Continuation-Passing C, Compiling Threads to Events Through Continuations Gabriel Kerneis, Juliusz Chroboczek
Total Page:16
File Type:pdf, Size:1020Kb
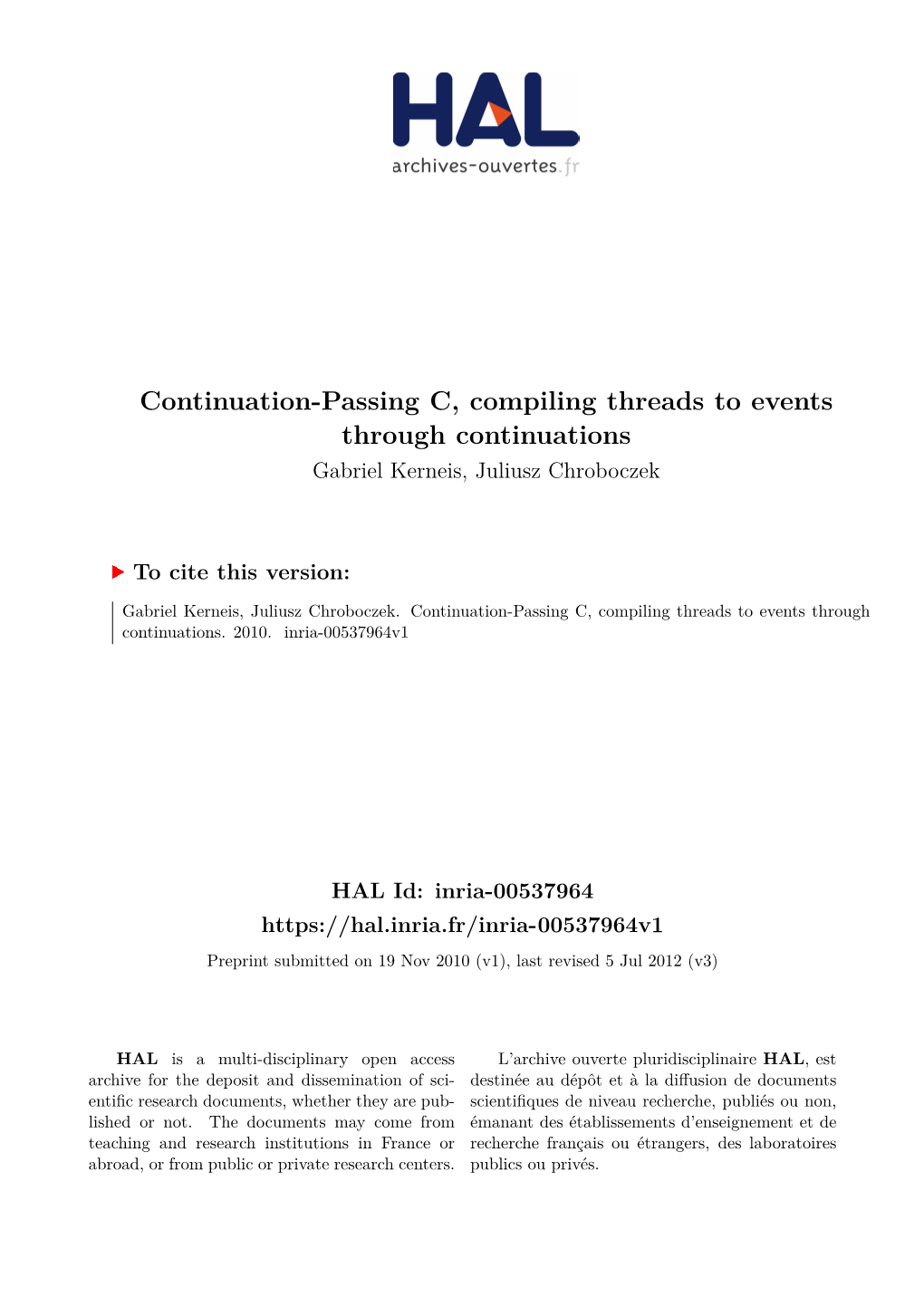
Load more
Recommended publications
-
The Spineless Tagless G-Machine Version
Implementing lazy functional languages on sto ck hardware the Spineless Tagless Gmachine Version Simon L Peyton Jones Department of Computing Science University of Glasgow G QQ simonp jdcsglasgowacuk July Abstract The Spineless Tagless Gmachine is an abstract machine designed to supp ort non strict higherorder functional languages This presentation of the machine falls into three parts Firstly we give a general discussion of the design issues involved in implementing nonstrict functional languages Next we present the STG language an austere but recognisablyfunctional language which as well as a denotational meaning has a welldened operational semantics The STG language is the abstract machine co de for the Spineless Tagless Gmachine Lastly we discuss the mapping of the STG language onto sto ck hardware The success of an abstract machine mo del dep ends largely on how ecient this mapping can b e made though this topic is often relegated to a short section Instead we give a detailed discussion of the design issues and the choices we have made Our principal target is the C language treating the C compiler as a p ortable assembler This pap er is to app ear in the Journal of Functional Programming Changes in Version large new section on comparing the STG machine with other designs Section proling material Section index Changes in Version prop er statement of the initial state of the machine Sec tion reformulation of CAF up dates Section new format for state transition rules separating the guards which govern the applicability of -
Hardware Synthesis from a Recursive Functional Language
Hardware Synthesis from a Recursive Functional Language Kuangya Zhai Richard Townsend Lianne Lairmore Martha A. Kim Stephen A. Edwards Columbia University, Department of Computer Science Technical Report CUCS-007-15 April 1, 2015 ABSTRACT to SystemVerilog. We use a single functional intermedi- Abstraction in hardware description languages stalled at the ate representation based on the Glasgow Haskell Compiler's register-transfer level decades ago, yet few alternatives have Core [23], allowing us to use Haskell and GHC as a source had much success, in part because they provide only mod- language. Reynolds [24] pioneered the methodology of trans- est gains in expressivity. We propose to make a much larger forming programs into successively restricted subsets, which jump: a compiler that synthesizes hardware from behav- inspired our compiler and many others [2, 15, 23]. ioral functional specifications. Our compiler translates gen- Figure 1 depicts our compiler's framework as a series of eral Haskell programs into a restricted intermediate repre- transformations yielding a hardware description. One pass sentation before applying a series of semantics-preserving eliminates polymorphism by making specialized copies of transformations, concluding with a simple syntax-directed types and functions. To implement recursion, our compiler translation to SystemVerilog. Here, we present the overall gives groups of recursive functions a stack and converts them framework for this compiler, focusing on the IRs involved into tail form. These functions are then scheduled over time and our method for translating general recursive functions by rewriting them to operate on infinite streams that model into equivalent hardware. We conclude with experimental discrete clock cycles. -
UNIVERSITY of CAMBRIDGE Computer Laboratory
UNIVERSITY OF CAMBRIDGE Computer Laboratory Computer Science Tripos Part Ib Compiler Construction http://www.cl.cam.ac.uk/Teaching/1112/CompConstr/ David J Greaves [email protected] 2011–2012 (Lent Term) Notes and slides: thanks due to Prof Alan Mycroft and his predecessors. Summary The first part of this course covers the design of the various parts of a fairly basic compiler for a pretty minimal language. The second part of the course considers various language features and concepts common to many programming languages, together with an outline of the kind of run-time data structures and operations they require. The course is intended to study compilation of a range of languages and accordingly syntax for example constructs will be taken from various languages (with the intention that the particular choice of syntax is reasonably clear). The target language of a compiler is generally machine code for some processor; this course will only use instructions common (modulo spelling) to x86, ARM and MIPS—with MIPS being favoured because of its use in the Part Ib course “Computer Design”. In terms of programming languages in which parts of compilers themselves are to be written, the preference varies between pseudo-code (as per the ‘Data Structures and Algorithms’ course) and language features (essentially) common to C/C++/Java/ML. The following books contain material relevant to the course. Compilers—Principles, Techniques, and Tools A.V.Aho, R.Sethi and J.D.Ullman Addison-Wesley (1986) Ellis Horwood (1982) Compiler Design in Java/C/ML (3 -
The Λ Abroad a Functional Approach to Software Components
The ¸ Abroad A Functional Approach To Software Components Een functionele benadering van software componenten (met een samenvatting in het Nederlands) Proefschrift ter verkrijging van de graad van doctor aan de Universiteit Utrecht op gezag van de Rector Magni¯cus, Prof. dr W.H. Gispen, ingevolge het besluit van het College voor Promoties in het openbaar te verdedigen op dinsdag 4 november 2003 des middags te 12.45 uur door Daniel Johannes Pieter Leijen geboren op 7 Juli 1973, te Alkmaar promotor: Prof. dr S.D. Swierstra, Universiteit Utrecht. co-promotor: dr H.J.M. Meijer, Microsoft Research. The work in this thesis has been carried out under the auspices of the research school IPA (Institute for Programming research and Algorithmics), and has been ¯nanced by Ordina. Printed by Febodruk 2003. Cover illustration shows the heavily cratered surface of the planet Mercury, photographed by the mariner 10. ISBN 90-9017528-8 Contents Dankwoord ix 1 Overview 1 2 H/Direct: a binary language interface for Haskell 5 2.1 Introduction ................................ 5 2.2 Background ................................ 6 2.2.1 Using the host or foreign language ............... 7 2.2.2 Using an IDL ........................... 8 2.2.3 Overview ............................. 9 2.3 The Foreign Function Interface ..................... 12 2.3.1 Foreign static import and export ................ 12 2.3.2 Variations on the theme ..................... 13 2.3.3 Stable pointers and foreign objects ............... 14 2.3.4 Dynamic import ......................... 15 2.3.5 Dynamic export ......................... 15 2.3.6 Implementing dynamic export .................. 18 2.3.7 Related work ........................... 19 iv Contents 2.4 Translating IDL to Haskell ...................... -
Œuf: Minimizing the Coq Extraction TCB
Œuf: Minimizing the Coq Extraction TCB Eric Mullen Stuart Pernsteiner James R. Wilcox University of Washington, USA University of Washington, USA University of Washington, USA USA USA USA Zachary Tatlock Dan Grossman University of Washington, USA University of Washington, USA USA USA Abstract ACM Reference Format: Verifying systems by implementing them in the program- Eric Mullen, Stuart Pernsteiner, James R. Wilcox, Zachary Tatlock, ming language of a proof assistant (e.g., Gallina for Coq) lets and Dan Grossman. 2018. Œuf: Minimizing the Coq Extraction TCB. In Proceedings of 7th ACM SIGPLAN International Conference on us directly leverage the full power of the proof assistant for Certified Programs and Proofs (CPP’18). ACM, New York, NY, USA, verifying the system. But, to execute such an implementation 14 pages. https://doi.org/10.1145/3167089 requires extraction, a large complicated process that is in the trusted computing base (TCB). This paper presents Œuf, a verified compiler from a subset 1 Introduction of Gallina to assembly. Œuf’s correctness theorem ensures that compilation preserves the semantics of the source Gal- In the past decade, a wide range of verified systems have lina program. We describe how Œuf’s specification can be been implemented in Gallina, Coq’s functional programming used as a foreign function interface to reason about the inter- language [8, 19, 20, 25, 26, 44, 45]. This approach eases veri- action between compiled Gallina programs and surrounding fication because Coq provides extensive built-in support for shim code. Additionally, Œuf maintains a small TCB for its reasoning about Gallina programs. Unfortunately, building front-end by reflecting Gallina programs to Œuf source and a system directly in Gallina typically incurs a substantial automatically ensuring equivalence using computational de- trusted computing base (TCB) to actually extract, compile, notation. -
Algol and Haskell
Spring 2014 Introduction to Haskell Shachar Itzhaky & Mooly Sagiv (original slides by Kathleen Fisher & John Mitchell) Part I: Lambda Calculus Computation Models • Turing Machines • Wang Machines • Counter Programs • Lambda Calculus Untyped Lambda Calculus Chapter 5 Benjamin Pierce Types and Programming Languages Basics • Repetitive expressions can be compactly represented using functional abstraction • Example: – (5 * 4* 3 * 2 * 1) + (7 * 6 * 5 * 4 * 3 * 2 *1) = – factorial(5) + factorial(7) – factorial(n) = if n = 0 then 1 else n * factorial(n-1) – factorial= n. if n = 0 then 0 else n factorial(n-1) Untyped Lambda Calculus t ::= terms x variable x. t abstraction t t application Terms can be represented as abstract syntax trees Syntactic Conventions • Applications associates to left e1 e2 e3 (e1 e2) e3 • The body of abstraction extends as far as possible • x. y. x y x x. (y. (x y) x) Free vs. Bound Variables • An occurrence of x is free in a term t if it is not in the body on an abstraction x. t – otherwise it is bound – x is a binder • Examples – z. x. y. x (y z) – (x. x) x • Terms w/o free variables are combinators – Identify function: id = x. x Operational Semantics ( x. t12) t2 [x ↦t2] t12 (-reduction) FV: t P(Var) is the set free variables of t FV(x) = {x} FV( x. t) = FV(t) – {x} FV (t1 t2) = FV(t1) FV(t2) [x↦s] x = s [x↦s] y = y if y x [x↦s] (y. t1) = y. [x ↦s] t1 if y x and yFV(s) [x↦s] (t1 t2) = ([x↦s] t1) ([x↦s] t2) Operational Semantics ( x. -
A Located Lambda Calculus
A located lambda calculus Ezra elias kilty Cooper Philip Wadler University of Edinburgh Abstract how to perform a splitting transformation to produce code for each Several recent language designs have offered a unified language for location. However, each of these works relied on concurrently- programming a distributed system; we call these “location-aware” running servers. Ours is the first work we’re aware of that shows languages. These languages provide constructs that allow the pro- how to implement a located language on top of a stateless server grammer to control the location (the choice of host, for example) model. where a piece of code should run, which can be useful for secu- Our technique involves three essential transformations: defunc- rity or performance reasons. On the other hand, a central mantra tionalization a` la Reynolds, CPS transformation, and a “trampo- of web engineering insists that web servers should be “stateless”: line” that allows tunnelling server-to-client requests within server that no “session state” should be maintained on behalf of individ- responses. ual clients—that is, no state that pertains to the particular point of We have implemented a version of this feature in the Links lan- the interaction at which a client program resides. Thus far, most guage (Cooper et al. 2006). In the current version, only calls to top- implementations of unified location-aware languages have ignored level functions can pass control between client and server. Here this precept, usually keeping a process for each client running on we provide a formalization and show how to relax the top level- the server, or otherwise storing state information in memory. -
Defining C Preprocessor Macro Libraries with Functional Programs
Computing and Informatics, Vol. 35, 2016, 819{851 DEFINING C PREPROCESSOR MACRO LIBRARIES WITH FUNCTIONAL PROGRAMS Boldizs´ar Nemeth´ , M´at´e Karacsony´ Zolt´an Kelemen, M´at´e Tejfel E¨otv¨osLor´andUniversity Department of Programming Languages and Compilers H-1117 P´azm´anyP´eters´et´any1/C, Budapest, Hungary e-mail: fnboldi, kmate, kelemzol, [email protected] Abstract. The preprocessor of the C language provides a standard way to generate code at compile time. However, writing and understanding these macros is difficult. Lack of typing, statelessness and uncommon syntax are the main reasons of this difficulty. Haskell is a high-level purely functional language with expressive type system, algebraic data types and many useful language extensions. These suggest that Haskell code can be written and maintained easier than preprocessor macros. Functional languages have certain similarities to macro languages. By using these similarities this paper describes a transformation that translates lambda expres- sions into preprocessor macros. Existing compilers for functional languages gener- ate lambda expressions from the source code as an intermediate representation. As a result it is possible to write Haskell code that will be translated into preprocessor macros that manipulate source code. This may result in faster development and maintenance of complex macro metaprograms. Keywords: C preprocessor, functional programming, Haskell, program transfor- mation, transcompiler Mathematics Subject Classification 2010: 68-N15 1 INTRODUCTION Preprocessor macros are fundamental elements of the C programming language. Besides being useful to define constants and common code snippets, they also allow 820 B. N´emeth,M. Kar´acsony,Z. Kelemen, M. Tejfel developers to extend the capabilities of the language without having to change the compiler itself. -
Promoting Functions to Type Families in Haskell Richard A
Bryn Mawr College Scholarship, Research, and Creative Work at Bryn Mawr College Computer Science Faculty Research and Computer Science Scholarship 2014 Promoting Functions to Type Families in Haskell Richard A. Eisenberg Bryn Mawr College, [email protected] Jan Stolarek Let us know how access to this document benefits ouy . Follow this and additional works at: http://repository.brynmawr.edu/compsci_pubs Part of the Computer Sciences Commons Custom Citation Richard A. Eisenberg "Promoting Functions to Type Families," ACM SIGPLAN Notices - Haskell '14 49.12 (December 2014): 95-106. This paper is posted at Scholarship, Research, and Creative Work at Bryn Mawr College. http://repository.brynmawr.edu/compsci_pubs/1 For more information, please contact [email protected]. Final draft submitted for publication at Haskell Symposium 2014 Promoting Functions to Type Families in Haskell Richard A. Eisenberg Jan Stolarek University of Pennsylvania Politechnika Łodzka´ [email protected] [email protected] Abstract In other words, is type-level programming expressive enough? Haskell, as implemented in the Glasgow Haskell Compiler (GHC), To begin to answer this question, we must define “enough.” In this is enriched with many extensions that support type-level program- paper, we choose to interpret “enough” as meaning that type-level ming, such as promoted datatypes, kind polymorphism, and type programming is at least as expressive as term-level programming. families. Yet, the expressiveness of the type-level language remains We wish to be able to take any pure term-level program and write limited. It is missing many features present at the term level, includ- an equivalent type-level one. -
Lambda-Dropping: Transforming Recursive Equations Into Programs with Block Structure Basic Research in Computer Science
BRICS Basic Research in Computer Science BRICS RS-99-27 Danvy & Schultz: Transforming Recursive Equations into Programs with Block Structure Lambda-Dropping: Transforming Recursive Equations into Programs with Block Structure Olivier Danvy Ulrik P. Schultz BRICS Report Series RS-99-27 ISSN 0909-0878 September 1999 Copyright c 1999, Olivier Danvy & Ulrik P. Schultz. BRICS, Department of Computer Science University of Aarhus. All rights reserved. Reproduction of all or part of this work is permitted for educational or research use on condition that this copyright notice is included in any copy. See back inner page for a list of recent BRICS Report Series publications. Copies may be obtained by contacting: BRICS Department of Computer Science University of Aarhus Ny Munkegade, building 540 DK–8000 Aarhus C Denmark Telephone: +45 8942 3360 Telefax: +45 8942 3255 Internet: [email protected] BRICS publications are in general accessible through the World Wide Web and anonymous FTP through these URLs: http://www.brics.dk ftp://ftp.brics.dk This document in subdirectory RS/99/27/ Lambda-Dropping: Transforming Recursive Equations into Programs with Block Structure ∗ Olivier Danvy Ulrik P. Schultz BRICS † Compose Project Department of Computer Science IRISA/INRIA University of Aarhus ‡ University of Rennes § July 30, 1998 Abstract Lambda-lifting a block-structured program transforms it into a set of recursive equations. We present the symmetric transformation: lambda- dropping. Lambda-dropping a set of recursive equations restores block structure and lexical scope. For lack of block structure and lexical scope, recursive equations must carry around all the parameters that any of their callees might possibly need. -
An Extensional Characterization of Lambda-Lifting and Lambda-Dropping Basic Research in Computer Science
BRICS Basic Research in Computer Science BRICS RS-99-21 O. Danvy: An Extensional Characterization of Lambda-Lifting and Lambda-Dropping An Extensional Characterization of Lambda-Lifting and Lambda-Dropping Olivier Danvy BRICS Report Series RS-99-21 ISSN 0909-0878 August 1999 Copyright c 1999, Olivier Danvy. BRICS, Department of Computer Science University of Aarhus. All rights reserved. Reproduction of all or part of this work is permitted for educational or research use on condition that this copyright notice is included in any copy. See back inner page for a list of recent BRICS Report Series publications. Copies may be obtained by contacting: BRICS Department of Computer Science University of Aarhus Ny Munkegade, building 540 DK–8000 Aarhus C Denmark Telephone: +45 8942 3360 Telefax: +45 8942 3255 Internet: [email protected] BRICS publications are in general accessible through the World Wide Web and anonymous FTP through these URLs: http://www.brics.dk ftp://ftp.brics.dk This document in subdirectory RS/99/21/ An Extensional Characterization of Lambda-Lifting and Lambda-Dropping ∗ Olivier Danvy BRICS † Department of Computer Science University of Aarhus ‡ August 25, 1999 Abstract Lambda-lifting and lambda-dropping respectively transform a block- structured functional program into recursive equations and vice versa. Lambda-lifting was developed in the early 80’s, whereas lambda-dropping is more recent. Both are split into an analysis and a transformation. Published work, however, has only concentrated on the analysis parts. We focus here on the transformation parts and more precisely on their correctness, which appears never to have been proven. -
First-Class Nonstandard Interpretations by Opening Closures
First-Class Nonstandard Interpretations by Opening Closures Jeffrey Mark Siskind Barak A. Pearlmutter School of Electrical and Computer Engineering Hamilton Institute Purdue University, USA NUI Maynooth, Ireland [email protected] [email protected] Abstract resent the complex number a + biasanArgandpaira, b.Ex- We motivate and discuss a novel functional programming construct tending the programming language to support complex arithmetic that allows convenient modular run-time nonstandard interpretation can be viewed as a nonstandard interpretation where real num- bers r are lifted to complex numbers r, 0, and operations such via reflection on closure environments. This map-closure con- +:R × R → R +:C × C → C struct encompasses both the ability to examine the contents of a as are lifted to . closure environment and to construct a new closure with a modi- a1,b1 + a2,b2 = a1 + a2,b1 + b2 fied environment. From the user’s perspective, map-closure is a powerful and useful construct that supports such tasks as tracing, One can accomplish this in SCHEME by redefining the arith- security logging, sandboxing, error checking, profiling, code in- metic primitives, such as +, to operate on combinations of native strumentation and metering, run-time code patching, and resource SCHEME reals and Argand pairs a, b represented as SCHEME monitoring. From the implementor’s perspective, map-closure pairs (a . b). For expository simplicity, we ignore the fact that is analogous to call/cc.Justascall/cc is a non-referentially- many of SCHEME’s numeric primitives can accept a variable num- transparent mechanism that reifies the continuations that are only ber of arguments.