Miniwebcoach
Total Page:16
File Type:pdf, Size:1020Kb
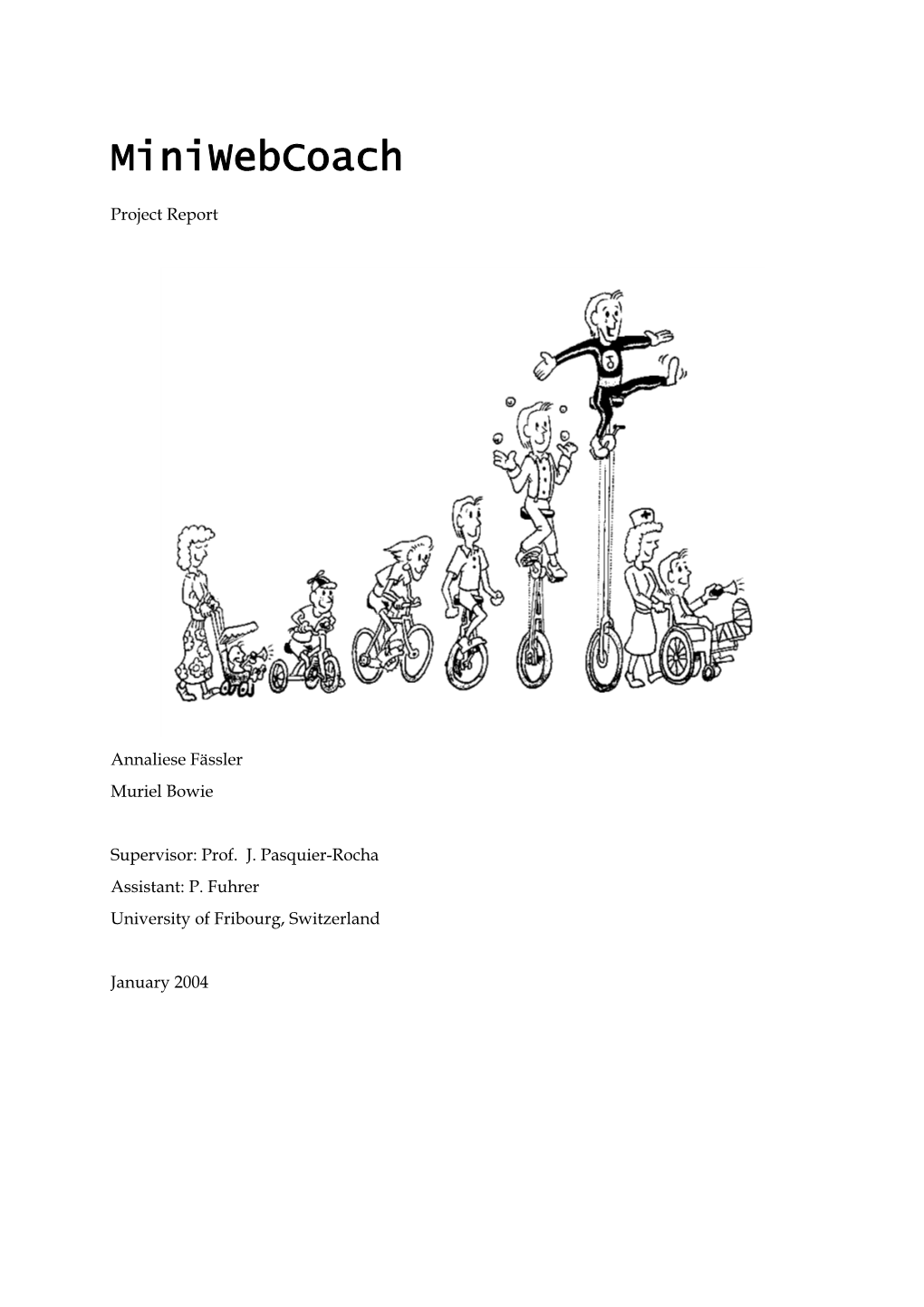
Load more
Recommended publications
-
(19) United States (12) Patent Application Publication (10) Pub
US 20110191743Al (19) United States (12) Patent Application Publication (10) Pub. No.: US 2011/0191743 A1 Cope et al. (43) Pub. Date: Aug. 4, 2011 (54) STACK MACROS AND PROJECT Publication Classi?cation EXTENSIBILITY FOR PROJECT STACKING (51) Int Cl AND SUPPORT SYSTEM G06F 9/44 (200601) (75) Inventors: Rod Cope, Broom?eld, CO (US); ( 52 ) US. Cl. ...................................................... .. 717/101 Eric Weidner, Highlands Ranch, (57) ABSTRACT CO (Us) A tool is provided for addressing a number of issues related to _ assembling software stacks including multiple uncoordinated (73) Asslgnee? OPENLOGIC, INC-i B1" Oom?eld, components such as open source projects. The tool identi?es CO (Us) individual projects for stacking, manages dependency rela tionships and provides an intuitive graphical interface to (21) App1_ NO_; 11/556,094 assist a user. A project ?lter is also provided for controlling access to or installation of projects in accordance With ?lter (22) Filed. N0“ 2 2006 criteria. In this manner, compliance With internal policies ' ’ regarding the use of open source or other software is facili . tated. The user can also add projects to the collection of Related U's' Apphcatlon Data supported projects and de?ne stack macros or stacros. Once (60) Provisional application No. 60/732,729, ?led on Nov. such stacros are de?ned, various functionality can be pro 2, 2005' vided analogous to that provided for individual projects. 1208 1210 1212 USER PARAMETERS USE PARAMETERS LICENSE PARAMETERS 1200 l A l / USER INTERFACE # ; FILTERS _ V USER SYSTEM 1204 1 202 1 21 4 PROJECTS 1206 Patent Application Publication Aug. -
Developing Java™ Web Applications
ECLIPSE WEB TOOLS PLATFORM the eclipse series SERIES EDITORS Erich Gamma ■ Lee Nackman ■ John Wiegand Eclipse is a universal tool platform, an open extensible integrated development envi- ronment (IDE) for anything and nothing in particular. Eclipse represents one of the most exciting initiatives hatched from the world of application development in a long time, and it has the considerable support of the leading companies and organ- izations in the technology sector. Eclipse is gaining widespread acceptance in both the commercial and academic arenas. The Eclipse Series from Addison-Wesley is the definitive series of books dedicated to the Eclipse platform. Books in the series promise to bring you the key technical information you need to analyze Eclipse, high-quality insight into this powerful technology, and the practical advice you need to build tools to support this evolu- tionary Open Source platform. Leading experts Erich Gamma, Lee Nackman, and John Wiegand are the series editors. Titles in the Eclipse Series John Arthorne and Chris Laffra Official Eclipse 3.0 FAQs 0-321-26838-5 Frank Budinsky, David Steinberg, Ed Merks, Ray Ellersick, and Timothy J. Grose Eclipse Modeling Framework 0-131-42542-0 David Carlson Eclipse Distilled 0-321-28815-7 Eric Clayberg and Dan Rubel Eclipse: Building Commercial-Quality Plug-Ins, Second Edition 0-321-42672-X Adrian Colyer,Andy Clement, George Harley, and Matthew Webster Eclipse AspectJ:Aspect-Oriented Programming with AspectJ and the Eclipse AspectJ Development Tools 0-321-24587-3 Erich Gamma and -
Soumaya HACHICHA Formation Expériences Professionnelles
Soumaya HACHICHA Ingénieur Informatique Née le : 13/11/1987 Mariée Sakiet Eddaier km 6, Rue cheikh Salemi N°60, 3011, Sfax, Tunisie (+216)53475698 @ [email protected] Formation 2008-2011 Cycle Ingénieur en informatique à l’Ecole Nationale d’Ingénieurs de Sfax, option ISI (Ingénierie des Systèmes Intelligents) Etude préparatoire en Mathématiques-Physiques - Institut 2006-2008 préparatoires aux études d’ingénieurs à Sfax 2005-2006 Baccalauréat, Science Mathématiques, Mention « Bien » Expériences professionnelles Période Sujet, outils et langages utilisés Recherche académique : Compression des maillages 3D multirésolution Depuis 2014 basée GPU Enseignement : Enseignante à l’ISET de Sidi Bouzid. 2011-2012 Matière enseignées : HTML (cours intégré et Tps), JavaScript (Tps), Commerce électronique (cours), 3P (suivie et direction des étudiants dans la réalisation de leurs projets). Sujet : Logiciel de contrôle d’accès et des présences des employés à 2011 distance en utilisant l’empreinte digitale comme étant une technique biométrique d’identification (Stage Projet Fin d’Etudes), Mention « Très 1 Bien » Outils : Visual C++ 2005, SQL SERVER 2008 Langages : C++, C++/CLI Sujet : Reconnaissance des caractères (Mini-projet) 2010 Outils : Visual C++ Langages : C++ - Stage technicien (1 mois) Sujet : Développement d’application de gestion de réservation dans un 2009-2010 hôtel (Projet Fin d’Année) Outils : SQL Server 2005, Delphi 2009 Langages : Delphi Eté 2009 Sujet : Stage ouvrier (1 mois) Sujet : Développement d’un site web de réservation -
Component-Based Development
Component-Based Development 2004-2005 Marco Scotto ([email protected]) Component-Based Development Outline ¾Introduction to Eclipse • Plug-in Architecture •Platform •JDT •PDE ¾HelloWorld Example ¾PDE Views Component-Based Development 2 Eclipse Project Aims ¾ Provide open platform for application development tools • Run on a wide range of operating systems • GUI and non-GUI ¾ Language-neutral • Permit unrestricted content types • HTML, Java, C, JSP, EJB, XML, GIF, … ¾ Facilitate seamless tool integration • At UI and deeper • Add new tools to existing installed products ¾ Attract community of tool developers • Including independent software vendors (ISVs) • Capitalize on popularity of Java for writing tools Component-Based Development 3 Eclipse Overview Another Eclipse Platform Tool Java Workbench Help Development Tools JFace (JDT) SWT Team Your Tool Plug-in Workspace Development Debug Environment (PDE) Their Platform Runtime Tool Eclipse Project Component-Based Development 4 Eclipse Origins ¾ Eclipse created by OTI and IBM teams responsible for IDE products • IBM VisualAge/Smalltalk (Smalltalk IDE) • IBM VisualAge/Java (Java IDE) • IBM VisualAge/Micro Edition (Java IDE) ¾ Initially staffed with 40 full-time developers ¾ Geographically dispersed development teams • OTI Ottawa, OTI Minneapolis, OTI Zurich, IBM Toronto, OTI Raleigh, IBM RTP, IBM St. Nazaire (France) ¾ Effort transitioned into open source project • IBM donated initial Eclipse code base Platform, JDT, PDE Component-Based Development 5 Brief History of Eclipse 1999 April -
Objectweb Consortium Sustainable Development of Next Generation Software Infrastructure
ObjectWeb Consortium Sustainable Development of Next Generation Software Infrastructure. Club Irisatech - February 4th, 2005. Christophe Ney ObjectWeb Executive Director Development Project Director, INRIA Rhône-Alpes Legal redistribution under the licensing terms of Creative Commons Attribution NonCommercial-NoDerivs 2.0 - © 2005 INRIA/ObjectWeb Agenda . ObjectWeb : A consortium to bring open-source middleware to the heart of the main stream market. JOnAS : ObjectWeb’s implementation of the J2EE specifications . ObjectWeb and Open-Source in Next Generation of GRID Software ObjectWeb Consortium NG Software Infrastructure e-business Middleware and Grid Software e-administration . Abstract network resources e-…. Ease development, deployment, management of distributed apps . Service Oriented Architecture . Convergence between Business/Computing Network . Stake for the future of Information Society Software Infrastructure is critical for everyone “… economic and social life becomes dependent upon a common computing infrastructure.” Professor Siobhan O’Mahony, Harvard Business School Software Infrastructure is shared by everybody Software Infrastructure “offers far more value when shared than when used in isolation” Nicholas Carr in “IT Doesn’t Matter”, Harvard Business Review ObjectWeb Consortium […] The importance of Software production goes beyond industrial and economic reasons. In highly-connected environments with pervasive computing, social, ethical and legal issues will have an ever increasing significance. The implementation of regulations -
Beginning Pojo.Pdf
Sam-Bodden_596-3 FRONT.fm Page i Friday, February 24, 2006 9:59 AM Beginning POJOs From Novice to Professional ■■■ Brian Sam-Bodden Sam-Bodden_596-3 FRONT.fm Page ii Friday, February 24, 2006 9:59 AM Beginning POJOs: From Novice to Professional Copyright © 2006 by Brian Sam-Bodden All rights reserved. No part of this work may be reproduced or transmitted in any form or by any means, electronic or mechanical, including photocopying, recording, or by any information storage or retrieval system, without the prior written permission of the copyright owner and the publisher. ISBN-13 (pbk): 978-159059-596-1 ISBN-10 (pbk): 1-59059-596-3 Printed and bound in the United States of America 9 8 7 6 5 4 3 2 1 Trademarked names may appear in this book. Rather than use a trademark symbol with every occurrence of a trademarked name, we use the names only in an editorial fashion and to the benefit of the trademark owner, with no intention of infringement of the trademark. Lead Editor: Steve Anglin Technical Reviewer: Dilip Thomas Editorial Board: Steve Anglin, Dan Appleman, Ewan Buckingham, Gary Cornell, Jason Gilmore, Jonathan Hassell, James Huddleston, Chris Mills, Matthew Moodie, Dominic Shakeshaft, Jim Sumser, Matt Wade Project Manager: Kylie Johnston Copy Edit Manager: Nicole LeClerc Copy Editor: Hastings Hart Assistant Production Director: Kari Brooks-Copony Production Editor: Katie Stence Compositor: Susan Glinert Proofreader: Lori Bring Indexer: Michael Brinkman Artist: April Milne Cover Designer: Kurt Krames Manufacturing Director: Tom Debolski Distributed to the book trade worldwide by Springer-Verlag New York, Inc., 233 Spring Street, 6th Floor, New York, NY 10013. -
Développons En Java Avec Eclipse
Développons en Java avec Eclipse Jean Michel DOUDOUX Table des matières Développons en Java avec Eclipse.....................................................................................................................1 Préambule............................................................................................................................................................2 A propos de ce document.........................................................................................................................2 Note de licence.........................................................................................................................................3 Marques déposées....................................................................................................................................3 Historique des versions............................................................................................................................3 Partie 1 : les bases pour l'utilisation d'Eclipse.................................................................................................5 1. Introduction.....................................................................................................................................................6 1.1. Les points forts d'Eclipse..................................................................................................................6 1.2. Les différentes versions d'Eclipse.....................................................................................................7 -
Web Tools Platform (WTP) 3.5
Web Tools Platform (WTP) 3.5 for the Kepler Simultaneous Release Review Full Release Review Materials June 6, 2013 Prepared by Chuck Bridgham and sub-project leads Table of Contents Introduction and Purpose ................................................................................................ 3 History............................................................................................................................. 3 Project Organization ....................................................................................................... 3 PMC Organization .......................................................................................................... 4 WTP 3.5 Goals and Plans ............................................................................................... 4 Features ........................................................................................................................... 5 Common Tools ................................................................................................................ 5 Server Tools .................................................................................................................... 5 JavaScript Development Tools (JSDT) ........................................................................... 6 Source Editing ................................................................................................................. 6 Web Service Tools.......................................................................................................... -
Web Tools Platform (WTP)
Web Tools Platform (WTP) 3.10 for the Photon Simultaneous Release Review Full Release Review Materials June 13, 2018 Prepared by Nitin Dahyabhai and sub-project leads Table of Contents Introduction and Purpose.................................................................................................2 History..............................................................................................................................2 Project Organization........................................................................................................2 PMC Organization...........................................................................................................3 WTP 3.10 Goals and Plans................................................................................................3 Features............................................................................................................................3 Common Tools.................................................................................................................4 Server Tools......................................................................................................................4 JavaScript Development Tools (JSDT)............................................................................4 Source Editing..................................................................................................................4 Web Service Tools............................................................................................................5 -
Eclipse Project Briefing Materials
[________________________] Eclipse project briefing materials. Copyright (c) 2002, 2003 IBM Corporation and others. All rights reserved. This content is made available to you by Eclipse.org under the terms and conditions of the Common Public License Version 1.0 ("CPL"), a copy of which is available at http://www.eclipse.org/legal/cpl-v10.html The most up-to-date briefing materials on the Eclipse project are found on the eclipse.org website at http://eclipse.org/eclipse/ 200303331 1 [________________________] Revision history: Mar. 31, 2003 – Updated for 2.1; new board members Sept. 4, 2002 – Initial version by Jim des Rivières (OTI) 200303331 2 EclipseEclipse ProjectProject 200303331 3 Eclipse Project Aims ■ Provide open platform for application development tools – Run on a wide range of operating systems – GUI and non-GUI ■ Language-neutral – Permit unrestricted content types – HTML, Java, C, JSP, EJB, XML, GIF, … ■ Facilitate seamless tool integration – At UI and deeper – Add new tools to existing installed products ■ Attract community of tool developers – Including independent software vendors (ISVs) – Capitalize on popularity of Java for writing tools 200303331 4 Eclipse Overview Another Eclipse Platform Tool Java Workbench Help Development Tools JFace (JDT) SWT Team Your Tool Plug-in Workspace Development Debug Environment (PDE) Their Platform Runtime Tool Eclipse Project 200303331 5 [________________________] Slides that provide non-technical background info on Eclipse. 200303331 6 Eclipse Origins ■ Eclipse created by OTI and IBM teams responsible for IDE products – IBM VisualAge/Smalltalk (Smalltalk IDE) – IBM VisualAge/Java (Java IDE) – IBM VisualAge/Micro Edition (Java IDE) ■ Initially staffed with 40 full-time developers ■ Geographically dispersed development teams – OTI Ottawa, OTI Minneapolis, OTI Zurich, IBM Toronto, OTI Raleigh, IBM RTP, IBM St. -
Herramientas Open Source Para La Construcción De Portales
Herramientas Open Source para la construcción de portales Nombre del Estudiante Esteve Almirall - ETIS Nombre del Consultor Dr. Jordi Delgado Fecha de Entrega 3-I-2005 INDICE RESUMEN ................................................................................. 5 JUSTIFICACIÓN Y OBJETIVOS.................................................. 6 ENFOQUE Y METODOLOGIA.............................................................. 8 PRODUCTOS OBTENIDOS................................................................ 8 BREVE DESCRIPCIÓN DE LOS CAPITULOS.............................................. 8 INTRODUCCIÓN ..................................................................... 10 QUE SE ENDIENTE POR SOFTWARE ABIERTO (OPEN SOURCE)..................... 10 LENGUAJES DE PROGRAMACIÓN PARA PLATAFORMAS .............................. 12 Descripción de lenguajes de programación ............................. 12 Comparativa entre los diferentes Lenguajes............................ 13 ESTUDIO DE PLATAFORMAS LIBRE DISTRIBUCIÓN................ 15 PLATAFORMAS PROVENIENTES DE ENTORNOS DE ELEARNING ..................... 15 PORTALES DE COLABORACIÓN Y PLATAFORMAS GRUPALES ........................ 20 Descripción de plataformas grupales...................................... 20 Comparativa de las mejores plataformas grupales ................... 22 Vistas de las herramientas grupales más destacadas................ 23 Valoración ......................................................................... 26 SISTEMAS E INFRAESTRUCTURA BASE.................................. -
Websphere Application Server V8.5 Migration Guide
IBM® WebSphere® Front cover WebSphere Application Server V8.5 Migration Guide Review case studies for competitive and version migrations Learn about the migration strategy and planning Become proficient with the Application Migration Toolkit Ersan Arik Sinan Konya Burak Cakil Hatice Meric Kurtcebe Eroglu Ross Pavitt Vasfi Gucer Dave Vines Rispna Jain Hakan Yildirim Levent Kaya Tayfun Yurdagul ibm.com/redbooks International Technical Support Organization WebSphere Application Server V8.5 Migration Guide November 2012 SG24-8048-00 Note: Before using this information and the product it supports, read the information in “Notices” on page xxi. First Edition (November 2012) This edition applies to the following IBM products: IBM WebSphere Application Server V8.5 IBM Rational Application Developer V8.5 IBM WebSphere Application Server Migration Toolkit V3.5 IBM DB2 Express Edition V10.1. © Copyright International Business Machines Corporation 2012. All rights reserved. Note to U.S. Government Users Restricted Rights -- Use, duplication or disclosure restricted by GSA ADP Schedule Contract with IBM Corp. Contents Figures . xi Tables . xvii Examples . xix Notices . xxi Trademarks . xxii Preface . xxiii The team who wrote this book . xxiii Now you can become a published author, too! . xxvi Comments welcome. xxvi Stay connected to IBM Redbooks . xxvi Part 1. WebSphere Application Server V8.5: Concepts and architecture . 1 Chapter 1. Overview of WebSphere Application Server V8.5 . 3 1.1 Overview of WebSphere Application Server . 4 1.2 WebSphere Application Server packaging . 4 1.3 WebSphere Application Server concepts . 6 1.3.1 Servers . 6 1.3.2 Nodes and node groups . 8 1.3.3 Cells, a deployment manager, and node agents.