Advanced Programming
Total Page:16
File Type:pdf, Size:1020Kb
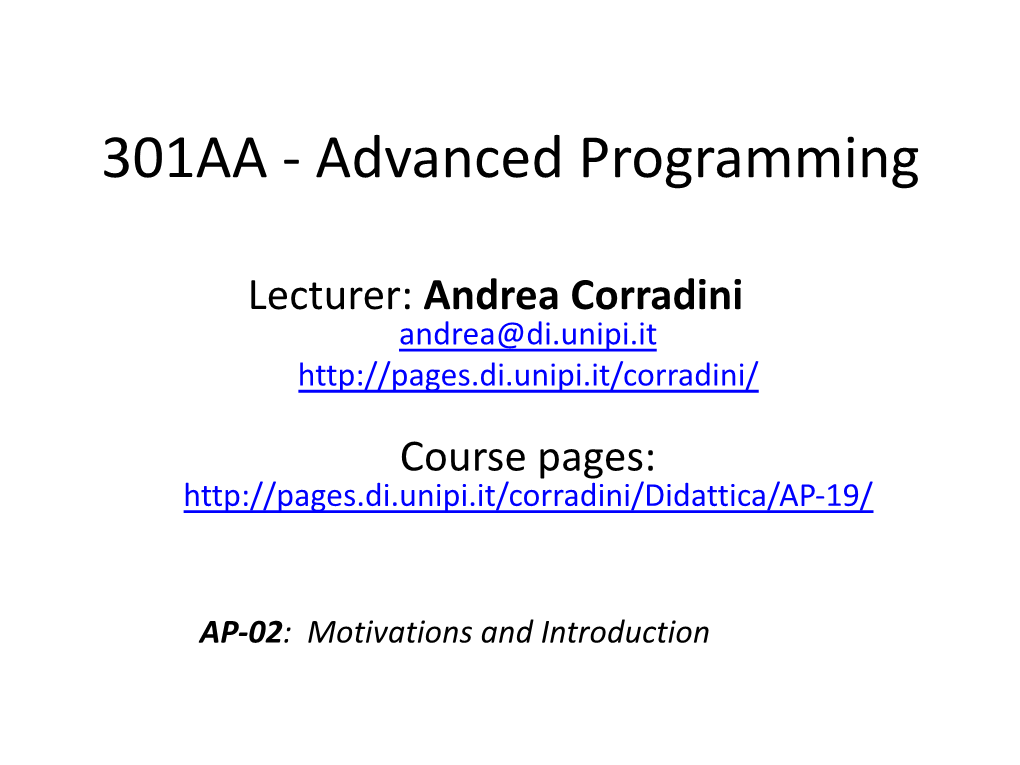
Load more
Recommended publications
-
What Is Spring Framework?
Software Engineering a.a. 2019-2020 Introduction to Spring Framework Prof. Luca Mainetti Università del Salento Roadmap ■ Introduction to Spring ■ Dependency Injection and IoC ■ Bean ■ AoP ■ Module Architecture Introduction to Spring Framework 2 Luca Mainetti What Is Spring Framework? ■ Spring is the most popular application development framework for Java enterprise ■ Open source Java platform since 2003. ■ Spring supports all main application servers and JEE standards ■ Spring handles the infrastructure so you can focus on your application ■ Current version: 5.0.X Introduction to Spring Framework 3 Luca Mainetti What does Spring offer? ■ Dependency Injection – Also known as IoC (Inversion of Control) ■ Aspect Oriented Programming – Runtime injection-based ■ Portable Service Abstractions – The rest of spring • ORM, DAO, Web MVC, Web, etc. • Allows access to these without knowing how they actually work Introduction to Spring Framework 4 Luca Mainetti Dependency Injection ■ The technology that actually defines Spring (Heart of Spring). ■ Dependency Injection helps us to keep our classes as indepedent as possible. – Increase reuse by applying low coupling – Easy testing – More understandable An injection is the passing of a dependency (a service) to a dependent object (a client). Passing the service to the client, rather than allowing a client to build or find the service, is the fundamental requirement of the pattern. Introduction to Spring Framework 5 Luca Mainetti Dependency Injection and Inversion of Control (IoC) In software engineering, inversion of control (IoC) describes a design in which custom-written portions of a computer program receive the flow of control from a generic, reusable library. ■ The Inversion of Control (IoC) is a general concept, and it can be expressed in many different ways and dependency Injection is merely one concrete example of Inversion of Control. -
Ioc Containers in Spring
301AA - Advanced Programming Lecturer: Andrea Corradini [email protected] http://pages.di.unipi.it/corradini/ AP-2018-11: Frameworks and Inversion of Control Frameworks and Inversion of Control • Recap: JavaBeans as Components • Frameworks, Component Frameworks and their features • Frameworks vs IDEs • Inversion of Control and Containers • Frameworks vs Libraries • Decoupling Components • Dependency Injection • IoC Containers in Spring 2 Components: a recap A software component is a unit of composition with contractually specified interfaces and explicit context dependencies only. A software component can be deployed independently and is subject to composition by third party. Clemens Szyperski, ECOOP 1996 • Examples: Java Beans, CLR Assemblies • Contractually specified interfaces: events, methods and properties • Explicit context dependencies: serializable, constructor with no argument • Subject to composition: connection to other beans – Using connection oriented programming (event source and listeners/delegates) 3 Towards Component Frameworks • Software Framework: A collection of common code providing generic functionality that can be selectively overridden or specialized by user code providing specific functionality • Application Framework: A software framework used to implement the standard structure of an application for a specific development environment. • Examples: – GUI Frameworks – Web Frameworks – Concurrency Frameworks 4 Examples of Frameworks Web Application Frameworks GUI Toolkits 5 Examples: General Software Frameworks – .NET – Windows platform. Provides language interoperability – Android SDK – Supports development of apps in Java (but does not use a JVM!) – Cocoa – Apple’s native OO API for macOS. Includes C standard library and the Objective-C runtime. – Eclipse – Cross-platform, easily extensible IDE with plugins 6 Examples: GUI Frameworks • Frameworks for Application with GUI – MFC - Microsoft Foundation Class Library. -
Inversion of Control in Spring – Using Annotation
Inversion of Control in Spring – Using Annotation In this chapter, we will configure Spring beans and the Dependency Injection using annotations. Spring provides support for annotation-based container configuration. We will go through bean management using stereotypical annotations and bean scope using annotations. We will then take a look at an annotation called @Required, which allows us to specify which dependencies are actually required. We will also see annotation-based dependency injections and life cycle annotations. We will use the autowired annotation to wire up dependencies in the same way as we did using XML in the previous chapter. You will then learn how to add dependencies by type and by name. We will also use qualifier to narrow down Dependency Injections. We will also understand how to perform Java-based configuration in Spring. We will then try to listen to and publish events in Spring. We will also see how to reference beans using Spring Expression Language (SpEL), invoke methods using SpEL, and use operators with SpEL. We will then discuss regular expressions using SpEL. Spring provides text message and internationalization, which we will learn to implement in our application. Here's a list of the topics covered in this chapter: • Container configuration using annotations • Java-based configuration in Spring • Event handling in Spring • Text message and internationalization [ 1 ] Inversion of Control in Spring – Using Annotation Container configuration using annotation Container configuration using Spring XML sometimes raises the possibility of delays in application development and maintenance due to size and complexity. To solve this issue, the Spring Framework supports container configuration using annotations without the need of a separate XML definition. -
Monadic Functional Reactive Programming
Monadic Functional Reactive Programming Atze van der Ploeg Centrum Wiskunde & Informatica [email protected] Abstract FRP is based on the notion of a reactive computation: a monadic Functional Reactive Programming (FRP) is a way to program reac- computation which may require the occurrence of external events tive systems in functional style, eliminating many of the problems to continue. The Monadic FRP variant of a signal is a signal that arise from imperative techniques. In this paper, we present an computation: a reactive computation that may also emit values alternative FRP formulation that is based on the notion of a reac- during the computation. tive computation: a monadic computation which may require the This novel formulation has two differences with other FRP occurrence of external events to continue. A signal computation approaches: is a reactive computation that may also emit values. In contrast to • In contrast to signals in other FRP formulations, signal compu- signals in other FRP formulations, signal computations can end, tations can end. This leads to a simple, monadic interface for leading to a monadic interface for sequencing behavioral changes. sequencing behavioral changes. This interface has several advantages: routing is implicit, sequencing • behaviors is easier and more intuitive than the switching combina- In other FRP approaches, either the entire FRP expression is tors found in other FRP approaches, and dynamic lists require much re-evaluated on each external stimulus, or impure techniques less boilerplate code. In other FRP approaches, either the entire are used to prevent redundant re-computations: re-computing FRP expression is re-evaluated on each external stimulus, or impure the current value of signal while the input it depends on has not techniques are used to prevent redundant re-computations. -
The Spring Framework: an Open Source Java Platform for Developing Robust Java Applications
International Journal of Innovative Technology and Exploring Engineering (IJITEE) ISSN: 2278-3075, Volume-3 Issue-2, July 2013 The Spring Framework: An Open Source Java Platform for Developing Robust Java Applications Dashrath Mane, Ketaki Chitnis, Namrata Ojha Abstract— The fundamental concepts of Spring Framework is Supported deployment platforms range from standalone presented in this paper.Spring framework is an open source Java applications to Tomcat and Java EE servers such as platform that provides comprehensive infrastructure support for WebSphere. Spring is also a first-class citizen on major developing robust Java applications very easily and very rapidly. cloud platforms with Java support, e.g. on Heroku, Google The Spring Framework is a lightweight solution and a potential App Engine, Amazon Elastic Beanstalk and VMware's one-stop-shop for building your enterprise-ready applications. Cloud Foundry.[1] IndexTerms— Aspect Oriented Programming, Dependency Injection, IoC Container, ORM. II. SPRING FRAMEWORK ARCHITECTURE Spring could potentially be a one-stop shop for all your I. INTRODUCTION enterprise applications; however, Spring is modular, Spring is the most popular application development allowing you to pick and choose which modules are framework for enterprise Java. Millions of developers applicable to you, without having to bring in the rest. around the world use Spring Framework to create high The Spring Framework provides about 20 modules which performing, easily testable, reusable code. Spring can be used based on an application requirement. framework is an open source Java platform and it was initially written by Rod Johnson and was first released under the Apache 2.0 license in June 2003. -
An Introduction to Dependency Inversion Page 1 of 12
Peruse Muse Infuse: An Introduction to Dependency Inversion Page 1 of 12 An Introduction to Dependency Inversion Originaly posted by AdrianK, 1-Nov-2010 at: http://www.morphological.geek.nz/blogs/viewpost/Peruse Muse Infuse/An Introduction to Dependency Inversion.aspx Tagged under: Architecture, Web Development, Patterns An Introduction to Dependency Inversion This article is for anyone (mainly developers, and specifically .Net developers) who is unfamiliar with Dependency Inversion or design patterns in general. The basic rationale behind the Dependency Inversion Principle [1] (DIP, or simply DI) is very simple; and like most other simple design principles it is suitable in many different situations. Once you understand it you’ll wonder how you managed without it. The key benefit it brings is control over dependencies – by removing them (or perhaps more correctly – by “inverting” them), and this isn’t just limited to “code” – you’ll find this also opens up new opportunities regarding how you can structure and run the actual development process. Implementing DI and is fairly straight forward and will often involve other design patterns, such as the Factory pattern [2] and Lazy Load pattern [3] ; and embodies several other principles including the Stable Abstractions Principle and Interface Segregation Principle. I’ll be using acronyms where I can, here’s a quick glossary for them. • BL: Business Logic, sometimes referred to as the Business Logic Layer. • DA: Data Access, often referred to as the DAL or Data Access Layer. • DI: can refer to either Dependency Inversion or Dependency Injection – both of which (at least for this article) essentially mean the same thing. -
Isolated Actors for Race-Free Concurrent Programming
Isolated Actors for Race-Free Concurrent Programming THÈSE NO 4874 (2010) PRÉSENTÉE LE 26 NOVEMBRE 2010 À LA FACULTÉ INFORMATIQUE ET COMMUNICATIONS LABORATOIRE DE MÉTHODES DE PROGRAMMATION 1 PROGRAMME DOCTORAL EN INFORMATIQUE, COMMUNICATIONS ET INFORMATION ÉCOLE POLYTECHNIQUE FÉDÉRALE DE LAUSANNE POUR L'OBTENTION DU GRADE DE DOCTEUR ÈS SCIENCES PAR Philipp HALLER acceptée sur proposition du jury: Prof. B. Faltings, président du jury Prof. M. Odersky, directeur de thèse Prof. D. Clarke, rapporteur Prof. V. Kuncak, rapporteur Prof. P. Müller, rapporteur Suisse 2010 ii Abstract Message-based concurrency using actors has the potential to scale from multi- core processors to distributed systems. However, several challenges remain until actor-based programming can be applied on a large scale. First, actor implementations must be efficient and highly scalable to meet the demands of large-scale distributed applications. Existing implementations for mainstream platforms achieve high performance and scalability only at the cost of flexibility and ease of use: the control inversion introduced by event-driven designs and the absence of fine-grained message filtering complicate the logic of application programs. Second, common requirements pertaining to performance and interoperability make programs prone to concurrency bugs: reusing code that relies on lower-level synchronization primitives may introduce livelocks; passing mutable messages by reference may lead to data races. This thesis describes the design and implementation of Scala Actors. Our sys- tem offers the efficiency and scalability required by large-scale production sys- tems, in some cases exceeding the performance of state-of-the-art JVM-based ac- tor implementations. At the same time, the programming model (a) avoids the control inversion of event-driven designs, and (b) supports a flexible message re- ception operation. -
Copyrighted Material
INDEX Symbols AnyVal class, 16–17, 18, 142 value classes and, 143–145 $ ApiKey class, 16 for printing variable value, 9–10 Applicative Functor, 170–172 in Scaladoc query, 99 areEqual method, 147, 149–150 ... (wildcard operator), 8 ArrayBuffer, implicit conversions into, 37–38 @ (at sign), for tags, 117 asynchronous call, 185 -> syntax, 11 at sign (@), for tags, 117 ATDD (Acceptance Test-Driven Development), 86 Atomic Variables, 183 A atto library, 70 abstract type members, 159–161 @author, 119, 120 abstract value members, 105 automatic conversions between types, implicits Acceptance Test-Driven Development (ATDD), for, 4 86 acceptance tests, 85, 90–92 actors (Akka), 198–200 B ad hoc polymorphism, 145, 146–149 add() function, 33 BDD (Business-Driven Development), 86 ADTs (Algebraic Data Types), case classes for, Big Data assets, Spark for, 200–202 6–7 Bloch, Joshua, Effective Java, 147 Akka (actors), 198–200 block elements, for documentation structure, Akka-streams, 192 110–113 Algebraic Data Types (ADTs), case classes for, Boolean, methods returning, 31 6–7 bounds, 149–155 alias, for tuple construction, 11 Bower dependencies, 212 Amazon Web ServicesCOPYRIGHTED S3, 134 Bower MATERIAL packages, 211 ammonite-repl, 62 Brown, Travis, 160 annotations, 117 build tool, integrating Scaladoc creation with, 133 self-type, 155–157 build.sbt fi les, 47 anonymous function, .map to apply to collection dependencies for, 86 elements, 10 locations of, 52 Any class, 142 specifying sub-projects, 52–53 Any type, 17 buildSettings, breakup of, 48 AnyRef class, 17, 18, 142, 143 bytecode, 188 215 Cake Pattern – documentation C context bounds, 149–150 contravariant type A occurs.. -
Type-Safe Polyvariadic Event Correlation 3
Type-safe Polyvariadic Event Correlation OLIVER BRAČEVAC, TU Darmstadt, Germany GUIDO SALVANESCHI, TU Darmstadt, Germany SEBASTIAN ERDWEG, Johannes Gutenberg University Mainz, Germany MIRA MEZINI, TU Darmstadt, Germany The pivotal role that event correlation technology plays in todays applications has lead to the emergence of different families of event correlation approaches with a multitude of specialized correlation semantics, including computation models that support the composition and extension of different semantics. However, type-safe embeddings of extensible and composable event patterns into statically-typed general- purpose programming languages have not been systematically explored so far. This is unfortunate, as type- safe embedding of event patterns is important to enable increased correctness of event correlation computa- tions as well as domain-specific optimizations. Event correlation technology has often adopted well-known and intuitive notations from database queries, for which approaches to type-safe embedding do exist. How- ever, we argue in the paper that these approaches, which are essentially descendants of the work on monadic comprehensions, are not well-suited for event correlations and, thus, cannot without further ado be reused/re- purposed for embedding event patterns. To close this gap we propose PolyJoin, a novel approach to type-safe embedding for fully polyvariadic event patterns with polymorphic correlation semantics. Our approach is based on a tagless final encoding with uncurried higher-order abstract syntax (HOAS) representation of event patterns with n variables, for arbitrary n ∈ N. Thus, our embedding is defined in terms of the host language without code generation and exploits the host language type system to model and type check the type system of the pattern language. -
Dependency Injection in Practice
Dependency Injection in practice Kristijan Horvat Software Architect twitter.com/khorvat2 What is Dependency Injection (DI) Dependency injection is a software design pattern that implements inversion of control for software libraries. Wikipedia Dependency Injection is a set of software design principles and patterns that enable us to develop loosely coupled code. Mark Seemann What is Inversion of Control (IoC) Inversion of Control (IoC) describes a design in which custom- written portions of a computer program receive the flow of control from a generic, reusable library. Wikipedia Inversion of Control is a common pattern among developers that helps assemble components from different projects into a cohesive application. ~ based on http://www.martinfowler.com/articles/injection.html (reworded). Martin Fowler When to use DI & IoC ? High Level perspective - when your software stack supports DI & IoC - when you have mid to large projects or teams - when we have long running projects - when your project tend to change over time (Agile) - when you plan to use unit testing - when you plan to deploy projects to cloud (Amazon, Azure, etc.) When to use DI & IoC ? Low Level perspective - when we want to avoid tight coupling of objects - when we need to easily add new functionality to code (Extensibility) - when we need to choose what components we use at run-time rather than compile- time (Late binding) - when we want multiple development teams to work on the same project (Parallel Development) - when we want independent components and isolated -
Event-Based Programming Without Inversion of Control
Event-Based Programming without Inversion of Control Philipp Haller, Martin Odersky Ecole Polytechnique Federalede Lausanne (EPFL) 1015 Lausanne, Switzerland 1 Introduction Concurrent programming is indispensable. On the one hand, distributed and mobile environments naturally involve concurrency. On the other hand, there is a general trend towards multi-core processors that are capable of running multiple threads in parallel. With actors there exists a computation model which is especially suited for con- current and distributed computations [16,1]. Actors are basically concurrent pro- cesses which communicate through asynchronous message passing. When com- bined with pattern matching for messages, actor-based process models have been proven to be very e ective, as the success of Erlang documents [3,25]. Erlang [4] is a dynamically typed functional programming language designed for programming real-time control systems. Examples of such systems are tele- phone exchanges, network simulators and distributed resource controllers. In these systems, large numbers of concurrent processes can be active simultane- ously. Moreover, it is generally dicult to predict the number of processes and their memory requirements as they vary with time. For the implementation of these processes, operating system threads and threads of virtual machines, such as the Java Virtual Machine [22], are usually too heavy- weight. The main reasons are: (1) Over-provisioning of stacks leads to quick ex- haustion of virtual address space and (2) locking mechanisms often lack suitable contention managers [12]. Therefore, Erlang implements concurrent processes by its own runtime system and not by the underlying operating system [5]. Actor abstractions as lightweight as Erlang's processes have been unavailable on popular virtual machines so far. -
The Scala Experiment – Can We Provide Better Language Support for Component Systems?
The Scala Experiment – Can We Provide Better Language Support for Component Systems? Martin Odersky, EPFL Invited Talk, ACM Symposium on Programming Languages and Systems (POPL), Charleston, South Carolina, January 11, 2006. 1 Component Software – State of the Art In principle, software should be constructed from re-usable parts (“components”). In practice, software is still most often written “from scratch”, more like a craft than an industry. Programming languages share part of the blame. Most existing languages offer only limited support for components. This holds in particular for statically typed languages such as Java and C#. 2 How To Do Better? Hypothesis 1: Languages for components need to be scalable; the same concepts should describe small as well as large parts. Hypothesis 2: Scalability can be achieved by unifying and generalizing functional and object-oriented programming concepts. 3 Why Unify FP and OOP? Both have complementary strengths for composition: Functional programming: Makes it easy to build interesting things from simple parts, using • higher-order functions, • algebraic types and pattern matching, • parametric polymorphism. Object-oriented programming: Makes it easy to adapt and extend complex systems, using • subtyping and inheritance, • dynamic configurations, • classes as partial abstractions. 4 An Experiment To validate our hypotheses we have designed and implemented a concrete programming language, Scala. An open-source distribution of Scala has been available since Jan 2004. Currently: ≈ 500 downloads per month. Version 2 of the language has been released 10 Jan 2006. A language by itself proves little; its applicability can only be validated by practical, serious use. 5 Scala Scala is an object-oriented and functional language which is completely interoperable with Java and .NET.