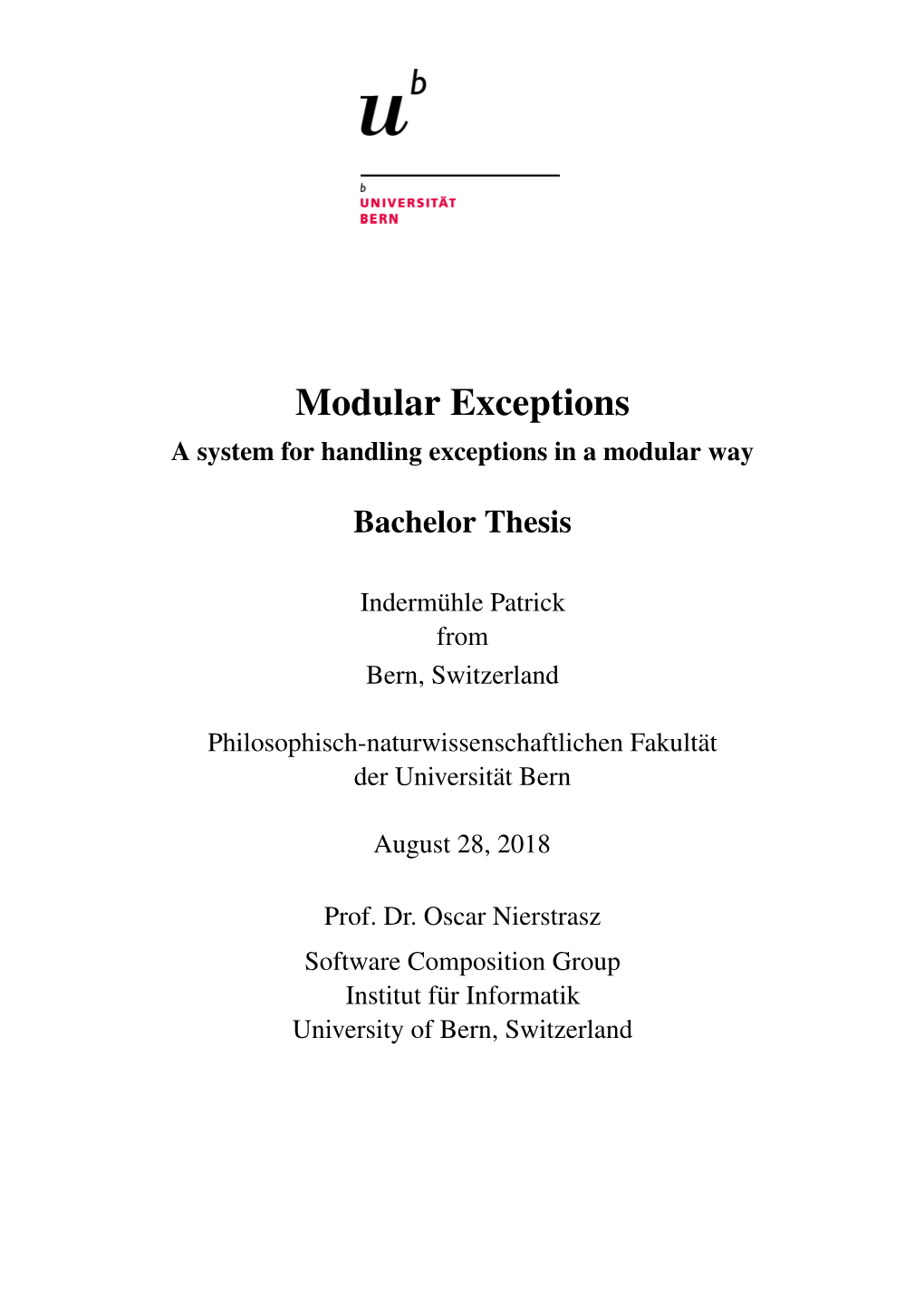
Load more
Recommended publications
-
The Basics of Exception Handling
The Basics of Exception Handling MIPS uses two coprocessors: C0 and C1 for additional help. C0 primarily helps with exception handling, and C1 helps with floating point arithmetic. Each coprocessor has a few registers. Interrupts Initiated outside the instruction stream Arrive asynchronously (at no specific time), Example: o I/O device status change o I/O device error condition Traps Occur due to something in instruction stream. Examples: o Unaligned address error o Arithmetic overflow o System call MIPS coprocessor C0 has a cause register (Register 13) that contains a 4-bit code to identify the cause of an exception Cause register pending exception code interrupt Bits 15-10 Bits 5-2 [Exception Code = 0 means I/O interrupt = 12 means arithmetic overflow etc] MIPS instructions that cause overflow (or some other violation) lead to an exception, which sets the exception code. It then switches to the kernel mode (designated by a bit in the status register of C0, register 12) and transfers control to a predefined address to invoke a routine (exception handler) for handling the exception. Interrupt Enable Status register Interrupt Mask 15-8 1 0 Kernel/User (EPC = Exception Program Counter, Reg 14 of C0) Memory L: add $t0, $t1, $t2 overflow! Return address (L+4) Exception handler routine is saved in EPC Next instruction Overflow ra ! EPC; jr ra Invalid instruction ra ! EPC; jr ra System Call ra ! EPC; jr ra The Exception Handler determines the cause of the exception by looking at the exception code bits. Then it jumps to the appropriate exception handling routine. -
A Framework for the Development of Multi-Level Reflective Applications
A Framework for the Development of Multi-Level Reflective Applications Federico Valentino, Andrés Ramos, Claudia Marcos and Jane Pryor ISISTAN Research Institute Fac. de Ciencias Exactas, UNICEN Pje. Arroyo Seco, B7001BBO Tandil, Bs. As., Argentina Tel/Fax: +54-2293-440362/3 E-mail: {fvalenti,aramos,cmarcos,jpryor}@exa.unicen.edu.ar URL: http://www.exa.unicen.edu.ar/~isistan Abstract. Computational reflection has become a useful technique for developing applications that are able to observe and modify their behaviour. Reflection has evolved to the point where it is being used to address a variety of application domains. This paper presents a reflective architecture that has been developed as a framework that provides a flexible reflective mechanism for the development of a wide range of applications. This architecture supports many meta-levels, where each one may contain one or more planes. A plane groups components that deal with the same functionality, and so enhances the separation of concerns in the system. The reflective mechanism permits different types of reflection, and also incorporates the runtime handling of potential conflicts between competing system components. The design and implementation of this framework are described, and an example illustrates its characteristics. 1. Introduction Reflective architectures provide a degree of flexibility that allows designers to adapt and add functionality to software systems in a transparent fashion. Since its onset, computational reflection has been proposed and used for the solving of many different problem domains in software engineering: aspect-oriented programming [PDF99, PBC00], multi-agent systems [Zun00], concurrency programming [WY88, MMY93], distributed systems [Str93, OI94, OIT92], and others. -
Support for Understanding and Writing Exception Handling Code
Moonstone: Support for Understanding and Writing Exception Handling Code Florian Kistner,y Mary Beth Kery,z Michael Puskas,x Steven Moore,z and Brad A. Myersz fl[email protected], [email protected], [email protected], [email protected], [email protected] y Department of Informatics, Technical University of Munich, Germany z Human-Computer Interaction Institute, Carnegie Mellon University, Pittsburgh, PA, USA x School of Computing, Informatics, and Decision Systems Engineering, Arizona State University, Tempe, AZ, USA Abstract—Moonstone is a new plugin for Eclipse that supports developers in understanding exception flow and in writing excep- tion handlers in Java. Understanding exception control flow is paramount for writing robust exception handlers, a task many de- velopers struggle with. To help with this understanding, we present two new kinds of information: ghost comments, which are transient overlays that reveal potential sources of exceptions directly in code, and annotated highlights of skipped code and associated handlers. To help developers write better handlers, Moonstone additionally provides project-specific recommendations, detects common bad practices, such as empty or inadequate handlers, and provides automatic resolutions, introducing programmers to advanced Java exception handling features, such as try-with- resources. We present findings from two formative studies that informed the design of Moonstone. We then show with a user study that Moonstone improves users’ understanding in certain Fig. 1. Overview of Moonstone’s features. areas and enables developers to amend exception handling code more quickly and correctly. it difficult to assess the behavior of the software system [1]. While novices particularly struggle with exception handling I. -
Programming with Exceptions in Jcilk$
View metadata, citation and similar papers at core.ac.uk brought to you by CORE provided by Elsevier - Publisher Connector Science of Computer Programming 63 (2006) 147–171 www.elsevier.com/locate/scico Programming with exceptions in JCilk$ John S. Danaher, I.-Ting Angelina Lee∗, Charles E. Leiserson Computer Science and Artificial Intelligence Laboratory, Massachusetts Institute of Technology, Cambridge, MA 02139, USA Received 29 December 2005; received in revised form 7 May 2006; accepted 18 May 2006 Available online 25 September 2006 Abstract JCilk extends the serial subset of the Java language by importing the fork-join primitives spawn and sync from the Cilk multithreaded language, thereby providing call-return semantics for multithreaded subcomputations. In addition, JCilk transparently integrates Java’s exception handling with multithreading by extending the semantics of Java’s try and catch constructs, but without adding new keywords. This extension is “faithful” in that it obeys Java’s ordinary serial semantics when executed on a single processor. When executed in parallel, however, an exception thrown by a JCilk computation causes its sibling computations to abort, which yields a clean semantics in which the enclosing cilk try block need only handle a single exception. The exception semantics of JCilk allows programs with speculative computations to be programmed easily. Speculation is essential in order to parallelize programs such as branch-and-bound or heuristic search. We show how JCilk’s linguistic mechanisms can be used to program the “queens” puzzle and a parallel alpha–beta search. We have implemented JCilk’s semantic model in a prototype compiler and runtime system, called JCilk-1. -
CS 6110 S11 Lecture 15 Exceptions and First-Class Continuations 26 February 2010
CS 6110 S11 Lecture 15 Exceptions and First-Class Continuations 26 February 2010 1 CPS and Strong Typing Now let us use CPS semantics to augment our previously defined FL language translation so that it supports runtime type checking. This time our translated expressions will be functions of ρ and k denoting an environment and a continuation, respectively. The term E[[e]]ρk represents a program that evaluates e in the environment ρ and sends the resulting value to the continuation k. As before, assume that we have an encoding of variable names x and a representation of environments ρ along with lookup and update functions lookup ρ x and update ρ x v. In addition, we want to catch type errors that may occur during evaluation. As before, we use integer tags to keep track of types: 4 4 4 Err = 0 Null = 1 Bool = 2 4 4 4 Num = 3 Tuple = 4 Func = 5 A tagged value is a value paired with its type tag; for example, (0; true). Using these tagged values, we can now define a translation that incorporates runtime type checking: 4 E[[x]]ρk = k (lookup ρ x) 4 E[[b]]ρk = k (Bool; b) 4 E[[n]]ρk = k (Num; n) 4 E[[nil]]ρk = k (Null; 0) 4 E[[(e1; : : : ; en)]]ρk = E[[e1 ]]ρ(λv1 :::: E[[e2 ]]ρ(λv2 :::: [[E ]]en ρ(λvn : k (Tuple; n; (v1; : : : ; vn))))) 4 E[[let x = e1 in e2 ]]ρk = E[[e1 ]]ρ(λp: E[[e2 ]] (update ρ x p) k) 4 E[[λx: e]]ρk = k (Func; λxk: E[[e]](update ρ x x)k) 4 E[[error]]ρk = k (Err; error): Now a function application can check that it is actually applying a function: 4 E[[e0 e1 ]]ρk = E[[e0 ]]ρ(λp: let (t; f) = p in if t =6 Func then error else E[[e1 ]]ρ(λv: fvk)) We can simplify this by defining a helper function check-fn: 4 check-fn = λkp: let (t; f) = p in if t =6 Func then error else kf: The helper function takes in a continuation and a tagged value, checks the type, strips off the tag, and passes the raw (untagged) value to the continuation. -
T. Wolf: on Exceptions As First-Class Objects in Ada 95
On Exceptions as First-Class Objects in Ada 95 Thomas Wolf Paranor AG, Switzerland e–mail: [email protected] Abstract. This short position paper argues that it might gramming languages that show a much better integration be beneficial to try to bring the exception model of of object orientation and exceptions, most notably Java. Ada 95 more in–line with the object–oriented model of In the remainder of this paper, I’ll try to make a case programming. In particular, it is felt that exceptions — being such an important concept for the development of for better integrating exception into the object–oriented fault–tolerant software — have deserved a promotion to paradigm in Ada 95. Section 2 discusses some problems first–class objects of the language. It is proposed to with the current model of exceptions. Section 3 argues resolve the somewhat awkward dichotomy of exceptions for an improved exception model that would solve these and exception occurrences as it exists now in Ada 95 by problems, and section 4 presents a brief summary. treating exceptions as (tagged) types, and exception occurrences as their instances. 2 Exceptions in Ada 95 Keywords. Object–Oriented Exception Handling, Soft- ware Fault Tolerance Exceptions in Ada 95 are seen as some kind of “special objects”: even their declaration looks more like that of an object than a type declaration: 1 Introduction Some_Exception : exception; The exception model of Ada 95 [ARM95] is still basi- Such exceptions can be raised (or thrown, as other lan- cally the same as it was in the original Ada 83 program- guages call it) through a raise statement: ming language; although some improvements did occur: raise Some_Exception; the introduction of the concept of exception occurrences, and some other, minor improvements concerning excep- or through a procedure that passes a string message tion names and messages. -
Engineering Java Byte Code to Detect Exception Information Flow
UNIVERSIDADE FEDERAL DO RIO GRANDE DO SUL INSTITUTO DE INFORMÁTICA CURSO DE BACHARELADO EM CIÊNCIA DA COMPUTAÇÃO DENYS HUPEL DA SILVA OLIVEIRA Engineering Java Byte Code to Detect Exception Information Flow Bachelor Thesis Dr. Raul Fernando Weber Advisor Porto Alegre, July 2010 CIP – CATALOGING-IN-PUBLICATION Oliveira, Denys Hupel da Silva Engineering Java Byte Code to Detect Exception Information Flow / Denys Hupel da Silva Oliveira. – Porto Alegre: PPGC da UFRGS, 2010. 41 f.: il. Final Report (Bachelor) – Universidade Federal do Rio Grande do Sul. Curso de Bacharelado em Ciência da Com- putação, Porto Alegre, BR–RS, 2010. Advisor: Raul Fernando Weber. 1. Information flow. 2. Java byte code. 3. Exception flow. 4. Static analysis. 5. Usage control. I. Weber, Raul Fernando. II. Título. UNIVERSIDADE FEDERAL DO RIO GRANDE DO SUL Reitor: Prof. Carlos Alexandre Netto Vice-Reitor: Prof. Rui Vicente Oppermann Pró-Reitora de Graduação: Profa. Valquiria Link Bassani Diretor do Instituto de Informática: Prof. Flávio Rech Wagner Coordenador do CIC: Prof. João César Netto Bibliotecária-Chefe do Instituto de Informática: Beatriz Regina Bastos Haro “The wisest mind has something yet to learn.” —GEORGE SANTAYANA ACKNOWLEDGEMENTS I would like to thank my family, who was always there for me, no matter good or bad times, always giving support and important advices. Many thanks to my friends(old ones and some met at the university), who gave me great moments during my graduation, helped me when necessary, and also understood times that I was very busy with university stuff. And I would also like to thank Raul Weber, who was my advisor in this thesis, and helped me whenever necessary. -
Mopping up Exceptions S
MOPping up Exceptions S. E. Mitchell, A. Burns and A. J. Wellings, Department of Computer Science, University of York, UK Abstract: This paper describes the development of a model for the reflective treatment of both application and environmentally sourced exceptions. We show how a variety of exception models can be implemented using an exception handler at the metalevel. The approach described allows for better separation of exceptional and normal error-free program code producing systems that are easier to understand and therefore maintain. Keywords: metalevel architecture, reflection, exceptions. 1 Introduction with particular emphasis on the relationship between concurrency and reflective The principle reason for the inclusion of exceptions. Finally, we evaluate the exception handling mechanisms in effectiveness of the work with respect to the programming languages is a desire to success of reflection in producing the separate error handling from the programs required separation of concerns. normal operation [Burns and Wellings, 1996]. This is in line with the justification 1.1 Non-reflective Exception Models for the use of reflection in system design – in that its use aids in the separation of The exception model that we explore within concerns. this paper is derived from the common non- reflective one of exceptions being raised Despite this desire for a separation, in many (thrown) and subsequently (possibly) caught approaches the handler code is still within a separate section of code (termed the intermingled with application code, albeit exception handler) usually at the end of an moved to the end of a program block. In this exception block. Exceptions that are not paper we explore the use of reflective caught by any exception handler currently principles to complete the separation of within scope are propagated back up to the concerns by attempting to operate on previous level – the caller – and the search exceptions at the metalevel. -
Finding Error-Handling Bugs in Systems Code Using Static Analysis∗
Finding Error-Handling Bugs in Systems Code Using Static Analysis∗ Cindy Rubio-González Ben Liblit Computer Sciences Department, University of Wisconsin–Madison 1210 W Dayton St., Madison, Wisconsin, United States of America {crubio, liblit}@cs.wisc.edu ABSTRACT error handling. Exceptional conditions must be considered Run-time errors are unavoidable whenever software interacts during all phases of software development [18], introducing with the physical world. Unchecked errors are especially interprocedural control flow that can be difficult to reason about pernicious in operating system file management code. Tran- [3, 19, 22]. As a result, error-handling code is usually scattered sient or permanent hardware failures are inevitable, and error- across different functions and files, making software more com- management bugs at the file system layer can cause silent, plex and less reliable. unrecoverable data corruption. Furthermore, even when devel- Modern programming languages such as Java, C++ and C# opers have the best of intentions, inaccurate documentation can provide exception-handling mechanisms. On the other hand, mislead programmers and cause software to fail in unexpected C does not have explicit exception-handling support, thus pro- ways. grammers have to emulate exceptions in a variety of ways. We use static program analysis to understand and make er- The return-code idiom is among the most popular idioms used ror handling in large systems more reliable. We apply our in large C programs, including operating systems. Errors are analyses to numerous Linux file systems and drivers, finding represented as simple integer codes, where each integer value hundreds of confirmed error-handling bugs that could lead to represents a different kind of error. -
Exception Handlers As Extensible Cases
Exception Handlers as Extensible Cases Matthias Blume Umut A. Acar Wonseok Chae Toyota Technological Institute at Chicago fblume,umut,[email protected] Abstract. Exceptions are an indispensable part of modern program- ming languages. They are, however, handled poorly, especially by higher- order languages such as Standard ML and Haskell: in both languages a well-typed program can unexpectedly fail due to an uncaught exception. In this paper, we propose a technique for type-safe exception handling. Our approach relies on representing exceptions as sums and assigning exception handlers polymorphic, extensible row types. Based on this rep- resentation, we describe an implicitly typed external language EL where well-typed programs do not raise any unhandled exceptions. EL relies on sums, extensible records, and polymorphism to represent exception- handling, and its type system is no more complicated than that for ex- isting languages with polymorphic extensible records. EL is translated into an internal language IL that is a variant of Sys- tem F extended with extensible records. The translation performs a CPS transformation to represent exception handlers as continuations. It also relies on duality to transform sums into records. (The details for this translation are given in an accompanying technical report.) We describe the implementation of a compiler for a concrete language based on EL. The compiler performs full type inference and translates EL-style source code to machine code. Type inference relieves the pro- grammer from having to provide explicit exception annotations. We be- lieve that this is the first practical proposal for integrating exceptions into the type system of a higher-order language. -
Spc564axx/Rpc564axx/Spc56elxx/Rpc56elxx Devices Exception Handling and Single/Double Bit Error
AN4417 Application note SPC564Axx/RPC564Axx/SPC56ELxx/RPC56ELxx devices Exception handling and single/double bit error Introduction This document provides an overview of SPC564Axx/RPC564Axx/SPC56ELxx/RPC56ELxx exception handling with main focus on different kinds of exception that the application code may face during the runtime like single and double bit errors in memories, MPU protection violation, AIPS access protection violation and others. It starts with the simple overview of Machine check interrupt highlighting important things from an application perspective. To get detailed view and to implement low level machine check interrupt handler, it is necessary to use Z4 Core User Manual which describes all the details about the Core exception and interrupts. The following part describes the reason of the exception, how to find it and what possibilities exist to remove the fault. October 2015 DocID025623 Rev 3 1/22 www.st.com Contents AN4417 Contents 1 Z4 Core exception overview . 5 1.1 Machine check interrupt (IVOR1) . 5 1.1.1 Machine check registers . 5 2 Machine check handler . 8 2.1 Low level handler . 8 2.1.1 Start phase . 9 2.1.2 Final phase . 9 2.1.3 Modification of the MCSRR0 register . 9 2.2 User handler . 10 3 SPC564Axx/RPC564Axx/SPC56ELxx/RPC56ELxx exception cases . 12 3.1 Flash 2b ECC error . 12 3.1.1 Cause of the exception . 12 3.1.2 Machine check exception status . 13 3.1.3 Flash 2b ECC error detection by ECSM . 13 3.1.4 ECSM_ESR.FNCE implementation note for SPC564A70 device only . 14 3.1.5 ECSM_ESR implementation for SPC56ELx/RPC56ELx devices only . -
Principles of Programming Languages Course Review
CSE 307: Principles of Programming Languages Course Review R. Sekar 1 / 57 Course Topics Introduction and History Syntax Values and types Names, Scopes and Bindings Variables and Constants Expressions Statements and Control-flow Procedures, Parameter Passing Runtime Environments Object-Oriented Programming Functional Programming Logic Programming 2 / 57 Programming Language A notational system for describing computation in a machine and human readable form Human readability requires: abstractions data abstractions, e.g., primitive and compound, structured data types, encapsulation, ... control abstractions, e.g., structured statements (if, switch, loops,...) procedure abstractions promotes reuse and maintainability 3 / 57 Computational Paradigms Imperative: characterized by variable assignment and sequential execution procedural object-oriented Declarative: Focus on specifying what, reduced emphasis on how. No variable assignment or sequential execution. functional logic 4 / 57 History of Programming Languages (1) 1940s: Programming by “wiring,” machine languages, assembly languages 1950s: FORTRAN, COBOL, LISP, APL 1960s: PL/I, Algol68, SNOBOL, Simula67, BASIC 1970s: Pascal, C, SML, Scheme, Smalltalk 1980s: Ada, Modula 2, Prolog, C++, Eiel 1990s: Java, Haskell 2000s: Javascript, PHP, Python, ... 5 / 57 History of Programming Languages (2) FORTRAN: the grandfather of high-level languages Emphasis on scientific computing Simple data structures (arrays) Control structures (goto, do-loops, subroutines) ALGOL: where most modern language concepts